@ -0,0 +1,16 @@ |
|||
version: 2 |
|||
updates: |
|||
# GitHub Actions |
|||
- package-ecosystem: "github-actions" |
|||
directory: "/" |
|||
schedule: |
|||
interval: "daily" |
|||
commit-message: |
|||
prefix: ⬆ |
|||
# Python |
|||
- package-ecosystem: "pip" |
|||
directory: "/" |
|||
schedule: |
|||
interval: "daily" |
|||
commit-message: |
|||
prefix: ⬆ |
@ -0,0 +1,51 @@ |
|||
# See https://pre-commit.com for more information |
|||
# See https://pre-commit.com/hooks.html for more hooks |
|||
repos: |
|||
- repo: https://github.com/pre-commit/pre-commit-hooks |
|||
rev: v4.3.0 |
|||
hooks: |
|||
- id: check-added-large-files |
|||
- id: check-toml |
|||
- id: check-yaml |
|||
args: |
|||
- --unsafe |
|||
- id: end-of-file-fixer |
|||
- id: trailing-whitespace |
|||
- repo: https://github.com/asottile/pyupgrade |
|||
rev: v2.37.1 |
|||
hooks: |
|||
- id: pyupgrade |
|||
args: |
|||
- --py3-plus |
|||
- --keep-runtime-typing |
|||
- repo: https://github.com/myint/autoflake |
|||
rev: v1.4 |
|||
hooks: |
|||
- id: autoflake |
|||
args: |
|||
- --recursive |
|||
- --in-place |
|||
- --remove-all-unused-imports |
|||
- --remove-unused-variables |
|||
- --expand-star-imports |
|||
- --exclude |
|||
- __init__.py |
|||
- --remove-duplicate-keys |
|||
- repo: https://github.com/pycqa/isort |
|||
rev: 5.10.1 |
|||
hooks: |
|||
- id: isort |
|||
name: isort (python) |
|||
- id: isort |
|||
name: isort (cython) |
|||
types: [cython] |
|||
- id: isort |
|||
name: isort (pyi) |
|||
types: [pyi] |
|||
- repo: https://github.com/psf/black |
|||
rev: 22.6.0 |
|||
hooks: |
|||
- id: black |
|||
ci: |
|||
autofix_commit_msg: 🎨 [pre-commit.ci] Auto format from pre-commit.com hooks |
|||
autoupdate_commit_msg: ⬆ [pre-commit.ci] pre-commit autoupdate |
@ -0,0 +1,267 @@ |
|||
# Generate Clients |
|||
|
|||
As **FastAPI** is based on the OpenAPI specification, you get automatic compatibility with many tools, including the automatic API docs (provided by Swagger UI). |
|||
|
|||
One particular advantage that is not necessarily obvious is that you can **generate clients** (sometimes called <abbr title="Software Development Kits">**SDKs**</abbr> ) for your API, for many different **programming languages**. |
|||
|
|||
## OpenAPI Client Generators |
|||
|
|||
There are many tools to generate clients from **OpenAPI**. |
|||
|
|||
A common tool is <a href="https://openapi-generator.tech/" class="external-link" target="_blank">OpenAPI Generator</a>. |
|||
|
|||
If you are building a **frontend**, a very interesting alternative is <a href="https://github.com/ferdikoomen/openapi-typescript-codegen" class="external-link" target="_blank">openapi-typescript-codegen</a>. |
|||
|
|||
## Generate a TypeScript Frontend Client |
|||
|
|||
Let's start with a simple FastAPI application: |
|||
|
|||
=== "Python 3.6 and above" |
|||
|
|||
```Python hl_lines="9-11 14-15 18 19 23" |
|||
{!> ../../../docs_src/generate_clients/tutorial001.py!} |
|||
``` |
|||
|
|||
=== "Python 3.9 and above" |
|||
|
|||
```Python hl_lines="7-9 12-13 16-17 21" |
|||
{!> ../../../docs_src/generate_clients/tutorial001_py39.py!} |
|||
``` |
|||
|
|||
Notice that the *path operations* define the models they use for request payload and response payload, using the models `Item` and `ResponseMessage`. |
|||
|
|||
### API Docs |
|||
|
|||
If you go to the API docs, you will see that it has the **schemas** for the data to be sent in requests and received in responses: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image01.png"> |
|||
|
|||
You can see those schemas because they were declared with the models in the app. |
|||
|
|||
That information is available in the app's **OpenAPI schema**, and then shown in the API docs (by Swagger UI). |
|||
|
|||
And that same information from the models that is included in OpenAPI is what can be used to **generate the client code**. |
|||
|
|||
### Generate a TypeScript Client |
|||
|
|||
Now that we have the app with the models, we can generate the client code for the frontend. |
|||
|
|||
#### Install `openapi-typescript-codegen` |
|||
|
|||
You can install `openapi-typescript-codegen` in your frontend code with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ npm install openapi-typescript-codegen --save-dev |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
#### Generate Client Code |
|||
|
|||
To generate the client code you can use the command line application `openapi` that would now be installed. |
|||
|
|||
Because it is installed in the local project, you probably wouldn't be able to call that command directly, but you would put it on your `package.json` file. |
|||
|
|||
It could look like this: |
|||
|
|||
```JSON hl_lines="7" |
|||
{ |
|||
"name": "frontend-app", |
|||
"version": "1.0.0", |
|||
"description": "", |
|||
"main": "index.js", |
|||
"scripts": { |
|||
"generate-client": "openapi --input http://localhost:8000/openapi.json --output ./src/client --client axios" |
|||
}, |
|||
"author": "", |
|||
"license": "", |
|||
"devDependencies": { |
|||
"openapi-typescript-codegen": "^0.20.1", |
|||
"typescript": "^4.6.2" |
|||
} |
|||
} |
|||
``` |
|||
|
|||
After having that NPM `generate-client` script there, you can run it with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ npm run generate-client |
|||
|
|||
[email protected] generate-client /home/user/code/frontend-app |
|||
> openapi --input http://localhost:8000/openapi.json --output ./src/client --client axios |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
That command will generate code in `./src/client` and will use `axios` (the frontend HTTP library) internally. |
|||
|
|||
### Try Out the Client Code |
|||
|
|||
Now you can import and use the client code, it could look like this, notice that you get autocompletion for the methods: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image02.png"> |
|||
|
|||
You will also get autocompletion for the payload to send: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image03.png"> |
|||
|
|||
!!! tip |
|||
Notice the autocompletion for `name` and `price`, that was defined in the FastAPI application, in the `Item` model. |
|||
|
|||
You will have inline errors for the data that you send: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image04.png"> |
|||
|
|||
The response object will also have autocompletion: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image05.png"> |
|||
|
|||
## FastAPI App with Tags |
|||
|
|||
In many cases your FastAPI app will be bigger, and you will probably use tags to separate different groups of *path operations*. |
