committed by
GitHub
199 changed files with 11796 additions and 1110 deletions
@ -2,7 +2,7 @@ name: Issue Manager |
|||
|
|||
on: |
|||
schedule: |
|||
- cron: "10 3 * * *" |
|||
- cron: "13 22 * * *" |
|||
issue_comment: |
|||
types: |
|||
- created |
|||
@ -16,6 +16,7 @@ on: |
|||
|
|||
permissions: |
|||
issues: write |
|||
pull-requests: write |
|||
|
|||
jobs: |
|||
issue-manager: |
|||
@ -26,7 +27,7 @@ jobs: |
|||
env: |
|||
GITHUB_CONTEXT: ${{ toJson(github) }} |
|||
run: echo "$GITHUB_CONTEXT" |
|||
- uses: tiangolo/[email protected].0 |
|||
- uses: tiangolo/[email protected].1 |
|||
with: |
|||
token: ${{ secrets.GITHUB_TOKEN }} |
|||
config: > |
|||
@ -35,8 +36,8 @@ jobs: |
|||
"delay": 864000, |
|||
"message": "Assuming the original need was handled, this will be automatically closed now. But feel free to add more comments or create new issues or PRs." |
|||
}, |
|||
"changes-requested": { |
|||
"waiting": { |
|||
"delay": 2628000, |
|||
"message": "As this PR had requested changes to be applied but has been inactive for a while, it's now going to be closed. But if there's anyone interested, feel free to create a new PR." |
|||
"message": "As this PR has been waiting for the original user for a while but seems to be inactive, it's now going to be closed. But if there's anyone interested, feel free to create a new PR." |
|||
} |
|||
} |
|||
|
@ -34,8 +34,7 @@ jobs: |
|||
if: ${{ github.event_name == 'workflow_dispatch' && github.event.inputs.debug_enabled == 'true' }} |
|||
with: |
|||
limit-access-to-actor: true |
|||
- uses: docker://tiangolo/latest-changes:0.3.0 |
|||
# - uses: tiangolo/latest-changes@main |
|||
- uses: tiangolo/[email protected] |
|||
with: |
|||
token: ${{ secrets.GITHUB_TOKEN }} |
|||
latest_changes_file: docs/en/docs/release-notes.md |
|||
|
@ -0,0 +1,298 @@ |
|||
# Environment Variables |
|||
|
|||
/// tip |
|||
|
|||
If you already know what "environment variables" are and how to use them, feel free to skip this. |
|||
|
|||
/// |
|||
|
|||
An environment variable (also known as "**env var**") is a variable that lives **outside** of the Python code, in the **operating system**, and could be read by your Python code (or by other programs as well). |
|||
|
|||
Environment variables could be useful for handling application **settings**, as part of the **installation** of Python, etc. |
|||
|
|||
## Create and Use Env Vars |
|||
|
|||
You can **create** and use environment variables in the **shell (terminal)**, without needing Python: |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// You could create an env var MY_NAME with |
|||
$ export MY_NAME="Wade Wilson" |
|||
|
|||
// Then you could use it with other programs, like |
|||
$ echo "Hello $MY_NAME" |
|||
|
|||
Hello Wade Wilson |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Create an env var MY_NAME |
|||
$ $Env:MY_NAME = "Wade Wilson" |
|||
|
|||
// Use it with other programs, like |
|||
$ echo "Hello $Env:MY_NAME" |
|||
|
|||
Hello Wade Wilson |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
## Read env vars in Python |
|||
|
|||
You could also create environment variables **outside** of Python, in the terminal (or with any other method), and then **read them in Python**. |
|||
|
|||
For example you could have a file `main.py` with: |
|||
|
|||
```Python hl_lines="3" |
|||
import os |
|||
|
|||
name = os.getenv("MY_NAME", "World") |
|||
print(f"Hello {name} from Python") |
|||
``` |
|||
|
|||
/// tip |
|||
|
|||
The second argument to <a href="https://docs.python.org/3.8/library/os.html#os.getenv" class="external-link" target="_blank">`os.getenv()`</a> is the default value to return. |
|||
|
|||
If not provided, it's `None` by default, here we provide `"World"` as the default value to use. |
|||
|
|||
/// |
|||
|
|||
Then you could call that Python program: |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Here we don't set the env var yet |
|||
$ python main.py |
|||
|
|||
// As we didn't set the env var, we get the default value |
|||
|
|||
Hello World from Python |
|||
|
|||
// But if we create an environment variable first |
|||
$ export MY_NAME="Wade Wilson" |
|||
|
|||
// And then call the program again |
|||
$ python main.py |
|||
|
|||
// Now it can read the environment variable |
|||
|
|||
Hello Wade Wilson from Python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Here we don't set the env var yet |
|||
$ python main.py |
|||
|
|||
// As we didn't set the env var, we get the default value |
|||
|
|||
Hello World from Python |
|||
|
|||
// But if we create an environment variable first |
|||
$ $Env:MY_NAME = "Wade Wilson" |
|||
|
|||
// And then call the program again |
|||
$ python main.py |
|||
|
|||
// Now it can read the environment variable |
|||
|
|||
Hello Wade Wilson from Python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
As environment variables can be set outside of the code, but can be read by the code, and don't have to be stored (committed to `git`) with the rest of the files, it's common to use them for configurations or **settings**. |
|||
|
|||
You can also create an environment variable only for a **specific program invocation**, that is only available to that program, and only for its duration. |
|||
|
|||
To do that, create it right before the program itself, on the same line: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Create an env var MY_NAME in line for this program call |
|||
$ MY_NAME="Wade Wilson" python main.py |
|||
|
|||
// Now it can read the environment variable |
|||
|
|||
Hello Wade Wilson from Python |
|||
|
|||
// The env var no longer exists afterwards |
|||
$ python main.py |
|||
|
|||
Hello World from Python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// tip |
|||
|
|||
You can read more about it at <a href="https://12factor.net/config" class="external-link" target="_blank">The Twelve-Factor App: Config</a>. |
|||
|
|||
/// |
|||
|
|||
## Types and Validation |
|||
|
|||
These environment variables can only handle **text strings**, as they are external to Python and have to be compatible with other programs and the rest of the system (and even with different operating systems, as Linux, Windows, macOS). |
|||
|
|||
That means that **any value** read in Python from an environment variable **will be a `str`**, and any conversion to a different type or any validation has to be done in code. |
|||
|
|||
You will learn more about using environment variables for handling **application settings** in the [Advanced User Guide - Settings and Environment Variables](./advanced/settings.md){.internal-link target=_blank}. |
|||
|
|||
## `PATH` Environment Variable |
|||
|
|||
There is a **special** environment variable called **`PATH`** that is used by the operating systems (Linux, macOS, Windows) to find programs to run. |
|||
|
|||
The value of the variable `PATH` is a long string that is made of directories separated by a colon `:` on Linux and macOS, and by a semicolon `;` on Windows. |
|||
|
|||
For example, the `PATH` environment variable could look like this: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
```plaintext |
|||
/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin |
|||
``` |
|||
|
|||
This means that the system should look for programs in the directories: |
|||
|
|||
* `/usr/local/bin` |
|||
* `/usr/bin` |
|||
* `/bin` |
|||
* `/usr/sbin` |
|||
* `/sbin` |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
```plaintext |
|||
C:\Program Files\Python312\Scripts;C:\Program Files\Python312;C:\Windows\System32 |
|||
``` |
|||
|
|||
This means that the system should look for programs in the directories: |
|||
|
|||
* `C:\Program Files\Python312\Scripts` |
|||
* `C:\Program Files\Python312` |
|||
* `C:\Windows\System32` |
|||
|
|||
//// |
|||
|
|||
When you type a **command** in the terminal, the operating system **looks for** the program in **each of those directories** listed in the `PATH` environment variable. |
|||
|
|||
For example, when you type `python` in the terminal, the operating system looks for a program called `python` in the **first directory** in that list. |
|||
|
|||
If it finds it, then it will **use it**. Otherwise it keeps looking in the **other directories**. |
|||
|
|||
### Installing Python and Updating the `PATH` |
|||
|
|||
When you install Python, you might be asked if you want to update the `PATH` environment variable. |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
Let's say you install Python and it ends up in a directory `/opt/custompython/bin`. |
|||
|
|||
If you say yes to update the `PATH` environment variable, then the installer will add `/opt/custompython/bin` to the `PATH` environment variable. |
|||
|
|||
It could look like this: |
|||
|
|||
```plaintext |
|||
/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/opt/custompython/bin |
|||
``` |
|||
|
|||
This way, when you type `python` in the terminal, the system will find the Python program in `/opt/custompython/bin` (the last directory) and use that one. |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
Let's say you install Python and it ends up in a directory `C:\opt\custompython\bin`. |
|||
|
|||
If you say yes to update the `PATH` environment variable, then the installer will add `C:\opt\custompython\bin` to the `PATH` environment variable. |
|||
|
|||
```plaintext |
|||
C:\Program Files\Python312\Scripts;C:\Program Files\Python312;C:\Windows\System32;C:\opt\custompython\bin |
|||
``` |
|||
|
|||
This way, when you type `python` in the terminal, the system will find the Python program in `C:\opt\custompython\bin` (the last directory) and use that one. |
