+
+#### Generate Client Code
+
+To generate the client code you can use the command line application `openapi` that would now be installed.
+
+Because it is installed in the local project, you probably wouldn't be able to call that command directly, but you would put it on your `package.json` file.
+
+It could look like this:
+
+```JSON hl_lines="7"
+{
+ "name": "frontend-app",
+ "version": "1.0.0",
+ "description": "",
+ "main": "index.js",
+ "scripts": {
+ "generate-client": "openapi --input http://localhost:8000/openapi.json --output ./src/client --client axios"
+ },
+ "author": "",
+ "license": "",
+ "devDependencies": {
+ "openapi-typescript-codegen": "^0.20.1",
+ "typescript": "^4.6.2"
+ }
+}
+```
+
+After having that NPM `generate-client` script there, you can run it with:
+
+
+
+That command will generate code in `./src/client` and will use `axios` (the frontend HTTP library) internally.
+
+### Try Out the Client Code
+
+Now you can import and use the client code, it could look like this, notice that you get autocompletion for the methods:
+
+
+
+You will also get autocompletion for the payload to send:
+
+
+
+!!! tip
+ Notice the autocompletion for `name` and `price`, that was defined in the FastAPI application, in the `Item` model.
+
+You will have inline errors for the data that you send:
+
+
+
+The response object will also have autocompletion:
+
+
+
+## FastAPI App with Tags
+
+In many cases your FastAPI app will be bigger, and you will probably use tags to separate different groups of *path operations*.
+
+For example, you could have a section for **items** and another section for **users**, and they could be separated by tags:
+
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="23 28 36"
+ {!> ../../../docs_src/generate_clients/tutorial002.py!}
+ ```
+
+=== "Python 3.9 and above"
+
+ ```Python hl_lines="21 26 34"
+ {!> ../../../docs_src/generate_clients/tutorial002_py39.py!}
+ ```
+
+### Generate a TypeScript Client with Tags
+
+If you generate a client for a FastAPI app using tags, it will normally also separate the client code based on the tags.
+
+This way you will be able to have things ordered and grouped correctly for the client code:
+
+
+
+In this case you have:
+
+* `ItemsService`
+* `UsersService`
+
+### Client Method Names
+
+Right now the generated method names like `createItemItemsPost` don't look very clean:
+
+```TypeScript
+ItemsService.createItemItemsPost({name: "Plumbus", price: 5})
+```
+
+...that's because the client generator uses the OpenAPI internal **operation ID** for each *path operation*.
+
+OpenAPI requires that each operation ID is unique across all the *path operations*, so FastAPI uses the **function name**, the **path**, and the **HTTP method/operation** to generate that operation ID, because that way it can make sure that the operation IDs are unique.
+
+But I'll show you how to improve that next. 🤓
+
+## Custom Operation IDs and Better Method Names
+
+You can **modify** the way these operation IDs are **generated** to make them simpler and have **simpler method names** in the clients.
+
+In this case you will have to ensure that each operation ID is **unique** in some other way.
+
+For example, you could make sure that each *path operation* has a tag, and then generate the operation ID based on the **tag** and the *path operation* **name** (the function name).
+
+### Custom Generate Unique ID Function
+
+FastAPI uses a **unique ID** for each *path operation*, it is used for the **operation ID** and also for the names of any needed custom models, for requests or responses.
+
+You can customize that function. It takes an `APIRoute` and outputs a string.
+
+For example, here it is using the first tag (you will probably have only one tag) and the *path operation* name (the function name).
+
+You can then pass that custom function to **FastAPI** as the `generate_unique_id_function` parameter:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="8-9 12"
+ {!> ../../../docs_src/generate_clients/tutorial003.py!}
+ ```
+
+=== "Python 3.9 and above"
+
+ ```Python hl_lines="6-7 10"
+ {!> ../../../docs_src/generate_clients/tutorial003_py39.py!}
+ ```
+
+### Generate a TypeScript Client with Custom Operation IDs
+
+Now if you generate the client again, you will see that it has the improved method names:
+
+
+
+As you see, the method names now have the tag and then the function name, now they don't include information from the URL path and the HTTP operation.
+
+### Preprocess the OpenAPI Specification for the Client Generator
+
+The generated code still has some **duplicated information**.
+
+We already know that this method is related to the **items** because that word is in the `ItemsService` (taken from the tag), but we still have the tag name prefixed in the method name too. 😕
+
+We will probably still want to keep it for OpenAPI in general, as that will ensure that the operation IDs are **unique**.
+
+But for the generated client we could **modify** the OpenAPI operation IDs right before generating the clients, just to make those method names nicer and **cleaner**.
+
+We could download the OpenAPI JSON to a file `openapi.json` and then we could **remove that prefixed tag** with a script like this:
+
+```Python
+{!../../../docs_src/generate_clients/tutorial004.py!}
+```
+
+With that, the operation IDs would be renamed from things like `items-get_items` to just `get_items`, that way the client generator can generate simpler method names.
+
+### Generate a TypeScript Client with the Preprocessed OpenAPI
+
+Now as the end result is in a file `openapi.json`, you would modify the `package.json` to use that local file, for example:
+
+```JSON hl_lines="7"
+{
+ "name": "frontend-app",
+ "version": "1.0.0",
+ "description": "",
+ "main": "index.js",
+ "scripts": {
+ "generate-client": "openapi --input ./openapi.json --output ./src/client --client axios"
+ },
+ "author": "",
+ "license": "",
+ "devDependencies": {
+ "openapi-typescript-codegen": "^0.20.1",
+ "typescript": "^4.6.2"
+ }
+}
+```
+
+After generating the new client, you would now have **clean method names**, with all the **autocompletion**, **inline errors**, etc:
+
+
+
+## Benefits
+
+When using the automatically generated clients you would **autocompletion** for:
+
+* Methods.
+* Request payloads in the body, query parameters, etc.
+* Response payloads.
+
+You would also have **inline errors** for everything.
+
+And whenever you update the backend code, and **regenerate** the frontend, it would have any new *path operations* available as methods, the old ones removed, and any other change would be reflected on the generated code. 🤓
+
+This also means that if something changed it will be **reflected** on the client code automatically. And if you **build** the client it will error out if you have any **mismatch** in the data used.
+
+So, you would **detect many errors** very early in the development cycle instead of having to wait for the errors to show up to your final users in production and then trying to debug where the problem is. ✨
diff --git a/docs/en/docs/advanced/openapi-callbacks.md b/docs/en/docs/advanced/openapi-callbacks.md
index 138c90dd7..656ddbb3f 100644
--- a/docs/en/docs/advanced/openapi-callbacks.md
+++ b/docs/en/docs/advanced/openapi-callbacks.md
@@ -31,7 +31,7 @@ It will have a *path operation* that will receive an `Invoice` body, and a query
This part is pretty normal, most of the code is probably already familiar to you:
-```Python hl_lines="10-14 37-54"
+```Python hl_lines="9-13 36-53"
{!../../../docs_src/openapi_callbacks/tutorial001.py!}
```
@@ -83,7 +83,7 @@ So we are going to use that same knowledge to document how the *external API* sh
First create a new `APIRouter` that will contain one or more callbacks.
-```Python hl_lines="5 26"
+```Python hl_lines="3 25"
{!../../../docs_src/openapi_callbacks/tutorial001.py!}
```
@@ -96,7 +96,7 @@ It should look just like a normal FastAPI *path operation*:
* It should probably have a declaration of the body it should receive, e.g. `body: InvoiceEvent`.
* And it could also have a declaration of the response it should return, e.g. `response_model=InvoiceEventReceived`.
-```Python hl_lines="17-19 22-23 29-33"
+```Python hl_lines="16-18 21-22 28-32"
{!../../../docs_src/openapi_callbacks/tutorial001.py!}
```
@@ -163,7 +163,7 @@ At this point you have the *callback path operation(s)* needed (the one(s) that
Now use the parameter `callbacks` in *your API's path operation decorator* to pass the attribute `.routes` (that's actually just a `list` of routes/*path operations*) from that callback router:
-```Python hl_lines="36"
+```Python hl_lines="35"
{!../../../docs_src/openapi_callbacks/tutorial001.py!}
```
diff --git a/docs/en/docs/advanced/security/http-basic-auth.md b/docs/en/docs/advanced/security/http-basic-auth.md
index 6c589cd9a..90c516808 100644
--- a/docs/en/docs/advanced/security/http-basic-auth.md
+++ b/docs/en/docs/advanced/security/http-basic-auth.md
@@ -34,13 +34,19 @@ Here's a more complete example.
Use a dependency to check if the username and password are correct.
-For this, use the Python standard module `secrets` to check the username and password:
+For this, use the Python standard module `secrets` to check the username and password.
-```Python hl_lines="1 11-13"
+`secrets.compare_digest()` needs to take `bytes` or a `str` that only contains ASCII characters (the ones in English), this means it wouldn't work with characters like `á`, as in `Sebastián`.
+
+To handle that, we first convert the `username` and `password` to `bytes` encoding them with UTF-8.
+
+Then we can use `secrets.compare_digest()` to ensure that `credentials.username` is `"stanleyjobson"`, and that `credentials.password` is `"swordfish"`.
+
+```Python hl_lines="1 11-21"
{!../../../docs_src/security/tutorial007.py!}
```
-This will ensure that `credentials.username` is `"stanleyjobson"`, and that `credentials.password` is `"swordfish"`. This would be similar to:
+This would be similar to:
```Python
if not (credentials.username == "stanleyjobson") or not (credentials.password == "swordfish"):
@@ -102,6 +108,6 @@ That way, using `secrets.compare_digest()` in your application code, it will be
After detecting that the credentials are incorrect, return an `HTTPException` with a status code 401 (the same returned when no credentials are provided) and add the header `WWW-Authenticate` to make the browser show the login prompt again:
-```Python hl_lines="15-19"
+```Python hl_lines="23-27"
{!../../../docs_src/security/tutorial007.py!}
```
diff --git a/docs/en/docs/advanced/security/oauth2-scopes.md b/docs/en/docs/advanced/security/oauth2-scopes.md
index 4bd9cfd04..be51325cd 100644
--- a/docs/en/docs/advanced/security/oauth2-scopes.md
+++ b/docs/en/docs/advanced/security/oauth2-scopes.md
@@ -16,11 +16,11 @@ In this section you will see how to manage authentication and authorization with
You don't necessarily need OAuth2 scopes, and you can handle authentication and authorization however you want.
But OAuth2 with scopes can be nicely integrated into your API (with OpenAPI) and your API docs.
-
+
Nevertheless, you still enforce those scopes, or any other security/authorization requirement, however you need, in your code.
In many cases, OAuth2 with scopes can be an overkill.
-
+
But if you know you need it, or you are curious, keep reading.
## OAuth2 scopes and OpenAPI
@@ -47,7 +47,7 @@ They are normally used to declare specific security permissions, for example:
In OAuth2 a "scope" is just a string that declares a specific permission required.
It doesn't matter if it has other characters like `:` or if it is a URL.
-
+
Those details are implementation specific.
For OAuth2 they are just strings.
@@ -115,7 +115,7 @@ In this case, it requires the scope `me` (it could require more than one scope).
!!! note
You don't necessarily need to add different scopes in different places.
-
+
We are doing it here to demonstrate how **FastAPI** handles scopes declared at different levels.
```Python hl_lines="4 139 166"
diff --git a/docs/en/docs/advanced/sql-databases-peewee.md b/docs/en/docs/advanced/sql-databases-peewee.md
index 48514d1e2..b4ea61367 100644
--- a/docs/en/docs/advanced/sql-databases-peewee.md
+++ b/docs/en/docs/advanced/sql-databases-peewee.md
@@ -182,7 +182,7 @@ Now let's check the file `sql_app/schemas.py`.
To avoid confusion between the Peewee *models* and the Pydantic *models*, we will have the file `models.py` with the Peewee models, and the file `schemas.py` with the Pydantic models.
These Pydantic models define more or less a "schema" (a valid data shape).
-
+
So this will help us avoiding confusion while using both.
### Create the Pydantic *models* / schemas
diff --git a/docs/en/docs/advanced/testing-dependencies.md b/docs/en/docs/advanced/testing-dependencies.md
index 79208e8dc..7bba82fb7 100644
--- a/docs/en/docs/advanced/testing-dependencies.md
+++ b/docs/en/docs/advanced/testing-dependencies.md
@@ -28,7 +28,7 @@ To override a dependency for testing, you put as a key the original dependency (
And then **FastAPI** will call that override instead of the original dependency.
-```Python hl_lines="26-27 30"
+```Python hl_lines="28-29 32"
{!../../../docs_src/dependency_testing/tutorial001.py!}
```
diff --git a/docs/en/docs/advanced/websockets.md b/docs/en/docs/advanced/websockets.md
index 878ad37dd..0e9bc5b06 100644
--- a/docs/en/docs/advanced/websockets.md
+++ b/docs/en/docs/advanced/websockets.md
@@ -2,6 +2,20 @@
You can use WebSockets with **FastAPI**.
+## Install `WebSockets`
+
+First you need to install `WebSockets`:
+
+
+
## WebSockets client
### In production
diff --git a/docs/en/docs/alternatives.md b/docs/en/docs/alternatives.md
index 28d0be8cc..68aa3150d 100644
--- a/docs/en/docs/alternatives.md
+++ b/docs/en/docs/alternatives.md
@@ -235,7 +235,7 @@ It was one of the first extremely fast Python frameworks based on `asyncio`. It
!!! check "Inspired **FastAPI** to"
Find a way to have a crazy performance.
-
+
That's why **FastAPI** is based on Starlette, as it is the fastest framework available (tested by third-party benchmarks).
### Falcon
@@ -333,7 +333,7 @@ Now APIStar is a set of tools to validate OpenAPI specifications, not a web fram
Exist.
The idea of declaring multiple things (data validation, serialization and documentation) with the same Python types, that at the same time provided great editor support, was something I considered a brilliant idea.
-
+
And after searching for a long time for a similar framework and testing many different alternatives, APIStar was the best option available.
Then APIStar stopped to exist as a server and Starlette was created, and was a new better foundation for such a system. That was the final inspiration to build **FastAPI**.
@@ -391,7 +391,7 @@ That's one of the main things that **FastAPI** adds on top, all based on Python
Handle all the core web parts. Adding features on top.
The class `FastAPI` itself inherits directly from the class `Starlette`.
-
+
So, anything that you can do with Starlette, you can do it directly with **FastAPI**, as it is basically Starlette on steroids.
### Uvicorn
diff --git a/docs/en/docs/async.md b/docs/en/docs/async.md
index 8194650fd..c14a2cbb7 100644
--- a/docs/en/docs/async.md
+++ b/docs/en/docs/async.md
@@ -26,7 +26,7 @@ async def read_results():
---
-If you are using a third party library that communicates with something (a database, an API, the file system, etc) and doesn't have support for using `await`, (this is currently the case for most database libraries), then declare your *path operation functions* as normally, with just `def`, like:
+If you are using a third party library that communicates with something (a database, an API, the file system, etc.) and doesn't have support for using `await`, (this is currently the case for most database libraries), then declare your *path operation functions* as normally, with just `def`, like:
```Python hl_lines="2"
@app.get('/')
@@ -45,7 +45,7 @@ If you just don't know, use normal `def`.
---
-**Note**: you can mix `def` and `async def` in your *path operation functions* as much as you need and define each one using the best option for you. FastAPI will do the right thing with them.
+**Note**: You can mix `def` and `async def` in your *path operation functions* as much as you need and define each one using the best option for you. FastAPI will do the right thing with them.
Anyway, in any of the cases above, FastAPI will still work asynchronously and be extremely fast.
@@ -102,87 +102,117 @@ To see the difference, imagine the following story about burgers:
### Concurrent Burgers
-
+You go with your crush to get fast food, you stand in line while the cashier takes the orders from the people in front of you. 😍
-You go with your crush 😍 to get fast food 🍔, you stand in line while the cashier 💁 takes the orders from the people in front of you.
+
-Then it's your turn, you place your order of 2 very fancy burgers 🍔 for your crush 😍 and you.
+Then it's your turn, you place your order of 2 very fancy burgers for your crush and you. 🍔🍔
-You pay 💸.
+
+
+The cashier says something to the cook in the kitchen so they know they have to prepare your burgers (even though they are currently preparing the ones for the previous clients).
+
+
+
+You pay. 💸
+
+The cashier gives you the number of your turn.
-The cashier 💁 says something to the cook in the kitchen 👨🍳 so they know they have to prepare your burgers 🍔 (even though they are currently preparing the ones for the previous clients).
+
-The cashier 💁 gives you the number of your turn.
+While you are waiting, you go with your crush and pick a table, you sit and talk with your crush for a long time (as your burgers are very fancy and take some time to prepare).
-While you are waiting, you go with your crush 😍 and pick a table, you sit and talk with your crush 😍 for a long time (as your burgers are very fancy and take some time to prepare ✨🍔✨).
+As you are sitting at the table with your crush, while you wait for the burgers, you can spend that time admiring how awesome, cute and smart your crush is ✨😍✨.
-As you are sitting on the table with your crush 😍, while you wait for the burgers 🍔, you can spend that time admiring how awesome, cute and smart your crush is ✨😍✨.
+
-While waiting and talking to your crush 😍, from time to time, you check the number displayed on the counter to see if it's your turn already.
+While waiting and talking to your crush, from time to time, you check the number displayed on the counter to see if it's your turn already.
-Then at some point, it finally is your turn. You go to the counter, get your burgers 🍔 and come back to the table.
+Then at some point, it finally is your turn. You go to the counter, get your burgers and come back to the table.
-You and your crush 😍 eat the burgers 🍔 and have a nice time ✨.
+
+
+You and your crush eat the burgers and have a nice time. ✨
+
+
+
+!!! info
+ Beautiful illustrations by Ketrina Thompson. 🎨
---
Imagine you are the computer / program 🤖 in that story.
-While you are at the line, you are just idle 😴, waiting for your turn, not doing anything very "productive". But the line is fast because the cashier 💁 is only taking the orders (not preparing them), so that's fine.
+While you are at the line, you are just idle 😴, waiting for your turn, not doing anything very "productive". But the line is fast because the cashier is only taking the orders (not preparing them), so that's fine.
-Then, when it's your turn, you do actual "productive" work 🤓, you process the menu, decide what you want, get your crush's 😍 choice, pay 💸, check that you give the correct bill or card, check that you are charged correctly, check that the order has the correct items, etc.
+Then, when it's your turn, you do actual "productive" work, you process the menu, decide what you want, get your crush's choice, pay, check that you give the correct bill or card, check that you are charged correctly, check that the order has the correct items, etc.
-But then, even though you still don't have your burgers 🍔, your work with the cashier 💁 is "on pause" ⏸, because you have to wait 🕙 for your burgers to be ready.
+But then, even though you still don't have your burgers, your work with the cashier is "on pause" ⏸, because you have to wait 🕙 for your burgers to be ready.
-But as you go away from the counter and sit on the table with a number for your turn, you can switch 🔀 your attention to your crush 😍, and "work" ⏯ 🤓 on that. Then you are again doing something very "productive" 🤓, as is flirting with your crush 😍.
+But as you go away from the counter and sit at the table with a number for your turn, you can switch 🔀 your attention to your crush, and "work" ⏯ 🤓 on that. Then you are again doing something very "productive" as is flirting with your crush 😍.
-Then the cashier 💁 says "I'm finished with doing the burgers" 🍔 by putting your number on the counter's display, but you don't jump like crazy immediately when the displayed number changes to your turn number. You know no one will steal your burgers 🍔 because you have the number of your turn, and they have theirs.
+Then the cashier 💁 says "I'm finished with doing the burgers" by putting your number on the counter's display, but you don't jump like crazy immediately when the displayed number changes to your turn number. You know no one will steal your burgers because you have the number of your turn, and they have theirs.
-So you wait for your crush 😍 to finish the story (finish the current work ⏯ / task being processed 🤓), smile gently and say that you are going for the burgers ⏸.
+So you wait for your crush to finish the story (finish the current work ⏯ / task being processed 🤓), smile gently and say that you are going for the burgers ⏸.
-Then you go to the counter 🔀, to the initial task that is now finished ⏯, pick the burgers 🍔, say thanks and take them to the table. That finishes that step / task of interaction with the counter ⏹. That in turn, creates a new task, of "eating burgers" 🔀 ⏯, but the previous one of "getting burgers" is finished ⏹.
+Then you go to the counter 🔀, to the initial task that is now finished ⏯, pick the burgers, say thanks and take them to the table. That finishes that step / task of interaction with the counter ⏹. That in turn, creates a new task, of "eating burgers" 🔀 ⏯, but the previous one of "getting burgers" is finished ⏹.
### Parallel Burgers
Now let's imagine these aren't "Concurrent Burgers", but "Parallel Burgers".
-You go with your crush 😍 to get parallel fast food 🍔.
+You go with your crush to get parallel fast food.
+
+You stand in line while several (let's say 8) cashiers that at the same time are cooks take the orders from the people in front of you.
-You stand in line while several (let's say 8) cashiers that at the same time are cooks 👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳 take the orders from the people in front of you.
+Everyone before you is waiting for their burgers to be ready before leaving the counter because each of the 8 cashiers goes and prepares the burger right away before getting the next order.
-Everyone before you is waiting 🕙 for their burgers 🍔 to be ready before leaving the counter because each of the 8 cashiers goes and prepares the burger right away before getting the next order.
+
-Then it's finally your turn, you place your order of 2 very fancy burgers 🍔 for your crush 😍 and you.
+Then it's finally your turn, you place your order of 2 very fancy burgers for your crush and you.
You pay 💸.
-The cashier goes to the kitchen 👨🍳.
+
-You wait, standing in front of the counter 🕙, so that no one else takes your burgers 🍔 before you do, as there are no numbers for turns.
+The cashier goes to the kitchen.
-As you and your crush 😍 are busy not letting anyone get in front of you and take your burgers whenever they arrive 🕙, you cannot pay attention to your crush 😞.
+You wait, standing in front of the counter 🕙, so that no one else takes your burgers before you do, as there are no numbers for turns.
-This is "synchronous" work, you are "synchronized" with the cashier/cook 👨🍳. You have to wait 🕙 and be there at the exact moment that the cashier/cook 👨🍳 finishes the burgers 🍔 and gives them to you, or otherwise, someone else might take them.
+
-Then your cashier/cook 👨🍳 finally comes back with your burgers 🍔, after a long time waiting 🕙 there in front of the counter.
+As you and your crush are busy not letting anyone get in front of you and take your burgers whenever they arrive, you cannot pay attention to your crush. 😞
-You take your burgers 🍔 and go to the table with your crush 😍.
+This is "synchronous" work, you are "synchronized" with the cashier/cook 👨🍳. You have to wait 🕙 and be there at the exact moment that the cashier/cook 👨🍳 finishes the burgers and gives them to you, or otherwise, someone else might take them.
-You just eat them, and you are done 🍔 ⏹.
+
-There was not much talk or flirting as most of the time was spent waiting 🕙 in front of the counter 😞.
+Then your cashier/cook 👨🍳 finally comes back with your burgers, after a long time waiting 🕙 there in front of the counter.
+
+
+
+You take your burgers and go to the table with your crush.
+
+You just eat them, and you are done. ⏹
+
+
+
+There was not much talk or flirting as most of the time was spent waiting 🕙 in front of the counter. 😞
+
+!!! info
+ Beautiful illustrations by Ketrina Thompson. 🎨
---
-In this scenario of the parallel burgers, you are a computer / program 🤖 with two processors (you and your crush 😍), both waiting 🕙 and dedicating their attention ⏯ to be "waiting on the counter" 🕙 for a long time.
+In this scenario of the parallel burgers, you are a computer / program 🤖 with two processors (you and your crush), both waiting 🕙 and dedicating their attention ⏯ to be "waiting on the counter" 🕙 for a long time.
-The fast food store has 8 processors (cashiers/cooks) 👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳. While the concurrent burgers store might have had only 2 (one cashier and one cook) 💁 👨🍳.
+The fast food store has 8 processors (cashiers/cooks). While the concurrent burgers store might have had only 2 (one cashier and one cook).
-But still, the final experience is not the best 😞.
+But still, the final experience is not the best. 😞
---
-This would be the parallel equivalent story for burgers 🍔.
+This would be the parallel equivalent story for burgers. 🍔
For a more "real life" example of this, imagine a bank.
@@ -208,11 +238,7 @@ This "waiting" 🕙 is measured in microseconds, but still, summing it all, it's
That's why it makes a lot of sense to use asynchronous ⏸🔀⏯ code for web APIs.
-Most of the existing popular Python frameworks (including Flask and Django) were created before the new asynchronous features in Python existed. So, the ways they can be deployed support parallel execution and an older form of asynchronous execution that is not as powerful as the new capabilities.
-
-Even though the main specification for asynchronous web Python (ASGI) was developed at Django, to add support for WebSockets.
-
-That kind of asynchronicity is what made NodeJS popular (even though NodeJS is not parallel) and that's the strength of Go as a programming language.
+This kind of asynchronicity is what made NodeJS popular (even though NodeJS is not parallel) and that's the strength of Go as a programming language.
And that's the same level of performance you get with **FastAPI**.
@@ -238,7 +264,7 @@ You could have turns as in the burgers example, first the living room, then the
It would take the same amount of time to finish with or without turns (concurrency) and you would have done the same amount of work.
-But in this case, if you could bring the 8 ex-cashier/cooks/now-cleaners 👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳, and each one of them (plus you) could take a zone of the house to clean it, you could do all the work in **parallel**, with the extra help, and finish much sooner.
+But in this case, if you could bring the 8 ex-cashier/cooks/now-cleaners, and each one of them (plus you) could take a zone of the house to clean it, you could do all the work in **parallel**, with the extra help, and finish much sooner.
In this scenario, each one of the cleaners (including you) would be a processor, doing their part of the job.
@@ -371,7 +397,7 @@ All that is what powers FastAPI (through Starlette) and what makes it have such
These are very technical details of how **FastAPI** works underneath.
- If you have quite some technical knowledge (co-routines, threads, blocking, etc) and are curious about how FastAPI handles `async def` vs normal `def`, go ahead.
+ If you have quite some technical knowledge (co-routines, threads, blocking, etc.) and are curious about how FastAPI handles `async def` vs normal `def`, go ahead.
### Path operation functions
diff --git a/docs/en/docs/contributing.md b/docs/en/docs/contributing.md
index 648c472fe..ca51c6e82 100644
--- a/docs/en/docs/contributing.md
+++ b/docs/en/docs/contributing.md
@@ -84,7 +84,17 @@ To check it worked, use:
If it shows the `pip` binary at `env/bin/pip` then it worked. 🎉
+Make sure you have the latest pip version on your virtual environment to avoid errors on the next steps:
+
!!! tip
Every time you install a new package with `pip` under that environment, activate the environment again.
diff --git a/docs/en/docs/css/custom.css b/docs/en/docs/css/custom.css
index 7d3503e49..066b51725 100644
--- a/docs/en/docs/css/custom.css
+++ b/docs/en/docs/css/custom.css
@@ -4,10 +4,21 @@
display: block;
}
+.termy {
+ /* For right to left languages */
+ direction: ltr;
+}
+
.termy [data-termynal] {
white-space: pre-wrap;
}
+a.external-link {
+ /* For right to left languages */
+ direction: ltr;
+ display: inline-block;
+}
+
a.external-link::after {
/* \00A0 is a non-breaking space
to make the mark be on the same line as the link
@@ -94,7 +105,7 @@ a.announce-link:hover {
.announce-wrapper .sponsor-badge {
display: block;
position: absolute;
- top: -5px;
+ top: -10px;
right: 0;
font-size: 0.5rem;
color: #999;
@@ -118,3 +129,18 @@ a.announce-link:hover {
.twitter {
color: #00acee;
}
+
+/* Right to left languages */
+code {
+ direction: ltr;
+ display: inline-block;
+}
+
+.md-content__inner h1 {
+ direction: ltr !important;
+}
+
+.illustration {
+ margin-top: 2em;
+ margin-bottom: 2em;
+}
diff --git a/docs/en/docs/deployment/docker.md b/docs/en/docs/deployment/docker.md
index 3f86efcce..8a542622e 100644
--- a/docs/en/docs/deployment/docker.md
+++ b/docs/en/docs/deployment/docker.md
@@ -142,7 +142,7 @@ Successfully installed fastapi pydantic uvicorn
* Create a `main.py` file with:
```Python
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -155,7 +155,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -350,7 +350,7 @@ If your FastAPI is a single file, for example, `main.py` without an `./app` dire
Then you would just have to change the corresponding paths to copy the file inside the `Dockerfile`:
```{ .dockerfile .annotate hl_lines="10 13" }
-FROM python:3.9
+FROM python:3.9
WORKDIR /code
diff --git a/docs/en/docs/deployment/manually.md b/docs/en/docs/deployment/manually.md
index 7fd1f4d4f..d6892b2c1 100644
--- a/docs/en/docs/deployment/manually.md
+++ b/docs/en/docs/deployment/manually.md
@@ -38,7 +38,7 @@ You can install an ASGI compatible server with:
!!! tip
By adding the `standard`, Uvicorn will install and use some recommended extra dependencies.
-
+
That including `uvloop`, the high-performance drop-in replacement for `asyncio`, that provides the big concurrency performance boost.
=== "Hypercorn"
@@ -59,7 +59,7 @@ You can install an ASGI compatible server with:
## Run the Server Program
-You can then your application the same way you have done in the tutorials, but without the `--reload` option, e.g.:
+You can then run your application the same way you have done in the tutorials, but without the `--reload` option, e.g.:
=== "Uvicorn"
@@ -89,7 +89,7 @@ You can then your application the same way you have done in the tutorials, but w
Remember to remove the `--reload` option if you were using it.
The `--reload` option consumes much more resources, is more unstable, etc.
-
+
It helps a lot during **development**, but you **shouldn't** use it in **production**.
## Hypercorn with Trio
diff --git a/docs/en/docs/features.md b/docs/en/docs/features.md
index 36f80783a..02bb3ac1f 100644
--- a/docs/en/docs/features.md
+++ b/docs/en/docs/features.md
@@ -190,11 +190,11 @@ With **FastAPI** you get all of **Pydantic**'s features (as FastAPI is based on
* Plays nicely with your **IDE/linter/brain**:
* Because pydantic data structures are just instances of classes you define; auto-completion, linting, mypy and your intuition should all work properly with your validated data.
* **Fast**:
- * in benchmarks Pydantic is faster than all other tested libraries.
+ * in benchmarks Pydantic is faster than all other tested libraries.
* Validate **complex structures**:
* Use of hierarchical Pydantic models, Python `typing`’s `List` and `Dict`, etc.
* And validators allow complex data schemas to be clearly and easily defined, checked and documented as JSON Schema.
* You can have deeply **nested JSON** objects and have them all validated and annotated.
-* **Extendible**:
+* **Extensible**:
* Pydantic allows custom data types to be defined or you can extend validation with methods on a model decorated with the validator decorator.
* 100% test coverage.
diff --git a/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-01.png b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-01.png
new file mode 100644
index 000000000..e0e77d3fc
Binary files /dev/null and b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-01.png differ
diff --git a/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-02.png b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-02.png
new file mode 100644
index 000000000..27f6e1271
Binary files /dev/null and b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-02.png differ
diff --git a/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-03.png b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-03.png
new file mode 100644
index 000000000..27472a8e0
Binary files /dev/null and b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-03.png differ
diff --git a/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-04.png b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-04.png
new file mode 100644
index 000000000..cf1d8dd45
Binary files /dev/null and b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-04.png differ
diff --git a/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-05.png b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-05.png
new file mode 100644
index 000000000..ab6e03669
Binary files /dev/null and b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-05.png differ
diff --git a/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-06.png b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-06.png
new file mode 100644
index 000000000..4bbf247c0
Binary files /dev/null and b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-06.png differ
diff --git a/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-07.png b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-07.png
new file mode 100644
index 000000000..7a0f4092d
Binary files /dev/null and b/docs/en/docs/img/async/concurrent-burgers/concurrent-burgers-07.png differ
diff --git a/docs/en/docs/img/async/parallel-burgers/parallel-burgers-01.png b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-01.png
new file mode 100644
index 000000000..92fc1a8a0
Binary files /dev/null and b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-01.png differ
diff --git a/docs/en/docs/img/async/parallel-burgers/parallel-burgers-02.png b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-02.png
new file mode 100644
index 000000000..9583b84dc
Binary files /dev/null and b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-02.png differ
diff --git a/docs/en/docs/img/async/parallel-burgers/parallel-burgers-03.png b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-03.png
new file mode 100644
index 000000000..bea9ff0d8
Binary files /dev/null and b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-03.png differ
diff --git a/docs/en/docs/img/async/parallel-burgers/parallel-burgers-04.png b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-04.png
new file mode 100644
index 000000000..b5c8a60bb
Binary files /dev/null and b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-04.png differ
diff --git a/docs/en/docs/img/async/parallel-burgers/parallel-burgers-05.png b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-05.png
new file mode 100644
index 000000000..45aca8e21
Binary files /dev/null and b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-05.png differ
diff --git a/docs/en/docs/img/async/parallel-burgers/parallel-burgers-06.png b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-06.png
new file mode 100644
index 000000000..c91c4b472
Binary files /dev/null and b/docs/en/docs/img/async/parallel-burgers/parallel-burgers-06.png differ
diff --git a/docs/en/docs/img/deployment/concepts/process-ram.drawio b/docs/en/docs/img/deployment/concepts/process-ram.drawio
index 51fc30ed3..b29c8a342 100644
--- a/docs/en/docs/img/deployment/concepts/process-ram.drawio
+++ b/docs/en/docs/img/deployment/concepts/process-ram.drawio
@@ -103,4 +103,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/concepts/process-ram.svg b/docs/en/docs/img/deployment/concepts/process-ram.svg
index cd086c36b..c1bf0d589 100644
--- a/docs/en/docs/img/deployment/concepts/process-ram.svg
+++ b/docs/en/docs/img/deployment/concepts/process-ram.svg
@@ -56,4 +56,4 @@
}
1 GBViewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/deployment/https/https.drawio b/docs/en/docs/img/deployment/https/https.drawio
index 31cfab96b..c4c8a3628 100644
--- a/docs/en/docs/img/deployment/https/https.drawio
+++ b/docs/en/docs/img/deployment/https/https.drawio
@@ -274,4 +274,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/https/https.svg b/docs/en/docs/img/deployment/https/https.svg
index e63345eba..69497518a 100644
--- a/docs/en/docs/img/deployment/https/https.svg
+++ b/docs/en/docs/img/deployment/https/https.svg
@@ -59,4 +59,4 @@
TLS HandshakeViewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/deployment/https/https03.drawio b/docs/en/docs/img/deployment/https/https03.drawio
index c5766086c..c178fd363 100644
--- a/docs/en/docs/img/deployment/https/https03.drawio
+++ b/docs/en/docs/img/deployment/https/https03.drawio
@@ -128,4 +128,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/https/https03.svg b/docs/en/docs/img/deployment/https/https03.svg
index e68e1c459..2badd1c7d 100644
--- a/docs/en/docs/img/deployment/https/https03.svg
+++ b/docs/en/docs/img/deployment/https/https03.svg
@@ -59,4 +59,4 @@
IP:...Viewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/deployment/https/https04.drawio b/docs/en/docs/img/deployment/https/https04.drawio
index ea357a6c1..78a6e919a 100644
--- a/docs/en/docs/img/deployment/https/https04.drawio
+++ b/docs/en/docs/img/deployment/https/https04.drawio
@@ -149,4 +149,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/https/https04.svg b/docs/en/docs/img/deployment/https/https04.svg
index 4c9b7999b..4513ac76b 100644
--- a/docs/en/docs/img/deployment/https/https04.svg
+++ b/docs/en/docs/img/deployment/https/https04.svg
@@ -59,4 +59,4 @@
IP:...Viewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/deployment/https/https05.drawio b/docs/en/docs/img/deployment/https/https05.drawio
index 9b8b7c6f7..236ecd841 100644
--- a/docs/en/docs/img/deployment/https/https05.drawio
+++ b/docs/en/docs/img/deployment/https/https05.drawio
@@ -163,4 +163,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/https/https05.svg b/docs/en/docs/img/deployment/https/https05.svg
index d11647b9b..ddcd2760a 100644
--- a/docs/en/docs/img/deployment/https/https05.svg
+++ b/docs/en/docs/img/deployment/https/https05.svg
@@ -59,4 +59,4 @@
IP:...Viewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/deployment/https/https06.drawio b/docs/en/docs/img/deployment/https/https06.drawio
index 5bb85813f..9dec13184 100644
--- a/docs/en/docs/img/deployment/https/https06.drawio
+++ b/docs/en/docs/img/deployment/https/https06.drawio
@@ -180,4 +180,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/https/https06.svg b/docs/en/docs/img/deployment/https/https06.svg
index 10e03b7c5..3695de40c 100644
--- a/docs/en/docs/img/deployment/https/https06.svg
+++ b/docs/en/docs/img/deployment/https/https06.svg
@@ -59,4 +59,4 @@
IP:...Viewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/deployment/https/https07.drawio b/docs/en/docs/img/deployment/https/https07.drawio
index 1ca994b22..aa8f4d6be 100644
--- a/docs/en/docs/img/deployment/https/https07.drawio
+++ b/docs/en/docs/img/deployment/https/https07.drawio
@@ -200,4 +200,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/https/https07.svg b/docs/en/docs/img/deployment/https/https07.svg
index e409d8871..551354cef 100644
--- a/docs/en/docs/img/deployment/https/https07.svg
+++ b/docs/en/docs/img/deployment/https/https07.svg
@@ -59,4 +59,4 @@
IP:...Viewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/deployment/https/https08.drawio b/docs/en/docs/img/deployment/https/https08.drawio
index 8a4f41056..794b192df 100644
--- a/docs/en/docs/img/deployment/https/https08.drawio
+++ b/docs/en/docs/img/deployment/https/https08.drawio
@@ -214,4 +214,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/deployment/https/https08.svg b/docs/en/docs/img/deployment/https/https08.svg
index 3047dd821..2d4680dcc 100644
--- a/docs/en/docs/img/deployment/https/https08.svg
+++ b/docs/en/docs/img/deployment/https/https08.svg
@@ -59,4 +59,4 @@
IP:...Viewer does not support full SVG 1.1
diff --git a/docs/en/docs/img/sponsors/budget-insight.svg b/docs/en/docs/img/sponsors/budget-insight.svg
new file mode 100644
index 000000000..d753727a1
--- /dev/null
+++ b/docs/en/docs/img/sponsors/budget-insight.svg
@@ -0,0 +1,22 @@
+
diff --git a/docs/en/docs/img/sponsors/classiq-banner.png b/docs/en/docs/img/sponsors/classiq-banner.png
new file mode 100644
index 000000000..8a0d6ac74
Binary files /dev/null and b/docs/en/docs/img/sponsors/classiq-banner.png differ
diff --git a/docs/en/docs/img/sponsors/classiq.png b/docs/en/docs/img/sponsors/classiq.png
new file mode 100644
index 000000000..189c6bbe8
Binary files /dev/null and b/docs/en/docs/img/sponsors/classiq.png differ
diff --git a/docs/en/docs/img/sponsors/docarray-top-banner.svg b/docs/en/docs/img/sponsors/docarray-top-banner.svg
new file mode 100644
index 000000000..b2eca3544
--- /dev/null
+++ b/docs/en/docs/img/sponsors/docarray-top-banner.svg
@@ -0,0 +1 @@
+
diff --git a/docs/en/docs/img/sponsors/docarray.svg b/docs/en/docs/img/sponsors/docarray.svg
new file mode 100644
index 000000000..f18df247e
--- /dev/null
+++ b/docs/en/docs/img/sponsors/docarray.svg
@@ -0,0 +1 @@
+
diff --git a/docs/en/docs/img/sponsors/doist-banner.svg b/docs/en/docs/img/sponsors/doist-banner.svg
new file mode 100644
index 000000000..3a4d9a295
--- /dev/null
+++ b/docs/en/docs/img/sponsors/doist-banner.svg
@@ -0,0 +1,46 @@
+
diff --git a/docs/en/docs/img/sponsors/doist.svg b/docs/en/docs/img/sponsors/doist.svg
new file mode 100644
index 000000000..b55855f06
--- /dev/null
+++ b/docs/en/docs/img/sponsors/doist.svg
@@ -0,0 +1,54 @@
+
diff --git a/docs/en/docs/img/sponsors/exoflare.png b/docs/en/docs/img/sponsors/exoflare.png
new file mode 100644
index 000000000..b5977d80b
Binary files /dev/null and b/docs/en/docs/img/sponsors/exoflare.png differ
diff --git a/docs/en/docs/img/sponsors/fastapi-course-bundle-banner.png b/docs/en/docs/img/sponsors/fastapi-course-bundle-banner.png
new file mode 100644
index 000000000..220d08638
Binary files /dev/null and b/docs/en/docs/img/sponsors/fastapi-course-bundle-banner.png differ
diff --git a/docs/en/docs/img/sponsors/imgwhale-banner.svg b/docs/en/docs/img/sponsors/imgwhale-banner.svg
new file mode 100644
index 000000000..db87cc4c9
--- /dev/null
+++ b/docs/en/docs/img/sponsors/imgwhale-banner.svg
@@ -0,0 +1,14 @@
+
diff --git a/docs/en/docs/img/sponsors/imgwhale.svg b/docs/en/docs/img/sponsors/imgwhale.svg
new file mode 100644
index 000000000..46aefd930
--- /dev/null
+++ b/docs/en/docs/img/sponsors/imgwhale.svg
@@ -0,0 +1,28 @@
+
diff --git a/docs/en/docs/img/sponsors/ines-course.jpg b/docs/en/docs/img/sponsors/ines-course.jpg
new file mode 100644
index 000000000..05158b387
Binary files /dev/null and b/docs/en/docs/img/sponsors/ines-course.jpg differ
diff --git a/docs/en/docs/img/sponsors/jina-ai-banner.png b/docs/en/docs/img/sponsors/jina-ai-banner.png
new file mode 100644
index 000000000..3ac6b44ad
Binary files /dev/null and b/docs/en/docs/img/sponsors/jina-ai-banner.png differ
diff --git a/docs/en/docs/img/sponsors/jina-ai.png b/docs/en/docs/img/sponsors/jina-ai.png
new file mode 100644
index 000000000..d6b0bfb4e
Binary files /dev/null and b/docs/en/docs/img/sponsors/jina-ai.png differ
diff --git a/docs/en/docs/img/sponsors/jina-top-banner.svg b/docs/en/docs/img/sponsors/jina-top-banner.svg
new file mode 100644
index 000000000..8b62cd619
--- /dev/null
+++ b/docs/en/docs/img/sponsors/jina-top-banner.svg
@@ -0,0 +1 @@
+
diff --git a/docs/en/docs/img/sponsors/jina2.svg b/docs/en/docs/img/sponsors/jina2.svg
new file mode 100644
index 000000000..6a48677ba
--- /dev/null
+++ b/docs/en/docs/img/sponsors/jina2.svg
@@ -0,0 +1 @@
+
diff --git a/docs/en/docs/img/sponsors/striveworks-banner.png b/docs/en/docs/img/sponsors/striveworks-banner.png
new file mode 100644
index 000000000..5206744b7
Binary files /dev/null and b/docs/en/docs/img/sponsors/striveworks-banner.png differ
diff --git a/docs/en/docs/img/sponsors/striveworks.png b/docs/en/docs/img/sponsors/striveworks.png
new file mode 100644
index 000000000..435ac536c
Binary files /dev/null and b/docs/en/docs/img/sponsors/striveworks.png differ
diff --git a/docs/en/docs/img/sponsors/striveworks2.png b/docs/en/docs/img/sponsors/striveworks2.png
new file mode 100644
index 000000000..bed9cb0a7
Binary files /dev/null and b/docs/en/docs/img/sponsors/striveworks2.png differ
diff --git a/docs/en/docs/img/sponsors/testdriven.svg b/docs/en/docs/img/sponsors/testdriven.svg
index 97741b9e0..6ba2daa3b 100644
--- a/docs/en/docs/img/sponsors/testdriven.svg
+++ b/docs/en/docs/img/sponsors/testdriven.svg
@@ -1 +1 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/tutorial/bigger-applications/package.drawio b/docs/en/docs/img/tutorial/bigger-applications/package.drawio
index 48f6e76fe..cab3de2ca 100644
--- a/docs/en/docs/img/tutorial/bigger-applications/package.drawio
+++ b/docs/en/docs/img/tutorial/bigger-applications/package.drawio
@@ -40,4 +40,4 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/tutorial/bigger-applications/package.svg b/docs/en/docs/img/tutorial/bigger-applications/package.svg
index a9cec926a..44da1dc30 100644
--- a/docs/en/docs/img/tutorial/bigger-applications/package.svg
+++ b/docs/en/docs/img/tutorial/bigger-applications/package.svg
@@ -1 +1 @@
-
\ No newline at end of file
+
diff --git a/docs/en/docs/img/tutorial/generate-clients/image01.png b/docs/en/docs/img/tutorial/generate-clients/image01.png
new file mode 100644
index 000000000..f23d57773
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image01.png differ
diff --git a/docs/en/docs/img/tutorial/generate-clients/image02.png b/docs/en/docs/img/tutorial/generate-clients/image02.png
new file mode 100644
index 000000000..f991352eb
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image02.png differ
diff --git a/docs/en/docs/img/tutorial/generate-clients/image03.png b/docs/en/docs/img/tutorial/generate-clients/image03.png
new file mode 100644
index 000000000..e2514b048
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image03.png differ
diff --git a/docs/en/docs/img/tutorial/generate-clients/image04.png b/docs/en/docs/img/tutorial/generate-clients/image04.png
new file mode 100644
index 000000000..777a695bb
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image04.png differ
diff --git a/docs/en/docs/img/tutorial/generate-clients/image05.png b/docs/en/docs/img/tutorial/generate-clients/image05.png
new file mode 100644
index 000000000..e1e53179d
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image05.png differ
diff --git a/docs/en/docs/img/tutorial/generate-clients/image06.png b/docs/en/docs/img/tutorial/generate-clients/image06.png
new file mode 100644
index 000000000..0e9a100ea
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image06.png differ
diff --git a/docs/en/docs/img/tutorial/generate-clients/image07.png b/docs/en/docs/img/tutorial/generate-clients/image07.png
new file mode 100644
index 000000000..27884960f
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image07.png differ
diff --git a/docs/en/docs/img/tutorial/generate-clients/image08.png b/docs/en/docs/img/tutorial/generate-clients/image08.png
new file mode 100644
index 000000000..509fbd340
Binary files /dev/null and b/docs/en/docs/img/tutorial/generate-clients/image08.png differ
diff --git a/docs/en/docs/index.md b/docs/en/docs/index.md
index 7de1e50df..24ce14e23 100644
--- a/docs/en/docs/index.md
+++ b/docs/en/docs/index.md
@@ -32,7 +32,6 @@ FastAPI is a modern, fast (high-performance), web framework for building APIs wi
The key features are:
* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance).
-
* **Fast to code**: Increase the speed to develop features by about 200% to 300%. *
* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. *
* **Intuitive**: Great editor support. Completion everywhere. Less time debugging.