|||
|
|||
For example, you could have a section for **items** and another section for **users**, and they could be separated by tags: |
|||
|
|||
|
|||
=== "Python 3.6 and above" |
|||
|
|||
```Python hl_lines="23 28 36" |
|||
{!> ../../../docs_src/generate_clients/tutorial002.py!} |
|||
``` |
|||
|
|||
=== "Python 3.9 and above" |
|||
|
|||
```Python hl_lines="21 26 34" |
|||
{!> ../../../docs_src/generate_clients/tutorial002_py39.py!} |
|||
``` |
|||
|
|||
### Generate a TypeScript Client with Tags |
|||
|
|||
If you generate a client for a FastAPI app using tags, it will normally also separate the client code based on the tags. |
|||
|
|||
This way you will be able to have things ordered and grouped correctly for the client code: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image06.png"> |
|||
|
|||
In this case you have: |
|||
|
|||
* `ItemsService` |
|||
* `UsersService` |
|||
|
|||
### Client Method Names |
|||
|
|||
Right now the generated method names like `createItemItemsPost` don't look very clean: |
|||
|
|||
```TypeScript |
|||
ItemsService.createItemItemsPost({name: "Plumbus", price: 5}) |
|||
``` |
|||
|
|||
...that's because the client generator uses the OpenAPI internal **operation ID** for each *path operation*. |
|||
|
|||
OpenAPI requires that each operation ID is unique across all the *path operations*, so FastAPI uses the **function name**, the **path**, and the **HTTP method/operation** to generate that operation ID, because that way it can make sure that the operation IDs are unique. |
|||
|
|||
But I'll show you how to improve that next. 🤓 |
|||
|
|||
## Custom Operation IDs and Better Method Names |
|||
|
|||
You can **modify** the way these operation IDs are **generated** to make them simpler and have **simpler method names** in the clients. |
|||
|
|||
In this case you will have to ensure that each operation ID is **unique** in some other way. |
|||
|
|||
For example, you could make sure that each *path operation* has a tag, and then generate the operation ID based on the **tag** and the *path operation* **name** (the function name). |
|||
|
|||
### Custom Generate Unique ID Function |
|||
|
|||
FastAPI uses a **unique ID** for each *path operation*, it is used for the **operation ID** and also for the names of any needed custom models, for requests or responses. |
|||
|
|||
You can customize that function. It takes an `APIRoute` and outputs a string. |
|||
|
|||
For example, here it is using the first tag (you will probably have only one tag) and the *path operation* name (the function name). |
|||
|
|||
You can then pass that custom function to **FastAPI** as the `generate_unique_id_function` parameter: |
|||
|
|||
=== "Python 3.6 and above" |
|||
|
|||
```Python hl_lines="8-9 12" |
|||
{!> ../../../docs_src/generate_clients/tutorial003.py!} |
|||
``` |
|||
|
|||
=== "Python 3.9 and above" |
|||
|
|||
```Python hl_lines="6-7 10" |
|||
{!> ../../../docs_src/generate_clients/tutorial003_py39.py!} |
|||
``` |
|||
|
|||
### Generate a TypeScript Client with Custom Operation IDs |
|||
|
|||
Now if you generate the client again, you will see that it has the improved method names: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image07.png"> |
|||
|
|||
As you see, the method names now have the tag and then the function name, now they don't include information from the URL path and the HTTP operation. |
|||
|
|||
### Preprocess the OpenAPI Specification for the Client Generator |
|||
|
|||
The generated code still has some **duplicated information**. |
|||
|
|||
We already know that this method is related to the **items** because that word is in the `ItemsService` (taken from the tag), but we still have the tag name prefixed in the method name too. 😕 |
|||
|
|||
We will probably still want to keep it for OpenAPI in general, as that will ensure that the operation IDs are **unique**. |
|||
|
|||
But for the generated client we could **modify** the OpenAPI operation IDs right before generating the clients, just to make those method names nicer and **cleaner**. |
|||
|
|||
We could download the OpenAPI JSON to a file `openapi.json` and then we could **remove that prefixed tag** with a script like this: |
|||
|
|||
```Python |
|||
{!../../../docs_src/generate_clients/tutorial004.py!} |
|||
``` |
|||
|
|||
With that, the operation IDs would be renamed from things like `items-get_items` to just `get_items`, that way the client generator can generate simpler method names. |
|||
|
|||
### Generate a TypeScript Client with the Preprocessed OpenAPI |
|||
|
|||
Now as the end result is in a file `openapi.json`, you would modify the `package.json` to use that local file, for example: |
|||
|
|||
```JSON hl_lines="7" |
|||
{ |
|||
"name": "frontend-app", |
|||
"version": "1.0.0", |
|||
"description": "", |
|||
"main": "index.js", |
|||
"scripts": { |
|||
"generate-client": "openapi --input ./openapi.json --output ./src/client --client axios" |
|||
}, |
|||
"author": "", |
|||
"license": "", |
|||
"devDependencies": { |
|||
"openapi-typescript-codegen": "^0.20.1", |
|||
"typescript": "^4.6.2" |
|||
} |
|||
} |
|||
``` |
|||
|
|||
After generating the new client, you would now have **clean method names**, with all the **autocompletion**, **inline errors**, etc: |
|||
|
|||
<img src="/img/tutorial/generate-clients/image08.png"> |
|||
|
|||
## Benefits |
|||
|
|||
When using the automatically generated clients you would **autocompletion** for: |
|||
|
|||
* Methods. |
|||
* Request payloads in the body, query parameters, etc. |
|||
* Response payloads. |
|||
|
|||
You would also have **inline errors** for everything. |
|||
|
|||
And whenever you update the backend code, and **regenerate** the frontend, it would have any new *path operations* available as methods, the old ones removed, and any other change would be reflected on the generated code. 🤓 |
|||
|
|||
This also means that if something changed it will be **reflected** on the client code automatically. And if you **build** the client it will error out if you have any **mismatch** in the data used. |
|||
|
|||
So, you would **detect many errors** very early in the development cycle instead of having to wait for the errors to show up to your final users in production and then trying to debug where the problem is. ✨ |
After Width: | Height: | Size: 36 KiB |
After Width: | Height: | Size: 5.2 KiB |
After Width: | Height: | Size: 6.1 KiB |
After Width: | Height: | Size: 52 KiB |
After Width: | Height: | Size: 91 KiB |
After Width: | Height: | Size: 10 KiB |