|||
|
|||
//// |
|||
|
|||
So, if you type: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
The system will **find** the `python` program in `/opt/custompython/bin` and run it. |
|||
|
|||
It would be roughly equivalent to typing: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ /opt/custompython/bin/python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
The system will **find** the `python` program in `C:\opt\custompython\bin\python` and run it. |
|||
|
|||
It would be roughly equivalent to typing: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ C:\opt\custompython\bin\python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
This information will be useful when learning about [Virtual Environments](virtual-environments.md){.internal-link target=_blank}. |
|||
|
|||
## Conclusion |
|||
|
|||
With this you should have a basic understanding of what **environment variables** are and how to use them in Python. |
|||
|
|||
You can also read more about them in the <a href="https://en.wikipedia.org/wiki/Environment_variable" class="external-link" target="_blank">Wikipedia for Environment Variable</a>. |
|||
|
|||
In many cases it's not very obvious how environment variables would be useful and applicable right away. But they keep showing up in many different scenarios when you are developing, so it's good to know about them. |
|||
|
|||
For example, you will need this information in the next section, about [Virtual Environments](virtual-environments.md). |
After Width: | Height: | Size: 44 KiB |
After Width: | Height: | Size: 61 KiB |
After Width: | Height: | Size: 44 KiB |
After Width: | Height: | Size: 43 KiB |
@ -0,0 +1,154 @@ |
|||
# Cookie Parameter Models |
|||
|
|||
If you have a group of **cookies** that are related, you can create a **Pydantic model** to declare them. 🍪 |
|||
|
|||
This would allow you to **re-use the model** in **multiple places** and also to declare validations and metadata for all the parameters at once. 😎 |
|||
|
|||
/// note |
|||
|
|||
This is supported since FastAPI version `0.115.0`. 🤓 |
|||
|
|||
/// |
|||
|
|||
/// tip |
|||
|
|||
This same technique applies to `Query`, `Cookie`, and `Header`. 😎 |
|||
|
|||
/// |
|||
|
|||
## Cookies with a Pydantic Model |
|||
|
|||
Declare the **cookie** parameters that you need in a **Pydantic model**, and then declare the parameter as `Cookie`: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="9-12 16" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial001_an_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9-12 16" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="10-13 17" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.10+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7-10 14" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial001_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="9-12 16" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial001.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
**FastAPI** will **extract** the data for **each field** from the **cookies** received in the request and give you the Pydantic model you defined. |
|||
|
|||
## Check the Docs |
|||
|
|||
You can see the defined cookies in the docs UI at `/docs`: |
|||
|
|||
<div class="screenshot"> |
|||
<img src="/img/tutorial/cookie-param-models/image01.png"> |
|||
</div> |
|||
|
|||
/// info |
|||
|
|||
Have in mind that, as **browsers handle cookies** in special ways and behind the scenes, they **don't** easily allow **JavaScript** to touch them. |
|||
|
|||
If you go to the **API docs UI** at `/docs` you will be able to see the **documentation** for cookies for your *path operations*. |
|||
|
|||
But even if you **fill the data** and click "Execute", because the docs UI works with **JavaScript**, the cookies won't be sent, and you will see an **error** message as if you didn't write any values. |
|||
|
|||
/// |
|||
|
|||
## Forbid Extra Cookies |
|||
|
|||
In some special use cases (probably not very common), you might want to **restrict** the cookies that you want to receive. |
|||
|
|||
Your API now has the power to control its own <abbr title="This is a joke, just in case. It has nothing to do with cookie consents, but it's funny that even the API can now reject the poor cookies. Have a cookie. 🍪">cookie consent</abbr>. 🤪🍪 |
|||
|
|||
You can use Pydantic's model configuration to `forbid` any `extra` fields: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial002_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="11" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial002_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/cookie_param_models/tutorial002.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
If a client tries to send some **extra cookies**, they will receive an **error** response. |
|||
|
|||
Poor cookie banners with all their effort to get your consent for the <abbr title="This is another joke. Don't pay attention to me. Have some coffee for your cookie. ☕">API to reject it</abbr>. 🍪 |
|||
|
|||
For example, if the client tries to send a `santa_tracker` cookie with a value of `good-list-please`, the client will receive an **error** response telling them that the `santa_tracker` <abbr title="Santa disapproves the lack of cookies. 🎅 Okay, no more cookie jokes.">cookie is not allowed</abbr>: |
|||
|
|||
```json |
|||
{ |
|||
"detail": [ |
|||
{ |
|||
"type": "extra_forbidden", |
|||
"loc": ["cookie", "santa_tracker"], |
|||
"msg": "Extra inputs are not permitted", |
|||
"input": "good-list-please", |
|||
} |
|||
] |
|||
} |
|||
``` |
|||
|
|||
## Summary |
|||
|
|||
You can use **Pydantic models** to declare <abbr title="Have a last cookie before you go. 🍪">**cookies**</abbr> in **FastAPI**. 😎 |
@ -0,0 +1,184 @@ |
|||
# Header Parameter Models |
|||
|
|||
If you have a group of related **header parameters**, you can create a **Pydantic model** to declare them. |
|||
|
|||
This would allow you to **re-use the model** in **multiple places** and also to declare validations and metadata for all the parameters at once. 😎 |
|||
|
|||
/// note |
|||
|
|||
This is supported since FastAPI version `0.115.0`. 🤓 |
|||
|
|||
/// |
|||
|
|||
## Header Parameters with a Pydantic Model |
|||
|
|||
Declare the **header parameters** that you need in a **Pydantic model**, and then declare the parameter as `Header`: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="9-14 18" |
|||
{!> ../../../docs_src/header_param_models/tutorial001_an_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9-14 18" |
|||
{!> ../../../docs_src/header_param_models/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="10-15 19" |
|||
{!> ../../../docs_src/header_param_models/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.10+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7-12 16" |
|||
{!> ../../../docs_src/header_param_models/tutorial001_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="9-14 18" |
|||
{!> ../../../docs_src/header_param_models/tutorial001_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7-12 16" |
|||
{!> ../../../docs_src/header_param_models/tutorial001_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
**FastAPI** will **extract** the data for **each field** from the **headers** in the request and give you the Pydantic model you defined. |
|||
|
|||
## Check the Docs |
|||
|
|||
You can see the required headers in the docs UI at `/docs`: |
|||
|
|||
<div class="screenshot"> |
|||
<img src="/img/tutorial/header-param-models/image01.png"> |
|||
</div> |
|||
|
|||
## Forbid Extra Headers |
|||
|
|||
In some special use cases (probably not very common), you might want to **restrict** the headers that you want to receive. |
|||
|
|||
You can use Pydantic's model configuration to `forbid` any `extra` fields: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/header_param_models/tutorial002_an_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/header_param_models/tutorial002_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="11" |
|||
{!> ../../../docs_src/header_param_models/tutorial002_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.10+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="8" |
|||
{!> ../../../docs_src/header_param_models/tutorial002_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/header_param_models/tutorial002_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/header_param_models/tutorial002.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
If a client tries to send some **extra headers**, they will receive an **error** response. |
|||
|
|||
For example, if the client tries to send a `tool` header with a value of `plumbus`, they will receive an **error** response telling them that the header parameter `tool` is not allowed: |
|||
|
|||
```json |
|||
{ |
|||
"detail": [ |
|||
{ |
|||
"type": "extra_forbidden", |
|||
"loc": ["header", "tool"], |
|||
"msg": "Extra inputs are not permitted", |
|||
"input": "plumbus", |
|||
} |
|||
] |
|||
} |
|||
``` |
|||
|
|||
## Summary |
|||
|
|||
You can use **Pydantic models** to declare **headers** in **FastAPI**. 😎 |
@ -0,0 +1,196 @@ |
|||
# Query Parameter Models |
|||
|
|||
If you have a group of **query parameters** that are related, you can create a **Pydantic model** to declare them. |
|||
|
|||
This would allow you to **re-use the model** in **multiple places** and also to declare validations and metadata for all the parameters at once. 😎 |
|||
|
|||
/// note |
|||
|
|||
This is supported since FastAPI version `0.115.0`. 🤓 |
