diff --git a/README.md b/README.md index 67275d29d..279a5fd71 100644 --- a/README.md +++ b/README.md @@ -262,12 +262,20 @@ You will see the automatic interactive API documentation (provided by http://127.0.0.1:8000/redoc. +There are two alternatives to Swagger UI: Redocly & Scalar. + +Go to http://127.0.0.1:8000/redoc. You will see the alternative automatic documentation (provided by ReDoc): 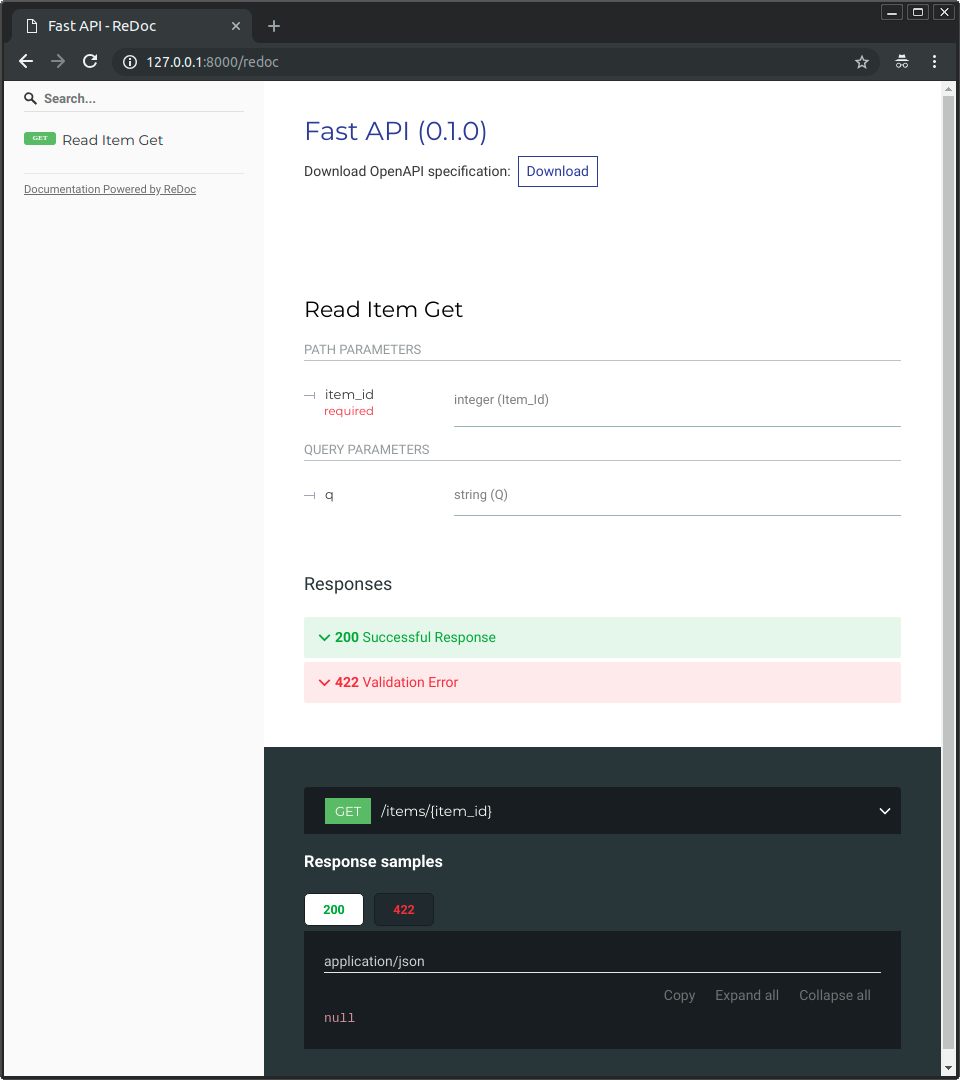 +And now, go to http://127.0.0.1:8000/scalar. + +You will see the alternative automatic documentation (provided by Scalar): + + + ## Example upgrade Now modify the file `main.py` to receive a body from a `PUT` request. @@ -324,12 +332,20 @@ Now go to http://127.0.0.1:8000/redoc. +There are two alternatives to Swagger UI: Redocly & Scalar. + +Go to http://127.0.0.1:8000/redoc. * The alternative documentation will also reflect the new query parameter and body: 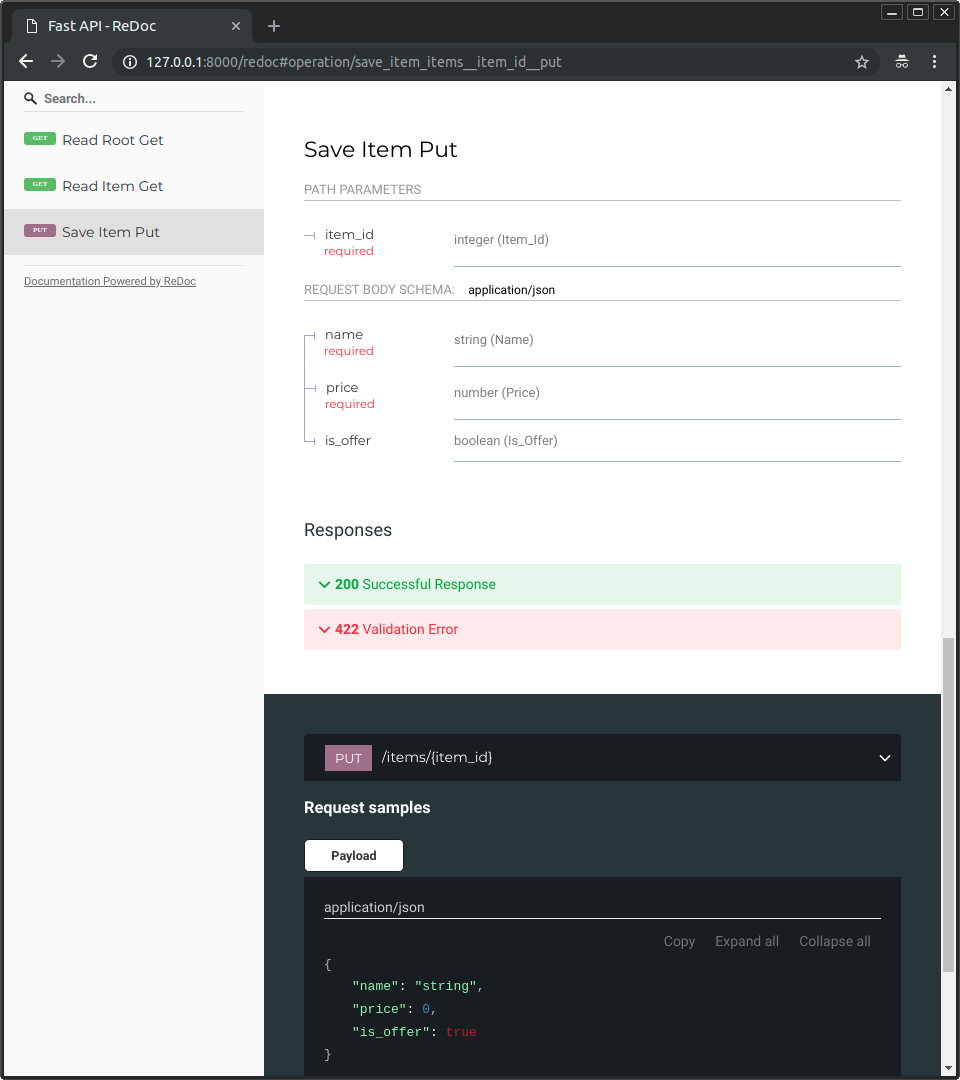 +And now, go to http://127.0.0.1:8000/scalar. + +* The alternative documentation will also reflect the new query parameter and body: + + + ### Recap In summary, you declare **once** the types of parameters, body, etc. as function parameters. diff --git a/docs/en/docs/features.md b/docs/en/docs/features.md index 6f0e74b3d..f8f93e081 100644 --- a/docs/en/docs/features.md +++ b/docs/en/docs/features.md @@ -24,10 +24,15 @@ Interactive API documentation and exploration web user interfaces. As the framew 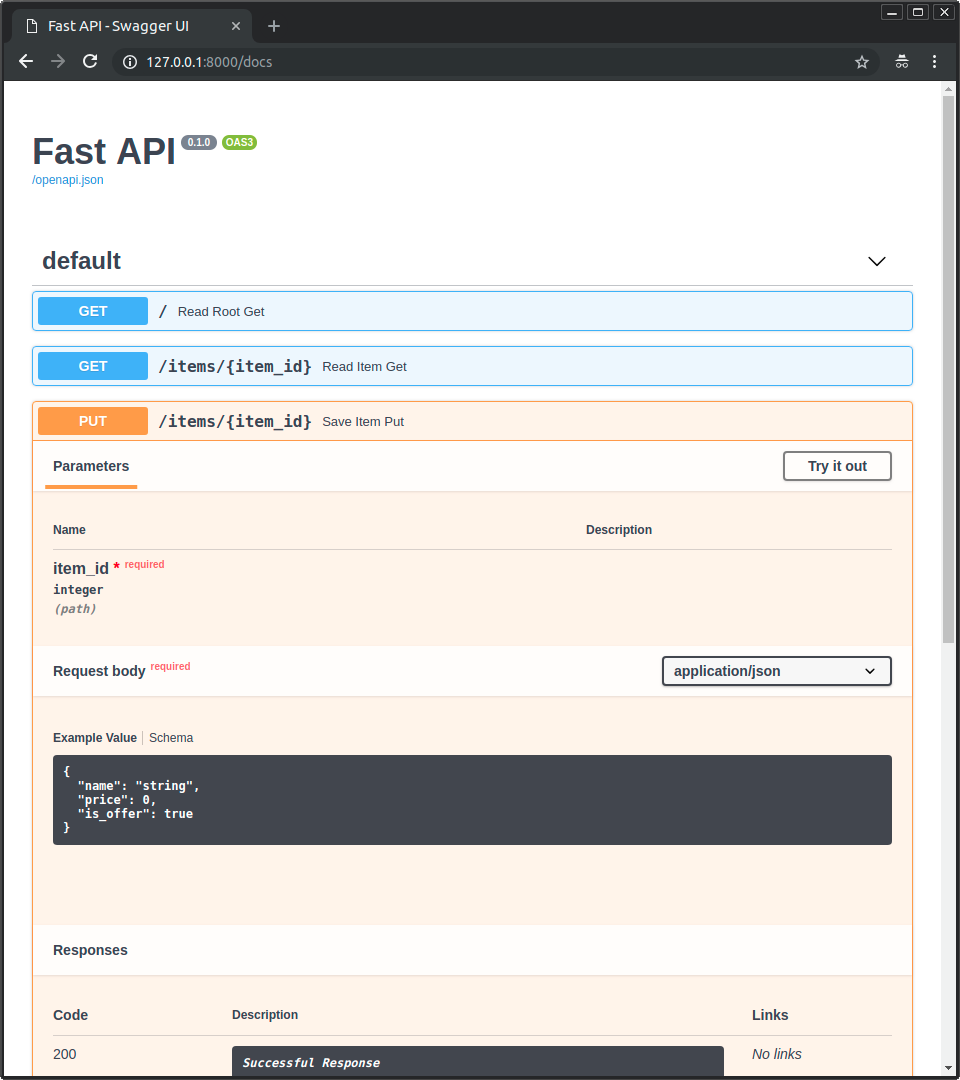 -* Alternative API documentation with ReDoc. +* Alternative API documentation with Redocly or Scalar +* ReDoc. 