After Width: | Height: | Size: 16 KiB |
After Width: | Height: | Size: 105 KiB |
After Width: | Height: | Size: 106 KiB |
After Width: | Height: | Size: 12 KiB |
After Width: | Height: | Size: 14 KiB |
After Width: | Height: | Size: 26 KiB |
After Width: | Height: | Size: 34 KiB |
After Width: | Height: | Size: 76 KiB |
After Width: | Height: | Size: 58 KiB |
After Width: | Height: | Size: 60 KiB |
After Width: | Height: | Size: 53 KiB |
After Width: | Height: | Size: 30 KiB |
After Width: | Height: | Size: 42 KiB |
After Width: | Height: | Size: 49 KiB |
After Width: | Height: | Size: 29 KiB |
@ -22,22 +22,16 @@ |
|||
</div> |
|||
</div> |
|||
<div id="announce-right" style="position: relative;"> |
|||
<!-- <div class="item"> |
|||
<a title="The launchpad for all your (team's) ideas" style="display: block; position: relative;" href="https://www.deta.sh/?ref=fastapi" target="_blank"> |
|||
<span class="sponsor-badge">sponsor</span> |
|||
<img class="sponsor-image" src="/img/sponsors/deta-banner.svg" /> |
|||
</a> |
|||
</div> --> |
|||
<div class="item"> |
|||
<a title="Get three courses at 10% off their current prices! Plus, we'll be donating 10% of all profits from sales of this bundle to the FastAPI team." style="display: block; position: relative;" href="https://testdriven.io/talkpython/" target="_blank"> |
|||
<span class="sponsor-badge">sponsor</span> |
|||
<img class="sponsor-image" src="/img/sponsors/fastapi-course-bundle-banner.svg" /> |
|||
<img class="sponsor-image" src="/img/sponsors/fastapi-course-bundle-banner.png" /> |
|||
</a> |
|||
</div> |
|||
<div class="item"> |
|||
<a title="Jina: build neural search-as-a-service for any kind of data in just minutes." style="display: block; position: relative;" href="https://bit.ly/2QSouzH" target="_blank"> |
|||
<a title="DiscoArt: Create compelling Disco Diffusion artworks in just one line" style="display: block; position: relative;" href="https://bit.ly/3PjOZqc" target="_blank"> |
|||
<span class="sponsor-badge">sponsor</span> |
|||
<img class="sponsor-image" src="/img/sponsors/jina-banner.svg" /> |
|||
<img class="sponsor-image" src="/img/sponsors/jina-ai-banner.png" /> |
|||
</a> |
|||
</div> |
|||
<div class="item"> |
|||
@ -47,17 +41,49 @@ |
|||
</a> |
|||
</div> |
|||
<div class="item"> |
|||
<a title="Dropbase - seamlessly collect, clean, and centralize data." style="display: block; position: relative;" href="https://www.dropbase.io/careers" target="_blank"> |
|||
<a title="The ultimate solution to unlimited and forever cloud storage." style="display: block; position: relative;" href="https://app.imgwhale.xyz/" target="_blank"> |
|||
<span class="sponsor-badge">sponsor</span> |
|||
<img class="sponsor-image" src="/img/sponsors/dropbase-banner.svg" /> |
|||
<img class="sponsor-image" src="/img/sponsors/imgwhale-banner.svg" /> |
|||
</a> |
|||
</div> |
|||
<div class="item"> |
|||
<a title="https://striveworks.us/careers" style="display: block; position: relative;" href="https://striveworks.us/careers?utm_source=fastapi&utm_medium=small_banner&utm_campaign=feb_march#openings" target="_blank"> |
|||
<a title="Help us migrate doist to FastAPI" style="display: block; position: relative;" href="https://doist.com/careers/9B437B1615-wa-senior-backend-engineer-python" target="_blank"> |
|||
<span class="sponsor-badge">sponsor</span> |
|||
<img class="sponsor-image" src="/img/sponsors/striveworks-banner.png" /> |
|||
<img class="sponsor-image" src="/img/sponsors/doist-banner.svg" /> |
|||
</a> |
|||
</div> |
|||
</div> |
|||
</div> |
|||
{% endblock %} |
|||
{%- block scripts %} |
|||
{{ super() }} |
|||
<!-- DocsQA integration start --> |
|||
<script src="https://cdn.jsdelivr.net/npm/[email protected]"></script> |
|||
<script> |
|||
// This prevents the global search from interfering with qa-bot's internal text input. |
|||
document.addEventListener('DOMContentLoaded', () => { |
|||
document.querySelectorAll('qa-bot').forEach((x) => { |
|||
x.addEventListener('keydown', (event) => { |
|||
event.stopPropagation(); |
|||
}); |
|||
}); |
|||
}); |
|||
</script> |
|||
<qa-bot |
|||
server="https://tiangolo-fastapi.docsqa.jina.ai" |
|||
theme="infer" |
|||
title="FastAPI Bot" |
|||
description="FastAPI framework, high performance, easy to learn, fast to code, ready for production" |
|||
style="font-size: 0.8rem" |
|||
> |
|||
<template> |
|||
<dl> |
|||
<dt>You can ask questions about FastAPI. Try:</dt> |
|||
<dd>How do you deploy FastAPI?</dd> |
|||
<dd>What are type hints?</dd> |
|||
<dd>What is OpenAPI?</dd> |
|||
</dl> |
|||
</template> |
|||
</qa-bot> |
|||
<!-- DocsQA integration end --> |
|||
{%- endblock %} |
|||
|
@ -0,0 +1,462 @@ |
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>فریمورک FastAPI، کارایی بالا، یادگیری آسان، کدنویسی سریع، آماده برای استفاده در محیط پروداکشن</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/tiangolo/fastapi/actions?query=workflow%3ATest" target="_blank"> |
|||
<img src="https://github.com/tiangolo/fastapi/workflows/Test/badge.svg" alt="Test"> |
|||
</a> |
|||
<a href="https://codecov.io/gh/tiangolo/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/codecov/c/github/tiangolo/fastapi?color=%2334D058" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/pyversions/fastapi.svg?color=%2334D058" alt="Supported Python versions"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**مستندات**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**کد منبع**: <a href="https://github.com/tiangolo/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
FastAPI یک وب فریمورک مدرن و سریع (با کارایی بالا) برای ایجاد APIهای متنوع (وب، وبسوکت و غبره) با زبان پایتون نسخه +۳.۶ است. این فریمورک با رعایت کامل راهنمای نوع داده (Type Hint) ایجاد شده است. |
|||
|
|||
ویژگیهای کلیدی این فریمورک عبارتند از: |
|||
|
|||
* **<abbr title="Fast">سرعت</abbr>**: کارایی بسیار بالا و قابل مقایسه با **NodeJS** و **Go** (با تشکر از Starlette و Pydantic). [یکی از سریعترین فریمورکهای پایتونی موجود](#performance). |
|||
|
|||
* **<abbr title="Fast to code">کدنویسی سریع</abbr>**: افزایش ۲۰۰ تا ۳۰۰ درصدی سرعت توسعه فابلیتهای جدید. * |
|||
* **<abbr title="Fewer bugs">باگ کمتر</abbr>**: کاهش ۴۰ درصدی خطاهای انسانی (برنامهنویسی). * |
|||
* **<abbr title="Intuitive">غریزی</abbr>**: پشتیبانی فوقالعاده در محیطهای توسعه یکپارچه (IDE). <abbr title="یا اتوکامپلیت، اتوکامپلشن، اینتلیسنس">تکمیل</abbr> در همه بخشهای کد. کاهش زمان رفع باگ. |
|||
* **<abbr title="Easy">آسان</abbr>**: طراحی شده برای یادگیری و استفاده آسان. کاهش زمان مورد نیاز برای مراجعه به مستندات. |
|||
