committed by
GitHub
599 changed files with 22774 additions and 15642 deletions
@ -1,7 +0,0 @@ |
|||
FROM python:3.7 |
|||
|
|||
RUN pip install httpx PyGithub "pydantic==1.5.1" |
|||
|
|||
COPY ./app /app |
|||
|
|||
CMD ["python", "/app/main.py"] |
@ -1,10 +0,0 @@ |
|||
name: "Watch docs previews in PRs" |
|||
description: "Check PRs and trigger new docs deploys" |
|||
author: "Sebastián Ramírez <tiangolo@gmail.com>" |
|||
inputs: |
|||
token: |
|||
description: 'Token for the repo. Can be passed in using {{ secrets.GITHUB_TOKEN }}' |
|||
required: true |
|||
runs: |
|||
using: 'docker' |
|||
image: 'Dockerfile' |
@ -1,101 +0,0 @@ |
|||
import logging |
|||
from datetime import datetime |
|||
from pathlib import Path |
|||
from typing import List, Union |
|||
|
|||
import httpx |
|||
from github import Github |
|||
from github.NamedUser import NamedUser |
|||
from pydantic import BaseModel, BaseSettings, SecretStr |
|||
|
|||
github_api = "https://api.github.com" |
|||
netlify_api = "https://api.netlify.com" |
|||
|
|||
|
|||
class Settings(BaseSettings): |
|||
input_token: SecretStr |
|||
github_repository: str |
|||
github_event_path: Path |
|||
github_event_name: Union[str, None] = None |
|||
|
|||
|
|||
class Artifact(BaseModel): |
|||
id: int |
|||
node_id: str |
|||
name: str |
|||
size_in_bytes: int |
|||
url: str |
|||
archive_download_url: str |
|||
expired: bool |
|||
created_at: datetime |
|||
updated_at: datetime |
|||
|
|||
|
|||
class ArtifactResponse(BaseModel): |
|||
total_count: int |
|||
artifacts: List[Artifact] |
|||
|
|||
|
|||
def get_message(commit: str) -> str: |
|||
return f"Docs preview for commit {commit} at" |
|||
|
|||
|
|||
if __name__ == "__main__": |
|||
logging.basicConfig(level=logging.INFO) |
|||
settings = Settings() |
|||
logging.info(f"Using config: {settings.json()}") |
|||
g = Github(settings.input_token.get_secret_value()) |
|||
repo = g.get_repo(settings.github_repository) |
|||
owner: NamedUser = repo.owner |
|||
headers = {"Authorization": f"token {settings.input_token.get_secret_value()}"} |
|||
prs = list(repo.get_pulls(state="open")) |
|||
response = httpx.get( |
|||
f"{github_api}/repos/{settings.github_repository}/actions/artifacts", |
|||
headers=headers, |
|||
) |
|||
data = response.json() |
|||
artifacts_response = ArtifactResponse.parse_obj(data) |
|||
for pr in prs: |
|||
logging.info("-----") |
|||
logging.info(f"Processing PR #{pr.number}: {pr.title}") |
|||
pr_comments = list(pr.get_issue_comments()) |
|||
pr_commits = list(pr.get_commits()) |
|||
last_commit = pr_commits[0] |
|||
for pr_commit in pr_commits: |
|||
if pr_commit.commit.author.date > last_commit.commit.author.date: |
|||
last_commit = pr_commit |
|||
commit = last_commit.commit.sha |
|||
logging.info(f"Last commit: {commit}") |
|||
message = get_message(commit) |
|||
notified = False |
|||
for pr_comment in pr_comments: |
|||
if message in pr_comment.body: |
|||
notified = True |
|||
logging.info(f"Docs preview was notified: {notified}") |
|||
if not notified: |
|||
artifact_name = f"docs-zip-{commit}" |
|||
use_artifact: Union[Artifact, None] = None |
|||
for artifact in artifacts_response.artifacts: |
|||
if artifact.name == artifact_name: |
|||
use_artifact = artifact |
|||
break |
|||
if not use_artifact: |
|||
logging.info("Artifact not available") |
|||
else: |
|||
logging.info(f"Existing artifact: {use_artifact.name}") |
|||
response = httpx.post( |
|||
"https://api.github.com/repos/tiangolo/fastapi/actions/workflows/preview-docs.yml/dispatches", |
|||
headers=headers, |
|||
json={ |
|||
"ref": "master", |
|||
"inputs": { |
|||
"pr": f"{pr.number}", |
|||
"name": artifact_name, |
|||
"commit": commit, |
|||
}, |
|||
}, |
|||
) |
|||
logging.info( |
|||
f"Trigger sent, response status: {response.status_code} - content: {response.content}" |
|||
) |
|||
logging.info("Finished") |
@ -1,466 +0,0 @@ |
|||
|
|||
{!../../../docs/missing-translation.md!} |
|||
|
|||
|
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>FastAPI framework, high performance, easy to learn, fast to code, ready for production</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/tiangolo/fastapi/actions?query=workflow%3ATest" target="_blank"> |
|||
<img src="https://github.com/tiangolo/fastapi/workflows/Test/badge.svg" alt="Test"> |
|||
</a> |
|||
<a href="https://codecov.io/gh/tiangolo/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/codecov/c/github/tiangolo/fastapi?color=%2334D058" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**Documentation**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**Source Code**: <a href="https://github.com/tiangolo/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
|
|||
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. |
|||
|
|||
The key features are: |
|||
|
|||
* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance). |
|||
|
|||
* **Fast to code**: Increase the speed to develop features by about 200% to 300%. * |
|||
* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. * |
|||
* **Intuitive**: Great editor support. <abbr title="also known as auto-complete, autocompletion, IntelliSense">Completion</abbr> everywhere. Less time debugging. |
|||
* **Easy**: Designed to be easy to use and learn. Less time reading docs. |
|||
* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs. |
|||
* **Robust**: Get production-ready code. With automatic interactive documentation. |
|||
* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (previously known as Swagger) and <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>* estimation based on tests on an internal development team, building production applications.</small> |
|||
|
|||
## Sponsors |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor -%} |
|||
{%- for sponsor in sponsors.silver -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">Other sponsors</a> |
|||
|
|||
## Opinions |
|||
|
|||
"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/tiangolo/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_I’m over the moon excited about **FastAPI**. It’s so fun!_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast host</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_" |
|||
|
|||
"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> founders - <a href="https://spacy.io" target="_blank">spaCy</a> creators</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, the FastAPI of CLIs |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
If you are building a <abbr title="Command Line Interface">CLI</abbr> app to be used in the terminal instead of a web API, check out <a href="https://typer.tiangolo.com/" class="external-link" target="_blank">**Typer**</a>. |
|||
|
|||
**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀 |
|||
|
|||
## Requirements |
|||
|
|||
Python 3.7+ |
|||
|
|||
FastAPI stands on the shoulders of giants: |
|||
|
|||
* <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> for the web parts. |
|||
* <a href="https://pydantic-docs.helpmanual.io/" class="external-link" target="_blank">Pydantic</a> for the data parts. |
|||
|
|||
## Installation |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install fastapi |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
You will also need an ASGI server, for production such as <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a> or <a href="https://gitlab.com/pgjones/hypercorn" class="external-link" target="_blank">Hypercorn</a>. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "uvicorn[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Example |
|||
|
|||
### Create it |
|||
|
|||
* Create a file `main.py` with: |
|||
|
|||
```Python |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Optional[str] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>Or use <code>async def</code>...</summary> |
|||
|
|||
If your code uses `async` / `await`, use `async def`: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Optional[str] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**Note**: |
|||
|
|||
If you don't know, check the _"In a hurry?"_ section about <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` and `await` in the docs</a>. |
|||
|
|||
</details> |
|||
|
|||
### Run it |
|||
|
|||
Run the server with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [28720] |
|||
INFO: Started server process [28722] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>About the command <code>uvicorn main:app --reload</code>...</summary> |
|||
|
|||
The command `uvicorn main:app` refers to: |
|||
|
|||
* `main`: the file `main.py` (the Python "module"). |
|||
* `app`: the object created inside of `main.py` with the line `app = FastAPI()`. |
|||
* `--reload`: make the server restart after code changes. Only do this for development. |
|||
|
|||
</details> |
|||
|
|||
### Check it |
|||
|
|||
Open your browser at <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a>. |
|||
|
|||
You will see the JSON response as: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
You already created an API that: |
|||
|
|||
* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`. |
|||
* Both _paths_ take `GET` <em>operations</em> (also known as HTTP _methods_). |
|||
* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`. |
|||
* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`. |
|||
|
|||
### Interactive API docs |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
You will see the automatic interactive API documentation (provided by <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>): |
|||
|
|||
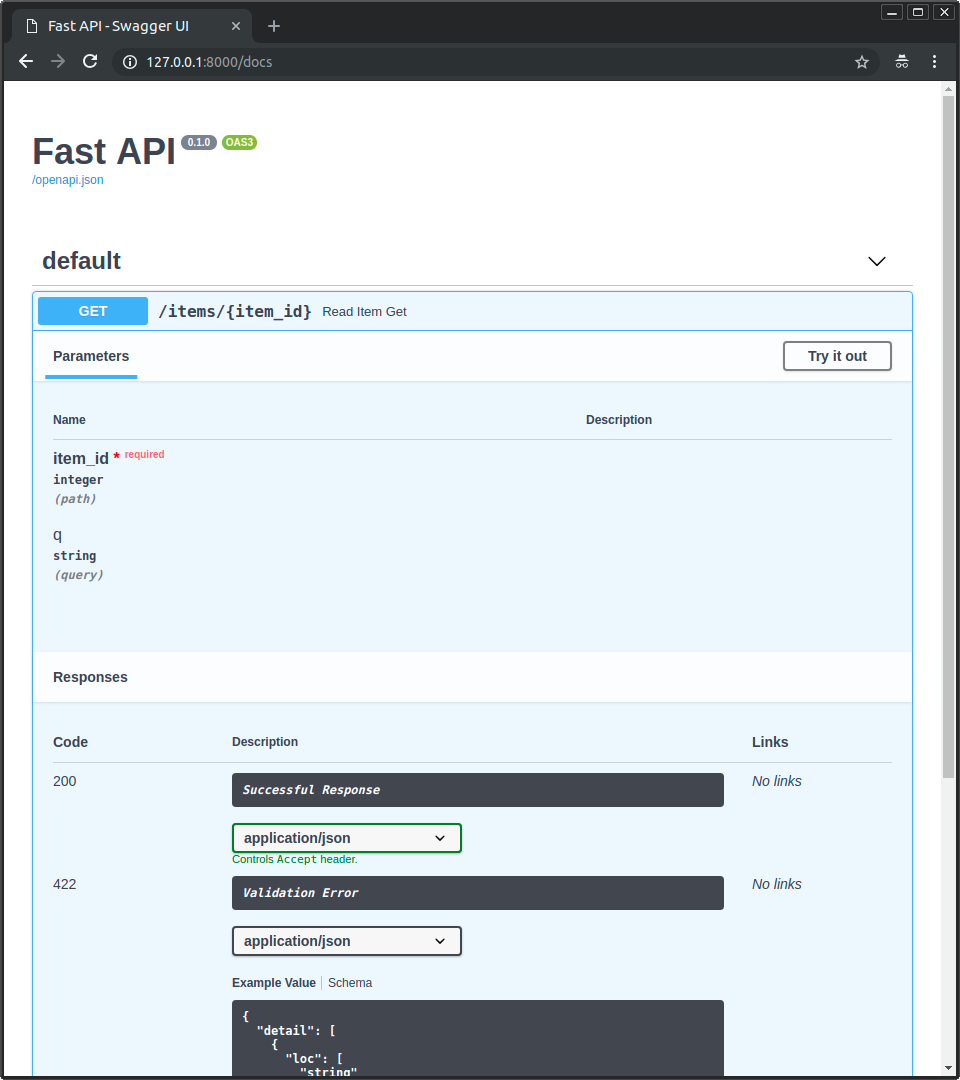 |
|||
|
|||
### Alternative API docs |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
You will see the alternative automatic documentation (provided by <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a>): |
|||
|
|||
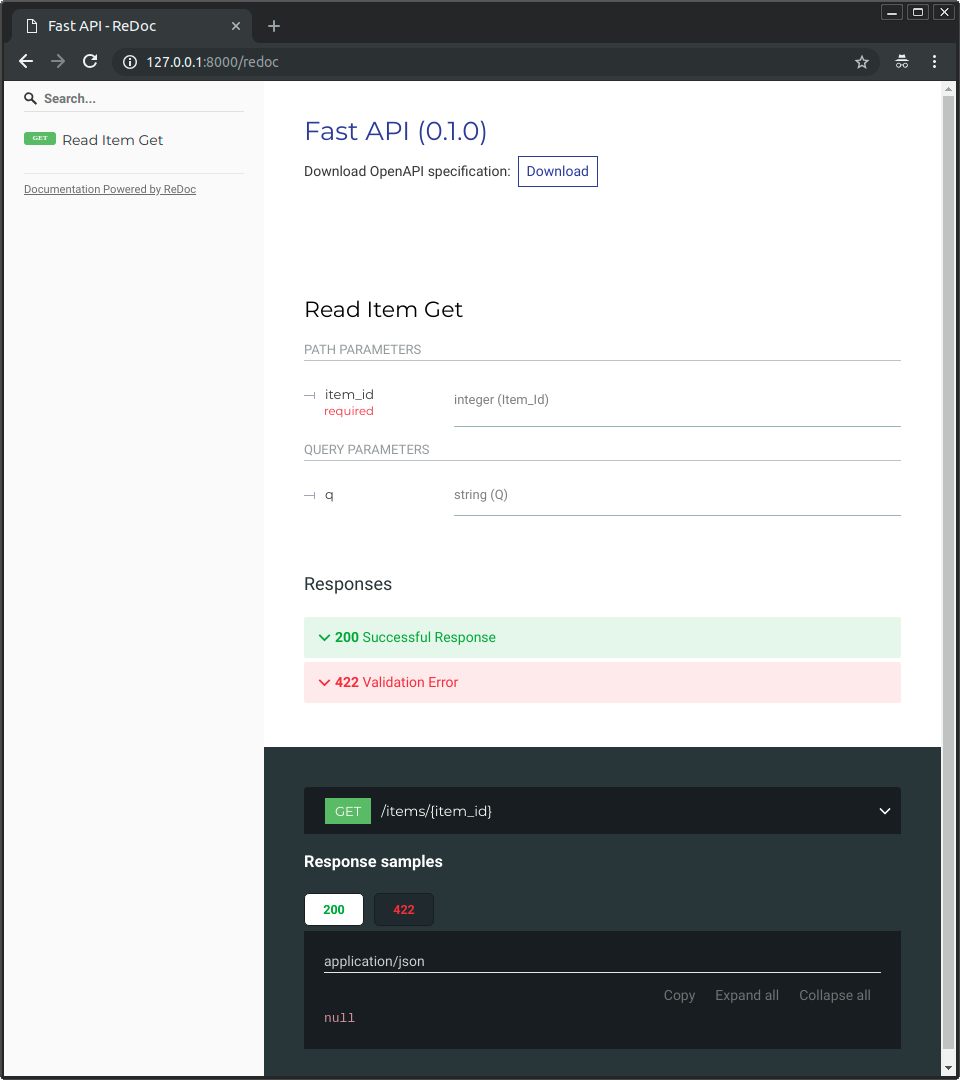 |
|||
|
|||
## Example upgrade |
|||
|
|||
Now modify the file `main.py` to receive a body from a `PUT` request. |
|||
|
|||
Declare the body using standard Python types, thanks to Pydantic. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Optional[bool] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Optional[str] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
The server should reload automatically (because you added `--reload` to the `uvicorn` command above). |
|||
|
|||
### Interactive API docs upgrade |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
* The interactive API documentation will be automatically updated, including the new body: |
|||
|
|||
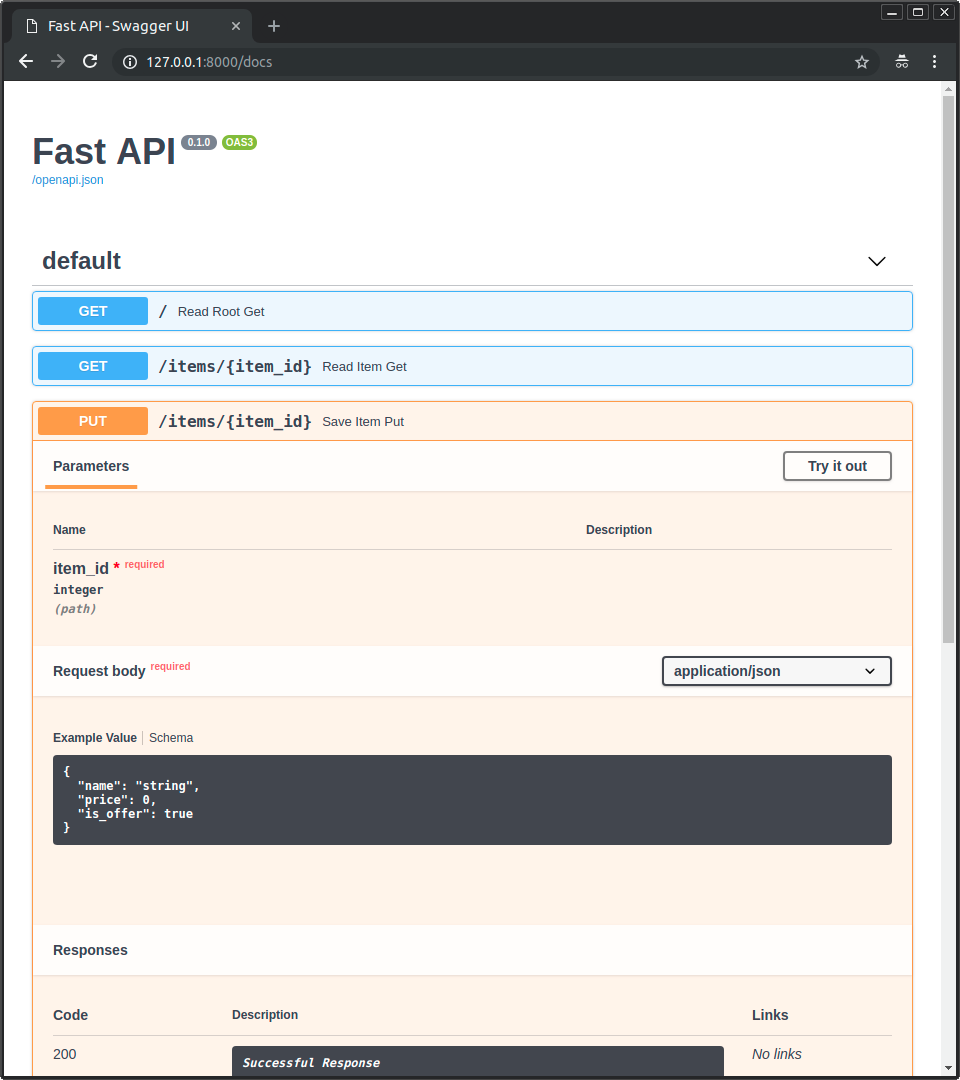 |
|||
|
|||
* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API: |
|||
|
|||
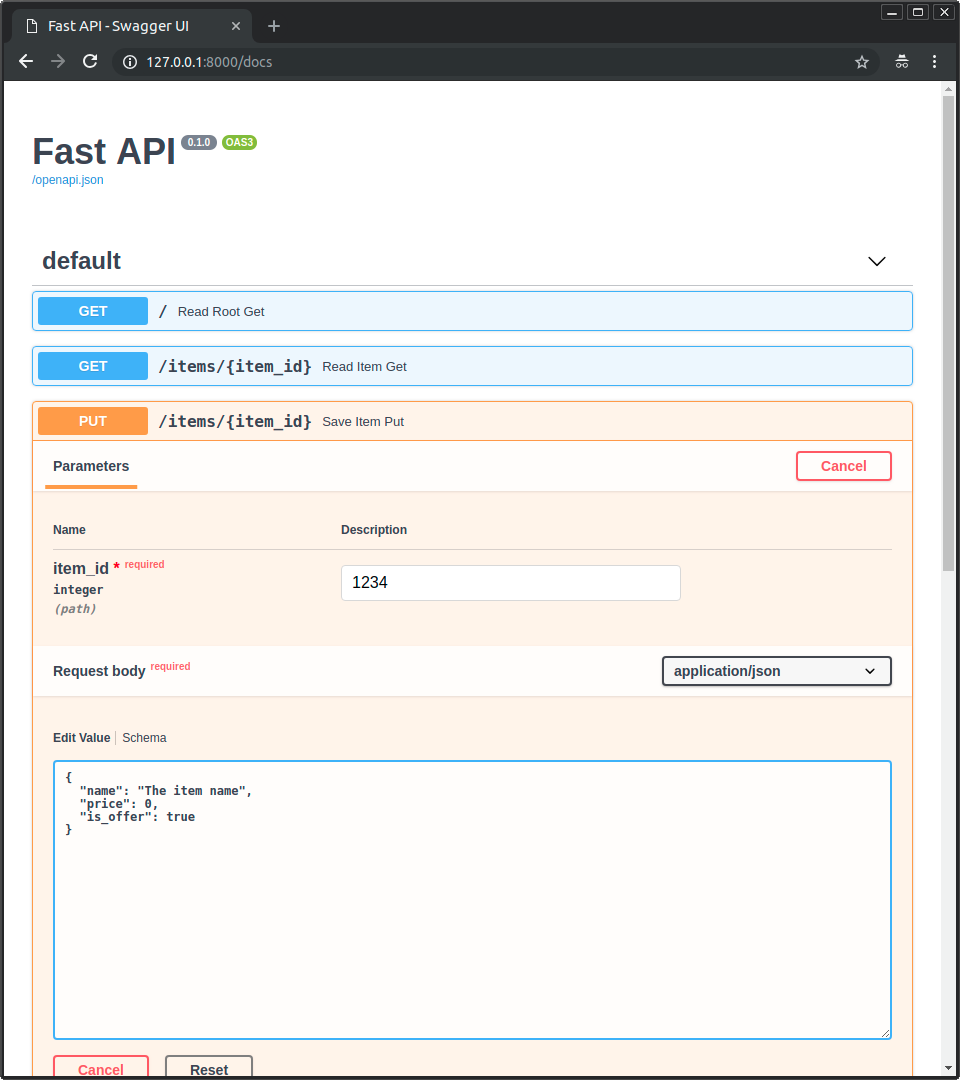 |
|||
|
|||
* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen: |
|||
|
|||
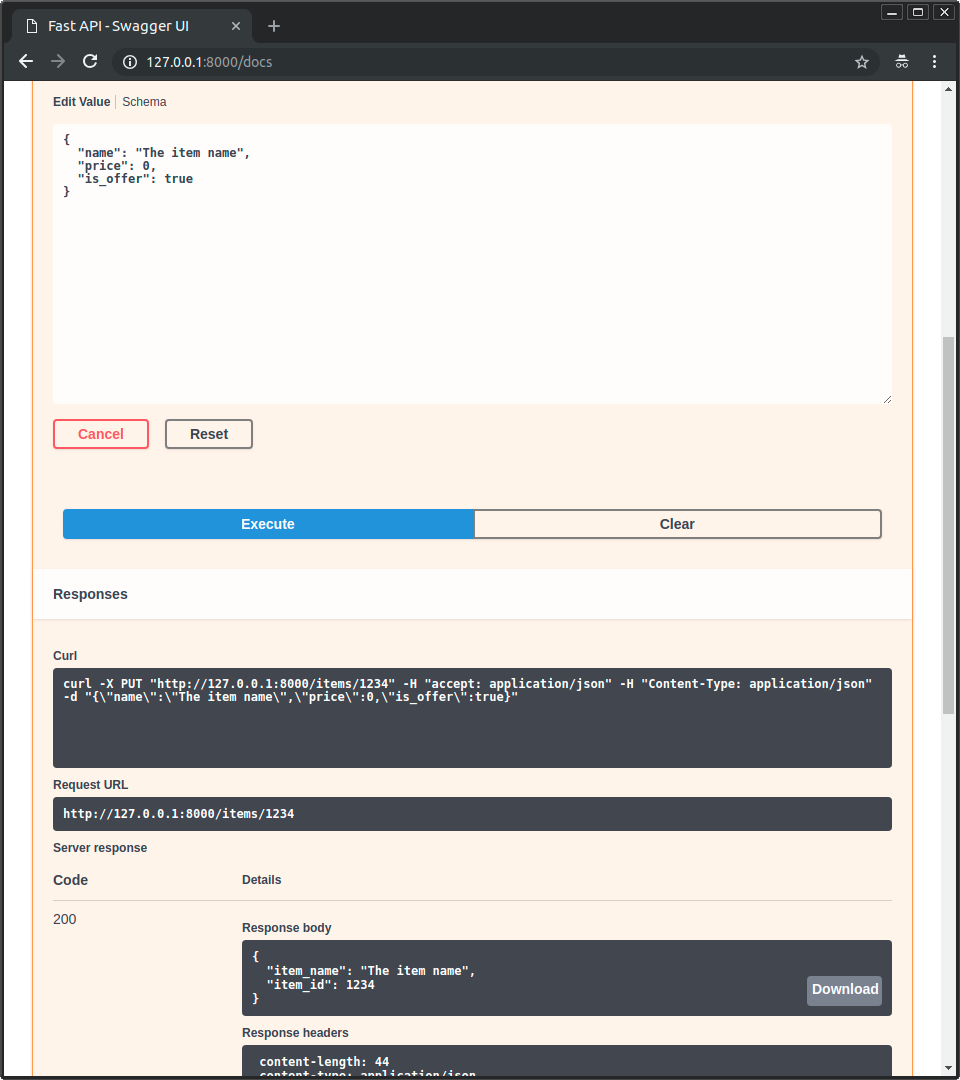 |
|||
|
|||
### Alternative API docs upgrade |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
* The alternative documentation will also reflect the new query parameter and body: |
|||
|
|||
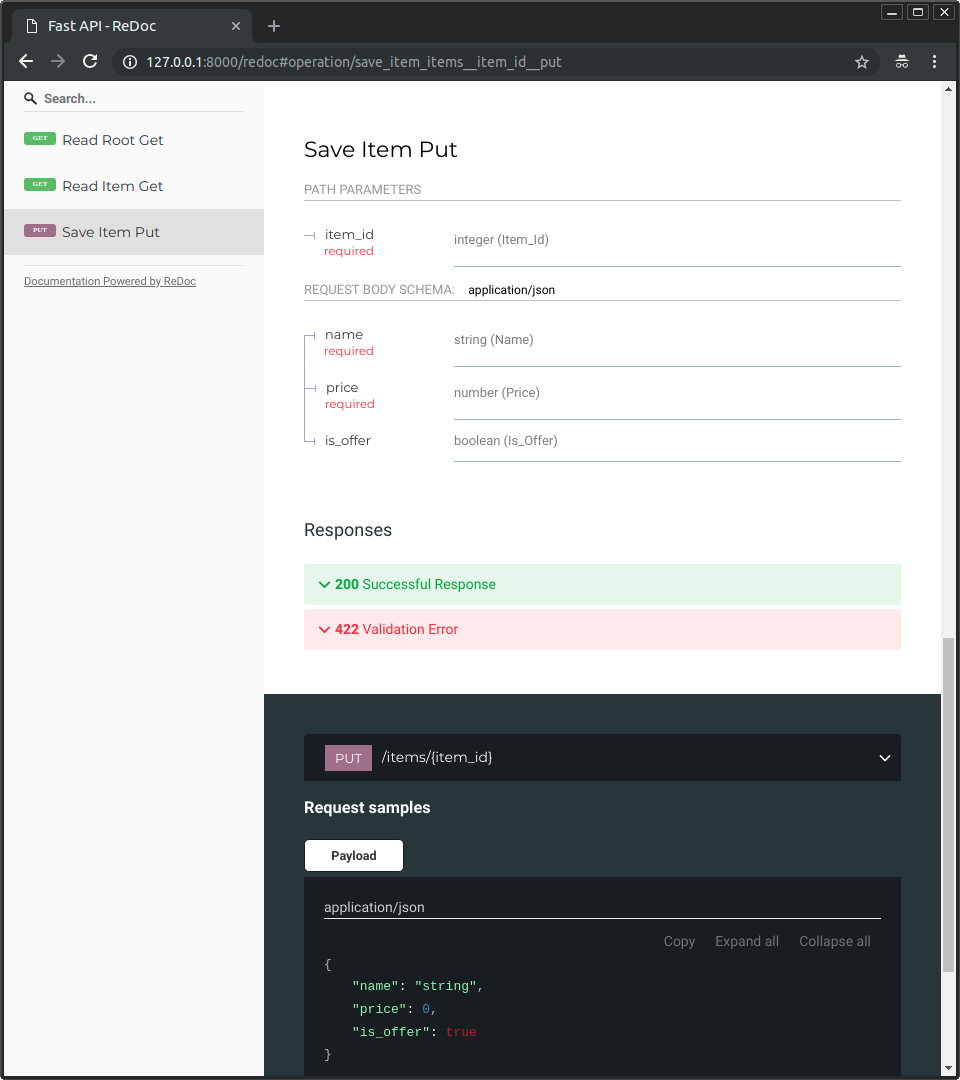 |
|||
|
|||
### Recap |
|||
|
|||
In summary, you declare **once** the types of parameters, body, etc. as function parameters. |
|||
|
|||
You do that with standard modern Python types. |
|||
|
|||
You don't have to learn a new syntax, the methods or classes of a specific library, etc. |
|||
|
|||
Just standard **Python 3.6+**. |
|||
|
|||
For example, for an `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
or for a more complex `Item` model: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...and with that single declaration you get: |
|||
|
|||
* Editor support, including: |
|||
* Completion. |
|||
* Type checks. |
|||
* Validation of data: |
|||
* Automatic and clear errors when the data is invalid. |
|||
* Validation even for deeply nested JSON objects. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of input data: coming from the network to Python data and types. Reading from: |
|||
* JSON. |
|||
* Path parameters. |
|||
* Query parameters. |
|||