|||
|
|||
/// |
|||
|
|||
## Query Parameters with a Pydantic Model |
|||
|
|||
Declare the **query parameters** that you need in a **Pydantic model**, and then declare the parameter as `Query`: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="9-13 17" |
|||
{!> ../../../docs_src/query_param_models/tutorial001_an_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="8-12 16" |
|||
{!> ../../../docs_src/query_param_models/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="10-14 18" |
|||
{!> ../../../docs_src/query_param_models/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.10+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="9-13 17" |
|||
{!> ../../../docs_src/query_param_models/tutorial001_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="8-12 16" |
|||
{!> ../../../docs_src/query_param_models/tutorial001_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="9-13 17" |
|||
{!> ../../../docs_src/query_param_models/tutorial001_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
**FastAPI** will **extract** the data for **each field** from the **query parameters** in the request and give you the Pydantic model you defined. |
|||
|
|||
## Check the Docs |
|||
|
|||
You can see the query parameters in the docs UI at `/docs`: |
|||
|
|||
<div class="screenshot"> |
|||
<img src="/img/tutorial/query-param-models/image01.png"> |
|||
</div> |
|||
|
|||
## Forbid Extra Query Parameters |
|||
|
|||
In some special use cases (probably not very common), you might want to **restrict** the query parameters that you want to receive. |
|||
|
|||
You can use Pydantic's model configuration to `forbid` any `extra` fields: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/query_param_models/tutorial002_an_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9" |
|||
{!> ../../../docs_src/query_param_models/tutorial002_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="11" |
|||
{!> ../../../docs_src/query_param_models/tutorial002_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.10+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/query_param_models/tutorial002_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="9" |
|||
{!> ../../../docs_src/query_param_models/tutorial002_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="11" |
|||
{!> ../../../docs_src/query_param_models/tutorial002.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
If a client tries to send some **extra** data in the **query parameters**, they will receive an **error** response. |
|||
|
|||
For example, if the client tries to send a `tool` query parameter with a value of `plumbus`, like: |
|||
|
|||
```http |
|||
https://example.com/items/?limit=10&tool=plumbus |
|||
``` |
|||
|
|||
They will receive an **error** response telling them that the query parameter `tool` is not allowed: |
|||
|
|||
```json |
|||
{ |
|||
"detail": [ |
|||
{ |
|||
"type": "extra_forbidden", |
|||
"loc": ["query", "tool"], |
|||
"msg": "Extra inputs are not permitted", |
|||
"input": "plumbus" |
|||
} |
|||
] |
|||
} |
|||
``` |
|||
|
|||
## Summary |
|||
|
|||
You can use **Pydantic models** to declare **query parameters** in **FastAPI**. 😎 |
|||
|
|||
/// tip |
|||
|
|||
Spoiler alert: you can also use Pydantic models to declare cookies and headers, but you will read about that later in the tutorial. 🤫 |
|||
|
|||
/// |
@ -0,0 +1,134 @@ |
|||
# Form Models |
|||
|
|||
You can use **Pydantic models** to declare **form fields** in FastAPI. |
|||
|
|||
/// info |
|||
|
|||
To use forms, first install <a href="https://github.com/Kludex/python-multipart" class="external-link" target="_blank">`python-multipart`</a>. |
|||
|
|||
Make sure you create a [virtual environment](../virtual-environments.md){.internal-link target=_blank}, activate it, and then install it, for example: |
|||
|
|||
```console |
|||
$ pip install python-multipart |
|||
``` |
|||
|
|||
/// |
|||
|
|||
/// note |
|||
|
|||
This is supported since FastAPI version `0.113.0`. 🤓 |
|||
|
|||
/// |
|||
|
|||
## Pydantic Models for Forms |
|||
|
|||
You just need to declare a **Pydantic model** with the fields you want to receive as **form fields**, and then declare the parameter as `Form`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9-11 15" |
|||
{!> ../../../docs_src/request_form_models/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="8-10 14" |
|||
{!> ../../../docs_src/request_form_models/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7-9 13" |
|||
{!> ../../../docs_src/request_form_models/tutorial001.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
**FastAPI** will **extract** the data for **each field** from the **form data** in the request and give you the Pydantic model you defined. |
|||
|
|||
## Check the Docs |
|||
|
|||
You can verify it in the docs UI at `/docs`: |
|||
|
|||
<div class="screenshot"> |
|||
<img src="/img/tutorial/request-form-models/image01.png"> |
|||
</div> |
|||
|
|||
## Forbid Extra Form Fields |
|||
|
|||
In some special use cases (probably not very common), you might want to **restrict** the form fields to only those declared in the Pydantic model. And **forbid** any **extra** fields. |
|||
|
|||
/// note |
|||
|
|||
This is supported since FastAPI version `0.114.0`. 🤓 |
|||
|
|||
/// |
|||
|
|||
You can use Pydantic's model configuration to `forbid` any `extra` fields: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="12" |
|||
{!> ../../../docs_src/request_form_models/tutorial002_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="11" |
|||
{!> ../../../docs_src/request_form_models/tutorial002_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefer to use the `Annotated` version if possible. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/request_form_models/tutorial002.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
If a client tries to send some extra data, they will receive an **error** response. |
|||
|
|||
For example, if the client tries to send the form fields: |
|||
|
|||
* `username`: `Rick` |
|||
* `password`: `Portal Gun` |
|||
* `extra`: `Mr. Poopybutthole` |
|||
|
|||
They will receive an error response telling them that the field `extra` is not allowed: |
|||
|
|||
```json |
|||
{ |
|||
"detail": [ |
|||
{ |
|||
"type": "extra_forbidden", |
|||
"loc": ["body", "extra"], |
|||
"msg": "Extra inputs are not permitted", |
|||
"input": "Mr. Poopybutthole" |
|||
} |
|||
] |
|||
} |
|||
``` |
|||
|
|||
## Summary |
|||
|
|||
You can use Pydantic models to declare form fields in FastAPI. 😎 |
@ -0,0 +1,844 @@ |
|||
# Virtual Environments |
|||
|
|||
When you work in Python projects you probably should use a **virtual environment** (or a similar mechanism) to isolate the packages you install for each project. |
|||
|
|||
/// info |
|||
|
|||
If you already know about virtual environments, how to create them and use them, you might want to skip this section. 🤓 |
|||
|
|||
/// |
|||
|
|||
/// tip |
|||
|
|||
A **virtual environment** is different than an **environment variable**. |
|||
|
|||
An **environment variable** is a variable in the system that can be used by programs. |
|||
|
|||
A **virtual environment** is a directory with some files in it. |
|||
|
|||
/// |
|||
|
|||
/// info |
|||
|
|||
This page will teach you how to use **virtual environments** and how they work. |
|||
|
|||
If you are ready to adopt a **tool that manages everything** for you (including installing Python), try <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">uv</a>. |
|||
|
|||
/// |
|||
|
|||
## Create a Project |
|||
|
|||
First, create a directory for your project. |
|||
|
|||
What I normally do is that I create a directory named `code` inside my home/user directory. |
|||
|
|||
And inside of that I create one directory per project. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Go to the home directory |
|||
$ cd |
|||
// Create a directory for all your code projects |
|||
$ mkdir code |
|||
// Enter into that code directory |
|||
$ cd code |
|||
// Create a directory for this project |
|||
$ mkdir awesome-project |
|||
// Enter into that project directory |
|||
$ cd awesome-project |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Create a Virtual Environment |
|||
|
|||
When you start working on a Python project **for the first time**, create a virtual environment **<abbr title="there are other options, this is a simple guideline">inside your project</abbr>**. |
|||
|
|||
/// tip |
|||
|
|||
You only need to do this **once per project**, not every time you work. |
|||
|
|||
/// |
|||
|
|||
//// tab | `venv` |
|||
|
|||
To create a virtual environment, you can use the `venv` module that comes with Python. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python -m venv .venv |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// details | What that command means |
|||
|
|||
* `python`: use the program called `python` |
|||
* `-m`: call a module as a script, we'll tell it which module next |
|||
* `venv`: use the module called `venv` that normally comes installed with Python |
|||
* `.venv`: create the virtual environment in the new directory `.venv` |
|||
|
|||
/// |
|||
|
|||
//// |
|||
|
|||
//// tab | `uv` |
|||
|
|||
If you have <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a> installed, you can use it to create a virtual environment. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uv venv |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// tip |
|||
|
|||
By default, `uv` will create a virtual environment in a directory called `.venv`. |
|||
|