* **<abbr title="Short">کوچک</abbr>**: کاهش تکرار در کد. چندین قابلیت برای هر پارامتر (منظور پارامترهای ورودی تابع هندلر میباشد، به بخش <a href="https://fastapi.tiangolo.com/#recap">خلاصه</a> در همین صفحه مراجعه شود). باگ کمتر. |
|||
* **<abbr title="Robust">استوار</abbr>**: ایجاد کدی آماده برای استفاده در محیط پروداکشن و تولید خودکار <abbr title="Interactive documentation">مستندات تعاملی</abbr> |
|||
* **<abbr title="Standards-based">مبتنی بر استانداردها</abbr>**: مبتنی بر (و منطبق با) استانداردهای متن باز مربوط به API: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (سوگر سابق) و <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>* تخمینها بر اساس تستهای انجام شده در یک تیم توسعه داخلی که مشغول ایجاد برنامههای کاربردی واقعی بودند صورت گرفته است.</small> |
|||
|
|||
## اسپانسرهای طلایی |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">دیگر اسپانسرها</a> |
|||
|
|||
## نظر دیگران در مورد FastAPI |
|||
|
|||
<div style="text-align: left; direction: ltr;"><em> [...] I'm using <strong>FastAPI</strong> a ton these days. [...] I'm actually planning to use it for all of my team's <strong>ML services at Microsoft</strong>. Some of them are getting integrated into the core <strong>Windows</strong> product and some <strong>Office</strong> products."</em></div> |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/tiangolo/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
<div style="text-align: left; direction: ltr;"><em>"We adopted the <strong>FastAPI</strong> library to spawn a <strong>REST</strong>server that can be queried to obtain <strong>predictions</strong>. [for Ludwig]"</em></div> |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
<div style="text-align: left; direction: ltr;">"<strong>Netflix</strong> is pleased to announce the open-source release of our <strong>crisis management</strong> orchestration framework: <strong>Dispatch</strong>! [built with <strong>FastAPI</strong>]"</div> |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
<div style="text-align: left; direction: ltr;">"<em>I’m over the moon excited about <strong>FastAPI</strong>. It’s so fun!"</em></div> |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast host</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
<div style="text-align: left; direction: ltr;">"<em>Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted <strong>Hug</strong> to be - it's really inspiring to see someone build that."</em></div> |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
<div style="text-align: left; direction: ltr;">"<em>If you're looking to learn one <strong>modern framework</strong> for building REST APIs, check out <strong>FastAPI</strong> [...] It's fast, easy to use and easy to learn [...]"</em></div> |
|||
|
|||
<div style="text-align: left; direction: ltr;">"<em>We've switched over to <strong>FastAPI</strong> for our <strong>APIs</strong> [...] I think you'll like it [...]</em>"</div> |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> founders - <a href="https://spacy.io" target="_blank">spaCy</a> creators</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, فریمورکی معادل FastAPI برای کار با <abbr title="CLI (Command Line Interface)">واسط خط فرمان</abbr> |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
اگر در حال ساختن برنامهای برای استفاده در <abbr title="Command Line Interface">CLI</abbr> (به جای استفاده در وب) هستید، میتوانید از <a href="https://typer.tiangolo.com/" class="external-link" target="_blank">**Typer**</a>. استفاده کنید. |
|||
|
|||
**Typer** دوقلوی کوچکتر FastAPI است و قرار است معادلی برای FastAPI در برنامههای CLI باشد.️ 🚀 |
|||
|
|||
## نیازمندیها |
|||
|
|||
پایتون +۳.۶ |
|||
|
|||
FastAPI مبتنی بر ابزارهای قدرتمند زیر است: |
|||
|
|||
* فریمورک <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> برای بخش وب. |
|||
* کتابخانه <a href="https://pydantic-docs.helpmanual.io/" class="external-link" target="_blank">Pydantic</a> برای بخش داده. |
|||
|
|||
## نصب |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install fastapi |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
نصب یک سرور پروداکشن نظیر <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a> یا <a href="https://gitlab.com/pgjones/hypercorn" class="external-link" target="_blank">Hypercorn</a> نیز جزء نیازمندیهاست. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "uvicorn[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## مثال |
|||
|
|||
### ایجاد کنید |
|||
* فایلی به نام `main.py` با محتوای زیر ایجاد کنید : |
|||
|
|||
```Python |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>همچنین میتوانید از <code>async def</code>... نیز استفاده کنید</summary> |
|||
|
|||
اگر در کدتان از `async` / `await` استفاده میکنید, از `async def` برای تعریف تابع خود استفاده کنید: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Optional[str] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**توجه**: |
|||
|
|||
اگر با `async / await` آشنا نیستید، به بخش _"عجله دارید?"_ در صفحه درباره <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` و `await` در مستندات</a> مراجعه کنید. |
|||
|
|||
|
|||
</details> |
|||
|
|||
### اجرا کنید |
|||
|
|||
با استفاده از دستور زیر سرور را اجرا کنید: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [28720] |
|||
INFO: Started server process [28722] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>درباره دستور <code>uvicorn main:app --reload</code>...</summary> |
|||
|
|||
دستور `uvicorn main:app` شامل موارد زیر است: |
|||
|
|||
* `main`: فایل `main.py` (ماژول پایتون ایجاد شده). |
|||
* `app`: شیء ایجاد شده در فایل `main.py` در خط `app = FastAPI()`. |
|||
* `--reload`: ریستارت کردن سرور با تغییر کد. تنها در هنگام توسعه از این گزینه استفاده شود.. |
|||
|
|||
</details> |
|||
|
|||
### بررسی کنید |
|||
|
|||
آدرس <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a> را در مرورگر خود باز کنید. |
|||
|
|||
پاسخ JSON زیر را مشاهده خواهید کرد: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
تا اینجا شما APIای ساختید که: |
|||
|
|||
* درخواستهای HTTP به _مسیرهای_ `/` و `/items/{item_id}` را دریافت میکند. |
|||
* هردو _مسیر_ <abbr title="operations در OpenAPI">عملیات</abbr> (یا HTTP _متد_) `GET` را پشتیبانی میکنند. |
|||
* _مسیر_ `/items/{item_id}` شامل <abbr title="Path Parameter">_پارامتر مسیر_</abbr> `item_id` از نوع `int` است. |
|||
* _مسیر_ `/items/{item_id}` شامل <abbr title="Query Parameter">_پارامتر پرسمان_</abbr> اختیاری `q` از نوع `str` است. |
|||
|
|||
### مستندات API تعاملی |
|||
|
|||
حال به آدرس <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a> بروید. |
|||
|
|||
مستندات API تعاملی (ایجاد شده به کمک <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>) را مشاهده خواهید کرد: |