* Cookies. |
|||
* Headers. |
|||
* Forms. |
|||
* Files. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of output data: converting from Python data and types to network data (as JSON): |
|||
* Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc). |
|||
* `datetime` objects. |
|||
* `UUID` objects. |
|||
* Database models. |
|||
* ...and many more. |
|||
* Automatic interactive API documentation, including 2 alternative user interfaces: |
|||
* Swagger UI. |
|||
* ReDoc. |
|||
|
|||
--- |
|||
|
|||
Coming back to the previous code example, **FastAPI** will: |
|||
|
|||
* Validate that there is an `item_id` in the path for `GET` and `PUT` requests. |
|||
* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests. |
|||
* If it is not, the client will see a useful, clear error. |
|||
* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests. |
|||
* As the `q` parameter is declared with `= None`, it is optional. |
|||
* Without the `None` it would be required (as is the body in the case with `PUT`). |
|||
* For `PUT` requests to `/items/{item_id}`, Read the body as JSON: |
|||
* Check that it has a required attribute `name` that should be a `str`. |
|||
* Check that it has a required attribute `price` that has to be a `float`. |
|||
* Check that it has an optional attribute `is_offer`, that should be a `bool`, if present. |
|||
* All this would also work for deeply nested JSON objects. |
|||
* Convert from and to JSON automatically. |
|||
* Document everything with OpenAPI, that can be used by: |
|||
* Interactive documentation systems. |
|||
* Automatic client code generation systems, for many languages. |
|||
* Provide 2 interactive documentation web interfaces directly. |
|||
|
|||
--- |
|||
|
|||
We just scratched the surface, but you already get the idea of how it all works. |
|||
|
|||
Try changing the line with: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
...from: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
...to: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
...and see how your editor will auto-complete the attributes and know their types: |
|||
|
|||
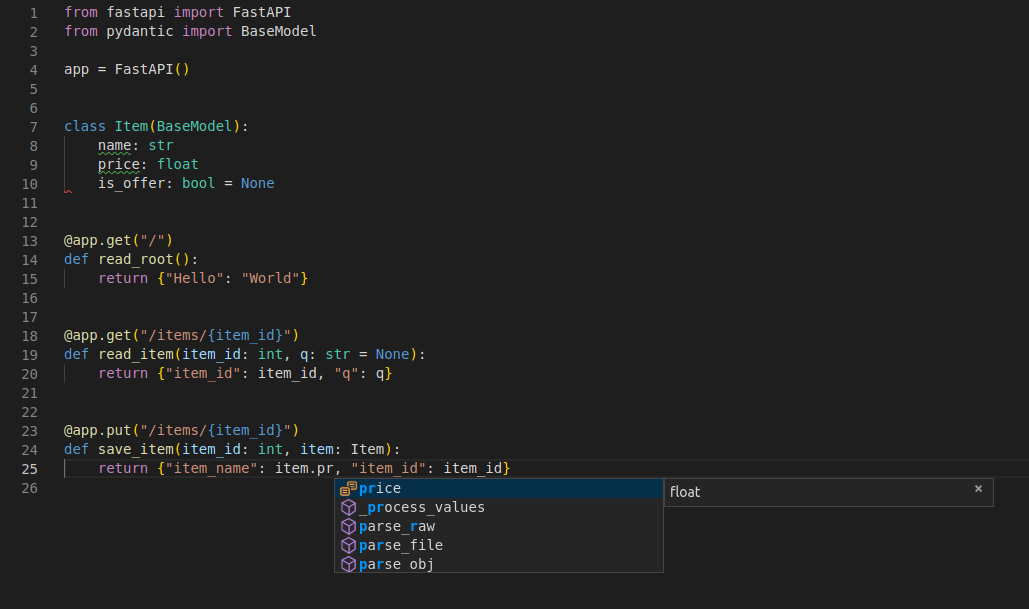 |
|||
|
|||
For a more complete example including more features, see the <a href="https://fastapi.tiangolo.com/tutorial/">Tutorial - User Guide</a>. |
|||
|
|||
**Spoiler alert**: the tutorial - user guide includes: |
|||
|
|||
* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**. |
|||
* How to set **validation constraints** as `maximum_length` or `regex`. |
|||
* A very powerful and easy to use **<abbr title="also known as components, resources, providers, services, injectables">Dependency Injection</abbr>** system. |
|||
* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth. |
|||
* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic). |
|||
* Many extra features (thanks to Starlette) as: |
|||
* **WebSockets** |
|||
* **GraphQL** |
|||
* extremely easy tests based on `requests` and `pytest` |
|||
* **CORS** |
|||
* **Cookie Sessions** |
|||
* ...and more. |
|||
|
|||
## Performance |
|||
|
|||
Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">one of the fastest Python frameworks available</a>, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*) |
|||
|
|||
To understand more about it, see the section <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">Benchmarks</a>. |
|||
|
|||
## Optional Dependencies |
|||
|
|||
Used by Pydantic: |
|||
|
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - for faster JSON <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>. |
|||
* <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - for email validation. |
|||
|
|||
Used by Starlette: |
|||
|
|||
* <a href="https://www.python-httpx.org" target="_blank"><code>httpx</code></a> - Required if you want to use the `TestClient`. |
|||
* <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - Required if you want to use the default template configuration. |
|||
* <a href="https://andrew-d.github.io/python-multipart/" target="_blank"><code>python-multipart</code></a> - Required if you want to support form <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>, with `request.form()`. |
|||
* <a href="https://pythonhosted.org/itsdangerous/" target="_blank"><code>itsdangerous</code></a> - Required for `SessionMiddleware` support. |
|||
* <a href="https://pyyaml.org/wiki/PyYAMLDocumentation" target="_blank"><code>pyyaml</code></a> - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI). |
|||
* <a href="https://graphene-python.org/" target="_blank"><code>graphene</code></a> - Required for `GraphQLApp` support. |
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - Required if you want to use `UJSONResponse`. |
|||
|
|||
Used by FastAPI / Starlette: |
|||
|
|||
* <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - for the server that loads and serves your application. |
|||
* <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - Required if you want to use `ORJSONResponse`. |
|||
|
|||
You can install all of these with `pip install fastapi[all]`. |
|||
|
|||
## License |
|||
|
|||
This project is licensed under the terms of the MIT license. |
@ -1,160 +0,0 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/az/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: en |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
@ -1,473 +0,0 @@ |
|||
|
|||
{!../../../docs/missing-translation.md!} |
|||
|
|||
|
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>FastAPI framework, high performance, easy to learn, fast to code, ready for production</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/tiangolo/fastapi/actions?query=workflow%3ATest+event%3Apush+branch%3Amaster" target="_blank"> |
|||
<img src="https://github.com/tiangolo/fastapi/workflows/Test/badge.svg?event=push&branch=master" alt="Test"> |
|||
</a> |
|||
<a href="https://coverage-badge.samuelcolvin.workers.dev/redirect/tiangolo/fastapi" target="_blank"> |
|||
<img src="https://coverage-badge.samuelcolvin.workers.dev/tiangolo/fastapi.svg" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/pyversions/fastapi.svg?color=%2334D058" alt="Supported Python versions"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**Documentation**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**Source Code**: <a href="https://github.com/tiangolo/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
|
|||
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. |
|||
|
|||
The key features are: |
|||
|
|||
* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance). |
|||
* **Fast to code**: Increase the speed to develop features by about 200% to 300%. * |
|||
* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. * |
|||
* **Intuitive**: Great editor support. <abbr title="also known as auto-complete, autocompletion, IntelliSense">Completion</abbr> everywhere. Less time debugging. |
|||
* **Easy**: Designed to be easy to use and learn. Less time reading docs. |
|||
* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs. |
|||
* **Robust**: Get production-ready code. With automatic interactive documentation. |
|||
* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (previously known as Swagger) and <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>* estimation based on tests on an internal development team, building production applications.</small> |
|||
|
|||
## Sponsors |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor -%} |
|||
{%- for sponsor in sponsors.silver -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">Other sponsors</a> |
|||
|
|||
## Opinions |
|||
|
|||
"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/tiangolo/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_I’m over the moon excited about **FastAPI**. It’s so fun!_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast host</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_" |
|||
|
|||
"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> founders - <a href="https://spacy.io" target="_blank">spaCy</a> creators</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_If anyone is looking to build a production Python API, I would highly recommend **FastAPI**. It is **beautifully designed**, **simple to use** and **highly scalable**, it has become a **key component** in our API first development strategy and is driving many automations and services such as our Virtual TAC Engineer._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Deon Pillsbury - <strong>Cisco</strong> <a href="https://www.linkedin.com/posts/deonpillsbury_cisco-cx-python-activity-6963242628536487936-trAp/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, the FastAPI of CLIs |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
If you are building a <abbr title="Command Line Interface">CLI</abbr> app to be used in the terminal instead of a web API, check out <a href="https://typer.tiangolo.com/" class="external-link" target="_blank">**Typer**</a>. |
|||
|
|||
**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀 |
|||
|
|||
## Requirements |
|||
|
|||
Python 3.7+ |
|||
|
|||
FastAPI stands on the shoulders of giants: |
|||
|
|||
* <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> for the web parts. |
|||
* <a href="https://pydantic-docs.helpmanual.io/" class="external-link" target="_blank">Pydantic</a> for the data parts. |
|||
|
|||
## Installation |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install fastapi |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
You will also need an ASGI server, for production such as <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a> or <a href="https://github.com/pgjones/hypercorn" class="external-link" target="_blank">Hypercorn</a>. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "uvicorn[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Example |
|||
|
|||
### Create it |
|||
|
|||
* Create a file `main.py` with: |
|||
|
|||
```Python |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>Or use <code>async def</code>...</summary> |
|||
|
|||
If your code uses `async` / `await`, use `async def`: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**Note**: |
|||
|
|||
If you don't know, check the _"In a hurry?"_ section about <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` and `await` in the docs</a>. |
|||
|
|||
</details> |
|||
|
|||
### Run it |
|||
|
|||
Run the server with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [28720] |
|||
INFO: Started server process [28722] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>About the command <code>uvicorn main:app --reload</code>...</summary> |
|||
|
|||
The command `uvicorn main:app` refers to: |
|||
|
|||
* `main`: the file `main.py` (the Python "module"). |
|||
* `app`: the object created inside of `main.py` with the line `app = FastAPI()`. |
|||
* `--reload`: make the server restart after code changes. Only do this for development. |
|||
|
|||
</details> |
|||
|
|||
### Check it |
|||
|
|||
Open your browser at <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a>. |
|||
|
|||
You will see the JSON response as: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
You already created an API that: |
|||
|
|||
* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`. |
|||
* Both _paths_ take `GET` <em>operations</em> (also known as HTTP _methods_). |
|||
* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`. |
|||
* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`. |
|||
|
|||
### Interactive API docs |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
You will see the automatic interactive API documentation (provided by <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>): |
|||
|
|||
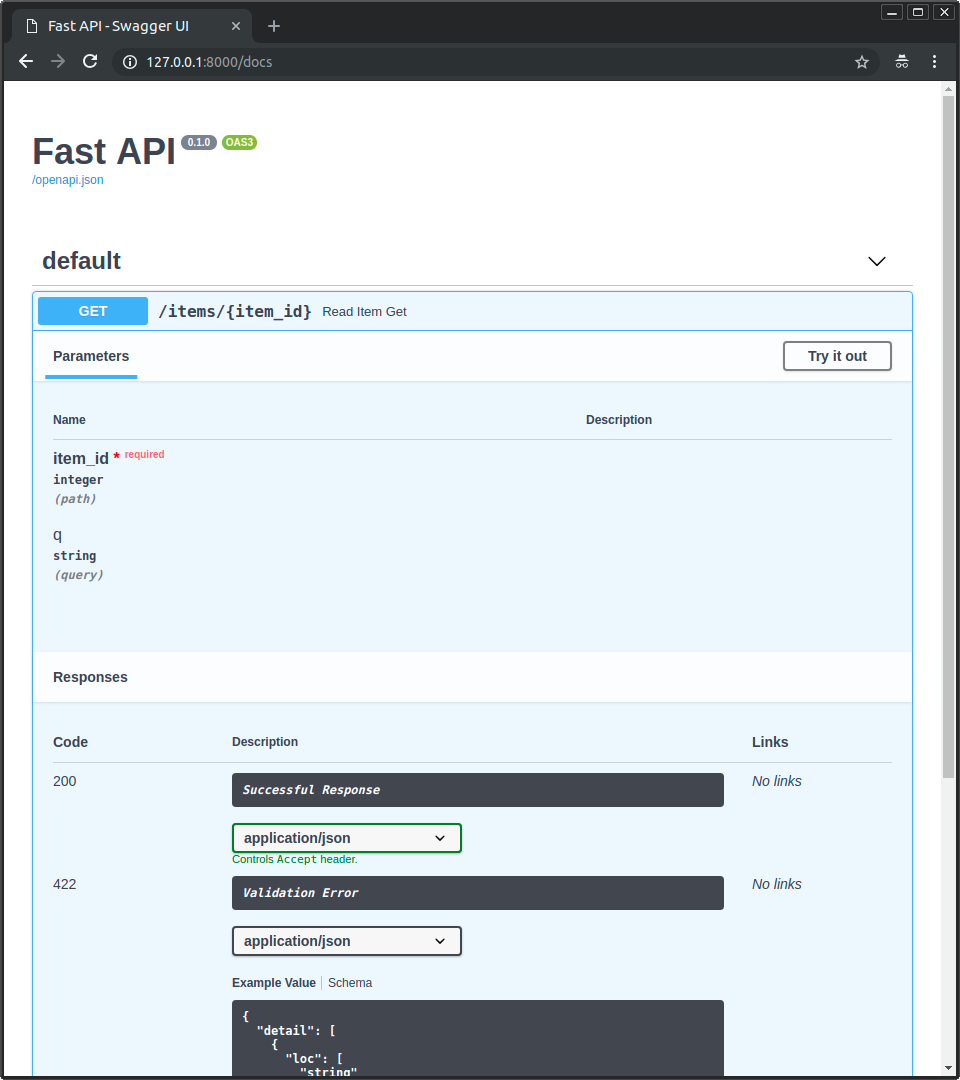 |
|||
|
|||
### Alternative API docs |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
You will see the alternative automatic documentation (provided by <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a>): |
|||
|
|||
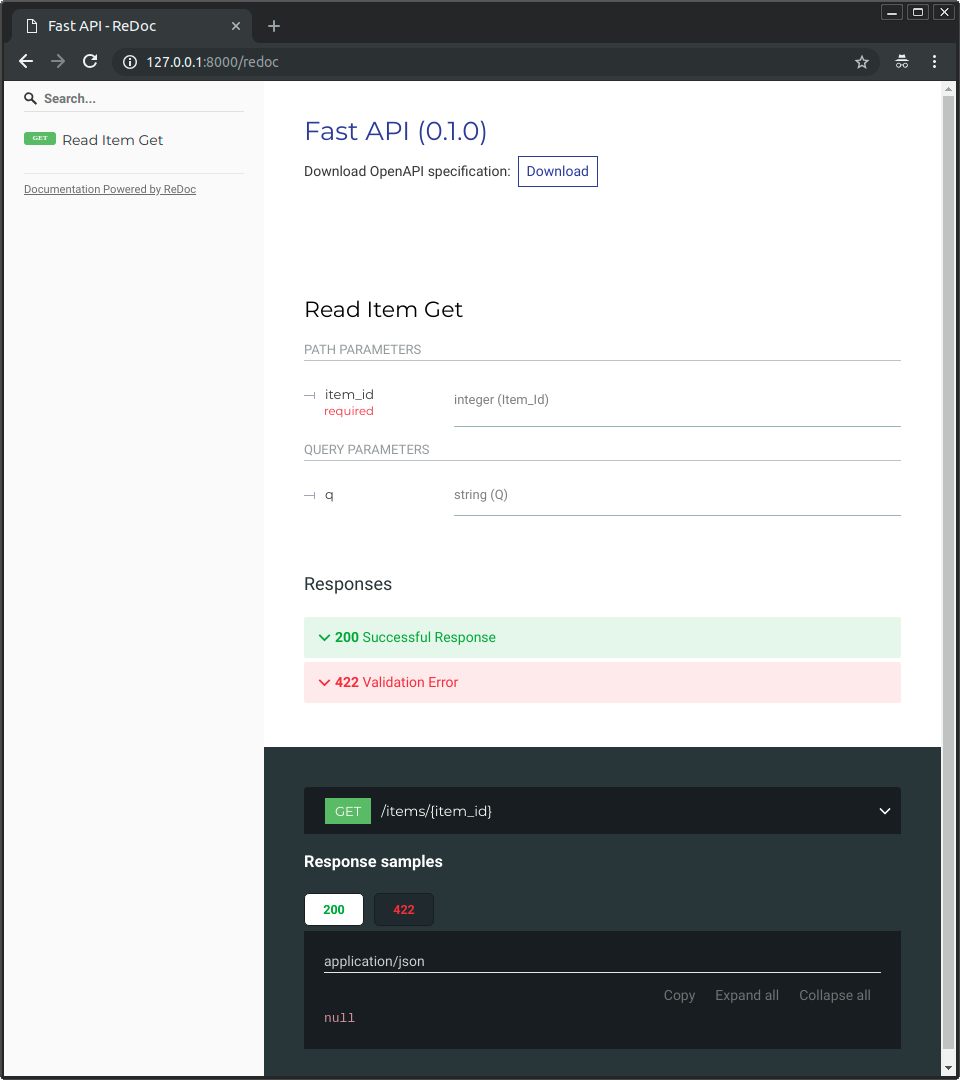 |
|||
|
|||
## Example upgrade |
|||
|
|||