@@ -148,7 +147,7 @@ $ pip install "uvicorn[standard]"
* Create a file `main.py` with:
```Python
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -161,7 +160,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -171,7 +170,7 @@ def read_item(item_id: int, q: Optional[str] = None):
If your code uses `async` / `await`, use `async def`:
```Python hl_lines="9 14"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -184,7 +183,7 @@ async def read_root():
@app.get("/items/{item_id}")
-async def read_item(item_id: int, q: Optional[str] = None):
+async def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -263,7 +262,7 @@ Now modify the file `main.py` to receive a body from a `PUT` request.
Declare the body using standard Python types, thanks to Pydantic.
```Python hl_lines="4 9-12 25-27"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
from pydantic import BaseModel
@@ -274,7 +273,7 @@ app = FastAPI()
class Item(BaseModel):
name: str
price: float
- is_offer: Optional[bool] = None
+ is_offer: Union[bool, None] = None
@app.get("/")
@@ -283,7 +282,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
diff --git a/docs/en/docs/js/termynal.js b/docs/en/docs/js/termynal.js
index 8b0e9339e..4ac32708a 100644
--- a/docs/en/docs/js/termynal.js
+++ b/docs/en/docs/js/termynal.js
@@ -72,14 +72,14 @@ class Termynal {
* Initialise the widget, get lines, clear container and start animation.
*/
init() {
- /**
+ /**
* Calculates width and height of Termynal container.
* If container is empty and lines are dynamically loaded, defaults to browser `auto` or CSS.
- */
+ */
const containerStyle = getComputedStyle(this.container);
- this.container.style.width = containerStyle.width !== '0px' ?
+ this.container.style.width = containerStyle.width !== '0px' ?
containerStyle.width : undefined;
- this.container.style.minHeight = containerStyle.height !== '0px' ?
+ this.container.style.minHeight = containerStyle.height !== '0px' ?
containerStyle.height : undefined;
this.container.setAttribute('data-termynal', '');
@@ -138,7 +138,7 @@ class Termynal {
restart.innerHTML = "restart ↻"
return restart
}
-
+
generateFinish() {
const finish = document.createElement('a')
finish.onclick = (e) => {
@@ -215,7 +215,7 @@ class Termynal {
/**
* Converts line data objects into line elements.
- *
+ *
* @param {Object[]} lineData - Dynamically loaded lines.
* @param {Object} line - Line data object.
* @returns {Element[]} - Array of line elements.
@@ -231,7 +231,7 @@ class Termynal {
/**
* Helper function for generating attributes string.
- *
+ *
* @param {Object} line - Line data object.
* @returns {string} - String of attributes.
*/
diff --git a/docs/en/docs/python-types.md b/docs/en/docs/python-types.md
index fe56dadec..3d22ee620 100644
--- a/docs/en/docs/python-types.md
+++ b/docs/en/docs/python-types.md
@@ -29,7 +29,7 @@ Calling this program outputs:
John Doe
```
-The function does the following:
+The function does the following:
* Takes a `first_name` and `last_name`.
* Converts the first letter of each one to upper case with `title()`.
@@ -158,7 +158,7 @@ The syntax using `typing` is **compatible** with all versions, from Python 3.6 t
As Python advances, **newer versions** come with improved support for these type annotations and in many cases you won't even need to import and use the `typing` module to declare the type annotations.
-If you can chose a more recent version of Python for your project, you will be able to take advantage of that extra simplicity. See some examples below.
+If you can choose a more recent version of Python for your project, you will be able to take advantage of that extra simplicity. See some examples below.
#### List
@@ -267,7 +267,7 @@ You can declare that a variable can be any of **several types**, for example, an
In Python 3.6 and above (including Python 3.10) you can use the `Union` type from `typing` and put inside the square brackets the possible types to accept.
-In Python 3.10 there's also an **alternative syntax** were you can put the possible types separated by a vertical bar (`|`).
+In Python 3.10 there's also an **alternative syntax** where you can put the possible types separated by a vertical bar (`|`).
=== "Python 3.6 and above"
@@ -317,6 +317,45 @@ This also means that in Python 3.10, you can use `Something | None`:
{!> ../../../docs_src/python_types/tutorial009_py310.py!}
```
+#### Using `Union` or `Optional`
+
+If you are using a Python version below 3.10, here's a tip from my very **subjective** point of view:
+
+* 🚨 Avoid using `Optional[SomeType]`
+* Instead ✨ **use `Union[SomeType, None]`** ✨.
+
+Both are equivalent and underneath they are the same, but I would recommend `Union` instead of `Optional` because the word "**optional**" would seem to imply that the value is optional, and it actually means "it can be `None`", even if it's not optional and is still required.
+
+I think `Union[SomeType, None]` is more explicit about what it means.
+
+It's just about the words and names. But those words can affect how you and your teammates think about the code.
+
+As an example, let's take this function:
+
+```Python hl_lines="1 4"
+{!../../../docs_src/python_types/tutorial009c.py!}
+```
+
+The parameter `name` is defined as `Optional[str]`, but it is **not optional**, you cannot call the function without the parameter:
+
+```Python
+say_hi() # Oh, no, this throws an error! 😱
+```
+
+The `name` parameter is **still required** (not *optional*) because it doesn't have a default value. Still, `name` accepts `None` as the value:
+
+```Python
+say_hi(name=None) # This works, None is valid 🎉
+```
+
+The good news is, once you are on Python 3.10 you won't have to worry about that, as you will be able to simply use `|` to define unions of types:
+
+```Python hl_lines="1 4"
+{!../../../docs_src/python_types/tutorial009c_py310.py!}
+```
+
+And then you won't have to worry about names like `Optional` and `Union`. 😎
+
#### Generic types
These types that take type parameters in square brackets are called **Generic types** or **Generics**, for example:
@@ -333,28 +372,28 @@ These types that take type parameters in square brackets are called **Generic ty
=== "Python 3.9 and above"
- You can use the same builtin types as generics (with square brakets and types inside):
-
+ You can use the same builtin types as generics (with square brackets and types inside):
+
* `list`
* `tuple`
* `set`
* `dict`
And the same as with Python 3.6, from the `typing` module:
-
+
* `Union`
* `Optional`
* ...and others.
=== "Python 3.10 and above"
- You can use the same builtin types as generics (with square brakets and types inside):
+ You can use the same builtin types as generics (with square brackets and types inside):
* `list`
* `tuple`
* `set`
* `dict`
-
+
And the same as with Python 3.6, from the `typing` module:
* `Union`
@@ -422,6 +461,9 @@ An example from the official Pydantic docs:
You will see a lot more of all this in practice in the [Tutorial - User Guide](tutorial/index.md){.internal-link target=_blank}.
+!!! tip
+ Pydantic has a special behavior when you use `Optional` or `Union[Something, None]` without a default value, you can read more about it in the Pydantic docs about Required Optional fields.
+
## Type hints in **FastAPI**
**FastAPI** takes advantage of these type hints to do several things.
diff --git a/docs/en/docs/release-notes.md b/docs/en/docs/release-notes.md
index 6d5ee8ea1..8acf8d004 100644
--- a/docs/en/docs/release-notes.md
+++ b/docs/en/docs/release-notes.md
@@ -2,7 +2,522 @@
## Latest Changes
+* 🐛 Make sure a parameter defined as required is kept required in OpenAPI even if defined as optional in another dependency. PR [#4319](https://github.com/tiangolo/fastapi/pull/4319) by [@cd17822](https://github.com/cd17822).
+* ♻ Internal small refactor, move `operation_id` parameter position in delete method for consistency with the code. PR [#4474](https://github.com/tiangolo/fastapi/pull/4474) by [@hiel](https://github.com/hiel).
+* ✨ Support Python internal description on Pydantic model's docstring. PR [#3032](https://github.com/tiangolo/fastapi/pull/3032) by [@Kludex](https://github.com/Kludex).
+* ✨ Update `ORJSONResponse` to support non `str` keys and serializing Numpy arrays. PR [#3892](https://github.com/tiangolo/fastapi/pull/3892) by [@baby5](https://github.com/baby5).
+* 🔧 Update sponsors, disable ImgWhale. PR [#5338](https://github.com/tiangolo/fastapi/pull/5338) by [@tiangolo](https://github.com/tiangolo).
+* 📝 Update docs for `ORJSONResponse` with details about improving performance. PR [#2615](https://github.com/tiangolo/fastapi/pull/2615) by [@falkben](https://github.com/falkben).
+* 📝 Add docs for creating a custom Response class. PR [#5331](https://github.com/tiangolo/fastapi/pull/5331) by [@tiangolo](https://github.com/tiangolo).
+* 📝 Add tip about using alias for form data fields. PR [#5329](https://github.com/tiangolo/fastapi/pull/5329) by [@tiangolo](https://github.com/tiangolo).
+* 🐛 Fix support for path parameters in WebSockets. PR [#3879](https://github.com/tiangolo/fastapi/pull/3879) by [@davidbrochart](https://github.com/davidbrochart).
+
+## 0.81.0
+
+### Features
+
+* ✨ Add ReDoc ` automatique.
## Validation de données
@@ -91,7 +91,7 @@ documentation générée automatiquement et interactive :
On voit bien dans la documentation que `item_id` est déclaré comme entier.
-## Les avantages d'avoir une documentation basée sur une norme, et la documentation alternative.
+## Les avantages d'avoir une documentation basée sur une norme, et la documentation alternative.
Le schéma généré suivant la norme OpenAPI,
il existe de nombreux outils compatibles.
@@ -102,7 +102,7 @@ sur
De la même façon, il existe bien d'autres outils compatibles, y compris des outils de génération de code
-pour de nombreux langages.
+pour de nombreux langages.
## Pydantic
diff --git a/docs/fr/docs/tutorial/query-params.md b/docs/fr/docs/tutorial/query-params.md
index f1f2a605d..7bf3b9e79 100644
--- a/docs/fr/docs/tutorial/query-params.md
+++ b/docs/fr/docs/tutorial/query-params.md
@@ -6,7 +6,7 @@ Quand vous déclarez des paramètres dans votre fonction de chemin qui ne font p
{!../../../docs_src/query_params/tutorial001.py!}
```
-La partie appelée requête (ou **query**) dans une URL est l'ensemble des paires clés-valeurs placées après le `?` , séparées par des `&`.
+La partie appelée requête (ou **query**) dans une URL est l'ensemble des paires clés-valeurs placées après le `?` , séparées par des `&`.
Par exemple, dans l'URL :
@@ -120,7 +120,7 @@ ou n'importe quelle autre variation de casse (tout en majuscules, uniquement la
## Multiples paramètres de chemin et de requête
-Vous pouvez déclarer plusieurs paramètres de chemin et paramètres de requête dans la même fonction, **FastAPI** saura comment les gérer.
+Vous pouvez déclarer plusieurs paramètres de chemin et paramètres de requête dans la même fonction, **FastAPI** saura comment les gérer.
Et vous n'avez pas besoin de les déclarer dans un ordre spécifique.
diff --git a/docs/fr/mkdocs.yml b/docs/fr/mkdocs.yml
index ff16e1d78..6bed7be73 100644
--- a/docs/fr/mkdocs.yml
+++ b/docs/fr/mkdocs.yml
@@ -5,13 +5,15 @@ theme:
name: material
custom_dir: overrides
palette:
- - scheme: default
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
primary: teal
accent: amber
toggle:
icon: material/lightbulb
name: Switch to light mode
- - scheme: slate
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
primary: teal
accent: amber
toggle:
@@ -40,15 +42,19 @@ nav:
- az: /az/
- de: /de/
- es: /es/
+ - fa: /fa/
- fr: /fr/
+ - he: /he/
- id: /id/
- it: /it/
- ja: /ja/
- ko: /ko/
+ - nl: /nl/
- pl: /pl/
- pt: /pt/
- ru: /ru/
- sq: /sq/
+ - sv: /sv/
- tr: /tr/
- uk: /uk/
- zh: /zh/
@@ -63,9 +69,11 @@ nav:
- tutorial/background-tasks.md
- async.md
- Déploiement:
+ - deployment/index.md
- deployment/docker.md
- project-generation.md
- alternatives.md
+- history-design-future.md
- external-links.md
markdown_extensions:
- toc:
@@ -84,6 +92,8 @@ markdown_extensions:
format: !!python/name:pymdownx.superfences.fence_code_format ''
- pymdownx.tabbed:
alternate_style: true
+- attr_list
+- md_in_html
extra:
analytics:
provider: google
@@ -112,8 +122,12 @@ extra:
name: de
- link: /es/
name: es - español
+ - link: /fa/
+ name: fa
- link: /fr/
name: fr - français
+ - link: /he/
+ name: he
- link: /id/
name: id
- link: /it/
@@ -122,6 +136,8 @@ extra:
name: ja - 日本語
- link: /ko/
name: ko - 한국어
+ - link: /nl/
+ name: nl
- link: /pl/
name: pl
- link: /pt/
@@ -130,6 +146,8 @@ extra:
name: ru - русский язык
- link: /sq/
name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
- link: /tr/
name: tr - Türkçe
- link: /uk/
diff --git a/docs/he/docs/index.md b/docs/he/docs/index.md
new file mode 100644
index 000000000..fa63d8cb7
--- /dev/null
+++ b/docs/he/docs/index.md
@@ -0,0 +1,464 @@
+
+
+---
+
+**תיעוד**: https://fastapi.tiangolo.com
+
+**קוד**: https://github.com/tiangolo/fastapi
+
+---
+
+FastAPI היא תשתית רשת מודרנית ומהירה (ביצועים גבוהים) לבניית ממשקי תכנות יישומים (API) עם פייתון 3.6+ בהתבסס על רמזי טיפוסים סטנדרטיים.
+
+תכונות המפתח הן:
+
+- **מהירה**: ביצועים גבוהים מאוד, בקנה אחד עם NodeJS ו - Go (תודות ל - Starlette ו - Pydantic). [אחת מתשתיות הפייתון המהירות ביותר](#performance).
+
+- **מהירה לתכנות**: הגבירו את מהירות פיתוח התכונות החדשות בכ - %200 עד %300. \*
+- **פחות שגיאות**: מנעו כ - %40 משגיאות אנוש (מפתחים). \*
+- **אינטואיטיבית**: תמיכת עורך מעולה. השלמה בכל מקום. פחות זמן ניפוי שגיאות.
+- **קלה**: מתוכננת להיות קלה לשימוש וללמידה. פחות זמן קריאת תיעוד.
+- **קצרה**: מזערו שכפול קוד. מספר תכונות מכל הכרזת פרמטר. פחות שגיאות.
+- **חסונה**: קבלו קוד מוכן לסביבת ייצור. עם תיעוד אינטרקטיבי אוטומטי.
+- **מבוססת סטנדרטים**: מבוססת על (ותואמת לחלוטין ל -) הסטדנרטים הפתוחים לממשקי תכנות יישומים: OpenAPI (ידועים לשעבר כ - Swagger) ו - JSON Schema.
+
+\* הערכה מבוססת על בדיקות של צוות פיתוח פנימי שבונה אפליקציות בסביבת ייצור.
+
+## נותני חסות
+
+
+
+{% if sponsors %}
+{% for sponsor in sponsors.gold -%}
+
+{% endfor -%}
+{%- for sponsor in sponsors.silver -%}
+
+{% endfor %}
+{% endif %}
+
+
+
+נותני חסות אחרים
+
+## דעות
+
+"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._"
+
+
+
+---
+
+"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_"
+
+
Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - Uber(ref)
+
+---
+
+"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_"
+
+
Kevin Glisson, Marc Vilanova, Forest Monsen - Netflix(ref)
+
+---
+
+"_I’m over the moon excited about **FastAPI**. It’s so fun!_"
+
+
+
+---
+
+"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._"
+
+
+
+---
+
+"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_"
+
+"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_"
+
+
+
+---
+
+## **Typer**, ה - FastAPI של ממשקי שורת פקודה (CLI).
+
+
+
+אם אתם בונים אפליקציית CLI לשימוש במסוף במקום ממשק רשת, העיפו מבט על **Typer**.
+
+**Typer** היא אחותה הקטנה של FastAPI. ומטרתה היא להיות ה - **FastAPI של ממשקי שורת פקודה**. ⌨️ 🚀
+
+## תלויות
+
+פייתון 3.6+
+
+FastAPI עומדת על כתפי ענקיות:
+
+- Starlette לחלקי הרשת.
+- Pydantic לחלקי המידע.
+
+## התקנה
+
+
+
+## דוגמא
+
+### צרו אותה
+
+- צרו קובץ בשם `main.py` עם:
+
+```Python
+from typing import Union
+
+from fastapi import FastAPI
+
+app = FastAPI()
+
+
+@app.get("/")
+def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+```
+
+
+או השתמשו ב - async def...
+
+אם הקוד שלכם משתמש ב - `async` / `await`, השתמשו ב - `async def`:
+
+```Python hl_lines="9 14"
+from typing import Union
+
+from fastapi import FastAPI
+
+app = FastAPI()
+
+
+@app.get("/")
+async def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+async def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+```
+
+**שימו לב**:
+
+אם אינכם יודעים, בדקו את פרק "ממהרים?" על `async` ו - `await` בתיעוד.
+
+
+
+### הריצו אותה
+
+התחילו את השרת עם:
+
+
+
+```console
+$ uvicorn main:app --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+INFO: Started reloader process [28720]
+INFO: Started server process [28722]
+INFO: Waiting for application startup.
+INFO: Application startup complete.
+```
+
+
+
+
+על הפקודה uvicorn main:app --reload...
+
+הפקודה `uvicorn main:app` מתייחסת ל:
+
+- `main`: הקובץ `main.py` (מודול פייתון).
+- `app`: האובייקט שנוצר בתוך `main.py` עם השורה app = FastAPI().
+- --reload: גרמו לשרת להתאתחל לאחר שינויים בקוד. עשו זאת רק בסביבת פיתוח.
+
+
+
+### בדקו אותה
+
+פתחו את הדפדפן שלכם בכתובת http://127.0.0.1:8000/items/5?q=somequery.
+
+אתם תראו תגובת JSON:
+
+```JSON
+{"item_id": 5, "q": "somequery"}
+```
+
+כבר יצרתם API ש:
+
+- מקבל בקשות HTTP בנתיבים `/` ו - /items/{item_id}.
+- שני ה _נתיבים_ מקבלים _בקשות_ `GET` (ידועות גם כ*מתודות* HTTP).
+- ה _נתיב_ /items/{item_id} כולל \*פרמטר נתיב\_ `item_id` שאמור להיות `int`.
+- ה _נתיב_ /items/{item_id} \*פרמטר שאילתא\_ אופציונלי `q`.
+
+### תיעוד API אינטרקטיבי
+
+כעת פנו לכתובת http://127.0.0.1:8000/docs.
+
+אתם תראו את התיעוד האוטומטי (מסופק על ידי Swagger UI):
+
+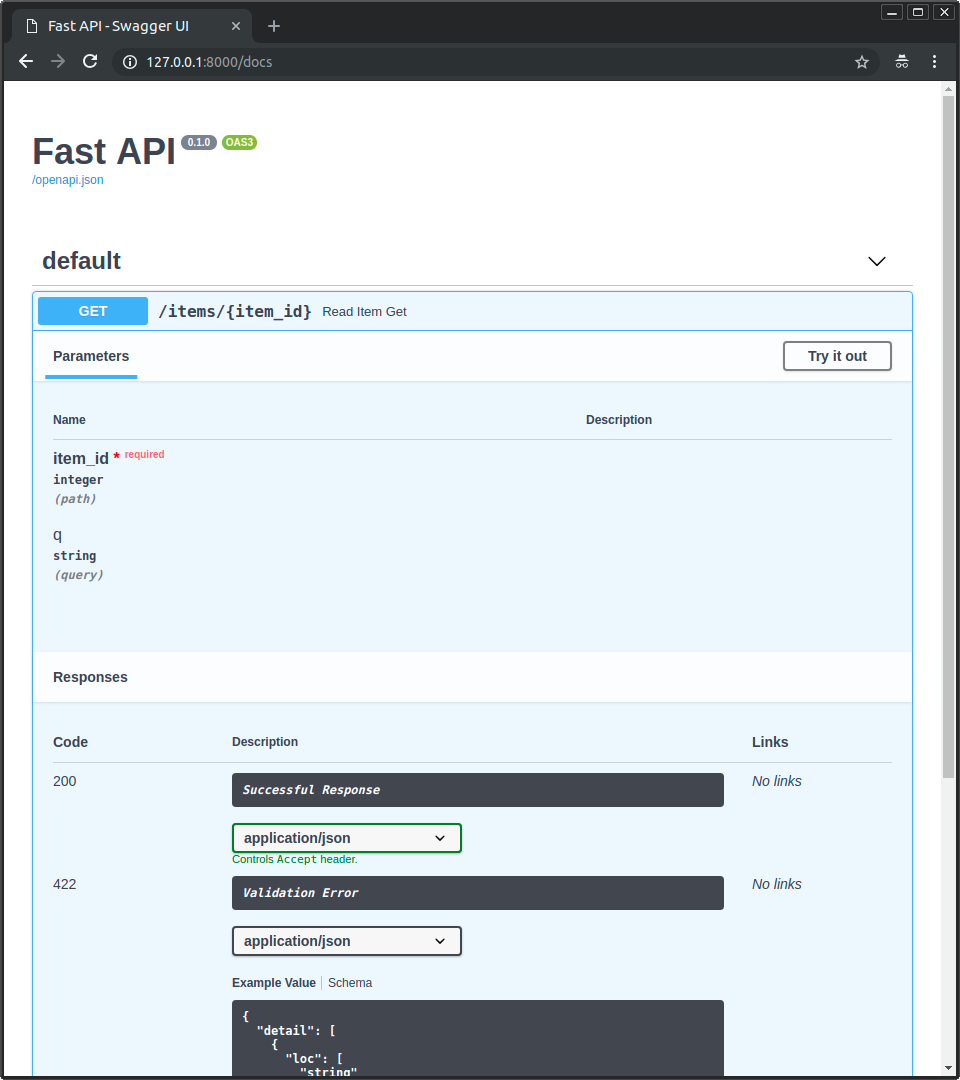
+
+### תיעוד אלטרנטיבי
+
+כעת פנו לכתובת http://127.0.0.1:8000/redoc.
+
+אתם תראו תיעוד אלטרנטיבי (מסופק על ידי ReDoc):
+
+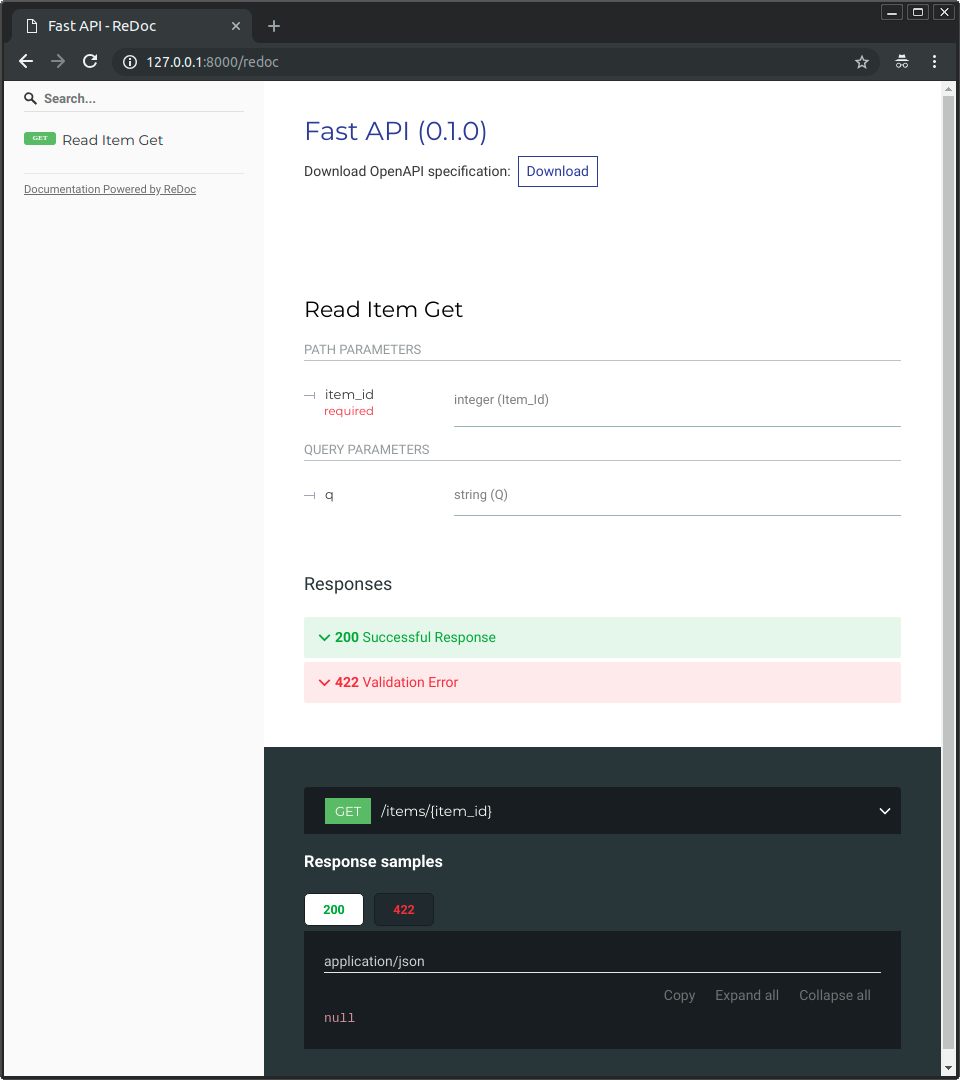
+
+## שדרוג לדוגמא
+
+כעת ערכו את הקובץ `main.py` כך שיוכל לקבל גוף מבקשת `PUT`.
+
+הגדירו את הגוף בעזרת רמזי טיפוסים סטנדרטיים, הודות ל - `Pydantic`.
+
+```Python hl_lines="4 9-12 25-27"
+from typing import Union
+
+from fastapi import FastAPI
+from pydantic import BaseModel
+
+app = FastAPI()
+
+
+class Item(BaseModel):
+ name: str
+ price: float
+ is_offer: Union[bool, None] = None
+
+
+@app.get("/")
+def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+
+
+@app.put("/items/{item_id}")
+def update_item(item_id: int, item: Item):
+ return {"item_name": item.name, "item_id": item_id}
+```
+
+השרת אמול להתאתחל אוטומטית (מאחר והוספתם --reload לפקודת `uvicorn` שלמעלה).
+
+### שדרוג התיעוד האינטרקטיבי
+
+כעת פנו לכתובת http://127.0.0.1:8000/docs.
+
+- התיעוד האוטומטי יתעדכן, כולל הגוף החדש:
+
+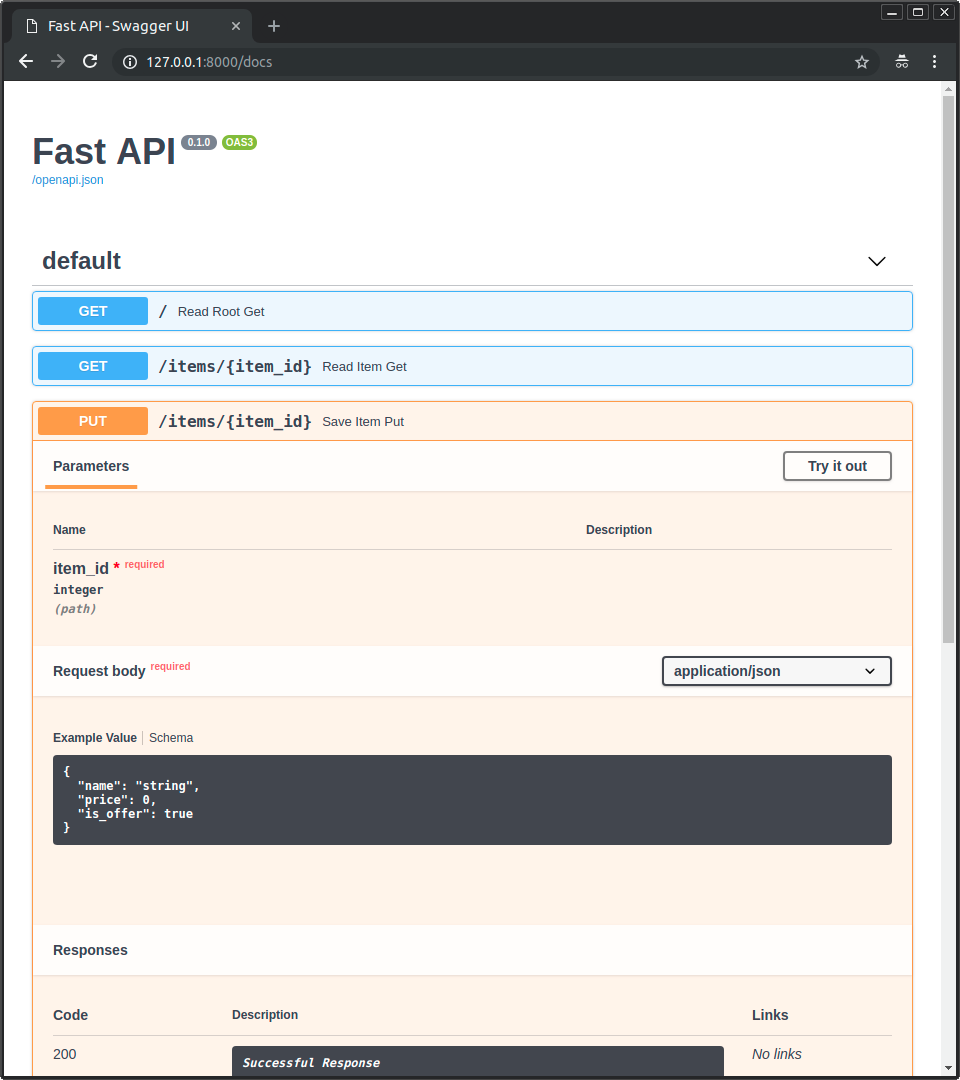
+
+- לחצו על הכפתור "Try it out", הוא יאפשר לכם למלא את הפרמטרים ולעבוד ישירות מול ה - API.
+
+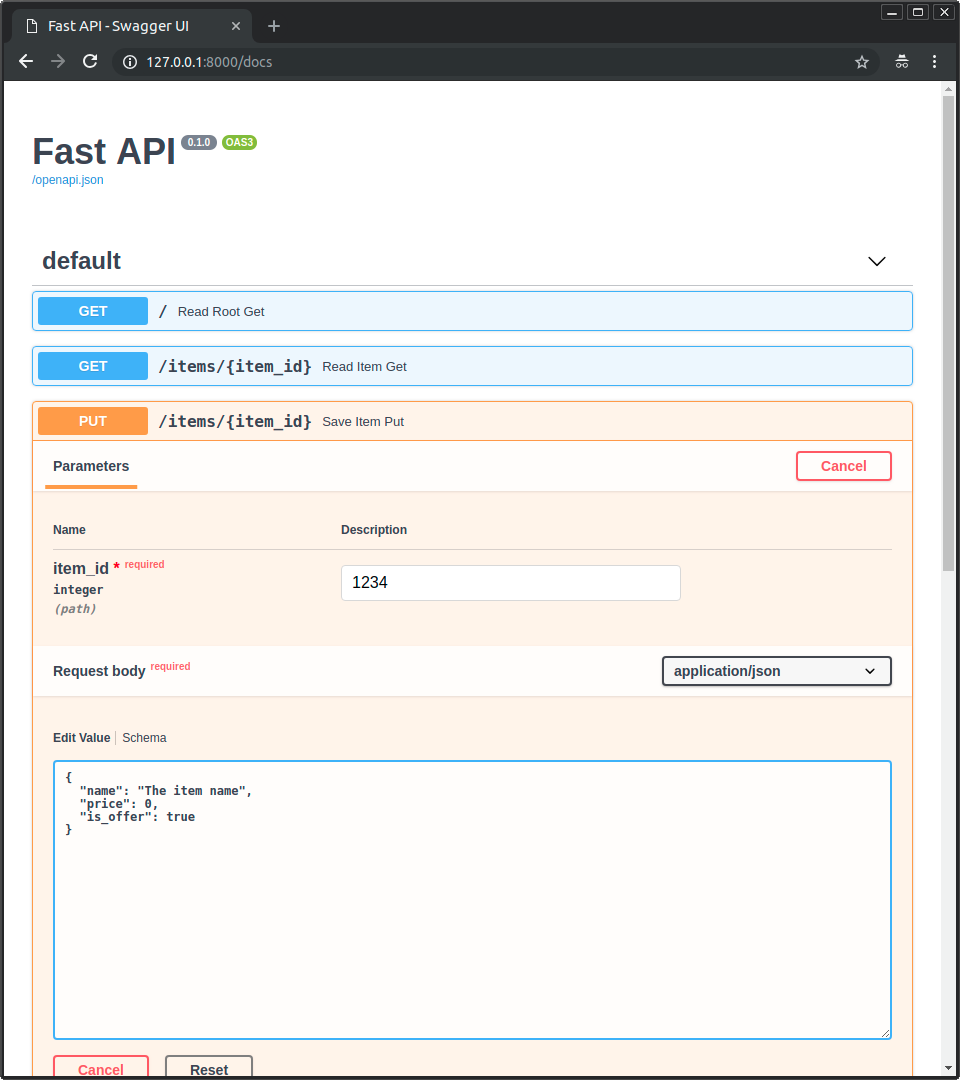
+
+- אחר כך לחצו על הכפתור "Execute", האתר יתקשר עם ה - API שלכם, ישלח את הפרמטרים, ישיג את התוצאות ואז יראה אותן על המסך:
+
+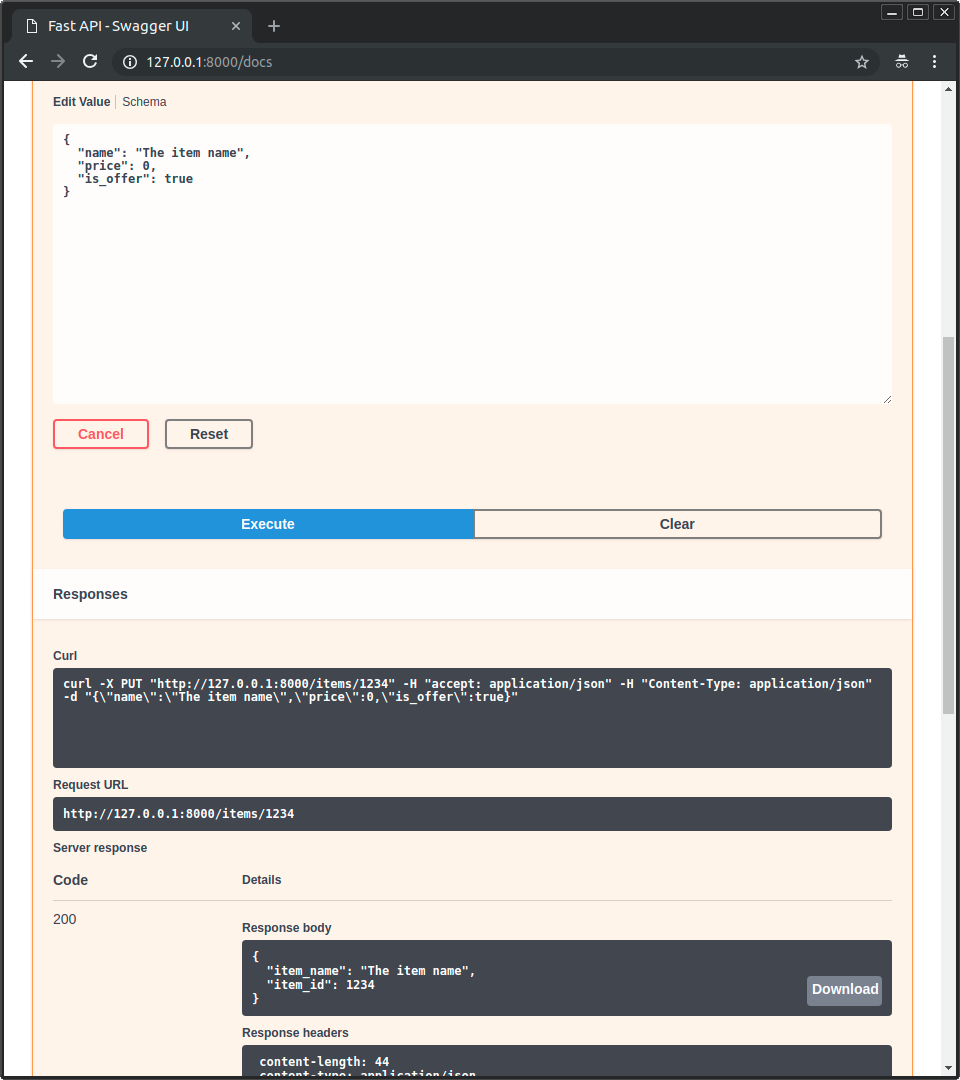
+
+### שדרוג התיעוד האלטרנטיבי
+
+כעת פנו לכתובת http://127.0.0.1:8000/redoc.
+
+- התיעוד האלטרנטיבי גם יראה את פרמטר השאילתא והגוף החדשים.
+
+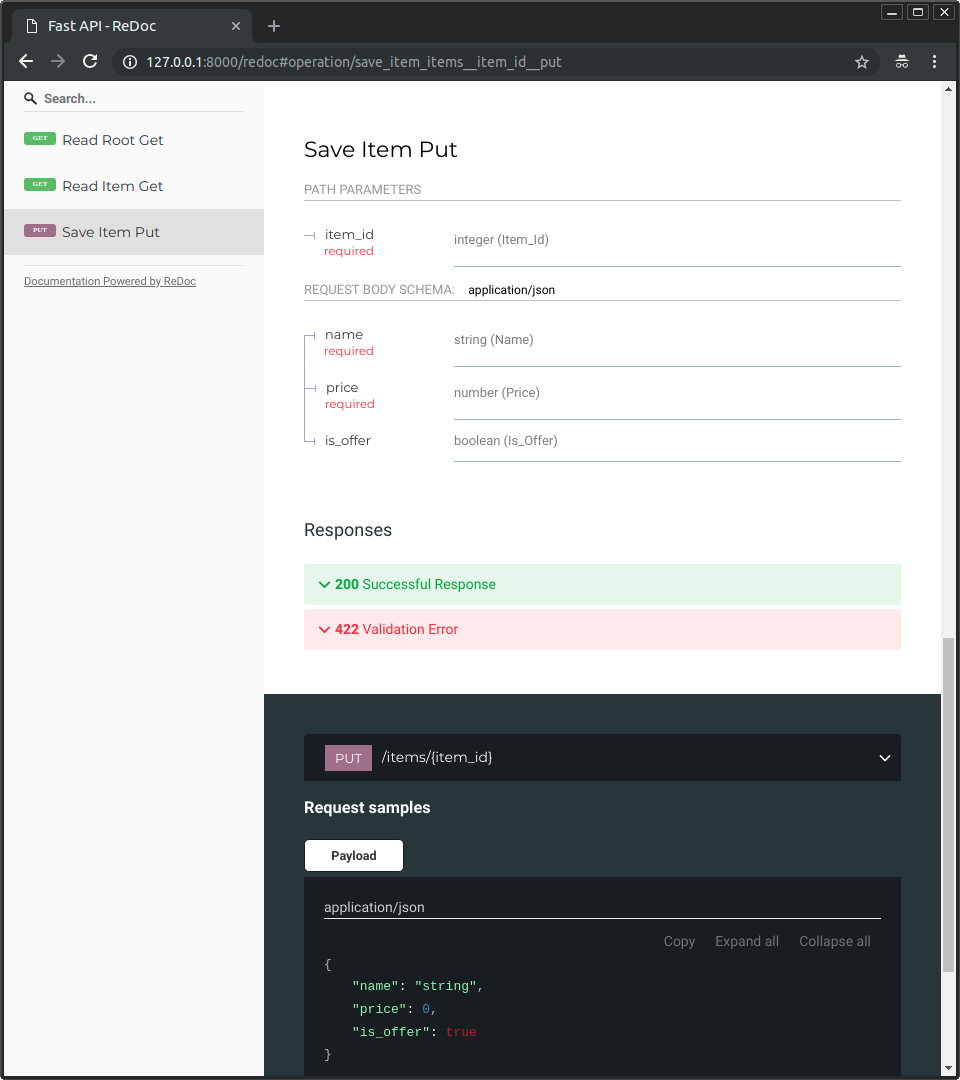
+
+### סיכום
+
+לסיכום, אתם מכריזים ** פעם אחת** על טיפוסי הפרמטרים, גוף וכו' כפרמטרים לפונקציה.
+
+אתם עושים את זה עם טיפוסי פייתון מודרניים.
+
+אתם לא צריכים ללמוד תחביר חדש, מתודות או מחלקות של ספרייה ספיציפית, וכו'
+
+רק **פייתון 3.6+** סטנדרטי.
+
+לדוגמא, ל - `int`:
+
+```Python
+item_id: int
+```
+
+או למודל `Item` מורכב יותר:
+
+```Python
+item: Item
+```
+
+...ועם הכרזת הטיפוס האחת הזו אתם מקבלים:
+
+- תמיכת עורך, כולל:
+ - השלמות.
+ - בדיקת טיפוסים.
+- אימות מידע:
+ - שגיאות ברורות ואטומטיות כאשר מוכנס מידע לא חוקי .
+ - אימות אפילו לאובייקטי JSON מקוננים.
+- המרה של מידע קלט: המרה של מידע שמגיע מהרשת למידע וטיפוסים של פייתון. קורא מ:
+ - JSON.
+ - פרמטרי נתיב.
+ - פרמטרי שאילתא.
+ - עוגיות.
+ - כותרות.
+ - טפסים.
+ - קבצים.
+- המרה של מידע פלט: המרה של מידע וטיפוסים מפייתון למידע רשת (כ - JSON):
+ - המירו טיפוסי פייתון (`str`, `int`, `float`, `bool`, `list`, etc).
+ - עצמי `datetime`.
+ - עצמי `UUID`.
+ - מודלי בסיסי נתונים.
+ - ...ורבים אחרים.
+- תיעוד API אוטומטי ואינטרקטיבית כולל שתי אלטרנטיבות לממשק המשתמש:
+ - Swagger UI.
+ - ReDoc.
+
+---
+
+בחזרה לדוגמאת הקוד הקודמת, **FastAPI** ידאג:
+
+- לאמת שיש `item_id` בנתיב בבקשות `GET` ו - `PUT`.
+- לאמת שה - `item_id` הוא מטיפוס `int` בבקשות `GET` ו - `PUT`.
+ - אם הוא לא, הלקוח יראה שגיאה ברורה ושימושית.
+- לבדוק האם קיים פרמטר שאילתא בשם `q` (קרי `http://127.0.0.1:8000/items/foo?q=somequery`) לבקשות `GET`.
+ - מאחר והפרמטר `q` מוגדר עם = None, הוא אופציונלי.
+ - לולא ה - `None` הוא היה חובה (כמו הגוף במקרה של `PUT`).
+- לבקשות `PUT` לנתיב /items/{item_id}, לקרוא את גוף הבקשה כ - JSON:
+ - לאמת שהוא כולל את מאפיין החובה `name` שאמור להיות מטיפוס `str`.
+ - לאמת שהוא כולל את מאפיין החובה `price` שחייב להיות מטיפוס `float`.
+ - לבדוק האם הוא כולל את מאפיין הרשות `is_offer` שאמור להיות מטיפוס `bool`, אם הוא נמצא.
+ - כל זה יעבוד גם לאובייקט JSON מקונן.
+- להמיר מ - JSON ול- JSON אוטומטית.
+- לתעד הכל באמצעות OpenAPI, תיעוד שבו יוכלו להשתמש:
+ - מערכות תיעוד אינטרקטיביות.
+ - מערכות ייצור קוד אוטומטיות, להרבה שפות.
+- לספק ישירות שתי מערכות תיעוד רשתיות.
+
+---
+
+רק גרדנו את קצה הקרחון, אבל כבר יש לכם רעיון של איך הכל עובד.
+
+נסו לשנות את השורה:
+
+```Python
+ return {"item_name": item.name, "item_id": item_id}
+```
+
+...מ:
+
+```Python
+ ... "item_name": item.name ...
+```
+
+...ל:
+
+```Python
+ ... "item_price": item.price ...
+```
+
+...וראו איך העורך שלכם משלים את המאפיינים ויודע את הטיפוסים שלהם:
+
+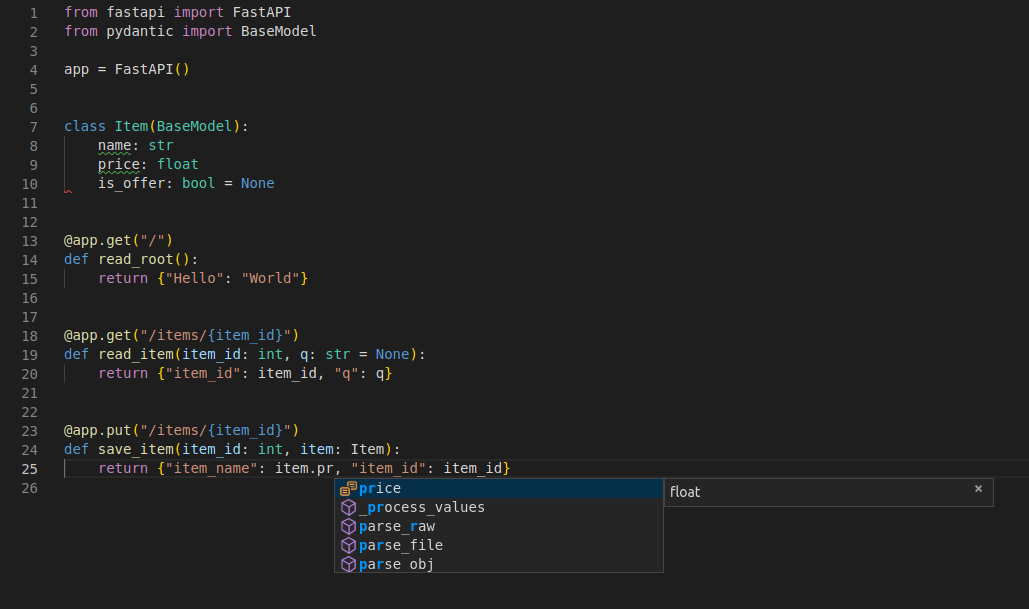
+
+לדוגמא יותר שלמה שכוללת עוד תכונות, ראו את המדריך - למשתמש.
+
+**התראת ספוילרים**: המדריך - למשתמש כולל:
+
+- הכרזה על **פרמטרים** ממקורות אחרים ושונים כגון: **כותרות**, **עוגיות**, **טפסים** ו - **קבצים**.
+- איך לקבוע **מגבלות אימות** בעזרת `maximum_length` או `regex`.
+- דרך חזקה וקלה להשתמש ב**הזרקת תלויות**.
+- אבטחה והתאמתות, כולל תמיכה ב - **OAuth2** עם **JWT** והתאמתות **HTTP Basic**.
+- טכניקות מתקדמות (אבל קלות באותה מידה) להכרזת אובייקטי JSON מקוננים (תודות ל - Pydantic).