|||
But you could customize it passing an additional argument with the directory name. |
|||
|
|||
/// |
|||
|
|||
//// |
|||
|
|||
That command creates a new virtual environment in a directory called `.venv`. |
|||
|
|||
/// details | `.venv` or other name |
|||
|
|||
You could create the virtual environment in a different directory, but there's a convention of calling it `.venv`. |
|||
|
|||
/// |
|||
|
|||
## Activate the Virtual Environment |
|||
|
|||
Activate the new virtual environment so that any Python command you run or package you install uses it. |
|||
|
|||
/// tip |
|||
|
|||
Do this **every time** you start a **new terminal session** to work on the project. |
|||
|
|||
/// |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/bin/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ .venv\Scripts\Activate.ps1 |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows Bash |
|||
|
|||
Or if you use Bash for Windows (e.g. <a href="https://gitforwindows.org/" class="external-link" target="_blank">Git Bash</a>): |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/Scripts/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
/// tip |
|||
|
|||
Every time you install a **new package** in that environment, **activate** the environment again. |
|||
|
|||
This makes sure that if you use a **terminal (<abbr title="command line interface">CLI</abbr>) program** installed by that package, you use the one from your virtual environment and not any other that could be installed globally, probably with a different version than what you need. |
|||
|
|||
/// |
|||
|
|||
## Check the Virtual Environment is Active |
|||
|
|||
Check that the virtual environment is active (the previous command worked). |
|||
|
|||
/// tip |
|||
|
|||
This is **optional**, but it's a good way to **check** that everything is working as expected and you are using the virtual environment you intended. |
|||
|
|||
/// |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ which python |
|||
|
|||
/home/user/code/awesome-project/.venv/bin/python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
If it shows the `python` binary at `.venv/bin/python`, inside of your project (in this case `awesome-project`), then it worked. 🎉 |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ Get-Command python |
|||
|
|||
C:\Users\user\code\awesome-project\.venv\Scripts\python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
If it shows the `python` binary at `.venv\Scripts\python`, inside of your project (in this case `awesome-project`), then it worked. 🎉 |
|||
|
|||
//// |
|||
|
|||
## Upgrade `pip` |
|||
|
|||
/// tip |
|||
|
|||
If you use <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a> you would use it to install things instead of `pip`, so you don't need to upgrade `pip`. 😎 |
|||
|
|||
/// |
|||
|
|||
If you are using `pip` to install packages (it comes by default with Python), you should **upgrade** it to the latest version. |
|||
|
|||
Many exotic errors while installing a package are solved by just upgrading `pip` first. |
|||
|
|||
/// tip |
|||
|
|||
You would normally do this **once**, right after you create the virtual environment. |
|||
|
|||
/// |
|||
|
|||
Make sure the virtual environment is active (with the command above) and then run: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python -m pip install --upgrade pip |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Add `.gitignore` |
|||
|
|||
If you are using **Git** (you should), add a `.gitignore` file to exclude everything in your `.venv` from Git. |
|||
|
|||
/// tip |
|||
|
|||
If you used <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a> to create the virtual environment, it already did this for you, you can skip this step. 😎 |
|||
|
|||
/// |
|||
|
|||
/// tip |
|||
|
|||
Do this **once**, right after you create the virtual environment. |
|||
|
|||
/// |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ echo "*" > .venv/.gitignore |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// details | What that command means |
|||
|
|||
* `echo "*"`: will "print" the text `*` in the terminal (the next part changes that a bit) |
|||
* `>`: anything printed to the terminal by the command to the left of `>` should not be printed but instead written to the file that goes to the right of `>` |
|||
* `.gitignore`: the name of the file where the text should be written |
|||
|
|||
And `*` for Git means "everything". So, it will ignore everything in the `.venv` directory. |
|||
|
|||
That command will create a file `.gitignore` with the content: |
|||
|
|||
```gitignore |
|||
* |
|||
``` |
|||
|
|||
/// |
|||
|
|||
## Install Packages |
|||
|
|||
After activating the environment, you can install packages in it. |
|||
|
|||
/// tip |
|||
|
|||
Do this **once** when installing or upgrading the packages your project needs. |
|||
|
|||
If you need to upgrade a version or add a new package you would **do this again**. |
|||
|
|||
/// |
|||
|
|||
### Install Packages Directly |
|||
|
|||
If you're in a hurry and don't want to use a file to declare your project's package requirements, you can install them directly. |
|||
|
|||
/// tip |
|||
|
|||
It's a (very) good idea to put the packages and versions your program needs in a file (for example `requirements.txt` or `pyproject.toml`). |
|||
|
|||
/// |
|||
|
|||
//// tab | `pip` |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "fastapi[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | `uv` |
|||
|
|||
If you have <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a>: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uv pip install "fastapi[standard]" |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
### Install from `requirements.txt` |
|||
|
|||
If you have a `requirements.txt`, you can now use it to install its packages. |
|||
|
|||
//// tab | `pip` |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install -r requirements.txt |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | `uv` |
|||
|
|||
If you have <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a>: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uv pip install -r requirements.txt |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
/// details | `requirements.txt` |
|||
|
|||
A `requirements.txt` with some packages could look like: |
|||
|
|||
```requirements.txt |
|||
fastapi[standard]==0.113.0 |
|||
pydantic==2.8.0 |
|||
``` |
|||
|
|||
/// |
|||
|
|||
## Run Your Program |
|||
|
|||
After you activated the virtual environment, you can run your program, and it will use the Python inside of your virtual environment with the packages you installed there. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python main.py |
|||
|
|||
Hello World |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Configure Your Editor |
|||
|
|||
You would probably use an editor, make sure you configure it to use the same virtual environment you created (it will probably autodetect it) so that you can get autocompletion and inline errors. |
|||
|
|||
For example: |
|||
|
|||
* <a href="https://code.visualstudio.com/docs/python/environments#_select-and-activate-an-environment" class="external-link" target="_blank">VS Code</a> |
|||
* <a href="https://www.jetbrains.com/help/pycharm/creating-virtual-environment.html" class="external-link" target="_blank">PyCharm</a> |
|||
|
|||
/// tip |
|||
|
|||
You normally have to do this only **once**, when you create the virtual environment. |
|||
|
|||
/// |
|||
|
|||
## Deactivate the Virtual Environment |
|||
|
|||
Once you are done working on your project you can **deactivate** the virtual environment. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ deactivate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
This way, when you run `python` it won't try to run it from that virtual environment with the packages installed there. |
|||
|
|||
## Ready to Work |
|||
|
|||
Now you're ready to start working on your project. |
|||
|
|||
|
|||
|
|||
/// tip |
|||
|
|||
Do you want to understand what's all that above? |
|||
|
|||
Continue reading. 👇🤓 |
|||
|
|||
/// |
|||
|
|||
## Why Virtual Environments |
|||
|
|||
To work with FastAPI you need to install <a href="https://www.python.org/" class="external-link" target="_blank">Python</a>. |
|||
|
|||
After that, you would need to **install** FastAPI and any other **packages** you want to use. |
|||
|
|||
To install packages you would normally use the `pip` command that comes with Python (or similar alternatives). |
|||
|
|||
Nevertheless, if you just use `pip` directly, the packages would be installed in your **global Python environment** (the global installation of Python). |
|||
|
|||
### The Problem |
|||
|
|||
So, what's the problem with installing packages in the global Python environment? |
|||
|
|||
At some point, you will probably end up writing many different programs that depend on **different packages**. And some of these projects you work on will depend on **different versions** of the same package. 😱 |
|||
|
|||
For example, you could create a project called `philosophers-stone`, this program depends on another package called **`harry`, using the version `1`**. So, you need to install `harry`. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
stone(philosophers-stone) -->|requires| harry-1[harry v1] |
|||
``` |
|||
|
|||
Then, at some point later, you create another project called `prisoner-of-azkaban`, and this project also depends on `harry`, but this project needs **`harry` version `3`**. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