Now modify the file `main.py` to receive a body from a `PUT` request. |
|||
|
|||
Declare the body using standard Python types, thanks to Pydantic. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Union[bool, None] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
The server should reload automatically (because you added `--reload` to the `uvicorn` command above). |
|||
|
|||
### Interactive API docs upgrade |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
* The interactive API documentation will be automatically updated, including the new body: |
|||
|
|||
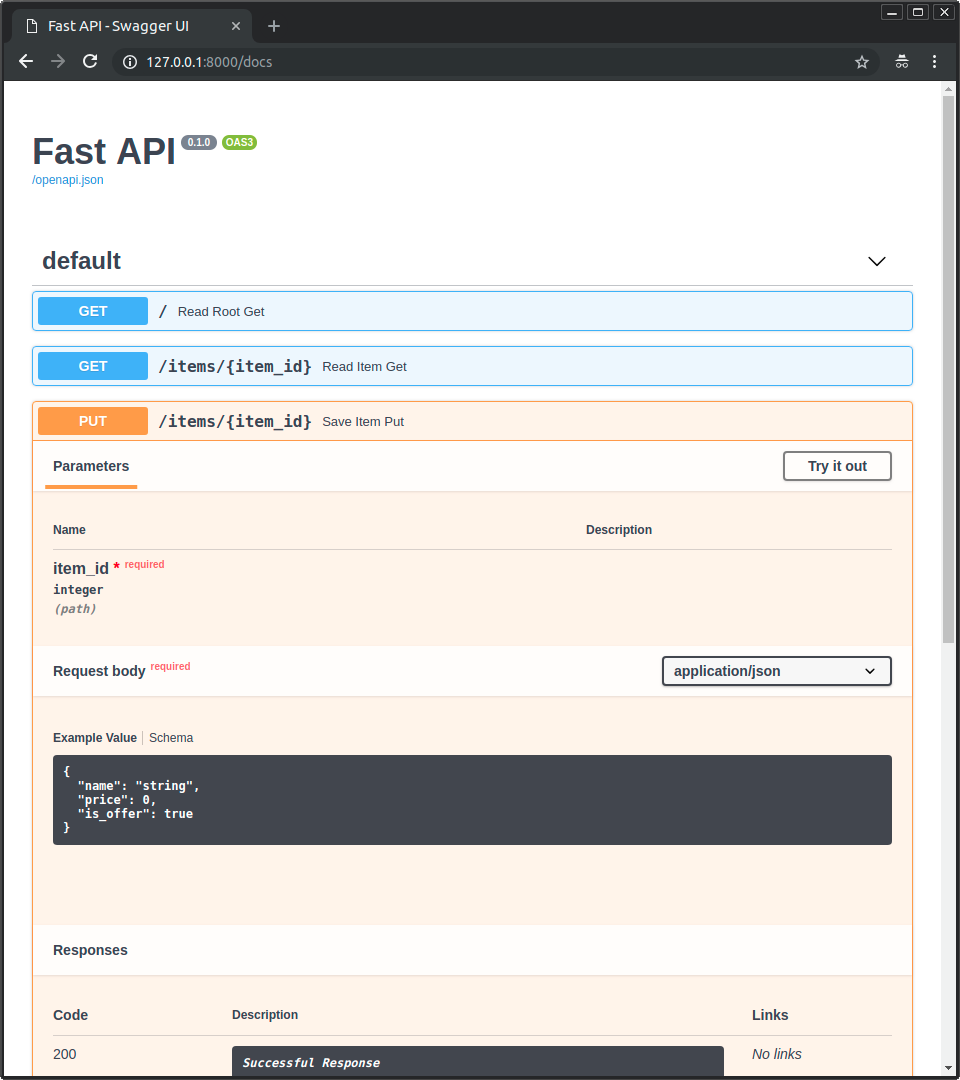 |
|||
|
|||
* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API: |
|||
|
|||
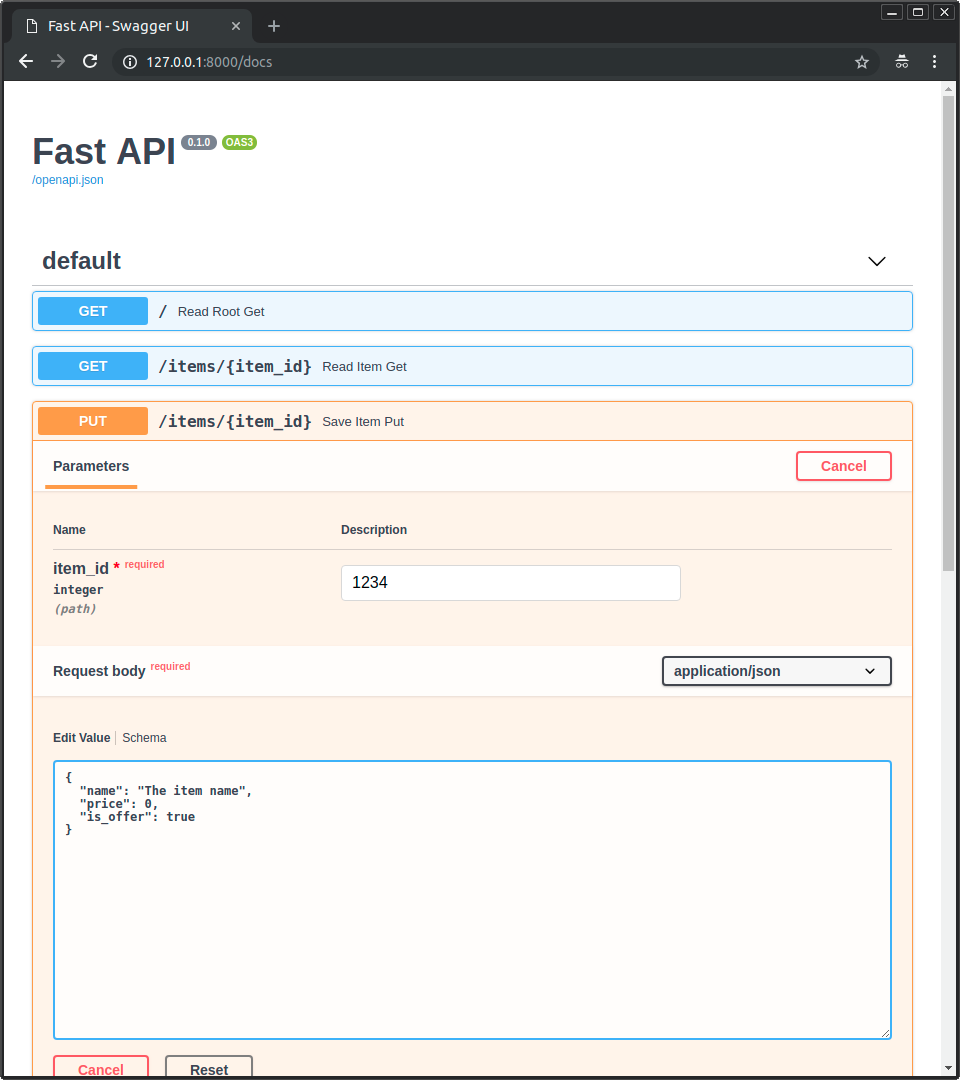 |
|||
|
|||
* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen: |
|||
|
|||
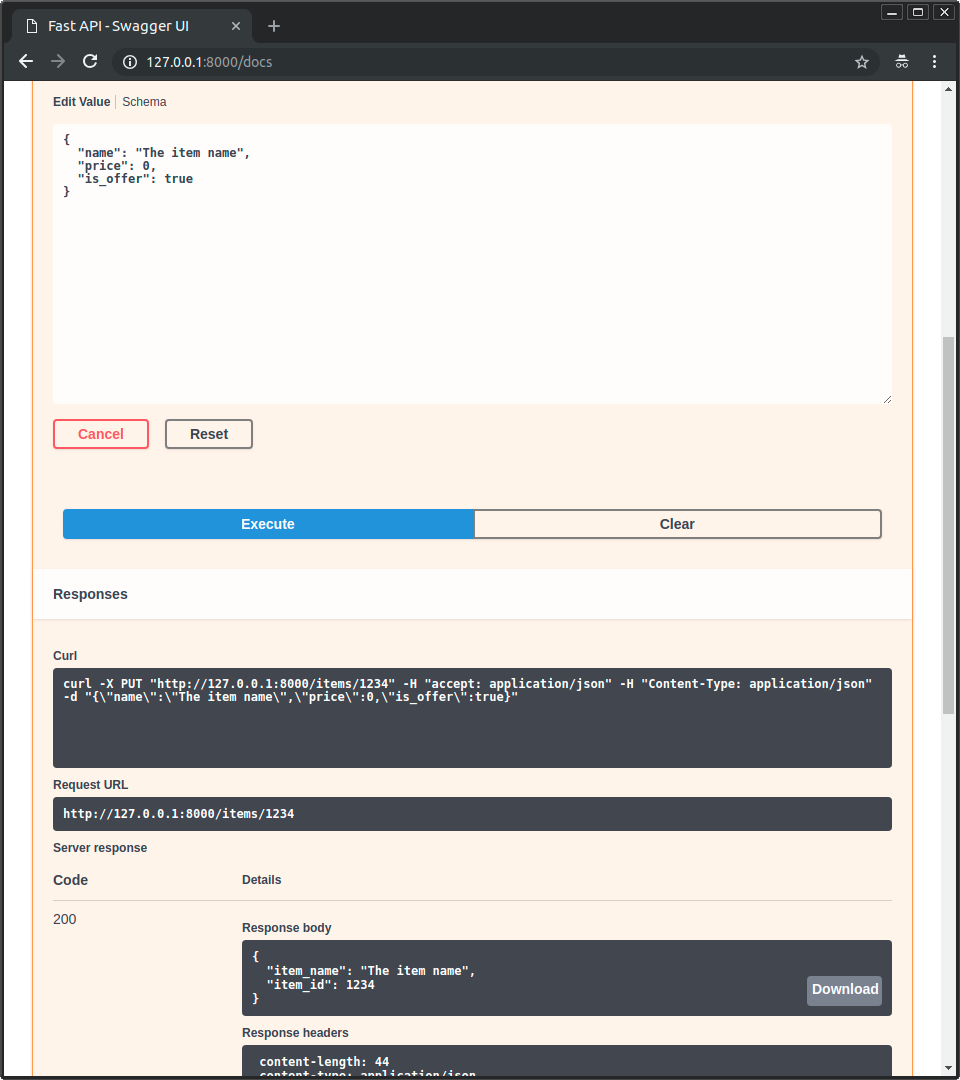 |
|||
|
|||
### Alternative API docs upgrade |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
* The alternative documentation will also reflect the new query parameter and body: |
|||
|
|||
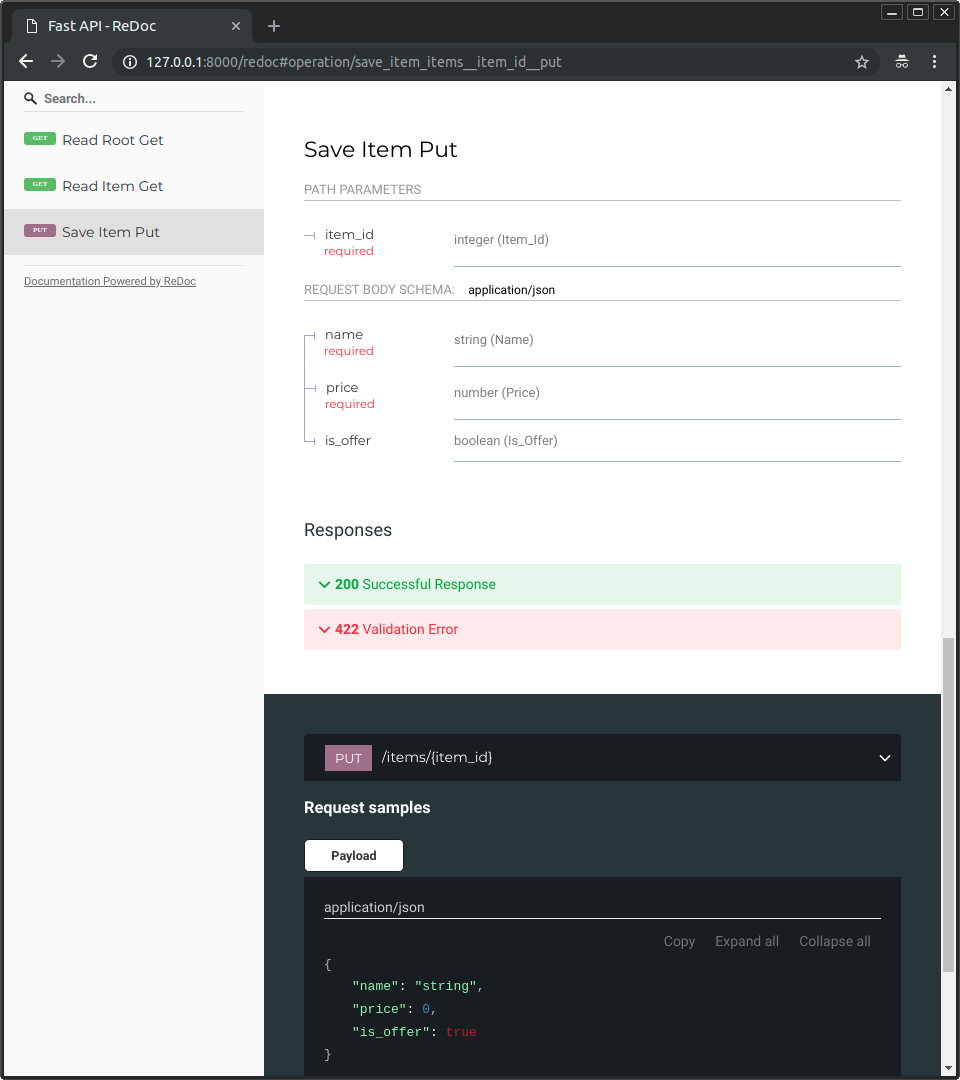 |
|||
|
|||
### Recap |
|||
|
|||
In summary, you declare **once** the types of parameters, body, etc. as function parameters. |
|||
|
|||
You do that with standard modern Python types. |
|||
|
|||
You don't have to learn a new syntax, the methods or classes of a specific library, etc. |
|||
|
|||
Just standard **Python 3.7+**. |
|||
|
|||
For example, for an `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
or for a more complex `Item` model: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...and with that single declaration you get: |
|||
|
|||
* Editor support, including: |
|||
* Completion. |
|||
* Type checks. |
|||
* Validation of data: |
|||
* Automatic and clear errors when the data is invalid. |
|||
* Validation even for deeply nested JSON objects. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of input data: coming from the network to Python data and types. Reading from: |
|||
* JSON. |
|||
* Path parameters. |
|||
* Query parameters. |
|||
* Cookies. |
|||
* Headers. |
|||
* Forms. |
|||
* Files. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of output data: converting from Python data and types to network data (as JSON): |
|||
* Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc). |
|||
* `datetime` objects. |
|||
* `UUID` objects. |
|||
* Database models. |
|||
* ...and many more. |
|||
* Automatic interactive API documentation, including 2 alternative user interfaces: |
|||
* Swagger UI. |
|||
* ReDoc. |
|||
|
|||
--- |
|||
|
|||
Coming back to the previous code example, **FastAPI** will: |
|||
|
|||
* Validate that there is an `item_id` in the path for `GET` and `PUT` requests. |
|||
* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests. |
|||
* If it is not, the client will see a useful, clear error. |
|||
* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests. |
|||
* As the `q` parameter is declared with `= None`, it is optional. |
|||
* Without the `None` it would be required (as is the body in the case with `PUT`). |
|||
* For `PUT` requests to `/items/{item_id}`, Read the body as JSON: |
|||
* Check that it has a required attribute `name` that should be a `str`. |
|||
* Check that it has a required attribute `price` that has to be a `float`. |
|||
* Check that it has an optional attribute `is_offer`, that should be a `bool`, if present. |
|||
* All this would also work for deeply nested JSON objects. |
|||
* Convert from and to JSON automatically. |
|||
* Document everything with OpenAPI, that can be used by: |
|||
* Interactive documentation systems. |
|||
* Automatic client code generation systems, for many languages. |
|||
* Provide 2 interactive documentation web interfaces directly. |
|||
|
|||
--- |
|||
|
|||
We just scratched the surface, but you already get the idea of how it all works. |
|||
|
|||
Try changing the line with: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
...from: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
...to: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
...and see how your editor will auto-complete the attributes and know their types: |
|||
|
|||
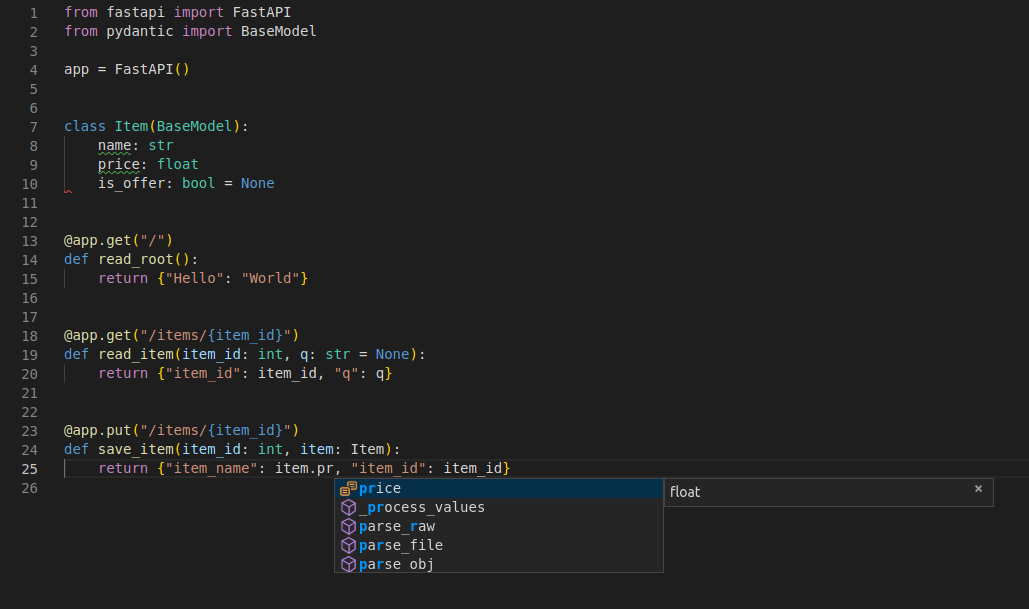 |
|||
|
|||
For a more complete example including more features, see the <a href="https://fastapi.tiangolo.com/tutorial/">Tutorial - User Guide</a>. |
|||
|
|||
**Spoiler alert**: the tutorial - user guide includes: |
|||
|
|||
* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**. |
|||
* How to set **validation constraints** as `maximum_length` or `regex`. |
|||
* A very powerful and easy to use **<abbr title="also known as components, resources, providers, services, injectables">Dependency Injection</abbr>** system. |
|||
* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth. |
|||
* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic). |
|||
* **GraphQL** integration with <a href="https://strawberry.rocks" class="external-link" target="_blank">Strawberry</a> and other libraries. |
|||
* Many extra features (thanks to Starlette) as: |
|||
* **WebSockets** |
|||
* extremely easy tests based on HTTPX and `pytest` |
|||
* **CORS** |
|||
* **Cookie Sessions** |
|||
* ...and more. |
|||
|
|||
## Performance |
|||
|
|||
Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">one of the fastest Python frameworks available</a>, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*) |
|||
|
|||
To understand more about it, see the section <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">Benchmarks</a>. |
|||
|
|||
## Optional Dependencies |
|||
|
|||
Used by Pydantic: |
|||
|
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - for faster JSON <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>. |
|||
* <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - for email validation. |
|||
|
|||
Used by Starlette: |
|||
|
|||
* <a href="https://www.python-httpx.org" target="_blank"><code>httpx</code></a> - Required if you want to use the `TestClient`. |
|||
* <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - Required if you want to use the default template configuration. |
|||
* <a href="https://andrew-d.github.io/python-multipart/" target="_blank"><code>python-multipart</code></a> - Required if you want to support form <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>, with `request.form()`. |
|||
* <a href="https://pythonhosted.org/itsdangerous/" target="_blank"><code>itsdangerous</code></a> - Required for `SessionMiddleware` support. |
|||
* <a href="https://pyyaml.org/wiki/PyYAMLDocumentation" target="_blank"><code>pyyaml</code></a> - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI). |
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - Required if you want to use `UJSONResponse`. |
|||
|
|||
Used by FastAPI / Starlette: |
|||
|
|||
* <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - for the server that loads and serves your application. |
|||
* <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - Required if you want to use `ORJSONResponse`. |
|||
|
|||
You can install all of these with `pip install "fastapi[all]"`. |
|||
|
|||
## License |
|||
|
|||
This project is licensed under the terms of the MIT license. |
@ -1,154 +0,0 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/cs/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: cs |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
@ -1,464 +0,0 @@ |
|||
|
|||
{!../../../docs/missing-translation.md!} |
|||
|
|||
|
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>FastAPI framework, high performance, easy to learn, fast to code, ready for production</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/tiangolo/fastapi/actions?query=workflow%3ATest" target="_blank"> |
|||
<img src="https://github.com/tiangolo/fastapi/workflows/Test/badge.svg" alt="Test"> |
|||
</a> |
|||
<a href="https://codecov.io/gh/tiangolo/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/codecov/c/github/tiangolo/fastapi?color=%2334D058" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**Documentation**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**Source Code**: <a href="https://github.com/tiangolo/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
|
|||
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. |
|||
|
|||
The key features are: |
|||
|
|||
* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance). |
|||
|
|||
* **Fast to code**: Increase the speed to develop features by about 200% to 300%. * |
|||
* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. * |
|||
* **Intuitive**: Great editor support. <abbr title="also known as auto-complete, autocompletion, IntelliSense">Completion</abbr> everywhere. Less time debugging. |
|||
* **Easy**: Designed to be easy to use and learn. Less time reading docs. |
|||
* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs. |
|||
* **Robust**: Get production-ready code. With automatic interactive documentation. |
|||
* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (previously known as Swagger) and <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>* estimation based on tests on an internal development team, building production applications.</small> |
|||
|
|||
## Sponsors |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor -%} |
|||
{%- for sponsor in sponsors.silver -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">Other sponsors</a> |
|||
|
|||
## Opinions |
|||
|
|||
"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/tiangolo/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_I’m over the moon excited about **FastAPI**. It’s so fun!_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast host</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_" |
|||
|
|||
"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> founders - <a href="https://spacy.io" target="_blank">spaCy</a> creators</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, the FastAPI of CLIs |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
If you are building a <abbr title="Command Line Interface">CLI</abbr> app to be used in the terminal instead of a web API, check out <a href="https://typer.tiangolo.com/" class="external-link" target="_blank">**Typer**</a>. |
|||
|
|||
**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀 |
|||
|
|||
## Requirements |
|||
|
|||
Python 3.7+ |
|||
|
|||
FastAPI stands on the shoulders of giants: |
|||
|
|||
* <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> for the web parts. |
|||
* <a href="https://pydantic-docs.helpmanual.io/" class="external-link" target="_blank">Pydantic</a> for the data parts. |
|||
|
|||
## Installation |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install fastapi |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
You will also need an ASGI server, for production such as <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a> or <a href="https://gitlab.com/pgjones/hypercorn" class="external-link" target="_blank">Hypercorn</a>. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "uvicorn[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Example |
|||
|
|||
### Create it |
|||
|
|||
* Create a file `main.py` with: |
|||
|
|||
```Python |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>Or use <code>async def</code>...</summary> |
|||
|
|||
If your code uses `async` / `await`, use `async def`: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**Note**: |
|||
|
|||
If you don't know, check the _"In a hurry?"_ section about <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` and `await` in the docs</a>. |
|||
|
|||
</details> |
|||
|
|||
### Run it |
|||
|
|||
Run the server with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [28720] |
|||
INFO: Started server process [28722] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>About the command <code>uvicorn main:app --reload</code>...</summary> |
|||
|
|||
The command `uvicorn main:app` refers to: |
|||
|
|||
* `main`: the file `main.py` (the Python "module"). |
|||