+- אינטרקציה עם **GraphQL** דרך Strawberry וספריות אחרות.
+- תכונות נוספות רבות (תודות ל - Starlette) כגון:
+ - **WebSockets**
+ - בדיקות קלות במיוחד מבוססות על `requests` ו - `pytest`
+ - **CORS**
+ - **Cookie Sessions**
+ - ...ועוד.
+
+## ביצועים
+
+בדיקות עצמאיות של TechEmpower הראו שאפליקציות **FastAPI** שרצות תחת Uvicorn הן מתשתיות הפייתון המהירות ביותר, רק מתחת ל - Starlette ו - Uvicorn עצמן (ש - FastAPI מבוססת עליהן). (\*)
+
+כדי להבין עוד על הנושא, ראו את הפרק Benchmarks.
+
+## תלויות אופציונליות
+
+בשימוש Pydantic:
+
+- ujson - "פרסור" JSON.
+- email_validator - לאימות כתובות אימייל.
+
+בשימוש Starlette:
+
+- requests - דרוש אם ברצונכם להשתמש ב - `TestClient`.
+- jinja2 - דרוש אם ברצונכם להשתמש בברירת המחדל של תצורת הטמפלייטים.
+- python-multipart - דרוש אם ברצונכם לתמוך ב "פרסור" טפסים, באצמעות request.form().
+- itsdangerous - דרוש אם ברצונכם להשתמש ב - `SessionMiddleware`.
+- pyyaml - דרוש אם ברצונכם להשתמש ב - `SchemaGenerator` של Starlette (כנראה שאתם לא צריכים את זה עם FastAPI).
+- ujson - דרוש אם ברצונכם להשתמש ב - `UJSONResponse`.
+
+בשימוש FastAPI / Starlette:
+
+- uvicorn - לשרת שטוען ומגיש את האפליקציה שלכם.
+- orjson - דרוש אם ברצונכם להשתמש ב - `ORJSONResponse`.
+
+תוכלו להתקין את כל אלו באמצעות pip install "fastapi[all]".
+
+## רשיון
+
+הפרויקט הזה הוא תחת התנאים של רשיון MIT.
diff --git a/docs/he/mkdocs.yml b/docs/he/mkdocs.yml
new file mode 100644
index 000000000..3279099b5
--- /dev/null
+++ b/docs/he/mkdocs.yml
@@ -0,0 +1,145 @@
+site_name: FastAPI
+site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production
+site_url: https://fastapi.tiangolo.com/he/
+theme:
+ name: material
+ custom_dir: overrides
+ palette:
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
+ primary: teal
+ accent: amber
+ toggle:
+ icon: material/lightbulb
+ name: Switch to light mode
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
+ primary: teal
+ accent: amber
+ toggle:
+ icon: material/lightbulb-outline
+ name: Switch to dark mode
+ features:
+ - search.suggest
+ - search.highlight
+ - content.tabs.link
+ icon:
+ repo: fontawesome/brands/github-alt
+ logo: https://fastapi.tiangolo.com/img/icon-white.svg
+ favicon: https://fastapi.tiangolo.com/img/favicon.png
+ language: he
+repo_name: tiangolo/fastapi
+repo_url: https://github.com/tiangolo/fastapi
+edit_uri: ''
+plugins:
+- search
+- markdownextradata:
+ data: data
+nav:
+- FastAPI: index.md
+- Languages:
+ - en: /
+ - az: /az/
+ - de: /de/
+ - es: /es/
+ - fa: /fa/
+ - fr: /fr/
+ - he: /he/
+ - id: /id/
+ - it: /it/
+ - ja: /ja/
+ - ko: /ko/
+ - nl: /nl/
+ - pl: /pl/
+ - pt: /pt/
+ - ru: /ru/
+ - sq: /sq/
+ - sv: /sv/
+ - tr: /tr/
+ - uk: /uk/
+ - zh: /zh/
+markdown_extensions:
+- toc:
+ permalink: true
+- markdown.extensions.codehilite:
+ guess_lang: false
+- mdx_include:
+ base_path: docs
+- admonition
+- codehilite
+- extra
+- pymdownx.superfences:
+ custom_fences:
+ - name: mermaid
+ class: mermaid
+ format: !!python/name:pymdownx.superfences.fence_code_format ''
+- pymdownx.tabbed:
+ alternate_style: true
+- attr_list
+- md_in_html
+extra:
+ analytics:
+ provider: google
+ property: UA-133183413-1
+ social:
+ - icon: fontawesome/brands/github-alt
+ link: https://github.com/tiangolo/fastapi
+ - icon: fontawesome/brands/discord
+ link: https://discord.gg/VQjSZaeJmf
+ - icon: fontawesome/brands/twitter
+ link: https://twitter.com/fastapi
+ - icon: fontawesome/brands/linkedin
+ link: https://www.linkedin.com/in/tiangolo
+ - icon: fontawesome/brands/dev
+ link: https://dev.to/tiangolo
+ - icon: fontawesome/brands/medium
+ link: https://medium.com/@tiangolo
+ - icon: fontawesome/solid/globe
+ link: https://tiangolo.com
+ alternate:
+ - link: /
+ name: en - English
+ - link: /az/
+ name: az
+ - link: /de/
+ name: de
+ - link: /es/
+ name: es - español
+ - link: /fa/
+ name: fa
+ - link: /fr/
+ name: fr - français
+ - link: /he/
+ name: he
+ - link: /id/
+ name: id
+ - link: /it/
+ name: it - italiano
+ - link: /ja/
+ name: ja - 日本語
+ - link: /ko/
+ name: ko - 한국어
+ - link: /nl/
+ name: nl
+ - link: /pl/
+ name: pl
+ - link: /pt/
+ name: pt - português
+ - link: /ru/
+ name: ru - русский язык
+ - link: /sq/
+ name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
+ - link: /tr/
+ name: tr - Türkçe
+ - link: /uk/
+ name: uk - українська мова
+ - link: /zh/
+ name: zh - 汉语
+extra_css:
+- https://fastapi.tiangolo.com/css/termynal.css
+- https://fastapi.tiangolo.com/css/custom.css
+extra_javascript:
+- https://fastapi.tiangolo.com/js/termynal.js
+- https://fastapi.tiangolo.com/js/custom.js
diff --git a/docs/he/overrides/.gitignore b/docs/he/overrides/.gitignore
new file mode 100644
index 000000000..e69de29bb
diff --git a/docs/id/docs/index.md b/docs/id/docs/index.md
index 95fb7ae21..041e0b754 100644
--- a/docs/id/docs/index.md
+++ b/docs/id/docs/index.md
@@ -135,7 +135,7 @@ You will also need an ASGI server, for production such as
```console
-$ pip install uvicorn[standard]
+$ pip install "uvicorn[standard]"
---> 100%
```
@@ -321,7 +321,7 @@ And now, go to requests - Required if you want to use the `TestClient`.
-* aiofiles - Required if you want to use `FileResponse` or `StaticFiles`.
* jinja2 - Required if you want to use the default template configuration.
* python-multipart - Required if you want to support form "parsing", with `request.form()`.
* itsdangerous - Required for `SessionMiddleware` support.
diff --git a/docs/id/docs/tutorial/index.md b/docs/id/docs/tutorial/index.md
new file mode 100644
index 000000000..8fec3c087
--- /dev/null
+++ b/docs/id/docs/tutorial/index.md
@@ -0,0 +1,80 @@
+# Tutorial - Pedoman Pengguna - Pengenalan
+
+Tutorial ini menunjukan cara menggunakan ***FastAPI*** dengan semua fitur-fiturnya, tahap demi tahap.
+
+Setiap bagian dibangun secara bertahap dari bagian sebelumnya, tetapi terstruktur untuk memisahkan banyak topik, sehingga kamu bisa secara langsung menuju ke topik spesifik untuk menyelesaikan kebutuhan API tertentu.
+
+Ini juga dibangun untuk digunakan sebagai referensi yang akan datang.
+
+Sehingga kamu dapat kembali lagi dan mencari apa yang kamu butuhkan dengan tepat.
+
+## Jalankan kode
+
+Semua blok-blok kode dapat dicopy dan digunakan langsung (Mereka semua sebenarnya adalah file python yang sudah teruji).
+
+Untuk menjalankan setiap contoh, copy kode ke file `main.py`, dan jalankan `uvicorn` dengan:
+
+
+
+```console
+$ uvicorn main:app --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+INFO: Started reloader process [28720]
+INFO: Started server process [28722]
+INFO: Waiting for application startup.
+INFO: Application startup complete.
+```
+
+
+
+**SANGAT disarankan** agar kamu menulis atau meng-copy kode, meng-editnya dan menjalankannya secara lokal.
+
+Dengan menggunakannya di dalam editor, benar-benar memperlihatkan manfaat dari FastAPI, melihat bagaimana sedikitnya kode yang harus kamu tulis, semua pengecekan tipe, pelengkapan otomatis, dll.
+
+---
+
+## Install FastAPI
+
+Langkah pertama adalah dengan meng-install FastAPI.
+
+Untuk tutorial, kamu mungkin hendak meng-instalnya dengan semua pilihan fitur dan dependensinya:
+
+
+
+---
+
+**Documentation**: https://fastapi.tiangolo.com
+
+**Source Code**: https://github.com/tiangolo/fastapi
+
+---
+
+FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints.
+
+The key features are:
+
+* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance).
+
+* **Fast to code**: Increase the speed to develop features by about 200% to 300%. *
+* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. *
+* **Intuitive**: Great editor support. Completion everywhere. Less time debugging.
+* **Easy**: Designed to be easy to use and learn. Less time reading docs.
+* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs.
+* **Robust**: Get production-ready code. With automatic interactive documentation.
+* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: OpenAPI (previously known as Swagger) and JSON Schema.
+
+* estimation based on tests on an internal development team, building production applications.
+
+## Sponsors
+
+
+
+{% if sponsors %}
+{% for sponsor in sponsors.gold -%}
+
+{% endfor -%}
+{%- for sponsor in sponsors.silver -%}
+
+{% endfor %}
+{% endif %}
+
+
+
+Other sponsors
+
+## Opinions
+
+"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._"
+
+
+
+---
+
+"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_"
+
+
Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - Uber(ref)
+
+---
+
+"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_"
+
+
Kevin Glisson, Marc Vilanova, Forest Monsen - Netflix(ref)
+
+---
+
+"_I’m over the moon excited about **FastAPI**. It’s so fun!_"
+
+
+
+---
+
+"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._"
+
+
+
+---
+
+"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_"
+
+"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_"
+
+
+
+---
+
+## **Typer**, the FastAPI of CLIs
+
+
+
+If you are building a CLI app to be used in the terminal instead of a web API, check out **Typer**.
+
+**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀
+
+## Requirements
+
+Python 3.6+
+
+FastAPI stands on the shoulders of giants:
+
+* Starlette for the web parts.
+* Pydantic for the data parts.
+
+## Installation
+
+
+
+## Example
+
+### Create it
+
+* Create a file `main.py` with:
+
+```Python
+from typing import Union
+
+from fastapi import FastAPI
+
+app = FastAPI()
+
+
+@app.get("/")
+def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+```
+
+
+Or use async def...
+
+If your code uses `async` / `await`, use `async def`:
+
+```Python hl_lines="9 14"
+from typing import Union
+
+from fastapi import FastAPI
+
+app = FastAPI()
+
+
+@app.get("/")
+async def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+async def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+```
+
+**Note**:
+
+If you don't know, check the _"In a hurry?"_ section about `async` and `await` in the docs.
+
+
+
+### Run it
+
+Run the server with:
+
+
+
+```console
+$ uvicorn main:app --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+INFO: Started reloader process [28720]
+INFO: Started server process [28722]
+INFO: Waiting for application startup.
+INFO: Application startup complete.
+```
+
+
+
+
+About the command uvicorn main:app --reload...
+
+The command `uvicorn main:app` refers to:
+
+* `main`: the file `main.py` (the Python "module").
+* `app`: the object created inside of `main.py` with the line `app = FastAPI()`.
+* `--reload`: make the server restart after code changes. Only do this for development.
+
+
+
+### Check it
+
+Open your browser at http://127.0.0.1:8000/items/5?q=somequery.
+
+You will see the JSON response as:
+
+```JSON
+{"item_id": 5, "q": "somequery"}
+```
+
+You already created an API that:
+
+* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`.
+* Both _paths_ take `GET` operations (also known as HTTP _methods_).
+* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`.
+* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`.
+
+### Interactive API docs
+
+Now go to http://127.0.0.1:8000/docs.
+
+You will see the automatic interactive API documentation (provided by Swagger UI):
+
+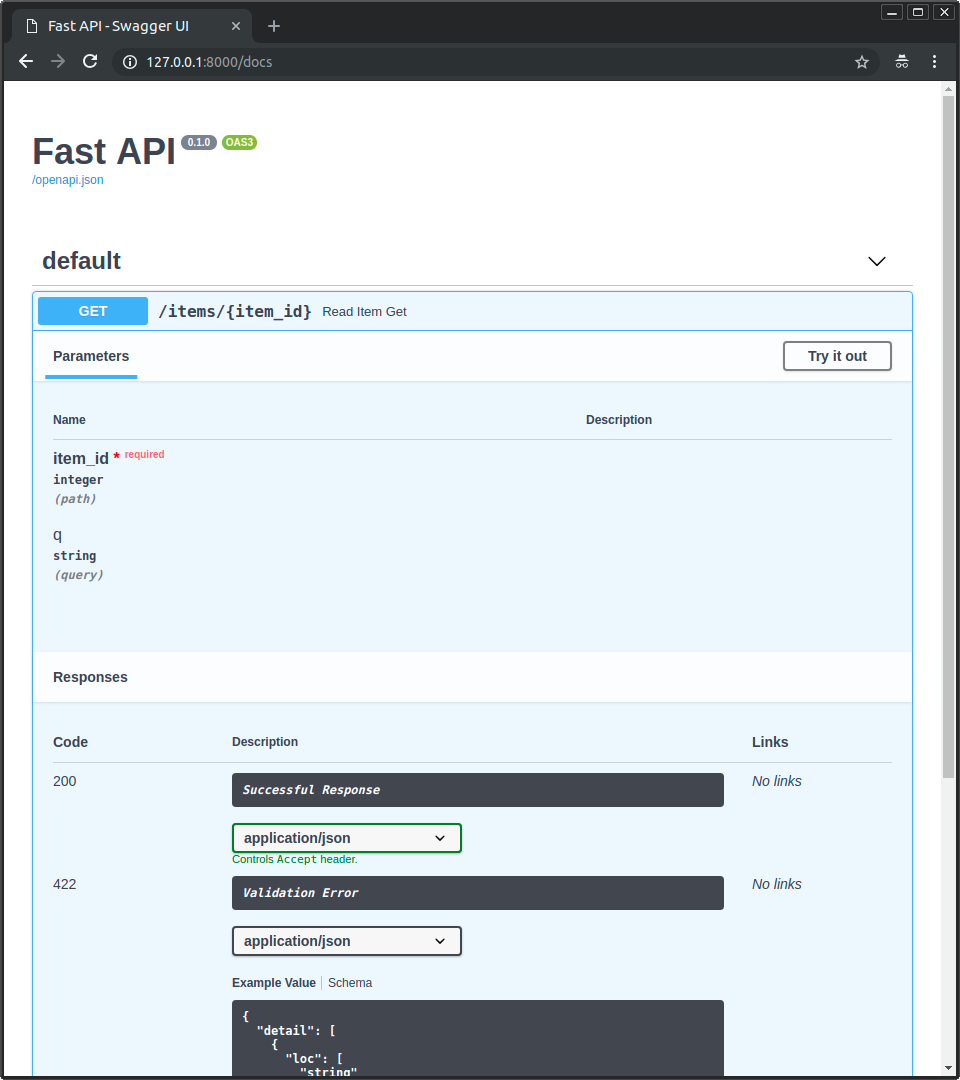
+
+### Alternative API docs
+
+And now, go to http://127.0.0.1:8000/redoc.
+
+You will see the alternative automatic documentation (provided by ReDoc):
+
+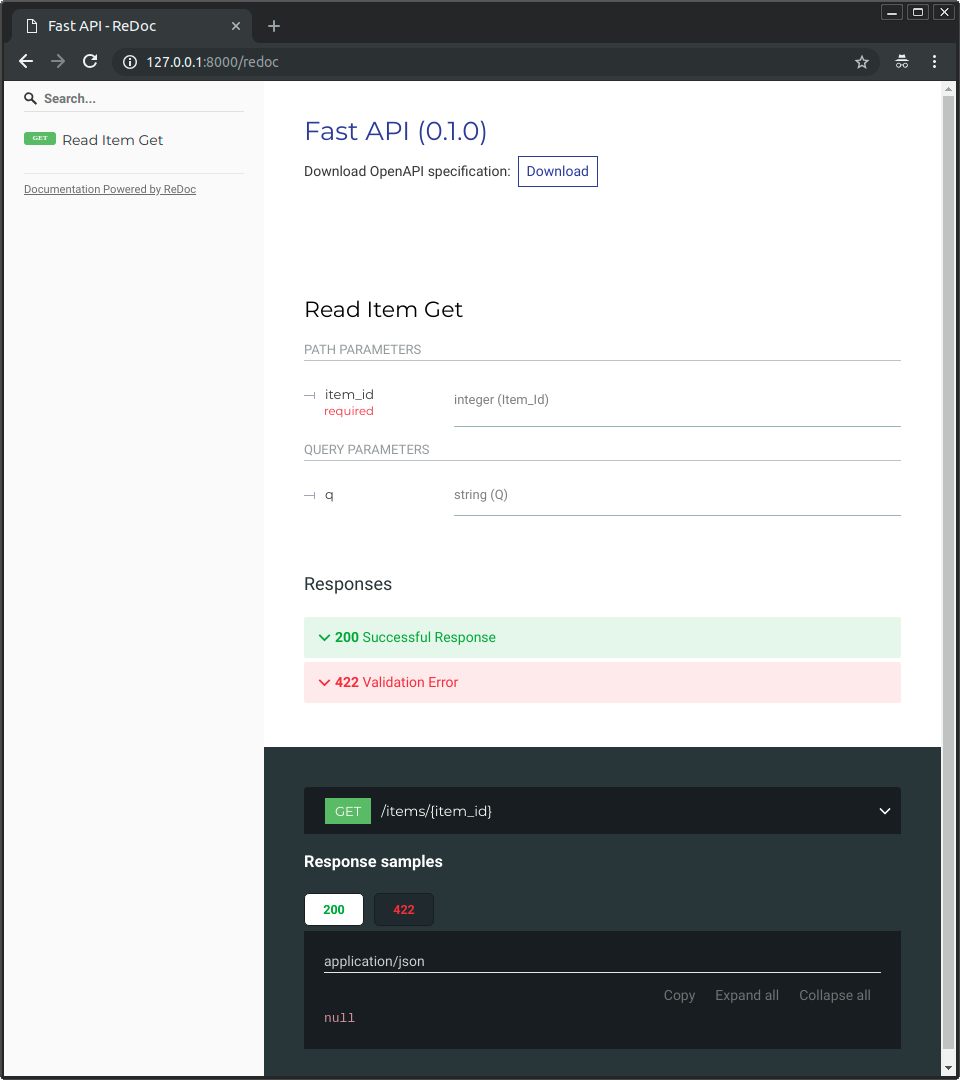
+
+## Example upgrade
+
+Now modify the file `main.py` to receive a body from a `PUT` request.
+
+Declare the body using standard Python types, thanks to Pydantic.
+
+```Python hl_lines="4 9-12 25-27"
+from typing import Union
+
+from fastapi import FastAPI
+from pydantic import BaseModel
+
+app = FastAPI()
+
+
+class Item(BaseModel):
+ name: str
+ price: float
+ is_offer: Union[bool, None] = None
+
+
+@app.get("/")
+def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+
+
+@app.put("/items/{item_id}")
+def update_item(item_id: int, item: Item):
+ return {"item_name": item.name, "item_id": item_id}
+```
+
+The server should reload automatically (because you added `--reload` to the `uvicorn` command above).
+
+### Interactive API docs upgrade
+
+Now go to http://127.0.0.1:8000/docs.
+
+* The interactive API documentation will be automatically updated, including the new body:
+
+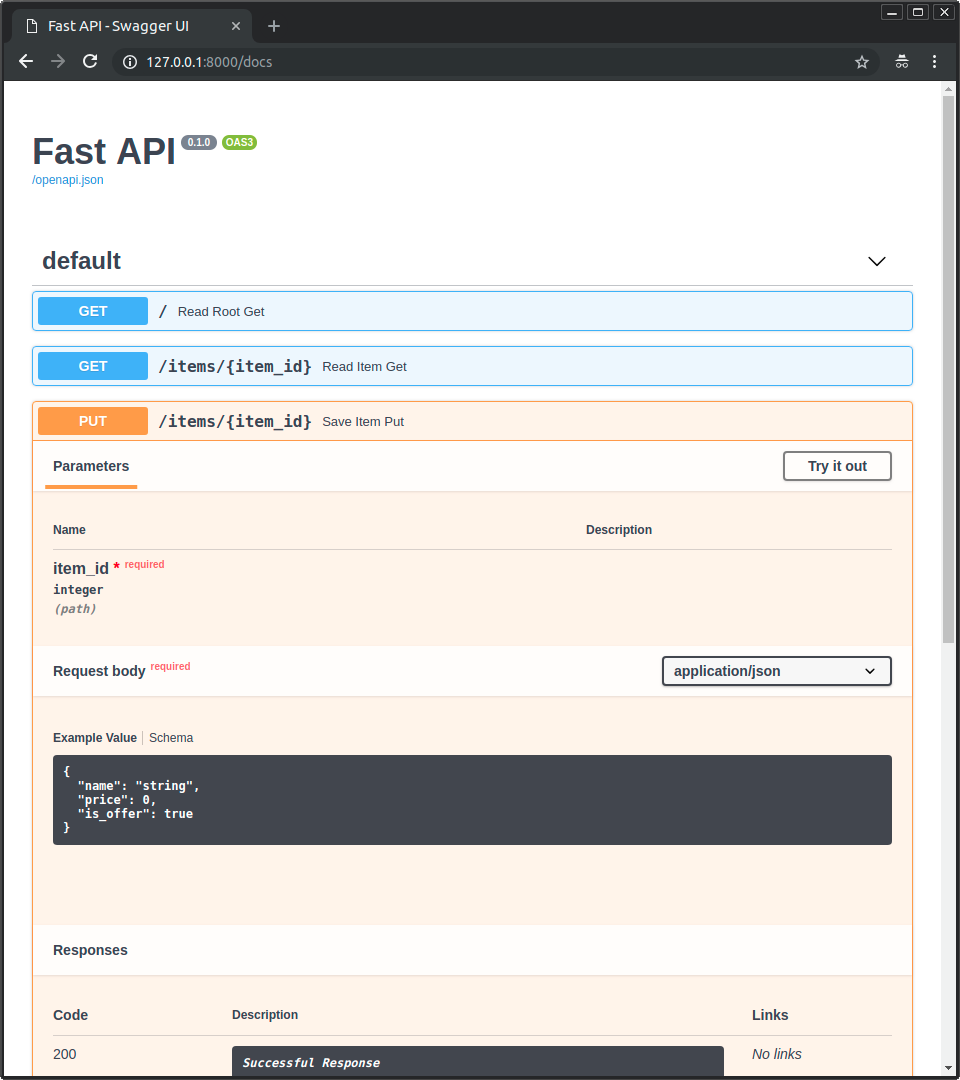
+
+* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API:
+
+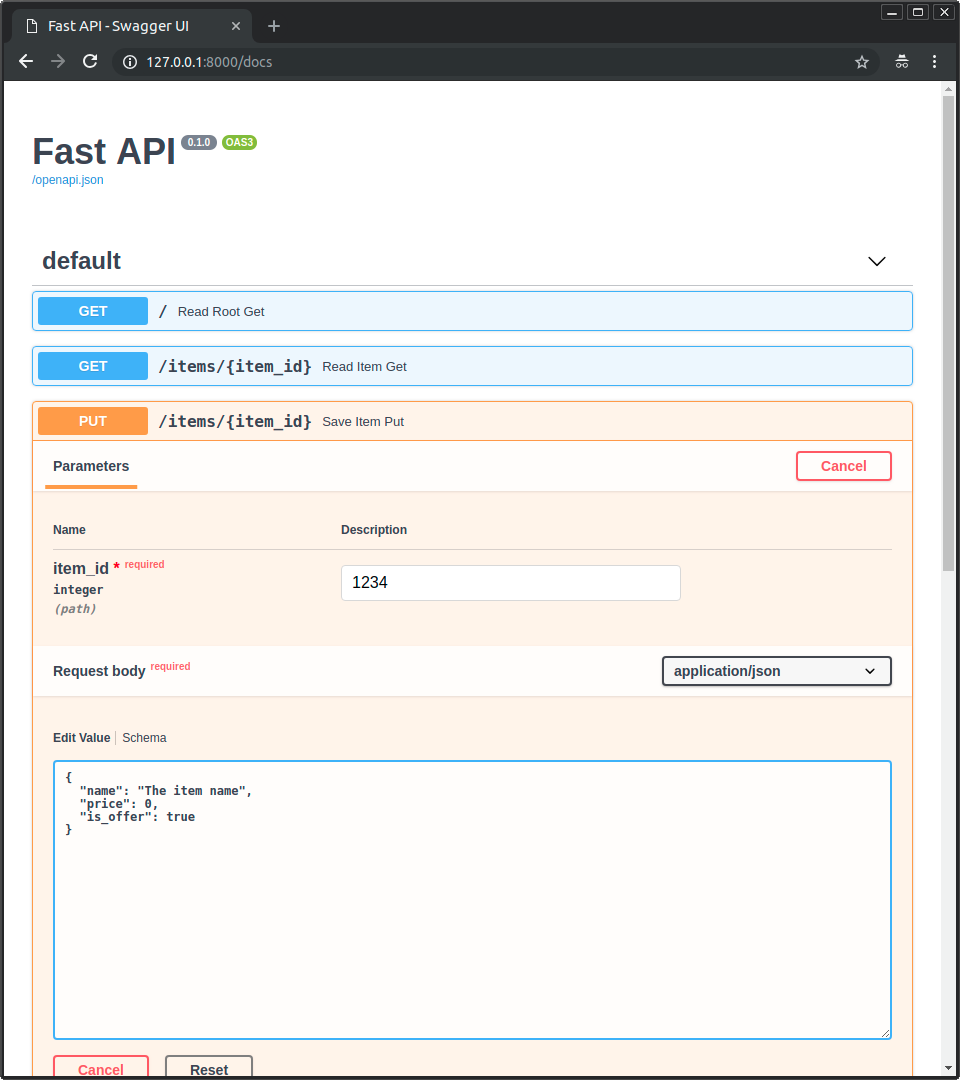
+
+* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen:
+
+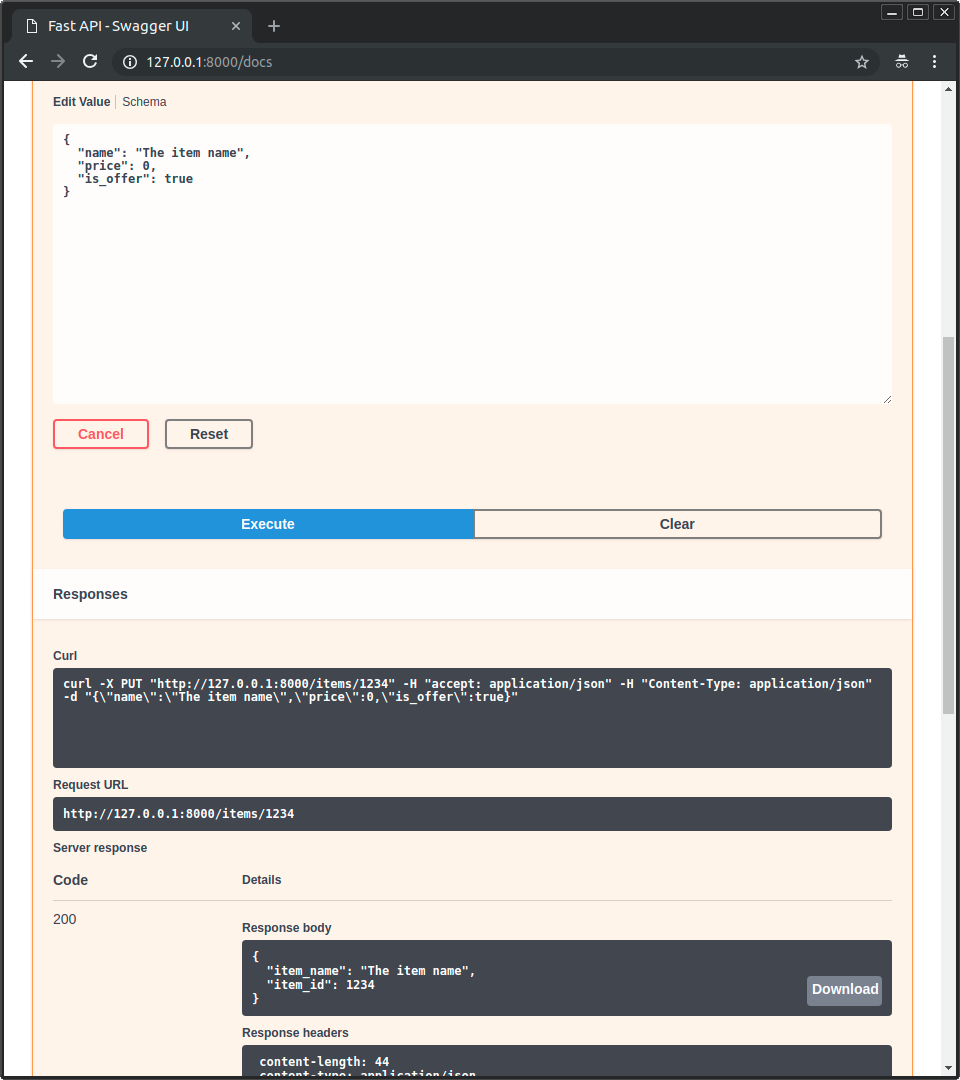
+
+### Alternative API docs upgrade
+
+And now, go to http://127.0.0.1:8000/redoc.
+
+* The alternative documentation will also reflect the new query parameter and body:
+
+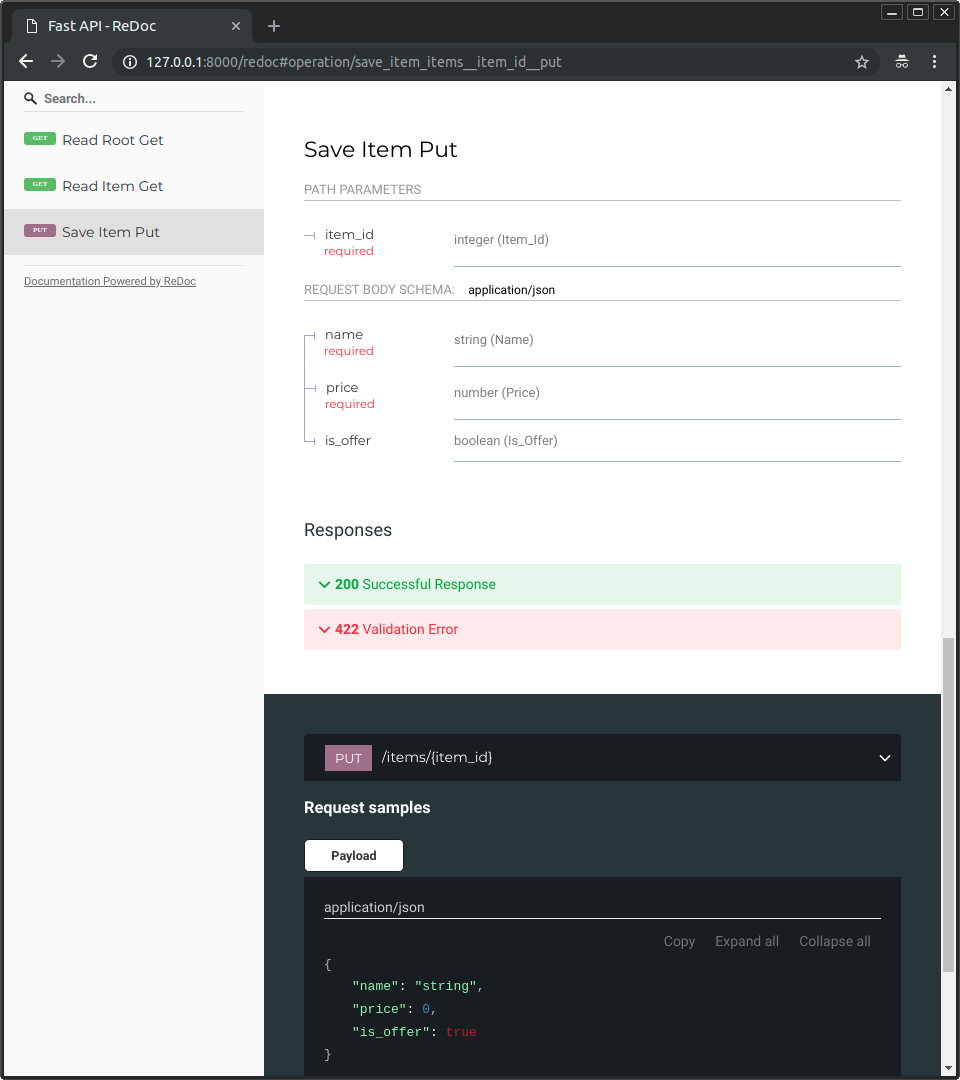
+
+### Recap
+
+In summary, you declare **once** the types of parameters, body, etc. as function parameters.
+
+You do that with standard modern Python types.
+
+You don't have to learn a new syntax, the methods or classes of a specific library, etc.
+
+Just standard **Python 3.6+**.
+
+For example, for an `int`:
+
+```Python
+item_id: int
+```
+
+or for a more complex `Item` model:
+
+```Python
+item: Item
+```
+
+...and with that single declaration you get:
+
+* Editor support, including:
+ * Completion.
+ * Type checks.
+* Validation of data:
+ * Automatic and clear errors when the data is invalid.
+ * Validation even for deeply nested JSON objects.
+* Conversion of input data: coming from the network to Python data and types. Reading from:
+ * JSON.
+ * Path parameters.
+ * Query parameters.
+ * Cookies.
+ * Headers.
+ * Forms.
+ * Files.
+* Conversion of output data: converting from Python data and types to network data (as JSON):
+ * Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc).
+ * `datetime` objects.
+ * `UUID` objects.
+ * Database models.
+ * ...and many more.
+* Automatic interactive API documentation, including 2 alternative user interfaces:
+ * Swagger UI.
+ * ReDoc.
+
+---
+
+Coming back to the previous code example, **FastAPI** will:
+
+* Validate that there is an `item_id` in the path for `GET` and `PUT` requests.
+* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests.
+ * If it is not, the client will see a useful, clear error.
+* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests.
+ * As the `q` parameter is declared with `= None`, it is optional.
+ * Without the `None` it would be required (as is the body in the case with `PUT`).
+* For `PUT` requests to `/items/{item_id}`, Read the body as JSON:
+ * Check that it has a required attribute `name` that should be a `str`.
+ * Check that it has a required attribute `price` that has to be a `float`.
+ * Check that it has an optional attribute `is_offer`, that should be a `bool`, if present.
+ * All this would also work for deeply nested JSON objects.
+* Convert from and to JSON automatically.
+* Document everything with OpenAPI, that can be used by:
+ * Interactive documentation systems.
+ * Automatic client code generation systems, for many languages.
+* Provide 2 interactive documentation web interfaces directly.
+
+---
+
+We just scratched the surface, but you already get the idea of how it all works.
+
+Try changing the line with:
+
+```Python
+ return {"item_name": item.name, "item_id": item_id}
+```
+
+...from:
+
+```Python
+ ... "item_name": item.name ...
+```
+
+...to:
+
+```Python
+ ... "item_price": item.price ...
+```
+
+...and see how your editor will auto-complete the attributes and know their types:
+
+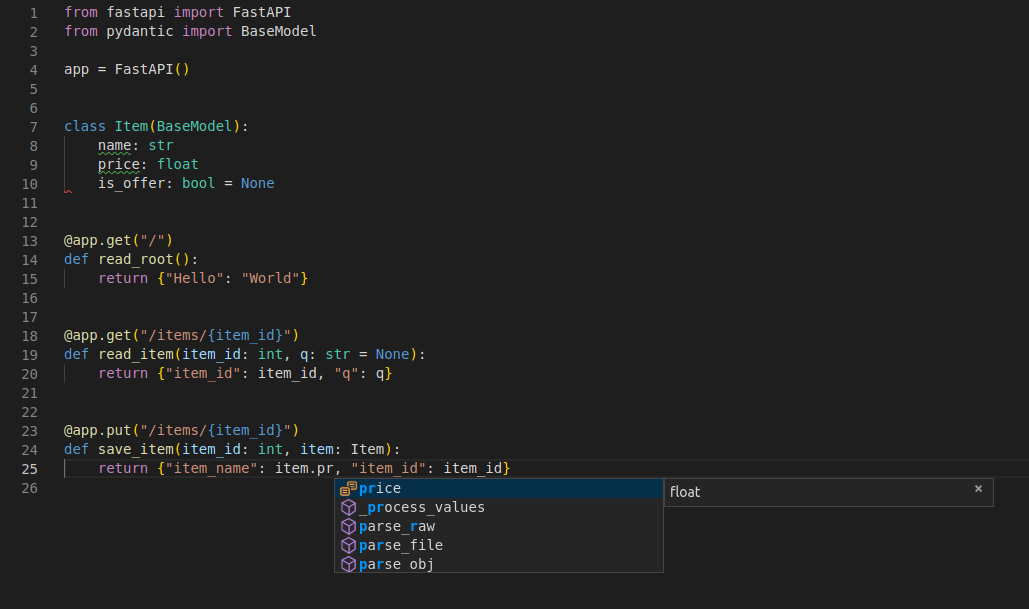
+
+For a more complete example including more features, see the Tutorial - User Guide.
+
+**Spoiler alert**: the tutorial - user guide includes:
+
+* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**.
+* How to set **validation constraints** as `maximum_length` or `regex`.
+* A very powerful and easy to use **Dependency Injection** system.
+* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth.
+* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic).
+* **GraphQL** integration with Strawberry and other libraries.
+* Many extra features (thanks to Starlette) as:
+ * **WebSockets**
+ * extremely easy tests based on `requests` and `pytest`
+ * **CORS**
+ * **Cookie Sessions**
+ * ...and more.
+
+## Performance
+
+Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as one of the fastest Python frameworks available, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*)
+
+To understand more about it, see the section Benchmarks.
+
+## Optional Dependencies
+
+Used by Pydantic:
+
+* ujson - for faster JSON "parsing".
+* email_validator - for email validation.
+
+Used by Starlette:
+
+* requests - Required if you want to use the `TestClient`.
+* jinja2 - Required if you want to use the default template configuration.
+* python-multipart - Required if you want to support form "parsing", with `request.form()`.
+* itsdangerous - Required for `SessionMiddleware` support.
+* pyyaml - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI).
+* ujson - Required if you want to use `UJSONResponse`.
+
+Used by FastAPI / Starlette:
+
+* uvicorn - for the server that loads and serves your application.
+* orjson - Required if you want to use `ORJSONResponse`.
+
+You can install all of these with `pip install "fastapi[all]"`.
+
+## License
+
+This project is licensed under the terms of the MIT license.
diff --git a/docs/nl/mkdocs.yml b/docs/nl/mkdocs.yml
new file mode 100644
index 000000000..6d46939f9
--- /dev/null
+++ b/docs/nl/mkdocs.yml
@@ -0,0 +1,145 @@
+site_name: FastAPI
+site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production
+site_url: https://fastapi.tiangolo.com/nl/
+theme:
+ name: material
+ custom_dir: overrides
+ palette:
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
+ primary: teal
+ accent: amber
+ toggle:
+ icon: material/lightbulb
+ name: Switch to light mode
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
+ primary: teal
+ accent: amber
+ toggle:
+ icon: material/lightbulb-outline
+ name: Switch to dark mode
+ features:
+ - search.suggest
+ - search.highlight
+ - content.tabs.link
+ icon:
+ repo: fontawesome/brands/github-alt
+ logo: https://fastapi.tiangolo.com/img/icon-white.svg
+ favicon: https://fastapi.tiangolo.com/img/favicon.png
+ language: nl
+repo_name: tiangolo/fastapi
+repo_url: https://github.com/tiangolo/fastapi
+edit_uri: ''
+plugins:
+- search
+- markdownextradata:
+ data: data
+nav:
+- FastAPI: index.md
+- Languages:
+ - en: /
+ - az: /az/
+ - de: /de/
+ - es: /es/
+ - fa: /fa/
+ - fr: /fr/
+ - he: /he/
+ - id: /id/
+ - it: /it/
+ - ja: /ja/
+ - ko: /ko/
+ - nl: /nl/
+ - pl: /pl/
+ - pt: /pt/
+ - ru: /ru/
+ - sq: /sq/
+ - sv: /sv/
+ - tr: /tr/
+ - uk: /uk/
+ - zh: /zh/
+markdown_extensions:
+- toc:
+ permalink: true
+- markdown.extensions.codehilite:
+ guess_lang: false
+- mdx_include:
+ base_path: docs
+- admonition
+- codehilite
+- extra
+- pymdownx.superfences:
+ custom_fences:
+ - name: mermaid
+ class: mermaid
+ format: !!python/name:pymdownx.superfences.fence_code_format ''
+- pymdownx.tabbed:
+ alternate_style: true
+- attr_list
+- md_in_html
+extra:
+ analytics:
+ provider: google
+ property: UA-133183413-1
+ social:
+ - icon: fontawesome/brands/github-alt
+ link: https://github.com/tiangolo/fastapi
+ - icon: fontawesome/brands/discord
+ link: https://discord.gg/VQjSZaeJmf
+ - icon: fontawesome/brands/twitter
+ link: https://twitter.com/fastapi
+ - icon: fontawesome/brands/linkedin
+ link: https://www.linkedin.com/in/tiangolo
+ - icon: fontawesome/brands/dev
+ link: https://dev.to/tiangolo
+ - icon: fontawesome/brands/medium
+ link: https://medium.com/@tiangolo
+ - icon: fontawesome/solid/globe
+ link: https://tiangolo.com
+ alternate:
+ - link: /
+ name: en - English
+ - link: /az/
+ name: az
+ - link: /de/
+ name: de
+ - link: /es/
+ name: es - español
+ - link: /fa/
+ name: fa
+ - link: /fr/
+ name: fr - français
+ - link: /he/
+ name: he
+ - link: /id/
+ name: id
+ - link: /it/
+ name: it - italiano
+ - link: /ja/
+ name: ja - 日本語
+ - link: /ko/
+ name: ko - 한국어
+ - link: /nl/
+ name: nl
+ - link: /pl/
+ name: pl
+ - link: /pt/
+ name: pt - português
+ - link: /ru/
+ name: ru - русский язык
+ - link: /sq/
+ name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
+ - link: /tr/
+ name: tr - Türkçe
+ - link: /uk/
+ name: uk - українська мова
+ - link: /zh/
+ name: zh - 汉语
+extra_css:
+- https://fastapi.tiangolo.com/css/termynal.css
+- https://fastapi.tiangolo.com/css/custom.css
+extra_javascript:
+- https://fastapi.tiangolo.com/js/termynal.js
+- https://fastapi.tiangolo.com/js/custom.js
diff --git a/docs/nl/overrides/.gitignore b/docs/nl/overrides/.gitignore
new file mode 100644
index 000000000..e69de29bb
diff --git a/docs/pl/docs/index.md b/docs/pl/docs/index.md
index 4a300ae63..9cc99ba72 100644
--- a/docs/pl/docs/index.md
+++ b/docs/pl/docs/index.md
@@ -130,7 +130,7 @@ Na serwerze produkcyjnym będziesz także potrzebował serwera ASGI, np.
```console
-$ pip install uvicorn[standard]
+$ pip install "uvicorn[standard]"
---> 100%
```
@@ -144,7 +144,7 @@ $ pip install uvicorn[standard]
* Utwórz plik o nazwie `main.py` z:
```Python
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -157,7 +157,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -167,7 +167,7 @@ def read_item(item_id: int, q: Optional[str] = None):
Jeżeli twój kod korzysta z `async` / `await`, użyj `async def`:
```Python hl_lines="9 14"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -180,7 +180,7 @@ async def read_root():
@app.get("/items/{item_id}")
-async def read_item(item_id: int, q: Optional[str] = None):
+async def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -258,7 +258,7 @@ Zmodyfikuj teraz plik `main.py`, aby otrzmywał treść (body) żądania `PUT`.
Zadeklaruj treść żądania, używając standardowych typów w Pythonie dzięki Pydantic.
```Python hl_lines="4 9-12 25-27"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
from pydantic import BaseModel
@@ -269,7 +269,7 @@ app = FastAPI()
class Item(BaseModel):
name: str
price: float
- is_offer: Optional[bool] = None
+ is_offer: Union[bool, None] = None
@app.get("/")
@@ -278,7 +278,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
diff --git a/docs/pl/docs/tutorial/first-steps.md b/docs/pl/docs/tutorial/first-steps.md
new file mode 100644
index 000000000..9406d703d
--- /dev/null
+++ b/docs/pl/docs/tutorial/first-steps.md
@@ -0,0 +1,334 @@
+# Pierwsze kroki
+
+Najprostszy plik FastAPI może wyglądać tak:
+
+```Python
+{!../../../docs_src/first_steps/tutorial001.py!}
+```
+
+Skopiuj to do pliku `main.py`.
+
+Uruchom serwer:
+
+
+
+!!! note
+ Polecenie `uvicorn main:app` odnosi się do:
+
+ * `main`: plik `main.py` ("moduł" Python).
+ * `app`: obiekt utworzony w pliku `main.py` w lini `app = FastAPI()`.
+ * `--reload`: sprawia, że serwer uruchamia się ponownie po zmianie kodu. Używany tylko w trakcie tworzenia oprogramowania.
+
+Na wyjściu znajduje się linia z czymś w rodzaju:
+
+```hl_lines="4"
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+```
+
+Ta linia pokazuje adres URL, pod którym Twoja aplikacja jest obsługiwana, na Twoim lokalnym komputerze.
+
+### Sprawdź to
+
+Otwórz w swojej przeglądarce http://127.0.0.1:8000.
+
+Zobaczysz odpowiedź w formacie JSON:
+
+```JSON
+{"message": "Hello World"}
+```
+
+### Interaktywna dokumentacja API
+
+Przejdź teraz do http://127.0.0.1:8000/docs.