|||
|
|||
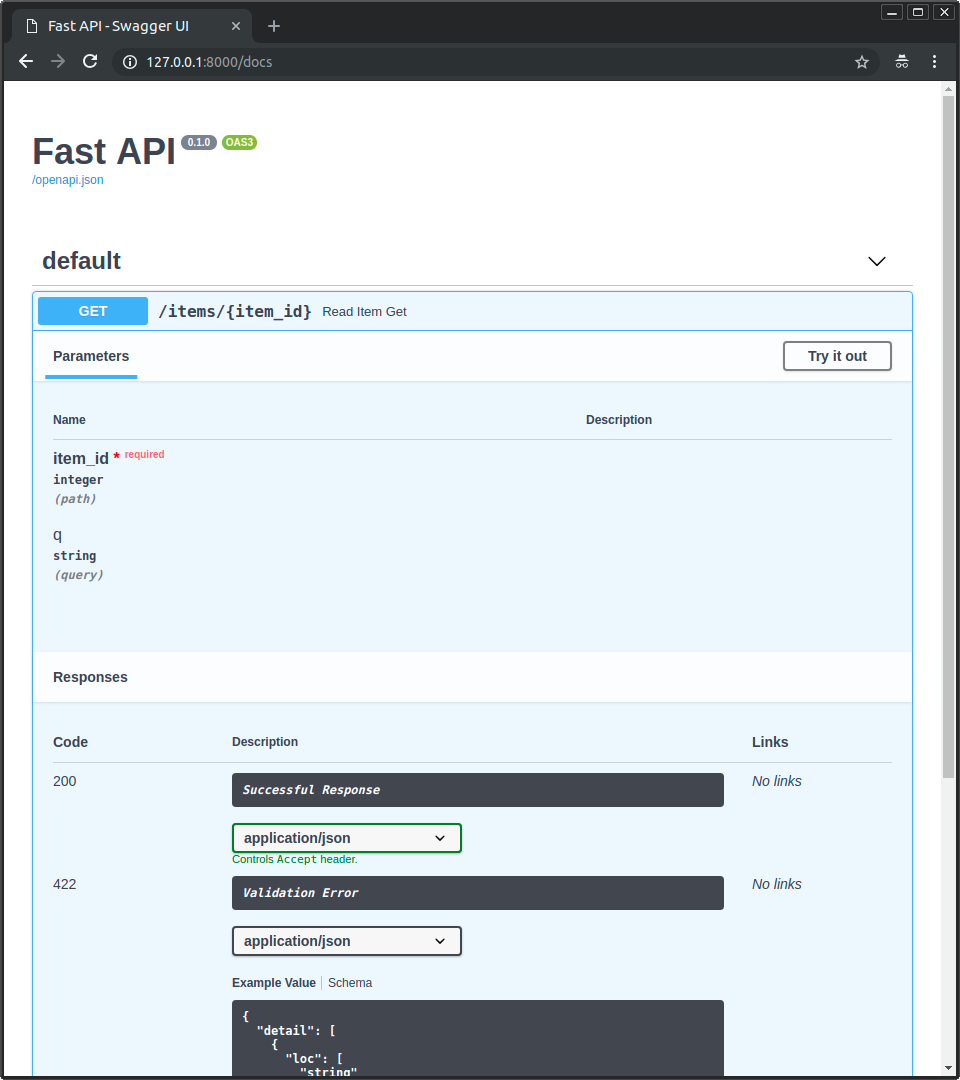 |
|||
|
|||
### مستندات API جایگزین |
|||
|
|||
حال به آدرس <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a> بروید. |
|||
|
|||
مستندات خودکار دیگری را مشاهده خواهید کرد که به کمک <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a> ایجاد میشود: |
|||
|
|||
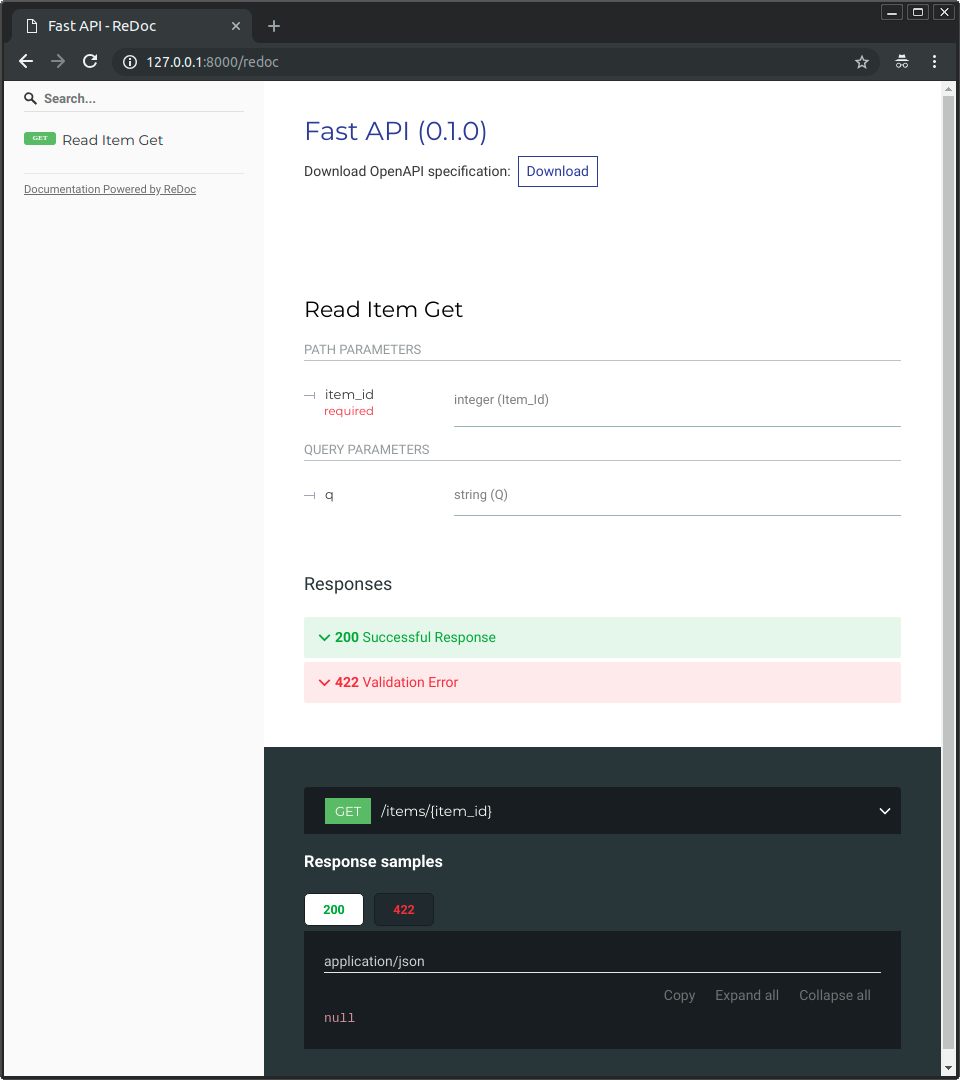 |
|||
|
|||
## تغییر مثال |
|||
|
|||
حال فایل `main.py` را مطابق زیر ویرایش کنید تا بتوانید <abbr title="Body">بدنه</abbr> یک درخواست `PUT` را دریافت کنید. |
|||
|
|||
به کمک Pydantic بدنه درخواست را با <abbr title="Type">انواع</abbr> استاندارد پایتون تعریف کنید. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Union[bool, None] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
سرور به صورت خودکار ریاستارت میشود (زیرا پیشتر از گزینه `--reload` در دستور `uvicorn` استفاده کردیم). |
|||
|
|||
### تغییر مستندات API تعاملی |
|||
|
|||
مجددا به آدرس <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a> بروید. |
|||
|
|||
* مستندات API تعاملی به صورت خودکار بهروز شده است و شامل بدنه تعریف شده در مرحله قبل است: |
|||
|
|||
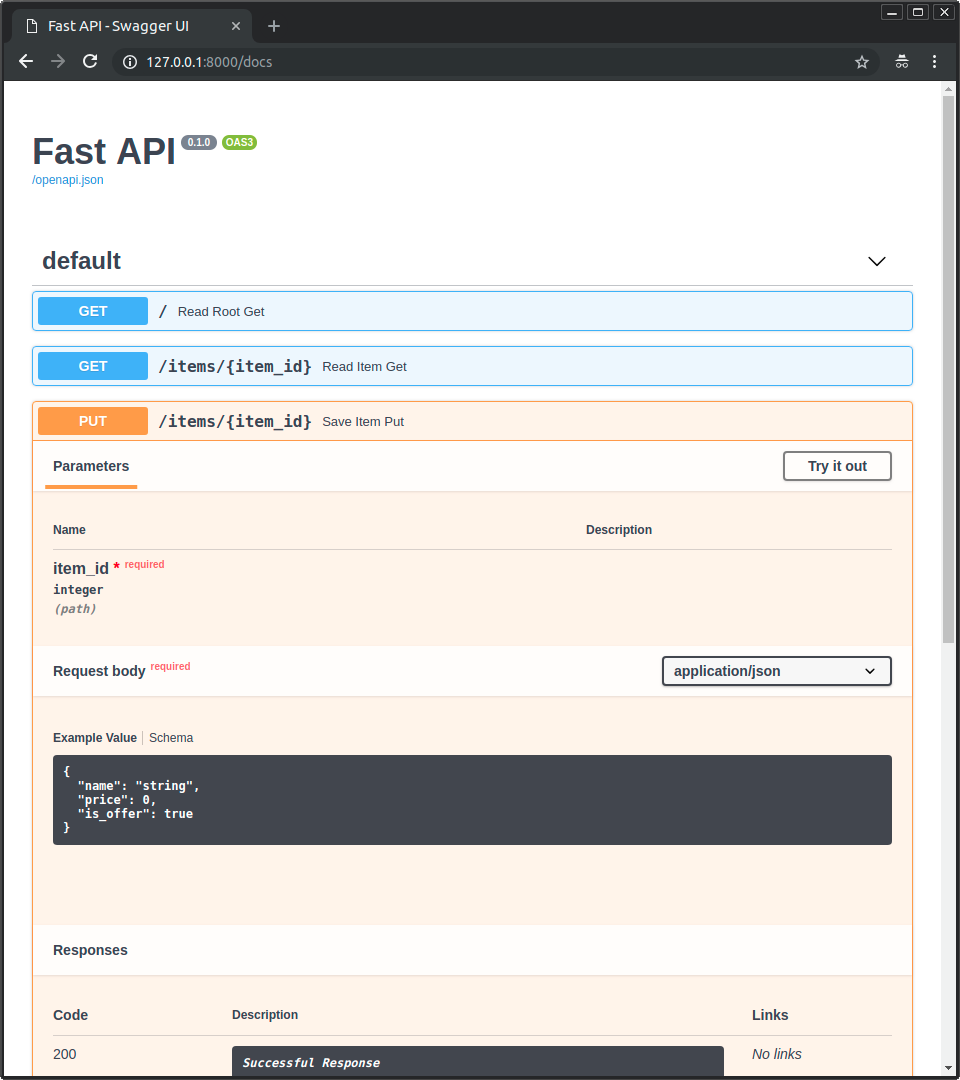 |
|||
|
|||
* روی دکمه "Try it out" کلیک کنید, اکنون میتوانید پارامترهای مورد نیاز هر API را مشخص کرده و به صورت مستقیم با آنها تعامل کنید: |
|||
|
|||
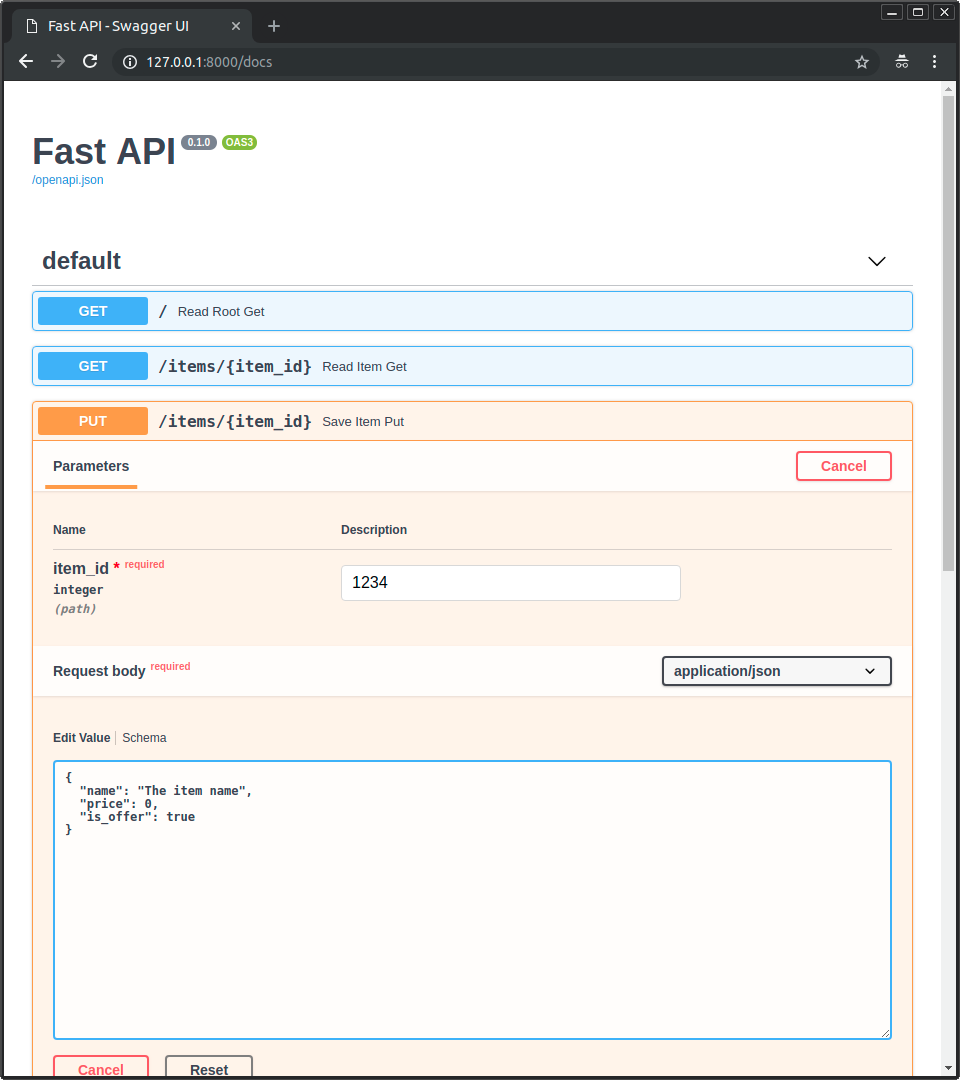 |
|||
|
|||
* سپس روی دکمه "Execute" کلیک کنید, خواهید دید که واسط کاریری با APIهای تعریف شده ارتباط برقرار کرده، پارامترهای مورد نیاز را به آنها ارسال میکند، سپس نتایج را دریافت کرده و در صفحه نشان میدهد: |
|||
|
|||
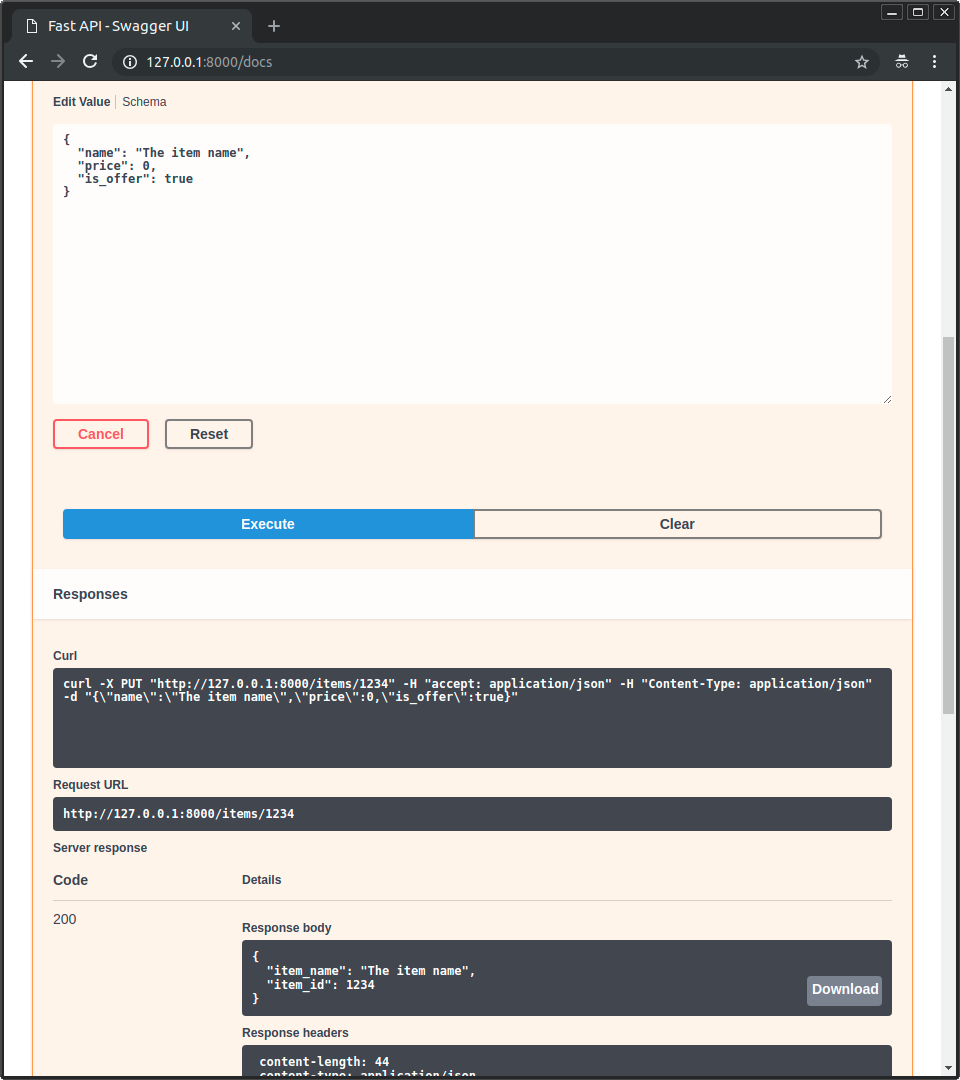 |
|||
|
|||
### تغییر مستندات API جایگزین |
|||
|
|||
حال به آدرس <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a> بروید. |
|||
|
|||
* خواهید دید که مستندات جایگزین نیز بهروزرسانی شده و شامل پارامتر پرسمان و بدنه تعریف شده میباشد: |
|||
|
|||
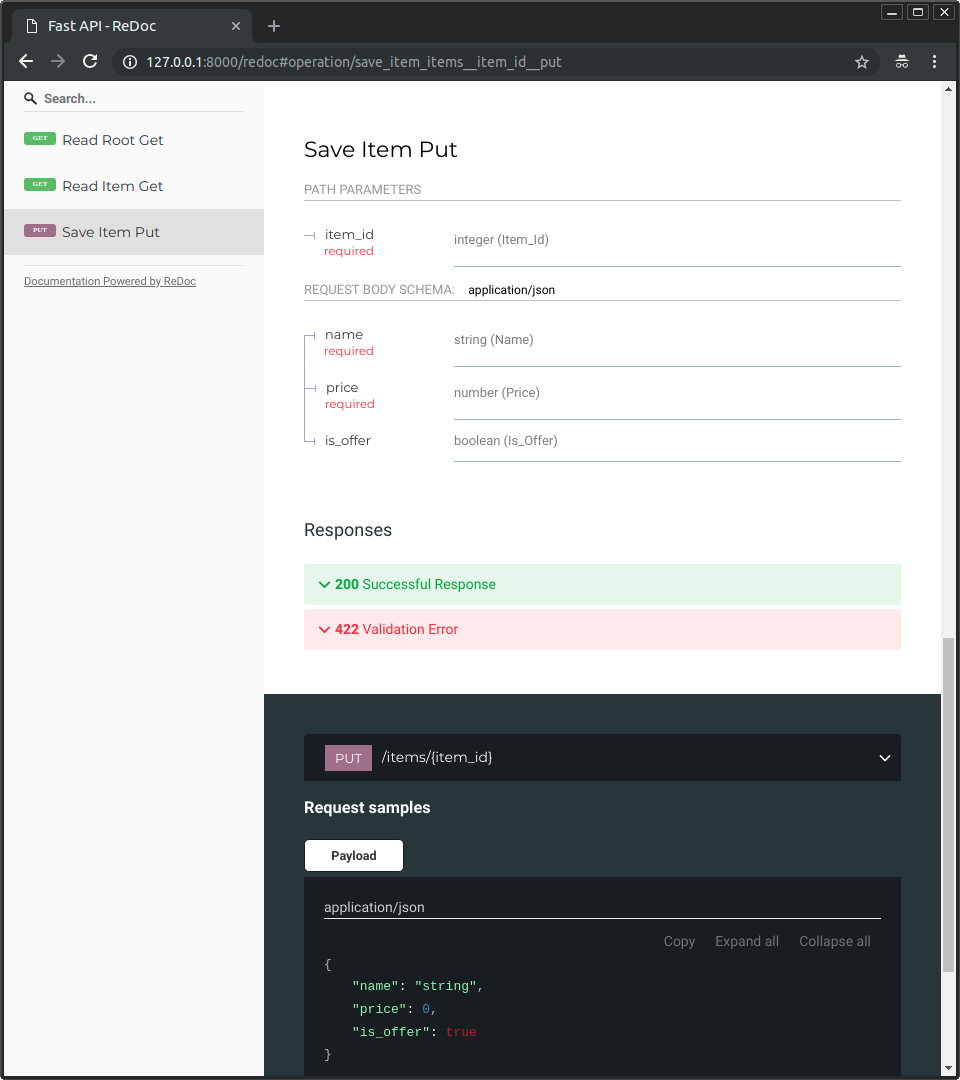 |
|||
|
|||
### خلاصه |
|||
|
|||
به طور خلاصه شما **یک بار** انواع پارامترها، بدنه و غیره را به عنوان پارامترهای ورودی تابع خود تعریف میکنید. |
|||
|
|||
این کار را با استفاده از انواع استاندارد و مدرن موجود در پایتون انجام میدهید. |
|||
|
|||
نیازی به یادگیری <abbr title="Syntax">نحو</abbr> جدید یا متدها و کلاسهای یک کتابخانه بخصوص و غیره نیست. |
|||
|
|||
تنها **پایتون +۳.۶**. |
|||
|
|||
به عنوان مثال برای یک پارامتر از نوع `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
یا برای یک مدل پیچیدهتر مثل `Item`: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...و با همین اعلان تمامی قابلیتهای زیر در دسترس قرار میگیرد: |
|||
|
|||
* پشتیبانی ویرایشگر متنی شامل: |
|||
* تکمیل کد. |
|||
* بررسی انواع داده. |
|||
* اعتبارسنجی داده: |
|||
* خطاهای خودکار و مشخص در هنگام نامعتبر بودن داده |
|||
* اعتبارسنجی، حتی برای اشیاء JSON تو در تو. |
|||
* <abbr title="serialization, parsing, marshalling">تبدیل</abbr> داده ورودی: که از شبکه رسیده به انواع و داده پایتونی. این داده شامل: |
|||
* JSON. |
|||
* <abbr title="Path parameters">پارامترهای مسیر</abbr>. |
|||
* <abbr title="Query parameters">پارامترهای پرسمان</abbr>. |
|||
* <abbr title="Cookies">کوکیها</abbr>. |
|||
* <abbr title="Headers">سرآیندها (هدرها)</abbr>. |
|||
* <abbr title="Forms">فرمها</abbr>. |
|||
* <abbr title="Files">فایلها</abbr>. |
|||
* <abbr title="serialization, parsing, marshalling">تبدیل</abbr> داده خروجی: تبدیل از انواع و داده پایتون به داده شبکه (مانند JSON): |
|||
* تبدیل انواع داده پایتونی (`str`, `int`, `float`, `bool`, `list` و غیره). |
|||
* اشیاء `datetime`. |
|||
* اشیاء `UUID`. |
|||
* qمدلهای پایگاهداده. |
|||
* و موارد بیشمار دیگر. |
|||
* دو مدل مستند API تعاملی خودکار : |
|||
* Swagger UI. |
|||
* ReDoc. |
|||
|
|||
--- |
|||
|
|||
به مثال قبلی باز میگردیم، در این مثال **FastAPI** موارد زیر را انجام میدهد: |
|||
|
|||
* اعتبارسنجی اینکه پارامتر `item_id` در مسیر درخواستهای `GET` و `PUT` موجود است . |
|||
* اعتبارسنجی اینکه پارامتر `item_id` در درخواستهای `GET` و `PUT` از نوع `int` است. |
|||
* اگر غیر از این موارد باشد، سرویسگیرنده خطای مفید و مشخصی دریافت خواهد کرد. |
|||
* بررسی وجود پارامتر پرسمان اختیاری `q` (مانند `http://127.0.0.1:8000/items/foo?q=somequery`) در درخواستهای `GET`. |
|||
* از آنجا که پارامتر `q` با `= None` مقداردهی شده است, این پارامتر اختیاری است. |
|||
* اگر از مقدار اولیه `None` استفاده نکنیم، این پارامتر الزامی خواهد بود (همانند بدنه درخواست در درخواست `PUT`). |
|||
* برای درخواستهای `PUT` به آدرس `/items/{item_id}`, بدنه درخواست باید از نوع JSON تعریف شده باشد: |
|||
* بررسی اینکه بدنه شامل فیلدی با نام `name` و از نوع `str` است. |
|||
* بررسی اینکه بدنه شامل فیلدی با نام `price` و از نوع `float` است. |
|||
* بررسی اینکه بدنه شامل فیلدی اختیاری با نام `is_offer` است, که در صورت وجود باید از نوع `bool` باشد. |
|||
* تمامی این موارد برای اشیاء JSON در هر عمقی قابل بررسی میباشد. |
|||
* تبدیل از/به JSON به صورت خودکار. |
|||
* مستندسازی همه چیز با استفاده از OpenAPI, که میتوان از آن برای موارد زیر استفاده کرد: |
|||
* سیستم مستندات تعاملی. |
|||
* تولید خودکار کد سرویسگیرنده در زبانهای برنامهنویسی بیشمار. |
|||
* فراهم سازی ۲ مستند تعاملی مبتنی بر وب به صورت پیشفرض . |
|||
|
|||
--- |
|||
|
|||
موارد ذکر شده تنها پارهای از ویژگیهای بیشمار FastAPI است اما ایدهای کلی از طرز کار آن در اختیار قرار میدهد. |
|||
|
|||
خط زیر را به این صورت تغییر دهید: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
از: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
به: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
در حین تایپ کردن توجه کنید که چگونه ویرایشگر، ویژگیهای کلاس `Item` را تشخیص داده و به تکمیل خودکار آنها کمک میکند: |
|||
|
|||
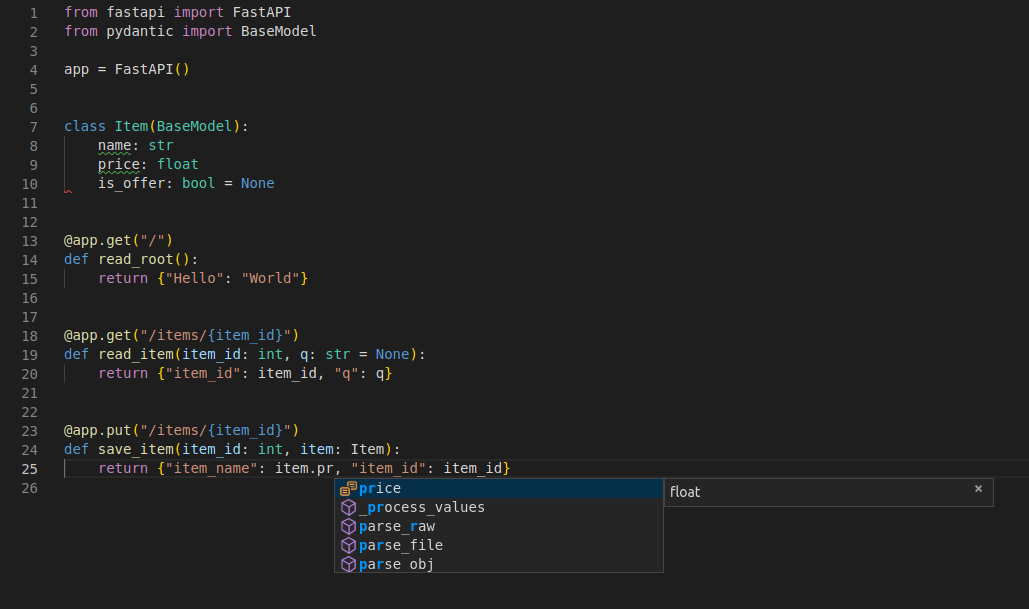 |
|||
|
|||
برای مشاهده مثالهای کاملتر که شامل قابلیتهای بیشتری از FastAPI باشد به بخش <a href="https://fastapi.tiangolo.com/tutorial/">آموزش - راهنمای کاربر</a> مراجعه کنید. |
|||
|
|||
**هشدار اسپویل**: بخش آموزش - راهنمای کاربر شامل موارد زیر است: |
|||
|
|||
* اعلان **پارامترهای** موجود در بخشهای دیگر درخواست، شامل: **سرآیند (هدر)ها**, **کوکیها**, **فیلدهای فرم** و **فایلها**. |
|||
* چگونگی تنظیم **<abbr title="Validation Constraints">محدودیتهای اعتبارسنجی</abbr>** به عنوان مثال `maximum_length` یا `regex`. |
|||
* سیستم **<abbr title="also known as components, resources, providers, services, injectables">Dependency Injection</abbr>** قوی و کاربردی. |
|||
* امنیت و تایید هویت, شامل پشتیبانی از **OAuth2** مبتنی بر **JWT tokens** و **HTTP Basic**. |
|||
* تکنیک پیشرفته برای تعریف **مدلهای چند سطحی JSON** (بر اساس Pydantic). |
|||
* قابلیتهای اضافی دیگر (بر اساس Starlette) شامل: |
|||
* **<abbr title="WebSocket">وبسوکت</abbr>** |
|||
* **GraphQL** |
|||
* تستهای خودکار آسان مبتنی بر `requests` و `pytest` |
|||