* `app`: the object created inside of `main.py` with the line `app = FastAPI()`. |
|||
* `--reload`: make the server restart after code changes. Only do this for development. |
|||
|
|||
</details> |
|||
|
|||
### Check it |
|||
|
|||
Open your browser at <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a>. |
|||
|
|||
You will see the JSON response as: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
You already created an API that: |
|||
|
|||
* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`. |
|||
* Both _paths_ take `GET` <em>operations</em> (also known as HTTP _methods_). |
|||
* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`. |
|||
* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`. |
|||
|
|||
### Interactive API docs |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
You will see the automatic interactive API documentation (provided by <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>): |
|||
|
|||
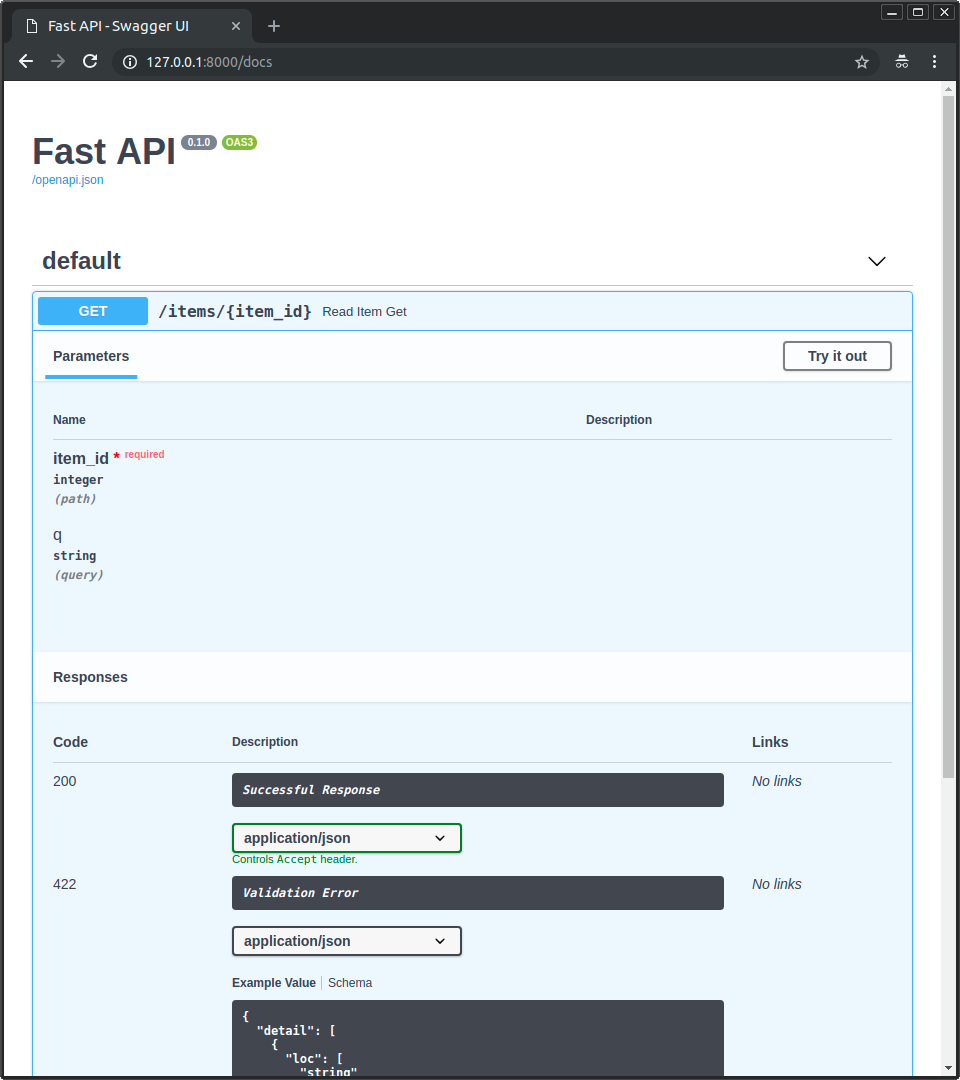 |
|||
|
|||
### Alternative API docs |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
You will see the alternative automatic documentation (provided by <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a>): |
|||
|
|||
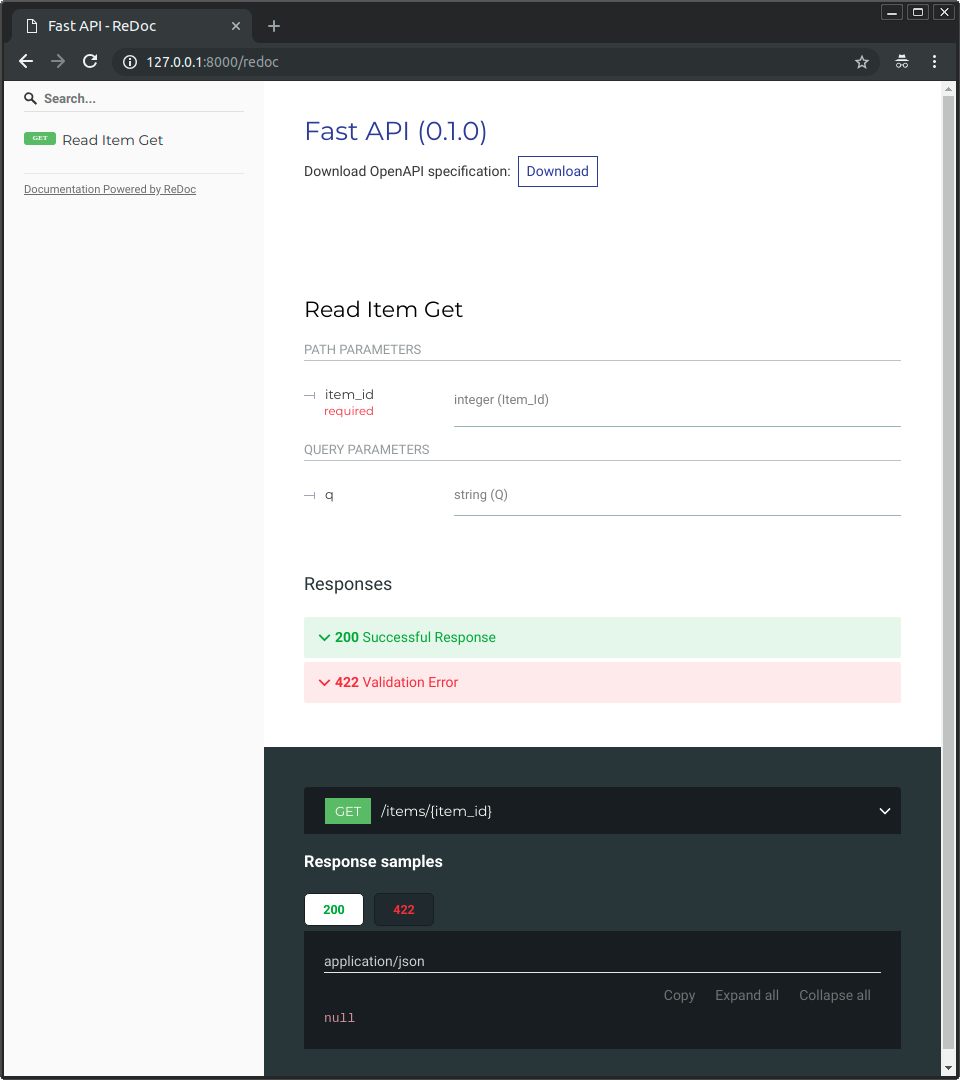 |
|||
|
|||
## Example upgrade |
|||
|
|||
Now modify the file `main.py` to receive a body from a `PUT` request. |
|||
|
|||
Declare the body using standard Python types, thanks to Pydantic. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Union[bool, None] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
The server should reload automatically (because you added `--reload` to the `uvicorn` command above). |
|||
|
|||
### Interactive API docs upgrade |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
* The interactive API documentation will be automatically updated, including the new body: |
|||
|
|||
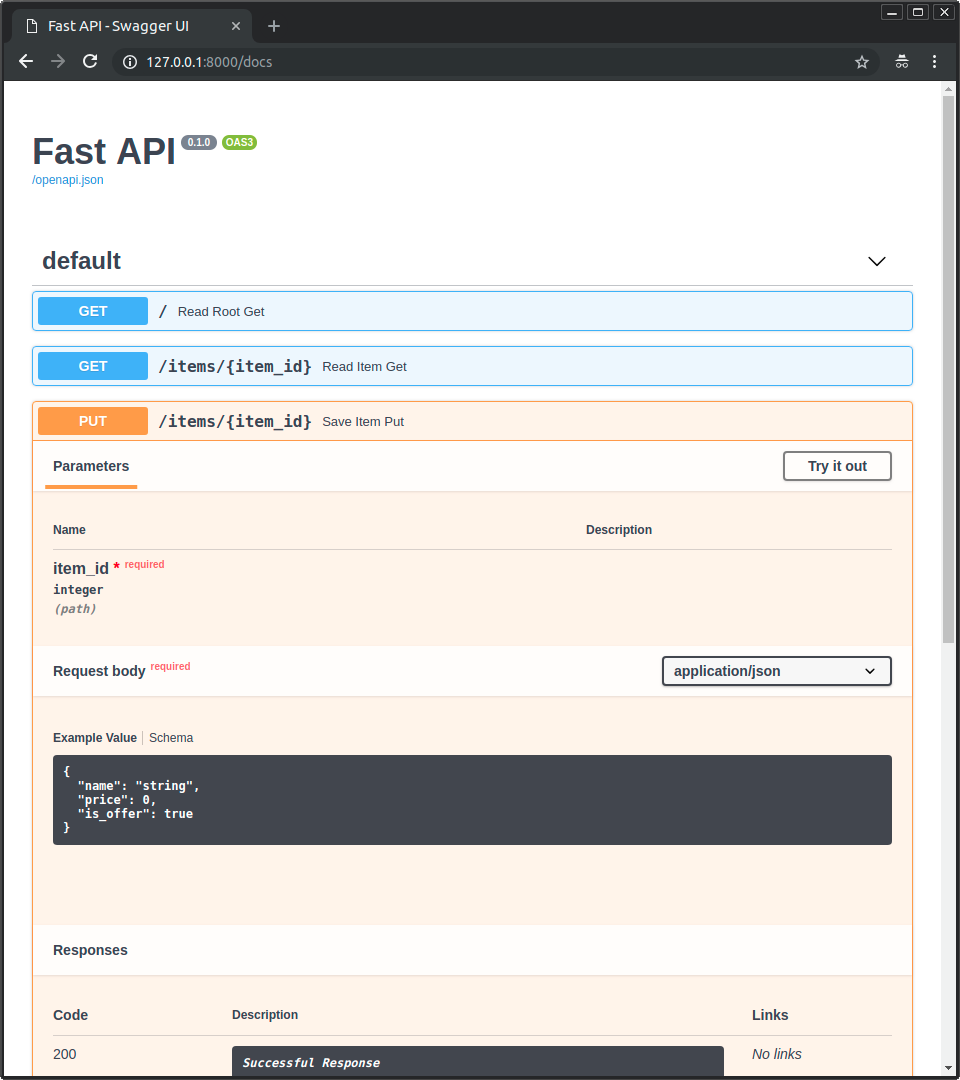 |
|||
|
|||
* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API: |
|||
|
|||
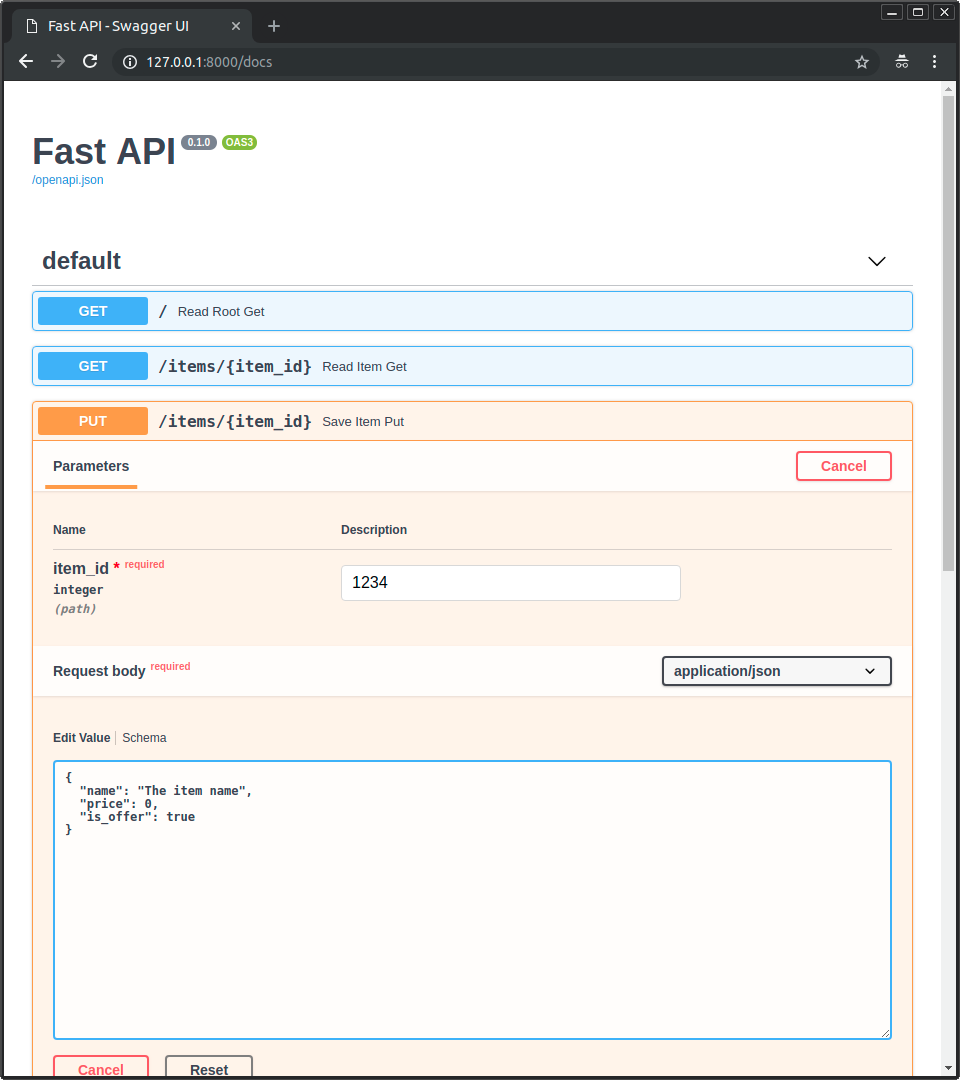 |
|||
|
|||
* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen: |
|||
|
|||
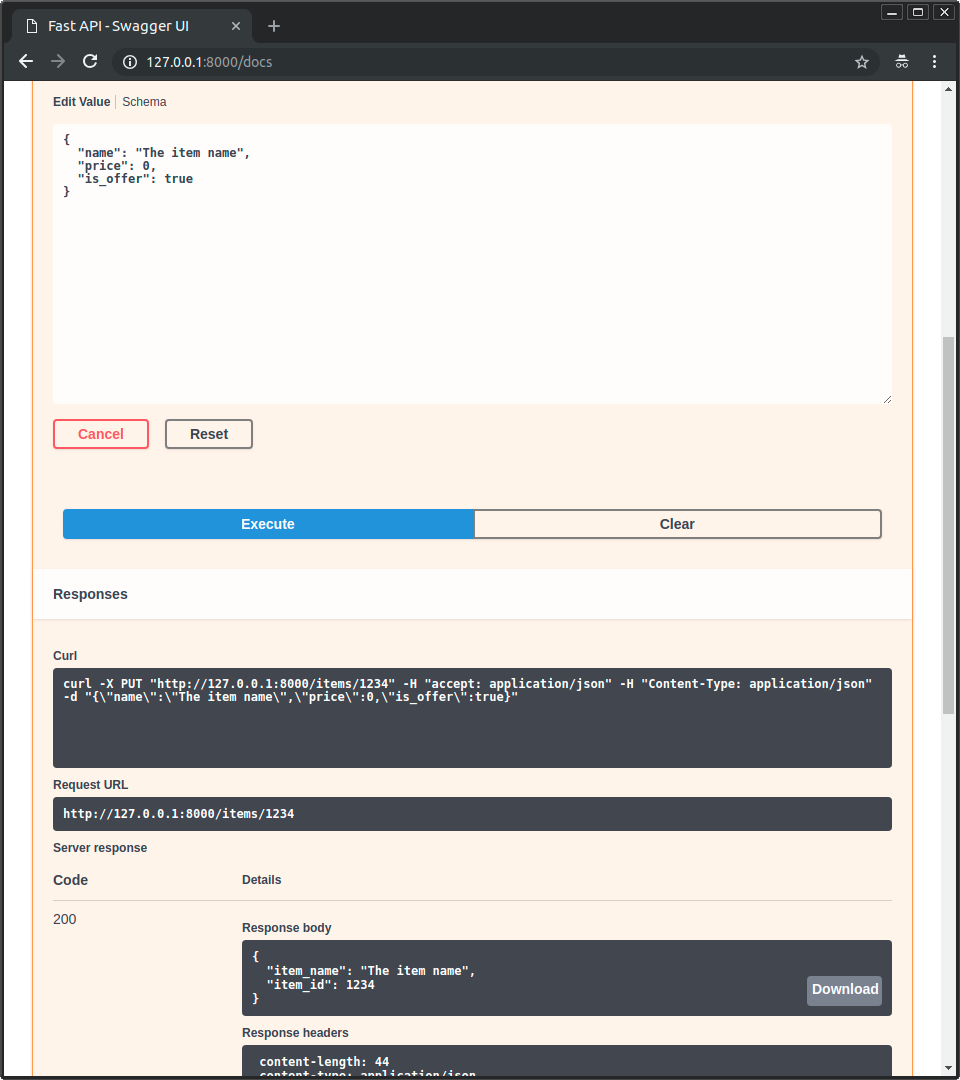 |
|||
|
|||
### Alternative API docs upgrade |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
* The alternative documentation will also reflect the new query parameter and body: |
|||
|
|||
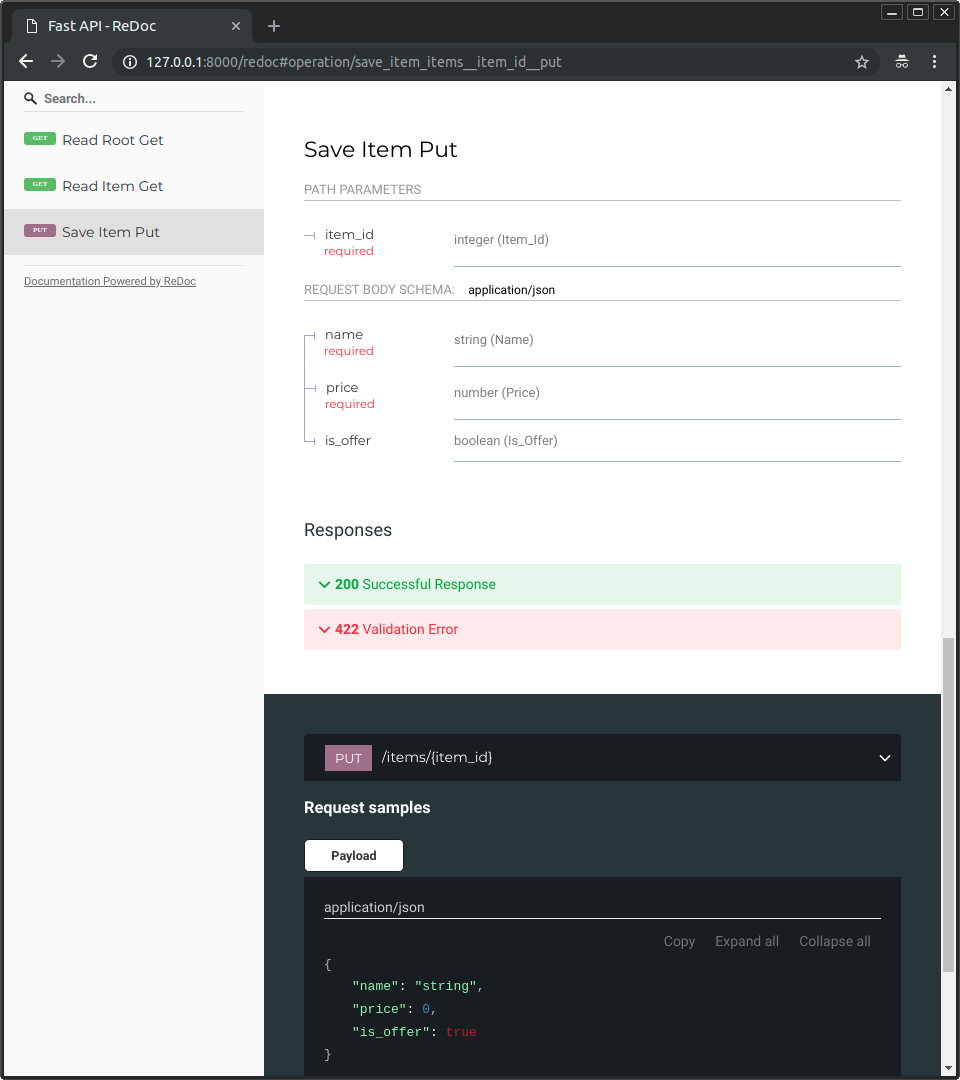 |
|||
|
|||
### Recap |
|||
|
|||
In summary, you declare **once** the types of parameters, body, etc. as function parameters. |
|||
|
|||
You do that with standard modern Python types. |
|||
|
|||
You don't have to learn a new syntax, the methods or classes of a specific library, etc. |
|||
|
|||
Just standard **Python 3.6+**. |
|||
|
|||
For example, for an `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
or for a more complex `Item` model: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...and with that single declaration you get: |
|||
|
|||
* Editor support, including: |
|||
* Completion. |
|||
* Type checks. |
|||
* Validation of data: |
|||
* Automatic and clear errors when the data is invalid. |
|||
* Validation even for deeply nested JSON objects. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of input data: coming from the network to Python data and types. Reading from: |
|||
* JSON. |
|||
* Path parameters. |
|||
* Query parameters. |
|||
* Cookies. |
|||
* Headers. |
|||
* Forms. |
|||
* Files. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of output data: converting from Python data and types to network data (as JSON): |
|||
* Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc). |
|||
* `datetime` objects. |
|||
* `UUID` objects. |
|||
* Database models. |
|||
* ...and many more. |
|||
* Automatic interactive API documentation, including 2 alternative user interfaces: |
|||
* Swagger UI. |
|||
* ReDoc. |
|||
|
|||
--- |
|||
|
|||
Coming back to the previous code example, **FastAPI** will: |
|||
|
|||
* Validate that there is an `item_id` in the path for `GET` and `PUT` requests. |
|||
* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests. |
|||
* If it is not, the client will see a useful, clear error. |
|||
* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests. |
|||
* As the `q` parameter is declared with `= None`, it is optional. |
|||
* Without the `None` it would be required (as is the body in the case with `PUT`). |
|||
* For `PUT` requests to `/items/{item_id}`, Read the body as JSON: |
|||
* Check that it has a required attribute `name` that should be a `str`. |
|||
* Check that it has a required attribute `price` that has to be a `float`. |
|||
* Check that it has an optional attribute `is_offer`, that should be a `bool`, if present. |
|||
* All this would also work for deeply nested JSON objects. |
|||
* Convert from and to JSON automatically. |
|||
* Document everything with OpenAPI, that can be used by: |
|||
* Interactive documentation systems. |
|||
* Automatic client code generation systems, for many languages. |
|||
* Provide 2 interactive documentation web interfaces directly. |
|||
|
|||
--- |
|||
|
|||
We just scratched the surface, but you already get the idea of how it all works. |
|||
|
|||
Try changing the line with: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
...from: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
...to: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
...and see how your editor will auto-complete the attributes and know their types: |
|||
|
|||
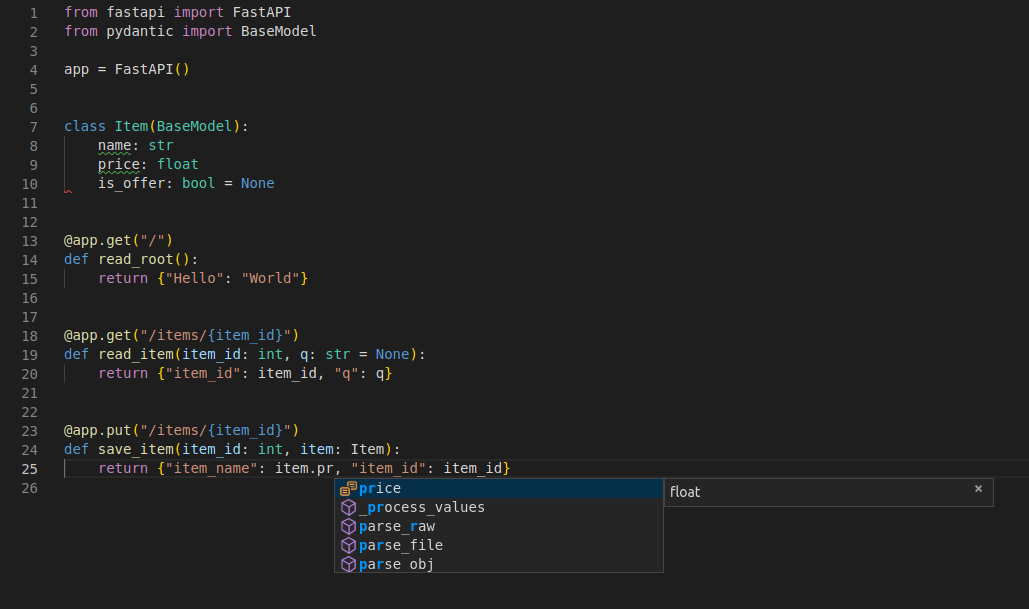 |
|||
|
|||
For a more complete example including more features, see the <a href="https://fastapi.tiangolo.com/tutorial/">Tutorial - User Guide</a>. |
|||
|
|||
**Spoiler alert**: the tutorial - user guide includes: |
|||
|
|||
* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**. |
|||
* How to set **validation constraints** as `maximum_length` or `regex`. |
|||
* A very powerful and easy to use **<abbr title="also known as components, resources, providers, services, injectables">Dependency Injection</abbr>** system. |
|||
* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth. |
|||
* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic). |
|||
* Many extra features (thanks to Starlette) as: |
|||
* **WebSockets** |
|||
* extremely easy tests based on `requests` and `pytest` |
|||
* **CORS** |
|||
* **Cookie Sessions** |
|||
* ...and more. |
|||
|
|||
## Performance |
|||
|
|||
Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">one of the fastest Python frameworks available</a>, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*) |
|||
|
|||
To understand more about it, see the section <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">Benchmarks</a>. |
|||
|
|||
## Optional Dependencies |
|||
|
|||
Used by Pydantic: |
|||
|
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - for faster JSON <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>. |
|||
* <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - for email validation. |
|||
|
|||
Used by Starlette: |
|||
|
|||
* <a href="https://www.python-httpx.org" target="_blank"><code>httpx</code></a> - Required if you want to use the `TestClient`. |
|||
* <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - Required if you want to use the default template configuration. |
|||
* <a href="https://andrew-d.github.io/python-multipart/" target="_blank"><code>python-multipart</code></a> - Required if you want to support form <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>, with `request.form()`. |
|||
* <a href="https://pythonhosted.org/itsdangerous/" target="_blank"><code>itsdangerous</code></a> - Required for `SessionMiddleware` support. |
|||
* <a href="https://pyyaml.org/wiki/PyYAMLDocumentation" target="_blank"><code>pyyaml</code></a> - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI). |
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - Required if you want to use `UJSONResponse`. |
|||
|
|||
Used by FastAPI / Starlette: |
|||
|
|||
* <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - for the server that loads and serves your application. |
|||
* <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - Required if you want to use `ORJSONResponse`. |
|||
|
|||
You can install all of these with `pip install fastapi[all]`. |
|||
|
|||
## License |
|||
|
|||
This project is licensed under the terms of the MIT license. |
@ -1,161 +1 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/de/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: de |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
- features.md |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
|||
INHERIT: ../en/mkdocs.yml |
|||
|
@ -1,264 +1 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/em/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: en |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
- features.md |
|||
- fastapi-people.md |
|||
- python-types.md |
|||
- 🔰 - 👩💻 🦮: |
|||
- tutorial/index.md |
|||
- tutorial/first-steps.md |
|||
- tutorial/path-params.md |
|||
- tutorial/query-params.md |
|||
- tutorial/body.md |
|||
- tutorial/query-params-str-validations.md |
|||
- tutorial/path-params-numeric-validations.md |
|||
- tutorial/body-multiple-params.md |
|||
- tutorial/body-fields.md |
|||
- tutorial/body-nested-models.md |
|||
- tutorial/schema-extra-example.md |
|||
- tutorial/extra-data-types.md |
|||
- tutorial/cookie-params.md |
|||
- tutorial/header-params.md |
|||
- tutorial/response-model.md |
|||
- tutorial/extra-models.md |
|||
- tutorial/response-status-code.md |
|||
- tutorial/request-forms.md |
|||
- tutorial/request-files.md |
|||
- tutorial/request-forms-and-files.md |
|||
- tutorial/handling-errors.md |
|||
- tutorial/path-operation-configuration.md |
|||
- tutorial/encoder.md |
|||
- tutorial/body-updates.md |
|||
- 🔗: |
|||
- tutorial/dependencies/index.md |
|||
- tutorial/dependencies/classes-as-dependencies.md |
|||
- tutorial/dependencies/sub-dependencies.md |
|||
- tutorial/dependencies/dependencies-in-path-operation-decorators.md |
|||
- tutorial/dependencies/global-dependencies.md |
|||
- tutorial/dependencies/dependencies-with-yield.md |
|||
- 💂♂: |
|||
- tutorial/security/index.md |
|||
- tutorial/security/first-steps.md |
|||
- tutorial/security/get-current-user.md |
|||
- tutorial/security/simple-oauth2.md |
|||
- tutorial/security/oauth2-jwt.md |
|||
- tutorial/middleware.md |
|||
- tutorial/cors.md |
|||
- tutorial/sql-databases.md |
|||
- tutorial/bigger-applications.md |
|||
- tutorial/background-tasks.md |
|||
- tutorial/metadata.md |
|||
- tutorial/static-files.md |
|||
- tutorial/testing.md |
|||
- tutorial/debugging.md |
|||
- 🏧 👩💻 🦮: |
|||
- advanced/index.md |
|||
- advanced/path-operation-advanced-configuration.md |
|||
- advanced/additional-status-codes.md |
|||
- advanced/response-directly.md |
|||
- advanced/custom-response.md |
|||
- advanced/additional-responses.md |
|||
- advanced/response-cookies.md |
|||
- advanced/response-headers.md |
|||
- advanced/response-change-status-code.md |
|||
- advanced/advanced-dependencies.md |
|||
- 🏧 💂♂: |
|||
- advanced/security/index.md |
|||
- advanced/security/oauth2-scopes.md |
|||
- advanced/security/http-basic-auth.md |
|||
- advanced/using-request-directly.md |
|||
- advanced/dataclasses.md |
|||
- advanced/middleware.md |
|||
- advanced/sql-databases-peewee.md |
|||
- advanced/async-sql-databases.md |
|||
- advanced/nosql-databases.md |
|||
- advanced/sub-applications.md |
|||
- advanced/behind-a-proxy.md |
|||
- advanced/templates.md |
|||
- advanced/graphql.md |
|||
- advanced/websockets.md |
|||
- advanced/events.md |
|||
- advanced/custom-request-and-route.md |
|||
- advanced/testing-websockets.md |
|||
- advanced/testing-events.md |
|||
- advanced/testing-dependencies.md |
|||
- advanced/testing-database.md |
|||
- advanced/async-tests.md |
|||
- advanced/settings.md |
|||
- advanced/conditional-openapi.md |
|||
- advanced/extending-openapi.md |
|||
- advanced/openapi-callbacks.md |
|||
- advanced/wsgi.md |
|||
- advanced/generate-clients.md |
|||