+
+Zobaczysz automatyczną i interaktywną dokumentację API (dostarczoną przez Swagger UI):
+
+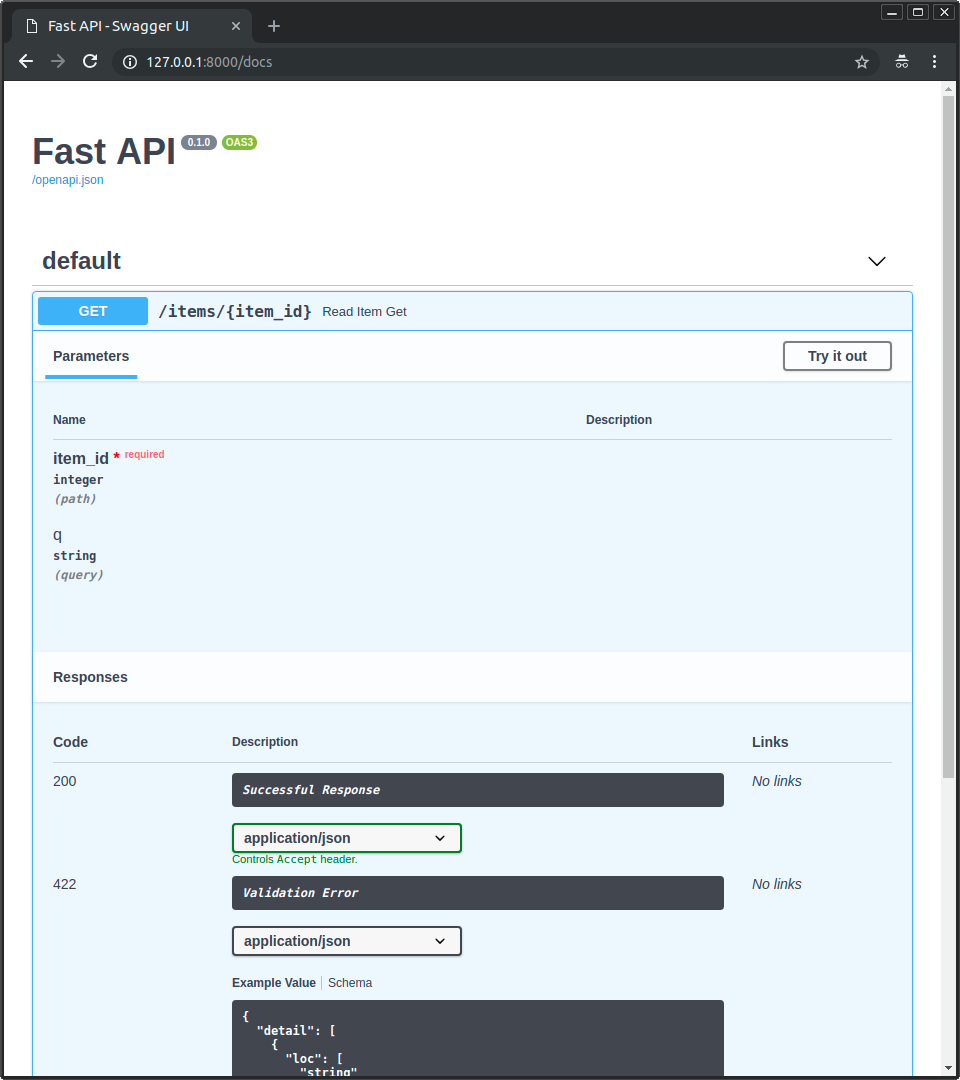
+
+### Alternatywna dokumentacja API
+
+Teraz przejdź do http://127.0.0.1:8000/redoc.
+
+Zobaczysz alternatywną automatycznie wygenerowaną dokumentację API (dostarczoną przez ReDoc):
+
+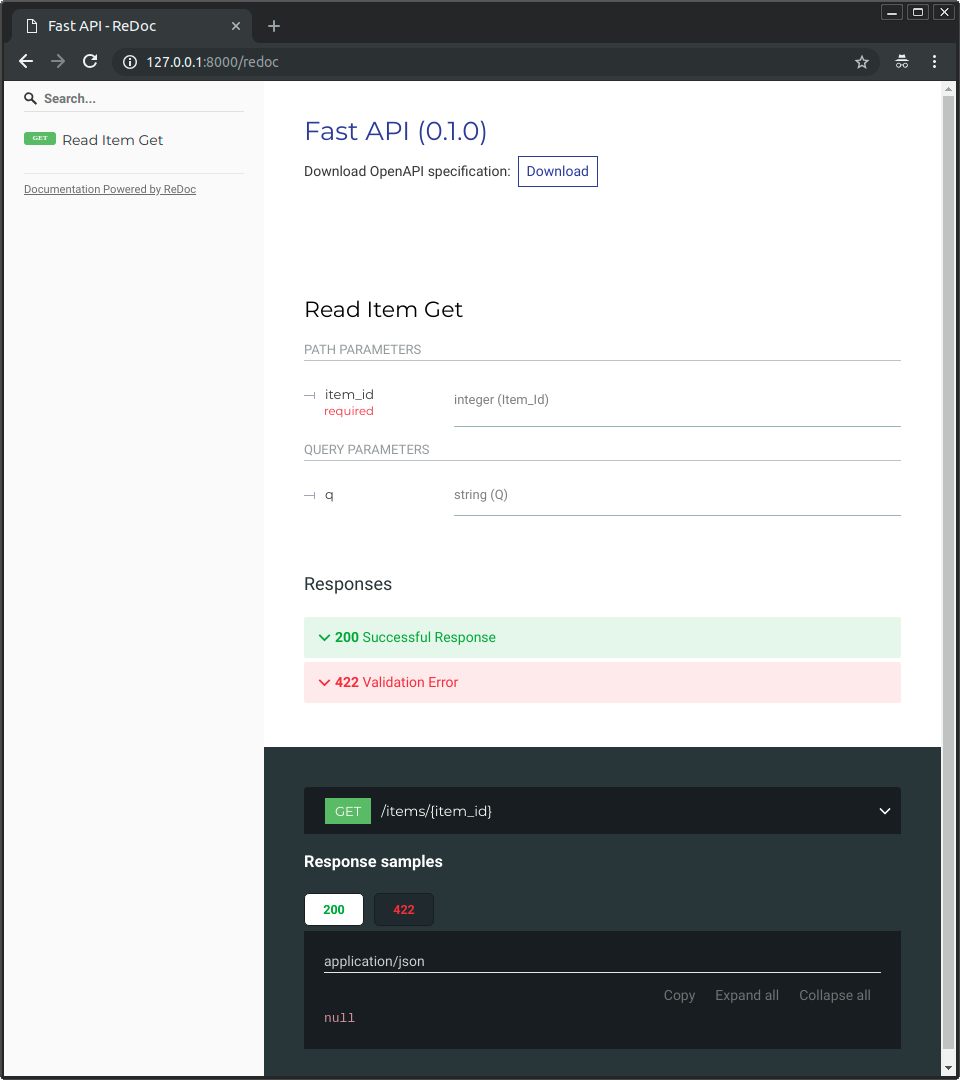
+
+### OpenAPI
+
+**FastAPI** generuje "schemat" z całym Twoim API przy użyciu standardu **OpenAPI** służącego do definiowania API.
+
+#### Schema
+
+"Schema" jest definicją lub opisem czegoś. Nie jest to kod, który go implementuje, ale po prostu abstrakcyjny opis.
+
+#### API "Schema"
+
+W typ przypadku, OpenAPI to specyfikacja, która dyktuje sposób definiowania schematu interfejsu API.
+
+Definicja schematu zawiera ścieżki API, możliwe parametry, które są przyjmowane przez endpointy, itp.
+
+#### "Schemat" danych
+
+Termin "schemat" może również odnosić się do wyglądu niektórych danych, takich jak zawartość JSON.
+
+W takim przypadku będzie to oznaczać atrybuty JSON, ich typy danych itp.
+
+#### OpenAPI i JSON Schema
+
+OpenAPI definiuje API Schema dla Twojego API, który zawiera definicje (lub "schematy") danych wysyłanych i odbieranych przez Twój interfejs API przy użyciu **JSON Schema**, standardu dla schematów danych w formacie JSON.
+
+#### Sprawdź `openapi.json`
+
+Jeśli jesteś ciekawy, jak wygląda surowy schemat OpenAPI, FastAPI automatycznie generuje JSON Schema z opisami wszystkich Twoich API.
+
+Możesz to zobaczyć bezpośrednio pod adresem: http://127.0.0.1:8000/openapi.json.
+
+Zobaczysz JSON zaczynający się od czegoś takiego:
+
+```JSON
+{
+ "openapi": "3.0.2",
+ "info": {
+ "title": "FastAPI",
+ "version": "0.1.0"
+ },
+ "paths": {
+ "/items/": {
+ "get": {
+ "responses": {
+ "200": {
+ "description": "Successful Response",
+ "content": {
+ "application/json": {
+
+
+
+...
+```
+
+#### Do czego służy OpenAPI
+
+Schemat OpenAPI jest tym, co zasila dwa dołączone interaktywne systemy dokumentacji.
+
+Istnieją dziesiątki alternatyw, wszystkie oparte na OpenAPI. Możesz łatwo dodać dowolną z nich do swojej aplikacji zbudowanej za pomocą **FastAPI**.
+
+Możesz go również użyć do automatycznego generowania kodu dla klientów, którzy komunikują się z Twoim API. Na przykład aplikacje frontendowe, mobilne lub IoT.
+
+## Przypomnijmy, krok po kroku
+
+### Krok 1: zaimportuj `FastAPI`
+
+```Python hl_lines="1"
+{!../../../docs_src/first_steps/tutorial001.py!}
+```
+
+`FastAPI` jest klasą, która zapewnia wszystkie funkcjonalności Twojego API.
+
+!!! note "Szczegóły techniczne"
+ `FastAPI` jest klasą, która dziedziczy bezpośrednio z `Starlette`.
+
+ Oznacza to, że możesz korzystać ze wszystkich funkcjonalności Starlette również w `FastAPI`.
+
+
+### Krok 2: utwórz instancję `FastAPI`
+
+```Python hl_lines="3"
+{!../../../docs_src/first_steps/tutorial001.py!}
+```
+
+Zmienna `app` będzie tutaj "instancją" klasy `FastAPI`.
+
+Będzie to główny punkt interakcji przy tworzeniu całego interfejsu API.
+
+Ta zmienna `app` jest tą samą zmienną, do której odnosi się `uvicorn` w poleceniu:
+
+
+
+```console
+$ uvicorn main:app --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+```
+
+
+
+Jeśli stworzysz swoją aplikację, np.:
+
+```Python hl_lines="3"
+{!../../../docs_src/first_steps/tutorial002.py!}
+```
+
+I umieścisz to w pliku `main.py`, to będziesz mógł tak wywołać `uvicorn`:
+
+
+
+```console
+$ uvicorn main:my_awesome_api --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+```
+
+
+
+### Krok 3: wykonaj *operację na ścieżce*
+
+#### Ścieżka
+
+"Ścieżka" tutaj odnosi się do ostatniej części adresu URL, zaczynając od pierwszego `/`.
+
+Więc, w adresie URL takim jak:
+
+```
+https://example.com/items/foo
+```
+
+...ścieżką będzie:
+
+```
+/items/foo
+```
+
+!!! info
+ "Ścieżka" jest zazwyczaj nazywana "path", "endpoint" lub "route'.
+
+Podczas budowania API, "ścieżka" jest głównym sposobem na oddzielenie "odpowiedzialności" i „zasobów”.
+
+#### Operacje
+
+"Operacje" tutaj odnoszą się do jednej z "metod" HTTP.
+
+Jedna z:
+
+* `POST`
+* `GET`
+* `PUT`
+* `DELETE`
+
+...i te bardziej egzotyczne:
+
+* `OPTIONS`
+* `HEAD`
+* `PATCH`
+* `TRACE`
+
+W protokole HTTP można komunikować się z każdą ścieżką za pomocą jednej (lub więcej) "metod".
+
+---
+
+Podczas tworzenia API zwykle używasz tych metod HTTP do wykonania określonej akcji.
+
+Zazwyczaj używasz:
+
+* `POST`: do tworzenia danych.
+* `GET`: do odczytywania danych.
+* `PUT`: do aktualizacji danych.
+* `DELETE`: do usuwania danych.
+
+Tak więc w OpenAPI każda z metod HTTP nazywana jest "operacją".
+
+Będziemy je również nazywali "**operacjami**".
+
+#### Zdefiniuj *dekorator operacji na ścieżce*
+
+```Python hl_lines="6"
+{!../../../docs_src/first_steps/tutorial001.py!}
+```
+
+`@app.get("/")` mówi **FastAPI** że funkcja poniżej odpowiada za obsługę żądań, które trafiają do:
+
+* ścieżki `/`
+* używając operacji get
+
+!!! info "`@decorator` Info"
+ Składnia `@something` jest w Pythonie nazywana "dekoratorem".
+
+ Umieszczasz to na szczycie funkcji. Jak ładną ozdobną czapkę (chyba stąd wzięła się nazwa).
+
+ "Dekorator" przyjmuje funkcję znajdującą się poniżej jego i coś z nią robi.
+
+ W naszym przypadku dekorator mówi **FastAPI**, że poniższa funkcja odpowiada **ścieżce** `/` z **operacją** `get`.
+
+ Jest to "**dekorator operacji na ścieżce**".
+
+Możesz również użyć innej operacji:
+
+* `@app.post()`
+* `@app.put()`
+* `@app.delete()`
+
+Oraz tych bardziej egzotycznych:
+
+* `@app.options()`
+* `@app.head()`
+* `@app.patch()`
+* `@app.trace()`
+
+!!! tip
+ Możesz dowolnie używać każdej operacji (metody HTTP).
+
+ **FastAPI** nie narzuca żadnego konkretnego znaczenia.
+
+ Informacje tutaj są przedstawione jako wskazówka, a nie wymóg.
+
+ Na przykład, używając GraphQL, normalnie wykonujesz wszystkie akcje używając tylko operacji `POST`.
+
+### Krok 4: zdefiniuj **funkcję obsługującą ścieżkę**
+
+To jest nasza "**funkcja obsługująca ścieżkę**":
+
+* **ścieżka**: to `/`.
+* **operacja**: to `get`.
+* **funkcja**: to funkcja poniżej "dekoratora" (poniżej `@app.get("/")`).
+
+```Python hl_lines="7"
+{!../../../docs_src/first_steps/tutorial001.py!}
+```
+
+Jest to funkcja Python.
+
+Zostanie ona wywołana przez **FastAPI** za każdym razem, gdy otrzyma żądanie do adresu URL "`/`" przy użyciu operacji `GET`.
+
+W tym przypadku jest to funkcja "asynchroniczna".
+
+---
+
+Możesz również zdefiniować to jako normalną funkcję zamiast `async def`:
+
+```Python hl_lines="7"
+{!../../../docs_src/first_steps/tutorial003.py!}
+```
+
+!!! note
+ Jeśli nie znasz różnicy, sprawdź [Async: *"In a hurry?"*](/async/#in-a-hurry){.internal-link target=_blank}.
+
+### Krok 5: zwróć zawartość
+
+```Python hl_lines="8"
+{!../../../docs_src/first_steps/tutorial001.py!}
+```
+
+Możesz zwrócić `dict`, `list`, pojedynczą wartość jako `str`, `int`, itp.
+
+Możesz również zwrócić modele Pydantic (więcej o tym później).
+
+Istnieje wiele innych obiektów i modeli, które zostaną automatycznie skonwertowane do formatu JSON (w tym ORM itp.). Spróbuj użyć swoich ulubionych, jest bardzo prawdopodobne, że są już obsługiwane.
+
+## Podsumowanie
+
+* Zaimportuj `FastAPI`.
+* Stwórz instancję `app`.
+* Dodaj **dekorator operacji na ścieżce** (taki jak `@app.get("/")`).
+* Napisz **funkcję obsługującą ścieżkę** (taką jak `def root(): ...` powyżej).
+* Uruchom serwer deweloperski (`uvicorn main:app --reload`).
diff --git a/docs/pl/docs/tutorial/index.md b/docs/pl/docs/tutorial/index.md
new file mode 100644
index 000000000..ed8752a95
--- /dev/null
+++ b/docs/pl/docs/tutorial/index.md
@@ -0,0 +1,80 @@
+# Samouczek - Wprowadzenie
+
+Ten samouczek pokaże Ci, krok po kroku, jak używać większości funkcji **FastAPI**.
+
+Każda część korzysta z poprzednich, ale jest jednocześnie osobnym tematem. Możesz przejść bezpośrednio do każdego rozdziału, jeśli szukasz rozwiązania konkretnego problemu.
+
+Samouczek jest tak zbudowany, żeby służył jako punkt odniesienia w przyszłości.
+
+Możesz wracać i sprawdzać dokładnie to czego potrzebujesz.
+
+## Wykonywanie kodu
+
+Wszystkie fragmenty kodu mogą być skopiowane bezpośrednio i użyte (są poprawnymi i przetestowanymi plikami).
+
+Żeby wykonać każdy przykład skopiuj kod to pliku `main.py` i uruchom `uvicorn` za pomocą:
+
+
+
+```console
+$ uvicorn main:app --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+INFO: Started reloader process [28720]
+INFO: Started server process [28722]
+INFO: Waiting for application startup.
+INFO: Application startup complete.
+```
+
+
+
+**BARDZO zalecamy** pisanie bądź kopiowanie kodu, edycję, a następnie wykonywanie go lokalnie.
+
+Użycie w Twoim edytorze jest tym, co pokazuje prawdziwe korzyści z FastAPI, pozwala zobaczyć jak mało kodu musisz napisać, wszystkie funkcje, takie jak kontrola typów, automatyczne uzupełnianie, itd.
+
+---
+
+## Instalacja FastAPI
+
+Jako pierwszy krok zainstaluj FastAPI.
+
+Na potrzeby samouczka możesz zainstalować również wszystkie opcjonalne biblioteki:
+
+
+
+...wliczając w to `uvicorn`, który będzie służył jako serwer wykonujacy Twój kod.
+
+!!! note
+ Możesz również wykonać instalację "krok po kroku".
+
+ Prawdopodobnie zechcesz to zrobić, kiedy będziesz wdrażać swoją aplikację w środowisku produkcyjnym:
+
+ ```
+ pip install fastapi
+ ```
+
+ Zainstaluj też `uvicorn`, który będzie służył jako serwer:
+
+ ```
+ pip install "uvicorn[standard]"
+ ```
+
+ Tak samo możesz zainstalować wszystkie dodatkowe biblioteki, których chcesz użyć.
+
+## Zaawansowany poradnik
+
+Jest też **Zaawansowany poradnik**, który możesz przeczytać po lekturze tego **Samouczka**.
+
+**Zaawansowany poradnik** opiera się na tym samouczku, używa tych samych pojęć, żeby pokazać Ci kilka dodatkowych funkcji.
+
+Najpierw jednak powinieneś przeczytać **Samouczek** (czytasz go teraz).
+
+Ten rozdział jest zaprojektowany tak, że możesz stworzyć kompletną aplikację używając tylko informacji tutaj zawartych, a następnie rozszerzać ją na różne sposoby, w zależności od potrzeb, używając kilku dodatkowych pomysłów z **Zaawansowanego poradnika**.
diff --git a/docs/pl/mkdocs.yml b/docs/pl/mkdocs.yml
index 3c1351a12..1cd129420 100644
--- a/docs/pl/mkdocs.yml
+++ b/docs/pl/mkdocs.yml
@@ -5,13 +5,15 @@ theme:
name: material
custom_dir: overrides
palette:
- - scheme: default
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
primary: teal
accent: amber
toggle:
icon: material/lightbulb
name: Switch to light mode
- - scheme: slate
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
primary: teal
accent: amber
toggle:
@@ -40,18 +42,25 @@ nav:
- az: /az/
- de: /de/
- es: /es/
+ - fa: /fa/
- fr: /fr/
+ - he: /he/
- id: /id/
- it: /it/
- ja: /ja/
- ko: /ko/
+ - nl: /nl/
- pl: /pl/
- pt: /pt/
- ru: /ru/
- sq: /sq/
+ - sv: /sv/
- tr: /tr/
- uk: /uk/
- zh: /zh/
+- Samouczek:
+ - tutorial/index.md
+ - tutorial/first-steps.md
markdown_extensions:
- toc:
permalink: true
@@ -69,6 +78,8 @@ markdown_extensions:
format: !!python/name:pymdownx.superfences.fence_code_format ''
- pymdownx.tabbed:
alternate_style: true
+- attr_list
+- md_in_html
extra:
analytics:
provider: google
@@ -97,8 +108,12 @@ extra:
name: de
- link: /es/
name: es - español
+ - link: /fa/
+ name: fa
- link: /fr/
name: fr - français
+ - link: /he/
+ name: he
- link: /id/
name: id
- link: /it/
@@ -107,6 +122,8 @@ extra:
name: ja - 日本語
- link: /ko/
name: ko - 한국어
+ - link: /nl/
+ name: nl
- link: /pl/
name: pl
- link: /pt/
@@ -115,6 +132,8 @@ extra:
name: ru - русский язык
- link: /sq/
name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
- link: /tr/
name: tr - Türkçe
- link: /uk/
diff --git a/docs/pt/docs/alternatives.md b/docs/pt/docs/alternatives.md
index 6559b7398..61ee4f900 100644
--- a/docs/pt/docs/alternatives.md
+++ b/docs/pt/docs/alternatives.md
@@ -331,7 +331,7 @@ Agora APIStar é um conjunto de ferramentas para validar especificações OpenAP
Existir.
A idéia de declarar múltiplas coisas (validação de dados, serialização e documentação) com os mesmos tipos Python, que ao mesmo tempo fornecesse grande suporte ao editor, era algo que eu considerava uma brilhante idéia.
-
+
E após procurar por um logo tempo por um framework similar e testar muitas alternativas diferentes, APIStar foi a melhor opção disponível.
Então APIStar parou de existir como um servidor e Starlette foi criado, e foi uma nova melhor fundação para tal sistema. Essa foi a inspiração final para construir **FastAPI**.
@@ -390,7 +390,7 @@ Essa é uma das principais coisas que **FastAPI** adiciona no topo, tudo baseado
Controlar todas as partes web centrais. Adiciona recursos no topo.
A classe `FastAPI` em si herda `Starlette`.
-
+
Então, qualquer coisa que você faz com Starlette, você pode fazer diretamente com **FastAPI**, pois ele é basicamente um Starlette com esteróides.
### Uvicorn
diff --git a/docs/pt/docs/async.md b/docs/pt/docs/async.md
index 44f4b5148..be1278a1b 100644
--- a/docs/pt/docs/async.md
+++ b/docs/pt/docs/async.md
@@ -94,7 +94,7 @@ Para "síncrono" (contrário de "assíncrono") também é utilizado o termo "seq
Essa idéia de código **assíncrono** descrito acima é algo às vezes chamado de **"concorrência"**. E é diferente de **"paralelismo"**.
-**Concorrência** e **paralelismo** ambos são relacionados a "diferentes coisas acontecendo mais ou menos ao mesmo tempo".
+**Concorrência** e **paralelismo** ambos são relacionados a "diferentes coisas acontecendo mais ou menos ao mesmo tempo".
Mas os detalhes entre *concorrência* e *paralelismo* são bem diferentes.
@@ -134,7 +134,7 @@ Mas então, embora você ainda não tenha os hambúrgueres, seu trabalho no caix
Mas enquanto você se afasta do balcão e senta na mesa com o número da sua chamada, você pode trocar sua atenção para seu _crush_ :heart_eyes:, e "trabalhar" nisso. Então você está novamente fazendo algo muito "produtivo", como flertar com seu _crush_ :heart_eyes:.
-Então o caixa diz que "seus hambúrgueres estão prontos" colocando seu número no balcão, mas você não corre que nem um maluco imediatamente quando o número exibido é o seu. Você sabe que ninguém irá roubar seus hambúrgueres porquê você tem o número de chamada, e os outros tem os números deles.
+Então o caixa diz que "seus hambúrgueres estão prontos" colocando seu número no balcão, mas você não corre que nem um maluco imediatamente quando o número exibido é o seu. Você sabe que ninguém irá roubar seus hambúrgueres porquê você tem o número de chamada, e os outros tem os números deles.
Então você espera que seu _crush_ :heart_eyes: termine a história que estava contando (terminar o trabalho atual / tarefa sendo processada), sorri gentilmente e diz que você está indo buscar os hambúrgueres.
@@ -358,9 +358,9 @@ Tudo isso é o que deixa o FastAPI poderoso (através do Starlette) e que o faz
!!! warning
Você pode provavelmente pular isso.
-
+
Esses são detalhes muito técnicos de como **FastAPI** funciona por baixo do capô.
-
+
Se você tem algum conhecimento técnico (corrotinas, threads, blocking etc) e está curioso sobre como o FastAPI controla o `async def` vs normal `def`, vá em frente.
### Funções de operação de rota
diff --git a/docs/pt/docs/benchmarks.md b/docs/pt/docs/benchmarks.md
index 7f7c95ba1..07461ce46 100644
--- a/docs/pt/docs/benchmarks.md
+++ b/docs/pt/docs/benchmarks.md
@@ -2,7 +2,7 @@
As comparações independentes da TechEmpower mostram as aplicações **FastAPI** rodando com Uvicorn como um dos _frameworks_ Python mais rápidos disponíveis, somente atrás dos próprios Starlette e Uvicorn (utilizados internamente pelo FastAPI). (*)
-Mas quando se checa _benchmarks_ e comparações você deveria ter o seguinte em mente.
+Mas quando se checa _benchmarks_ e comparações você deveria ter o seguinte em mente.
## Comparações e velocidade
diff --git a/docs/pt/docs/deployment.md b/docs/pt/docs/deployment.md
index cd820cbd3..2272467fd 100644
--- a/docs/pt/docs/deployment.md
+++ b/docs/pt/docs/deployment.md
@@ -336,7 +336,7 @@ Você apenas precisa instalar um servidor ASGI compatível como:
```console
- $ pip install uvicorn[standard]
+ $ pip install "uvicorn[standard]"
---> 100%
```
diff --git a/docs/pt/docs/deployment/deta.md b/docs/pt/docs/deployment/deta.md
new file mode 100644
index 000000000..9271bba42
--- /dev/null
+++ b/docs/pt/docs/deployment/deta.md
@@ -0,0 +1,258 @@
+# Implantação FastAPI na Deta
+
+Nessa seção você aprenderá sobre como realizar a implantação de uma aplicação **FastAPI** na Deta utilizando o plano gratuito. 🎁
+
+Isso tudo levará aproximadamente **10 minutos**.
+
+!!! info "Informação"
+ Deta é uma patrocinadora do **FastAPI**. 🎉
+
+## Uma aplicação **FastAPI** simples
+
+* Crie e entre em um diretório para a sua aplicação, por exemplo, `./fastapideta/`.
+
+### Código FastAPI
+
+* Crie o arquivo `main.py` com:
+
+```Python
+from fastapi import FastAPI
+
+app = FastAPI()
+
+
+@app.get("/")
+def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+def read_item(item_id: int):
+ return {"item_id": item_id}
+```
+
+### Requisitos
+
+Agora, no mesmo diretório crie o arquivo `requirements.txt` com:
+
+```text
+fastapi
+```
+
+!!! tip "Dica"
+ Você não precisa instalar Uvicorn para realizar a implantação na Deta, embora provavelmente queira instalá-lo para testar seu aplicativo localmente.
+
+### Estrutura de diretório
+
+Agora você terá o diretório `./fastapideta/` com dois arquivos:
+
+```
+.
+└── main.py
+└── requirements.txt
+```
+
+## Crie uma conta gratuita na Deta
+
+Agora crie uma conta gratuita na Deta, você precisará apenas de um email e senha.
+
+Você nem precisa de um cartão de crédito.
+
+## Instale a CLI
+
+Depois de ter sua conta criada, instale Deta CLI:
+
+=== "Linux, macOS"
+
+
+
+Após a instalação, abra um novo terminal para que a CLI seja detectada.
+
+Em um novo terminal, confirme se foi instalado corretamente com:
+
+
+
+```console
+$ deta --help
+
+Deta command line interface for managing deta micros.
+Complete documentation available at https://docs.deta.sh
+
+Usage:
+ deta [flags]
+ deta [command]
+
+Available Commands:
+ auth Change auth settings for a deta micro
+
+...
+```
+
+
+
+!!! tip "Dica"
+ Se você tiver problemas ao instalar a CLI, verifique a documentação oficial da Deta.
+
+## Login pela CLI
+
+Agora faça login na Deta pela CLI com:
+
+
+
+```console
+$ deta login
+
+Please, log in from the web page. Waiting..
+Logged in successfully.
+```
+
+
+
+Isso abrirá um navegador da Web e autenticará automaticamente.
+
+## Implantação com Deta
+
+Em seguida, implante seu aplicativo com a Deta CLI:
+
+
+
+Você verá uma mensagem JSON semelhante a:
+
+```JSON hl_lines="4"
+{
+ "name": "fastapideta",
+ "runtime": "python3.7",
+ "endpoint": "https://qltnci.deta.dev",
+ "visor": "enabled",
+ "http_auth": "enabled"
+}
+```
+
+!!! tip "Dica"
+ Sua implantação terá um URL `"endpoint"` diferente.
+
+## Confira
+
+Agora, abra seu navegador na URL do `endpoint`. No exemplo acima foi `https://qltnci.deta.dev`, mas o seu será diferente.
+
+Você verá a resposta JSON do seu aplicativo FastAPI:
+
+```JSON
+{
+ "Hello": "World"
+}
+```
+
+Agora vá para o `/docs` da sua API, no exemplo acima seria `https://qltnci.deta.dev/docs`.
+
+Ele mostrará sua documentação como:
+
+
+
+## Permitir acesso público
+
+Por padrão, a Deta lidará com a autenticação usando cookies para sua conta.
+
+Mas quando estiver pronto, você pode torná-lo público com:
+
+
+
+Agora você pode compartilhar essa URL com qualquer pessoa e elas conseguirão acessar sua API. 🚀
+
+## HTTPS
+
+Parabéns! Você realizou a implantação do seu app FastAPI na Deta! 🎉 🍰
+
+Além disso, observe que a Deta lida corretamente com HTTPS para você, para que você não precise cuidar disso e tenha a certeza de que seus clientes terão uma conexão criptografada segura. ✅ 🔒
+
+## Verifique o Visor
+
+Na UI da sua documentação (você estará em um URL como `https://qltnci.deta.dev/docs`) envie um request para *operação de rota* `/items/{item_id}`.
+
+Por exemplo com ID `5`.
+
+Agora vá para https://web.deta.sh.
+
+Você verá que há uma seção à esquerda chamada "Micros" com cada um dos seus apps.
+
+Você verá uma aba com "Detalhes", e também a aba "Visor", vá para "Visor".
+
+Lá você pode inspecionar as solicitações recentes enviadas ao seu aplicativo.
+
+Você também pode editá-los e reproduzi-los novamente.
+
+
+
+## Saiba mais
+
+Em algum momento, você provavelmente desejará armazenar alguns dados para seu aplicativo de uma forma que persista ao longo do tempo. Para isso você pode usar Deta Base, que também tem um generoso **nível gratuito**.
+
+Você também pode ler mais na documentação da Deta.
+
+## Conceitos de implantação
+
+Voltando aos conceitos que discutimos em [Deployments Concepts](./concepts.md){.internal-link target=_blank}, veja como cada um deles seria tratado com a Deta:
+
+* **HTTPS**: Realizado pela Deta, eles fornecerão um subdomínio e lidarão com HTTPS automaticamente.
+* **Executando na inicialização**: Realizado pela Deta, como parte de seu serviço.
+* **Reinicialização**: Realizado pela Deta, como parte de seu serviço.
+* **Replicação**: Realizado pela Deta, como parte de seu serviço.
+* **Memória**: Limite predefinido pela Deta, você pode contatá-los para aumentá-lo.
+* **Etapas anteriores a inicialização**: Não suportado diretamente, você pode fazê-lo funcionar com o sistema Cron ou scripts adicionais.
+
+!!! note "Nota"
+ O Deta foi projetado para facilitar (e gratuitamente) a implantação rápida de aplicativos simples.
+
+ Ele pode simplificar vários casos de uso, mas, ao mesmo tempo, não suporta outros, como o uso de bancos de dados externos (além do próprio sistema de banco de dados NoSQL da Deta), máquinas virtuais personalizadas, etc.
+
+ Você pode ler mais detalhes na documentação da Deta para ver se é a escolha certa para você.
diff --git a/docs/pt/docs/features.md b/docs/pt/docs/features.md
index 20014fe2d..2b7836a6f 100644
--- a/docs/pt/docs/features.md
+++ b/docs/pt/docs/features.md
@@ -191,7 +191,7 @@ Com **FastAPI** você terá todos os recursos do **Pydantic** (já que FastAPI u
* Vai bem com o/a seu/sua **IDE/linter/cérebro**:
* Como as estruturas de dados do Pydantic são apenas instâncias de classes que você define, a auto completação, _linting_, _mypy_ e a sua intuição devem funcionar corretamente com seus dados validados.
* **Rápido**:
- * em _benchmarks_, o Pydantic é mais rápido que todas as outras bibliotecas testadas.
+ * em _benchmarks_, o Pydantic é mais rápido que todas as outras bibliotecas testadas.
* Valida **estruturas complexas**:
* Use modelos hierárquicos do Pydantic, `List` e `Dict` do `typing` do Python, etc.
* Validadores permitem que esquemas de dados complexos sejam limpos e facilmente definidos, conferidos e documentados como JSON Schema.
diff --git a/docs/pt/docs/help-fastapi.md b/docs/pt/docs/help-fastapi.md
new file mode 100644
index 000000000..d82ce3414
--- /dev/null
+++ b/docs/pt/docs/help-fastapi.md
@@ -0,0 +1,148 @@
+# Ajuda FastAPI - Obter Ajuda
+
+Você gosta do **FastAPI**?
+
+Você gostaria de ajudar o FastAPI, outros usários, e o autor?
+
+Ou você gostaria de obter ajuda relacionada ao **FastAPI**??
+
+Existem métodos muito simples de ajudar (A maioria das ajudas podem ser feitas com um ou dois cliques).
+
+E também existem vários modos de se conseguir ajuda.
+
+## Inscreva-se na newsletter
+
+Você pode se inscrever (pouco frequente) [**FastAPI e amigos** newsletter](/newsletter/){.internal-link target=_blank} para receber atualizações:
+
+* Notícias sobre FastAPI e amigos 🚀
+* Tutoriais 📝
+* Recursos ✨
+* Mudanças de última hora 🚨
+* Truques e dicas ✅
+
+## Siga o FastAPI no twitter
+
+Siga @fastapi no **Twitter** para receber as últimas notícias sobre o **FastAPI**. 🐦
+
+## Favorite o **FastAPI** no GitHub
+
+Você pode "favoritar" o FastAPI no GitHub (clicando na estrela no canto superior direito): https://github.com/tiangolo/fastapi. ⭐️
+
+Favoritando, outros usuários poderão encontrar mais facilmente e verão que já foi útil para muita gente.
+
+## Acompanhe novos updates no repositorio do GitHub
+
+Você pode "acompanhar" (watch) o FastAPI no GitHub (clicando no botão com um "olho" no canto superior direito): https://github.com/tiangolo/fastapi. 👀
+
+Podendo selecionar apenas "Novos Updates".
+
+Fazendo isto, serão enviadas notificações (em seu email) sempre que tiver novos updates (uma nova versão) com correções de bugs e novos recursos no **FastAPI**
+
+## Conect-se com o autor
+
+Você pode se conectar comigo (Sebastián Ramírez / `tiangolo`), o autor.
+
+Você pode:
+
+* Me siga no **GitHub**.
+ * Ver também outros projetos Open Source criados por mim que podem te ajudar.
+ * Me seguir para saber quando um novo projeto Open Source for criado.
+* Me siga no **Twitter**.
+ * Me dizer o motivo pelo o qual você está usando o FastAPI(Adoro ouvir esse tipo de comentário).
+ * Saber quando eu soltar novos anúncios ou novas ferramentas.
+ * Também é possivel seguir o @fastapi no Twitter (uma conta aparte).
+* Conect-se comigo no **Linkedin**.
+ * Saber quando eu fizer novos anúncios ou novas ferramentas (apesar de que uso o twitter com mais frequência 🤷♂).
+* Ler meus artigos (ou me seguir) no **Dev.to** ou no **Medium**.
+ * Ficar por dentro de novas ideias, artigos, e ferramentas criadas por mim.
+ * Me siga para saber quando eu publicar algo novo.
+
+## Tweete sobre **FastAPI**
+
+Tweete sobre o **FastAPI** e compartilhe comigo e com os outros o porque de gostar do FastAPI. 🎉
+
+Adoro ouvir sobre como o **FastAPI** é usado, o que você gosta nele, em qual projeto/empresa está sendo usado, etc.
+
+## Vote no FastAPI
+
+* Vote no **FastAPI** no Slant.
+* Vote no **FastAPI** no AlternativeTo.
+
+## Responda perguntas no GitHub
+
+Você pode acompanhar as perguntas existentes e tentar ajudar outros, . 🤓
+
+Ajudando a responder as questões de varias pessoas, você pode se tornar um [Expert em FastAPI](fastapi-people.md#experts){.internal-link target=_blank} oficial. 🎉
+
+## Acompanhe o repositório do GitHub
+
+Você pode "acompanhar" (watch) o FastAPI no GitHub (clicando no "olho" no canto superior direito): https://github.com/tiangolo/fastapi. 👀
+
+Se você selecionar "Acompanhando" (Watching) em vez de "Apenas Lançamentos" (Releases only) você receberá notificações quando alguém tiver uma nova pergunta.
+
+Assim podendo tentar ajudar a resolver essas questões.
+
+## Faça perguntas
+
+É possível criar uma nova pergunta no repositório do GitHub, por exemplo:
+
+* Faça uma **pergunta** ou pergunte sobre um **problema**.
+* Sugira novos **recursos**.
+
+**Nota**: Se você fizer uma pergunta, então eu gostaria de pedir que você também ajude os outros com suas respectivas perguntas. 😉
+
+## Crie um Pull Request
+
+É possível [contribuir](contributing.md){.internal-link target=_blank} no código fonte fazendo Pull Requests, por exemplo:
+
+* Para corrigir um erro de digitação que você encontrou na documentação.
+* Para compartilhar um artigo, video, ou podcast criados por você sobre o FastAPI editando este arquivo.
+ * Não se esqueça de adicionar o link no começo da seção correspondente.
+* Para ajudar [traduzir a documentação](contributing.md#translations){.internal-link target=_blank} para sua lingua.
+ * Também é possivel revisar as traduções já existentes.
+* Para propor novas seções na documentação.
+* Para corrigir um bug/questão.
+* Para adicionar um novo recurso.
+
+## Entre no chat
+
+Entre no 👥 server de conversa do Discord 👥 e conheça novas pessoas da comunidade
+do FastAPI.
+
+!!! dica
+ Para perguntas, pergunte nas questões do GitHub, lá tem um chance maior de você ser ajudado sobre o FastAPI [FastAPI Experts](fastapi-people.md#experts){.internal-link target=_blank}.
+
+ Use o chat apenas para outro tipo de assunto.
+
+Também existe o chat do Gitter, porém ele não possuí canais e recursos avançados, conversas são mais engessadas, por isso o Discord é mais recomendado.
+
+### Não faça perguntas no chat
+
+Tenha em mente que os chats permitem uma "conversa mais livre", dessa forma é muito fácil fazer perguntas que são muito genéricas e dificeís de responder, assim você pode acabar não sendo respondido.
+
+Nas questões do GitHub o template irá te guiar para que você faça a sua pergunta de um jeito mais correto, fazendo com que você receba respostas mais completas, e até mesmo que você mesmo resolva o problema antes de perguntar. E no GitHub eu garanto que sempre irei responder todas as perguntas, mesmo que leve um tempo. Eu pessoalmente não consigo fazer isso via chat. 😅
+
+Conversas no chat não são tão fáceis de serem encontrados quanto no GitHub, então questões e respostas podem se perder dentro da conversa. E apenas as que estão nas questões do GitHub contam para você se tornar um [Expert em FastAPI](fastapi-people.md#experts){.internal-link target=_blank}, então você receberá mais atenção nas questões do GitHub.
+
+Por outro lado, existem milhares de usuários no chat, então tem uma grande chance de você encontrar alguém para trocar uma idéia por lá em qualquer horário. 😄
+
+## Patrocine o autor
+
+Você também pode ajudar o autor financeiramente (eu) através do GitHub sponsors.
+
+Lá você pode me pagar um cafézinho ☕️ como agradecimento. 😄
+
+E você também pode se tornar um patrocinador Prata ou Ouro do FastAPI. 🏅🎉
+
+## Patrocine as ferramente que potencializam o FastAPI
+
+Como você viu na documentação, o FastAPI se apoia em nos gigantes, Starlette e Pydantic.
+
+Patrocine também:
+
+* Samuel Colvin (Pydantic)
+* Encode (Starlette, Uvicorn)
+
+---
+
+Muito Obrigado! 🚀
diff --git a/docs/pt/docs/index.md b/docs/pt/docs/index.md
index 848fff08a..51af486b9 100644
--- a/docs/pt/docs/index.md
+++ b/docs/pt/docs/index.md
@@ -124,7 +124,7 @@ Você também precisará de um servidor ASGI para produção, tal como
```console
-$ pip install uvicorn[standard]
+$ pip install "uvicorn[standard]"
---> 100%
```
@@ -138,7 +138,7 @@ $ pip install uvicorn[standard]
* Crie um arquivo `main.py` com:
```Python
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -151,7 +151,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -161,7 +161,7 @@ def read_item(item_id: int, q: Optional[str] = None):
Se seu código utiliza `async` / `await`, use `async def`:
```Python hl_lines="9 14"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -174,7 +174,7 @@ async def read_root():
@app.get("/items/{item_id}")
-async def read_item(item_id: int, q: Optional[str] = None):
+async def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -253,6 +253,8 @@ Agora modifique o arquivo `main.py` para receber um corpo para uma requisição
Declare o corpo utilizando tipos padrão Python, graças ao Pydantic.
```Python hl_lines="4 9-12 25-27"
+from typing import Union
+
from fastapi import FastAPI
from pydantic import BaseModel
@@ -262,7 +264,7 @@ app = FastAPI()
class Item(BaseModel):
name: str
price: float
- is_offer: Optional[bool] = None
+ is_offer: Union[bool] = None
@app.get("/")
@@ -271,7 +273,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
@@ -365,7 +367,7 @@ Voltando ao código do exemplo anterior, **FastAPI** irá:
* Como o parâmetro `q` é declarado com `= None`, ele é opcional.
* Sem o `None` ele poderia ser obrigatório (como o corpo no caso de `PUT`).
* Para requisições `PUT` para `/items/{item_id}`, lerá o corpo como JSON e:
- * Verifica que tem um atributo obrigatório `name` que deve ser `str`.
+ * Verifica que tem um atributo obrigatório `name` que deve ser `str`.
* Verifica que tem um atributo obrigatório `price` que deve ser `float`.
* Verifica que tem an atributo opcional `is_offer`, que deve ser `bool`, se presente.
* Tudo isso também funciona para objetos JSON profundamente aninhados.
@@ -434,7 +436,6 @@ Usados por Pydantic:
Usados por Starlette:
* requests - Necessário se você quiser utilizar o `TestClient`.
-* aiofiles - Necessário se você quiser utilizar o `FileResponse` ou `StaticFiles`.
* jinja2 - Necessário se você quiser utilizar a configuração padrão de templates.
* python-multipart - Necessário se você quiser suporte com "parsing" de formulário, com `request.form()`.
* itsdangerous - Necessário para suporte a `SessionMiddleware`.
diff --git a/docs/pt/docs/python-types.md b/docs/pt/docs/python-types.md
index df70afd40..9f12211c7 100644
--- a/docs/pt/docs/python-types.md
+++ b/docs/pt/docs/python-types.md
@@ -313,4 +313,3 @@ O importante é que, usando tipos padrão de Python, em um único local (em vez
!!! info "Informação"
Se você já passou por todo o tutorial e voltou para ver mais sobre os tipos, um bom recurso é a "cheat sheet" do `mypy` .
-
diff --git a/docs/pt/docs/tutorial/background-tasks.md b/docs/pt/docs/tutorial/background-tasks.md
new file mode 100644
index 000000000..625fa2b11
--- /dev/null
+++ b/docs/pt/docs/tutorial/background-tasks.md
@@ -0,0 +1,94 @@
+# Tarefas em segundo plano
+
+Você pode definir tarefas em segundo plano a serem executadas _ após _ retornar uma resposta.
+
+Isso é útil para operações que precisam acontecer após uma solicitação, mas que o cliente realmente não precisa esperar a operação ser concluída para receber a resposta.
+
+Isso inclui, por exemplo:
+
+- Envio de notificações por email após a realização de uma ação:
+ - Como conectar-se a um servidor de e-mail e enviar um e-mail tende a ser "lento" (vários segundos), você pode retornar a resposta imediatamente e enviar a notificação por e-mail em segundo plano.
+- Processando dados:
+ - Por exemplo, digamos que você receba um arquivo que deve passar por um processo lento, você pode retornar uma resposta de "Aceito" (HTTP 202) e processá-lo em segundo plano.
+
+## Usando `BackgroundTasks`
+
+Primeiro, importe `BackgroundTasks` e defina um parâmetro em sua _função de operação de caminho_ com uma declaração de tipo de `BackgroundTasks`:
+
+```Python hl_lines="1 13"
+{!../../../docs_src/background_tasks/tutorial001.py!}
+```
+
+O **FastAPI** criará o objeto do tipo `BackgroundTasks` para você e o passará como esse parâmetro.
+
+## Criar uma função de tarefa
+
+Crie uma função a ser executada como tarefa em segundo plano.
+
+É apenas uma função padrão que pode receber parâmetros.
+
+Pode ser uma função `async def` ou `def` normal, o **FastAPI** saberá como lidar com isso corretamente.
+
+Nesse caso, a função de tarefa gravará em um arquivo (simulando o envio de um e-mail).
+
+E como a operação de gravação não usa `async` e `await`, definimos a função com `def` normal:
+
+```Python hl_lines="6-9"
+{!../../../docs_src/background_tasks/tutorial001.py!}
+```
+
+## Adicionar a tarefa em segundo plano
+
+Dentro de sua _função de operação de caminho_, passe sua função de tarefa para o objeto _tarefas em segundo plano_ com o método `.add_task()`:
+
+```Python hl_lines="14"
+{!../../../docs_src/background_tasks/tutorial001.py!}
+```
+
+`.add_task()` recebe como argumentos:
+
+- Uma função de tarefa a ser executada em segundo plano (`write_notification`).
+- Qualquer sequência de argumentos que deve ser passada para a função de tarefa na ordem (`email`).
+- Quaisquer argumentos nomeados que devem ser passados para a função de tarefa (`mensagem = "alguma notificação"`).
+
+## Injeção de dependência
+
+Usar `BackgroundTasks` também funciona com o sistema de injeção de dependência, você pode declarar um parâmetro do tipo `BackgroundTasks` em vários níveis: em uma _função de operação de caminho_, em uma dependência (confiável), em uma subdependência, etc.
+
+O **FastAPI** sabe o que fazer em cada caso e como reutilizar o mesmo objeto, de forma que todas as tarefas em segundo plano sejam mescladas e executadas em segundo plano posteriormente:
+
+```Python hl_lines="13 15 22 25"
+{!../../../docs_src/background_tasks/tutorial002.py!}
+```
+
+Neste exemplo, as mensagens serão gravadas no arquivo `log.txt` _após_ o envio da resposta.