azkaban(prisoner-of-azkaban) --> |requires| harry-3[harry v3] |
|||
``` |
|||
|
|||
But now the problem is, if you install the packages globally (in the global environment) instead of in a local **virtual environment**, you will have to choose which version of `harry` to install. |
|||
|
|||
If you want to run `philosophers-stone` you will need to first install `harry` version `1`, for example with: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "harry==1" |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
And then you would end up with `harry` version `1` installed in your global Python environment. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
subgraph global[global env] |
|||
harry-1[harry v1] |
|||
end |
|||
subgraph stone-project[philosophers-stone project] |
|||
stone(philosophers-stone) -->|requires| harry-1 |
|||
end |
|||
``` |
|||
|
|||
But then if you want to run `prisoner-of-azkaban`, you will need to uninstall `harry` version `1` and install `harry` version `3` (or just installing version `3` would automatically uninstall version `1`). |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "harry==3" |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
And then you would end up with `harry` version `3` installed in your global Python environment. |
|||
|
|||
And if you try to run `philosophers-stone` again, there's a chance it would **not work** because it needs `harry` version `1`. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
subgraph global[global env] |
|||
harry-1[<strike>harry v1</strike>] |
|||
style harry-1 fill:#ccc,stroke-dasharray: 5 5 |
|||
harry-3[harry v3] |
|||
end |
|||
subgraph stone-project[philosophers-stone project] |
|||
stone(philosophers-stone) -.-x|⛔️| harry-1 |
|||
end |
|||
subgraph azkaban-project[prisoner-of-azkaban project] |
|||
azkaban(prisoner-of-azkaban) --> |requires| harry-3 |
|||
end |
|||
``` |
|||
|
|||
/// tip |
|||
|
|||
It's very common in Python packages to try the best to **avoid breaking changes** in **new versions**, but it's better to be safe, and install newer versions intentionally and when you can run the tests to check everything is working correctly. |
|||
|
|||
/// |
|||
|
|||
Now, imagine that with **many** other **packages** that all your **projects depend on**. That's very difficult to manage. And you would probably end up running some projects with some **incompatible versions** of the packages, and not knowing why something isn't working. |
|||
|
|||
Also, depending on your operating system (e.g. Linux, Windows, macOS), it could have come with Python already installed. And in that case it probably had some packages pre-installed with some specific versions **needed by your system**. If you install packages in the global Python environment, you could end up **breaking** some of the programs that came with your operating system. |
|||
|
|||
## Where are Packages Installed |
|||
|
|||
When you install Python, it creates some directories with some files in your computer. |
|||
|
|||
Some of these directories are the ones in charge of having all the packages you install. |
|||
|
|||
When you run: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Don't run this now, it's just an example 🤓 |
|||
$ pip install "fastapi[standard]" |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
That will download a compressed file with the FastAPI code, normally from <a href="https://pypi.org/project/fastapi/" class="external-link" target="_blank">PyPI</a>. |
|||
|
|||
It will also **download** files for other packages that FastAPI depends on. |
|||
|
|||
Then it will **extract** all those files and put them in a directory in your computer. |
|||
|
|||
By default, it will put those files downloaded and extracted in the directory that comes with your Python installation, that's the **global environment**. |
|||
|
|||
## What are Virtual Environments |
|||
|
|||
The solution to the problems of having all the packages in the global environment is to use a **virtual environment for each project** you work on. |
|||
|
|||
A virtual environment is a **directory**, very similar to the global one, where you can install the packages for a project. |
|||
|
|||
This way, each project will have its own virtual environment (`.venv` directory) with its own packages. |
|||
|
|||
```mermaid |
|||
flowchart TB |
|||
subgraph stone-project[philosophers-stone project] |
|||
stone(philosophers-stone) --->|requires| harry-1 |
|||
subgraph venv1[.venv] |
|||
harry-1[harry v1] |
|||
end |
|||
end |
|||
subgraph azkaban-project[prisoner-of-azkaban project] |
|||
azkaban(prisoner-of-azkaban) --->|requires| harry-3 |
|||
subgraph venv2[.venv] |
|||
harry-3[harry v3] |
|||
end |
|||
end |
|||
stone-project ~~~ azkaban-project |
|||
``` |
|||
|
|||
## What Does Activating a Virtual Environment Mean |
|||
|
|||
When you activate a virtual environment, for example with: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/bin/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ .venv\Scripts\Activate.ps1 |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows Bash |
|||
|
|||
Or if you use Bash for Windows (e.g. <a href="https://gitforwindows.org/" class="external-link" target="_blank">Git Bash</a>): |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/Scripts/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
That command will create or modify some [environment variables](environment-variables.md){.internal-link target=_blank} that will be available for the next commands. |
|||
|
|||
One of those variables is the `PATH` variable. |
|||
|
|||
/// tip |
|||
|
|||
You can learn more about the `PATH` environment variable in the [Environment Variables](environment-variables.md#path-environment-variable){.internal-link target=_blank} section. |
|||
|
|||
/// |
|||
|
|||
Activating a virtual environment adds its path `.venv/bin` (on Linux and macOS) or `.venv\Scripts` (on Windows) to the `PATH` environment variable. |
|||
|
|||
Let's say that before activating the environment, the `PATH` variable looked like this: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
```plaintext |
|||
/usr/bin:/bin:/usr/sbin:/sbin |
|||
``` |
|||
|
|||
That means that the system would look for programs in: |
|||
|
|||
* `/usr/bin` |
|||
* `/bin` |
|||
* `/usr/sbin` |
|||
* `/sbin` |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
```plaintext |
|||
C:\Windows\System32 |
|||
``` |
|||
|
|||
That means that the system would look for programs in: |
|||
|
|||
* `C:\Windows\System32` |
|||
|
|||
//// |
|||
|
|||
After activating the virtual environment, the `PATH` variable would look something like this: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
```plaintext |
|||
/home/user/code/awesome-project/.venv/bin:/usr/bin:/bin:/usr/sbin:/sbin |
|||
``` |
|||
|
|||
That means that the system will now start looking first look for programs in: |
|||
|
|||
```plaintext |
|||
/home/user/code/awesome-project/.venv/bin |
|||
``` |
|||
|
|||
before looking in the other directories. |
|||
|
|||
So, when you type `python` in the terminal, the system will find the Python program in |
|||
|
|||
```plaintext |
|||
/home/user/code/awesome-project/.venv/bin/python |
|||
``` |
|||
|
|||
and use that one. |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
```plaintext |
|||
C:\Users\user\code\awesome-project\.venv\Scripts;C:\Windows\System32 |
|||
``` |
|||
|
|||
That means that the system will now start looking first look for programs in: |
|||
|
|||
```plaintext |
|||
C:\Users\user\code\awesome-project\.venv\Scripts |
|||
``` |
|||
|
|||
before looking in the other directories. |
|||
|
|||
So, when you type `python` in the terminal, the system will find the Python program in |
|||
|
|||
```plaintext |
|||
C:\Users\user\code\awesome-project\.venv\Scripts\python |
|||
``` |
|||
|
|||
and use that one. |
|||
|
|||
//// |
|||
|
|||
An important detail is that it will put the virtual environment path at the **beginning** of the `PATH` variable. The system will find it **before** finding any other Python available. This way, when you run `python`, it will use the Python **from the virtual environment** instead of any other `python` (for example, a `python` from a global environment). |
|||
|
|||
Activating a virtual environment also changes a couple of other things, but this is one of the most important things it does. |
|||
|
|||
## Checking a Virtual Environment |
|||
|
|||
When you check if a virtual environment is active, for example with: |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ which python |
|||
|
|||
/home/user/code/awesome-project/.venv/bin/python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ Get-Command python |
|||
|
|||
C:\Users\user\code\awesome-project\.venv\Scripts\python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
That means that the `python` program that will be used is the one **in the virtual environment**. |
|||
|
|||
you use `which` in Linux and macOS and `Get-Command` in Windows PowerShell. |
|||
|
|||
The way that command works is that it will go and check in the `PATH` environment variable, going through **each path in order**, looking for the program called `python`. Once it finds it, it will **show you the path** to that program. |
|||
|
|||
The most important part is that when you call `python`, that is the exact "`python`" that will be executed. |
|||
|
|||
So, you can confirm if you are in the correct virtual environment. |
|||
|
|||
/// tip |
|||
|
|||