- async.md |
|||
- 🛠️: |
|||
- deployment/index.md |
|||
- deployment/versions.md |
|||
- deployment/https.md |
|||
- deployment/manually.md |
|||
- deployment/concepts.md |
|||
- deployment/deta.md |
|||
- deployment/server-workers.md |
|||
- deployment/docker.md |
|||
- project-generation.md |
|||
- alternatives.md |
|||
- history-design-future.md |
|||
- external-links.md |
|||
- benchmarks.md |
|||
- help-fastapi.md |
|||
- contributing.md |
|||
- release-notes.md |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
|||
INHERIT: ../en/mkdocs.yml |
|||
|
@ -0,0 +1,51 @@ |
|||
# OpenAPI Webhooks |
|||
|
|||
There are cases where you want to tell your API **users** that your app could call *their* app (sending a request) with some data, normally to **notify** of some type of **event**. |
|||
|
|||
This means that instead of the normal process of your users sending requests to your API, it's **your API** (or your app) that could **send requests to their system** (to their API, their app). |
|||
|
|||
This is normally called a **webhook**. |
|||
|
|||
## Webhooks steps |
|||
|
|||
The process normally is that **you define** in your code what is the message that you will send, the **body of the request**. |
|||
|
|||
You also define in some way at which **moments** your app will send those requests or events. |
|||
|
|||
And **your users** define in some way (for example in a web dashboard somewhere) the **URL** where your app should send those requests. |
|||
|
|||
All the **logic** about how to register the URLs for webhooks and the code to actually send those requests is up to you. You write it however you want to in **your own code**. |
|||
|
|||
## Documenting webhooks with **FastAPI** and OpenAPI |
|||
|
|||
With **FastAPI**, using OpenAPI, you can define the names of these webhooks, the types of HTTP operations that your app can send (e.g. `POST`, `PUT`, etc.) and the request **bodies** that your app would send. |
|||
|
|||
This can make it a lot easier for your users to **implement their APIs** to receive your **webhook** requests, they might even be able to autogenerate some of their own API code. |
|||
|
|||
!!! info |
|||
Webhooks are available in OpenAPI 3.1.0 and above, supported by FastAPI `0.99.0` and above. |
|||
|
|||
## An app with webhooks |
|||
|
|||
When you create a **FastAPI** application, there is a `webhooks` attribute that you can use to define *webhooks*, the same way you would define *path operations*, for example with `@app.webhooks.post()`. |
|||
|
|||
```Python hl_lines="9-13 36-53" |
|||
{!../../../docs_src/openapi_webhooks/tutorial001.py!} |
|||
``` |
|||
|
|||
The webhooks that you define will end up in the **OpenAPI** schema and the automatic **docs UI**. |
|||
|
|||
!!! info |
|||
The `app.webhooks` object is actually just an `APIRouter`, the same type you would use when structuring your app with multiple files. |
|||
|
|||
Notice that with webhooks you are actually not declaring a *path* (like `/items/`), the text you pass there is just an **identifier** of the webhook (the name of the event), for example in `@app.webhooks.post("new-subscription")`, the webhook name is `new-subscription`. |
|||
|
|||
This is because it is expected that **your users** would define the actual **URL path** where they want to receive the webhook request in some other way (e.g. a web dashboard). |
|||
|
|||
### Check the docs |
|||
|
|||
Now you can start your app with Uvicorn and go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
You will see your docs have the normal *path operations* and now also some **webhooks**: |
|||
|
|||
<img src="/img/tutorial/openapi-webhooks/image01.png"> |
After Width: | Height: | Size: 10 KiB |
After Width: | Height: | Size: 6.2 KiB |
After Width: | Height: | Size: 5.6 KiB |
Before Width: | Height: | Size: 88 KiB After Width: | Height: | Size: 84 KiB |
After Width: | Height: | Size: 85 KiB |
@ -1,5 +1,5 @@ |
|||
# FastAPI and friends newsletter |
|||
|
|||
<iframe class="mj-w-res-iframe" frameborder="0" scrolling="no" marginheight="0" marginwidth="0" src="https://app.mailjet.com/widget/iframe/6gQ4/GDo" width="100%"></iframe> |
|||
<iframe data-w-type="embedded" frameborder="0" scrolling="no" marginheight="0" marginwidth="0" src="https://xr4n4.mjt.lu/wgt/xr4n4/hj5/form?c=40a44fa4" width="100%" style="height: 0;"></iframe> |
|||
|
|||
<script type="text/javascript" src="https://app.mailjet.com/statics/js/iframeResizer.min.js"></script> |
|||
<script type="text/javascript" src="https://app.mailjet.com/pas-nc-embedded-v1.js"></script> |
|||
|
@ -0,0 +1,228 @@ |
|||
# Copyright (c) 2016-2023 Martin Donath <martin.donath@squidfunk.com> |
|||
|
|||
# Permission is hereby granted, free of charge, to any person obtaining a copy |
|||
# of this software and associated documentation files (the "Software"), to |
|||
# deal in the Software without restriction, including without limitation the |
|||
# rights to use, copy, modify, merge, publish, distribute, sublicense, and/or |
|||
# sell copies of the Software, and to permit persons to whom the Software is |
|||
# furnished to do so, subject to the following conditions: |
|||
|
|||
# The above copyright notice and this permission notice shall be included in |
|||
# all copies or substantial portions of the Software. |
|||
|
|||
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
|||
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
|||
# FITNESS FOR A PARTICULAR PURPOSE AND NON-INFRINGEMENT. IN NO EVENT SHALL THE |
|||
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
|||
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING |
|||
# FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS |
|||
# IN THE SOFTWARE. |
|||
|
|||
# ----------------------------------------------------------------------------- |
|||
# Configuration |
|||
# ----------------------------------------------------------------------------- |
|||
|
|||
# The same default card with a a configurable logo |
|||
|
|||
# Definitions |
|||
definitions: |
|||
|
|||
# Background image |
|||
- &background_image >- |
|||
{{ layout.background_image or "" }} |
|||
|
|||
# Background color (default: indigo) |
|||
- &background_color >- |
|||
{%- if layout.background_color -%} |
|||
{{ layout.background_color }} |
|||
{%- else -%} |
|||
{%- set palette = config.theme.palette or {} -%} |
|||
{%- if not palette is mapping -%} |
|||
{%- set palette = palette | first -%} |
|||
{%- endif -%} |
|||
{%- set primary = palette.get("primary", "indigo") -%} |
|||
{%- set primary = primary.replace(" ", "-") -%} |
|||
{{ { |
|||
"red": "#ef5552", |
|||
"pink": "#e92063", |
|||
"purple": "#ab47bd", |
|||
"deep-purple": "#7e56c2", |
|||
"indigo": "#4051b5", |
|||
"blue": "#2094f3", |
|||
"light-blue": "#02a6f2", |
|||
"cyan": "#00bdd6", |
|||
"teal": "#009485", |
|||
"green": "#4cae4f", |
|||
"light-green": "#8bc34b", |
|||
"lime": "#cbdc38", |
|||
"yellow": "#ffec3d", |
|||
"amber": "#ffc105", |
|||
"orange": "#ffa724", |
|||
"deep-orange": "#ff6e42", |
|||
"brown": "#795649", |
|||
"grey": "#757575", |
|||
"blue-grey": "#546d78", |
|||
"black": "#000000", |
|||
"white": "#ffffff" |
|||
}[primary] or "#4051b5" }} |
|||
{%- endif -%} |
|||
|
|||
# Text color (default: white) |
|||
- &color >- |
|||
{%- if layout.color -%} |
|||
{{ layout.color }} |
|||
{%- else -%} |
|||
{%- set palette = config.theme.palette or {} -%} |
|||
{%- if not palette is mapping -%} |
|||
{%- set palette = palette | first -%} |
|||
{%- endif -%} |
|||
{%- set primary = palette.get("primary", "indigo") -%} |
|||
{%- set primary = primary.replace(" ", "-") -%} |
|||
{{ { |
|||
"red": "#ffffff", |
|||
"pink": "#ffffff", |
|||
"purple": "#ffffff", |
|||
"deep-purple": "#ffffff", |
|||
"indigo": "#ffffff", |
|||
"blue": "#ffffff", |
|||
"light-blue": "#ffffff", |
|||
"cyan": "#ffffff", |
|||
"teal": "#ffffff", |
|||
"green": "#ffffff", |
|||
"light-green": "#ffffff", |
|||
"lime": "#000000", |
|||
"yellow": "#000000", |
|||
"amber": "#000000", |
|||
"orange": "#000000", |
|||
"deep-orange": "#ffffff", |
|||
"brown": "#ffffff", |
|||
"grey": "#ffffff", |
|||
"blue-grey": "#ffffff", |
|||
"black": "#ffffff", |
|||
"white": "#000000" |
|||
}[primary] or "#ffffff" }} |
|||
{%- endif -%} |
|||
|
|||
# Font family (default: Roboto) |
|||
- &font_family >- |
|||
{%- if layout.font_family -%} |
|||
{{ layout.font_family }} |
|||
{%- elif config.theme.font != false -%} |
|||
{{ config.theme.font.get("text", "Roboto") }} |
|||
{%- else -%} |
|||
Roboto |
|||
{%- endif -%} |
|||
|
|||
# Site name |
|||
- &site_name >- |
|||
{{ config.site_name }} |
|||
|
|||
# Page title |
|||
- &page_title >- |
|||
{{ page.meta.get("title", page.title) }} |
|||
|
|||
# Page title with site name |
|||
- &page_title_with_site_name >- |
|||
{%- if not page.is_homepage -%} |
|||
{{ page.meta.get("title", page.title) }} - {{ config.site_name }} |
|||
{%- else -%} |
|||
{{ page.meta.get("title", page.title) }} |
|||
{%- endif -%} |
|||
|
|||
# Page description |
|||
- &page_description >- |
|||
{{ page.meta.get("description", config.site_description) or "" }} |
|||
|
|||
|
|||
# Start of custom modified logic |
|||
# Logo |
|||
- &logo >- |
|||
{%- if layout.logo -%} |
|||
{{ layout.logo }} |
|||
{%- elif config.theme.logo -%} |
|||
{{ config.docs_dir }}/{{ config.theme.logo }} |
|||
{%- endif -%} |
|||
# End of custom modified logic |
|||
|
|||
# Logo (icon) |
|||
- &logo_icon >- |
|||
{{ config.theme.icon.logo or "" }} |
|||
|
|||
# Meta tags |
|||
tags: |
|||
|
|||
# Open Graph |
|||
og:type: website |
|||
og:title: *page_title_with_site_name |
|||
og:description: *page_description |
|||
og:image: "{{ image.url }}" |
|||
og:image:type: "{{ image.type }}" |
|||
og:image:width: "{{ image.width }}" |
|||
og:image:height: "{{ image.height }}" |
|||
og:url: "{{ page.canonical_url }}" |
|||
|
|||
# Twitter |
|||
twitter:card: summary_large_image |
|||
twitter.title: *page_title_with_site_name |
|||
twitter:description: *page_description |
|||
twitter:image: "{{ image.url }}" |
|||
|
|||
# ----------------------------------------------------------------------------- |
|||
# Specification |
|||
# ----------------------------------------------------------------------------- |
|||
|
|||
# Card size and layers |
|||
size: { width: 1200, height: 630 } |
|||
layers: |
|||
|
|||
# Background |
|||
- background: |
|||
image: *background_image |
|||
color: *background_color |
|||
|
|||
# Logo |
|||
- size: { width: 144, height: 144 } |
|||
offset: { x: 992, y: 64 } |
|||
background: |
|||
image: *logo |
|||
icon: |
|||
value: *logo_icon |
|||
color: *color |
|||
|
|||
# Site name |
|||
- size: { width: 832, height: 42 } |
|||
offset: { x: 64, y: 64 } |
|||
typography: |
|||
content: *site_name |
|||
color: *color |
|||
font: |
|||
family: *font_family |
|||
style: Bold |
|||
|
|||
# Page title |
|||
- size: { width: 832, height: 310 } |
|||
offset: { x: 62, y: 160 } |
|||
typography: |
|||
content: *page_title |
|||
align: start |
|||
color: *color |
|||
line: |
|||
amount: 3 |
|||
height: 1.25 |
|||
font: |
|||
family: *font_family |
|||
style: Bold |
|||
|
|||
# Page description |
|||
- size: { width: 832, height: 64 } |
|||
offset: { x: 64, y: 512 } |
|||
typography: |
|||
content: *page_description |
|||
align: start |
|||
color: *color |
|||
line: |
|||
amount: 2 |
|||
height: 1.5 |
|||
font: |
|||
family: *font_family |
|||
style: Regular |
@ -0,0 +1,7 @@ |
|||
plugins: |
|||
social: |
|||
cards_layout_dir: ../en/layouts |
|||
cards_layout: custom |
|||
cards_layout_options: |
|||
logo: ../en/docs/img/icon-white.svg |
|||
typeset: |
@ -0,0 +1,6 @@ |
|||
# Define this here and not in the main mkdocs.yml file because that one is auto |
|||
# updated and written, and the script would remove the env var |
|||
INHERIT: !ENV [INSIDERS_FILE, '../en/mkdocs.no-insiders.yml'] |
|||
markdown_extensions: |
|||
pymdownx.highlight: |
|||
linenums: !ENV [LINENUMS, false] |
@ -1,170 +1 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/es/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: es |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