* **CORS** |
|||
* **Cookie Sessions** |
|||
* و موارد بیشمار دیگر. |
|||
|
|||
## کارایی |
|||
|
|||
معیار (بنچمارک)های مستقل TechEmpower حاکی از آن است که برنامههای **FastAPI** که تحت Uvicorn اجرا میشود، <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">یکی از سریعترین فریمورکهای مبتنی بر پایتون</a>, است که کمی ضعیفتر از Starlette و Uvicorn عمل میکند (فریمورک و سروری که FastAPI بر اساس آنها ایجاد شده است) (*) |
|||
|
|||
برای درک بهتری از این موضوع به بخش <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">بنچمارکها</a> مراجعه کنید. |
|||
|
|||
## نیازمندیهای اختیاری |
|||
|
|||
استفاده شده توسط Pydantic: |
|||
|
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - برای <abbr title="تبدیل دادههای موجود در درخواستهای HTTP به داده پایتونی">"تجزیه (parse)"</abbr> سریعتر JSON . |
|||
* <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - برای اعتبارسنجی آدرسهای ایمیل. |
|||
|
|||
استفاده شده توسط Starlette: |
|||
|
|||
* <a href="https://requests.readthedocs.io" target="_blank"><code>requests</code></a> - در صورتی که میخواهید از `TestClient` استفاده کنید. |
|||
* <a href="https://github.com/Tinche/aiofiles" target="_blank"><code>aiofiles</code></a> - در صورتی که میخواهید از `FileResponse` و `StaticFiles` استفاده کنید. |
|||
* <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - در صورتی که بخواهید از پیکربندی پیشفرض برای قالبها استفاده کنید. |
|||
* <a href="https://andrew-d.github.io/python-multipart/" target="_blank"><code>python-multipart</code></a> - در صورتی که بخواهید با استفاده از `request.form()` از قابلیت <abbr title="تبدیل رشته متنی موجود در درخواست HTTP به انواع داده پایتون">"تجزیه (parse)"</abbr> فرم استفاده کنید. |
|||
* <a href="https://pythonhosted.org/itsdangerous/" target="_blank"><code>itsdangerous</code></a> - در صورتی که بخواید از `SessionMiddleware` پشتیبانی کنید. |
|||
* <a href="https://pyyaml.org/wiki/PyYAMLDocumentation" target="_blank"><code>pyyaml</code></a> - برای پشتیبانی `SchemaGenerator` در Starlet (به احتمال زیاد برای کار کردن با FastAPI به آن نیازی پیدا نمیکنید.). |
|||
* <a href="https://graphene-python.org/" target="_blank"><code>graphene</code></a> - در صورتی که از `GraphQLApp` پشتیبانی میکنید. |
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - در صورتی که بخواهید از `UJSONResponse` استفاده کنید. |
|||
|
|||
استفاده شده توسط FastAPI / Starlette: |
|||
|
|||
* <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - برای سرور اجرا کننده برنامه وب. |
|||
* <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - در صورتی که بخواهید از `ORJSONResponse` استفاده کنید. |
|||
|
|||
میتوان همه این موارد را با استفاده از دستور `pip install fastapi[all]`. به صورت یکجا نصب کرد. |
|||
|
|||
## لایسنس |
|||
|
|||
این پروژه مشمول قوانین و مقررات لایسنس MIT است. |
@ -0,0 +1,143 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/fa/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: fa |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- de: /de/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: UA-133183413-1 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /de/ |
|||
name: de |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
@ -0,0 +1,464 @@ |
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>תשתית FastAPI, ביצועים גבוהים, קלה ללמידה, מהירה לתכנות, מוכנה לסביבת ייצור</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/tiangolo/fastapi/actions?query=workflow%3ATest+event%3Apush+branch%3Amaster" target="_blank"> |
|||
<img src="https://github.com/tiangolo/fastapi/workflows/Test/badge.svg?event=push&branch=master" alt="Test"> |
|||
</a> |
|||
<a href="https://codecov.io/gh/tiangolo/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/codecov/c/github/tiangolo/fastapi?color=%2334D058" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/pyversions/fastapi.svg?color=%2334D058" alt="Supported Python versions"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**תיעוד**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**קוד**: <a href="https://github.com/tiangolo/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
|
|||
FastAPI היא תשתית רשת מודרנית ומהירה (ביצועים גבוהים) לבניית ממשקי תכנות יישומים (API) עם פייתון 3.6+ בהתבסס על רמזי טיפוסים סטנדרטיים. |
|||
|
|||
תכונות המפתח הן: |
|||
|
|||
- **מהירה**: ביצועים גבוהים מאוד, בקנה אחד עם NodeJS ו - Go (תודות ל - Starlette ו - Pydantic). [אחת מתשתיות הפייתון המהירות ביותר](#performance). |
|||
|
|||
- **מהירה לתכנות**: הגבירו את מהירות פיתוח התכונות החדשות בכ - %200 עד %300. \* |
|||
- **פחות שגיאות**: מנעו כ - %40 משגיאות אנוש (מפתחים). \* |
|||
- **אינטואיטיבית**: תמיכת עורך מעולה. <abbr title="ידועה גם כהשלמה אוטומטית או IntelliSense">השלמה</abbr> בכל מקום. פחות זמן ניפוי שגיאות. |
|||
- **קלה**: מתוכננת להיות קלה לשימוש וללמידה. פחות זמן קריאת תיעוד. |
|||
- **קצרה**: מזערו שכפול קוד. מספר תכונות מכל הכרזת פרמטר. פחות שגיאות. |
|||
- **חסונה**: קבלו קוד מוכן לסביבת ייצור. עם תיעוד אינטרקטיבי אוטומטי. |
|||
- **מבוססת סטנדרטים**: מבוססת על (ותואמת לחלוטין ל -) הסטדנרטים הפתוחים לממשקי תכנות יישומים: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (ידועים לשעבר כ - Swagger) ו - <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>\* הערכה מבוססת על בדיקות של צוות פיתוח פנימי שבונה אפליקציות בסביבת ייצור.</small> |
|||
|
|||
## נותני חסות |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor -%} |
|||
{%- for sponsor in sponsors.silver -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">נותני חסות אחרים</a> |
|||
|
|||
## דעות |
|||
|
|||
"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/tiangolo/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_I’m over the moon excited about **FastAPI**. It’s so fun!_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast host</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_" |
|||
|
|||
"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> founders - <a href="https://spacy.io" target="_blank">spaCy</a> creators</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, ה - FastAPI של ממשקי שורת פקודה (CLI). |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
אם אתם בונים אפליקציית <abbr title="ממשק שורת פקודה">CLI</abbr> לשימוש במסוף במקום ממשק רשת, העיפו מבט על <a href="https://typer.tiangolo.com/" class="external-link" target="_blank">**Typer**</a>. |
|||
|
|||
**Typer** היא אחותה הקטנה של FastAPI. ומטרתה היא להיות ה - **FastAPI של ממשקי שורת פקודה**. ⌨️ 🚀 |
|||
|
|||
## תלויות |
|||
|
|||
פייתון 3.6+ |
|||
|
|||
FastAPI עומדת על כתפי ענקיות: |
|||
|
|||
- <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> לחלקי הרשת. |
|||