It's easy to activate one virtual environment, get one Python, and then **go to another project**. |
|||
|
|||
And the second project **wouldn't work** because you are using the **incorrect Python**, from a virtual environment for another project. |
|||
|
|||
It's useful being able to check what `python` is being used. 🤓 |
|||
|
|||
/// |
|||
|
|||
## Why Deactivate a Virtual Environment |
|||
|
|||
For example, you could be working on a project `philosophers-stone`, **activate that virtual environment**, install packages and work with that environment. |
|||
|
|||
And then you want to work on **another project** `prisoner-of-azkaban`. |
|||
|
|||
You go to that project: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ cd ~/code/prisoner-of-azkaban |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
If you don't deactivate the virtual environment for `philosophers-stone`, when you run `python` in the terminal, it will try to use the Python from `philosophers-stone`. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ cd ~/code/prisoner-of-azkaban |
|||
|
|||
$ python main.py |
|||
|
|||
// Error importing sirius, it's not installed 😱 |
|||
Traceback (most recent call last): |
|||
File "main.py", line 1, in <module> |
|||
import sirius |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
But if you deactivate the virtual environment and activate the new one for `prisoner-of-askaban` then when you run `python` it will use the Python from the virtual environment in `prisoner-of-azkaban`. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ cd ~/code/prisoner-of-azkaban |
|||
|
|||
// You don't need to be in the old directory to deactivate, you can do it wherever you are, even after going to the other project 😎 |
|||
$ deactivate |
|||
|
|||
// Activate the virtual environment in prisoner-of-azkaban/.venv 🚀 |
|||
$ source .venv/bin/activate |
|||
|
|||
// Now when you run python, it will find the package sirius installed in this virtual environment ✨ |
|||
$ python main.py |
|||
|
|||
I solemnly swear 🐺 |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Alternatives |
|||
|
|||
This is a simple guide to get you started and teach you how everything works **underneath**. |
|||
|
|||
There are many **alternatives** to managing virtual environments, package dependencies (requirements), projects. |
|||
|
|||
Once you are ready and want to use a tool to **manage the entire project**, packages dependencies, virtual environments, etc. I would suggest you try <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">uv</a>. |
|||
|
|||
`uv` can do a lot of things, it can: |
|||
|
|||
* **Install Python** for you, including different versions |
|||
* Manage the **virtual environment** for your projects |
|||
* Install **packages** |
|||
* Manage package **dependencies and versions** for your project |
|||
* Make sure you have an **exact** set of packages and versions to install, including their dependencies, so that you can be sure that you can run your project in production exactly the same as in your computer while developing, this is called **locking** |
|||
* And many other things |
|||
|
|||
## Conclusion |
|||
|
|||
If you read and understood all this, now **you know much more** about virtual environments than many developers out there. 🤓 |
|||
|
|||
Knowing these details will most probably be useful in a future time when you are debugging something that seems complex, but you will know **how it all works underneath**. 😎 |
@ -0,0 +1,5 @@ |
|||
# 学習 |
|||
|
|||
ここでは、**FastAPI** を学習するための入門セクションとチュートリアルを紹介します。 |
|||
|
|||
これは、FastAPIを学習するにあたっての**書籍**や**コース**であり、**公式**かつ推奨される方法とみなすことができます 😎 |
@ -0,0 +1,28 @@ |
|||
# Full Stack FastAPI 템플릿 |
|||
|
|||
템플릿은 일반적으로 특정 설정과 함께 제공되지만, 유연하고 커스터마이징이 가능하게 디자인 되었습니다. 이 특성들은 여러분이 프로젝트의 요구사항에 맞춰 수정, 적용을 할 수 있게 해주고, 템플릿이 완벽한 시작점이 되게 해줍니다. 🏁 |
|||
|
|||
많은 초기 설정, 보안, 데이터베이스 및 일부 API 엔드포인트가 이미 준비되어 있으므로, 여러분은 이 템플릿을 (프로젝트를) 시작하는 데 사용할 수 있습니다. |
|||
|
|||
GitHub 저장소: <a href="https://github.com/tiangolo/full-stack-fastapi-template" class="external-link" target="_blank">Full Stack FastAPI 템플릿</a> |
|||
|
|||
## Full Stack FastAPI 템플릿 - 기술 스택과 기능들 |
|||
|
|||
- ⚡ [**FastAPI**](https://fastapi.tiangolo.com): Python 백엔드 API. |
|||
- 🧰 [SQLModel](https://sqlmodel.tiangolo.com): Python SQL 데이터 상호작용을 위한 (ORM). |
|||
- 🔍 [Pydantic](https://docs.pydantic.dev): FastAPI에 의해 사용되는, 데이터 검증과 설정관리. |
|||
- 💾 [PostgreSQL](https://www.postgresql.org): SQL 데이터베이스. |
|||
- 🚀 [React](https://react.dev): 프론트엔드. |
|||
- 💃 TypeScript, hooks, Vite 및 기타 현대적인 프론트엔드 스택을 사용. |
|||
- 🎨 [Chakra UI](https://chakra-ui.com): 프론트엔드 컴포넌트. |
|||
- 🤖 자동으로 생성된 프론트엔드 클라이언트. |
|||
- 🧪 E2E 테스트를 위한 Playwright. |
|||
- 🦇 다크 모드 지원. |
|||
- 🐋 [Docker Compose](https://www.docker.com): 개발 환경과 프로덕션(운영). |
|||
- 🔒 기본으로 지원되는 안전한 비밀번호 해싱. |
|||
- 🔑 JWT 토큰 인증. |
|||
- 📫 이메일 기반 비밀번호 복구. |
|||
- ✅ [Pytest]를 이용한 테스트(https://pytest.org). |
|||
- 📞 [Traefik](https://traefik.io): 리버스 프록시 / 로드 밸런서. |
|||
- 🚢 Docker Compose를 이용한 배포 지침: 자동 HTTPS 인증서를 처리하기 위한 프론트엔드 Traefik 프록시 설정 방법을 포함. |
|||
- 🏭 GitHub Actions를 기반으로 CI (지속적인 통합) 및 CD (지속적인 배포). |
@ -0,0 +1,201 @@ |
|||
# Functionaliteit |
|||
|
|||
## FastAPI functionaliteit |
|||
|
|||
**FastAPI** biedt je het volgende: |
|||
|
|||
### Gebaseerd op open standaarden |
|||
|
|||
* <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank"><strong>OpenAPI</strong></a> voor het maken van API's, inclusief declaraties van <abbr title="ook bekend als: endpoints, routess">pad</abbr><abbr title="ook bekend als HTTP-methoden, zoals POST, GET, PUT, DELETE">bewerkingen</abbr>, parameters, request bodies, beveiliging, enz. |
|||
* Automatische datamodel documentatie met <a href="https://json-schema.org/" class="external-link" target="_blank"><strong>JSON Schema</strong></a> (aangezien OpenAPI zelf is gebaseerd op JSON Schema). |
|||
* Ontworpen op basis van deze standaarden, na zorgvuldig onderzoek. In plaats van achteraf deze laag er bovenop te bouwen. |
|||
* Dit maakt het ook mogelijk om automatisch **clientcode te genereren** in verschillende programmeertalen. |
|||
|
|||
### Automatische documentatie |
|||
|
|||
Interactieve API-documentatie en verkenning van webgebruikersinterfaces. Aangezien dit framework is gebaseerd op OpenAPI, zijn er meerdere documentatie opties mogelijk, waarvan er standaard 2 zijn inbegrepen. |
|||
|
|||
* <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank"><strong>Swagger UI</strong></a>, met interactieve interface, maakt het mogelijk je API rechtstreeks vanuit de browser aan te roepen en te testen. |
|||
|
|||
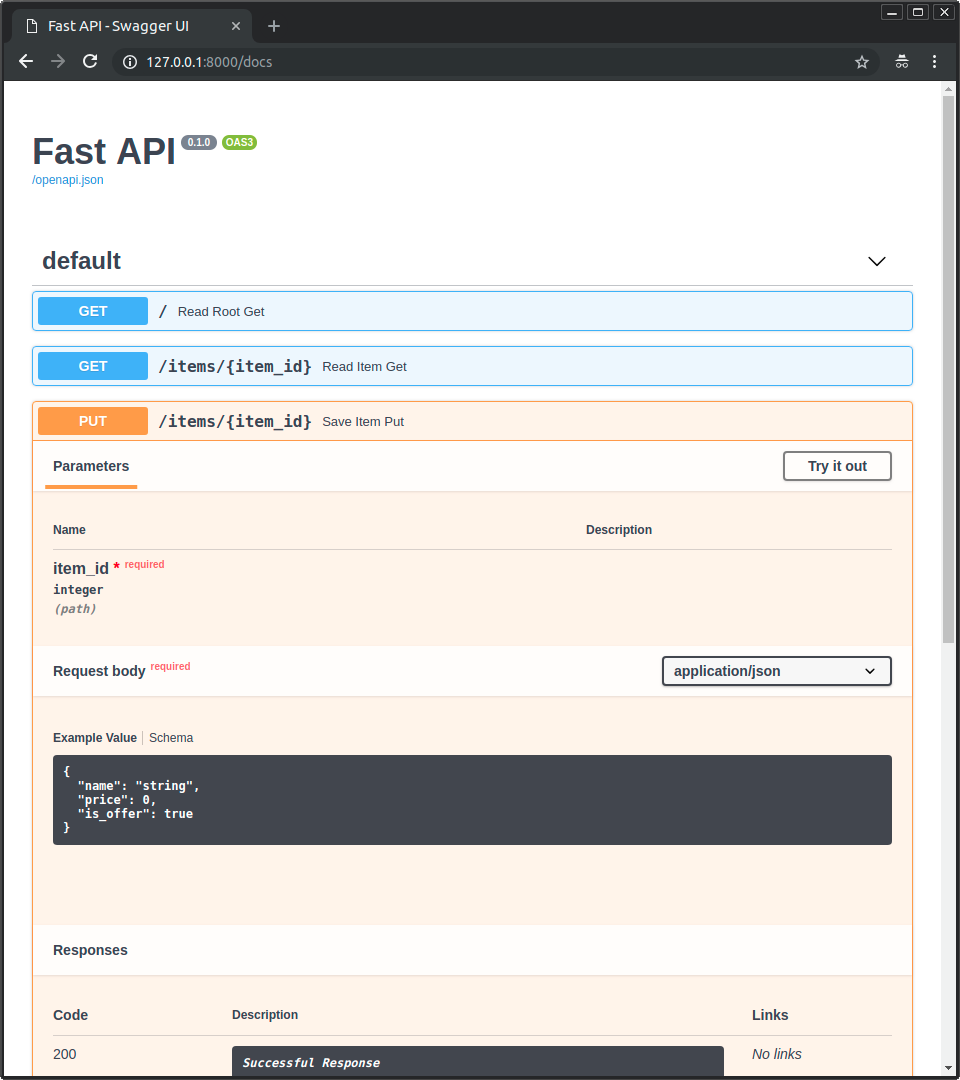 |
|||
|
|||
* Alternatieve API-documentatie met <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank"><strong>ReDoc</strong></a>. |
|||
|
|||
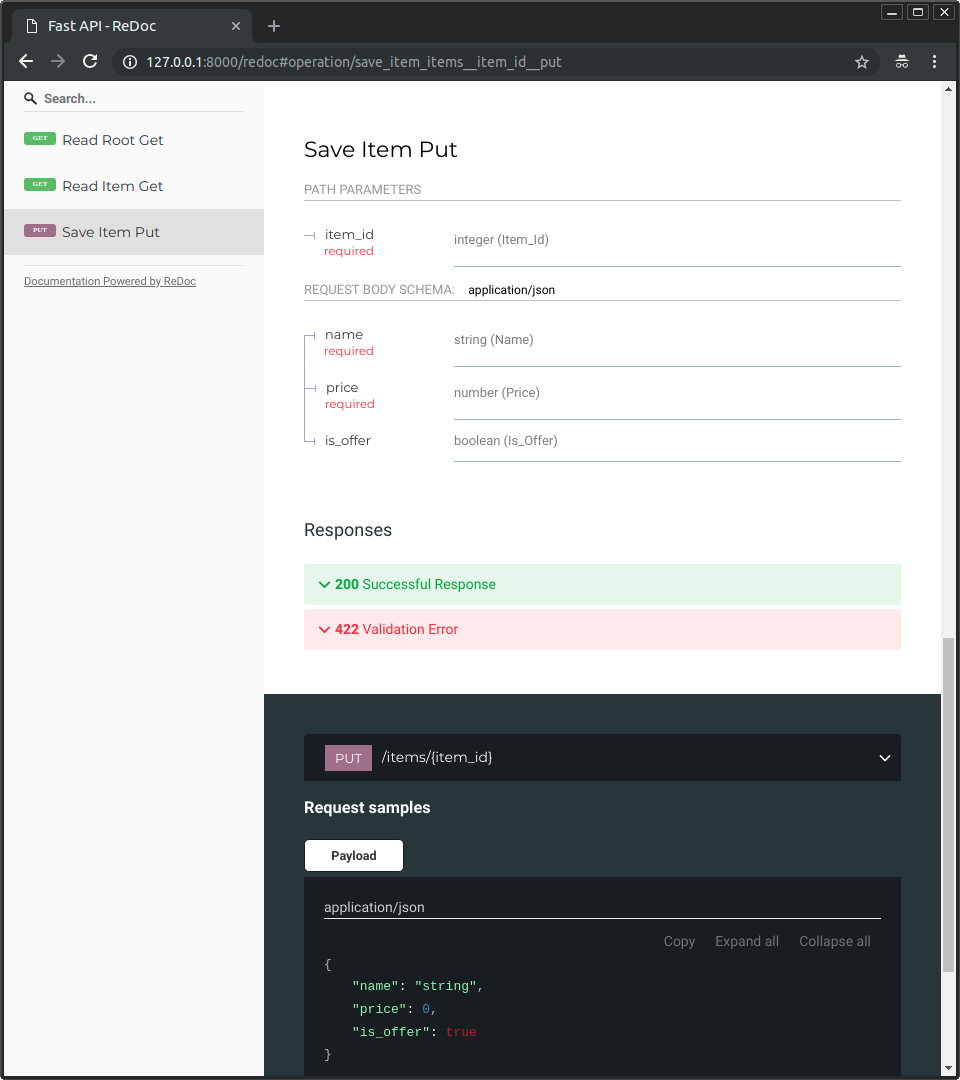 |
|||
|
|||
### Gewoon Moderne Python |
|||
|
|||
Het is allemaal gebaseerd op standaard **Python type** declaraties (dankzij Pydantic). Je hoeft dus geen nieuwe syntax te leren. Het is gewoon standaard moderne Python. |
|||
|
|||
Als je een opfriscursus van 2 minuten nodig hebt over het gebruik van Python types (zelfs als je FastAPI niet gebruikt), bekijk dan deze korte tutorial: [Python Types](python-types.md){.internal-link target=_blank}. |
|||
|
|||
Je schrijft gewoon standaard Python met types: |
|||
|
|||
```Python |
|||
from datetime import date |
|||
|
|||
from pydantic import BaseModel |
|||
|
|||
# Declareer een variabele als een str |
|||
# en krijg editorondersteuning in de functie |
|||
def main(user_id: str): |
|||
return user_id |
|||
|
|||
|
|||
# Een Pydantic model |
|||
class User(BaseModel): |
|||
id: int |
|||
name: str |
|||
joined: date |
|||
``` |
|||
|
|||
Vervolgens kan je het op deze manier gebruiken: |
|||
|
|||
```Python |
|||
my_user: User = User(id=3, name="John Doe", joined="2018-07-19") |
|||
|
|||
second_user_data = { |
|||
"id": 4, |
|||
"name": "Mary", |
|||
"joined": "2018-11-30", |
|||
} |
|||
|
|||
my_second_user: User = User(**second_user_data) |
|||
``` |
|||
|
|||
/// info |
|||
|
|||
`**second_user_data` betekent: |
|||
|
|||
Geef de sleutels (keys) en waarden (values) van de `second_user_data` dict direct door als sleutel-waarden argumenten, gelijk aan: `User(id=4, name=“Mary”, joined=“2018-11-30”)` |
|||
|
|||
/// |
|||
|
|||
### Editor-ondersteuning |
|||
|
|||
Het gehele framework is ontworpen om eenvoudig en intuïtief te zijn in gebruik. Alle beslissingen zijn getest op meerdere code-editors nog voordat het daadwerkelijke ontwikkelen begon, om zo de beste ontwikkelervaring te garanderen. |
|||
|
|||
Uit enquêtes onder Python ontwikkelaars blijkt maar al te duidelijk dat "(automatische) code aanvulling" <a href="https://www.jetbrains.com/research/python-developers-survey-2017/#tools-and-features" class="external-link" target="_blank">een van de meest gebruikte functionaliteiten is</a>. |
|||
|
|||
Het hele **FastAPI** framework is daarop gebaseerd. Automatische code aanvulling werkt overal. |
|||
|
|||
Je hoeft zelden terug te vallen op de documentatie. |
|||
|
|||
Zo kan je editor je helpen: |
|||
|
|||
* in <a href="https://code.visualstudio.com/" class="external-link" target="_blank">Visual Studio Code</a>: |
|||
|
|||
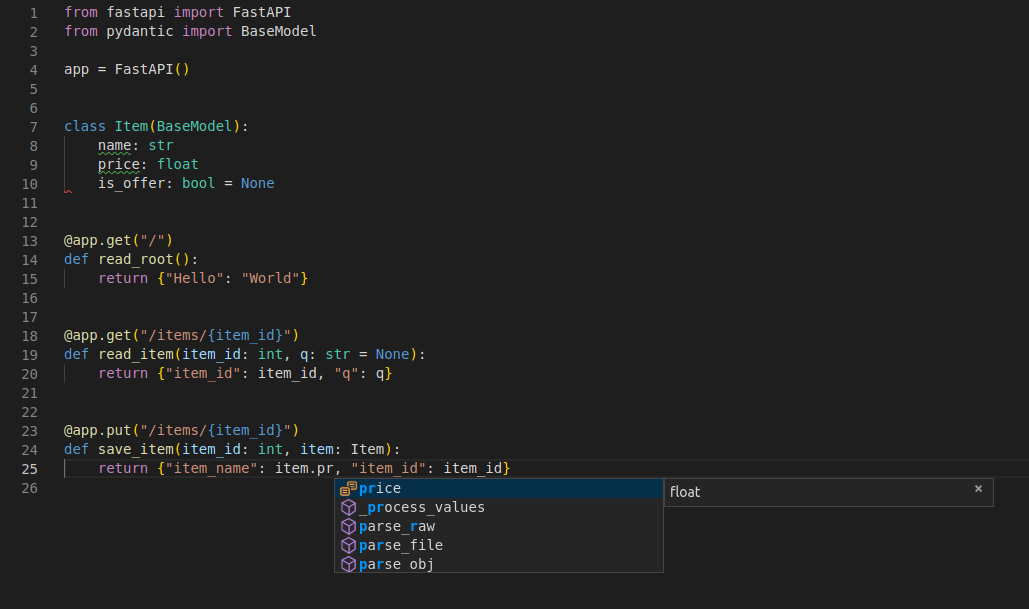 |
|||
|
|||
* in <a href="https://www.jetbrains.com/pycharm/" class="external-link" target="_blank">PyCharm</a>: |
|||
|
|||
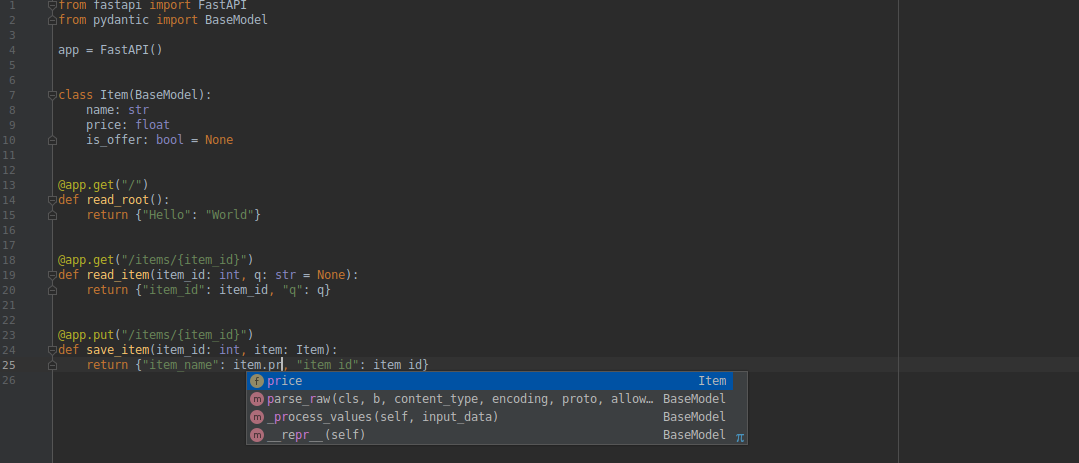 |
|||
|
|||
Je krijgt autocomletion die je voorheen misschien zelfs voor onmogelijk had gehouden. Zoals bijvoorbeeld de `price` key in een JSON body (die genest had kunnen zijn) die afkomstig is van een request. |