- features.md |
|||
- python-types.md |
|||
- Tutorial - Guía de Usuario: |
|||
- tutorial/index.md |
|||
- tutorial/first-steps.md |
|||
- tutorial/path-params.md |
|||
- tutorial/query-params.md |
|||
- Guía de Usuario Avanzada: |
|||
- advanced/index.md |
|||
- async.md |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
|||
INHERIT: ../en/mkdocs.yml |
|||
|
@ -0,0 +1,72 @@ |
|||
# زیر برنامه ها - اتصال |
|||
|
|||
اگر نیاز دارید که دو برنامه مستقل FastAPI، با OpenAPI مستقل و رابطهای کاربری اسناد خود داشته باشید، میتوانید یک برنامه |
|||
اصلی داشته باشید و یک (یا چند) زیر برنامه را به آن متصل کنید. |
|||
|
|||
## اتصال (mount) به یک برنامه **FastAPI** |
|||
|
|||
کلمه "Mounting" به معنای افزودن یک برنامه کاملاً مستقل در یک مسیر خاص است، که پس از آن مدیریت همه چیز در آن مسیر، با path operations (عملیات های مسیر) اعلام شده در آن زیر برنامه می باشد. |
|||
|
|||
### برنامه سطح بالا |
|||
|
|||
ابتدا برنامه اصلی سطح بالا، **FastAPI** و path operations آن را ایجاد کنید: |
|||
|
|||
|
|||
```Python hl_lines="3 6-8" |
|||
{!../../../docs_src/sub_applications/tutorial001.py!} |
|||
``` |
|||
|
|||
### زیر برنامه |
|||
|
|||
سپس، زیر برنامه خود و path operations آن را ایجاد کنید. |
|||
|
|||
این زیر برنامه فقط یکی دیگر از برنامه های استاندارد FastAPI است، اما این برنامه ای است که متصل می شود: |
|||
|
|||
```Python hl_lines="11 14-16" |
|||
{!../../../docs_src/sub_applications/tutorial001.py!} |
|||
``` |
|||
|
|||
### اتصال زیر برنامه |
|||
|
|||
در برنامه سطح بالا `app` اتصال زیر برنامه `subapi` در این نمونه `/subapi` در مسیر قرار میدهد و میشود: |
|||
|
|||
```Python hl_lines="11 19" |
|||
{!../../../docs_src/sub_applications/tutorial001.py!} |
|||
``` |
|||
|
|||
### اسناد API خودکار را بررسی کنید |
|||
|
|||
برنامه را با استفاده از ‘uvicorn‘ اجرا کنید، اگر فایل شما ‘main.py‘ نام دارد، دستور زیر را وارد کنید: |
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
<span style="color: green;">INFO</span>: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
صفحه مستندات را در آدرس <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a> باز کنید. |
|||
|
|||
اسناد API خودکار برنامه اصلی را مشاهده خواهید کرد که فقط شامل path operations خود می شود: |
|||
|
|||
<img src="/img/tutorial/sub-applications/image01.png"> |
|||
|
|||
و سپس اسناد زیر برنامه را در آدرس <a href="http://127.0.0.1:8000/subapi/docs" class="external-link" target="_blank">http://127.0.0.1:8000/subapi/docs</a>. باز کنید. |
|||
|
|||
اسناد API خودکار برای زیر برنامه را خواهید دید، که فقط شامل path operations خود می شود، همه در زیر مسیر `/subapi` قرار دارند: |
|||
|
|||
<img src="/img/tutorial/sub-applications/image02.png"> |
|||
|
|||
اگر سعی کنید با هر یک از این دو رابط کاربری تعامل داشته باشید، آنها به درستی کار می کنند، زیرا مرورگر می تواند با هر یک از برنامه ها یا زیر برنامه های خاص صحبت کند. |
|||
|
|||
### جرئیات فنی : `root_path` |
|||
|
|||
هنگامی که یک زیر برنامه را همانطور که در بالا توضیح داده شد متصل می کنید, FastAPI با استفاده از مکانیزمی از مشخصات ASGI به نام `root_path` ارتباط مسیر mount را برای زیر برنامه انجام می دهد. |
|||
|
|||
به این ترتیب، زیر برنامه می داند که از آن پیشوند مسیر برای رابط کاربری اسناد (docs UI) استفاده کند. |
|||
|
|||
و زیر برنامه ها نیز می تواند زیر برنامه های متصل شده خود را داشته باشد و همه چیز به درستی کار کند، زیرا FastAPI تمام این مسیرهای `root_path` را به طور خودکار مدیریت می کند. |
|||
|
|||
در بخش [پشت پراکسی](./behind-a-proxy.md){.internal-link target=_blank}. درباره `root_path` و نحوه استفاده درست از آن بیشتر خواهید آموخت. |
@ -1,160 +1 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/fa/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: fa |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
|||
INHERIT: ../en/mkdocs.yml |
|||
|
@ -1,189 +1 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/fr/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: fr |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
- features.md |
|||
- fastapi-people.md |
|||
- python-types.md |
|||
- Tutoriel - Guide utilisateur: |
|||
- tutorial/first-steps.md |
|||
- tutorial/path-params.md |
|||
- tutorial/query-params.md |
|||
- tutorial/body.md |
|||
- tutorial/background-tasks.md |
|||
- tutorial/debugging.md |
|||
- Guide utilisateur avancé: |
|||
- advanced/index.md |
|||
- advanced/path-operation-advanced-configuration.md |
|||
- advanced/additional-status-codes.md |
|||
- advanced/response-directly.md |
|||
- advanced/additional-responses.md |
|||
- async.md |
|||
- Déploiement: |
|||
- deployment/index.md |
|||
- deployment/versions.md |
|||
- deployment/https.md |
|||
- deployment/deta.md |
|||
- deployment/docker.md |
|||
- deployment/manually.md |
|||
- project-generation.md |
|||
- alternatives.md |
|||
- history-design-future.md |
|||
- external-links.md |
|||
- help-fastapi.md |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
|||
INHERIT: ../en/mkdocs.yml |
|||
|
@ -1,160 +1 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/he/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: he |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
|||
INHERIT: ../en/mkdocs.yml |
|||
|
@ -1,467 +0,0 @@ |
|||
|
|||
{!../../../docs/missing-translation.md!} |
|||
|
|||
|
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>FastAPI framework, high performance, easy to learn, fast to code, ready for production</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/tiangolo/fastapi/actions?query=workflow%3ATest+event%3Apush+branch%3Amaster" target="_blank"> |
|||
<img src="https://github.com/tiangolo/fastapi/workflows/Test/badge.svg?event=push&branch=master" alt="Test"> |
|||
</a> |
|||
<a href="https://coverage-badge.samuelcolvin.workers.dev/redirect/tiangolo/fastapi" target="_blank"> |
|||
<img src="https://coverage-badge.samuelcolvin.workers.dev/tiangolo/fastapi.svg" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/pyversions/fastapi.svg?color=%2334D058" alt="Supported Python versions"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**Documentation**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**Source Code**: <a href="https://github.com/tiangolo/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
|
|||
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. |
|||
|
|||
The key features are: |
|||
|
|||
* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance). |
|||
* **Fast to code**: Increase the speed to develop features by about 200% to 300%. * |
|||
* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. * |
|||
* **Intuitive**: Great editor support. <abbr title="also known as auto-complete, autocompletion, IntelliSense">Completion</abbr> everywhere. Less time debugging. |
|||
* **Easy**: Designed to be easy to use and learn. Less time reading docs. |
|||
* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs. |
|||
* **Robust**: Get production-ready code. With automatic interactive documentation. |
|||
* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (previously known as Swagger) and <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>* estimation based on tests on an internal development team, building production applications.</small> |
|||
|
|||
## Sponsors |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor -%} |
|||
{%- for sponsor in sponsors.silver -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">Other sponsors</a> |
|||
|
|||
## Opinions |
|||
|
|||
"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/tiangolo/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_I’m over the moon excited about **FastAPI**. It’s so fun!_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast host</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_" |
|||
|
|||
"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> founders - <a href="https://spacy.io" target="_blank">spaCy</a> creators</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, the FastAPI of CLIs |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
If you are building a <abbr title="Command Line Interface">CLI</abbr> app to be used in the terminal instead of a web API, check out <a href="https://typer.tiangolo.com/" class="external-link" target="_blank">**Typer**</a>. |
|||
|
|||
**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀 |
|||
|
|||
## Requirements |
|||
|
|||
Python 3.7+ |
|||
|
|||
FastAPI stands on the shoulders of giants: |
|||
|
|||
* <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> for the web parts. |
|||
* <a href="https://pydantic-docs.helpmanual.io/" class="external-link" target="_blank">Pydantic</a> for the data parts. |
|||
|
|||
## Installation |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install fastapi |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
You will also need an ASGI server, for production such as <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a> or <a href="https://github.com/pgjones/hypercorn" class="external-link" target="_blank">Hypercorn</a>. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "uvicorn[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Example |
|||
|
|||
### Create it |
|||
|
|||
* Create a file `main.py` with: |
|||
|
|||
```Python |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>Or use <code>async def</code>...</summary> |
|||
|
|||
If your code uses `async` / `await`, use `async def`: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**Note**: |
|||
|
|||
If you don't know, check the _"In a hurry?"_ section about <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` and `await` in the docs</a>. |
|||
|
|||
</details> |
|||
|
|||
### Run it |
|||
|
|||
Run the server with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [28720] |
|||
INFO: Started server process [28722] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>About the command <code>uvicorn main:app --reload</code>...</summary> |
|||
|
|||
The command `uvicorn main:app` refers to: |
|||
|
|||
* `main`: the file `main.py` (the Python "module"). |
|||
* `app`: the object created inside of `main.py` with the line `app = FastAPI()`. |
|||
* `--reload`: make the server restart after code changes. Only do this for development. |
|||
|
|||
</details> |
|||
|
|||
### Check it |
|||
|
|||
Open your browser at <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a>. |
|||
|
|||
You will see the JSON response as: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
You already created an API that: |
|||
|
|||
* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`. |
|||
* Both _paths_ take `GET` <em>operations</em> (also known as HTTP _methods_). |
|||
* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`. |
|||
* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`. |
|||
|
|||
### Interactive API docs |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
You will see the automatic interactive API documentation (provided by <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>): |
|||
|
|||
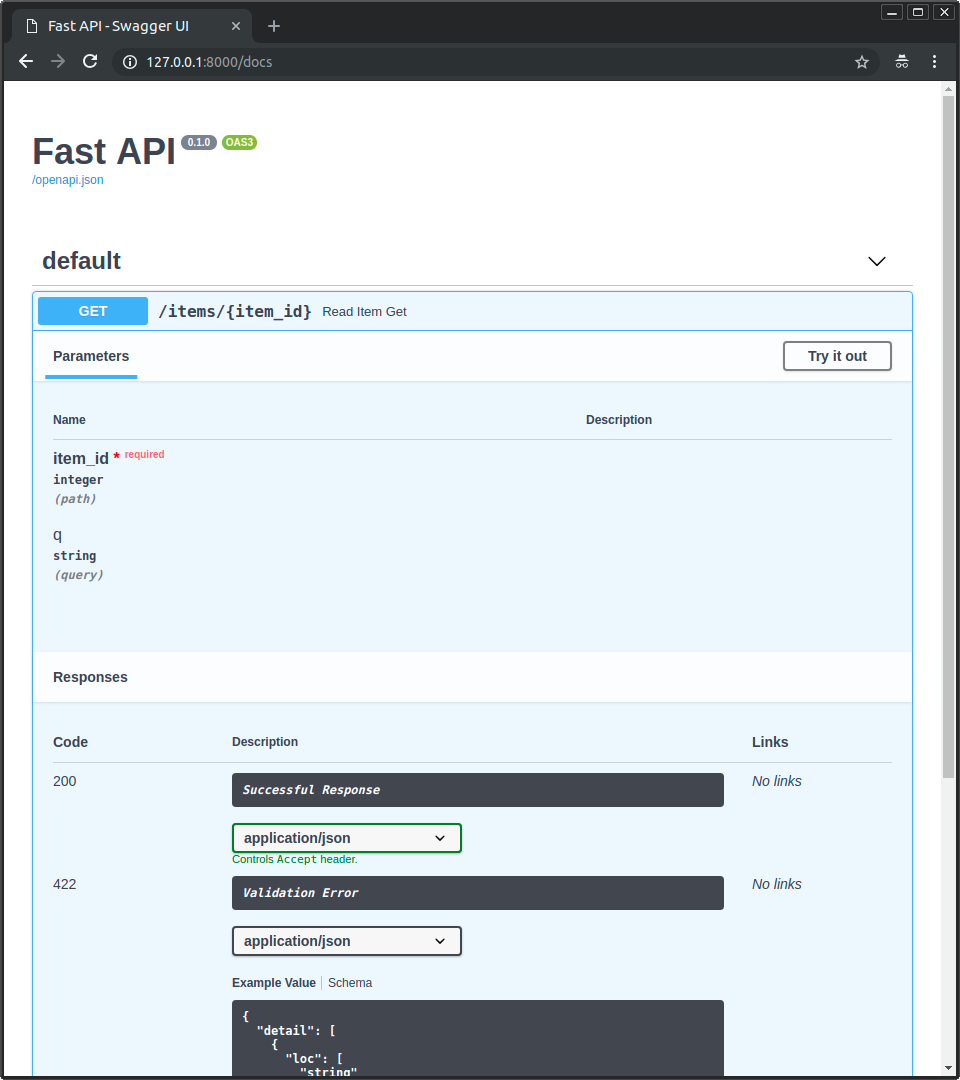 |
|||
|
|||
### Alternative API docs |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
You will see the alternative automatic documentation (provided by <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a>): |
|||
|
|||
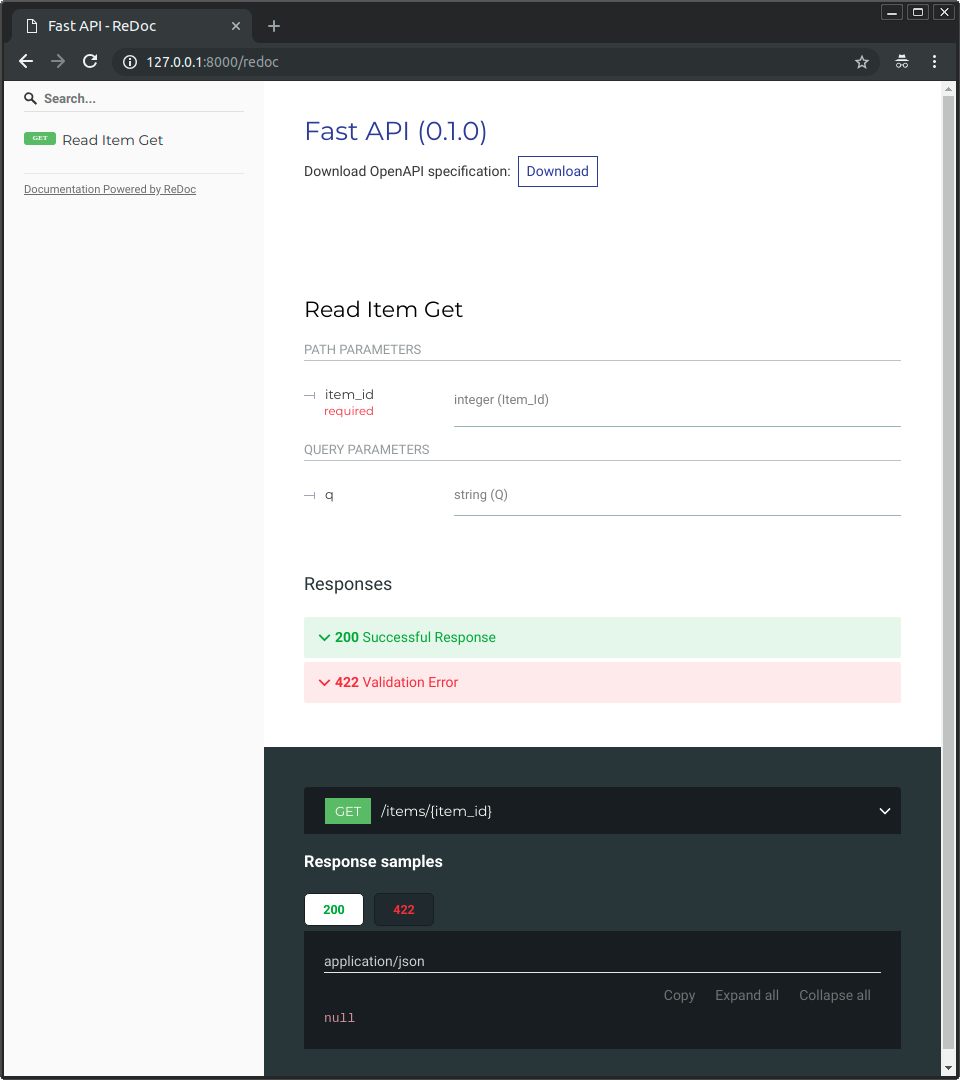 |
|||
|
|||
## Example upgrade |
|||
|
|||
Now modify the file `main.py` to receive a body from a `PUT` request. |
|||
|
|||
Declare the body using standard Python types, thanks to Pydantic. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Union[bool, None] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
The server should reload automatically (because you added `--reload` to the `uvicorn` command above). |
|||
|
|||
### Interactive API docs upgrade |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
* The interactive API documentation will be automatically updated, including the new body: |
|||
|
|||
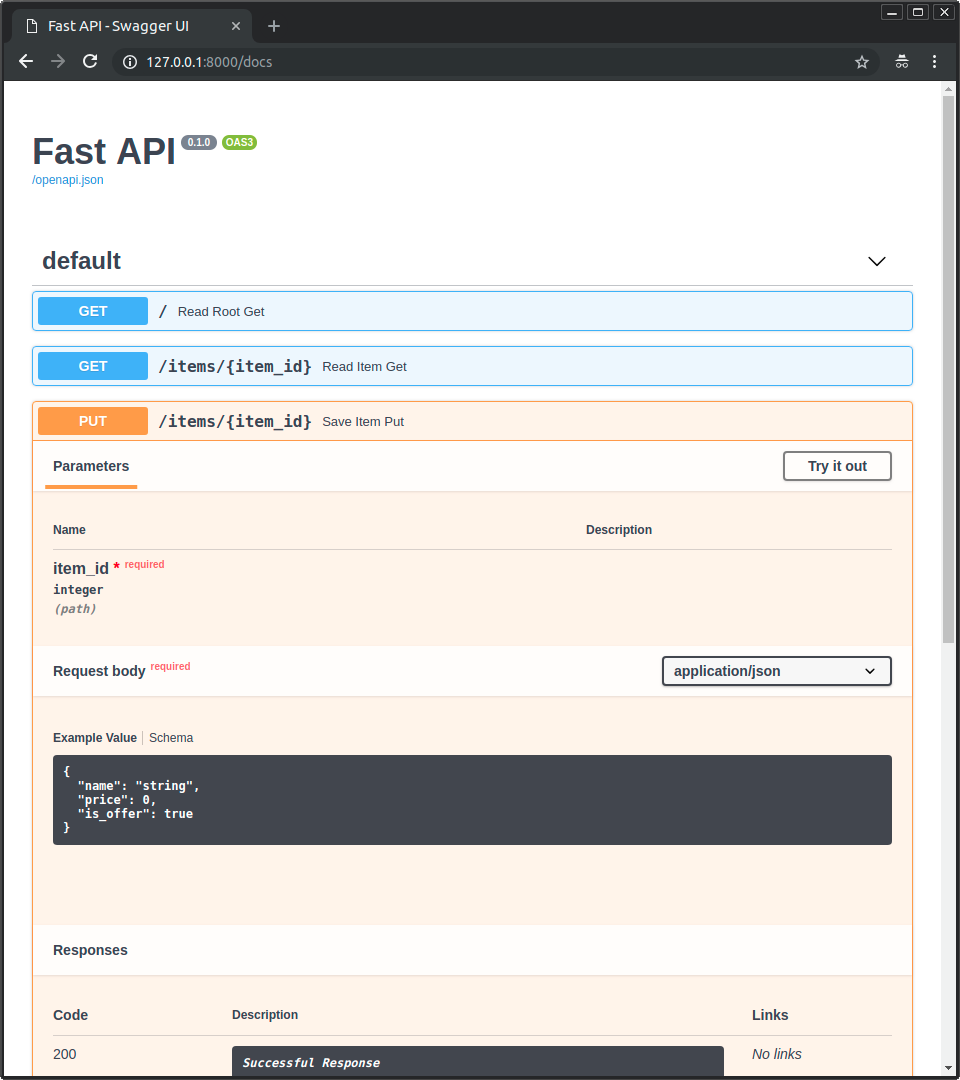 |
|||
|
|||
* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API: |
|||
|
|||
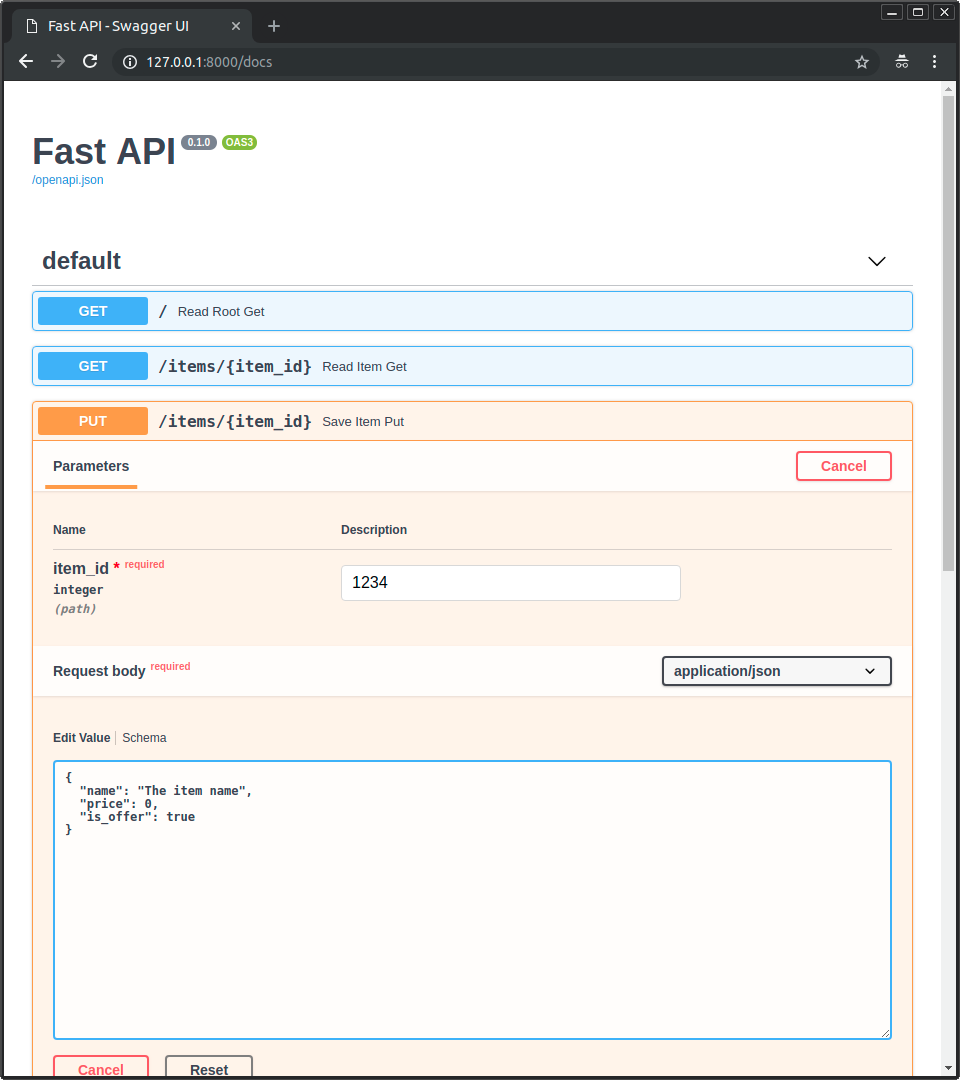 |
|||
|
|||
* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen: |