+
+Se houver uma consulta na solicitação, ela será gravada no log em uma tarefa em segundo plano.
+
+E então outra tarefa em segundo plano gerada na _função de operação de caminho_ escreverá uma mensagem usando o parâmetro de caminho `email`.
+
+## Detalhes técnicos
+
+A classe `BackgroundTasks` vem diretamente de `starlette.background`.
+
+Ela é importada/incluída diretamente no FastAPI para que você possa importá-la do `fastapi` e evitar a importação acidental da alternativa `BackgroundTask` (sem o `s` no final) de `starlette.background`.
+
+Usando apenas `BackgroundTasks` (e não `BackgroundTask`), é então possível usá-la como um parâmetro de _função de operação de caminho_ e deixar o **FastAPI** cuidar do resto para você, assim como ao usar o objeto `Request` diretamente.
+
+Ainda é possível usar `BackgroundTask` sozinho no FastAPI, mas você deve criar o objeto em seu código e retornar uma Starlette `Response` incluindo-o.
+
+Você pode ver mais detalhes na documentação oficiais da Starlette para tarefas em segundo plano .
+
+## Ressalva
+
+Se você precisa realizar cálculos pesados em segundo plano e não necessariamente precisa que seja executado pelo mesmo processo (por exemplo, você não precisa compartilhar memória, variáveis, etc), você pode se beneficiar do uso de outras ferramentas maiores, como Celery .
+
+Eles tendem a exigir configurações mais complexas, um gerenciador de fila de mensagens/tarefas, como RabbitMQ ou Redis, mas permitem que você execute tarefas em segundo plano em vários processos e, especialmente, em vários servidores.
+
+Para ver um exemplo, verifique os [Geradores de projeto](../project-generation.md){.internal-link target=\_blank}, todos incluem celery já configurado.
+
+Mas se você precisa acessar variáveis e objetos do mesmo aplicativo **FastAPI**, ou precisa realizar pequenas tarefas em segundo plano (como enviar uma notificação por e-mail), você pode simplesmente usar `BackgroundTasks`.
+
+## Recapitulando
+
+Importe e use `BackgroundTasks` com parâmetros em _funções de operação de caminho_ e dependências para adicionar tarefas em segundo plano.
diff --git a/docs/pt/docs/tutorial/body.md b/docs/pt/docs/tutorial/body.md
new file mode 100644
index 000000000..99e05ab77
--- /dev/null
+++ b/docs/pt/docs/tutorial/body.md
@@ -0,0 +1,165 @@
+# Corpo da Requisição
+
+Quando você precisa enviar dados de um cliente (como de um navegador web) para sua API, você o envia como um **corpo da requisição**.
+
+O corpo da **requisição** é a informação enviada pelo cliente para sua API. O corpo da **resposta** é a informação que sua API envia para o cliente.
+
+Sua API quase sempre irá enviar um corpo na **resposta**. Mas os clientes não necessariamente precisam enviar um corpo em toda **requisição**.
+
+Para declarar um corpo da **requisição**, você utiliza os modelos do Pydantic com todos os seus poderes e benefícios.
+
+!!! info "Informação"
+ Para enviar dados, você deve usar utilizar um dos métodos: `POST` (Mais comum), `PUT`, `DELETE` ou `PATCH`.
+
+ Enviar um corpo em uma requisição `GET` não tem um comportamento definido nas especificações, porém é suportado pelo FastAPI, apenas para casos de uso bem complexos/extremos.
+
+ Como é desencorajado, a documentação interativa com Swagger UI não irá mostrar a documentação para o corpo da requisição para um `GET`, e proxies que intermediarem podem não suportar o corpo da requisição.
+
+## Importe o `BaseModel` do Pydantic
+
+Primeiro, você precisa importar `BaseModel` do `pydantic`:
+
+```Python hl_lines="4"
+{!../../../docs_src/body/tutorial001.py!}
+```
+
+## Crie seu modelo de dados
+
+Então você declara seu modelo de dados como uma classe que herda `BaseModel`.
+
+Utilize os tipos Python padrão para todos os atributos:
+
+```Python hl_lines="7-11"
+{!../../../docs_src/body/tutorial001.py!}
+```
+
+Assim como quando declaramos parâmetros de consulta, quando um atributo do modelo possui um valor padrão, ele se torna opcional. Caso contrário, se torna obrigatório. Use `None` para torná-lo opcional.
+
+Por exemplo, o modelo acima declara um JSON "`object`" (ou `dict` no Python) como esse:
+
+```JSON
+{
+ "name": "Foo",
+ "description": "Uma descrição opcional",
+ "price": 45.2,
+ "tax": 3.5
+}
+```
+
+...como `description` e `tax` são opcionais (Com um valor padrão de `None`), esse JSON "`object`" também é válido:
+
+```JSON
+{
+ "name": "Foo",
+ "price": 45.2
+}
+```
+
+## Declare como um parâmetro
+
+Para adicionar o corpo na *função de operação de rota*, declare-o da mesma maneira que você declarou parâmetros de rota e consulta:
+
+```Python hl_lines="18"
+{!../../../docs_src/body/tutorial001.py!}
+```
+
+...E declare o tipo como o modelo que você criou, `Item`.
+
+## Resultados
+
+Apenas com esse declaração de tipos do Python, o **FastAPI** irá:
+
+* Ler o corpo da requisição como um JSON.
+* Converter os tipos correspondentes (se necessário).
+* Validar os dados.
+ * Se algum dados for inválido, irá retornar um erro bem claro, indicando exatamente onde e o que está incorreto.
+* Entregar a você a informação recebida no parâmetro `item`.
+ * Como você o declarou na função como do tipo `Item`, você também terá o suporte do editor (completação, etc) para todos os atributos e seus tipos.
+* Gerar um Esquema JSON com as definições do seu modelo, você também pode utilizá-lo em qualquer lugar que quiser, se fizer sentido para seu projeto.
+* Esses esquemas farão parte do esquema OpenAPI, e utilizados nas UIs de documentação automática.
+
+## Documentação automática
+
+Os esquemas JSON dos seus modelos farão parte do esquema OpenAPI gerado para sua aplicação, e aparecerão na documentação interativa da API:
+
+
+
+E também serão utilizados em cada *função de operação de rota* que utilizá-los:
+
+
+
+## Suporte do editor de texto:
+
+No seu editor de texto, dentro da função você receberá dicas de tipos e completação em todo lugar (isso não aconteceria se você recebesse um `dict` em vez de um modelo Pydantic):
+
+
+
+Você também poderá receber verificações de erros para operações de tipos incorretas:
+
+
+
+Isso não é por acaso, todo o framework foi construído em volta deste design.
+
+E foi imensamente testado na fase de design, antes de qualquer implementação, para garantir que funcionaria para todos os editores de texto.
+
+Houveram mudanças no próprio Pydantic para que isso fosse possível.
+
+As capturas de tela anteriores foram capturas no Visual Studio Code.
+
+Mas você terá o mesmo suporte do editor no PyCharm e na maioria dos editores Python:
+
+
+
+!!! tip "Dica"
+ Se você utiliza o PyCharm como editor, você pode utilizar o Plugin do Pydantic para o PyCharm .
+
+ Melhora o suporte do editor para seus modelos Pydantic com::
+
+ * completação automática
+ * verificação de tipos
+ * refatoração
+ * buscas
+ * inspeções
+
+## Use o modelo
+
+Dentro da função, você pode acessar todos os atributos do objeto do modelo diretamente:
+
+```Python hl_lines="21"
+{!../../../docs_src/body/tutorial002.py!}
+```
+
+## Corpo da requisição + parâmetros de rota
+
+Você pode declarar parâmetros de rota e corpo da requisição ao mesmo tempo.
+
+O **FastAPI** irá reconhecer que os parâmetros da função que combinam com parâmetros de rota devem ser **retirados da rota**, e parâmetros da função que são declarados como modelos Pydantic sejam **retirados do corpo da requisição**.
+
+```Python hl_lines="17-18"
+{!../../../docs_src/body/tutorial003.py!}
+```
+
+## Corpo da requisição + parâmetros de rota + parâmetros de consulta
+
+Você também pode declarar parâmetros de **corpo**, **rota** e **consulta**, ao mesmo tempo.
+
+O **FastAPI** irá reconhecer cada um deles e retirar a informação do local correto.
+
+```Python hl_lines="18"
+{!../../../docs_src/body/tutorial004.py!}
+```
+
+Os parâmetros da função serão reconhecidos conforme abaixo:
+
+* Se o parâmetro também é declarado na **rota**, será utilizado como um parâmetro de rota.
+* Se o parâmetro é de um **tipo único** (como `int`, `float`, `str`, `bool`, etc) será interpretado como um parâmetro de **consulta**.
+* Se o parâmetro é declarado como um **modelo Pydantic**, será interpretado como o **corpo** da requisição.
+
+!!! note "Observação"
+ O FastAPI saberá que o valor de `q` não é obrigatório por causa do valor padrão `= None`.
+
+ O `Union` em `Union[str, None]` não é utilizado pelo FastAPI, mas permite ao seu editor de texto lhe dar um suporte melhor e detectar erros.
+
+## Sem o Pydantic
+
+Se você não quer utilizar os modelos Pydantic, você também pode utilizar o parâmetro **Body**. Veja a documentação para [Body - Parâmetros múltiplos: Valores singulares no body](body-multiple-params.md#singular-values-in-body){.internal-link target=_blank}.
diff --git a/docs/pt/docs/tutorial/cookie-params.md b/docs/pt/docs/tutorial/cookie-params.md
new file mode 100644
index 000000000..1a60e3571
--- /dev/null
+++ b/docs/pt/docs/tutorial/cookie-params.md
@@ -0,0 +1,33 @@
+# Parâmetros de Cookie
+
+Você pode definir parâmetros de Cookie da mesma maneira que define paramêtros com `Query` e `Path`.
+
+## Importe `Cookie`
+
+Primeiro importe `Cookie`:
+
+```Python hl_lines="3"
+{!../../../docs_src/cookie_params/tutorial001.py!}
+```
+
+## Declare parâmetros de `Cookie`
+
+Então declare os paramêtros de cookie usando a mesma estrutura que em `Path` e `Query`.
+
+O primeiro valor é o valor padrão, você pode passar todas as validações adicionais ou parâmetros de anotação:
+
+```Python hl_lines="9"
+{!../../../docs_src/cookie_params/tutorial001.py!}
+```
+
+!!! note "Detalhes Técnicos"
+ `Cookie` é uma classe "irmã" de `Path` e `Query`. Ela também herda da mesma classe em comum `Param`.
+
+ Mas lembre-se que quando você importa `Query`, `Path`, `Cookie` e outras de `fastapi`, elas são na verdade funções que retornam classes especiais.
+
+!!! info "Informação"
+ Para declarar cookies, você precisa usar `Cookie`, caso contrário, os parâmetros seriam interpretados como parâmetros de consulta.
+
+## Recapitulando
+
+Declare cookies com `Cookie`, usando o mesmo padrão comum que utiliza-se em `Query` e `Path`.
diff --git a/docs/pt/docs/tutorial/extra-data-types.md b/docs/pt/docs/tutorial/extra-data-types.md
new file mode 100644
index 000000000..e4b9913dc
--- /dev/null
+++ b/docs/pt/docs/tutorial/extra-data-types.md
@@ -0,0 +1,66 @@
+# Tipos de dados extras
+
+Até agora, você tem usado tipos de dados comuns, tais como:
+
+* `int`
+* `float`
+* `str`
+* `bool`
+
+Mas você também pode usar tipos de dados mais complexos.
+
+E você ainda terá os mesmos recursos que viu até agora:
+
+* Ótimo suporte do editor.
+* Conversão de dados das requisições recebidas.
+* Conversão de dados para os dados da resposta.
+* Validação de dados.
+* Anotação e documentação automáticas.
+
+## Outros tipos de dados
+
+Aqui estão alguns dos tipos de dados adicionais que você pode usar:
+
+* `UUID`:
+ * Um "Identificador Universalmente Único" padrão, comumente usado como ID em muitos bancos de dados e sistemas.
+ * Em requisições e respostas será representado como uma `str`.
+* `datetime.datetime`:
+ * O `datetime.datetime` do Python.
+ * Em requisições e respostas será representado como uma `str` no formato ISO 8601, exemplo: `2008-09-15T15:53:00+05:00`.
+* `datetime.date`:
+ * O `datetime.date` do Python.
+ * Em requisições e respostas será representado como uma `str` no formato ISO 8601, exemplo: `2008-09-15`.
+* `datetime.time`:
+ * O `datetime.time` do Python.
+ * Em requisições e respostas será representado como uma `str` no formato ISO 8601, exemplo: `14:23:55.003`.
+* `datetime.timedelta`:
+ * O `datetime.timedelta` do Python.
+ * Em requisições e respostas será representado como um `float` de segundos totais.
+ * O Pydantic também permite representá-lo como uma "codificação ISO 8601 diferença de tempo", cheque a documentação para mais informações.
+* `frozenset`:
+ * Em requisições e respostas, será tratado da mesma forma que um `set`:
+ * Nas requisições, uma lista será lida, eliminando duplicadas e convertendo-a em um `set`.
+ * Nas respostas, o `set` será convertido para uma `list`.
+ * O esquema gerado vai especificar que os valores do `set` são unicos (usando o `uniqueItems` do JSON Schema).
+* `bytes`:
+ * O `bytes` padrão do Python.
+ * Em requisições e respostas será representado como uma `str`.
+ * O esquema gerado vai especificar que é uma `str` com o "formato" `binary`.
+* `Decimal`:
+ * O `Decimal` padrão do Python.
+ * Em requisições e respostas será representado como um `float`.
+* Você pode checar todos os tipos de dados válidos do Pydantic aqui: Tipos de dados do Pydantic.
+
+## Exemplo
+
+Aqui está um exemplo de *operação de rota* com parâmetros utilizando-se de alguns dos tipos acima.
+
+```Python hl_lines="1 3 12-16"
+{!../../../docs_src/extra_data_types/tutorial001.py!}
+```
+
+Note que os parâmetros dentro da função tem seu tipo de dados natural, e você pode, por exemplo, realizar manipulações normais de data, como:
+
+```Python hl_lines="18-19"
+{!../../../docs_src/extra_data_types/tutorial001.py!}
+```
diff --git a/docs/pt/docs/tutorial/handling-errors.md b/docs/pt/docs/tutorial/handling-errors.md
new file mode 100644
index 000000000..97a2e3eac
--- /dev/null
+++ b/docs/pt/docs/tutorial/handling-errors.md
@@ -0,0 +1,251 @@
+# Manipulação de erros
+
+Há diversas situações em que você precisa notificar um erro a um cliente que está utilizando a sua API.
+
+Esse cliente pode ser um browser com um frontend, o código de outra pessoa, um dispositivo IoT, etc.
+
+Pode ser que você precise comunicar ao cliente que:
+
+* O cliente não tem direitos para realizar aquela operação.
+* O cliente não tem acesso aquele recurso.
+* O item que o cliente está tentando acessar não existe.
+* etc.
+
+
+Nesses casos, você normalmente retornaria um **HTTP status code** próximo ao status code na faixa do status code **400** (do 400 ao 499).
+
+Isso é bastante similar ao caso do HTTP status code 200 (do 200 ao 299). Esses "200" status codes significam que, de algum modo, houve sucesso na requisição.
+
+Os status codes na faixa dos 400 significam que houve um erro por parte do cliente.
+
+Você se lembra de todos aqueles erros (e piadas) a respeito do "**404 Not Found**"?
+
+## Use o `HTTPException`
+
+Para retornar ao cliente *responses* HTTP com erros, use o `HTTPException`.
+
+### Import `HTTPException`
+
+```Python hl_lines="1"
+{!../../../docs_src/handling_errors/tutorial001.py!}
+```
+
+### Lance o `HTTPException` no seu código.
+
+`HTTPException`, ao fundo, nada mais é do que a conjunção entre uma exceção comum do Python e informações adicionais relevantes para APIs.
+
+E porque é uma exceção do Python, você não **retorna** (return) o `HTTPException`, você lança o (raise) no seu código.
+
+Isso também significa que, se você está escrevendo uma função de utilidade, a qual você está chamando dentro da sua função de operações de caminhos, e você lança o `HTTPException` dentro da função de utilidade, o resto do seu código não será executado dentro da função de operações de caminhos. Ao contrário, o `HTTPException` irá finalizar a requisição no mesmo instante e enviará o erro HTTP oriundo do `HTTPException` para o cliente.
+
+O benefício de lançar uma exceção em vez de retornar um valor ficará mais evidente na seção sobre Dependências e Segurança.
+
+Neste exemplo, quando o cliente pede, na requisição, por um item cujo ID não existe, a exceção com o status code `404` é lançada:
+
+```Python hl_lines="11"
+{!../../../docs_src/handling_errors/tutorial001.py!}
+```
+
+### A response resultante
+
+
+Se o cliente faz uma requisição para `http://example.com/items/foo` (um `item_id` `"foo"`), esse cliente receberá um HTTP status code 200, e uma resposta JSON:
+
+
+```
+{
+ "item": "The Foo Wrestlers"
+}
+```
+
+Mas se o cliente faz uma requisição para `http://example.com/items/bar` (ou seja, um não existente `item_id "bar"`), esse cliente receberá um HTTP status code 404 (o erro "não encontrado" — *not found error*), e uma resposta JSON:
+
+```JSON
+{
+ "detail": "Item not found"
+}
+```
+
+!!! tip "Dica"
+ Quando você lançar um `HTTPException`, você pode passar qualquer valor convertível em JSON como parâmetro de `detail`, e não apenas `str`.
+
+ Você pode passar um `dict` ou um `list`, etc.
+ Esses tipos de dados são manipulados automaticamente pelo **FastAPI** e convertidos em JSON.
+
+
+## Adicione headers customizados
+
+Há certas situações em que é bastante útil poder adicionar headers customizados no HTTP error. Exemplo disso seria adicionar headers customizados para tipos de segurança.
+
+Você provavelmente não precisará utilizar esses headers diretamente no seu código.
+
+Mas caso você precise, para um cenário mais complexo, você pode adicionar headers customizados:
+
+```Python hl_lines="14"
+{!../../../docs_src/handling_errors/tutorial002.py!}
+```
+
+## Instalando manipuladores de exceções customizados
+
+Você pode adicionar manipuladores de exceção customizados com a mesma seção de utilidade de exceções presentes no Starlette
+
+Digamos que você tenha uma exceção customizada `UnicornException` que você (ou uma biblioteca que você use) precise lançar (`raise`).
+
+Nesse cenário, se você precisa manipular essa exceção de modo global com o FastAPI, você pode adicionar um manipulador de exceção customizada com `@app.exception_handler()`.
+
+```Python hl_lines="5-7 13-18 24"
+{!../../../docs_src/handling_errors/tutorial003.py!}
+```
+
+Nesse cenário, se você fizer uma requisição para `/unicorns/yolo`, a *operação de caminho* vai lançar (`raise`) o `UnicornException`.
+
+Essa exceção será manipulada, contudo, pelo `unicorn_exception_handler`.
+
+Dessa forma você receberá um erro "limpo", com o HTTP status code `418` e um JSON com o conteúdo:
+
+```JSON
+{"message": "Oops! yolo did something. There goes a rainbow..."}
+```
+
+!!! note "Detalhes Técnicos"
+ Você também pode usar `from starlette.requests import Request` and `from starlette.responses import JSONResponse`.
+
+ **FastAPI** disponibiliza o mesmo `starlette.responses` através do `fastapi.responses` por conveniência ao desenvolvedor. Contudo, a maior parte das respostas disponíveis vem diretamente do Starlette. O mesmo acontece com o `Request`.
+
+## Sobrescreva o manipulador padrão de exceções
+
+**FastAPI** tem alguns manipuladores padrão de exceções.
+
+Esses manipuladores são os responsáveis por retornar o JSON padrão de respostas quando você lança (`raise`) o `HTTPException` e quando a requisição tem dados invalidos.
+
+Você pode sobrescrever esses manipuladores de exceção com os seus próprios manipuladores.
+
+## Sobrescreva exceções de validação da requisição
+
+Quando a requisição contém dados inválidos, **FastAPI** internamente lança para o `RequestValidationError`.
+
+Para sobrescrevê-lo, importe o `RequestValidationError` e use-o com o `@app.exception_handler(RequestValidationError)` para decorar o manipulador de exceções.
+
+```Python hl_lines="2 14-16"
+{!../../../docs_src/handling_errors/tutorial004.py!}
+```
+
+Se você for ao `/items/foo`, em vez de receber o JSON padrão com o erro:
+
+```JSON
+{
+ "detail": [
+ {
+ "loc": [
+ "path",
+ "item_id"
+ ],
+ "msg": "value is not a valid integer",
+ "type": "type_error.integer"
+ }
+ ]
+}
+```
+
+você receberá a versão em texto:
+
+```
+1 validation error
+path -> item_id
+ value is not a valid integer (type=type_error.integer)
+```
+
+### `RequestValidationError` vs `ValidationError`
+
+!!! warning "Aviso"
+ Você pode pular estes detalhes técnicos caso eles não sejam importantes para você neste momento.
+
+`RequestValidationError` é uma subclasse do `ValidationError` existente no Pydantic.
+
+**FastAPI** faz uso dele para que você veja o erro no seu log, caso você utilize um modelo de Pydantic em `response_model`, e seus dados tenham erro.
+
+Contudo, o cliente ou usuário não terão acesso a ele. Ao contrário, o cliente receberá um "Internal Server Error" com o HTTP status code `500`.
+
+E assim deve ser porque seria um bug no seu código ter o `ValidationError` do Pydantic na sua *response*, ou em qualquer outro lugar do seu código (que não na requisição do cliente).
+
+E enquanto você conserta o bug, os clientes / usuários não deveriam ter acesso às informações internas do erro, porque, desse modo, haveria exposição de uma vulnerabilidade de segurança.
+
+Do mesmo modo, você pode sobreescrever o `HTTPException`.
+
+Por exemplo, você pode querer retornar uma *response* em *plain text* ao invés de um JSON para os seguintes erros:
+
+```Python hl_lines="3-4 9-11 22"
+{!../../../docs_src/handling_errors/tutorial004.py!}
+```
+
+!!! note "Detalhes Técnicos"
+ Você pode usar `from starlette.responses import PlainTextResponse`.
+
+ **FastAPI** disponibiliza o mesmo `starlette.responses` como `fastapi.responses`, como conveniência a você, desenvolvedor. Contudo, a maior parte das respostas disponíveis vem diretamente do Starlette.
+
+
+### Use o body do `RequestValidationError`.
+
+O `RequestValidationError` contém o `body` que ele recebeu de dados inválidos.
+
+Você pode utilizá-lo enquanto desenvolve seu app para conectar o *body* e debugá-lo, e assim retorná-lo ao usuário, etc.
+
+Tente enviar um item inválido como este:
+
+```JSON
+{
+ "title": "towel",
+ "size": "XL"
+}
+```
+
+Você receberá uma *response* informando-o de que a data é inválida, e contendo o *body* recebido:
+
+```JSON hl_lines="12-15"
+{
+ "detail": [
+ {
+ "loc": [
+ "body",
+ "size"
+ ],
+ "msg": "value is not a valid integer",
+ "type": "type_error.integer"
+ }
+ ],
+ "body": {
+ "title": "towel",
+ "size": "XL"
+ }
+}
+```
+
+#### O `HTTPException` do FastAPI vs o `HTTPException` do Starlette.
+
+O **FastAPI** tem o seu próprio `HTTPException`.
+
+E a classe de erro `HTTPException` do **FastAPI** herda da classe de erro do `HTTPException` do Starlette.
+
+A diferença entre os dois é a de que o `HTTPException` do **FastAPI** permite que você adicione *headers* que serão incluídos nas *responses*.
+
+Esses *headers* são necessários/utilizados internamente pelo OAuth 2.0 e também por outras utilidades de segurança.
+
+Portanto, você pode continuar lançando o `HTTPException` do **FastAPI** normalmente no seu código.
+
+Porém, quando você registrar um manipulador de exceção, você deve registrá-lo através do `HTTPException` do Starlette.
+
+Dessa forma, se qualquer parte do código interno, extensão ou plug-in do Starlette lançar o `HTTPException`, o seu manipulador de exceção poderá capturar esse lançamento e tratá-lo.
+
+```Python
+from starlette.exceptions import HTTPException as StarletteHTTPException
+```
+
+### Re-use os manipulares de exceção do **FastAPI**
+
+Se você quer usar a exceção em conjunto com o mesmo manipulador de exceção *default* do **FastAPI**, você pode importar e re-usar esses manipuladores de exceção do `fastapi.exception_handlers`:
+
+```Python hl_lines="2-5 15 21"
+{!../../../docs_src/handling_errors/tutorial006.py!}
+```
+
+Nesse exemplo você apenas imprime (`print`) o erro com uma mensagem expressiva. Mesmo assim, dá para pegar a ideia. Você pode usar a exceção e então apenas re-usar o manipulador de exceção *default*.
diff --git a/docs/pt/docs/tutorial/header-params.md b/docs/pt/docs/tutorial/header-params.md
new file mode 100644
index 000000000..94ee784cd
--- /dev/null
+++ b/docs/pt/docs/tutorial/header-params.md
@@ -0,0 +1,128 @@
+# Parâmetros de Cabeçalho
+
+Você pode definir parâmetros de Cabeçalho da mesma maneira que define paramêtros com `Query`, `Path` e `Cookie`.
+
+## importe `Header`
+
+Primeiro importe `Header`:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="3"
+ {!> ../../../docs_src/header_params/tutorial001.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="1"
+ {!> ../../../docs_src/header_params/tutorial001_py310.py!}
+ ```
+
+## Declare parâmetros de `Header`
+
+Então declare os paramêtros de cabeçalho usando a mesma estrutura que em `Path`, `Query` e `Cookie`.
+
+O primeiro valor é o valor padrão, você pode passar todas as validações adicionais ou parâmetros de anotação:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="9"
+ {!> ../../../docs_src/header_params/tutorial001.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="7"
+ {!> ../../../docs_src/header_params/tutorial001_py310.py!}
+ ```
+
+!!! note "Detalhes Técnicos"
+ `Header` é uma classe "irmã" de `Path`, `Query` e `Cookie`. Ela também herda da mesma classe em comum `Param`.
+
+ Mas lembre-se que quando você importa `Query`, `Path`, `Header`, e outras de `fastapi`, elas são na verdade funções que retornam classes especiais.
+
+!!! info
+ Para declarar headers, você precisa usar `Header`, caso contrário, os parâmetros seriam interpretados como parâmetros de consulta.
+
+## Conversão automática
+
+`Header` tem algumas funcionalidades a mais em relação a `Path`, `Query` e `Cookie`.
+
+A maioria dos cabeçalhos padrão são separados pelo caractere "hífen", também conhecido como "sinal de menos" (`-`).
+
+Mas uma variável como `user-agent` é inválida em Python.
+
+Portanto, por padrão, `Header` converterá os caracteres de nomes de parâmetros de sublinhado (`_`) para hífen (`-`) para extrair e documentar os cabeçalhos.
+
+Além disso, os cabeçalhos HTTP não diferenciam maiúsculas de minúsculas, portanto, você pode declará-los com o estilo padrão do Python (também conhecido como "snake_case").
+
+Portanto, você pode usar `user_agent` como faria normalmente no código Python, em vez de precisar colocar as primeiras letras em maiúsculas como `User_Agent` ou algo semelhante.
+
+Se por algum motivo você precisar desabilitar a conversão automática de sublinhados para hífens, defina o parâmetro `convert_underscores` de `Header` para `False`:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="10"
+ {!> ../../../docs_src/header_params/tutorial002.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="8"
+ {!> ../../../docs_src/header_params/tutorial002_py310.py!}
+ ```
+
+!!! warning "Aviso"
+ Antes de definir `convert_underscores` como `False`, lembre-se de que alguns proxies e servidores HTTP não permitem o uso de cabeçalhos com sublinhados.
+
+## Cabeçalhos duplicados
+
+É possível receber cabeçalhos duplicados. Isso significa, o mesmo cabeçalho com vários valores.
+
+Você pode definir esses casos usando uma lista na declaração de tipo.
+
+Você receberá todos os valores do cabeçalho duplicado como uma `list` Python.
+
+Por exemplo, para declarar um cabeçalho de `X-Token` que pode aparecer mais de uma vez, você pode escrever:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="9"
+ {!> ../../../docs_src/header_params/tutorial003.py!}
+ ```
+
+=== "Python 3.9 and above"
+
+ ```Python hl_lines="9"
+ {!> ../../../docs_src/header_params/tutorial003_py39.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="7"
+ {!> ../../../docs_src/header_params/tutorial003_py310.py!}
+ ```
+
+Se você se comunicar com essa *operação de caminho* enviando dois cabeçalhos HTTP como:
+
+```
+X-Token: foo
+X-Token: bar
+```
+
+A resposta seria como:
+
+```JSON
+{
+ "X-Token values": [
+ "bar",
+ "foo"
+ ]
+}
+```
+
+## Recapitulando
+
+Declare cabeçalhos com `Header`, usando o mesmo padrão comum que utiliza-se em `Query`, `Path` e `Cookie`.
+
+E não se preocupe com sublinhados em suas variáveis, FastAPI cuidará da conversão deles.
diff --git a/docs/pt/docs/tutorial/index.md b/docs/pt/docs/tutorial/index.md
index f93fd8d75..b1abd32bc 100644
--- a/docs/pt/docs/tutorial/index.md
+++ b/docs/pt/docs/tutorial/index.md
@@ -43,7 +43,7 @@ Para o tutorial, você deve querer instalá-lo com todas as dependências e recu
```console
-$ pip install fastapi[all]
+$ pip install "fastapi[all]"
---> 100%
```
@@ -64,7 +64,7 @@ $ pip install fastapi[all]
Também instale o `uvicorn` para funcionar como servidor:
```
- pip install uvicorn[standard]
+ pip install "uvicorn[standard]"
```
E o mesmo para cada dependência opcional que você quiser usar.
diff --git a/docs/pt/docs/tutorial/path-params.md b/docs/pt/docs/tutorial/path-params.md
index 20913a564..5de3756ed 100644
--- a/docs/pt/docs/tutorial/path-params.md
+++ b/docs/pt/docs/tutorial/path-params.md
@@ -72,7 +72,7 @@ O mesmo erro apareceria se você tivesse fornecido um `float` ao invés de um `i
## Documentação
-Quando você abrir o seu navegador em http://127.0.0.1:8000/docs, você verá de forma automática e interativa a documtação da API como:
+Quando você abrir o seu navegador em http://127.0.0.1:8000/docs, você verá de forma automática e interativa a documentação da API como:
diff --git a/docs/pt/docs/tutorial/query-params-str-validations.md b/docs/pt/docs/tutorial/query-params-str-validations.md
index baac5f493..9a9e071db 100644
--- a/docs/pt/docs/tutorial/query-params-str-validations.md
+++ b/docs/pt/docs/tutorial/query-params-str-validations.md
@@ -8,12 +8,12 @@ Vamos utilizar essa aplicação como exemplo:
{!../../../docs_src/query_params_str_validations/tutorial001.py!}
```
-O parâmetro de consulta `q` é do tipo `Optional[str]`, o que significa que é do tipo `str` mas que também pode ser `None`, e de fato, o valor padrão é `None`, então o FastAPI saberá que não é obrigatório.
+O parâmetro de consulta `q` é do tipo `Union[str, None]`, o que significa que é do tipo `str` mas que também pode ser `None`, e de fato, o valor padrão é `None`, então o FastAPI saberá que não é obrigatório.
!!! note "Observação"
O FastAPI saberá que o valor de `q` não é obrigatório por causa do valor padrão `= None`.
- O `Optional` em `Optional[str]` não é usado pelo FastAPI, mas permitirá que seu editor lhe dê um melhor suporte e detecte erros.
+ O `Union` em `Union[str, None]` não é usado pelo FastAPI, mas permitirá que seu editor lhe dê um melhor suporte e detecte erros.
## Validação adicional
@@ -35,18 +35,18 @@ Agora utilize-o como valor padrão do seu parâmetro, definindo o parâmetro `ma
{!../../../docs_src/query_params_str_validations/tutorial002.py!}
```
-Note que substituímos o valor padrão de `None` para `Query(None)`, o primeiro parâmetro de `Query` serve para o mesmo propósito: definir o valor padrão do parâmetro.
+Note que substituímos o valor padrão de `None` para `Query(default=None)`, o primeiro parâmetro de `Query` serve para o mesmo propósito: definir o valor padrão do parâmetro.
Então:
```Python
-q: Optional[str] = Query(None)
+q: Union[str, None] = Query(default=None)
```
...Torna o parâmetro opcional, da mesma maneira que:
```Python
-q: Optional[str] = None
+q: Union[str, None] = None
```
Mas o declara explicitamente como um parâmetro de consulta.
@@ -61,17 +61,17 @@ Mas o declara explicitamente como um parâmetro de consulta.
Ou com:
```Python
- = Query(None)
+ = Query(default=None)
```
E irá utilizar o `None` para detectar que o parâmetro de consulta não é obrigatório.
- O `Optional` é apenas para permitir que seu editor de texto lhe dê um melhor suporte.
+ O `Union` é apenas para permitir que seu editor de texto lhe dê um melhor suporte.
Então, podemos passar mais parâmetros para `Query`. Neste caso, o parâmetro `max_length` que se aplica a textos:
```Python
-q: str = Query(None, max_length=50)
+q: str = Query(default=None, max_length=50)
```
Isso irá validar os dados, mostrar um erro claro quando os dados forem inválidos, e documentar o parâmetro na *operação de rota* do esquema OpenAPI..
@@ -80,7 +80,7 @@ Isso irá validar os dados, mostrar um erro claro quando os dados forem inválid
Você também pode incluir um parâmetro `min_length`:
-```Python hl_lines="9"
+```Python hl_lines="10"
{!../../../docs_src/query_params_str_validations/tutorial003.py!}
```
@@ -88,7 +88,7 @@ Você também pode incluir um parâmetro `min_length`:
Você pode definir uma expressão regular que combine com um padrão esperado pelo parâmetro:
-```Python hl_lines="10"
+```Python hl_lines="11"
{!../../../docs_src/query_params_str_validations/tutorial004.py!}
```
@@ -126,13 +126,13 @@ q: str
em vez desta:
```Python
-q: Optional[str] = None
+q: Union[str, None] = None
```
Mas agora nós o estamos declarando como `Query`, conforme abaixo:
```Python
-q: Optional[str] = Query(None, min_length=3)
+q: Union[str, None] = Query(default=None, min_length=3)
```
Então, quando você precisa declarar um parâmetro obrigatório utilizando o `Query`, você pode utilizar `...` como o primeiro argumento:
diff --git a/docs/pt/docs/tutorial/query-params.md b/docs/pt/docs/tutorial/query-params.md
new file mode 100644
index 000000000..189724396
--- /dev/null
+++ b/docs/pt/docs/tutorial/query-params.md
@@ -0,0 +1,224 @@
+# Parâmetros de Consulta
+
+Quando você declara outros parâmetros na função que não fazem parte dos parâmetros da rota, esses parâmetros são automaticamente interpretados como parâmetros de "consulta".
+
+```Python hl_lines="9"
+{!../../../docs_src/query_params/tutorial001.py!}
+```
+
+A consulta é o conjunto de pares chave-valor que vai depois de `?` na URL, separado pelo caractere `&`.
+
+Por exemplo, na URL:
+
+```
+http://127.0.0.1:8000/items/?skip=0&limit=10
+```
+
+...os parâmetros da consulta são:
+
+* `skip`: com o valor `0`
+* `limit`: com o valor `10`
+
+Como eles são parte da URL, eles são "naturalmente" strings.
+
+Mas quando você declara eles com os tipos do Python (no exemplo acima, como `int`), eles são convertidos para aquele tipo e validados em relação a ele.
+
+Todo o processo que era aplicado para parâmetros de rota também é aplicado para parâmetros de consulta:
+
+* Suporte do editor (obviamente)
+* "Parsing" de dados
+* Validação de dados
+* Documentação automática
+
+## Valores padrão
+
+Como os parâmetros de consulta não são uma parte fixa da rota, eles podem ser opcionais e podem ter valores padrão.
+
+No exemplo acima eles tem valores padrão de `skip=0` e `limit=10`.
+
+Então, se você for até a URL:
+
+```
+http://127.0.0.1:8000/items/
+```
+
+Seria o mesmo que ir para:
+
+```
+http://127.0.0.1:8000/items/?skip=0&limit=10
+```
+
+Mas, se por exemplo você for para:
+
+```
+http://127.0.0.1:8000/items/?skip=20
+```
+
+Os valores dos parâmetros na sua função serão:
+
+* `skip=20`: Por que você definiu isso na URL
+* `limit=10`: Por que esse era o valor padrão
+
+## Parâmetros opcionais
+
+Da mesma forma, você pode declarar parâmetros de consulta opcionais, definindo o valor padrão para `None`:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="9"
+ {!> ../../../docs_src/query_params/tutorial002.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="7"
+ {!> ../../../docs_src/query_params/tutorial002_py310.py!}
+ ```
+
+Nesse caso, o parâmetro da função `q` será opcional, e `None` será o padrão.
+
+!!! check "Verificar"
+ Você também pode notar que o **FastAPI** é esperto o suficiente para perceber que o parâmetro da rota `item_id` é um parâmetro da rota, e `q` não é, portanto, `q` é o parâmetro de consulta.
+
+
+## Conversão dos tipos de parâmetros de consulta
+
+Você também pode declarar tipos `bool`, e eles serão convertidos:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="9"
+ {!> ../../../docs_src/query_params/tutorial003.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="7"
+ {!> ../../../docs_src/query_params/tutorial003_py310.py!}
+ ```
+
+Nesse caso, se você for para:
+
+```
+http://127.0.0.1:8000/items/foo?short=1
+```
+
+ou
+
+```
+http://127.0.0.1:8000/items/foo?short=True
+```
+
+ou
+
+```
+http://127.0.0.1:8000/items/foo?short=true
+```
+
+ou
+
+```
+http://127.0.0.1:8000/items/foo?short=on
+```
+
+ou
+
+```
+http://127.0.0.1:8000/items/foo?short=yes
+```
+
+ou qualquer outra variação (tudo em maiúscula, primeira letra em maiúscula, etc), a sua função vai ver o parâmetro `short` com um valor `bool` de `True`. Caso contrário `False`.
+
+## Múltiplos parâmetros de rota e consulta
+
+Você pode declarar múltiplos parâmetros de rota e parâmetros de consulta ao mesmo tempo, o **FastAPI** vai saber o quê é o quê.
+
+E você não precisa declarar eles em nenhuma ordem específica.
+
+Eles serão detectados pelo nome:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="8 10"
+ {!> ../../../docs_src/query_params/tutorial004.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="6 8"
+ {!> ../../../docs_src/query_params/tutorial004_py310.py!}
+ ```
+
+## Parâmetros de consulta obrigatórios
+
+Quando você declara um valor padrão para parâmetros que não são de rota (até agora, nós vimos apenas parâmetros de consulta), então eles não são obrigatórios.
+
+Caso você não queira adicionar um valor específico mas queira apenas torná-lo opcional, defina o valor padrão como `None`.
+
+Porém, quando você quiser fazer com que o parâmetro de consulta seja obrigatório, você pode simplesmente não declarar nenhum valor como padrão.
+
+```Python hl_lines="6-7"
+{!../../../docs_src/query_params/tutorial005.py!}
+```
+
+Aqui o parâmetro de consulta `needy` é um valor obrigatório, do tipo `str`.
+
+Se você abrir no seu navegador a URL:
+
+```
+http://127.0.0.1:8000/items/foo-item
+```
+
+... sem adicionar o parâmetro obrigatório `needy`, você verá um erro como:
+
+```JSON
+{
+ "detail": [
+ {
+ "loc": [
+ "query",
+ "needy"
+ ],
+ "msg": "field required",
+ "type": "value_error.missing"
+ }
+ ]
+}
+```
+
+Como `needy` é um parâmetro obrigatório, você precisaria defini-lo na URL:
+
+```
+http://127.0.0.1:8000/items/foo-item?needy=sooooneedy
+```
+
+...isso deve funcionar:
+
+```JSON
+{
+ "item_id": "foo-item",
+ "needy": "sooooneedy"
+}
+```
+
+E claro, você pode definir alguns parâmetros como obrigatórios, alguns possuindo um valor padrão, e outros sendo totalmente opcionais:
+
+=== "Python 3.6 and above"
+
+ ```Python hl_lines="10"
+ {!> ../../../docs_src/query_params/tutorial006.py!}
+ ```
+
+=== "Python 3.10 and above"
+
+ ```Python hl_lines="8"
+ {!> ../../../docs_src/query_params/tutorial006_py310.py!}
+ ```
+Nesse caso, existem 3 parâmetros de consulta:
+
+* `needy`, um `str` obrigatório.
+* `skip`, um `int` com o valor padrão `0`.
+* `limit`, um `int` opcional.
+
+!!! tip "Dica"
+ Você também poderia usar `Enum` da mesma forma que com [Path Parameters](path-params.md#predefined-values){.internal-link target=_blank}.
diff --git a/docs/pt/docs/tutorial/security/first-steps.md b/docs/pt/docs/tutorial/security/first-steps.md
new file mode 100644
index 000000000..ed07d1c96
--- /dev/null
+++ b/docs/pt/docs/tutorial/security/first-steps.md
@@ -0,0 +1,181 @@
+# Segurança - Primeiros Passos
+
+Vamos imaginar que você tem a sua API **backend** em algum domínio.
+
+E você tem um **frontend** em outro domínio ou em um path diferente no mesmo domínio (ou em uma aplicação mobile).
+
+E você quer uma maneira de o frontend autenticar o backend, usando um **username** e **senha**.
+
+Nós podemos usar o **OAuth2** junto com o **FastAPI**.
+
+Mas, vamos poupar-lhe o tempo de ler toda a especificação apenas para achar as pequenas informações que você precisa.
+
+Vamos usar as ferramentas fornecidas pela **FastAPI** para lidar com segurança.
+
+## Como Parece
+
+Vamos primeiro usar o código e ver como funciona, e depois voltaremos para entender o que está acontecendo.
+
+## Crie um `main.py`
+Copie o exemplo em um arquivo `main.py`:
+
+```Python
+{!../../../docs_src/security/tutorial001.py!}
+```
+
+## Execute-o
+
+!!! informação
+ Primeiro, instale `python-multipart`.
+
+ Ex: `pip install python-multipart`.
+
+ Isso ocorre porque o **OAuth2** usa "dados de um formulário" para mandar o **username** e **senha**.
+
+Execute esse exemplo com:
+
+
+
+```console
+$ uvicorn main:app --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+```
+
+
+
+## Verifique-o
+
+Vá até a documentação interativa em: http://127.0.0.1:8000/docs.
+
+Você verá algo deste tipo:
+
+
+
+!!! marque o "botão de Autorizar!"
+ Você já tem um novo "botão de autorizar!".
+
+ E seu *path operation* tem um pequeno cadeado no canto superior direito que você pode clicar.
+
+E se você clicar, você terá um pequeno formulário de autorização para digitar o `username` e `senha` (e outros campos opcionais):
+
+
+
+!!! nota
+ Não importa o que você digita no formulário, não vai funcionar ainda. Mas nós vamos chegar lá.
+
+Claro que este não é o frontend para os usuários finais, mas é uma ótima ferramenta automática para documentar interativamente toda sua API.
+
+Pode ser usado pelo time de frontend (que pode ser você no caso).
+
+Pode ser usado por aplicações e sistemas third party (de terceiros).
+
+E também pode ser usada por você mesmo, para debugar, checar e testar a mesma aplicação.
+
+## O Fluxo da `senha`
+
+Agora vamos voltar um pouco e entender o que é isso tudo.
+
+O "fluxo" da `senha` é um dos caminhos ("fluxos") definidos no OAuth2, para lidar com a segurança e autenticação.
+
+OAuth2 foi projetado para que o backend ou a API pudesse ser independente do servidor que autentica o usuário.
+
+Mas nesse caso, a mesma aplicação **FastAPI** irá lidar com a API e a autenticação.
+
+Então, vamos rever de um ponto de vista simplificado:
+
+* O usuário digita o `username` e a `senha` no frontend e aperta `Enter`.
+* O frontend (rodando no browser do usuário) manda o `username` e a `senha` para uma URL específica na sua API (declarada com `tokenUrl="token"`).
+* A API checa aquele `username` e `senha`, e responde com um "token" (nós não implementamos nada disso ainda).
+ * Um "token" é apenas uma string com algum conteúdo que nós podemos utilizar mais tarde para verificar o usuário.
+ * Normalmente, um token é definido para expirar depois de um tempo.
+ * Então, o usuário terá que se logar de novo depois de um tempo.
+ * E se o token for roubado, o risco é menor. Não é como se fosse uma chave permanente que vai funcionar para sempre (na maioria dos casos).
+ * O frontend armazena aquele token temporariamente em algum lugar.
+ * O usuário clica no frontend para ir à outra seção daquele frontend do aplicativo web.
+ * O frontend precisa buscar mais dados daquela API.
+ * Mas precisa de autenticação para aquele endpoint em específico.
+ * Então, para autenticar com nossa API, ele manda um header de `Autorização` com o valor `Bearer` mais o token.
+ * Se o token contém `foobar`, o conteúdo do header de `Autorização` será: `Bearer foobar`.
+
+## **FastAPI**'s `OAuth2PasswordBearer`
+
+**FastAPI** fornece várias ferramentas, em diferentes níveis de abstração, para implementar esses recursos de segurança.
+
+Neste exemplo, nós vamos usar o **OAuth2** com o fluxo de **Senha**, usando um token **Bearer**. Fazemos isso usando a classe `OAuth2PasswordBearer`.
+
+!!! informação
+ Um token "bearer" não é a única opção.
+
+ Mas é a melhor no nosso caso.
+
+ E talvez seja a melhor para a maioria dos casos, a não ser que você seja um especialista em OAuth2 e saiba exatamente o porquê de existir outras opções que se adequam melhor às suas necessidades.
+
+ Nesse caso, **FastAPI** também fornece as ferramentas para construir.
+
+Quando nós criamos uma instância da classe `OAuth2PasswordBearer`, nós passamos pelo parâmetro `tokenUrl` Esse parâmetro contém a URL que o client (o frontend rodando no browser do usuário) vai usar para mandar o `username` e `senha` para obter um token.
+
+```Python hl_lines="6"
+{!../../../docs_src/security/tutorial001.py!}
+```
+
+!!! dica
+ Esse `tokenUrl="token"` se refere a uma URL relativa que nós não criamos ainda. Como é uma URL relativa, é equivalente a `./token`.
+
+ Porque estamos usando uma URL relativa, se sua API estava localizada em `https://example.com/`, então irá referir-se à `https://example.com/token`. Mas se sua API estava localizada em `https://example.com/api/v1/`, então irá referir-se à `https://example.com/api/v1/token`.