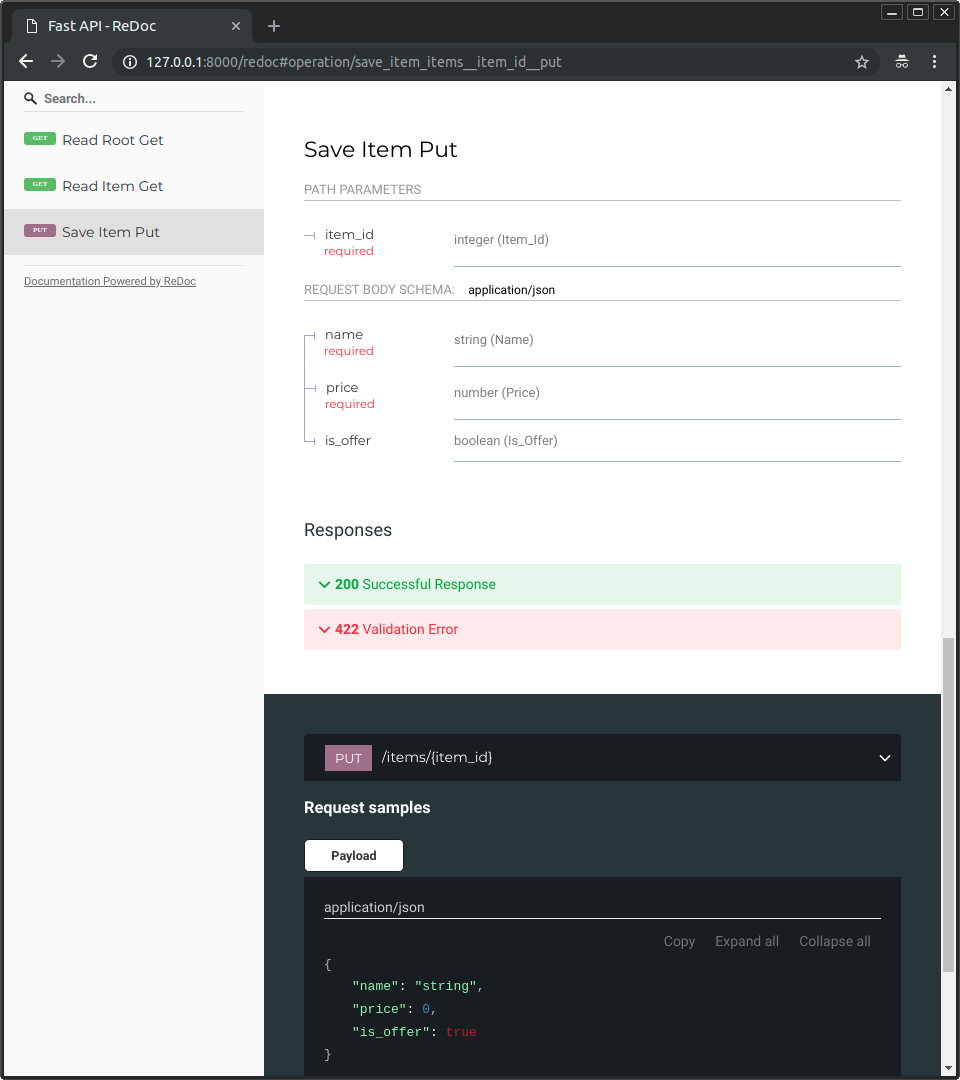 +* Scalar. + + + ### Just Modern Python It's all based on standard **Python 3.6 type** declarations (thanks to Pydantic). No new syntax to learn. Just standard modern Python. diff --git a/docs/en/docs/how-to/custom-docs-ui-assets.md b/docs/en/docs/how-to/custom-docs-ui-assets.md index 9726be2c7..ca92b9ca0 100644 --- a/docs/en/docs/how-to/custom-docs-ui-assets.md +++ b/docs/en/docs/how-to/custom-docs-ui-assets.md @@ -103,6 +103,10 @@ And **ReDoc** uses the file: * `redoc.standalone.js` +And **Scalar** uses the file: + +* `scalar.standalone.js` + After that, your file structure could look like: ``` @@ -112,6 +116,7 @@ After that, your file structure could look like: │ ├── main.py └── static ├── redoc.standalone.js + ├── scalar.standalone.js ├── swagger-ui-bundle.js └── swagger-ui.css ``` diff --git a/docs/en/docs/img/index/index-07-scalar-simple.png b/docs/en/docs/img/index/index-07-scalar-simple.png new file mode 100644 index 000000000..69463b408 Binary files /dev/null and b/docs/en/docs/img/index/index-07-scalar-simple.png differ diff --git a/docs/en/docs/img/index/index-08-scalar-02.png b/docs/en/docs/img/index/index-08-scalar-02.png new file mode 100644 index 000000000..e69de29bb diff --git a/docs/en/docs/reference/openapi/docs.md b/docs/en/docs/reference/openapi/docs.md index ab620833e..baa4d5550 100644 --- a/docs/en/docs/reference/openapi/docs.md +++ b/docs/en/docs/reference/openapi/docs.md @@ -1,11 +1,13 @@ # OpenAPI `docs` -Utilities to handle OpenAPI automatic UI documentation, including Swagger UI (by default at `/docs`) and ReDoc (by default at `/redoc`). +Utilities to handle OpenAPI automatic UI