|||
|
|||
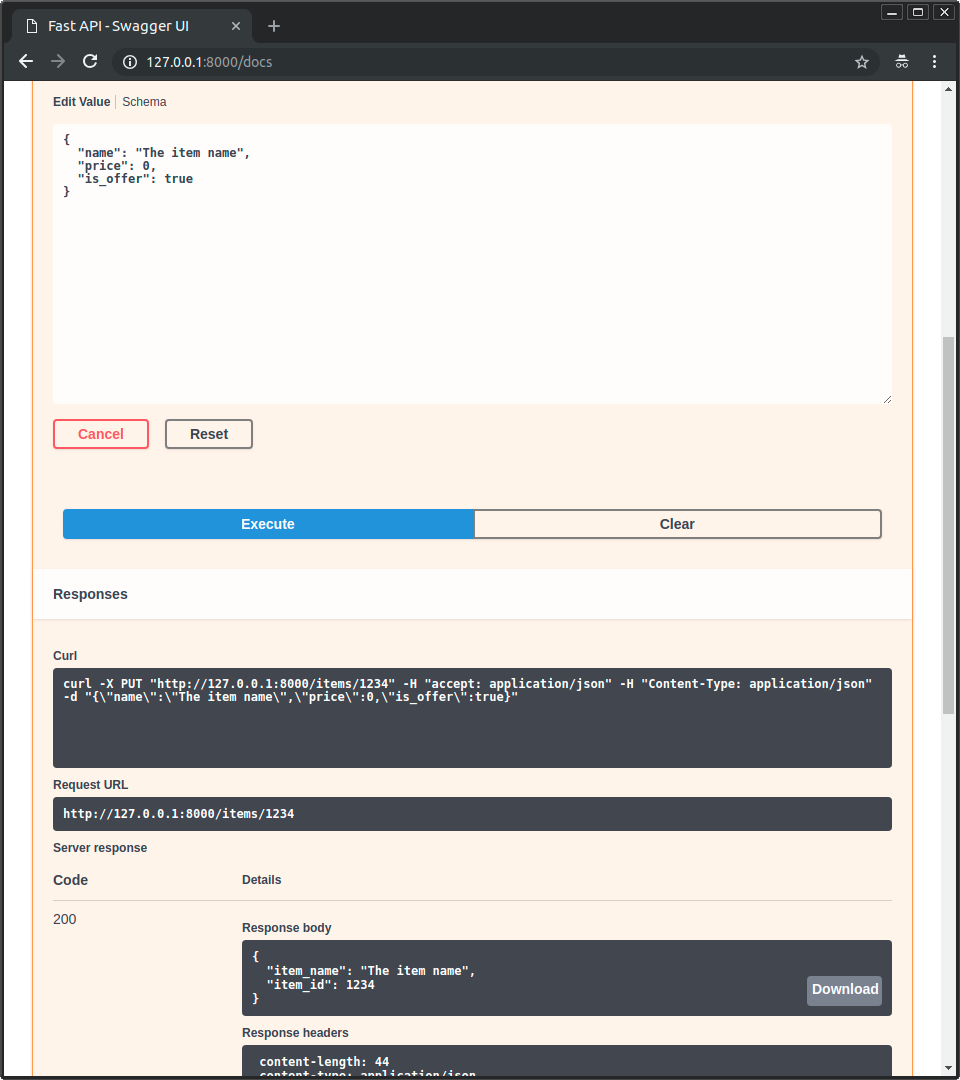 |
|||
|
|||
### Alternative API docs upgrade |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
* The alternative documentation will also reflect the new query parameter and body: |
|||
|
|||
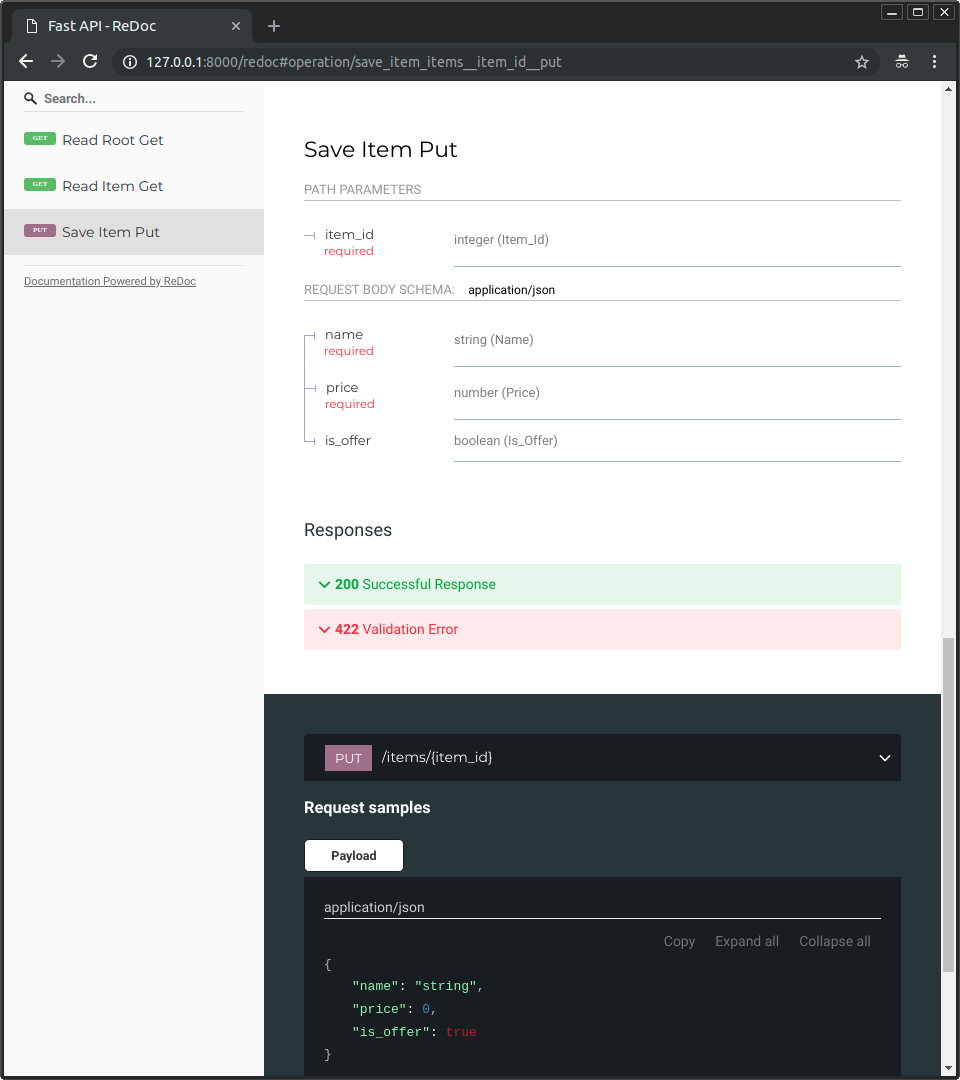 |
|||
|
|||
### Recap |
|||
|
|||
In summary, you declare **once** the types of parameters, body, etc. as function parameters. |
|||
|
|||
You do that with standard modern Python types. |
|||
|
|||
You don't have to learn a new syntax, the methods or classes of a specific library, etc. |
|||
|
|||
Just standard **Python 3.7+**. |
|||
|
|||
For example, for an `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
or for a more complex `Item` model: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...and with that single declaration you get: |
|||
|
|||
* Editor support, including: |
|||
* Completion. |
|||
* Type checks. |
|||
* Validation of data: |
|||
* Automatic and clear errors when the data is invalid. |
|||
* Validation even for deeply nested JSON objects. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of input data: coming from the network to Python data and types. Reading from: |
|||
* JSON. |
|||
* Path parameters. |
|||
* Query parameters. |
|||
* Cookies. |
|||
* Headers. |
|||
* Forms. |
|||
* Files. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of output data: converting from Python data and types to network data (as JSON): |
|||
* Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc). |
|||
* `datetime` objects. |
|||
* `UUID` objects. |
|||
* Database models. |
|||
* ...and many more. |
|||
* Automatic interactive API documentation, including 2 alternative user interfaces: |
|||
* Swagger UI. |
|||
* ReDoc. |
|||
|
|||
--- |
|||
|
|||
Coming back to the previous code example, **FastAPI** will: |
|||
|
|||
* Validate that there is an `item_id` in the path for `GET` and `PUT` requests. |
|||
* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests. |
|||
* If it is not, the client will see a useful, clear error. |
|||
* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests. |
|||
* As the `q` parameter is declared with `= None`, it is optional. |
|||
* Without the `None` it would be required (as is the body in the case with `PUT`). |
|||
* For `PUT` requests to `/items/{item_id}`, Read the body as JSON: |
|||
* Check that it has a required attribute `name` that should be a `str`. |
|||
* Check that it has a required attribute `price` that has to be a `float`. |
|||
* Check that it has an optional attribute `is_offer`, that should be a `bool`, if present. |
|||
* All this would also work for deeply nested JSON objects. |
|||
* Convert from and to JSON automatically. |
|||
* Document everything with OpenAPI, that can be used by: |
|||
* Interactive documentation systems. |
|||
* Automatic client code generation systems, for many languages. |
|||
* Provide 2 interactive documentation web interfaces directly. |
|||
|
|||
--- |
|||
|
|||
We just scratched the surface, but you already get the idea of how it all works. |
|||
|
|||
Try changing the line with: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
...from: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
...to: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
...and see how your editor will auto-complete the attributes and know their types: |
|||
|
|||
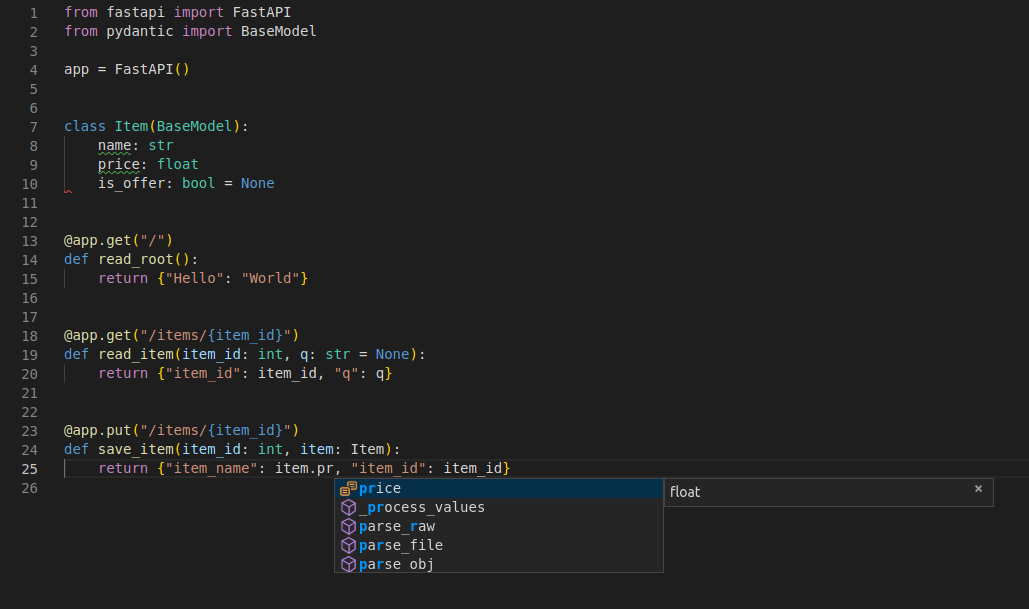 |
|||
|
|||
For a more complete example including more features, see the <a href="https://fastapi.tiangolo.com/tutorial/">Tutorial - User Guide</a>. |
|||
|
|||
**Spoiler alert**: the tutorial - user guide includes: |
|||
|
|||
* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**. |
|||
* How to set **validation constraints** as `maximum_length` or `regex`. |
|||
* A very powerful and easy to use **<abbr title="also known as components, resources, providers, services, injectables">Dependency Injection</abbr>** system. |
|||
* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth. |
|||
* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic). |
|||
* **GraphQL** integration with <a href="https://strawberry.rocks" class="external-link" target="_blank">Strawberry</a> and other libraries. |
|||
* Many extra features (thanks to Starlette) as: |
|||
* **WebSockets** |
|||
* extremely easy tests based on HTTPX and `pytest` |
|||
* **CORS** |
|||
* **Cookie Sessions** |
|||
* ...and more. |
|||
|
|||
## Performance |
|||
|
|||
Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">one of the fastest Python frameworks available</a>, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*) |
|||
|
|||
To understand more about it, see the section <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">Benchmarks</a>. |
|||
|
|||
## Optional Dependencies |
|||
|
|||
Used by Pydantic: |
|||
|
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - for faster JSON <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>. |
|||
* <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - for email validation. |
|||
|
|||
Used by Starlette: |
|||
|
|||
* <a href="https://www.python-httpx.org" target="_blank"><code>httpx</code></a> - Required if you want to use the `TestClient`. |
|||
* <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - Required if you want to use the default template configuration. |
|||
* <a href="https://andrew-d.github.io/python-multipart/" target="_blank"><code>python-multipart</code></a> - Required if you want to support form <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>, with `request.form()`. |
|||
* <a href="https://pythonhosted.org/itsdangerous/" target="_blank"><code>itsdangerous</code></a> - Required for `SessionMiddleware` support. |
|||
* <a href="https://pyyaml.org/wiki/PyYAMLDocumentation" target="_blank"><code>pyyaml</code></a> - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI). |
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - Required if you want to use `UJSONResponse`. |
|||
|
|||
Used by FastAPI / Starlette: |
|||
|
|||
* <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - for the server that loads and serves your application. |
|||
* <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - Required if you want to use `ORJSONResponse`. |
|||
|
|||
You can install all of these with `pip install "fastapi[all]"`. |
|||
|
|||
## License |
|||
|
|||
This project is licensed under the terms of the MIT license. |
@ -1,160 +0,0 @@ |
|||
site_name: FastAPI |
|||
site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production |
|||
site_url: https://fastapi.tiangolo.com/hy/ |
|||
theme: |
|||
name: material |
|||
custom_dir: overrides |
|||
palette: |
|||
- media: '(prefers-color-scheme: light)' |
|||
scheme: default |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb |
|||
name: Switch to light mode |
|||
- media: '(prefers-color-scheme: dark)' |
|||
scheme: slate |
|||
primary: teal |
|||
accent: amber |
|||
toggle: |
|||
icon: material/lightbulb-outline |
|||
name: Switch to dark mode |
|||
features: |
|||
- search.suggest |
|||
- search.highlight |
|||
- content.tabs.link |
|||
icon: |
|||
repo: fontawesome/brands/github-alt |
|||
logo: https://fastapi.tiangolo.com/img/icon-white.svg |
|||
favicon: https://fastapi.tiangolo.com/img/favicon.png |
|||
language: hy |
|||
repo_name: tiangolo/fastapi |
|||
repo_url: https://github.com/tiangolo/fastapi |
|||
edit_uri: '' |
|||
plugins: |
|||
- search |
|||
- markdownextradata: |
|||
data: data |
|||
nav: |
|||
- FastAPI: index.md |
|||
- Languages: |
|||
- en: / |
|||
- az: /az/ |
|||
- cs: /cs/ |
|||
- de: /de/ |
|||
- em: /em/ |
|||
- es: /es/ |
|||
- fa: /fa/ |
|||
- fr: /fr/ |
|||
- he: /he/ |
|||
- hy: /hy/ |
|||
- id: /id/ |
|||
- it: /it/ |
|||
- ja: /ja/ |
|||
- ko: /ko/ |
|||
- lo: /lo/ |
|||
- nl: /nl/ |
|||
- pl: /pl/ |
|||
- pt: /pt/ |
|||
- ru: /ru/ |
|||
- sq: /sq/ |
|||
- sv: /sv/ |
|||
- ta: /ta/ |
|||
- tr: /tr/ |
|||
- uk: /uk/ |
|||
- zh: /zh/ |
|||
markdown_extensions: |
|||
- toc: |
|||
permalink: true |
|||
- markdown.extensions.codehilite: |
|||
guess_lang: false |
|||
- mdx_include: |
|||
base_path: docs |
|||
- admonition |
|||
- codehilite |
|||
- extra |
|||
- pymdownx.superfences: |
|||
custom_fences: |
|||
- name: mermaid |
|||
class: mermaid |
|||
format: !!python/name:pymdownx.superfences.fence_code_format '' |
|||
- pymdownx.tabbed: |
|||
alternate_style: true |
|||
- attr_list |
|||
- md_in_html |
|||
extra: |
|||
analytics: |
|||
provider: google |
|||
property: G-YNEVN69SC3 |
|||
social: |
|||
- icon: fontawesome/brands/github-alt |
|||
link: https://github.com/tiangolo/fastapi |
|||
- icon: fontawesome/brands/discord |
|||
link: https://discord.gg/VQjSZaeJmf |
|||
- icon: fontawesome/brands/twitter |
|||
link: https://twitter.com/fastapi |
|||
- icon: fontawesome/brands/linkedin |
|||
link: https://www.linkedin.com/in/tiangolo |
|||
- icon: fontawesome/brands/dev |
|||
link: https://dev.to/tiangolo |
|||
- icon: fontawesome/brands/medium |
|||
link: https://medium.com/@tiangolo |
|||
- icon: fontawesome/solid/globe |
|||
link: https://tiangolo.com |
|||
alternate: |
|||
- link: / |
|||
name: en - English |
|||
- link: /az/ |
|||
name: az |
|||
- link: /cs/ |
|||
name: cs |
|||
- link: /de/ |
|||
name: de |
|||
- link: /em/ |
|||
name: 😉 |
|||
- link: /es/ |
|||
name: es - español |
|||
- link: /fa/ |
|||
name: fa |
|||
- link: /fr/ |
|||
name: fr - français |
|||
- link: /he/ |
|||
name: he |
|||
- link: /hy/ |
|||
name: hy |
|||
- link: /id/ |
|||
name: id |
|||
- link: /it/ |
|||
name: it - italiano |
|||
- link: /ja/ |
|||
name: ja - 日本語 |
|||
- link: /ko/ |
|||
name: ko - 한국어 |
|||
- link: /lo/ |
|||
name: lo - ພາສາລາວ |
|||
- link: /nl/ |
|||
name: nl |
|||
- link: /pl/ |
|||
name: pl |
|||
- link: /pt/ |
|||
name: pt - português |
|||
- link: /ru/ |
|||
name: ru - русский язык |
|||
- link: /sq/ |
|||
name: sq - shqip |
|||
- link: /sv/ |
|||
name: sv - svenska |
|||
- link: /ta/ |
|||
name: ta - தமிழ் |
|||
- link: /tr/ |
|||
name: tr - Türkçe |
|||
- link: /uk/ |
|||
name: uk - українська мова |
|||
- link: /zh/ |
|||
name: zh - 汉语 |
|||
extra_css: |
|||
- https://fastapi.tiangolo.com/css/termynal.css |
|||
- https://fastapi.tiangolo.com/css/custom.css |
|||
extra_javascript: |
|||
- https://fastapi.tiangolo.com/js/termynal.js |
|||
- https://fastapi.tiangolo.com/js/custom.js |
@ -1,466 +0,0 @@ |
|||
|
|||
{!../../../docs/missing-translation.md!} |
|||
|
|||
|
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>FastAPI framework, high performance, easy to learn, fast to code, ready for production</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/tiangolo/fastapi/actions?query=workflow%3ATest" target="_blank"> |
|||
<img src="https://github.com/tiangolo/fastapi/workflows/Test/badge.svg" alt="Test"> |
|||
</a> |
|||
<a href="https://codecov.io/gh/tiangolo/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/codecov/c/github/tiangolo/fastapi?color=%2334D058" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**Documentation**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**Source Code**: <a href="https://github.com/tiangolo/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
|
|||
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints. |
|||
|
|||
The key features are: |
|||
|
|||
* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance). |
|||
|
|||
* **Fast to code**: Increase the speed to develop features by about 200% to 300%. * |
|||
* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. * |
|||
* **Intuitive**: Great editor support. <abbr title="also known as auto-complete, autocompletion, IntelliSense">Completion</abbr> everywhere. Less time debugging. |
|||
* **Easy**: Designed to be easy to use and learn. Less time reading docs. |
|||
* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs. |
|||
* **Robust**: Get production-ready code. With automatic interactive documentation. |
|||
* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (previously known as Swagger) and <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>* estimation based on tests on an internal development team, building production applications.</small> |
|||
|
|||
## Sponsors |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor -%} |
|||
{%- for sponsor in sponsors.silver -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">Other sponsors</a> |
|||
|
|||
## Opinions |
|||
|
|||
"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/tiangolo/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_I’m over the moon excited about **FastAPI**. It’s so fun!_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast host</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_" |
|||
|
|||
"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> founders - <a href="https://spacy.io" target="_blank">spaCy</a> creators</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, the FastAPI of CLIs |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
If you are building a <abbr title="Command Line Interface">CLI</abbr> app to be used in the terminal instead of a web API, check out <a href="https://typer.tiangolo.com/" class="external-link" target="_blank">**Typer**</a>. |
|||
|
|||
**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀 |
|||
|
|||
## Requirements |
|||
|
|||
Python 3.7+ |
|||
|
|||
FastAPI stands on the shoulders of giants: |
|||
|
|||
* <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> for the web parts. |
|||
* <a href="https://pydantic-docs.helpmanual.io/" class="external-link" target="_blank">Pydantic</a> for the data parts. |
|||
|
|||
## Installation |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install fastapi |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
You will also need an ASGI server, for production such as <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a> or <a href="https://gitlab.com/pgjones/hypercorn" class="external-link" target="_blank">Hypercorn</a>. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "uvicorn[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Example |
|||
|
|||
### Create it |
|||
|
|||
* Create a file `main.py` with: |
|||
|
|||
```Python |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Optional[str] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>Or use <code>async def</code>...</summary> |
|||
|
|||
If your code uses `async` / `await`, use `async def`: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Optional[str] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**Note**: |
|||
|
|||
If you don't know, check the _"In a hurry?"_ section about <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` and `await` in the docs</a>. |
|||
|
|||
</details> |
|||
|
|||
### Run it |
|||
|
|||
Run the server with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uvicorn main:app --reload |
|||
|
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [28720] |
|||
INFO: Started server process [28722] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>About the command <code>uvicorn main:app --reload</code>...</summary> |
|||
|
|||
The command `uvicorn main:app` refers to: |
|||
|
|||
* `main`: the file `main.py` (the Python "module"). |
|||
* `app`: the object created inside of `main.py` with the line `app = FastAPI()`. |
|||
* `--reload`: make the server restart after code changes. Only do this for development. |
|||
|
|||
</details> |
|||
|
|||
### Check it |
|||
|
|||
Open your browser at <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a>. |
|||
|
|||
You will see the JSON response as: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
You already created an API that: |
|||
|
|||
* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`. |
|||
* Both _paths_ take `GET` <em>operations</em> (also known as HTTP _methods_). |
|||
* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`. |
|||
* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`. |
|||
|
|||
### Interactive API docs |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
You will see the automatic interactive API documentation (provided by <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>): |
|||
|
|||
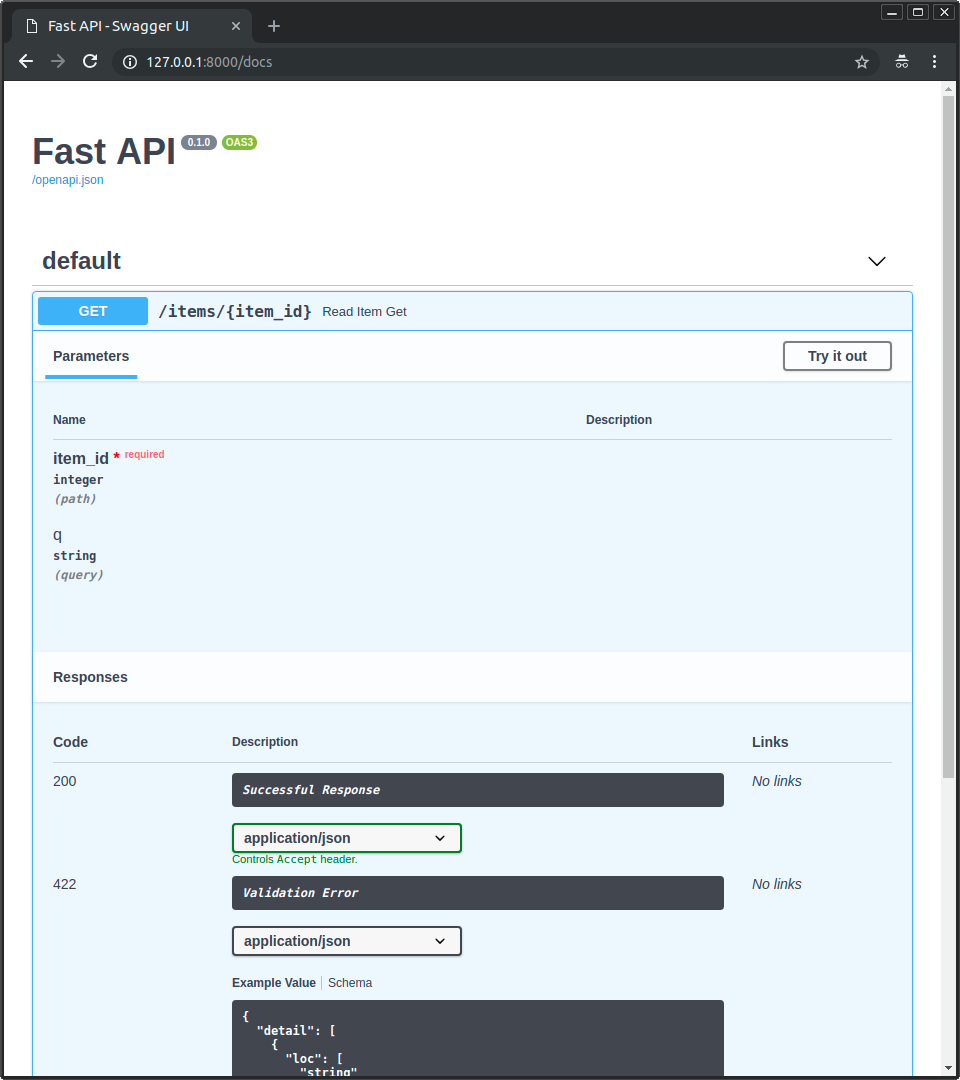 |
|||
|
|||
### Alternative API docs |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
You will see the alternative automatic documentation (provided by <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a>): |
|||
|
|||
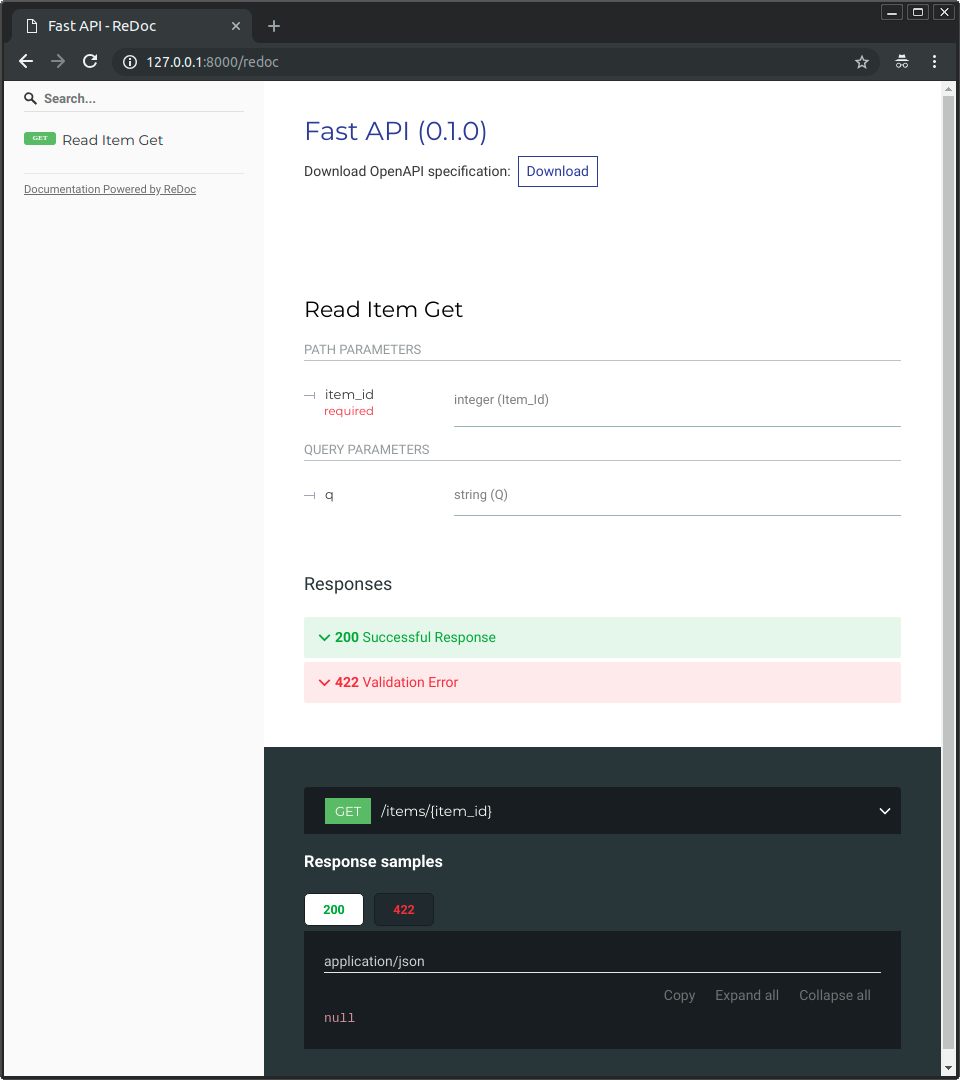 |
|||
|
|||
## Example upgrade |
|||
|
|||
Now modify the file `main.py` to receive a body from a `PUT` request. |
|||
|
|||
Declare the body using standard Python types, thanks to Pydantic. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Optional |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Optional[bool] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Optional[str] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
The server should reload automatically (because you added `--reload` to the `uvicorn` command above). |
|||
|
|||
### Interactive API docs upgrade |
|||
|
|||
Now go to <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
* The interactive API documentation will be automatically updated, including the new body: |
|||
|
|||
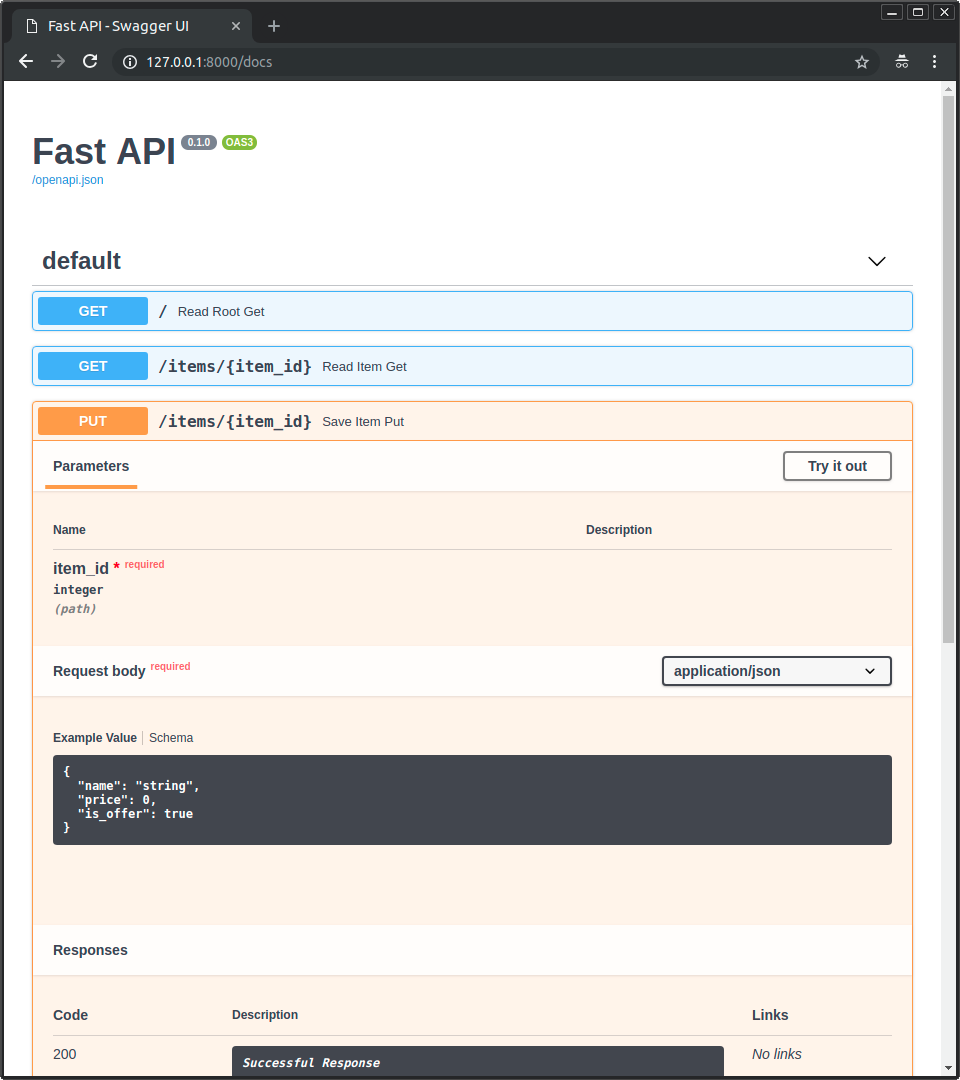 |
|||
|
|||
* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API: |
|||
|
|||
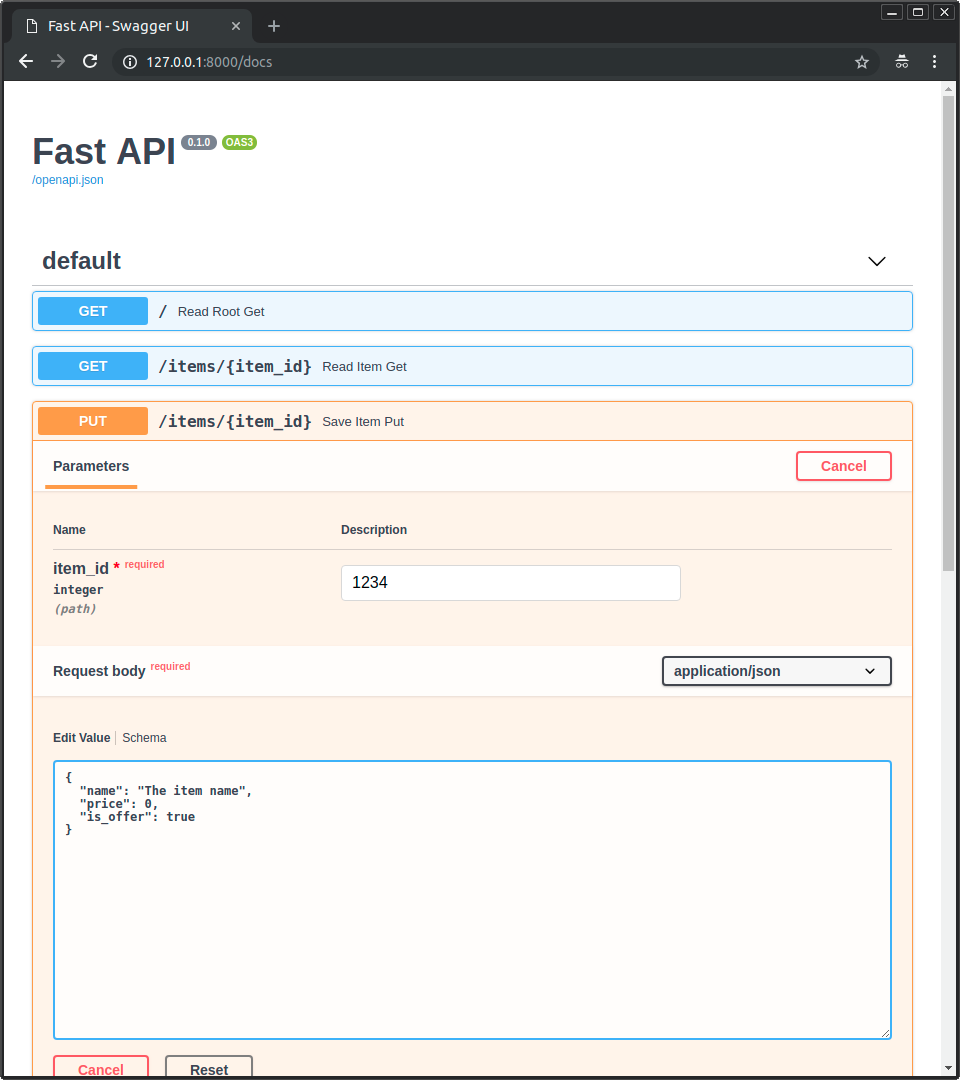 |
|||
|
|||
* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen: |
|||
|
|||
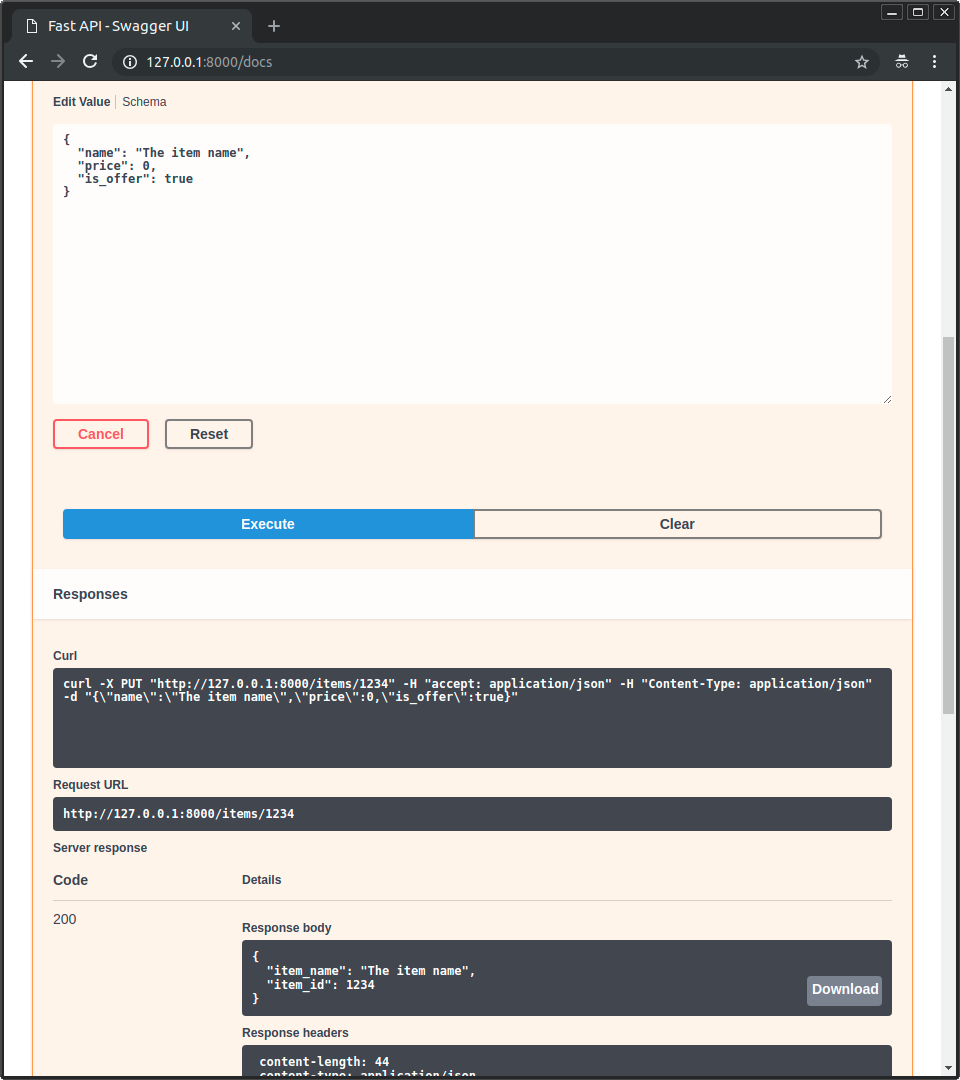 |
|||
|
|||
### Alternative API docs upgrade |
|||
|
|||
And now, go to <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
* The alternative documentation will also reflect the new query parameter and body: |
|||
|
|||
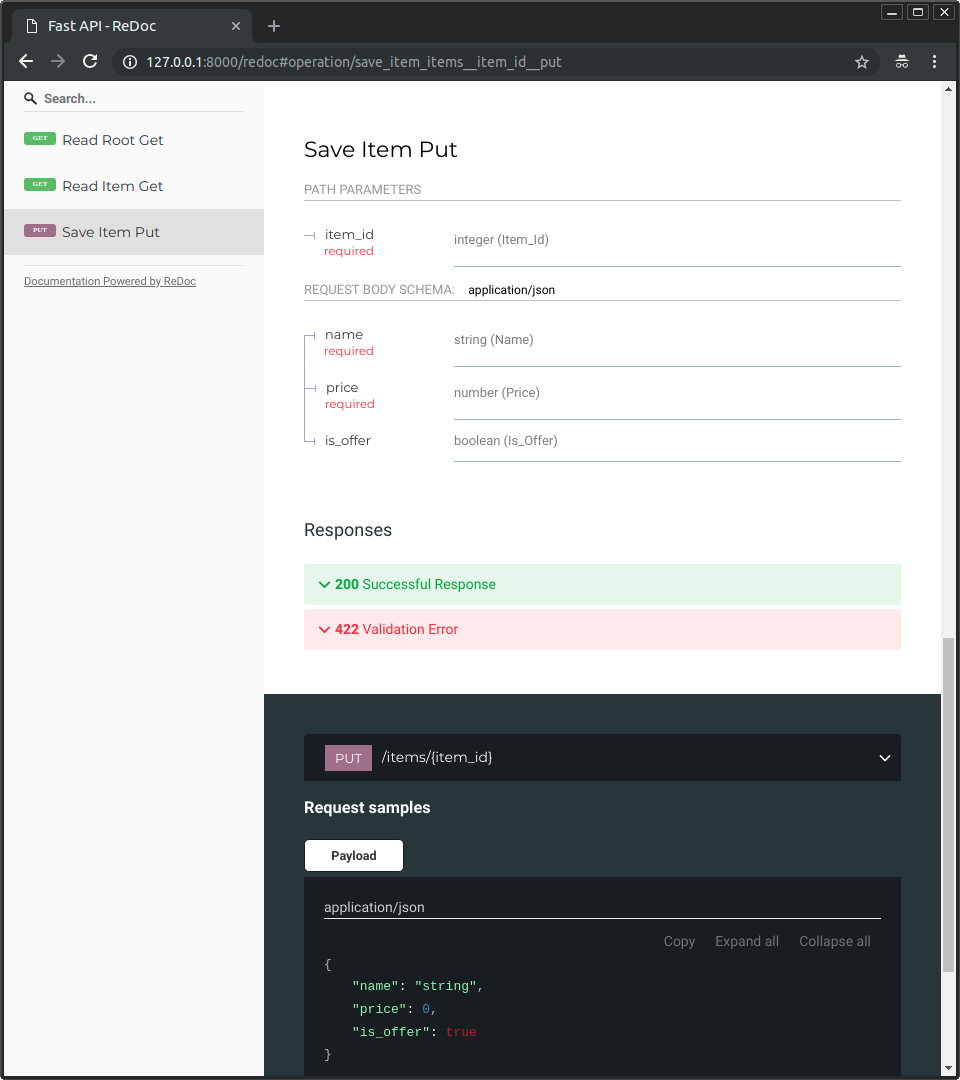 |
|||
|
|||
### Recap |
|||
|
|||
In summary, you declare **once** the types of parameters, body, etc. as function parameters. |
|||
|
|||
You do that with standard modern Python types. |
|||
|
|||
You don't have to learn a new syntax, the methods or classes of a specific library, etc. |
|||
|
|||
Just standard **Python 3.6+**. |
|||
|
|||
For example, for an `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
or for a more complex `Item` model: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...and with that single declaration you get: |
|||
|
|||
* Editor support, including: |
|||
* Completion. |
|||
* Type checks. |
|||
* Validation of data: |
|||
* Automatic and clear errors when the data is invalid. |
|||
* Validation even for deeply nested JSON objects. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of input data: coming from the network to Python data and types. Reading from: |
|||
* JSON. |
|||
* Path parameters. |
|||
* Query parameters. |
|||
* Cookies. |
|||
* Headers. |
|||
* Forms. |
|||
* Files. |
|||
* <abbr title="also known as: serialization, parsing, marshalling">Conversion</abbr> of output data: converting from Python data and types to network data (as JSON): |
|||
* Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc). |
|||
* `datetime` objects. |
|||
* `UUID` objects. |
|||
* Database models. |
|||
* ...and many more. |
|||
* Automatic interactive API documentation, including 2 alternative user interfaces: |
|||
* Swagger UI. |
|||
* ReDoc. |
|||
|
|||
--- |
|||
|
|||
Coming back to the previous code example, **FastAPI** will: |
|||
|
|||
* Validate that there is an `item_id` in the path for `GET` and `PUT` requests. |
|||
* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests. |
|||
* If it is not, the client will see a useful, clear error. |
|||
* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests. |
|||
* As the `q` parameter is declared with `= None`, it is optional. |
|||
* Without the `None` it would be required (as is the body in the case with `PUT`). |
|||
* For `PUT` requests to `/items/{item_id}`, Read the body as JSON: |
|||
* Check that it has a required attribute `name` that should be a `str`. |
|||
* Check that it has a required attribute `price` that has to be a `float`. |
|||
* Check that it has an optional attribute `is_offer`, that should be a `bool`, if present. |
|||
* All this would also work for deeply nested JSON objects. |
|||
* Convert from and to JSON automatically. |
|||
* Document everything with OpenAPI, that can be used by: |
|||
* Interactive documentation systems. |
|||
* Automatic client code generation systems, for many languages. |
|||
* Provide 2 interactive documentation web interfaces directly. |
|||
|
|||
--- |
|||
|
|||
We just scratched the surface, but you already get the idea of how it all works. |
|||
|
|||
Try changing the line with: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
...from: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
...to: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
...and see how your editor will auto-complete the attributes and know their types: |
|||
|
|||
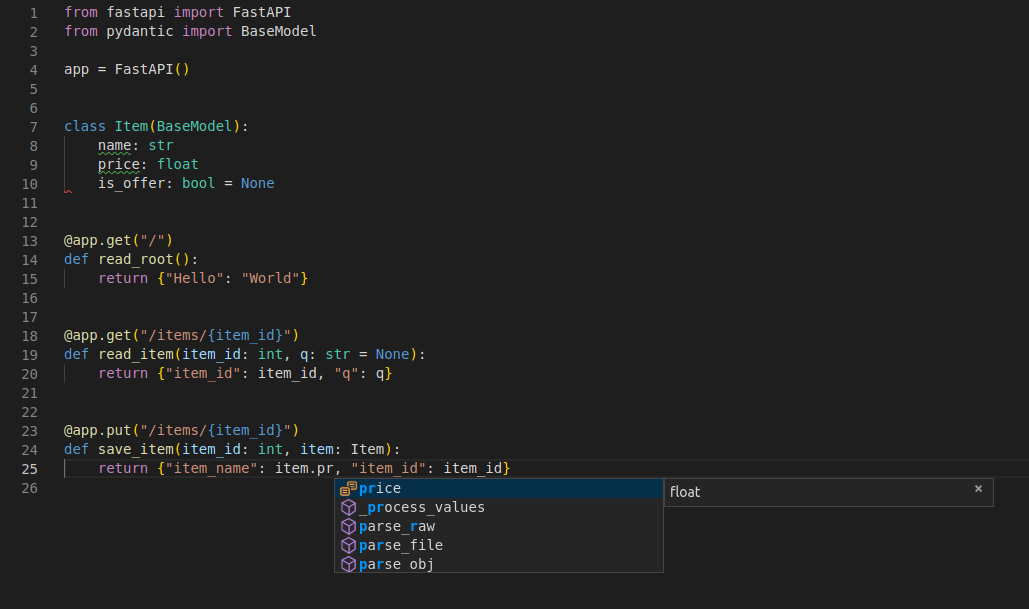 |
|||
|
|||
For a more complete example including more features, see the <a href="https://fastapi.tiangolo.com/tutorial/">Tutorial - User Guide</a>. |
|||
|
|||
**Spoiler alert**: the tutorial - user guide includes: |
|||
|
|||
* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**. |
|||
* How to set **validation constraints** as `maximum_length` or `regex`. |
|||
* A very powerful and easy to use **<abbr title="also known as components, resources, providers, services, injectables">Dependency Injection</abbr>** system. |
|||
* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth. |
|||
* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic). |
|||
* Many extra features (thanks to Starlette) as: |
|||
* **WebSockets** |
|||
* **GraphQL** |
|||
* extremely easy tests based on HTTPX and `pytest` |
|||
* **CORS** |
|||
* **Cookie Sessions** |
|||
* ...and more. |
|||
|
|||
## Performance |
|||
|
|||
Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">one of the fastest Python frameworks available</a>, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*) |
|||
|
|||
To understand more about it, see the section <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">Benchmarks</a>. |
|||
|
|||
## Optional Dependencies |
|||
|
|||
Used by Pydantic: |
|||
|
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - for faster JSON <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>. |
|||
* <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - for email validation. |
|||
|
|||
Used by Starlette: |
|||
|
|||
* <a href="https://www.python-httpx.org" target="_blank"><code>httpx</code></a> - Required if you want to use the `TestClient`. |
|||
* <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - Required if you want to use the default template configuration. |
|||
* <a href="https://andrew-d.github.io/python-multipart/" target="_blank"><code>python-multipart</code></a> - Required if you want to support form <abbr title="converting the string that comes from an HTTP request into Python data">"parsing"</abbr>, with `request.form()`. |
|||
* <a href="https://pythonhosted.org/itsdangerous/" target="_blank"><code>itsdangerous</code></a> - Required for `SessionMiddleware` support. |
|||
* <a href="https://pyyaml.org/wiki/PyYAMLDocumentation" target="_blank"><code>pyyaml</code></a> - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI). |
|||
* <a href="https://graphene-python.org/" target="_blank"><code>graphene</code></a> - Required for `GraphQLApp` support. |
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - Required if you want to use `UJSONResponse`. |
|||
|
|||
Used by FastAPI / Starlette: |
|||
|
|||
* <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - for the server that loads and serves your application. |
|||
* <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - Required if you want to use `ORJSONResponse`. |
|||
|
|||
You can install all of these with `pip install fastapi[all]`. |
|||
|
|||
## License |
|||
|
|||
This project is licensed under the terms of the MIT license. |
Some files were not shown because too many files changed in this diff
Loading…
Reference in new issue