|||
|
|||
Je hoeft niet langer de verkeerde keys in te typen, op en neer te gaan tussen de documentatie, of heen en weer te scrollen om te checken of je `username` of toch `user_name` had gebruikt. |
|||
|
|||
### Kort |
|||
|
|||
Dit framework heeft voor alles verstandige **standaardinstellingen**, met overal optionele configuraties. Alle parameters kunnen worden verfijnd zodat het past bij wat je nodig hebt, om zo de API te kunnen definiëren die jij nodig hebt. |
|||
|
|||
Maar standaard werkt alles **“gewoon”**. |
|||
|
|||
### Validatie |
|||
|
|||
* Validatie voor de meeste (of misschien wel alle?) Python **datatypes**, inclusief: |
|||
* JSON objecten (`dict`). |
|||
* JSON array (`list`) die itemtypes definiëren. |
|||
* String (`str`) velden, die min en max lengtes hebben. |
|||
* Getallen (`int`, `float`) met min en max waarden, enz. |
|||
|
|||
* Validatie voor meer exotische typen, zoals: |
|||
* URL. |
|||
* E-mail. |
|||
* UUID. |
|||
* ...en anderen. |
|||
|
|||
Alle validatie wordt uitgevoerd door het beproefde en robuuste **Pydantic**. |
|||
|
|||
### Beveiliging en authenticatie |
|||
|
|||
Beveiliging en authenticatie is geïntegreerd. Zonder compromissen te doen naar databases of datamodellen. |
|||
|
|||
Alle beveiligingsschema's gedefinieerd in OpenAPI, inclusief: |
|||
|
|||
* HTTP Basic. |
|||
* **OAuth2** (ook met **JWT tokens**). Bekijk de tutorial over [OAuth2 with JWT](tutorial/security/oauth2-jwt.md){.internal-link target=_blank}. |
|||
* API keys in: |
|||
* Headers. |
|||
* Query parameters. |
|||
* Cookies, enz. |
|||
|
|||
Plus alle beveiligingsfuncties van Starlette (inclusief **sessiecookies**). |
|||
|
|||
Gebouwd als een herbruikbare tool met componenten die makkelijk te integreren zijn in en met je systemen, datastores, relationele en NoSQL databases, enz. |
|||
|
|||
### Dependency Injection |
|||
|
|||
FastAPI bevat een uiterst eenvoudig, maar uiterst krachtig <abbr title='ook bekend als "componenten", "bronnen", "diensten", "aanbieders"'><strong>Dependency Injection</strong></abbr> systeem. |
|||
|
|||
* Zelfs dependencies kunnen dependencies hebben, waardoor een hiërarchie of **“graph” van dependencies** ontstaat. |
|||
* Allemaal **automatisch afgehandeld** door het framework. |
|||
* Alle dependencies kunnen data nodig hebben van request, de vereiste **padoperaties veranderen** en automatische documentatie verstrekken. |
|||
* **Automatische validatie** zelfs voor *padoperatie* parameters gedefinieerd in dependencies. |
|||
* Ondersteuning voor complexe gebruikersauthenticatiesystemen, **databaseverbindingen**, enz. |
|||
* **Geen compromisen** met databases, gebruikersinterfaces, enz. Maar eenvoudige integratie met ze allemaal. |
|||
|
|||
### Ongelimiteerde "plug-ins" |
|||
|
|||
Of anders gezegd, je hebt ze niet nodig, importeer en gebruik de code die je nodig hebt. |
|||
|
|||
Elke integratie is ontworpen om eenvoudig te gebruiken (met afhankelijkheden), zodat je een “plug-in" kunt maken in 2 regels code, met dezelfde structuur en syntax die wordt gebruikt voor je *padbewerkingen*. |
|||
|
|||
### Getest |
|||
|
|||
* 100% <abbr title="De hoeveelheid code die automatisch wordt getest">van de code is getest</abbr>. |
|||
* 100% <abbr title="Python type annotaties, hiermee kunnen je editor en externe tools je beter ondersteunen">type geannoteerde</abbr> codebase. |
|||
* Wordt gebruikt in productietoepassingen. |
|||
|
|||
## Starlette functies |
|||
|
|||
**FastAPI** is volledig verenigbaar met (en gebaseerd op) <a href="https://www.starlette.io/" class="external-link" target="_blank"><strong>Starlette</strong></a>. |
|||
|
|||
`FastAPI` is eigenlijk een subklasse van `Starlette`. Dus als je Starlette al kent of gebruikt, zal de meeste functionaliteit op dezelfde manier werken. |
|||
|
|||
Met **FastAPI** krijg je alle functies van **Starlette** (FastAPI is gewoon Starlette op steroïden): |
|||
|
|||
* Zeer indrukwekkende prestaties. Het is <a href="https://github.com/encode/starlette#performance" class="external-link" target="_blank">een van de snelste Python frameworks, vergelijkbaar met **NodeJS** en **Go**</a>. |
|||
* **WebSocket** ondersteuning. |
|||
* Taken in de achtergrond tijdens het proces. |
|||
* Opstart- en afsluit events. |
|||
* Test client gebouwd op HTTPX. |
|||
* **CORS**, GZip, Statische bestanden, Streaming reacties. |
|||
* **Sessie en Cookie** ondersteuning. |
|||
* 100% van de code is getest. |
|||
* 100% type geannoteerde codebase. |
|||
|
|||
## Pydantic functionaliteit |
|||
|
|||
**FastAPI** is volledig verenigbaar met (en gebaseerd op) Pydantic. Dus alle extra <a href="https://docs.pydantic.dev/" class="external-link" target="_blank"><strong>Pydantic</strong></a> code die je nog hebt werkt ook. |
|||
|
|||
Inclusief externe pakketten die ook gebaseerd zijn op Pydantic, zoals <abbr title="Object-Relational Mapper">ORM</abbr>s, <abbr title="Object-Document Mapper">ODM</abbr>s voor databases. |
|||
|
|||
Dit betekent ook dat je in veel gevallen het object dat je van een request krijgt **direct naar je database** kunt sturen, omdat alles automatisch wordt gevalideerd. |
|||
|
|||
Hetzelfde geldt ook andersom, in veel gevallen kun je dus het object dat je krijgt van de database **direct doorgeven aan de client**. |
|||
|
|||
Met **FastAPI** krijg je alle functionaliteit van **Pydantic** (omdat FastAPI is gebaseerd op Pydantic voor alle dataverwerking): |
|||
|
|||
* **Geen brainfucks**: |
|||
* Je hoeft geen nieuwe microtaal voor schemadefinities te leren. |
|||
* Als je bekend bent Python types, weet je hoe je Pydantic moet gebruiken. |
|||
* Werkt goed samen met je **<abbr title=“Integrated Development Environment, vergelijkbaar met een code editor”>IDE</abbr>/<abbr title=“Een programma dat controleert op fouten in de code”>linter</abbr>/hersenen**: |
|||
* Doordat pydantic's datastructuren enkel instanties zijn van klassen, die je definieert, werkt automatische aanvulling, linting, mypy en je intuïtie allemaal goed met je gevalideerde data. |
|||
* Valideer **complexe structuren**: |
|||
* Gebruik van hiërarchische Pydantic modellen, Python `typing`'s `List` en `Dict`, enz. |
|||
* Met validators kunnen complexe dataschema's duidelijk en eenvoudig worden gedefinieerd, gecontroleerd en gedocumenteerd als JSON Schema. |
|||
* Je kunt diep **geneste JSON** objecten laten valideren en annoteren. |
|||
* **Uitbreidbaar**: |
|||
* Met Pydantic kunnen op maat gemaakte datatypen worden gedefinieerd of je kunt validatie uitbreiden met methoden op een model dat is ingericht met de decorator validator. |
|||
* 100% van de code is getest. |
@ -0,0 +1,494 @@ |
|||
# FastAPI |
|||
|
|||
<style> |
|||
.md-content .md-typeset h1 { display: none; } |
|||
</style> |
|||
|
|||
<p align="center"> |
|||
<a href="https://fastapi.tiangolo.com"><img src="https://fastapi.tiangolo.com/img/logo-margin/logo-teal.png" alt="FastAPI"></a> |
|||
</p> |
|||
<p align="center"> |
|||
<em>FastAPI framework, zeer goede prestaties, eenvoudig te leren, snel te programmeren, klaar voor productie</em> |
|||
</p> |
|||
<p align="center"> |
|||
<a href="https://github.com/fastapi/fastapi/actions?query=workflow%3ATest+event%3Apush+branch%3Amaster" target="_blank"> |
|||
<img src="https://github.com/fastapi/fastapi/workflows/Test/badge.svg?event=push&branch=master" alt="Test"> |
|||
</a> |
|||
<a href="https://coverage-badge.samuelcolvin.workers.dev/redirect/fastapi/fastapi" target="_blank"> |
|||
<img src="https://coverage-badge.samuelcolvin.workers.dev/fastapi/fastapi.svg" alt="Coverage"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/v/fastapi?color=%2334D058&label=pypi%20package" alt="Package version"> |
|||
</a> |
|||
<a href="https://pypi.org/project/fastapi" target="_blank"> |
|||
<img src="https://img.shields.io/pypi/pyversions/fastapi.svg?color=%2334D058" alt="Supported Python versions"> |
|||
</a> |
|||
</p> |
|||
|
|||
--- |
|||
|
|||
**Documentatie**: <a href="https://fastapi.tiangolo.com" target="_blank">https://fastapi.tiangolo.com</a> |
|||
|
|||
**Broncode**: <a href="https://github.com/fastapi/fastapi" target="_blank">https://github.com/tiangolo/fastapi</a> |
|||
|
|||
--- |
|||
|
|||
FastAPI is een modern, snel (zeer goede prestaties), web framework voor het bouwen van API's in Python, gebruikmakend van standaard Python type-hints. |
|||
|
|||
De belangrijkste kenmerken zijn: |
|||
|
|||
* **Snel**: Zeer goede prestaties, vergelijkbaar met **NodeJS** en **Go** (dankzij Starlette en Pydantic). [Een van de snelste beschikbare Python frameworks](#prestaties). |
|||
* **Snel te programmeren**: Verhoog de snelheid om functionaliteit te ontwikkelen met ongeveer 200% tot 300%. * |
|||
* **Minder bugs**: Verminder ongeveer 40% van de door mensen (ontwikkelaars) veroorzaakte fouten. * |
|||
* **Intuïtief**: Buitengewoon goede ondersteuning voor editors. <abbr title="ook bekend als automatisch aanvullen, automatisch aanvullen, IntelliSense">Overal automische code aanvulling</abbr>. Minder tijd kwijt aan debuggen. |
|||
* **Eenvoudig**: Ontworpen om gemakkelijk te gebruiken en te leren. Minder tijd nodig om documentatie te lezen. |
|||
* **Kort**: Minimaliseer codeduplicatie. Elke parameterdeclaratie ondersteunt meerdere functionaliteiten. Minder bugs. |
|||
* **Robust**: Code gereed voor productie. Met automatische interactieve documentatie. |
|||
* **Standards-based**: Gebaseerd op (en volledig verenigbaar met) open standaarden voor API's: <a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> (voorheen bekend als Swagger) en <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a>. |