+
+ Usar uma URL relativa é importante para garantir que sua aplicação continue funcionando, mesmo em um uso avançado tipo [Atrás de um Proxy](../../advanced/behind-a-proxy.md){.internal-link target=_blank}.
+
+Esse parâmetro não cria um endpoint / *path operation*, mas declara que a URL `/token` vai ser aquela que o client deve usar para obter o token. Essa informação é usada no OpenAPI, e depois na API Interativa de documentação de sistemas.
+
+Em breve também criaremos o atual path operation.
+
+!!! informação
+ Se você é um "Pythonista" muito rigoroso, você pode não gostar do estilo do nome do parâmetro `tokenUrl` em vez de `token_url`.
+
+ Isso ocorre porque está utilizando o mesmo nome que está nas especificações do OpenAPI. Então, se você precisa investigar mais sobre qualquer um desses esquemas de segurança, você pode simplesmente copiar e colar para encontrar mais informações sobre isso.
+
+A variável `oauth2_scheme` é um instância de `OAuth2PasswordBearer`, mas também é um "callable".
+
+Pode ser chamada de:
+
+```Python
+oauth2_scheme(some, parameters)
+```
+
+Então, pode ser usado com `Depends`.
+
+## Use-o
+
+Agora você pode passar aquele `oauth2_scheme` em uma dependência com `Depends`.
+
+```Python hl_lines="10"
+{!../../../docs_src/security/tutorial001.py!}
+```
+
+Esse dependência vai fornecer uma `str` que é atribuído ao parâmetro `token da *função do path operation*
+
+A **FastAPI** saberá que pode usar essa dependência para definir um "esquema de segurança" no esquema da OpenAPI (e na documentação da API automática).
+
+!!! informação "Detalhes técnicos"
+ **FastAPI** saberá que pode usar a classe `OAuth2PasswordBearer` (declarada na dependência) para definir o esquema de segurança na OpenAPI porque herda de `fastapi.security.oauth2.OAuth2`, que por sua vez herda de `fastapi.security.base.Securitybase`.
+
+ Todos os utilitários de segurança que se integram com OpenAPI (e na documentação da API automática) herdam de `SecurityBase`, é assim que **FastAPI** pode saber como integrá-los no OpenAPI.
+
+## O que ele faz
+
+Ele irá e olhará na requisição para aquele header de `Autorização`, verificará se o valor é `Bearer` mais algum token, e vai retornar o token como uma `str`
+
+Se ele não ver o header de `Autorização` ou o valor não tem um token `Bearer`, vai responder com um código de erro 401 (`UNAUTHORIZED`) diretamente.
+
+Você nem precisa verificar se o token existe para retornar um erro. Você pode ter certeza de que se a sua função for executada, ela terá um `str` nesse token.
+
+Você já pode experimentar na documentação interativa:
+
+
+
+Não estamos verificando a validade do token ainda, mas isso já é um começo
+
+## Recapitulando
+
+Então, em apenas 3 ou 4 linhas extras, você já tem alguma forma primitiva de segurança.
diff --git a/docs/pt/mkdocs.yml b/docs/pt/mkdocs.yml
index f202f306d..5e4ceb66f 100644
--- a/docs/pt/mkdocs.yml
+++ b/docs/pt/mkdocs.yml
@@ -5,13 +5,15 @@ theme:
name: material
custom_dir: overrides
palette:
- - scheme: default
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
primary: teal
accent: amber
toggle:
icon: material/lightbulb
name: Switch to light mode
- - scheme: slate
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
primary: teal
accent: amber
toggle:
@@ -40,15 +42,19 @@ nav:
- az: /az/
- de: /de/
- es: /es/
+ - fa: /fa/
- fr: /fr/
+ - he: /he/
- id: /id/
- it: /it/
- ja: /ja/
- ko: /ko/
+ - nl: /nl/
- pl: /pl/
- pt: /pt/
- ru: /ru/
- sq: /sq/
+ - sv: /sv/
- tr: /tr/
- uk: /uk/
- zh: /zh/
@@ -58,8 +64,14 @@ nav:
- tutorial/index.md
- tutorial/first-steps.md
- tutorial/path-params.md
+ - tutorial/query-params.md
+ - tutorial/body.md
- tutorial/body-fields.md
+ - tutorial/extra-data-types.md
- tutorial/query-params-str-validations.md
+ - tutorial/cookie-params.md
+ - tutorial/header-params.md
+ - tutorial/handling-errors.md
- Segurança:
- tutorial/security/index.md
- Guia de Usuário Avançado:
@@ -68,10 +80,12 @@ nav:
- deployment/index.md
- deployment/versions.md
- deployment/https.md
+ - deployment/deta.md
- alternatives.md
- history-design-future.md
- external-links.md
- benchmarks.md
+- help-fastapi.md
markdown_extensions:
- toc:
permalink: true
@@ -89,6 +103,8 @@ markdown_extensions:
format: !!python/name:pymdownx.superfences.fence_code_format ''
- pymdownx.tabbed:
alternate_style: true
+- attr_list
+- md_in_html
extra:
analytics:
provider: google
@@ -117,8 +133,12 @@ extra:
name: de
- link: /es/
name: es - español
+ - link: /fa/
+ name: fa
- link: /fr/
name: fr - français
+ - link: /he/
+ name: he
- link: /id/
name: id
- link: /it/
@@ -127,6 +147,8 @@ extra:
name: ja - 日本語
- link: /ko/
name: ko - 한국어
+ - link: /nl/
+ name: nl
- link: /pl/
name: pl
- link: /pt/
@@ -135,6 +157,8 @@ extra:
name: ru - русский язык
- link: /sq/
name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
- link: /tr/
name: tr - Türkçe
- link: /uk/
diff --git a/docs/ru/docs/async.md b/docs/ru/docs/async.md
new file mode 100644
index 000000000..4c44fc22d
--- /dev/null
+++ b/docs/ru/docs/async.md
@@ -0,0 +1,505 @@
+# Конкурентность и async / await
+
+Здесь приведена подробная информация об использовании синтаксиса `async def` при написании *функций обработки пути*, а также рассмотрены основы асинхронного программирования, конкурентности и параллелизма.
+
+## Нет времени?
+
+TL;DR:
+
+Допустим, вы используете сторонюю библиотеку, которая требует вызова с ключевым словом `await`:
+
+```Python
+results = await some_library()
+```
+
+В этом случае *функции обработки пути* необходимо объявлять с использованием синтаксиса `async def`:
+
+```Python hl_lines="2"
+@app.get('/')
+async def read_results():
+ results = await some_library()
+ return results
+```
+
+!!! note
+ `await` можно использовать только внутри функций, объявленных с использованием `async def`.
+
+---
+
+Если вы обращаетесь к сторонней библиотеке, которая с чем-то взаимодействует
+(с базой данных, API, файловой системой и т. д.), и не имеет поддержки синтаксиса `await`
+(что относится сейчас к большинству библиотек для работы с базами данных), то
+объявляйте *функции обработки пути* обычным образом с помощью `def`, например:
+
+```Python hl_lines="2"
+@app.get('/')
+def results():
+ results = some_library()
+ return results
+```
+
+---
+
+Если вашему приложению (странным образом) не нужно ни с чем взаимодействовать и, соответственно,
+ожидать ответа, используйте `async def`.
+
+---
+
+Если вы не уверены, используйте обычный синтаксис `def`.
+
+---
+
+**Примечание**: при необходимости можно смешивать `def` и `async def` в *функциях обработки пути*
+и использовать в каждом случае наиболее подходящий синтаксис. А FastAPI сделает с этим всё, что нужно.
+
+В любом из описанных случаев FastAPI работает асинхронно и очень быстро.
+
+Однако придерживаясь указанных советов, можно получить дополнительную оптимизацию производительности.
+
+## Технические подробности
+
+Современные версии Python поддерживают разработку так называемого **"асинхронного кода"** посредством написания **"сопрограмм"** с использованием синтаксиса **`async` и `await`**.
+
+Ниже разберём эту фразу по частям:
+
+* **Асинхронный код**
+* **`async` и `await`**
+* **Сопрограммы**
+
+## Асинхронный код
+
+Асинхронный код означает, что в языке 💬 есть возможность сообщить машине / программе 🤖,
+что в определённой точке кода ей 🤖 нужно будет ожидать завершения выполнения *чего-то ещё* в другом месте. Допустим это *что-то ещё* называется "медленный файл" 📝.
+
+И пока мы ждём завершения работы с "медленным файлом" 📝, компьютер может переключиться для выполнения других задач.
+
+Но при каждой возможности компьютер / программа 🤖 будет возвращаться обратно. Например, если он 🤖 опять окажется в режиме ожидания, или когда закончит всю работу. В этом случае компьютер 🤖 проверяет, не завершена ли какая-нибудь из текущих задач.
+
+Потом он 🤖 берёт первую выполненную задачу (допустим, наш "медленный файл" 📝) и продолжает работу, производя с ней необходимые действия.
+
+Вышеупомянутое "что-то ещё", завершения которого приходится ожидать, обычно относится к достаточно "медленным" операциям I/O (по сравнению со скоростью работы процессора и оперативной памяти), например:
+
+* отправка данных от клиента по сети
+* получение клиентом данных, отправленных вашей программой по сети
+* чтение системой содержимого файла с диска и передача этих данных программе
+* запись на диск данных, которые программа передала системе
+* обращение к удалённому API
+* ожидание завершения операции с базой данных
+* получение результатов запроса к базе данных
+* и т. д.
+
+Поскольку в основном время тратится на ожидание выполнения операций I/O,
+их обычно называют операциями, ограниченными скоростью ввода-вывода.
+
+Код называют "асинхронным", потому что компьютеру / программе не требуется "синхронизироваться" с медленной задачей и,
+будучи в простое, ожидать момента её завершения, с тем чтобы забрать результат и продолжить работу.
+
+Вместо этого в "асинхронной" системе завершённая задача может немного подождать (буквально несколько микросекунд),
+пока компьютер / программа занимается другими важными вещами, с тем чтобы потом вернуться,
+забрать результаты выполнения и начать их обрабатывать.
+
+"Синхронное" исполнение (в противовес "асинхронному") также называют "последовательным",
+потому что компьютер / программа последовательно выполняет все требуемые шаги перед тем, как перейти к следующей задаче,
+даже если в процессе приходится ждать.
+
+### Конкурентность и бургеры
+
+Тот **асинхронный** код, о котором идёт речь выше, иногда называют **"конкурентностью"**. Она отличается от **"параллелизма"**.
+
+Да, **конкурентность** и **параллелизм** подразумевают, что разные вещи происходят примерно в одно время.
+
+Но внутреннее устройство **конкурентности** и **параллелизма** довольно разное.
+
+Чтобы это понять, представьте такую картину:
+
+### Конкурентные бургеры
+
+
+
+Вы идёте со своей возлюбленной 😍 в фастфуд 🍔 и становитесь в очередь, в это время кассир 💁 принимает заказы у посетителей перед вами.
+
+Когда наконец подходит очередь, вы заказываете парочку самых вкусных и навороченных бургеров 🍔, один для своей возлюбленной 😍, а другой себе.
+
+Отдаёте деньги 💸.
+
+Кассир 💁 что-то говорит поварам на кухне 👨🍳, теперь они знают, какие бургеры нужно будет приготовить 🍔
+(но пока они заняты бургерами предыдущих клиентов).
+
+Кассир 💁 отдаёт вам чек с номером заказа.
+
+В ожидании еды вы идёте со своей возлюбленной 😍 выбрать столик, садитесь и довольно продолжительное время общаетесь 😍
+(поскольку ваши бургеры самые навороченные, готовятся они не так быстро ✨🍔✨).
+
+Сидя за столиком с возлюбленной 😍 в ожидании бургеров 🍔, вы отлично проводите время,
+восхищаясь её великолепием, красотой и умом ✨😍✨.
+
+Всё ещё ожидая заказ и болтая со своей возлюбленной 😍, время от времени вы проверяете,
+какой номер горит над прилавком, и не подошла ли уже ваша очередь.
+
+И вот наконец настаёт этот момент, и вы идёте к стойке, чтобы забрать бургеры 🍔 и вернуться за столик.
+
+Вы со своей возлюбленной 😍 едите бургеры 🍔 и отлично проводите время ✨.
+
+---
+
+А теперь представьте, что в этой небольшой истории вы компьютер / программа 🤖.
+
+В очереди вы просто глазеете по сторонам 😴, ждёте и ничего особо "продуктивного" не делаете.
+Но очередь движется довольно быстро, поскольку кассир 💁 только принимает заказы (а не занимается приготовлением еды), так что ничего страшного.
+
+Когда подходит очередь вы наконец предпринимаете "продуктивные" действия 🤓: просматриваете меню, выбираете в нём что-то, узнаёте, что хочет ваша возлюбленная 😍, собираетесь оплатить 💸, смотрите, какую достали карту, проверяете, чтобы с вас списали верную сумму, и что в заказе всё верно и т. д.
+
+И хотя вы всё ещё не получили бургеры 🍔, ваша работа с кассиром 💁 ставится "на паузу" ⏸,
+поскольку теперь нужно ждать 🕙, когда заказ приготовят.
+
+Но отойдя с номерком от прилавка, вы садитесь за столик и можете переключить 🔀 внимание
+на свою возлюбленную 😍 и "работать" ⏯ 🤓 уже над этим. И вот вы снова очень
+"продуктивны" 🤓, мило болтаете вдвоём и всё такое 😍.
+
+В какой-то момент кассир 💁 поместит на табло ваш номер, подразумевая, что бургеры готовы 🍔, но вы не станете подскакивать как умалишённый, лишь только увидев на экране свою очередь. Вы уверены, что ваши бургеры 🍔 никто не утащит, ведь у вас свой номерок, а у других свой.
+
+Поэтому вы подождёте, пока возлюбленная 😍 закончит рассказывать историю (закончите текущую работу ⏯ / задачу в обработке 🤓),
+и мило улыбнувшись, скажете, что идёте забирать заказ ⏸.
+
+И вот вы подходите к стойке 🔀, к первоначальной задаче, которая уже завершена ⏯, берёте бургеры 🍔, говорите спасибо и относите заказ за столик. На этом заканчивается этап / задача взаимодействия с кассой ⏹.
+В свою очередь порождается задача "поедание бургеров" 🔀 ⏯, но предыдущая ("получение бургеров") завершена ⏹.
+
+### Параллельные бургеры
+
+Теперь представим, что вместо бургерной "Конкурентные бургеры" вы решили сходить в "Параллельные бургеры".
+
+И вот вы идёте со своей возлюбленной 😍 отведать параллельного фастфуда 🍔.
+
+Вы становитесь в очередь пока несколько (пусть будет 8) кассиров, которые по совместительству ещё и повары 👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳, принимают заказы у посетителей перед вами.
+
+При этом клиенты не отходят от стойки и ждут 🕙 получения еды, поскольку каждый
+из 8 кассиров идёт на кухню готовить бургеры 🍔, а только потом принимает следующий заказ.
+
+Наконец настаёт ваша очередь, и вы просите два самых навороченных бургера 🍔, один для дамы сердца 😍, а другой себе.
+
+Ни о чём не жалея, расплачиваетесь 💸.
+
+И кассир уходит на кухню 👨🍳.
+
+Вам приходится ждать перед стойкой 🕙, чтобы никто по случайности не забрал ваши бургеры 🍔, ведь никаких номерков у вас нет.
+
+Поскольку вы с возлюбленной 😍 хотите получить заказ вовремя 🕙, и следите за тем, чтобы никто не вклинился в очередь,
+у вас не получается уделять должного внимание своей даме сердца 😞.
+
+Это "синхронная" работа, вы "синхронизированы" с кассиром/поваром 👨🍳. Приходится ждать 🕙 у стойки,
+когда кассир/повар 👨🍳 закончит делать бургеры 🍔 и вручит вам заказ, иначе его случайно может забрать кто-то другой.
+
+Наконец кассир/повар 👨🍳 возвращается с бургерами 🍔 после невыносимо долгого ожидания 🕙 за стойкой.
+
+Вы скорее забираете заказ 🍔 и идёте с возлюбленной 😍 за столик.
+
+Там вы просто едите эти бургеры, и на этом всё 🍔 ⏹.
+
+Вам не особо удалось пообщаться, потому что большую часть времени 🕙 пришлось провести у кассы 😞.
+
+---
+
+В описанном сценарии вы компьютер / программа 🤖 с двумя исполнителями (вы и ваша возлюбленная 😍),
+на протяжении долгого времени 🕙 вы оба уделяете всё внимание ⏯ задаче "ждать на кассе".
+
+В этом ресторане быстрого питания 8 исполнителей (кассиров/поваров) 👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳.
+Хотя в бургерной конкурентного типа было всего два (один кассир и один повар) 💁 👨🍳.
+
+Несмотря на обилие работников, опыт в итоге получился не из лучших 😞.
+
+---
+
+Так бы выглядел аналог истории про бургерную 🍔 в "параллельном" мире.
+
+Вот более реалистичный пример. Представьте себе банк.
+
+До недавних пор в большинстве банков было несколько кассиров 👨💼👨💼👨💼👨💼 и длинные очереди 🕙🕙🕙🕙🕙🕙🕙🕙.
+
+Каждый кассир обслуживал одного клиента, потом следующего 👨💼⏯.
+
+Нужно было долгое время 🕙 стоять перед окошком вместе со всеми, иначе пропустишь свою очередь.
+
+Сомневаюсь, что у вас бы возникло желание прийти с возлюбленной 😍 в банк 🏦 оплачивать налоги.
+
+### Выводы о бургерах
+
+В нашей истории про поход в фастфуд за бургерами приходится много ждать 🕙,
+поэтому имеет смысл организовать конкурентную систему ⏸🔀⏯.
+
+И то же самое с большинством веб-приложений.
+
+Пользователей очень много, но ваш сервер всё равно вынужден ждать 🕙 запросы по их слабому интернет-соединению.
+
+Потом снова ждать 🕙, пока вернётся ответ.
+
+
+Это ожидание 🕙 измеряется микросекундами, но если всё сложить, то набегает довольно много времени.
+
+Вот почему есть смысл использовать асинхронное ⏸🔀⏯ программирование при построении веб-API.
+
+Большинство популярных фреймворков (включая Flask и Django) создавались
+до появления в Python новых возможностей асинхронного программирования. Поэтому
+их можно разворачивать с поддержкой параллельного исполнения или асинхронного
+программирования старого типа, которое не настолько эффективно.
+
+При том, что основная спецификация асинхронного взаимодействия Python с веб-сервером
+(ASGI)
+была разработана командой Django для внедрения поддержки веб-сокетов.
+
+Именно асинхронность сделала NodeJS таким популярным (несмотря на то, что он не параллельный),
+и в этом преимущество Go как языка программирования.
+
+И тот же уровень производительности даёт **FastAPI**.
+
+Поскольку можно использовать преимущества параллелизма и асинхронности вместе,
+вы получаете производительность лучше, чем у большинства протестированных NodeJS фреймворков
+и на уровне с Go, который является компилируемым языком близким к C (всё благодаря Starlette).
+
+### Получается, конкурентность лучше параллелизма?
+
+Нет! Мораль истории совсем не в этом.
+
+Конкурентность отличается от параллелизма. Она лучше в **конкретных** случаях, где много времени приходится на ожидание.
+Вот почему она зачастую лучше параллелизма при разработке веб-приложений. Но это не значит, что конкурентность лучше в любых сценариях.
+
+Давайте посмотрим с другой стороны, представьте такую картину:
+
+> Вам нужно убраться в большом грязном доме.
+
+*Да, это вся история*.
+
+---
+
+Тут не нужно нигде ждать 🕙, просто есть куча работы в разных частях дома.
+
+Можно организовать очередь как в примере с бургерами, сначала гостиная, потом кухня,
+но это ни на что не повлияет, поскольку вы нигде не ждёте 🕙, а просто трёте да моете.
+
+И понадобится одинаковое количество времени с очередью (конкурентностью) и без неё,
+и работы будет сделано тоже одинаковое количество.
+
+Однако в случае, если бы вы могли привести 8 бывших кассиров/поваров, а ныне уборщиков 👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳👩🍳👨🍳,
+и каждый из них (вместе с вами) взялся бы за свой участок дома,
+с такой помощью вы бы закончили намного быстрее, делая всю работу **параллельно**.
+
+В описанном сценарии каждый уборщик (включая вас) был бы исполнителем, занятым на своём участке работы.
+
+И поскольку большую часть времени выполнения занимает реальная работа (а не ожидание),
+а работу в компьютере делает ЦП,
+такие задачи называют ограниченными производительностью процессора.
+
+---
+
+Ограничение по процессору проявляется в операциях, где требуется выполнять сложные математические вычисления.
+
+Например:
+
+* Обработка **звука** или **изображений**.
+* **Компьютерное зрение**: изображение состоит из миллионов пикселей, в каждом пикселе 3 составляющих цвета,
+обработка обычно требует проведения расчётов по всем пикселям сразу.
+* **Машинное обучение**: здесь обычно требуется умножение "матриц" и "векторов".
+Представьте гигантскую таблицу с числами в Экселе, и все их надо одновременно перемножить.
+* **Глубокое обучение**: это область *машинного обучения*, поэтому сюда подходит то же описание.
+Просто у вас будет не одна таблица в Экселе, а множество. В ряде случаев используется
+специальный процессор для создания и / или использования построенных таким образом моделей.
+
+### Конкурентность + параллелизм: Веб + машинное обучение
+
+**FastAPI** предоставляет возможности конкуретного программирования,
+которое очень распространено в веб-разработке (именно этим славится NodeJS).
+
+Кроме того вы сможете использовать все преимущества параллелизма и
+многопроцессорности (когда несколько процессов работают параллельно),
+если рабочая нагрузка предполагает **ограничение по процессору**,
+как, например, в системах машинного обучения.
+
+Необходимо также отметить, что Python является главным языком в области
+**дата-сайенс**,
+машинного обучения и, особенно, глубокого обучения. Всё это делает FastAPI
+отличным вариантом (среди многих других) для разработки веб-API и приложений
+в области дата-сайенс / машинного обучения.
+
+Как добиться такого параллелизма в эксплуатации описано в разделе [Развёртывание](deployment/index.md){.internal-link target=_blank}.
+
+## `async` и `await`
+
+В современных версиях Python разработка асинхронного кода реализована очень интуитивно.
+Он выглядит как обычный "последовательный" код и самостоятельно выполняет "ожидание", когда это необходимо.
+
+Если некая операция требует ожидания перед тем, как вернуть результат, и
+поддерживает современные возможности Python, код можно написать следующим образом:
+
+```Python
+burgers = await get_burgers(2)
+```
+
+Главное здесь слово `await`. Оно сообщает интерпретатору, что необходимо дождаться ⏸
+пока `get_burgers(2)` закончит свои дела 🕙, и только после этого сохранить результат в `burgers`.
+Зная это, Python может пока переключиться на выполнение других задач 🔀 ⏯
+(например получение следующего запроса).
+
+Чтобы ключевое слово `await` сработало, оно должно находиться внутри функции,
+которая поддерживает асинхронность. Для этого вам просто нужно объявить её как `async def`:
+
+```Python hl_lines="1"
+async def get_burgers(number: int):
+ # Готовим бургеры по специальному асинхронному рецепту
+ return burgers
+```
+
+...вместо `def`:
+
+```Python hl_lines="2"
+# Это не асинхронный код
+def get_sequential_burgers(number: int):
+ # Готовим бургеры последовательно по шагам
+ return burgers
+```
+
+Объявление `async def` указывает интерпретатору, что внутри этой функции
+следует ожидать выражений `await`, и что можно поставить выполнение такой функции на "паузу" ⏸ и
+переключиться на другие задачи 🔀, с тем чтобы вернуться сюда позже.
+
+Если вы хотите вызвать функцию с `async def`, вам нужно "ожидать" её.
+Поэтому такое не сработает:
+
+```Python
+# Это не заработает, поскольку get_burgers объявлена с использованием async def
+burgers = get_burgers(2)
+```
+
+---
+
+Если сторонняя библиотека требует вызывать её с ключевым словом `await`,
+необходимо писать *функции обработки пути* с использованием `async def`, например:
+
+```Python hl_lines="2-3"
+@app.get('/burgers')
+async def read_burgers():
+ burgers = await get_burgers(2)
+ return burgers
+```
+
+### Технические подробности
+
+Как вы могли заметить, `await` может применяться только в функциях, объявленных с использованием `async def`.
+
+
+Но выполнение такой функции необходимо "ожидать" с помощью `await`.
+Это означает, что её можно вызвать только из другой функции, которая тоже объявлена с `async def`.
+
+Но как же тогда появилась первая курица? В смысле... как нам вызвать первую асинхронную функцию?
+
+При работе с **FastAPI** просто не думайте об этом, потому что "первой" функцией является ваша *функция обработки пути*,
+и дальше с этим разберётся FastAPI.
+
+Кроме того, если хотите, вы можете использовать синтаксис `async` / `await` и без FastAPI.
+
+### Пишите свой асинхронный код
+
+Starlette (и **FastAPI**) основаны на AnyIO, что делает их совместимыми как со стандартной библиотекой asyncio в Python, так и с Trio.
+
+В частности, вы можете напрямую использовать AnyIO в тех проектах, где требуется более сложная логика работы с конкурентностью.
+
+Даже если вы не используете FastAPI, вы можете писать асинхронные приложения с помощью AnyIO, чтобы они были максимально совместимыми и получали его преимущества (например *структурную конкурентность*).
+
+### Другие виды асинхронного программирования
+
+Стиль написания кода с `async` и `await` появился в языке Python относительно недавно.
+
+Но он сильно облегчает работу с асинхронным кодом.
+
+Ровно такой же синтаксис (ну или почти такой же) недавно был включён в современные версии JavaScript (в браузере и NodeJS).
+
+До этого поддержка асинхронного кода была реализована намного сложнее, и его было труднее воспринимать.
+
+В предыдущих версиях Python для этого использовались потоки или Gevent. Но такой код намного сложнее понимать, отлаживать и мысленно представлять.
+
+Что касается JavaScript (в браузере и NodeJS), раньше там использовали для этой цели
+"обратные вызовы". Что выливалось в
+ад обратных вызовов.
+
+## Сопрограммы
+
+**Корути́на** (или же сопрограмма) — это крутое словечко для именования той сущности,
+которую возвращает функция `async def`. Python знает, что её можно запустить, как и обычную функцию,
+но кроме того сопрограмму можно поставить на паузу ⏸ в том месте, где встретится слово `await`.
+
+Всю функциональность асинхронного программирования с использованием `async` и `await`
+часто обобщают словом "корутины". Они аналогичны "горутинам", ключевой особенности
+языка Go.
+
+## Заключение
+
+В самом начале была такая фраза:
+
+> Современные версии Python поддерживают разработку так называемого
+**"асинхронного кода"** посредством написания **"сопрограмм"** с использованием
+синтаксиса **`async` и `await`**.
+
+Теперь всё должно звучать понятнее. ✨
+
+На этом основана работа FastAPI (посредством Starlette), и именно это
+обеспечивает его высокую производительность.
+
+## Очень технические подробности
+
+!!! warning
+ Этот раздел читать не обязательно.
+
+ Здесь приводятся подробности внутреннего устройства **FastAPI**.
+
+ Но если вы обладаете техническими знаниями (корутины, потоки, блокировка и т. д.)
+ и вам интересно, как FastAPI обрабатывает `async def` в отличие от обычных `def`,
+ читайте дальше.
+
+### Функции обработки пути
+
+Когда вы объявляете *функцию обработки пути* обычным образом с ключевым словом `def`
+вместо `async def`, FastAPI ожидает её выполнения, запустив функцию во внешнем
+пуле потоков, а не напрямую (это бы заблокировало сервер).
+
+Если ранее вы использовали другой асинхронный фреймворк, который работает иначе,
+и привыкли объявлять простые вычислительные *функции* через `def` ради
+незначительного прироста скорости (порядка 100 наносекунд), обратите внимание,
+что с **FastAPI** вы получите противоположный эффект. В таком случае больше подходит
+`async def`, если только *функция обработки пути* не использует код, приводящий
+к блокировке I/O.
+
+
+
+Но в любом случае велика вероятность, что **FastAPI** [окажется быстрее](/#performance){.internal-link target=_blank}
+другого фреймворка (или хотя бы на уровне с ним).
+
+### Зависимости
+
+То же относится к зависимостям. Если это обычная функция `def`, а не `async def`,
+она запускается во внешнем пуле потоков.
+
+### Подзависимости
+
+Вы можете объявить множество ссылающихся друг на друга зависимостей и подзависимостей
+(в виде параметров при определении функции). Какие-то будут созданы с помощью `async def`,
+другие обычным образом через `def`, и такая схема вполне работоспособна. Функции,
+объявленные с помощью `def` будут запускаться на внешнем потоке (из пула),
+а не с помощью `await`.
+
+### Другие служебные функции
+
+Любые другие служебные функции, которые вы вызываете напрямую, можно объявлять
+с использованием `def` или `async def`. FastAPI не будет влиять на то, как вы
+их запускаете.
+
+Этим они отличаются от функций, которые FastAPI вызывает самостоятельно:
+*функции обработки пути* и зависимости.
+
+Если служебная функция объявлена с помощью `def`, она будет вызвана напрямую
+(как вы и написали в коде), а не в отдельном потоке. Если же она объявлена с
+помощью `async def`, её вызов должен осуществляться с ожиданием через `await`.
+
+---
+
+
+Ещё раз повторим, что все эти технические подробности полезны, только если вы специально их искали.
+
+В противном случае просто ознакомьтесь с основными принципами в разделе выше: Нет времени?.
diff --git a/docs/ru/docs/deployment/versions.md b/docs/ru/docs/deployment/versions.md
new file mode 100644
index 000000000..91b9038e9
--- /dev/null
+++ b/docs/ru/docs/deployment/versions.md
@@ -0,0 +1,87 @@
+# О версиях FastAPI
+
+**FastAPI** уже используется в продакшене во многих приложениях и системах. Покрытие тестами поддерживается на уровне 100%. Однако его разработка все еще продолжается.
+
+Часто добавляются новые функции, регулярно исправляются баги, код продолжает постоянно совершенствоваться.
+
+По указанным причинам текущие версии до сих пор `0.x.x`. Это говорит о том, что каждая версия может содержать обратно несовместимые изменения, следуя соглашению о Семантическом Версионировании.
+
+Уже сейчас вы можете создавать приложения в продакшене, используя **FastAPI** (и скорее всего так и делаете), главное убедиться в том, что вы используете версию, которая корректно работает с вашим кодом.
+
+## Закрепите вашу версию `fastapi`
+
+Первым делом вам следует "закрепить" конкретную последнюю используемую версию **FastAPI**, которая корректно работает с вашим приложением.
+
+Например, в своём приложении вы используете версию `0.45.0`.
+
+Если вы используете файл `requirements.txt`, вы можете указать версию следующим способом:
+
+```txt
+fastapi==0.45.0
+```
+
+это означает, что вы будете использовать именно версию `0.45.0`.
+
+Или вы можете закрепить версию следующим способом:
+
+```txt
+fastapi>=0.45.0,<0.46.0
+```
+
+это значит, что вы используете версии `0.45.0` или выше, но меньше чем `0.46.0`. Например, версия `0.45.2` все еще будет подходить.
+
+Если вы используете любой другой инструмент для управления зависимостями, например Poetry, Pipenv или др., у них у всех имеется способ определения специфической версии для ваших пакетов.
+
+## Доступные версии
+
+Вы можете посмотреть доступные версии (например, проверить последнюю на данный момент) в [примечаниях к выпуску](../release-notes.md){.internal-link target=_blank}.
+
+## О версиях
+
+Следуя соглашению о Семантическом Версионировании, любые версии ниже `1.0.0` потенциально могут добавить обратно несовместимые изменения.
+
+FastAPI следует соглашению в том, что любые изменения "ПАТЧ"-версии предназначены для исправления багов и внесения обратно совместимых изменений.
+
+!!! Подсказка
+ "ПАТЧ" - это последнее число. Например, в `0.2.3`, ПАТЧ-версия - это `3`.
+
+Итак, вы можете закрепить версию следующим образом:
+
+```txt
+fastapi>=0.45.0,<0.46.0
+```
+
+Обратно несовместимые изменения и новые функции добавляются в "МИНОРНЫЕ" версии.
+
+!!! Подсказка
+ "МИНОРНАЯ" версия - это число в середине. Например, в `0.2.3` МИНОРНАЯ версия - это `2`.
+
+## Обновление версий FastAPI
+
+Вам следует добавить тесты для вашего приложения.
+
+С помощью **FastAPI** это очень просто (благодаря Starlette), см. документацию: [Тестирование](../tutorial/testing.md){.internal-link target=_blank}
+
+После создания тестов вы можете обновить свою версию **FastAPI** до более новой. После этого следует убедиться, что ваш код работает корректно, запустив тесты.
+
+Если все работает корректно, или после внесения необходимых изменений все ваши тесты проходят, только тогда вы можете закрепить вашу новую версию `fastapi`.
+
+## О Starlette
+
+Не следует закреплять версию `starlette`.
+
+Разные версии **FastAPI** будут использовать более новые версии Starlette.
+
+Так что решение об используемой версии Starlette, вы можете оставить **FastAPI**.
+
+## О Pydantic
+
+Pydantic включает свои собственные тесты для **FastAPI**, так что новые версии Pydantic (выше `1.0.0`) всегда совместимы с FastAPI.
+
+Вы можете закрепить любую версию Pydantic, которая вам подходит, выше `1.0.0` и ниже `2.0.0`.
+
+Например:
+
+```txt
+pydantic>=1.2.0,<2.0.0
+```
diff --git a/docs/ru/docs/index.md b/docs/ru/docs/index.md
index 0c2506b87..448e2c707 100644
--- a/docs/ru/docs/index.md
+++ b/docs/ru/docs/index.md
@@ -1,50 +1,48 @@
-
-{!../../../docs/missing-translation.md!}
-
-
- FastAPI framework, high performance, easy to learn, fast to code, ready for production
+ Готовый к внедрению высокопроизводительный фреймворк, простой в изучении и разработке.
---
-**Documentation**: https://fastapi.tiangolo.com
+**Документация**: https://fastapi.tiangolo.com
-**Source Code**: https://github.com/tiangolo/fastapi
+**Исходный код**: https://github.com/tiangolo/fastapi
---
-FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints.
-
-The key features are:
+FastAPI — это современный, быстрый (высокопроизводительный) веб-фреймворк для создания API используя Python 3.6+, в основе которого лежит стандартная аннотация типов Python.
-* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance).
+Ключевые особенности:
-* **Fast to code**: Increase the speed to develop features by about 200% to 300%. *
-* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. *
-* **Intuitive**: Great editor support. Completion everywhere. Less time debugging.
-* **Easy**: Designed to be easy to use and learn. Less time reading docs.
-* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs.
-* **Robust**: Get production-ready code. With automatic interactive documentation.
-* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: OpenAPI (previously known as Swagger) and JSON Schema.
+* **Скорость**: Очень высокая производительность, на уровне **NodeJS** и **Go** (благодаря Starlette и Pydantic). [Один из самых быстрых фреймворков Python](#_10).
+* **Быстрота разработки**: Увеличьте скорость разработки примерно на 200–300%. *
+* **Меньше ошибок**: Сократите примерно на 40% количество ошибок, вызванных человеком (разработчиком). *
+* **Интуитивно понятный**: Отличная поддержка редактора. Автозавершение везде. Меньше времени на отладку.
+* **Лёгкость**: Разработан так, чтобы его было легко использовать и осваивать. Меньше времени на чтение документации.
+* **Краткость**: Сведите к минимуму дублирование кода. Каждый объявленный параметр - определяет несколько функций. Меньше ошибок.
+* **Надежность**: Получите готовый к работе код. С автоматической интерактивной документацией.
+* **На основе стандартов**: Основан на открытых стандартах API и полностью совместим с ними: OpenAPI (ранее известном как Swagger) и JSON Schema.
-* estimation based on tests on an internal development team, building production applications.
+* оценка на основе тестов внутренней команды разработчиков, создающих производственные приложения.
-## Sponsors
+## Спонсоры
@@ -59,66 +57,66 @@ The key features are:
-Other sponsors
+Другие спонсоры
-## Opinions
+## Отзывы
-"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._"
+"_В последнее время я много где использую **FastAPI**. [...] На самом деле я планирую использовать его для всех **сервисов машинного обучения моей команды в Microsoft**. Некоторые из них интегрируются в основной продукт **Windows**, а некоторые — в продукты **Office**._"
---
-"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_"
+"_Мы использовали библиотеку **FastAPI** для создания сервера **REST**, к которому можно делать запросы для получения **прогнозов**. [для Ludwig]_"
Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - Uber(ref)
---
-"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_"
+"_**Netflix** рада объявить о выпуске опенсорсного фреймворка для оркестровки **антикризисного управления**: **Dispatch**! [создана с помощью **FastAPI**]_"
Kevin Glisson, Marc Vilanova, Forest Monsen - Netflix(ref)
---
-"_I’m over the moon excited about **FastAPI**. It’s so fun!_"
+"_Я в полном восторге от **FastAPI**. Это так весело!_"
---
-"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._"
+"_Честно говоря, то, что вы создали, выглядит очень солидно и отполировано. Во многих смыслах я хотел, чтобы **Hug** был именно таким — это действительно вдохновляет, когда кто-то создаёт такое._"
---
-"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_"
+"_Если вы хотите изучить какой-нибудь **современный фреймворк** для создания REST API, ознакомьтесь с **FastAPI** [...] Он быстрый, лёгкий и простой в изучении [...]_"
-"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_"
+"_Мы перешли на **FastAPI** для наших **API** [...] Я думаю, вам тоже понравится [...]_"
---
-## **Typer**, the FastAPI of CLIs
+## **Typer**, интерфейс командной строки для FastAPI
-If you are building a CLI app to be used in the terminal instead of a web API, check out **Typer**.
+Если вы создаете приложение CLI для использования в терминале вместо веб-API, ознакомьтесь с **Typer**.
-**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀
+**Typer** — младший брат FastAPI. И он предназначен для использования в качестве **интерфейса командной строки для FastAPI**. ⌨️ 🚀
-## Requirements
+## Зависимости
Python 3.6+
-FastAPI stands on the shoulders of giants:
+FastAPI стоит на плечах гигантов:
-* Starlette for the web parts.
-* Pydantic for the data parts.
+* Starlette для части связанной с вебом.
+* Pydantic для части связанной с данными.
-## Installation
+## Установка
@@ -130,26 +128,26 @@ $ pip install fastapi
-You will also need an ASGI server, for production such as Uvicorn or Hypercorn.
+Вам также понадобится сервер ASGI для производства, такой как Uvicorn или Hypercorn.
-About the command uvicorn main:app --reload...
+О команде uvicorn main:app --reload...
-The command `uvicorn main:app` refers to:
+Команда `uvicorn main:app` относится к:
-* `main`: the file `main.py` (the Python "module").
-* `app`: the object created inside of `main.py` with the line `app = FastAPI()`.
-* `--reload`: make the server restart after code changes. Only do this for development.
+* `main`: файл `main.py` (модуль Python).
+* `app`: объект, созданный внутри `main.py` с помощью строки `app = FastAPI()`.
+* `--reload`: перезапуск сервера после изменения кода. Делайте это только во время разработки.
-### Check it
+### Проверка
-Open your browser at http://127.0.0.1:8000/items/5?q=somequery.
+Откройте браузер на http://127.0.0.1:8000/items/5?q=somequery.
-You will see the JSON response as:
+Вы увидите следующий JSON ответ:
```JSON
{"item_id": 5, "q": "somequery"}
```
-You already created an API that:
+Вы уже создали API, который:
-* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`.
-* Both _paths_ take `GET` operations (also known as HTTP _methods_).
-* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`.
-* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`.
+* Получает HTTP-запросы по _путям_ `/` и `/items/{item_id}`.
+* И первый и второй _путь_ используют `GET` операции (также известные как HTTP _методы_).
+* _путь_ `/items/{item_id}` имеет _параметр пути_ `item_id`, который должен быть `int`.
+* _путь_ `/items/{item_id}` имеет необязательный `str` _параметр запроса_ `q`.
-### Interactive API docs
+### Интерактивная документация по API
-Now go to http://127.0.0.1:8000/docs.
+Перейдите на http://127.0.0.1:8000/docs.
-You will see the automatic interactive API documentation (provided by Swagger UI):
+Вы увидите автоматическую интерактивную документацию API (предоставленную Swagger UI):
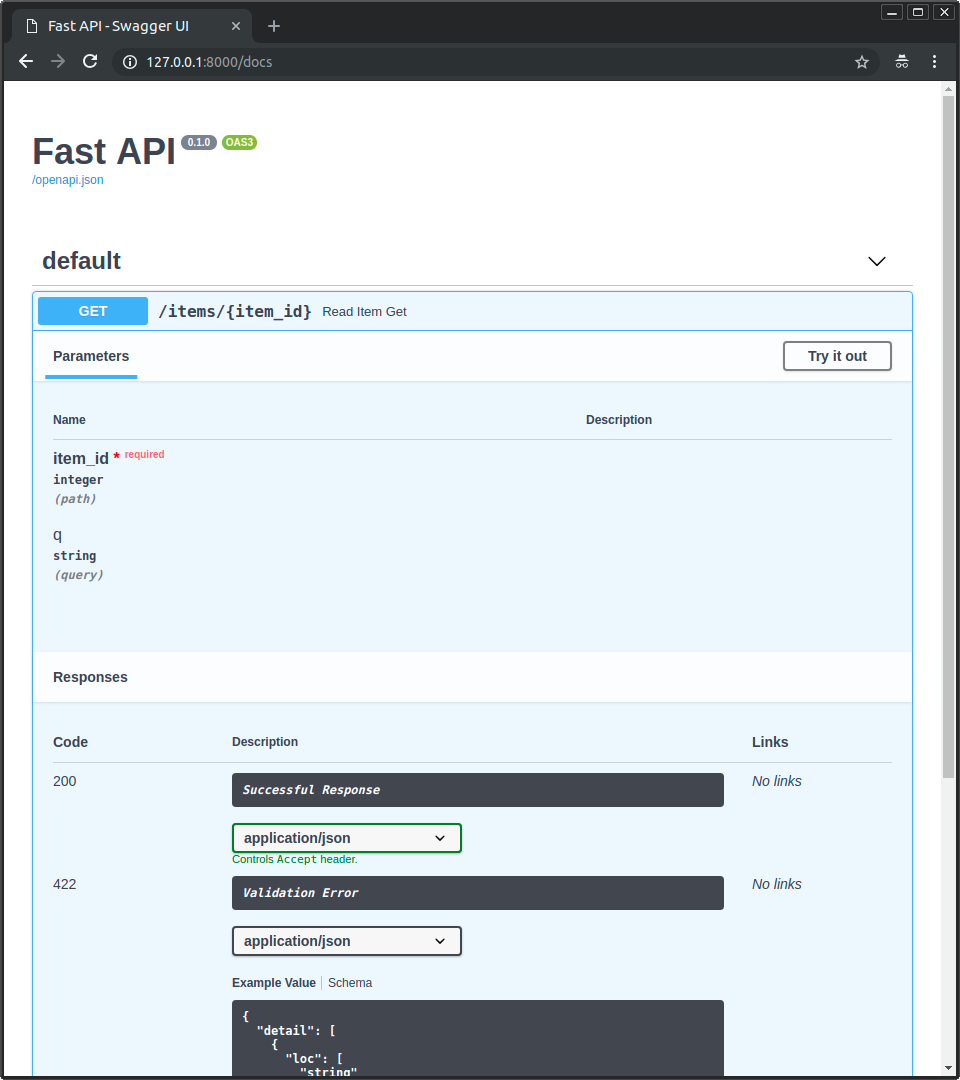
-### Alternative API docs
+### Альтернативная документация по API
-And now, go to http://127.0.0.1:8000/redoc.
+А теперь перейдите на http://127.0.0.1:8000/redoc.
-You will see the alternative automatic documentation (provided by ReDoc):
+Вы увидите альтернативную автоматическую документацию (предоставленную ReDoc):
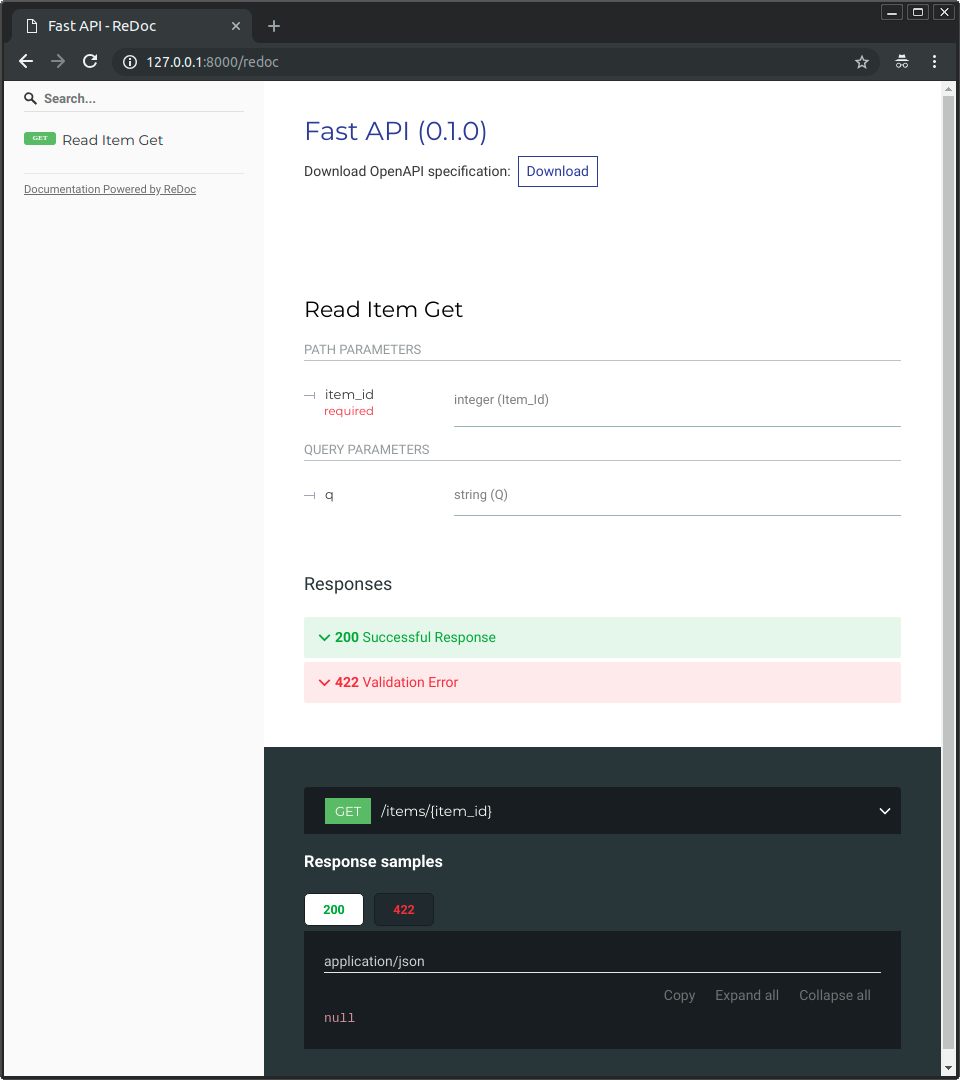
-## Example upgrade
+## Пример обновления
-Now modify the file `main.py` to receive a body from a `PUT` request.