- <a href="https://pydantic-docs.helpmanual.io/" class="external-link" target="_blank">Pydantic</a> לחלקי המידע. |
|||
|
|||
## התקנה |
|||
|
|||
<div dir="ltr" class="termy"> |
|||
|
|||
```console |
|||
$ pip install fastapi |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
תצטרכו גם שרת ASGI כגון <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a> או <a href="https://gitlab.com/pgjones/hypercorn" class="external-link" target="_blank">Hypercorn</a>. |
|||
|
|||
<div dir="ltr" class="termy"> |
|||
|
|||
```console |
|||
$ pip install "uvicorn[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## דוגמא |
|||
|
|||
### צרו אותה |
|||
|
|||
- צרו קובץ בשם `main.py` עם: |
|||
|
|||
```Python |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>או השתמשו ב - <code>async def</code>...</summary> |
|||
|
|||
אם הקוד שלכם משתמש ב - `async` / `await`, השתמשו ב - `async def`: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**שימו לב**: |
|||
|
|||
אם אינכם יודעים, בדקו את פרק "ממהרים?" על <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` ו - `await` בתיעוד</a>. |
|||
|
|||
</details> |
|||
|
|||
### הריצו אותה |
|||
|
|||
התחילו את השרת עם: |
|||
|
|||
<div dir="ltr" class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [28720] |
|||
INFO: Started server process [28722] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>על הפקודה <code>uvicorn main:app --reload</code>...</summary> |
|||
|
|||
הפקודה `uvicorn main:app` מתייחסת ל: |
|||
|
|||
- `main`: הקובץ `main.py` (מודול פייתון). |
|||
- `app`: האובייקט שנוצר בתוך `main.py` עם השורה <code dir="ltr">app = FastAPI()</code>. |
|||
- <code dir="ltr">--reload</code>: גרמו לשרת להתאתחל לאחר שינויים בקוד. עשו זאת רק בסביבת פיתוח. |
|||
|
|||
</details> |
|||
|
|||
### בדקו אותה |
|||
|
|||
פתחו את הדפדפן שלכם בכתובת <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a>. |
|||
|
|||
אתם תראו תגובת JSON: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
כבר יצרתם API ש: |
|||
|
|||
- מקבל בקשות HTTP בנתיבים `/` ו - <code dir="ltr">/items/{item_id}</code>. |
|||
- שני ה _נתיבים_ מקבלים _בקשות_ `GET` (ידועות גם כ*מתודות* HTTP). |
|||
- ה _נתיב_ <code dir="ltr">/items/{item_id}</code> כולל \*פרמטר נתיב\_ `item_id` שאמור להיות `int`. |
|||
- ה _נתיב_ <code dir="ltr">/items/{item_id}</code> \*פרמטר שאילתא\_ אופציונלי `q`. |
|||
|
|||
### תיעוד API אינטרקטיבי |
|||
|
|||
כעת פנו לכתובת <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
אתם תראו את התיעוד האוטומטי (מסופק על ידי <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>): |
|||
|
|||
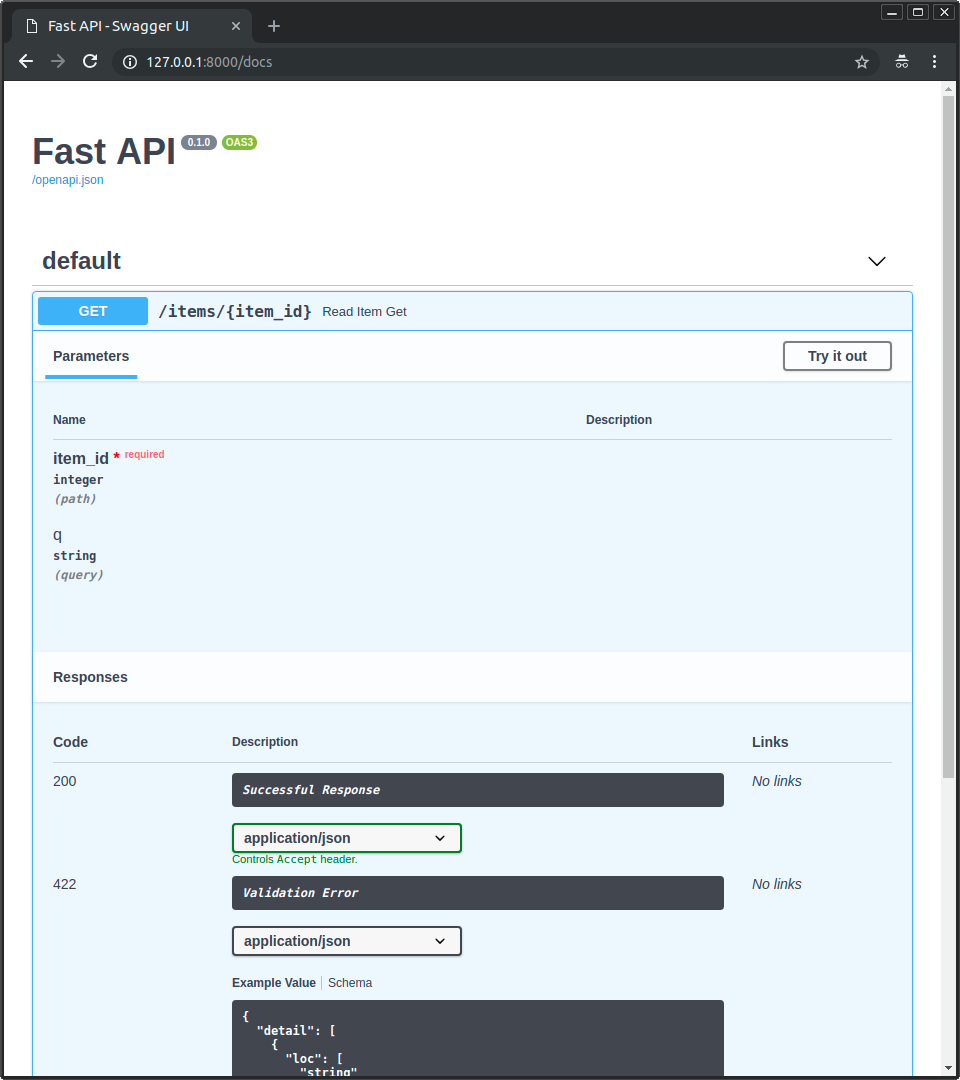 |
|||
|
|||
### תיעוד אלטרנטיבי |
|||
|
|||
כעת פנו לכתובת <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
אתם תראו תיעוד אלטרנטיבי (מסופק על ידי <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a>): |
|||
|
|||
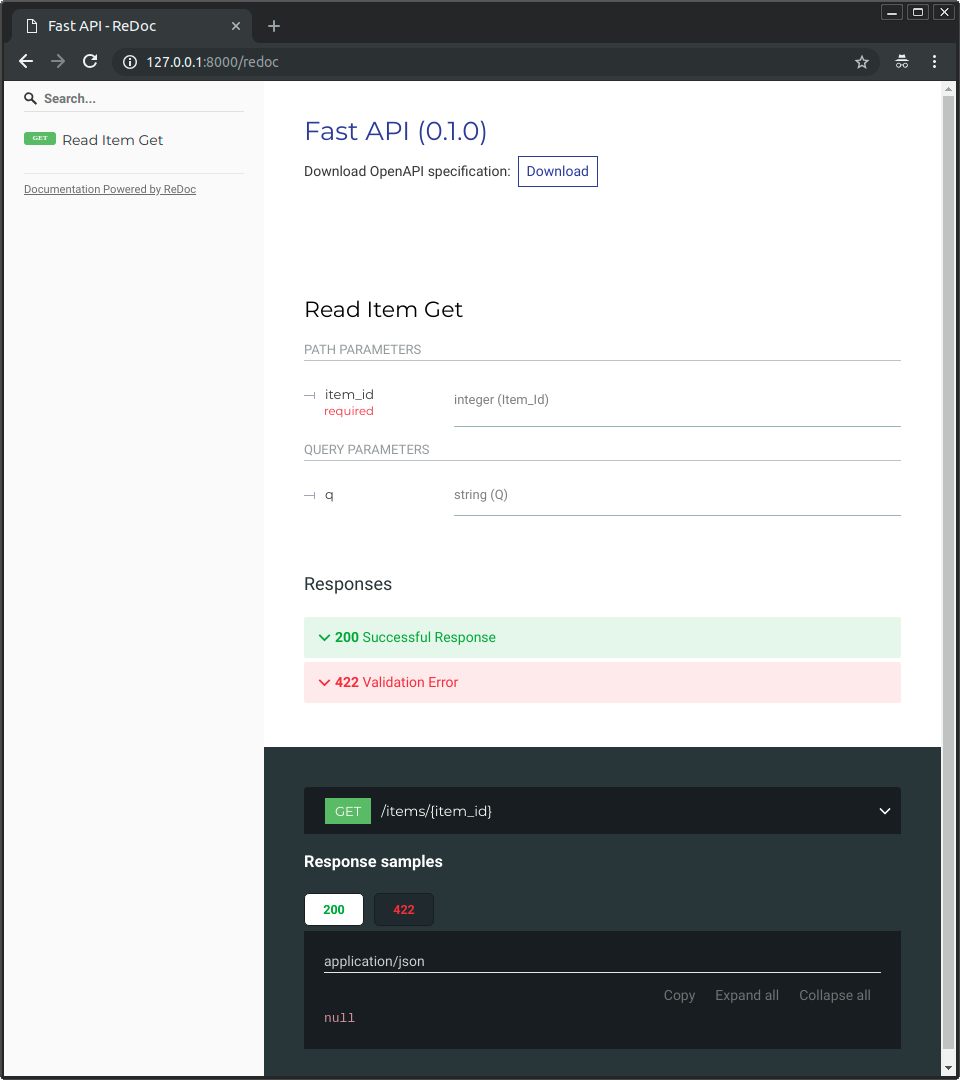 |
|||
|
|||
## שדרוג לדוגמא |
|||
|
|||
כעת ערכו את הקובץ `main.py` כך שיוכל לקבל גוף מבקשת `PUT`. |
|||
|
|||
הגדירו את הגוף בעזרת רמזי טיפוסים סטנדרטיים, הודות ל - `Pydantic`. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Union[bool, None] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
השרת אמול להתאתחל אוטומטית (מאחר והוספתם <code dir="ltr">--reload</code> לפקודת `uvicorn` שלמעלה). |
|||
|
|||
### שדרוג התיעוד האינטרקטיבי |
|||
|
|||
כעת פנו לכתובת <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
- התיעוד האוטומטי יתעדכן, כולל הגוף החדש: |
|||
|
|||
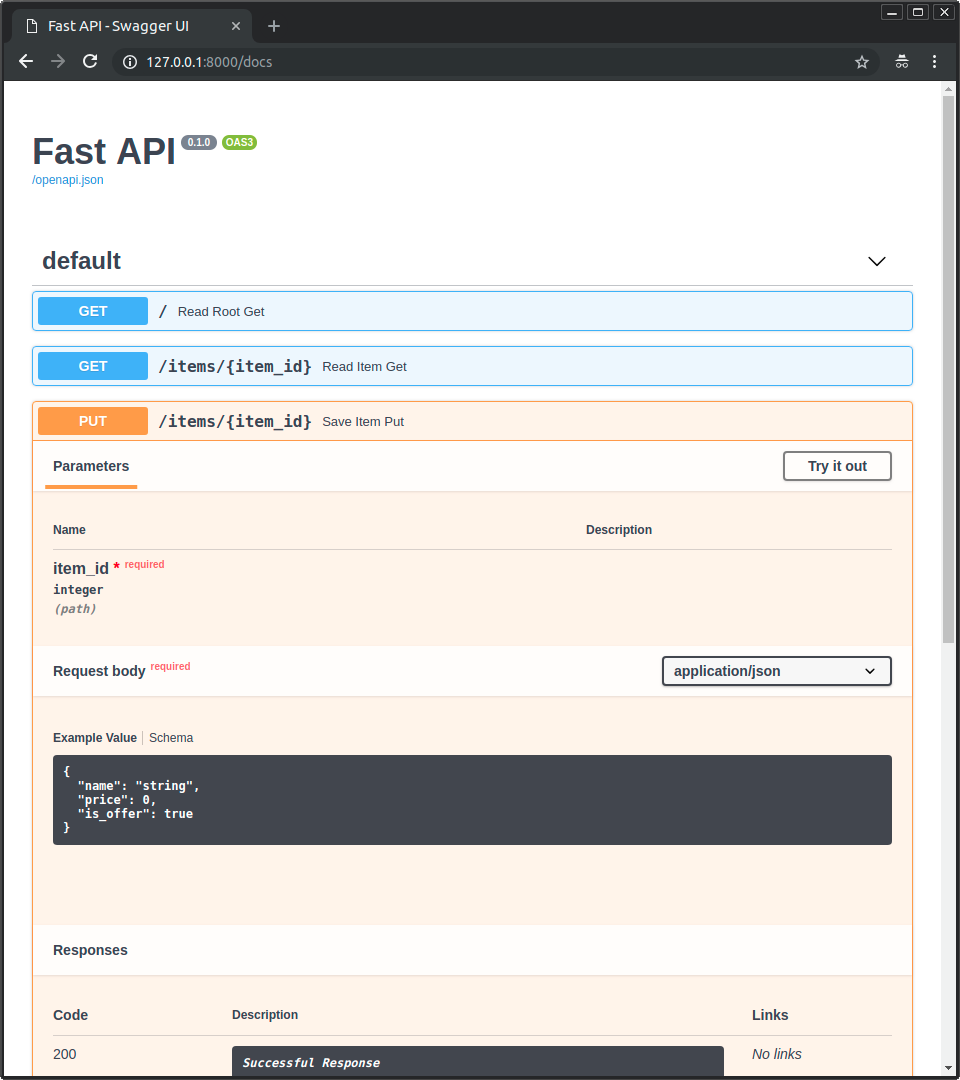 |
|||
|
|||
- לחצו על הכפתור "Try it out", הוא יאפשר לכם למלא את הפרמטרים ולעבוד ישירות מול ה - API. |
|||
|
|||
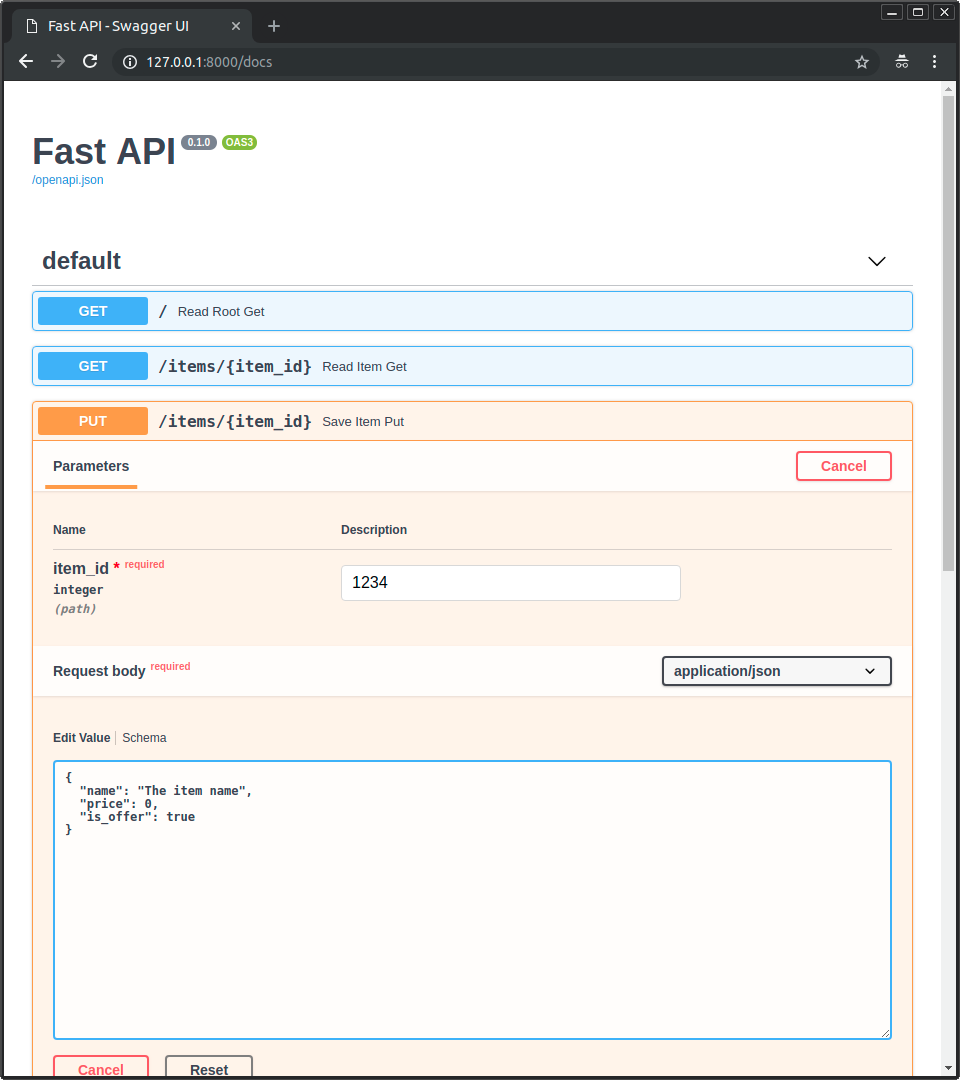 |
|||
|
|||
- אחר כך לחצו על הכפתור "Execute", האתר יתקשר עם ה - API שלכם, ישלח את הפרמטרים, ישיג את התוצאות ואז יראה אותן על המסך: |
|||
|
|||
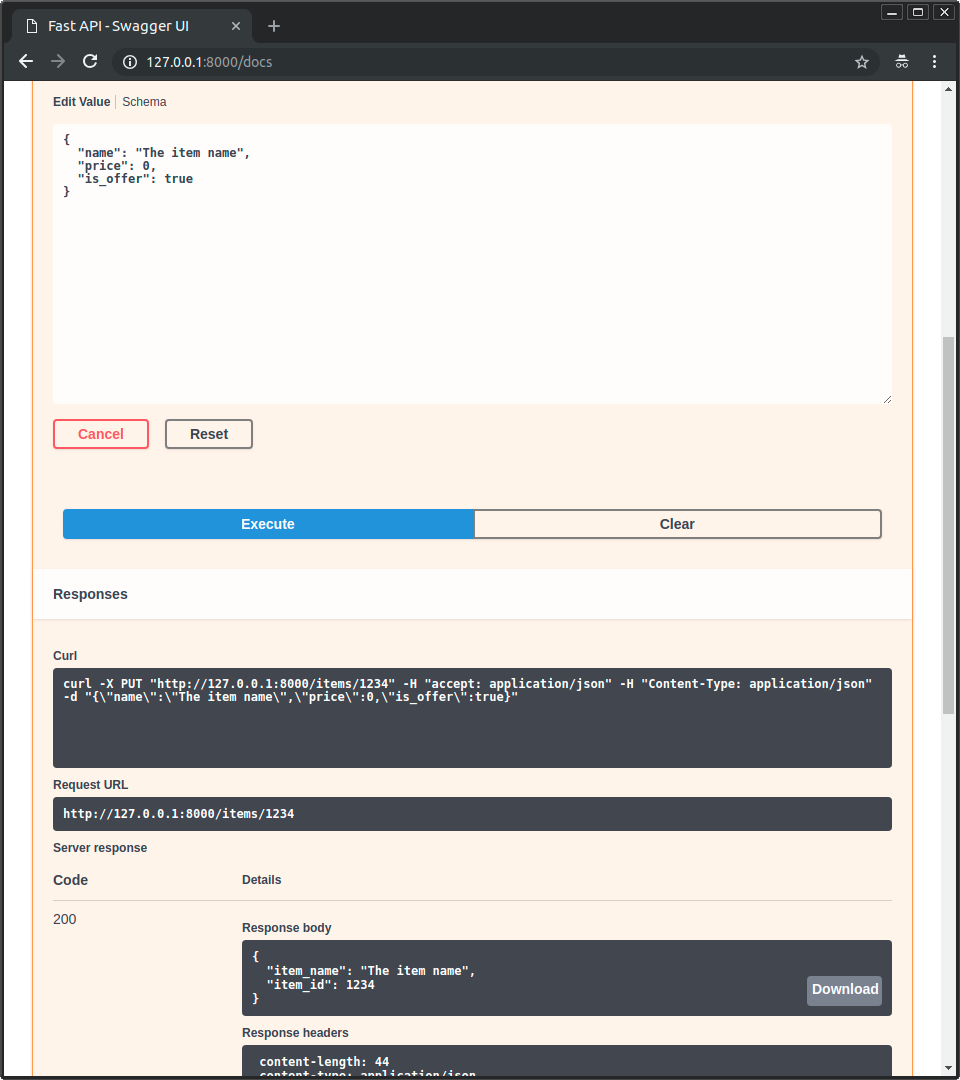 |
|||
|
|||
### שדרוג התיעוד האלטרנטיבי |
|||
|
|||
כעת פנו לכתובת <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
- התיעוד האלטרנטיבי גם יראה את פרמטר השאילתא והגוף החדשים. |
|||
|
|||
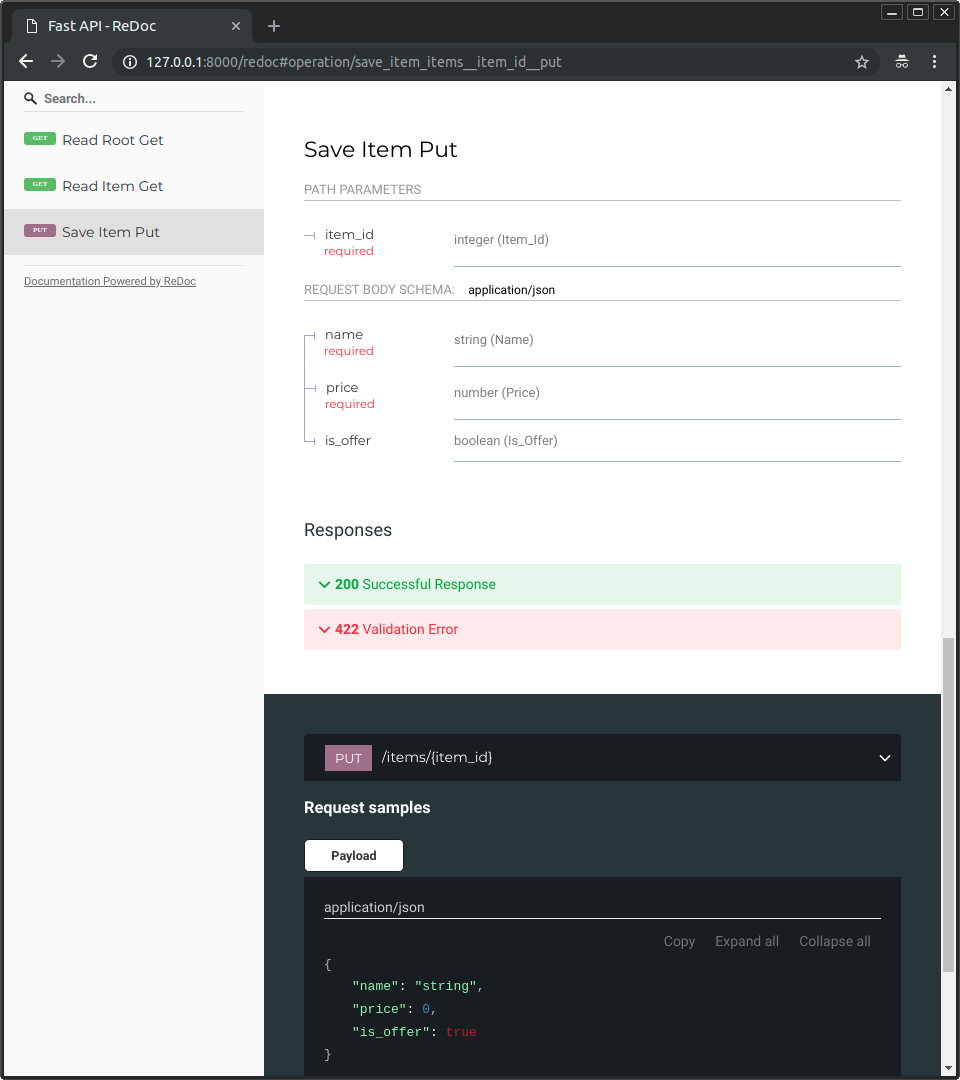 |
|||
|
|||
### סיכום |
|||
|
|||
לסיכום, אתם מכריזים ** פעם אחת** על טיפוסי הפרמטרים, גוף וכו' כפרמטרים לפונקציה. |
|||
|
|||
אתם עושים את זה עם טיפוסי פייתון מודרניים. |
|||
|
|||
אתם לא צריכים ללמוד תחביר חדש, מתודות או מחלקות של ספרייה ספיציפית, וכו' |
|||
|
|||
רק **פייתון 3.6+** סטנדרטי. |
|||
|
|||
לדוגמא, ל - `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
או למודל `Item` מורכב יותר: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...ועם הכרזת הטיפוס האחת הזו אתם מקבלים: |
|||
|
|||
- תמיכת עורך, כולל: |
|||
- השלמות. |
|||
- בדיקת טיפוסים. |
|||
- אימות מידע: |
|||
- שגיאות ברורות ואטומטיות כאשר מוכנס מידע לא חוקי . |
|||
- אימות אפילו לאובייקטי JSON מקוננים. |
|||
- <abbr title="ידועה גם כ: פרסור, סיריאליזציה">המרה</abbr> של מידע קלט: המרה של מידע שמגיע מהרשת למידע וטיפוסים של פייתון. קורא מ: |
|||
- JSON. |
|||
- פרמטרי נתיב. |
|||
- פרמטרי שאילתא. |
|||
- עוגיות. |
|||
- כותרות. |
|||
- טפסים. |
|||
- קבצים. |
|||
- <abbr title="ידועה גם כ: פרסור, סיריאליזציה">המרה</abbr> של מידע פלט: המרה של מידע וטיפוסים מפייתון למידע רשת (כ - JSON): |
|||
- המירו טיפוסי פייתון (`str`, `int`, `float`, `bool`, `list`, etc). |
|||
- עצמי `datetime`. |
|||
- עצמי `UUID`. |
|||
- מודלי בסיסי נתונים. |
|||
- ...ורבים אחרים. |
|||
- תיעוד API אוטומטי ואינטרקטיבית כולל שתי אלטרנטיבות לממשק המשתמש: |
|||
- Swagger UI. |
|||
- ReDoc. |
|||
|
|||
--- |
|||
|
|||
בחזרה לדוגמאת הקוד הקודמת, **FastAPI** ידאג: |
|||
|
|||
- לאמת שיש `item_id` בנתיב בבקשות `GET` ו - `PUT`. |
|||
- לאמת שה - `item_id` הוא מטיפוס `int` בבקשות `GET` ו - `PUT`. |
|||
- אם הוא לא, הלקוח יראה שגיאה ברורה ושימושית. |
|||
- לבדוק האם קיים פרמטר שאילתא בשם `q` (קרי `http://127.0.0.1:8000/items/foo?q=somequery`) לבקשות `GET`. |
|||
- מאחר והפרמטר `q` מוגדר עם <code dir="ltr"> = None</code>, הוא אופציונלי. |
|||
- לולא ה - `None` הוא היה חובה (כמו הגוף במקרה של `PUT`). |
|||
- לבקשות `PUT` לנתיב <code dir="ltr">/items/{item_id}</code>, לקרוא את גוף הבקשה כ - JSON: |
|||
- לאמת שהוא כולל את מאפיין החובה `name` שאמור להיות מטיפוס `str`. |
|||
- לאמת שהוא כולל את מאפיין החובה `price` שחייב להיות מטיפוס `float`. |
|||
- לבדוק האם הוא כולל את מאפיין הרשות `is_offer` שאמור להיות מטיפוס `bool`, אם הוא נמצא. |
|||
- כל זה יעבוד גם לאובייקט JSON מקונן. |
|||
- להמיר מ - JSON ול- JSON אוטומטית. |
|||
- לתעד הכל באמצעות OpenAPI, תיעוד שבו יוכלו להשתמש: |
|||
- מערכות תיעוד אינטרקטיביות. |
|||
- מערכות ייצור קוד אוטומטיות, להרבה שפות. |
|||
- לספק ישירות שתי מערכות תיעוד רשתיות. |
|||
|
|||
--- |
|||
|
|||
רק גרדנו את קצה הקרחון, אבל כבר יש לכם רעיון של איך הכל עובד. |