documentation, including Swagger UI (by default at `/docs`), ReDoc (by default at `/redoc`) & Scalar (by default at `/scalar`). ::: fastapi.openapi.docs.get_swagger_ui_html ::: fastapi.openapi.docs.get_redoc_html +::: fastapi.openapi.docs.get_scalar_html + ::: fastapi.openapi.docs.get_swagger_ui_oauth2_redirect_html ::: fastapi.openapi.docs.swagger_ui_default_parameters diff --git a/docs_src/custom_docs_ui/tutorial001.py b/docs_src/custom_docs_ui/tutorial001.py index 4384433e3..0af2c6760 100644 --- a/docs_src/custom_docs_ui/tutorial001.py +++ b/docs_src/custom_docs_ui/tutorial001.py @@ -2,10 +2,11 @@ from fastapi import FastAPI from fastapi.openapi.docs import ( get_redoc_html, get_swagger_ui_html, + get_scalar_html, get_swagger_ui_oauth2_redirect_html, ) -app = FastAPI(docs_url=None, redoc_url=None) +app = FastAPI(docs_url=None, redoc_url=None, scalar_url=None) @app.get("/docs", include_in_schema=False) @@ -32,6 +33,13 @@ async def redoc_html(): redoc_js_url="https://unpkg.com/redoc@next/bundles/redoc.standalone.js", ) +@app.get("/scalar", include_in_schema=False) +async def scalar_html(): + return get_scalar_html( + openapi_url=app.openapi_url, + title=app.title + " - Scalar", + scalar_js_url="https://cdn.jsdelivr.net/npm/@scalar/api-reference", + ) @app.get("/users/{username}") async def read_user(username: str): diff --git a/docs_src/custom_docs_ui/tutorial002.py b/docs_src/custom_docs_ui/tutorial002.py index 23ea368f8..94f05dd35 100644 --- a/docs_src/custom_docs_ui/tutorial002.py +++ b/docs_src/custom_docs_ui/tutorial002.py @@ -2,11 +2,12 @@ from fastapi import FastAPI from fastapi.openapi.docs import ( get_redoc_html, get_swagger_ui_html, + get_scalar_html, get_swagger_ui_oauth2_redirect_html, ) from fastapi.staticfiles import StaticFiles -app = FastAPI(docs_url=None, redoc_url=None) +app = FastAPI(docs_url=None, redoc_url=None, scalar_url=None) app.mount("/static", StaticFiles(directory="static"), name="static") @@ -35,6 +36,14 @@ async def redoc_html(): redoc_js_url="/static/redoc.standalone.js", ) +@app.get("/scalar", include_in_schema=False) +async def scalar_html(): + return get_scalar_html( + openapi_url=app.openapi_url, + title=app.title + " - Scalar", + scalar_js_url="/static/scalar.standalone.js", + ) + @app.get("/users/{username}") async def read_user(username: str): diff --git a/fastapi/applications.py b/fastapi/applications.py index d3edcc880..264fa8cb4 100644 --- a/fastapi/applications.py +++ b/fastapi/applications.py @@ -24,6 +24,7 @@ from fastapi.exceptions import RequestValidationError, WebSocketRequestValidatio from fastapi.logger import logger from fastapi.openapi.docs import ( get_redoc_html, + get_scalar_html, get_swagger_ui_html, get_swagger_ui_oauth2_redirect_html, ) @@ -444,6 +445,30 @@ class FastAPI(Starlette): """ ), ] = "/redoc", + scalar_url: Annotated[ + Optional[str], + Doc( + """ + The path to the alternative automatic interactive API documentation + provided by ReDoc. + + The default URL is `/scalar`. You can disable it by setting it to `None`. + + If `openapi_url` is set to `None`, this will be automatically disabled. + + Read more in the + [FastAPI docs for Metadata and Docs URLs](https://fastapi.tiangolo.com/tutorial/metadata/#docs-urls). + + **Example** + + ```python + from