+Теперь измените файл `main.py`, чтобы получить тело ответа из `PUT` запроса.
-Declare the body using standard Python types, thanks to Pydantic.
+Объявите тело, используя стандартную типизацию Python, спасибо Pydantic.
```Python hl_lines="4 9-12 25-27"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
from pydantic import BaseModel
@@ -275,7 +273,7 @@ app = FastAPI()
class Item(BaseModel):
name: str
price: float
- is_offer: Optional[bool] = None
+ is_offer: Union[bool, None] = None
@app.get("/")
@@ -284,7 +282,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
@@ -293,175 +291,173 @@ def update_item(item_id: int, item: Item):
return {"item_name": item.name, "item_id": item_id}
```
-The server should reload automatically (because you added `--reload` to the `uvicorn` command above).
+Сервер должен перезагрузиться автоматически (потому что вы добавили `--reload` к команде `uvicorn` выше).
-### Interactive API docs upgrade
+### Интерактивное обновление документации API
-Now go to http://127.0.0.1:8000/docs.
+Перейдите на http://127.0.0.1:8000/docs.
-* The interactive API documentation will be automatically updated, including the new body:
+* Интерактивная документация API будет автоматически обновляться, включая новое тело:
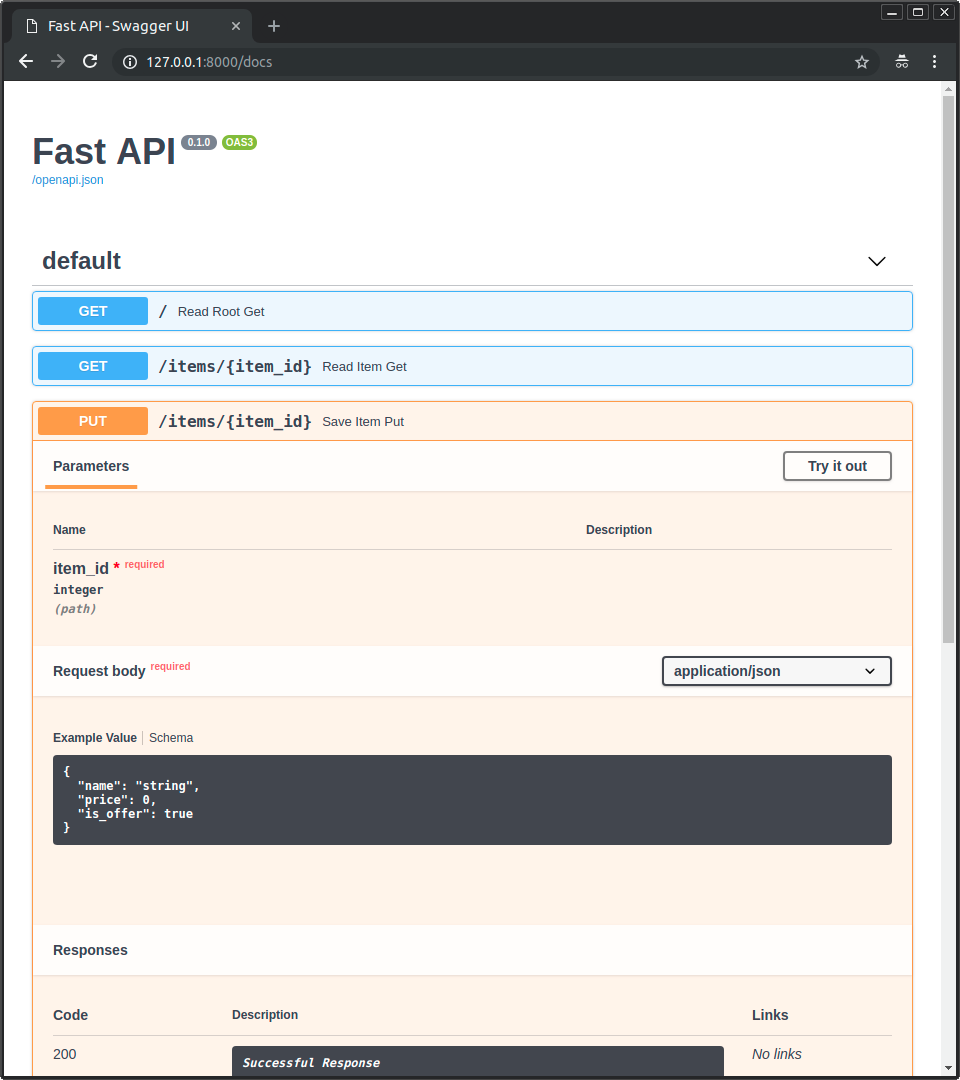
-* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API:
+* Нажмите на кнопку "Try it out", это позволит вам заполнить параметры и напрямую взаимодействовать с API:
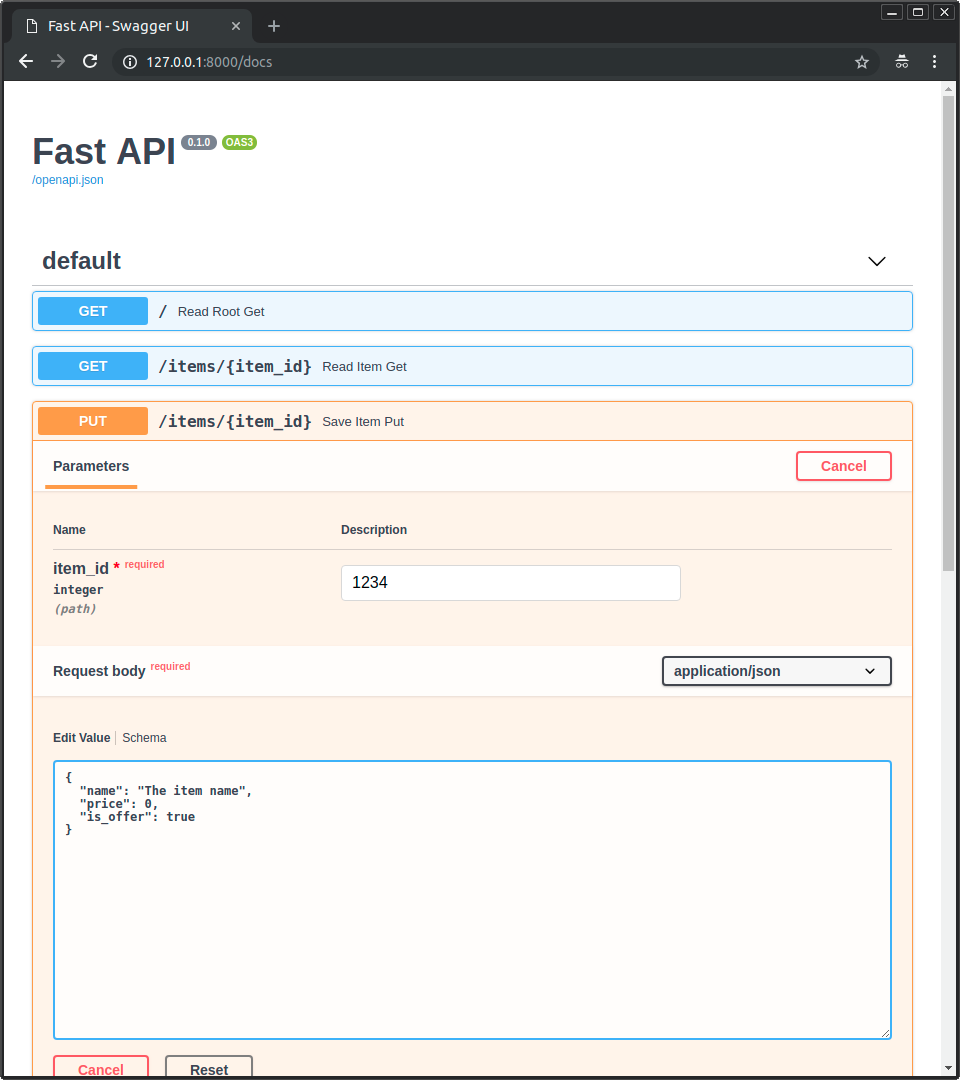
-* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen:
+* Затем нажмите кнопку "Execute", пользовательский интерфейс свяжется с вашим API, отправит параметры, получит результаты и отобразит их на экране:
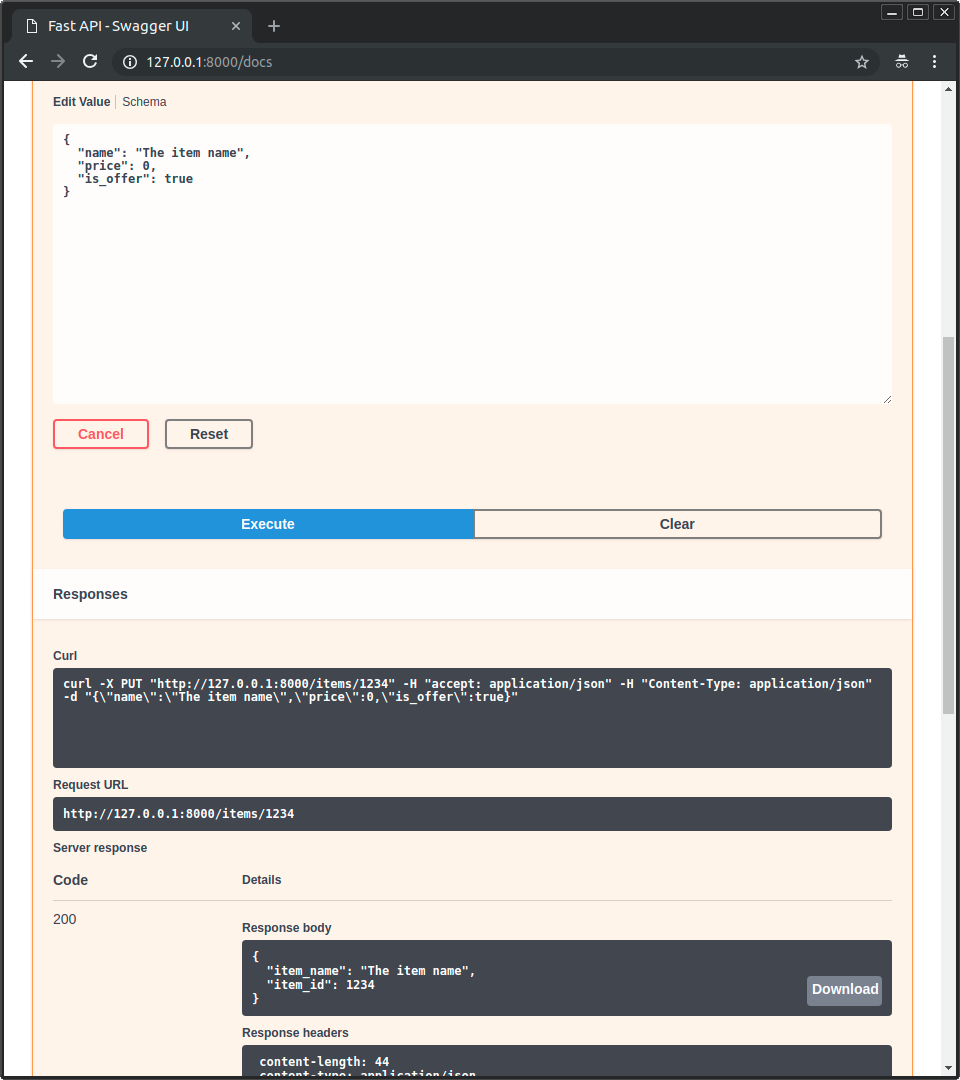
-### Alternative API docs upgrade
+### Альтернативное обновление документации API
-And now, go to http://127.0.0.1:8000/redoc.
+А теперь перейдите на http://127.0.0.1:8000/redoc.
-* The alternative documentation will also reflect the new query parameter and body:
+* Альтернативная документация также будет отражать новый параметр и тело запроса:
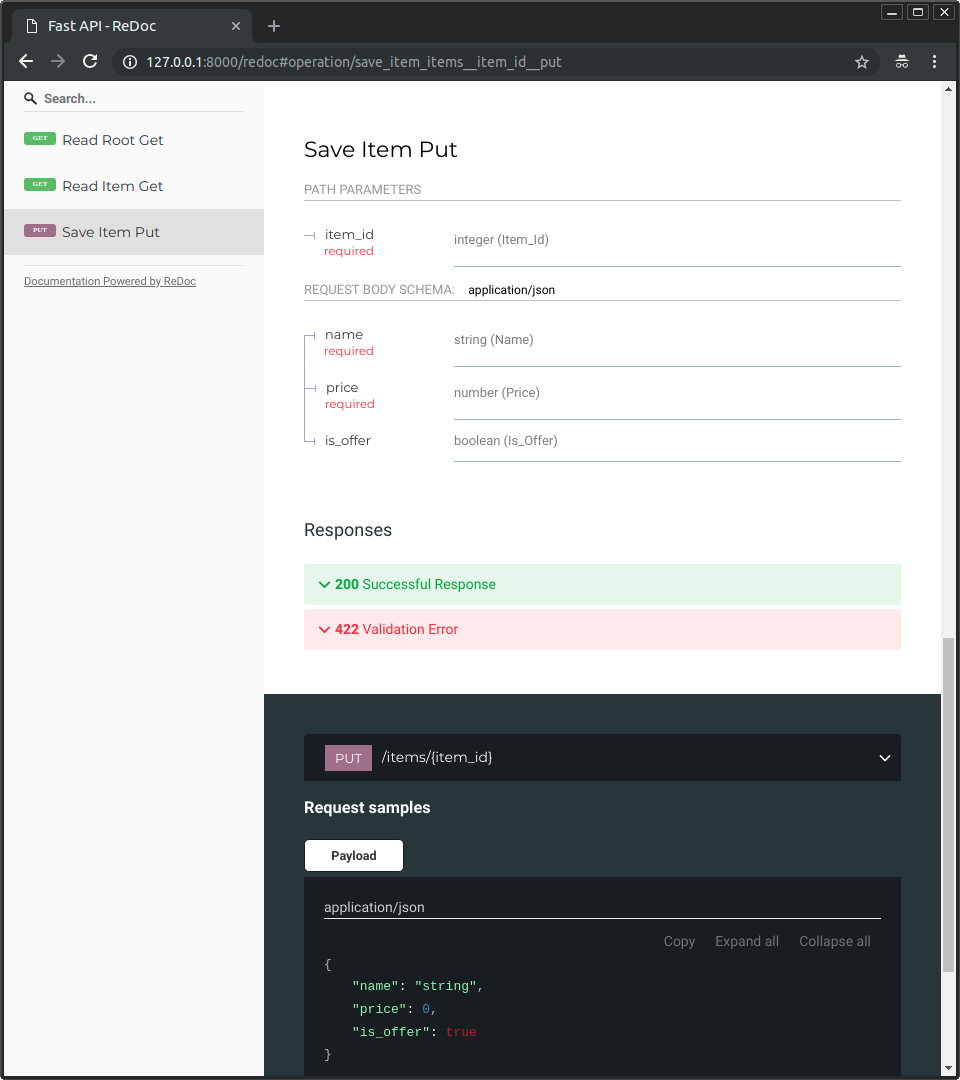
-### Recap
+### Подведём итоги
-In summary, you declare **once** the types of parameters, body, etc. as function parameters.
+Таким образом, вы объявляете **один раз** типы параметров, тело и т. д. в качестве параметров функции.
-You do that with standard modern Python types.
+Вы делаете это испльзуя стандартную современную типизацию Python.
-You don't have to learn a new syntax, the methods or classes of a specific library, etc.
+Вам не нужно изучать новый синтаксис, методы или классы конкретной библиотеки и т. д.
-Just standard **Python 3.6+**.
+Только стандартный **Python 3.6+**.
-For example, for an `int`:
+Например, для `int`:
```Python
item_id: int
```
-or for a more complex `Item` model:
+или для более сложной модели `Item`:
```Python
item: Item
```
-...and with that single declaration you get:
+... и с этим единственным объявлением вы получаете:
-* Editor support, including:
- * Completion.
- * Type checks.
-* Validation of data:
- * Automatic and clear errors when the data is invalid.
- * Validation even for deeply nested JSON objects.
-* Conversion of input data: coming from the network to Python data and types. Reading from:
+* Поддержка редактора, в том числе:
+ * Автозавершение.
+ * Проверка типов.
+* Валидация данных:
+ * Автоматические и четкие ошибки, когда данные недействительны.
+ * Проверка даже для глубоко вложенных объектов JSON.
+* Преобразование входных данных: поступающие из сети в объекты Python с соблюдением типов. Чтение из:
* JSON.
- * Path parameters.
- * Query parameters.
+ * Параметров пути.
+ * Параметров запроса.
* Cookies.
- * Headers.
- * Forms.
- * Files.
-* Conversion of output data: converting from Python data and types to network data (as JSON):
- * Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc).
- * `datetime` objects.
- * `UUID` objects.
- * Database models.
- * ...and many more.
-* Automatic interactive API documentation, including 2 alternative user interfaces:
+ * Заголовков.
+ * Форм.
+ * Файлов.
+* Преобразование выходных данных: преобразование объектов Python в данные передаваемые по сети интернет (такие как JSON):
+ * Преобразование типов Python (`str`, `int`, `float`, `bool`, `list`, и т.д.).
+ * Объекты `datetime`.
+ * Объекты `UUID`.
+ * Модели баз данных.
+ * ...и многое другое.
+* Автоматическая интерактивная документация по API, включая 2 альтернативных пользовательских интерфейса:
* Swagger UI.
* ReDoc.
---
-Coming back to the previous code example, **FastAPI** will:
-
-* Validate that there is an `item_id` in the path for `GET` and `PUT` requests.
-* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests.
- * If it is not, the client will see a useful, clear error.
-* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests.
- * As the `q` parameter is declared with `= None`, it is optional.
- * Without the `None` it would be required (as is the body in the case with `PUT`).
-* For `PUT` requests to `/items/{item_id}`, Read the body as JSON:
- * Check that it has a required attribute `name` that should be a `str`.
- * Check that it has a required attribute `price` that has to be a `float`.
- * Check that it has an optional attribute `is_offer`, that should be a `bool`, if present.
- * All this would also work for deeply nested JSON objects.
-* Convert from and to JSON automatically.
-* Document everything with OpenAPI, that can be used by:
- * Interactive documentation systems.
- * Automatic client code generation systems, for many languages.
-* Provide 2 interactive documentation web interfaces directly.
+Возвращаясь к предыдущему примеру кода, **FastAPI** будет:
+
+* Проверять наличие `item_id` в пути для запросов `GET` и `PUT`.
+* Проверять, что `item_id` имеет тип `int` для запросов `GET` и `PUT`.
+ * Если это не так, клиент увидит полезную чёткую ошибку.
+* Проверять, есть ли необязательный параметр запроса с именем `q` (например, `http://127.0.0.1:8000/items/foo?q=somequery`) для `GET` запросов.
+ * Поскольку параметр `q` объявлен с `= None`, он является необязательным.
+ * Без `None` он был бы необходим (как тело в случае с `PUT`).
+* Для `PUT` запросов к `/items/{item_id}` читать тело как JSON:
+ * Проверять, что у него есть обязательный атрибут `name`, который должен быть `str`.
+ * Проверять, что у него есть обязательный атрибут `price`, который должен быть `float`.
+ * Проверять, что у него есть необязательный атрибут `is_offer`, который должен быть `bool`, если он присутствует.
+ * Все это также будет работать для глубоко вложенных объектов JSON.
+* Преобразовывать из и в JSON автоматически.
+* Документировать с помощью OpenAPI все, что может быть использовано:
+ * Системы интерактивной документации.
+ * Системы автоматической генерации клиентского кода для многих языков.
+* Обеспечит 2 интерактивных веб-интерфейса документации напрямую.
---
-We just scratched the surface, but you already get the idea of how it all works.
+Мы только немного копнули поверхность, но вы уже поняли, как все это работает.
-Try changing the line with:
+Попробуйте изменить строку с помощью:
```Python
return {"item_name": item.name, "item_id": item_id}
```
-...from:
+...из:
```Python
... "item_name": item.name ...
```
-...to:
+...в:
```Python
... "item_price": item.price ...
```
-...and see how your editor will auto-complete the attributes and know their types:
+... и посмотрите, как ваш редактор будет автоматически заполнять атрибуты и узнавать их типы:
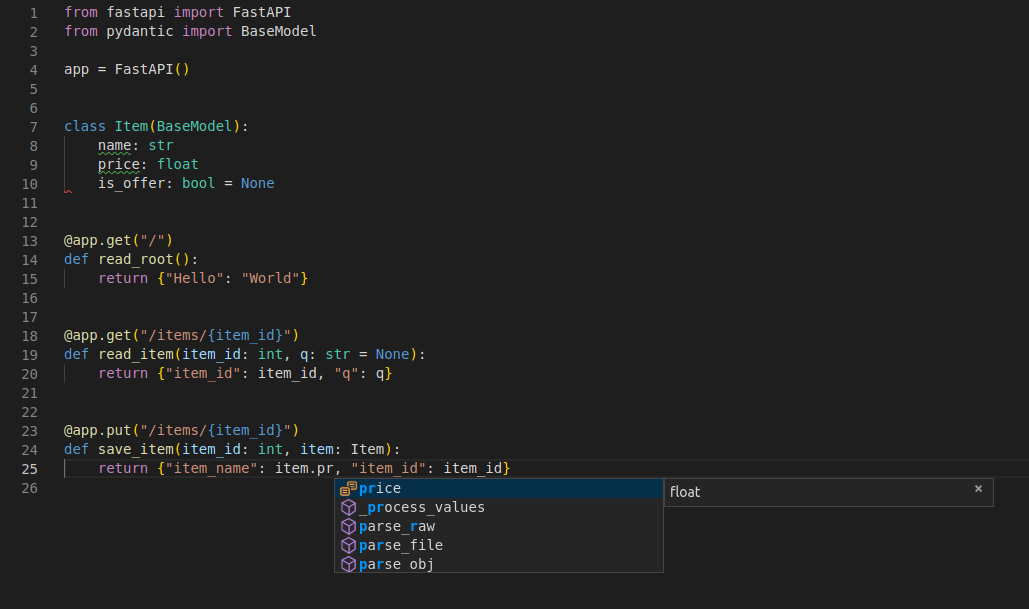
-For a more complete example including more features, see the Tutorial - User Guide.
+Более полный пример с дополнительными функциями см. в Учебное руководство - Руководство пользователя.
-**Spoiler alert**: the tutorial - user guide includes:
+**Осторожно, спойлер**: руководство пользователя включает в себя:
-* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**.
-* How to set **validation constraints** as `maximum_length` or `regex`.
-* A very powerful and easy to use **Dependency Injection** system.
-* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth.
-* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic).
-* Many extra features (thanks to Starlette) as:
- * **WebSockets**
- * **GraphQL**
- * extremely easy tests based on `requests` and `pytest`
+* Объявление **параметров** из других мест, таких как: **заголовки**, **cookies**, **поля формы** и **файлы**.
+* Как установить **ограничительные проверки** такие как `maximum_length` или `regex`.
+* Очень мощная и простая в использовании система **внедрения зависимостей**.
+* Безопасность и аутентификация, включая поддержку **OAuth2** с **токенами JWT** и **HTTP Basic** аутентификацию.
+* Более продвинутые (но столь же простые) методы объявления **глубоко вложенных моделей JSON** (спасибо Pydantic).
+* **GraphQL** интеграция с Strawberry и другими библиотеками.
+* Множество дополнительных функций (благодаря Starlette), таких как:
+ * **Веб-сокеты**
+ * очень простые тесты на основе `requests` и `pytest`
* **CORS**
- * **Cookie Sessions**
- * ...and more.
+ * **Cookie сеансы(сессии)**
+ * ...и многое другое.
-## Performance
+## Производительность
-Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as one of the fastest Python frameworks available, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*)
+Независимые тесты TechEmpower показывают приложения **FastAPI**, работающие под управлением Uvicorn, как один из самых быстрых доступных фреймворков Python, уступающий только самим Starlette и Uvicorn (используемых внутри FastAPI). (*)
-To understand more about it, see the section Benchmarks.
+Чтобы узнать больше об этом, см. раздел Тесты производительности.
-## Optional Dependencies
+## Необязательные зависимости
-Used by Pydantic:
+Используется Pydantic:
-* ujson - for faster JSON "parsing".
-* email_validator - for email validation.
+* ujson - для более быстрого JSON "парсинга".
+* email_validator - для проверки электронной почты.
-Used by Starlette:
+Используется Starlette:
-* requests - Required if you want to use the `TestClient`.
-* aiofiles - Required if you want to use `FileResponse` or `StaticFiles`.
-* jinja2 - Required if you want to use the default template configuration.
-* python-multipart - Required if you want to support form "parsing", with `request.form()`.
-* itsdangerous - Required for `SessionMiddleware` support.
-* pyyaml - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI).
-* graphene - Required for `GraphQLApp` support.
-* ujson - Required if you want to use `UJSONResponse`.
+* requests - Обязательно, если вы хотите использовать `TestClient`.
+* jinja2 - Обязательно, если вы хотите использовать конфигурацию шаблона по умолчанию.
+* python-multipart - Обязательно, если вы хотите поддерживать форму "парсинга" с помощью `request.form()`.
+* itsdangerous - Обязательно, для поддержки `SessionMiddleware`.
+* pyyaml - Обязательно, для поддержки `SchemaGenerator` Starlette (возможно, вам это не нужно с FastAPI).
+* ujson - Обязательно, если вы хотите использовать `UJSONResponse`.
-Used by FastAPI / Starlette:
+Используется FastAPI / Starlette:
-* uvicorn - for the server that loads and serves your application.
-* orjson - Required if you want to use `ORJSONResponse`.
+* uvicorn - сервер, который загружает и обслуживает ваше приложение.
+* orjson - Обязательно, если вы хотите использовать `ORJSONResponse`.
-You can install all of these with `pip install fastapi[all]`.
+Вы можете установить все это с помощью `pip install "fastapi[all]"`.
-## License
+## Лицензия
-This project is licensed under the terms of the MIT license.
+Этот проект распространяется на условиях лицензии MIT.
diff --git a/docs/ru/docs/python-types.md b/docs/ru/docs/python-types.md
index 99670363c..7523083c8 100644
--- a/docs/ru/docs/python-types.md
+++ b/docs/ru/docs/python-types.md
@@ -1,6 +1,6 @@
# Введение в аннотации типов Python
-Python имеет поддержку необязательных аннотаций типов.
+Python имеет поддержку необязательных аннотаций типов.
**Аннотации типов** являются специальным синтаксисом, который позволяет определять тип переменной.
diff --git a/docs/ru/docs/tutorial/background-tasks.md b/docs/ru/docs/tutorial/background-tasks.md
new file mode 100644
index 000000000..e608f6c8f
--- /dev/null
+++ b/docs/ru/docs/tutorial/background-tasks.md
@@ -0,0 +1,102 @@
+# Фоновые задачи
+
+Вы можете создавать фоновые задачи, которые будут выполнятся *после* возвращения ответа сервером.
+
+Это может быть полезно для функций, которые должны выполниться после получения запроса, но ожидание их выполнения необязательно для пользователя.
+
+К примеру:
+
+* Отправка писем на почту после выполнения каких-либо действий:
+ * Т.к. соединение с почтовым сервером и отправка письма идут достаточно "долго" (несколько секунд), вы можете отправить ответ пользователю, а отправку письма выполнить в фоне.
+* Обработка данных:
+ * К примеру, если вы получаете файл, который должен пройти через медленный процесс, вы можете отправить ответ "Accepted" (HTTP 202) и отправить работу с файлом в фон.
+
+## Использование класса `BackgroundTasks`
+
+Сначала импортируйте `BackgroundTasks`, потом добавьте в функцию параметр с типом `BackgroundTasks`:
+
+```Python hl_lines="1 13"
+{!../../../docs_src/background_tasks/tutorial001.py!}
+```
+
+**FastAPI** создаст объект класса `BackgroundTasks` для вас и запишет его в параметр.
+
+## Создание функции для фоновой задачи
+
+Создайте функцию, которую хотите запустить в фоне.
+
+Это совершенно обычная функция, которая может принимать параметры.
+
+Она может быть как асинхронной `async def`, так и обычной `def` функцией, **FastAPI** знает, как правильно ее выполнить.
+
+В нашем примере фоновая задача будет вести запись в файл (симулируя отправку письма).
+
+Так как операция записи не использует `async` и `await`, мы определим ее как обычную `def`:
+
+```Python hl_lines="6-9"
+{!../../../docs_src/background_tasks/tutorial001.py!}
+```
+
+## Добавление фоновой задачи
+
+Внутри функции вызовите метод `.add_task()` у объекта *background tasks* и передайте ему функцию, которую хотите выполнить в фоне:
+
+```Python hl_lines="14"
+{!../../../docs_src/background_tasks/tutorial001.py!}
+```
+
+`.add_task()` принимает следующие аргументы:
+
+* Функцию, которая будет выполнена в фоне (`write_notification`). Обратите внимание, что передается объект функции, без скобок.
+* Любое упорядоченное количество аргументов, которые принимает функция (`email`).
+* Любое количество именованных аргументов, которые принимает функция (`message="some notification"`).
+
+## Встраивание зависимостей
+
+Класс `BackgroundTasks` также работает с системой встраивания зависимостей, вы можете определить `BackgroundTasks` на разных уровнях: как параметр функции, как завимость, как подзависимость и так далее.
+
+**FastAPI** знает, что нужно сделать в каждом случае и как переиспользовать тот же объект `BackgroundTasks`, так чтобы все фоновые задачи собрались и запустились вместе в фоне:
+
+=== "Python 3.6 и выше"
+
+ ```Python hl_lines="13 15 22 25"
+ {!> ../../../docs_src/background_tasks/tutorial002.py!}
+ ```
+
+=== "Python 3.10 и выше"
+
+ ```Python hl_lines="11 13 20 23"
+ {!> ../../../docs_src/background_tasks/tutorial002_py310.py!}
+ ```
+
+В этом примере сообщения будут записаны в `log.txt` *после* того, как ответ сервера был отправлен.
+
+Если бы в запросе была очередь `q`, она бы первой записалась в `log.txt` фоновой задачей (потому что вызывается в зависимости `get_query`).
+
+После другая фоновая задача, которая была сгенерирована в функции, запишет сообщение из параметра `email`.
+
+## Технические детали
+
+Класс `BackgroundTasks` основан на `starlette.background`.
+
+Он интегрирован в FastAPI, так что вы можете импортировать его прямо из `fastapi` и избежать случайного импорта `BackgroundTask` (без `s` на конце) из `starlette.background`.
+
+При использовании `BackgroundTasks` (а не `BackgroundTask`), вам достаточно только определить параметр функции с типом `BackgroundTasks` и **FastAPI** сделает все за вас, также как при использовании объекта `Request`.
+
+Вы все равно можете использовать `BackgroundTask` из `starlette` в FastAPI, но вам придется самостоятельно создавать объект фоновой задачи и вручную обработать `Response` внутри него.
+
+Вы можете подробнее изучить его в Официальной документации Starlette для BackgroundTasks.
+
+## Предостережение
+
+Если вам нужно выполнить тяжелые вычисления в фоне, которым необязательно быть запущенными в одном процессе с приложением **FastAPI** (к примеру, вам не нужны обрабатываемые переменные или вы не хотите делиться памятью процесса и т.д.), вы можете использовать более серьезные инструменты, такие как Celery.
+
+Их тяжелее настраивать, также им нужен брокер сообщений наподобие RabbitMQ или Redis, но зато они позволяют вам запускать фоновые задачи в нескольких процессах и даже на нескольких серверах.
+
+Для примера, посмотрите [Project Generators](../project-generation.md){.internal-link target=_blank}, там есть проект с уже настроенным Celery.
+
+Но если вам нужен доступ к общим переменным и объектам вашего **FastAPI** приложения или вам нужно выполнять простые фоновые задачи (наподобие отправки письма из примера) вы можете просто использовать `BackgroundTasks`.
+
+## Резюме
+
+Для создания фоновых задач вам необходимо импортировать `BackgroundTasks` и добавить его в функцию, как параметр с типом `BackgroundTasks`.
diff --git a/docs/ru/mkdocs.yml b/docs/ru/mkdocs.yml
index 6e17c287e..2cb5eb8e0 100644
--- a/docs/ru/mkdocs.yml
+++ b/docs/ru/mkdocs.yml
@@ -5,13 +5,15 @@ theme:
name: material
custom_dir: overrides
palette:
- - scheme: default
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
primary: teal
accent: amber
toggle:
icon: material/lightbulb
name: Switch to light mode
- - scheme: slate
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
primary: teal
accent: amber
toggle:
@@ -40,18 +42,29 @@ nav:
- az: /az/
- de: /de/
- es: /es/
+ - fa: /fa/
- fr: /fr/
+ - he: /he/
- id: /id/
- it: /it/
- ja: /ja/
- ko: /ko/
+ - nl: /nl/
- pl: /pl/
- pt: /pt/
- ru: /ru/
- sq: /sq/
+ - sv: /sv/
- tr: /tr/
- uk: /uk/
- zh: /zh/
+- python-types.md
+- Учебник - руководство пользователя:
+ - tutorial/background-tasks.md
+- external-links.md
+- async.md
+- Развёртывание:
+ - deployment/versions.md
markdown_extensions:
- toc:
permalink: true
@@ -69,6 +82,8 @@ markdown_extensions:
format: !!python/name:pymdownx.superfences.fence_code_format ''
- pymdownx.tabbed:
alternate_style: true
+- attr_list
+- md_in_html
extra:
analytics:
provider: google
@@ -97,8 +112,12 @@ extra:
name: de
- link: /es/
name: es - español
+ - link: /fa/
+ name: fa
- link: /fr/
name: fr - français
+ - link: /he/
+ name: he
- link: /id/
name: id
- link: /it/
@@ -107,6 +126,8 @@ extra:
name: ja - 日本語
- link: /ko/
name: ko - 한국어
+ - link: /nl/
+ name: nl
- link: /pl/
name: pl
- link: /pt/
@@ -115,6 +136,8 @@ extra:
name: ru - русский язык
- link: /sq/
name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
- link: /tr/
name: tr - Türkçe
- link: /uk/
diff --git a/docs/sq/docs/index.md b/docs/sq/docs/index.md
index 95fb7ae21..2b64003fe 100644
--- a/docs/sq/docs/index.md
+++ b/docs/sq/docs/index.md
@@ -135,7 +135,7 @@ You will also need an ASGI server, for production such as
```console
-$ pip install uvicorn[standard]
+$ pip install "uvicorn[standard]"
---> 100%
```
@@ -149,7 +149,7 @@ $ pip install uvicorn[standard]
* Create a file `main.py` with:
```Python
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -162,7 +162,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -172,7 +172,7 @@ def read_item(item_id: int, q: Optional[str] = None):
If your code uses `async` / `await`, use `async def`:
```Python hl_lines="9 14"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -185,7 +185,7 @@ async def read_root():
@app.get("/items/{item_id}")
-async def read_item(item_id: int, q: Optional[str] = None):
+async def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -264,7 +264,7 @@ Now modify the file `main.py` to receive a body from a `PUT` request.
Declare the body using standard Python types, thanks to Pydantic.
```Python hl_lines="4 9-12 25-27"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
from pydantic import BaseModel
@@ -275,7 +275,7 @@ app = FastAPI()
class Item(BaseModel):
name: str
price: float
- is_offer: Optional[bool] = None
+ is_offer: Union[bool, None] = None
@app.get("/")
@@ -284,7 +284,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
@@ -321,7 +321,7 @@ And now, go to requests - Required if you want to use the `TestClient`.
-* aiofiles - Required if you want to use `FileResponse` or `StaticFiles`.
* jinja2 - Required if you want to use the default template configuration.
* python-multipart - Required if you want to support form "parsing", with `request.form()`.
* itsdangerous - Required for `SessionMiddleware` support.
diff --git a/docs/sq/mkdocs.yml b/docs/sq/mkdocs.yml
index d9c3dad4c..b07f3bc63 100644
--- a/docs/sq/mkdocs.yml
+++ b/docs/sq/mkdocs.yml
@@ -5,13 +5,15 @@ theme:
name: material
custom_dir: overrides
palette:
- - scheme: default
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
primary: teal
accent: amber
toggle:
icon: material/lightbulb
name: Switch to light mode
- - scheme: slate
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
primary: teal
accent: amber
toggle:
@@ -40,15 +42,19 @@ nav:
- az: /az/
- de: /de/
- es: /es/
+ - fa: /fa/
- fr: /fr/
+ - he: /he/
- id: /id/
- it: /it/
- ja: /ja/
- ko: /ko/
+ - nl: /nl/
- pl: /pl/
- pt: /pt/
- ru: /ru/
- sq: /sq/
+ - sv: /sv/
- tr: /tr/
- uk: /uk/
- zh: /zh/
@@ -69,6 +75,8 @@ markdown_extensions:
format: !!python/name:pymdownx.superfences.fence_code_format ''
- pymdownx.tabbed:
alternate_style: true
+- attr_list
+- md_in_html
extra:
analytics:
provider: google
@@ -97,8 +105,12 @@ extra:
name: de
- link: /es/
name: es - español
+ - link: /fa/
+ name: fa
- link: /fr/
name: fr - français
+ - link: /he/
+ name: he
- link: /id/
name: id
- link: /it/
@@ -107,6 +119,8 @@ extra:
name: ja - 日本語
- link: /ko/
name: ko - 한국어
+ - link: /nl/
+ name: nl
- link: /pl/
name: pl
- link: /pt/
@@ -115,6 +129,8 @@ extra:
name: ru - русский язык
- link: /sq/
name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
- link: /tr/
name: tr - Türkçe
- link: /uk/
diff --git a/docs/sv/docs/index.md b/docs/sv/docs/index.md
new file mode 100644
index 000000000..fd52f994c
--- /dev/null
+++ b/docs/sv/docs/index.md
@@ -0,0 +1,468 @@
+
+{!../../../docs/missing-translation.md!}
+
+
+
+
+
+
+ FastAPI framework, high performance, easy to learn, fast to code, ready for production
+
+
+---
+
+**Documentation**: https://fastapi.tiangolo.com
+
+**Source Code**: https://github.com/tiangolo/fastapi
+
+---
+
+FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints.
+
+The key features are:
+
+* **Fast**: Very high performance, on par with **NodeJS** and **Go** (thanks to Starlette and Pydantic). [One of the fastest Python frameworks available](#performance).
+
+* **Fast to code**: Increase the speed to develop features by about 200% to 300%. *
+* **Fewer bugs**: Reduce about 40% of human (developer) induced errors. *
+* **Intuitive**: Great editor support. Completion everywhere. Less time debugging.
+* **Easy**: Designed to be easy to use and learn. Less time reading docs.
+* **Short**: Minimize code duplication. Multiple features from each parameter declaration. Fewer bugs.
+* **Robust**: Get production-ready code. With automatic interactive documentation.
+* **Standards-based**: Based on (and fully compatible with) the open standards for APIs: OpenAPI (previously known as Swagger) and JSON Schema.
+
+* estimation based on tests on an internal development team, building production applications.
+
+## Sponsors
+
+
+
+{% if sponsors %}
+{% for sponsor in sponsors.gold -%}
+
+{% endfor -%}
+{%- for sponsor in sponsors.silver -%}
+
+{% endfor %}
+{% endif %}
+
+
+
+Other sponsors
+
+## Opinions
+
+"_[...] I'm using **FastAPI** a ton these days. [...] I'm actually planning to use it for all of my team's **ML services at Microsoft**. Some of them are getting integrated into the core **Windows** product and some **Office** products._"
+
+
+
+---
+
+"_We adopted the **FastAPI** library to spawn a **REST** server that can be queried to obtain **predictions**. [for Ludwig]_"
+
+
Piero Molino, Yaroslav Dudin, and Sai Sumanth Miryala - Uber(ref)
+
+---
+
+"_**Netflix** is pleased to announce the open-source release of our **crisis management** orchestration framework: **Dispatch**! [built with **FastAPI**]_"
+
+
Kevin Glisson, Marc Vilanova, Forest Monsen - Netflix(ref)
+
+---
+
+"_I’m over the moon excited about **FastAPI**. It’s so fun!_"
+
+
+
+---
+
+"_Honestly, what you've built looks super solid and polished. In many ways, it's what I wanted **Hug** to be - it's really inspiring to see someone build that._"
+
+
+
+---
+
+"_If you're looking to learn one **modern framework** for building REST APIs, check out **FastAPI** [...] It's fast, easy to use and easy to learn [...]_"
+
+"_We've switched over to **FastAPI** for our **APIs** [...] I think you'll like it [...]_"
+
+
+
+---
+
+## **Typer**, the FastAPI of CLIs
+
+
+
+If you are building a CLI app to be used in the terminal instead of a web API, check out **Typer**.
+
+**Typer** is FastAPI's little sibling. And it's intended to be the **FastAPI of CLIs**. ⌨️ 🚀
+
+## Requirements
+
+Python 3.6+
+
+FastAPI stands on the shoulders of giants:
+
+* Starlette for the web parts.
+* Pydantic for the data parts.
+
+## Installation
+
+
+
+## Example
+
+### Create it
+
+* Create a file `main.py` with:
+
+```Python
+from typing import Union
+
+from fastapi import FastAPI
+
+app = FastAPI()
+
+
+@app.get("/")
+def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+```
+
+
+Or use async def...
+
+If your code uses `async` / `await`, use `async def`:
+
+```Python hl_lines="9 14"
+from typing import Union
+
+from fastapi import FastAPI
+
+app = FastAPI()
+
+
+@app.get("/")
+async def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+async def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+```
+
+**Note**:
+
+If you don't know, check the _"In a hurry?"_ section about `async` and `await` in the docs.
+
+
+
+### Run it
+
+Run the server with:
+
+
+
+```console
+$ uvicorn main:app --reload
+
+INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
+INFO: Started reloader process [28720]
+INFO: Started server process [28722]
+INFO: Waiting for application startup.
+INFO: Application startup complete.
+```
+
+
+
+
+About the command uvicorn main:app --reload...
+
+The command `uvicorn main:app` refers to:
+
+* `main`: the file `main.py` (the Python "module").
+* `app`: the object created inside of `main.py` with the line `app = FastAPI()`.
+* `--reload`: make the server restart after code changes. Only do this for development.
+
+
+
+### Check it
+
+Open your browser at http://127.0.0.1:8000/items/5?q=somequery.
+
+You will see the JSON response as:
+
+```JSON
+{"item_id": 5, "q": "somequery"}
+```
+
+You already created an API that:
+
+* Receives HTTP requests in the _paths_ `/` and `/items/{item_id}`.
+* Both _paths_ take `GET` operations (also known as HTTP _methods_).
+* The _path_ `/items/{item_id}` has a _path parameter_ `item_id` that should be an `int`.
+* The _path_ `/items/{item_id}` has an optional `str` _query parameter_ `q`.
+
+### Interactive API docs
+
+Now go to http://127.0.0.1:8000/docs.
+
+You will see the automatic interactive API documentation (provided by Swagger UI):
+
+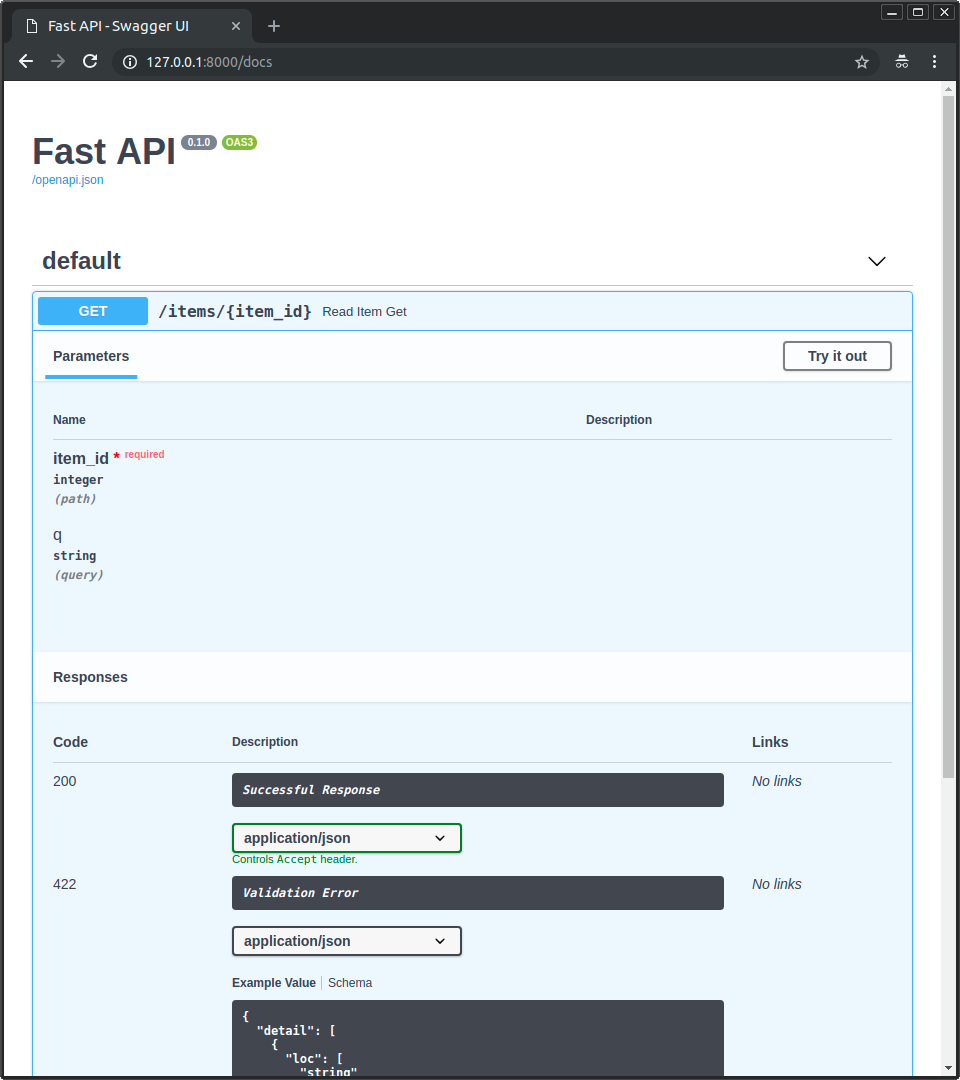
+
+### Alternative API docs
+
+And now, go to http://127.0.0.1:8000/redoc.