|||
|
|||
נסו לשנות את השורה: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
...מ: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
...ל: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
...וראו איך העורך שלכם משלים את המאפיינים ויודע את הטיפוסים שלהם: |
|||
|
|||
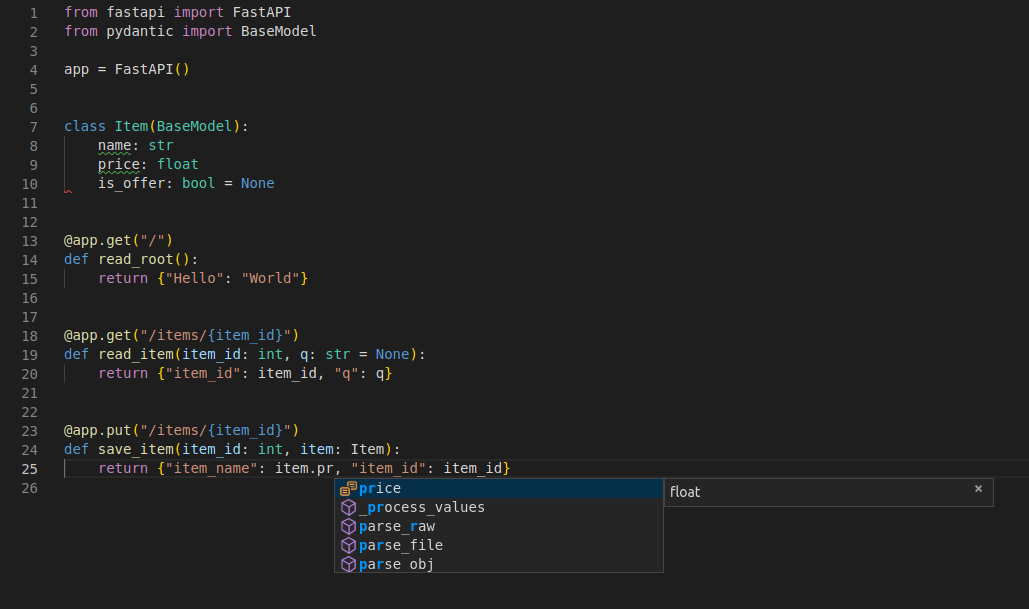 |
|||
|
|||
לדוגמא יותר שלמה שכוללת עוד תכונות, ראו את ה<a href="https://fastapi.tiangolo.com/tutorial/">מדריך - למשתמש</a>. |
|||
|
|||
**התראת ספוילרים**: המדריך - למשתמש כולל: |
|||
|
|||
- הכרזה על **פרמטרים** ממקורות אחרים ושונים כגון: **כותרות**, **עוגיות**, **טפסים** ו - **קבצים**. |
|||
- איך לקבוע **מגבלות אימות** בעזרת `maximum_length` או `regex`. |
|||
- דרך חזקה וקלה להשתמש ב**<abbr title="ידועה גם כרכיבים, משאבים, ספקים, שירותים, מוזרקים">הזרקת תלויות</abbr>**. |
|||
- אבטחה והתאמתות, כולל תמיכה ב - **OAuth2** עם **JWT** והתאמתות **HTTP Basic**. |
|||
- טכניקות מתקדמות (אבל קלות באותה מידה) להכרזת אובייקטי JSON מקוננים (תודות ל - Pydantic). |
|||
- אינטרקציה עם **GraphQL** דרך <a href="https://strawberry.rocks" class="external-link" target="_blank">Strawberry</a> וספריות אחרות. |
|||
- תכונות נוספות רבות (תודות ל - Starlette) כגון: |
|||
- **WebSockets** |
|||
- בדיקות קלות במיוחד מבוססות על `requests` ו - `pytest` |
|||
- **CORS** |
|||
- **Cookie Sessions** |
|||
- ...ועוד. |
|||
|
|||
## ביצועים |
|||
|
|||
בדיקות עצמאיות של TechEmpower הראו שאפליקציות **FastAPI** שרצות תחת Uvicorn הן <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">מתשתיות הפייתון המהירות ביותר</a>, רק מתחת ל - Starlette ו - Uvicorn עצמן (ש - FastAPI מבוססת עליהן). (\*) |
|||
|
|||
כדי להבין עוד על הנושא, ראו את הפרק <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">Benchmarks</a>. |
|||
|
|||
## תלויות אופציונליות |
|||
|
|||
בשימוש Pydantic: |
|||
|
|||
- <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - <abbr title="המרת המחרוזת שמגיעה מבקשת HTTP למידע פייתון">"פרסור"</abbr> JSON. |
|||
- <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - לאימות כתובות אימייל. |
|||
|
|||
בשימוש Starlette: |
|||
|
|||
- <a href="https://requests.readthedocs.io" target="_blank"><code>requests</code></a> - דרוש אם ברצונכם להשתמש ב - `TestClient`. |
|||
- <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - דרוש אם ברצונכם להשתמש בברירת המחדל של תצורת הטמפלייטים. |
|||
- <a href="https://andrew-d.github.io/python-multipart/" target="_blank"><code>python-multipart</code></a> - דרוש אם ברצונכם לתמוך ב <abbr title="המרת המחרוזת שמגיעה מבקשת HTTP למידע פייתון">"פרסור"</abbr> טפסים, באצמעות <code dir="ltr">request.form()</code>. |
|||
- <a href="https://pythonhosted.org/itsdangerous/" target="_blank"><code>itsdangerous</code></a> - דרוש אם ברצונכם להשתמש ב - `SessionMiddleware`. |
|||
- <a href="https://pyyaml.org/wiki/PyYAMLDocumentation" target="_blank"><code>pyyaml</code></a> - דרוש אם ברצונכם להשתמש ב - `SchemaGenerator` של Starlette (כנראה שאתם לא צריכים את זה עם FastAPI). |
|||
- <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - דרוש אם ברצונכם להשתמש ב - `UJSONResponse`. |
|||
|
|||
בשימוש FastAPI / Starlette: |
|||
|
|||
- <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - לשרת שטוען ומגיש את האפליקציה שלכם. |
|||
- <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - דרוש אם ברצונכם להשתמש ב - `ORJSONResponse`. |
|||
|
|||
תוכלו להתקין את כל אלו באמצעות <code dir="ltr">pip install "fastapi[all]"</code>. |
|||
|
|||
## רשיון |
|||
|
|||
הפרויקט הזה הוא תחת התנאים של רשיון MIT. |
@ -0,0 +1,143 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/he/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: he |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- de: /de/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: UA-133183413-1 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /de/ |
|||
name: de |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
@ -0,0 +1,58 @@ |
|||
# 条件付き OpenAPI |
|||
|
|||
必要であれば、設定と環境変数を利用して、環境に応じて条件付きでOpenAPIを構成することが可能です。また、完全にOpenAPIを無効にすることもできます。 |
|||
|
|||
## セキュリティとAPI、およびドキュメントについて |
|||
|
|||
本番環境においてドキュメントのUIを非表示にすることによって、APIを保護しようと *すべきではありません*。 |
|||
|
|||
それは、APIのセキュリティの強化にはならず、*path operations* は依然として利用可能です。 |
|||
|
|||
もしセキュリティ上の欠陥がソースコードにあるならば、それは存在したままです。 |
|||
|
|||
ドキュメンテーションを非表示にするのは、単にあなたのAPIへのアクセス方法を難解にするだけでなく、同時にあなた自身の本番環境でのAPIのデバッグを困難にしてしまう可能性があります。単純に、 <a href="https://en.wikipedia.org/wiki/Security_through_obscurity" class="external-link" target="_blank">Security through obscurity</a> の一つの形態として考えられるでしょう。 |
|||
|
|||
もしあなたのAPIのセキュリティを強化したいなら、いくつかのよりよい方法があります。例を示すと、 |
|||
|
|||
* リクエストボディとレスポンスのためのPydanticモデルの定義を見直す。 |
|||
* 依存関係に基づきすべての必要なパーミッションとロールを設定する。 |
|||
* パスワードを絶対に平文で保存しない。パスワードハッシュのみを保存する。 |
|||
* PasslibやJWTトークンに代表される、よく知られた暗号化ツールを使って実装する。 |
|||
* そして必要なところでは、もっと細かいパーミッション制御をOAuth2スコープを使って行う。 |
|||
* など |
|||
|
|||
それでも、例えば本番環境のような特定の環境のみで、あるいは環境変数の設定によってAPIドキュメントをどうしても無効にしたいという、非常に特殊なユースケースがあるかもしれません。 |
|||
|
|||
## 設定と環境変数による条件付き OpenAPI |
|||
|
|||
生成するOpenAPIとドキュメントUIの構成は、共通のPydanticの設定を使用して簡単に切り替えられます。 |
|||
|
|||
例えば、 |
|||
|
|||
```Python hl_lines="6 11" |
|||
{!../../../docs_src/conditional_openapi/tutorial001.py!} |
|||
``` |
|||
|
|||
ここでは `openapi_url` の設定を、デフォルトの `"/openapi.json"` のまま宣言しています。 |
|||
|
|||
そして、これを `FastAPI` appを作る際に使います。 |
|||
|
|||
それから、以下のように `OPENAPI_URL` という環境変数を空文字列に設定することによってOpenAPI (UIドキュメントを含む) を無効化することができます。 |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ OPENAPI_URL= uvicorn main:app |
|||
|
|||
<span style="color: green;">INFO</span>: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
すると、以下のように `/openapi.json`, `/docs`, `/redoc` のどのURLにアクセスしても、 `404 Not Found` エラーが返ってくるようになります。 |
|||
|
|||
```JSON |
|||
{ |
|||
"detail": "Not Found" |
|||
} |
|||
``` |
@ -0,0 +1,24 @@ |
|||
# ユーザーガイド 応用編 |
|||
|
|||
## さらなる機能 |
|||
|
|||
[チュートリアル - ユーザーガイド](../tutorial/){.internal-link target=_blank}により、**FastAPI**の主要な機能は十分に理解できたことでしょう。 |
|||
|
|||
以降のセクションでは、チュートリアルでは説明しきれなかったオプションや設定、および機能について説明します。 |
|||
|
|||
!!! tip "豆知識" |
|||
以降のセクションは、 **必ずしも"応用編"ではありません**。 |
|||
|
|||
ユースケースによっては、その中から解決策を見つけられるかもしれません。 |
|||
|
|||
## 先にチュートリアルを読む |
|||
|
|||
[チュートリアル - ユーザーガイド](../tutorial/){.internal-link target=_blank}の知識があれば、**FastAPI**の主要な機能を利用することができます。 |
|||
|
|||
以降のセクションは、すでにチュートリアルを読んで、その主要なアイデアを理解できていることを前提としています。 |
|||
|
|||
## テスト駆動開発のコース |
|||
|
|||
このセクションの内容を補完するために脱初心者用コースを受けたい場合は、**TestDriven.io**による、<a href="https://testdriven.io/courses/tdd-fastapi/" class="external-link" target="_blank">Test-Driven Development with FastAPI and Docker</a>を確認するのがよいかもしれません。 |
|||
|
|||
現在、このコースで得られた利益の10%が**FastAPI**の開発のために寄付されています。🎉 😄 |