fastapi import FastAPI + + app = FastAPI(docs_url="/documentation", scalar_url="scalar-docs") + ``` + """ + ), + ] = "/scalar", swagger_ui_oauth2_redirect_url: Annotated[ Optional[str], Doc( @@ -833,6 +858,7 @@ class FastAPI(Starlette): self.root_path_in_servers = root_path_in_servers self.docs_url = docs_url self.redoc_url = redoc_url + self.scalar_url = scalar_url self.swagger_ui_oauth2_redirect_url = swagger_ui_oauth2_redirect_url self.swagger_ui_init_oauth = swagger_ui_init_oauth self.swagger_ui_parameters = swagger_ui_parameters @@ -1047,6 +1073,17 @@ class FastAPI(Starlette): ) self.add_route(self.redoc_url, redoc_html, include_in_schema=False) + + if self.openapi_url and self.scalar_url: + + async def scalar_html(req: Request) -> HTMLResponse: + root_path = req.scope.get("root_path", "").rstrip("/") + openapi_url = root_path + self.openapi_url + return get_scalar_html( + openapi_url=openapi_url, title=self.title + " - Scalar" + ) + + self.add_route(self.scalar_url, scalar_html, include_in_schema=False) async def __call__(self, scope: Scope, receive: Receive, send: Send) -> None: if self.root_path: diff --git a/fastapi/openapi/docs.py b/fastapi/openapi/docs.py index 69473d19c..c5f1454db 100644 --- a/fastapi/openapi/docs.py +++ b/fastapi/openapi/docs.py @@ -157,6 +157,95 @@ def get_swagger_ui_html( """ return HTMLResponse(html) +def get_scalar_html( + *, + openapi_url: Annotated[ + str, + Doc( + """ + The OpenAPI URL that Scalar should load and use. + + This is normally done automatically by FastAPI using the default URL + `/openapi.json`. + """ + ), + ], + title: Annotated[ + str, + Doc( + """ + The HTML `