+
+You will see the alternative automatic documentation (provided by ReDoc):
+
+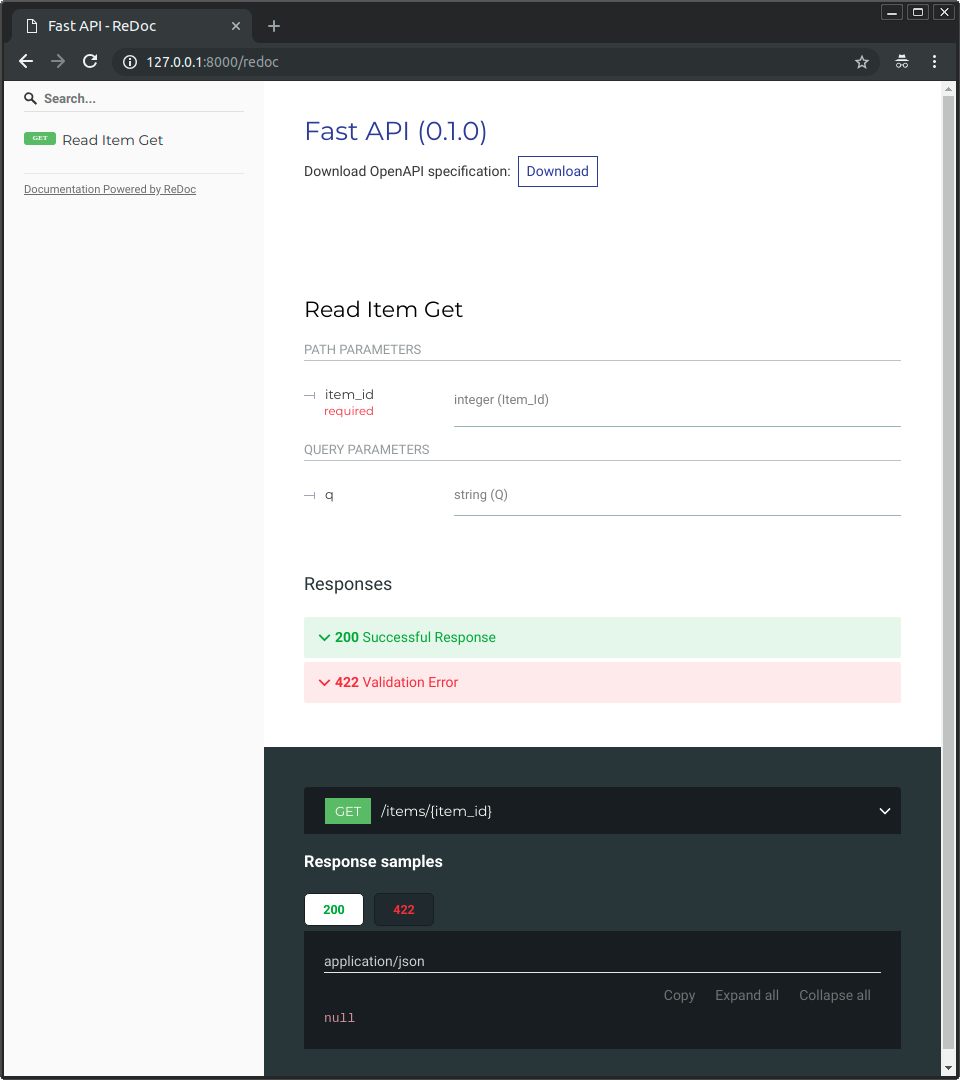
+
+## Example upgrade
+
+Now modify the file `main.py` to receive a body from a `PUT` request.
+
+Declare the body using standard Python types, thanks to Pydantic.
+
+```Python hl_lines="4 9-12 25-27"
+from typing import Union
+
+from fastapi import FastAPI
+from pydantic import BaseModel
+
+app = FastAPI()
+
+
+class Item(BaseModel):
+ name: str
+ price: float
+ is_offer: Union[bool, None] = None
+
+
+@app.get("/")
+def read_root():
+ return {"Hello": "World"}
+
+
+@app.get("/items/{item_id}")
+def read_item(item_id: int, q: Union[str, None] = None):
+ return {"item_id": item_id, "q": q}
+
+
+@app.put("/items/{item_id}")
+def update_item(item_id: int, item: Item):
+ return {"item_name": item.name, "item_id": item_id}
+```
+
+The server should reload automatically (because you added `--reload` to the `uvicorn` command above).
+
+### Interactive API docs upgrade
+
+Now go to http://127.0.0.1:8000/docs.
+
+* The interactive API documentation will be automatically updated, including the new body:
+
+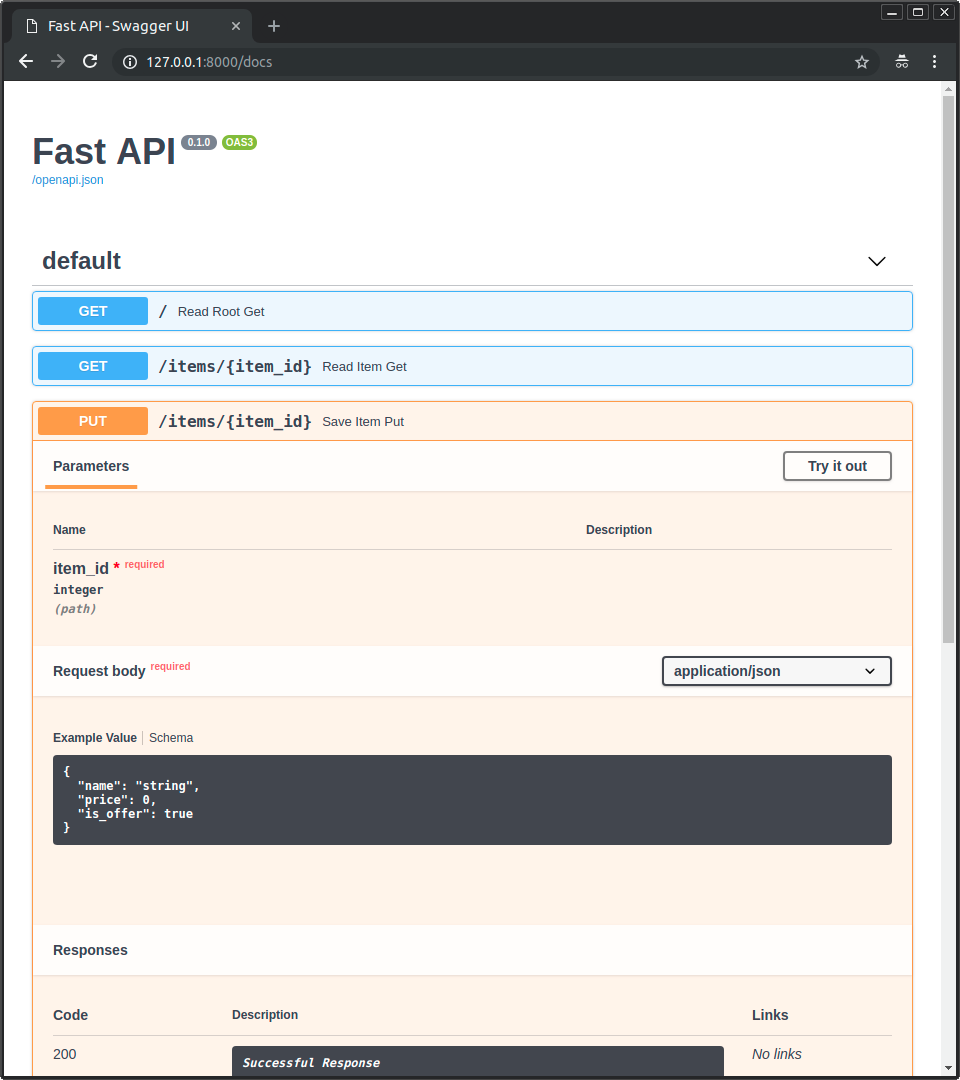
+
+* Click on the button "Try it out", it allows you to fill the parameters and directly interact with the API:
+
+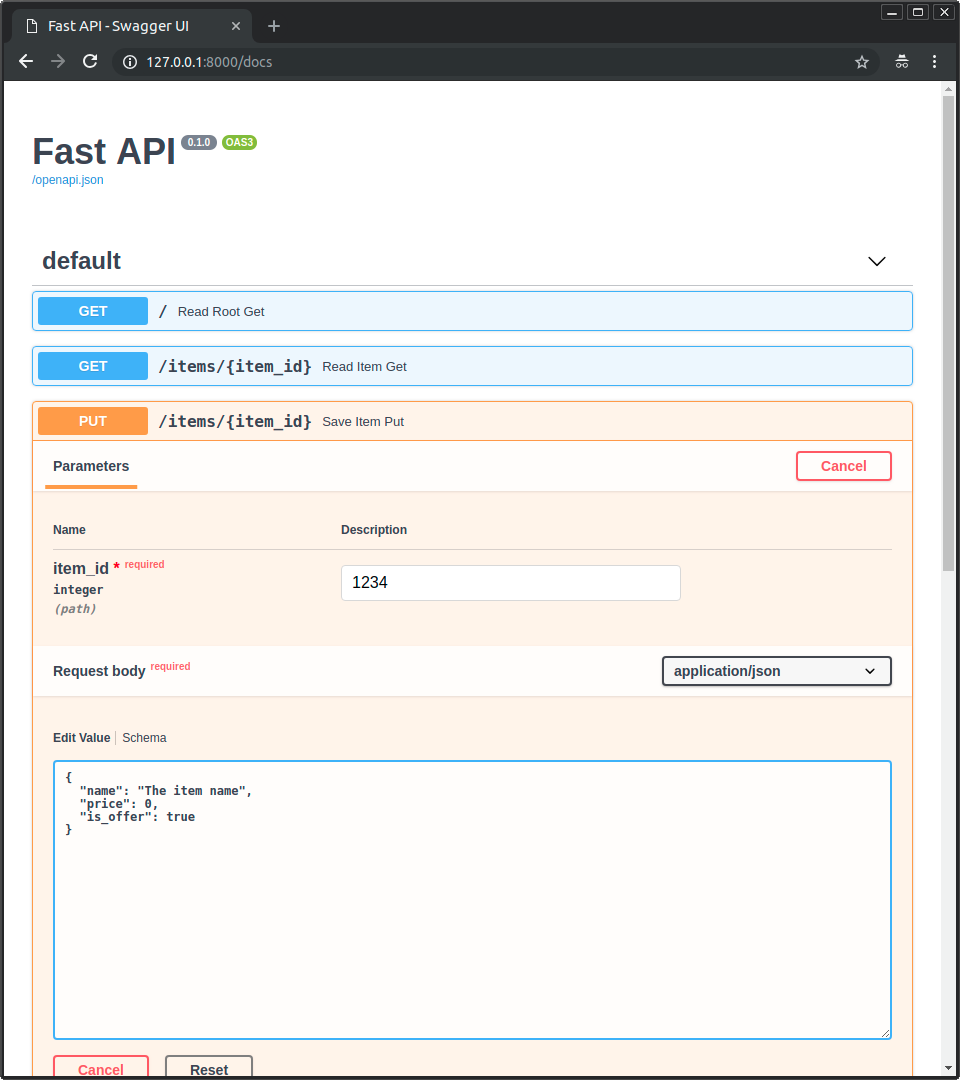
+
+* Then click on the "Execute" button, the user interface will communicate with your API, send the parameters, get the results and show them on the screen:
+
+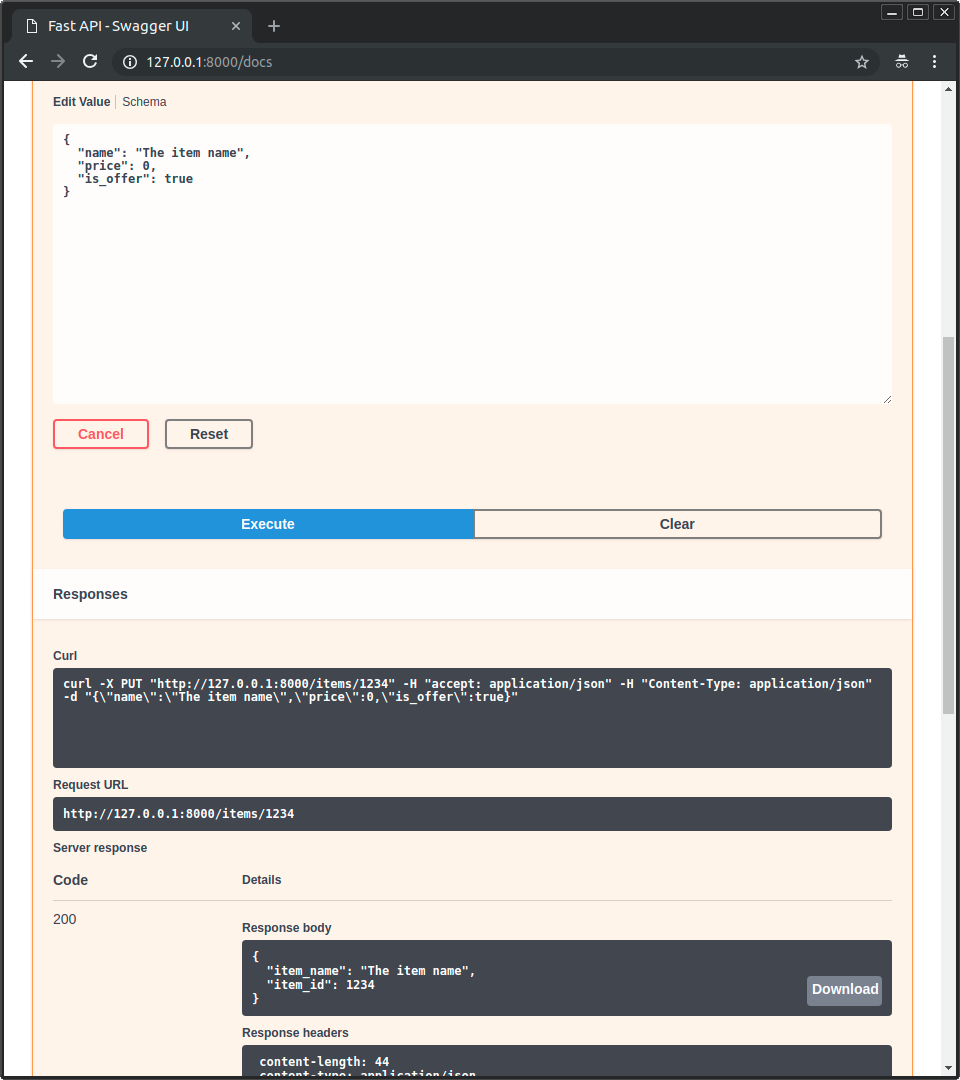
+
+### Alternative API docs upgrade
+
+And now, go to http://127.0.0.1:8000/redoc.
+
+* The alternative documentation will also reflect the new query parameter and body:
+
+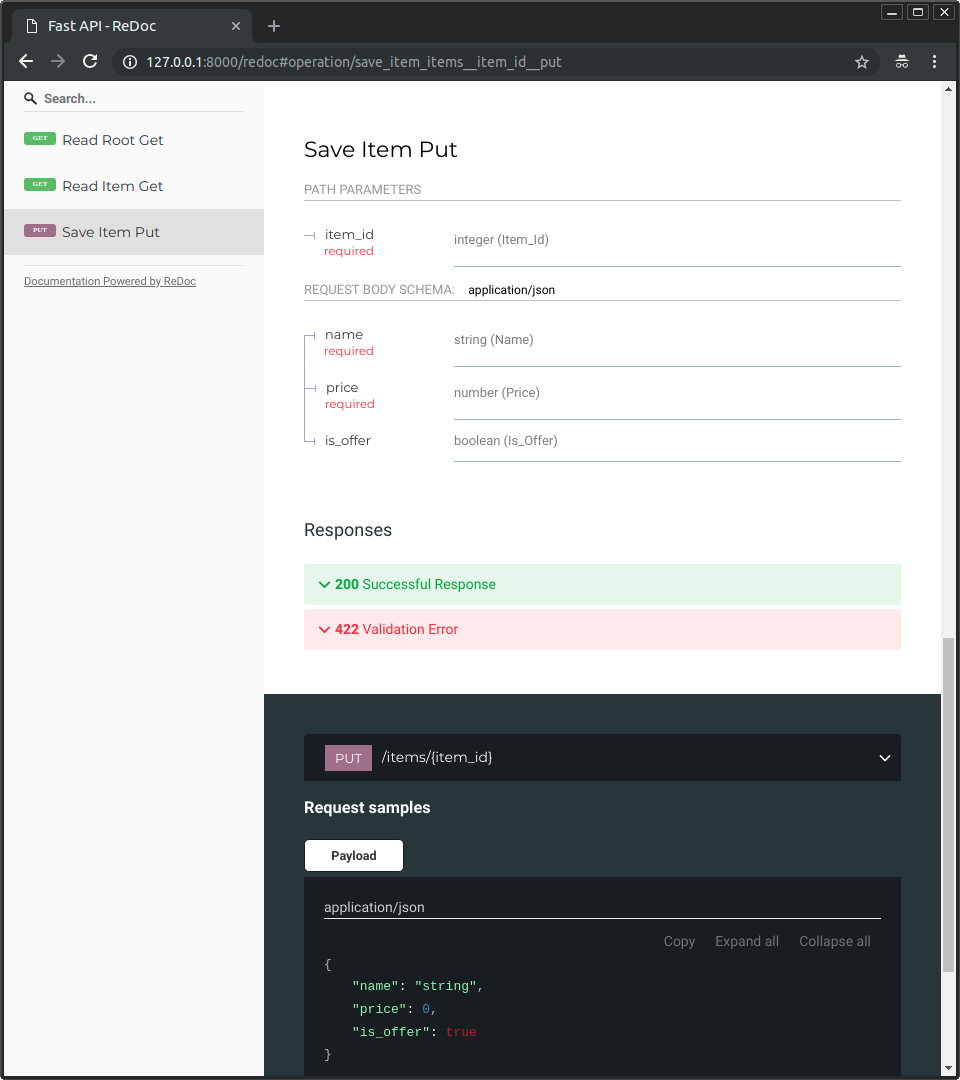
+
+### Recap
+
+In summary, you declare **once** the types of parameters, body, etc. as function parameters.
+
+You do that with standard modern Python types.
+
+You don't have to learn a new syntax, the methods or classes of a specific library, etc.
+
+Just standard **Python 3.6+**.
+
+For example, for an `int`:
+
+```Python
+item_id: int
+```
+
+or for a more complex `Item` model:
+
+```Python
+item: Item
+```
+
+...and with that single declaration you get:
+
+* Editor support, including:
+ * Completion.
+ * Type checks.
+* Validation of data:
+ * Automatic and clear errors when the data is invalid.
+ * Validation even for deeply nested JSON objects.
+* Conversion of input data: coming from the network to Python data and types. Reading from:
+ * JSON.
+ * Path parameters.
+ * Query parameters.
+ * Cookies.
+ * Headers.
+ * Forms.
+ * Files.
+* Conversion of output data: converting from Python data and types to network data (as JSON):
+ * Convert Python types (`str`, `int`, `float`, `bool`, `list`, etc).
+ * `datetime` objects.
+ * `UUID` objects.
+ * Database models.
+ * ...and many more.
+* Automatic interactive API documentation, including 2 alternative user interfaces:
+ * Swagger UI.
+ * ReDoc.
+
+---
+
+Coming back to the previous code example, **FastAPI** will:
+
+* Validate that there is an `item_id` in the path for `GET` and `PUT` requests.
+* Validate that the `item_id` is of type `int` for `GET` and `PUT` requests.
+ * If it is not, the client will see a useful, clear error.
+* Check if there is an optional query parameter named `q` (as in `http://127.0.0.1:8000/items/foo?q=somequery`) for `GET` requests.
+ * As the `q` parameter is declared with `= None`, it is optional.
+ * Without the `None` it would be required (as is the body in the case with `PUT`).
+* For `PUT` requests to `/items/{item_id}`, Read the body as JSON:
+ * Check that it has a required attribute `name` that should be a `str`.
+ * Check that it has a required attribute `price` that has to be a `float`.
+ * Check that it has an optional attribute `is_offer`, that should be a `bool`, if present.
+ * All this would also work for deeply nested JSON objects.
+* Convert from and to JSON automatically.
+* Document everything with OpenAPI, that can be used by:
+ * Interactive documentation systems.
+ * Automatic client code generation systems, for many languages.
+* Provide 2 interactive documentation web interfaces directly.
+
+---
+
+We just scratched the surface, but you already get the idea of how it all works.
+
+Try changing the line with:
+
+```Python
+ return {"item_name": item.name, "item_id": item_id}
+```
+
+...from:
+
+```Python
+ ... "item_name": item.name ...
+```
+
+...to:
+
+```Python
+ ... "item_price": item.price ...
+```
+
+...and see how your editor will auto-complete the attributes and know their types:
+
+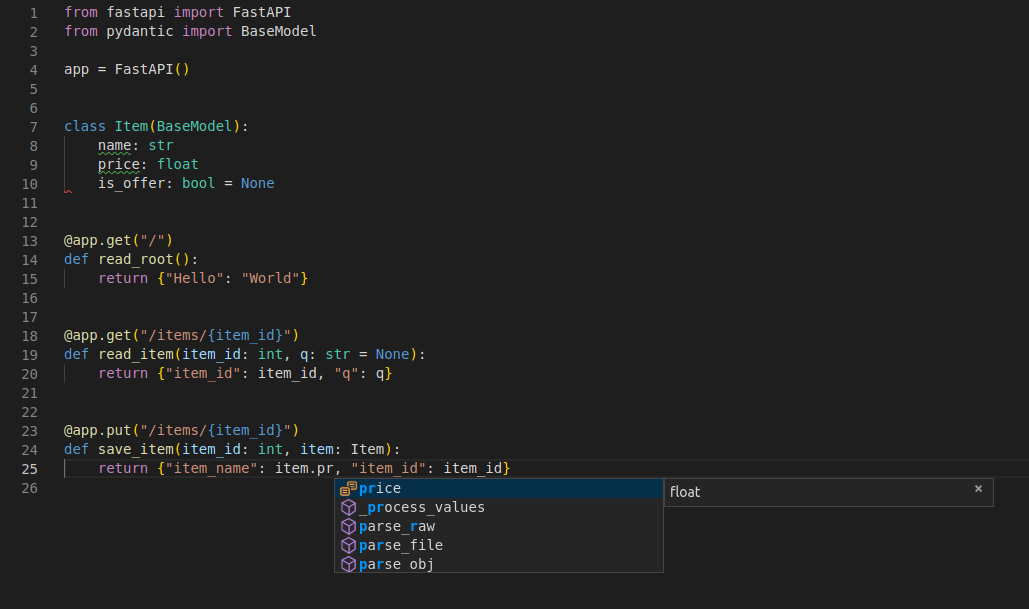
+
+For a more complete example including more features, see the Tutorial - User Guide.
+
+**Spoiler alert**: the tutorial - user guide includes:
+
+* Declaration of **parameters** from other different places as: **headers**, **cookies**, **form fields** and **files**.
+* How to set **validation constraints** as `maximum_length` or `regex`.
+* A very powerful and easy to use **Dependency Injection** system.
+* Security and authentication, including support for **OAuth2** with **JWT tokens** and **HTTP Basic** auth.
+* More advanced (but equally easy) techniques for declaring **deeply nested JSON models** (thanks to Pydantic).
+* **GraphQL** integration with Strawberry and other libraries.
+* Many extra features (thanks to Starlette) as:
+ * **WebSockets**
+ * extremely easy tests based on `requests` and `pytest`
+ * **CORS**
+ * **Cookie Sessions**
+ * ...and more.
+
+## Performance
+
+Independent TechEmpower benchmarks show **FastAPI** applications running under Uvicorn as one of the fastest Python frameworks available, only below Starlette and Uvicorn themselves (used internally by FastAPI). (*)
+
+To understand more about it, see the section Benchmarks.
+
+## Optional Dependencies
+
+Used by Pydantic:
+
+* ujson - for faster JSON "parsing".
+* email_validator - for email validation.
+
+Used by Starlette:
+
+* requests - Required if you want to use the `TestClient`.
+* jinja2 - Required if you want to use the default template configuration.
+* python-multipart - Required if you want to support form "parsing", with `request.form()`.
+* itsdangerous - Required for `SessionMiddleware` support.
+* pyyaml - Required for Starlette's `SchemaGenerator` support (you probably don't need it with FastAPI).
+* ujson - Required if you want to use `UJSONResponse`.
+
+Used by FastAPI / Starlette:
+
+* uvicorn - for the server that loads and serves your application.
+* orjson - Required if you want to use `ORJSONResponse`.
+
+You can install all of these with `pip install "fastapi[all]"`.
+
+## License
+
+This project is licensed under the terms of the MIT license.
diff --git a/docs/sv/mkdocs.yml b/docs/sv/mkdocs.yml
new file mode 100644
index 000000000..3332d232d
--- /dev/null
+++ b/docs/sv/mkdocs.yml
@@ -0,0 +1,145 @@
+site_name: FastAPI
+site_description: FastAPI framework, high performance, easy to learn, fast to code, ready for production
+site_url: https://fastapi.tiangolo.com/sv/
+theme:
+ name: material
+ custom_dir: overrides
+ palette:
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
+ primary: teal
+ accent: amber
+ toggle:
+ icon: material/lightbulb
+ name: Switch to light mode
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
+ primary: teal
+ accent: amber
+ toggle:
+ icon: material/lightbulb-outline
+ name: Switch to dark mode
+ features:
+ - search.suggest
+ - search.highlight
+ - content.tabs.link
+ icon:
+ repo: fontawesome/brands/github-alt
+ logo: https://fastapi.tiangolo.com/img/icon-white.svg
+ favicon: https://fastapi.tiangolo.com/img/favicon.png
+ language: sv
+repo_name: tiangolo/fastapi
+repo_url: https://github.com/tiangolo/fastapi
+edit_uri: ''
+plugins:
+- search
+- markdownextradata:
+ data: data
+nav:
+- FastAPI: index.md
+- Languages:
+ - en: /
+ - az: /az/
+ - de: /de/
+ - es: /es/
+ - fa: /fa/
+ - fr: /fr/
+ - he: /he/
+ - id: /id/
+ - it: /it/
+ - ja: /ja/
+ - ko: /ko/
+ - nl: /nl/
+ - pl: /pl/
+ - pt: /pt/
+ - ru: /ru/
+ - sq: /sq/
+ - sv: /sv/
+ - tr: /tr/
+ - uk: /uk/
+ - zh: /zh/
+markdown_extensions:
+- toc:
+ permalink: true
+- markdown.extensions.codehilite:
+ guess_lang: false
+- mdx_include:
+ base_path: docs
+- admonition
+- codehilite
+- extra
+- pymdownx.superfences:
+ custom_fences:
+ - name: mermaid
+ class: mermaid
+ format: !!python/name:pymdownx.superfences.fence_code_format ''
+- pymdownx.tabbed:
+ alternate_style: true
+- attr_list
+- md_in_html
+extra:
+ analytics:
+ provider: google
+ property: UA-133183413-1
+ social:
+ - icon: fontawesome/brands/github-alt
+ link: https://github.com/tiangolo/fastapi
+ - icon: fontawesome/brands/discord
+ link: https://discord.gg/VQjSZaeJmf
+ - icon: fontawesome/brands/twitter
+ link: https://twitter.com/fastapi
+ - icon: fontawesome/brands/linkedin
+ link: https://www.linkedin.com/in/tiangolo
+ - icon: fontawesome/brands/dev
+ link: https://dev.to/tiangolo
+ - icon: fontawesome/brands/medium
+ link: https://medium.com/@tiangolo
+ - icon: fontawesome/solid/globe
+ link: https://tiangolo.com
+ alternate:
+ - link: /
+ name: en - English
+ - link: /az/
+ name: az
+ - link: /de/
+ name: de
+ - link: /es/
+ name: es - español
+ - link: /fa/
+ name: fa
+ - link: /fr/
+ name: fr - français
+ - link: /he/
+ name: he
+ - link: /id/
+ name: id
+ - link: /it/
+ name: it - italiano
+ - link: /ja/
+ name: ja - 日本語
+ - link: /ko/
+ name: ko - 한국어
+ - link: /nl/
+ name: nl
+ - link: /pl/
+ name: pl
+ - link: /pt/
+ name: pt - português
+ - link: /ru/
+ name: ru - русский язык
+ - link: /sq/
+ name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
+ - link: /tr/
+ name: tr - Türkçe
+ - link: /uk/
+ name: uk - українська мова
+ - link: /zh/
+ name: zh - 汉语
+extra_css:
+- https://fastapi.tiangolo.com/css/termynal.css
+- https://fastapi.tiangolo.com/css/custom.css
+extra_javascript:
+- https://fastapi.tiangolo.com/js/termynal.js
+- https://fastapi.tiangolo.com/js/custom.js
diff --git a/docs/sv/overrides/.gitignore b/docs/sv/overrides/.gitignore
new file mode 100644
index 000000000..e69de29bb
diff --git a/docs/tr/docs/features.md b/docs/tr/docs/features.md
index c06c27c16..0bcda4e9c 100644
--- a/docs/tr/docs/features.md
+++ b/docs/tr/docs/features.md
@@ -6,7 +6,7 @@
### Açık standartları temel alır
-* API oluşturma işlemlerinde OpenAPI buna path operasyonları parametreleri, body talebi, güvenlik gibi şeyler dahil olmak üzere deklare bunların deklare edilmesi.
+* API oluşturma işlemlerinde OpenAPI buna path operasyonları parametreleri, body talebi, güvenlik gibi şeyler dahil olmak üzere deklare bunların deklare edilmesi.
* Otomatik olarak data modelinin JSON Schema ile beraber dokümante edilmesi (OpenAPI'n kendisi zaten JSON Schema'ya dayanıyor).
* Titiz bir çalışmanın sonucunda yukarıdaki standartlara uygun bir framework oluşturduk. Standartları pastanın üzerine sonradan eklenmiş bir çilek olarak görmedik.
* Ayrıca bu bir çok dilde kullanılabilecek **client code generator** kullanımına da izin veriyor.
@@ -74,7 +74,7 @@ my_second_user: User = User(**second_user_data)
### Editor desteği
-Bütün framework kullanılması kolay ve sezgileri güçlü olması için tasarlandı, verilen bütün kararlar geliştiricilere en iyi geliştirme deneyimini yaşatmak üzere, bir çok editör üzerinde test edildi.
+Bütün framework kullanılması kolay ve sezgileri güçlü olması için tasarlandı, verilen bütün kararlar geliştiricilere en iyi geliştirme deneyimini yaşatmak üzere, bir çok editör üzerinde test edildi.
Son yapılan Python geliştiricileri anketinde, açık ara en çok kullanılan özellik "oto-tamamlama" idi..
@@ -135,7 +135,7 @@ Bütün güvenlik şemaları OpenAPI'da tanımlanmış durumda, kapsadıkları:
Bütün güvenlik özellikleri Starlette'den geliyor (**session cookies'de** dahil olmak üzere).
-Bütün hepsi tekrardan kullanılabilir aletler ve bileşenler olarak, kolayca sistemlerinize, data depolarınıza, ilişkisel ve NoSQL databaselerinize entegre edebileceğiniz şekilde yapıldı.
+Bütün hepsi tekrardan kullanılabilir aletler ve bileşenler olarak, kolayca sistemlerinize, data depolarınıza, ilişkisel ve NoSQL databaselerinize entegre edebileceğiniz şekilde yapıldı.
### Dependency injection
@@ -198,7 +198,7 @@ Aynı şekilde, databaseden gelen objeyi de **direkt olarak isteğe** de tamamiy
* Kullandığın geliştirme araçları ile iyi çalışır **IDE/linter/brain**:
* Pydantic'in veri yapıları aslında sadece senin tanımladığın classlar; Bu yüzden doğrulanmış dataların ile otomatik tamamlama, linting ve mypy'ı kullanarak sorunsuz bir şekilde çalışabilirsin
* **Hızlı**:
- * Benchmarklarda, Pydantic'in diğer bütün test edilmiş bütün kütüphanelerden daha hızlı.
+ * Benchmarklarda, Pydantic'in diğer bütün test edilmiş bütün kütüphanelerden daha hızlı.
* **En kompleks** yapıları bile doğrula:
* Hiyerarşik Pydantic modellerinin kullanımı ile beraber, Python `typing`’s `List` and `Dict`, vs gibi şeyleri doğrula.
* Doğrulayıcılar en kompleks data şemalarının bile temiz ve kolay bir şekilde tanımlanmasına izin veriyor, ve hepsi JSON şeması olarak dokümante ediliyor
@@ -206,4 +206,3 @@ Aynı şekilde, databaseden gelen objeyi de **direkt olarak isteğe** de tamamiy
* **Genişletilebilir**:
* Pydantic özelleştirilmiş data tiplerinin tanımlanmasının yapılmasına izin veriyor ayrıca validator decoratorü ile senin doğrulamaları genişletip, kendi doğrulayıcılarını yazmana izin veriyor.
* 100% test kapsayıcılığı.
-
diff --git a/docs/tr/docs/index.md b/docs/tr/docs/index.md
index 88660f7eb..0d5337c87 100644
--- a/docs/tr/docs/index.md
+++ b/docs/tr/docs/index.md
@@ -28,7 +28,7 @@
---
-FastAPI, Python 3.6+'nın standart type hintlerine dayanan modern ve hızlı (yüksek performanslı) API'lar oluşturmak için kullanılabilecek web framework'ü.
+FastAPI, Python 3.6+'nın standart type hintlerine dayanan modern ve hızlı (yüksek performanslı) API'lar oluşturmak için kullanılabilecek web framework'ü.
Ana özellikleri:
@@ -143,7 +143,7 @@ Uygulamanı kullanılabilir hale getirmek için
```console
-$ pip install uvicorn[standard]
+$ pip install "uvicorn[standard]"
---> 100%
```
@@ -157,7 +157,7 @@ $ pip install uvicorn[standard]
* `main.py` adında bir dosya oluştur :
```Python
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -170,7 +170,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -180,7 +180,7 @@ def read_item(item_id: int, q: Optional[str] = None):
Eğer kodunda `async` / `await` var ise, `async def` kullan:
```Python hl_lines="9 14"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -193,7 +193,7 @@ async def read_root():
@app.get("/items/{item_id}")
-async def read_item(item_id: int, q: Optional[str] = None):
+async def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -272,7 +272,7 @@ Senin için alternatif olarak (requests - Eğer `TestClient` kullanmak istiyorsan gerekli.
-* aiofiles - `FileResponse` ya da `StaticFiles` kullanmak istiyorsan gerekli.
* jinja2 - Eğer kendine ait template konfigürasyonu oluşturmak istiyorsan gerekli
* python-multipart - Form kullanmak istiyorsan gerekli ("dönüşümü").
* itsdangerous - `SessionMiddleware` desteği için gerekli.
diff --git a/docs/tr/docs/python-types.md b/docs/tr/docs/python-types.md
index 7e46bd031..3b9ab9050 100644
--- a/docs/tr/docs/python-types.md
+++ b/docs/tr/docs/python-types.md
@@ -29,7 +29,7 @@ Programın çıktısı:
John Doe
```
-Fonksiyon sırayla şunları yapar:
+Fonksiyon sırayla şunları yapar:
* `first_name` ve `last_name` değerlerini alır.
* `title()` ile değişkenlerin ilk karakterlerini büyütür.
diff --git a/docs/tr/mkdocs.yml b/docs/tr/mkdocs.yml
index f6ed7f5b9..e29d25936 100644
--- a/docs/tr/mkdocs.yml
+++ b/docs/tr/mkdocs.yml
@@ -5,13 +5,15 @@ theme:
name: material
custom_dir: overrides
palette:
- - scheme: default
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
primary: teal
accent: amber
toggle:
icon: material/lightbulb
name: Switch to light mode
- - scheme: slate
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
primary: teal
accent: amber
toggle:
@@ -40,15 +42,19 @@ nav:
- az: /az/
- de: /de/
- es: /es/
+ - fa: /fa/
- fr: /fr/
+ - he: /he/
- id: /id/
- it: /it/
- ja: /ja/
- ko: /ko/
+ - nl: /nl/
- pl: /pl/
- pt: /pt/
- ru: /ru/
- sq: /sq/
+ - sv: /sv/
- tr: /tr/
- uk: /uk/
- zh: /zh/
@@ -72,6 +78,8 @@ markdown_extensions:
format: !!python/name:pymdownx.superfences.fence_code_format ''
- pymdownx.tabbed:
alternate_style: true
+- attr_list
+- md_in_html
extra:
analytics:
provider: google
@@ -100,8 +108,12 @@ extra:
name: de
- link: /es/
name: es - español
+ - link: /fa/
+ name: fa
- link: /fr/
name: fr - français
+ - link: /he/
+ name: he
- link: /id/
name: id
- link: /it/
@@ -110,6 +122,8 @@ extra:
name: ja - 日本語
- link: /ko/
name: ko - 한국어
+ - link: /nl/
+ name: nl
- link: /pl/
name: pl
- link: /pt/
@@ -118,6 +132,8 @@ extra:
name: ru - русский язык
- link: /sq/
name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
- link: /tr/
name: tr - Türkçe
- link: /uk/
diff --git a/docs/uk/docs/index.md b/docs/uk/docs/index.md
index 95fb7ae21..2b64003fe 100644
--- a/docs/uk/docs/index.md
+++ b/docs/uk/docs/index.md
@@ -135,7 +135,7 @@ You will also need an ASGI server, for production such as
```console
-$ pip install uvicorn[standard]
+$ pip install "uvicorn[standard]"
---> 100%
```
@@ -149,7 +149,7 @@ $ pip install uvicorn[standard]
* Create a file `main.py` with:
```Python
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -162,7 +162,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -172,7 +172,7 @@ def read_item(item_id: int, q: Optional[str] = None):
If your code uses `async` / `await`, use `async def`:
```Python hl_lines="9 14"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
@@ -185,7 +185,7 @@ async def read_root():
@app.get("/items/{item_id}")
-async def read_item(item_id: int, q: Optional[str] = None):
+async def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
```
@@ -264,7 +264,7 @@ Now modify the file `main.py` to receive a body from a `PUT` request.
Declare the body using standard Python types, thanks to Pydantic.
```Python hl_lines="4 9-12 25-27"
-from typing import Optional
+from typing import Union
from fastapi import FastAPI
from pydantic import BaseModel
@@ -275,7 +275,7 @@ app = FastAPI()
class Item(BaseModel):
name: str
price: float
- is_offer: Optional[bool] = None
+ is_offer: Union[bool, None] = None
@app.get("/")
@@ -284,7 +284,7 @@ def read_root():
@app.get("/items/{item_id}")
-def read_item(item_id: int, q: Optional[str] = None):
+def read_item(item_id: int, q: Union[str, None] = None):
return {"item_id": item_id, "q": q}
@@ -321,7 +321,7 @@ And now, go to requests - Required if you want to use the `TestClient`.
-* aiofiles - Required if you want to use `FileResponse` or `StaticFiles`.
* jinja2 - Required if you want to use the default template configuration.
* python-multipart - Required if you want to support form "parsing", with `request.form()`.
* itsdangerous - Required for `SessionMiddleware` support.
diff --git a/docs/uk/mkdocs.yml b/docs/uk/mkdocs.yml
index d0de8cc0e..711328771 100644
--- a/docs/uk/mkdocs.yml
+++ b/docs/uk/mkdocs.yml
@@ -5,13 +5,15 @@ theme:
name: material
custom_dir: overrides
palette:
- - scheme: default
+ - media: '(prefers-color-scheme: light)'
+ scheme: default
primary: teal
accent: amber
toggle:
icon: material/lightbulb
name: Switch to light mode
- - scheme: slate
+ - media: '(prefers-color-scheme: dark)'
+ scheme: slate
primary: teal
accent: amber
toggle:
@@ -40,15 +42,19 @@ nav:
- az: /az/
- de: /de/
- es: /es/
+ - fa: /fa/
- fr: /fr/
+ - he: /he/
- id: /id/
- it: /it/
- ja: /ja/
- ko: /ko/
+ - nl: /nl/
- pl: /pl/
- pt: /pt/
- ru: /ru/
- sq: /sq/
+ - sv: /sv/
- tr: /tr/
- uk: /uk/
- zh: /zh/
@@ -69,6 +75,8 @@ markdown_extensions:
format: !!python/name:pymdownx.superfences.fence_code_format ''
- pymdownx.tabbed:
alternate_style: true
+- attr_list
+- md_in_html
extra:
analytics:
provider: google
@@ -97,8 +105,12 @@ extra:
name: de
- link: /es/
name: es - español
+ - link: /fa/
+ name: fa
- link: /fr/
name: fr - français
+ - link: /he/
+ name: he
- link: /id/
name: id
- link: /it/
@@ -107,6 +119,8 @@ extra:
name: ja - 日本語
- link: /ko/
name: ko - 한국어
+ - link: /nl/
+ name: nl
- link: /pl/
name: pl
- link: /pt/
@@ -115,6 +129,8 @@ extra:
name: ru - русский язык
- link: /sq/
name: sq - shqip
+ - link: /sv/
+ name: sv - svenska
- link: /tr/
name: tr - Türkçe
- link: /uk/
diff --git a/docs/zh/docs/advanced/additional-status-codes.md b/docs/zh/docs/advanced/additional-status-codes.md
index 1cb724f1d..54ec9775b 100644
--- a/docs/zh/docs/advanced/additional-status-codes.md
+++ b/docs/zh/docs/advanced/additional-status-codes.md
@@ -14,7 +14,7 @@
要实现它,导入 `JSONResponse`,然后在其中直接返回你的内容,并将 `status_code` 设置为为你要的值。
-```Python hl_lines="2 19"
+```Python hl_lines="4 25"
{!../../../docs_src/additional_status_codes/tutorial001.py!}
```
@@ -22,7 +22,7 @@
当你直接返回一个像上面例子中的 `Response` 对象时,它会直接返回。
FastAPI 不会用模型等对该响应进行序列化。
-
+
确保其中有你想要的数据,且返回的值为合法的 JSON(如果你使用 `JSONResponse` 的话)。
!!! note "技术细节"
diff --git a/docs/zh/docs/advanced/custom-response.md b/docs/zh/docs/advanced/custom-response.md
index 5f1a74e9e..155ce2882 100644
--- a/docs/zh/docs/advanced/custom-response.md
+++ b/docs/zh/docs/advanced/custom-response.md
@@ -60,7 +60,7 @@
正如你在 [直接返回响应](response-directly.md){.internal-link target=_blank} 中了解到的,你也可以通过直接返回响应在 *路径操作* 中直接重载响应。
和上面一样的例子,返回一个 `HTMLResponse` 看起来可能是这样:
-
+
```Python hl_lines="2 7 19"
{!../../../docs_src/custom_response/tutorial003.py!}
```
diff --git a/docs/zh/docs/advanced/response-cookies.md b/docs/zh/docs/advanced/response-cookies.md
new file mode 100644
index 000000000..3e53c5319
--- /dev/null
+++ b/docs/zh/docs/advanced/response-cookies.md
@@ -0,0 +1,47 @@
+# 响应Cookies
+
+## 使用 `Response` 参数
+
+你可以在 *路径函数* 中定义一个类型为 `Response`的参数,这样你就可以在这个临时响应对象中设置cookie了。
+
+```Python hl_lines="1 8-9"
+{!../../../docs_src/response_cookies/tutorial002.py!}
+```
+
+而且你还可以根据你的需要响应不同的对象,比如常用的 `dict`,数据库model等。
+
+如果你定义了 `response_model`,程序会自动根据`response_model`来过滤和转换你响应的对象。
+
+**FastAPI** 会使用这个 *临时* 响应对象去装在这些cookies信息 (同样还有headers和状态码等信息), 最终会将这些信息和通过`response_model`转化过的数据合并到最终的响应里。
+
+你也可以在depend中定义`Response`参数,并设置cookie和header。
+
+## 直接响应 `Response`
+
+你还可以在直接响应`Response`时直接创建cookies。
+
+你可以参考[Return a Response Directly](response-directly.md){.internal-link target=_blank}来创建response
+
+然后设置Cookies,并返回:
+
+```Python hl_lines="10-12"
+{!../../../docs_src/response_cookies/tutorial001.py!}
+```
+
+!!! tip
+ 需要注意,如果你直接反馈一个response对象,而不是使用`Response`入参,FastAPI则会直接反馈你封装的response对象。
+
+ 所以你需要确保你响应数据类型的正确性,如:你可以使用`JSONResponse`来兼容JSON的场景。
+
+ 同时,你也应当仅反馈通过`response_model`过滤过的数据。
+
+### 更多信息
+
+!!! note "技术细节"
+ 你也可以使用`from starlette.responses import Response` 或者 `from starlette.responses import JSONResponse`。
+
+ 为了方便开发者,**FastAPI** 封装了相同数据类型,如`starlette.responses` 和 `fastapi.responses`。不过大部分response对象都是直接引用自Starlette。
+
+ 因为`Response`对象可以非常便捷的设置headers和cookies,所以 **FastAPI** 同时也封装了`fastapi.Response`。
+
+如果你想查看所有可用的参数和选项,可以参考 Starlette帮助文档
diff --git a/docs/zh/docs/advanced/response-directly.md b/docs/zh/docs/advanced/response-directly.md
index 05926a9c8..797a878eb 100644
--- a/docs/zh/docs/advanced/response-directly.md
+++ b/docs/zh/docs/advanced/response-directly.md
@@ -10,7 +10,7 @@
直接返回响应可能会有用处,比如返回自定义的响应头和 cookies。
-## 返回 `Response`
+## 返回 `Response`
事实上,你可以返回任意 `Response` 或者任意 `Response` 的子类。
@@ -62,4 +62,3 @@
但是你仍可以参考 [OpenApI 中的额外响应](additional-responses.md){.internal-link target=_blank} 给响应编写文档。
在后续的章节中你可以了解到如何使用/声明这些自定义的 `Response` 的同时还保留自动化的数据转换和文档等。
-
diff --git a/docs/zh/docs/benchmarks.md b/docs/zh/docs/benchmarks.md
index c133d51b7..8991c72cd 100644
--- a/docs/zh/docs/benchmarks.md
+++ b/docs/zh/docs/benchmarks.md
@@ -22,7 +22,7 @@
* 具有最佳性能,因为除了服务器本身外,它没有太多额外的代码。
* 您不会直接在 Uvicorn 中编写应用程序。这意味着您的代码至少必须包含 Starlette(或 **FastAPI**)提供的代码。如果您这样做了(即直接在 Uvicorn 中编写应用程序),最终的应用程序会和使用了框架并且最小化了应用代码和 bug 的情况具有相同的性能损耗。
* 如果要对比与 Uvicorn 对标的服务器,请将其与 Daphne,Hypercorn,uWSGI等应用服务器进行比较。
-* **Starlette**:
+* **Starlette**:
* 在 Uvicorn 后使用 Starlette,性能会略有下降。实际上,Starlette 使用 Uvicorn运行。因此,由于必须执行更多的代码,它只会比 Uvicorn 更慢。
* 但它为您提供了构建简单的网络程序的工具,并具有基于路径的路由等功能。
* 如果想对比与 Starlette 对标的开发框架,请将其与 Sanic,Flask,Django 等网络框架(或微框架)进行比较。
diff --git a/docs/zh/docs/contributing.md b/docs/zh/docs/contributing.md
index 402668c47..95500d12b 100644
--- a/docs/zh/docs/contributing.md
+++ b/docs/zh/docs/contributing.md
@@ -497,4 +497,4 @@ $ bash scripts/test-cov-html.sh