|||
|
|||
<small>* schatting op basis van testen met een intern ontwikkelteam en bouwen van productieapplicaties.</small> |
|||
|
|||
## Sponsors |
|||
|
|||
<!-- sponsors --> |
|||
|
|||
{% if sponsors %} |
|||
{% for sponsor in sponsors.gold -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor -%} |
|||
{%- for sponsor in sponsors.silver -%} |
|||
<a href="{{ sponsor.url }}" target="_blank" title="{{ sponsor.title }}"><img src="{{ sponsor.img }}" style="border-radius:15px"></a> |
|||
{% endfor %} |
|||
{% endif %} |
|||
|
|||
<!-- /sponsors --> |
|||
|
|||
<a href="https://fastapi.tiangolo.com/fastapi-people/#sponsors" class="external-link" target="_blank">Overige sponsoren</a> |
|||
|
|||
## Meningen |
|||
|
|||
"_[...] Ik gebruik **FastAPI** heel vaak tegenwoordig. [...] Ik ben van plan om het te gebruiken voor alle **ML-services van mijn team bij Microsoft**. Sommige van deze worden geïntegreerd in het kernproduct van **Windows** en sommige **Office**-producten._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kabir Khan - <strong>Microsoft</strong> <a href="https://github.com/fastapi/fastapi/pull/26" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_We hebben de **FastAPI** library gebruikt om een **REST** server te maken die bevraagd kan worden om **voorspellingen** te maken. [voor Ludwig]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Piero Molino, Yaroslav Dudin en Sai Sumanth Miryala - <strong>Uber</strong> <a href="https://eng.uber.com/ludwig-v0-2/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_**Netflix** is verheugd om een open-source release aan te kondigen van ons **crisismanagement**-orkestratieframework: **Dispatch**! [gebouwd met **FastAPI**]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Kevin Glisson, Marc Vilanova, Forest Monsen - <strong>Netflix</strong> <a href="https://netflixtechblog.com/introducing-dispatch-da4b8a2a8072" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Ik ben super enthousiast over **FastAPI**. Het is zo leuk!_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Brian Okken - <strong><a href="https://pythonbytes.fm/episodes/show/123/time-to-right-the-py-wrongs?time_in_sec=855" target="_blank">Python Bytes</a> podcast presentator</strong> <a href="https://twitter.com/brianokken/status/1112220079972728832" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Wat je hebt gebouwd ziet er echt super solide en gepolijst uit. In veel opzichten is het wat ik wilde dat **Hug** kon zijn - het is echt inspirerend om iemand dit te zien bouwen._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Timothy Crosley - <strong><a href="https://www.hug.rest/" target="_blank">Hug</a> creator</strong> <a href="https://news.ycombinator.com/item?id=19455465" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"Wie geïnteresseerd is in een **modern framework** voor het bouwen van REST API's, bekijkt best eens **FastAPI** [...] Het is snel, gebruiksvriendelijk en gemakkelijk te leren [...]_" |
|||
|
|||
"_We zijn overgestapt naar **FastAPI** voor onze **API's** [...] Het gaat jou vast ook bevallen [...]_" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Ines Montani - Matthew Honnibal - <strong><a href="https://explosion.ai" target="_blank">Explosion AI</a> oprichters - <a href="https://spacy.io" target="_blank">spaCy</a> ontwikkelaars</strong> <a href="https://twitter.com/_inesmontani/status/1144173225322143744" target="_blank"><small>(ref)</small></a> - <a href="https://twitter.com/honnibal/status/1144031421859655680" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
"_Wie een Python API wil bouwen voor productie, kan ik ten stelligste **FastAPI** aanraden. Het is **prachtig ontworpen**, **eenvoudig te gebruiken** en **gemakkelijk schaalbaar**, het is een **cruciale component** geworden in onze strategie om API's centraal te zetten, en het vereenvoudigt automatisering en diensten zoals onze Virtual TAC Engineer._" |
|||
|
|||
<div style="text-align: right; margin-right: 10%;">Deon Pillsbury - <strong>Cisco</strong> <a href="https://www.linkedin.com/posts/deonpillsbury_cisco-cx-python-activity-6963242628536487936-trAp/" target="_blank"><small>(ref)</small></a></div> |
|||
|
|||
--- |
|||
|
|||
## **Typer**, de FastAPI van CLIs |
|||
|
|||
<a href="https://typer.tiangolo.com" target="_blank"><img src="https://typer.tiangolo.com/img/logo-margin/logo-margin-vector.svg" style="width: 20%;"></a> |
|||
|
|||
Als je een <abbr title="Command Line Interface">CLI</abbr>-app bouwt die in de terminal moet worden gebruikt in plaats van een web-API, gebruik dan <a href="https://typer.tiangolo.com/ " class="external-link" target="_blank">**Typer**</a>. |
|||
|
|||
**Typer** is het kleine broertje van FastAPI. En het is bedoeld als de **FastAPI van CLI's**. ️ |
|||
|
|||
## Vereisten |
|||
|
|||
FastAPI staat op de schouders van reuzen: |
|||
|
|||
* <a href="https://www.starlette.io/" class="external-link" target="_blank">Starlette</a> voor de webonderdelen. |
|||
* <a href="https://docs.pydantic.dev/" class="external-link" target="_blank">Pydantic</a> voor de datadelen. |
|||
|
|||
## Installatie |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "fastapi[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
**Opmerking**: Zet `"fastapi[standard]"` tussen aanhalingstekens om ervoor te zorgen dat het werkt in alle terminals. |
|||
|
|||
## Voorbeeld |
|||
|
|||
### Creëer het |
|||
|
|||
* Maak het bestand `main.py` aan met daarin: |
|||
|
|||
```Python |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
<details markdown="1"> |
|||
<summary>Of maak gebruik van <code>async def</code>...</summary> |
|||
|
|||
Als je code gebruik maakt van `async` / `await`, gebruik dan `async def`: |
|||
|
|||
```Python hl_lines="9 14" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
@app.get("/") |
|||
async def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
async def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
``` |
|||
|
|||
**Opmerking**: |
|||
|
|||
Als je het niet weet, kijk dan in het gedeelte _"Heb je haast?"_ over <a href="https://fastapi.tiangolo.com/async/#in-a-hurry" target="_blank">`async` en `await` in de documentatie</a>. |
|||
|
|||
</details> |
|||
|
|||
### Voer het uit |
|||
|
|||
Run de server met: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ fastapi dev main.py |
|||
|
|||
╭────────── FastAPI CLI - Development mode ───────────╮ |
|||
│ │ |
|||
│ Serving at: http://127.0.0.1:8000 │ |
|||
│ │ |
|||
│ API docs: http://127.0.0.1:8000/docs │ |
|||
│ │ |
|||
│ Running in development mode, for production use: │ |
|||
│ │ |
|||
│ fastapi run │ |
|||
│ │ |
|||
╰─────────────────────────────────────────────────────╯ |
|||
|
|||
INFO: Will watch for changes in these directories: ['/home/user/code/awesomeapp'] |
|||
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) |
|||
INFO: Started reloader process [2248755] using WatchFiles |
|||
INFO: Started server process [2248757] |
|||
INFO: Waiting for application startup. |
|||
INFO: Application startup complete. |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
<details markdown="1"> |
|||
<summary>Over het commando <code>fastapi dev main.py</code>...</summary> |
|||
|
|||
Het commando `fastapi dev` leest het `main.py` bestand, detecteert de **FastAPI** app, en start een server met <a href="https://www.uvicorn.org" class="external-link" target="_blank">Uvicorn</a>. |
|||
|
|||
Standaard zal dit commando `fastapi dev` starten met "auto-reload" geactiveerd voor ontwikkeling op het lokale systeem. |
|||
|
|||
Je kan hier meer over lezen in de <a href="https://fastapi.tiangolo.com/fastapi-cli/" target="_blank">FastAPI CLI documentatie</a>. |
|||
|
|||
</details> |
|||
|
|||
### Controleer het |
|||
|
|||
Open je browser op <a href="http://127.0.0.1:8000/items/5?q=somequery" class="external-link" target="_blank">http://127.0.0.1:8000/items/5?q=somequery</a>. |
|||
|
|||
Je zult een JSON response zien: |
|||
|
|||
```JSON |
|||
{"item_id": 5, "q": "somequery"} |
|||
``` |
|||
|
|||
Je hebt een API gemaakt die: |
|||
|
|||
* HTTP verzoeken kan ontvangen op de _paden_ `/` en `/items/{item_id}`. |
|||
* Beide _paden_ hebben `GET` <em>operaties</em> (ook bekend als HTTP _methoden_). |
|||
* Het _pad_ `/items/{item_id}` heeft een _pad parameter_ `item_id` dat een `int` moet zijn. |
|||
* Het _pad_ `/items/{item_id}` heeft een optionele `str` _query parameter_ `q`. |
|||
|
|||
### Interactieve API documentatie |
|||
|
|||
Ga naar <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
Je ziet de automatische interactieve API documentatie (verstrekt door <a href="https://github.com/swagger-api/swagger-ui" class="external-link" target="_blank">Swagger UI</a>): |
|||
|
|||
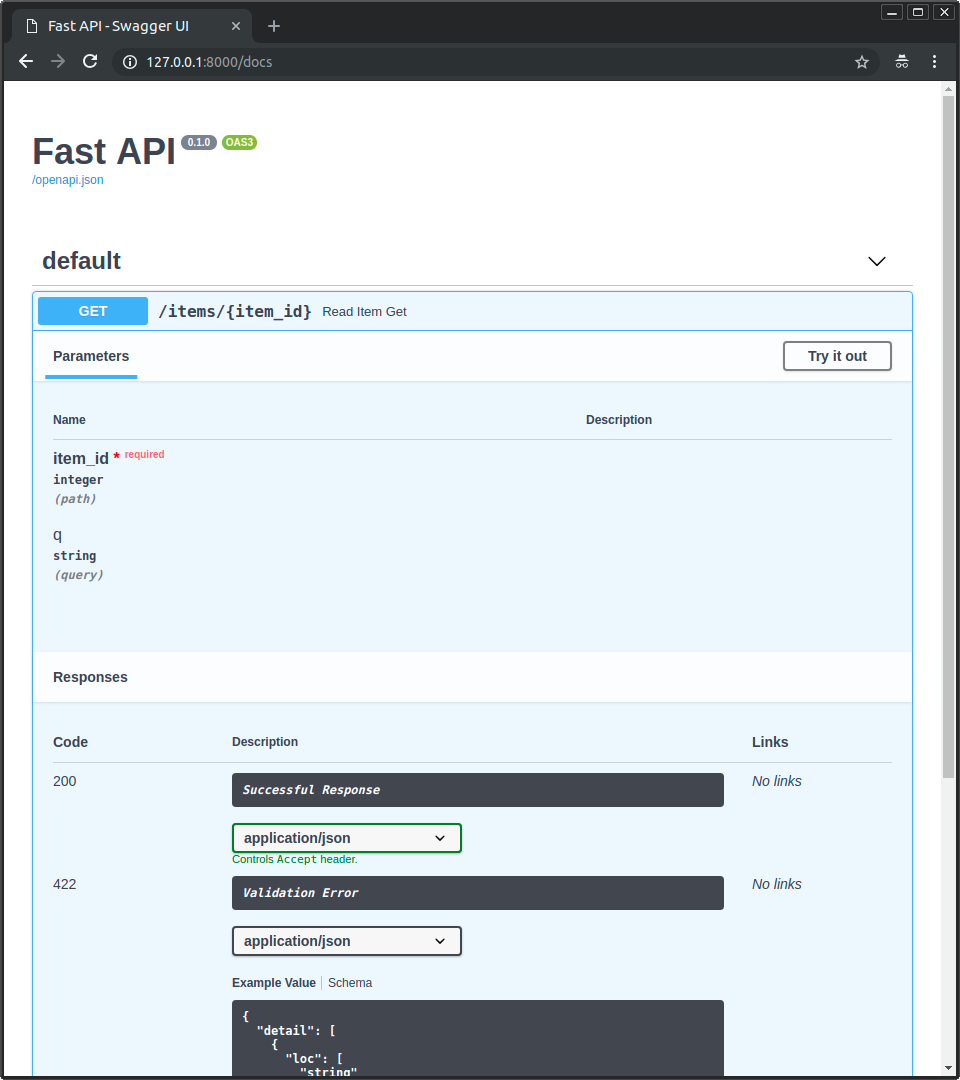 |
|||
|
|||
### Alternatieve API documentatie |
|||
|
|||
Ga vervolgens naar <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
Je ziet de automatische interactieve API documentatie (verstrekt door <a href="https://github.com/Rebilly/ReDoc" class="external-link" target="_blank">ReDoc</a>): |
|||
|
|||
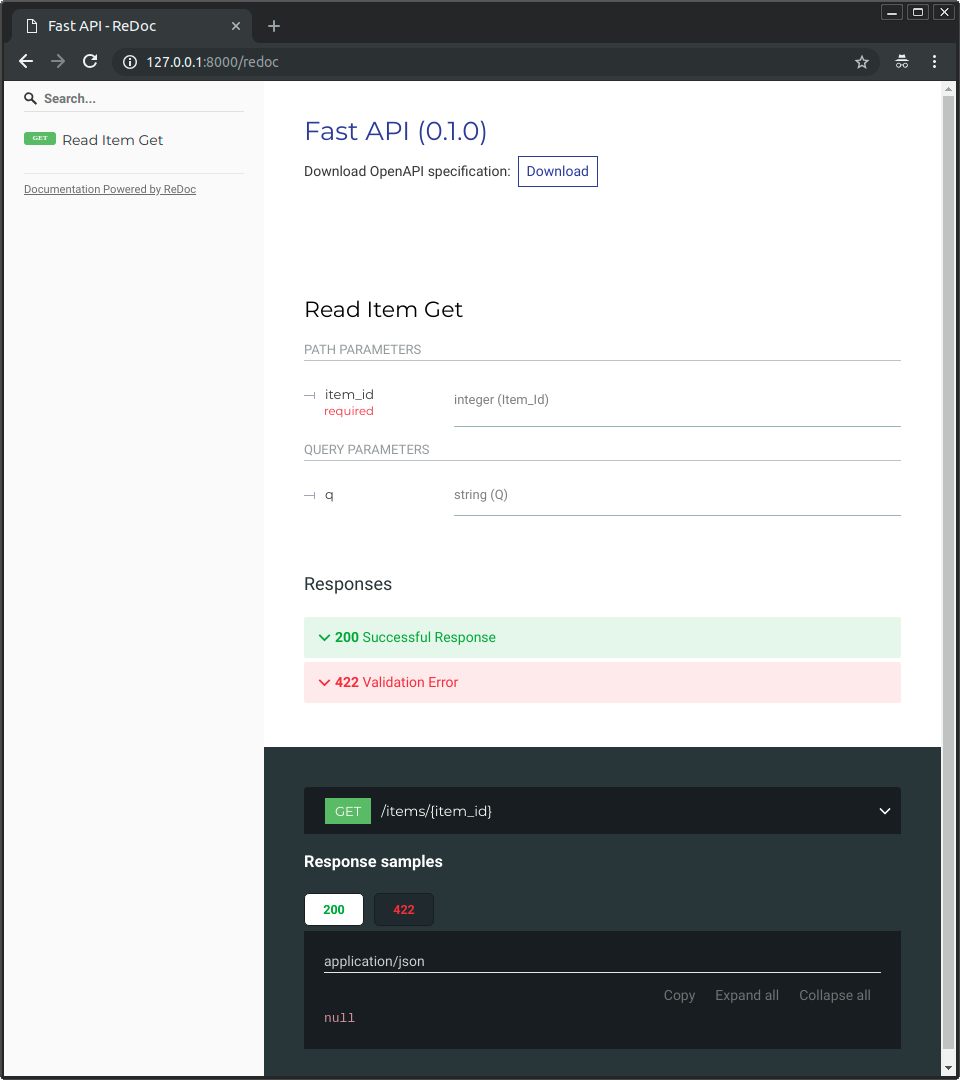 |
|||
|
|||
## Voorbeeld upgrade |
|||
|
|||
Pas nu het bestand `main.py` aan om de body van een `PUT` request te ontvangen. |
|||
|
|||
Dankzij Pydantic kunnen we de body declareren met standaard Python types. |
|||
|
|||
```Python hl_lines="4 9-12 25-27" |
|||
from typing import Union |
|||
|
|||
from fastapi import FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Item(BaseModel): |
|||
name: str |
|||
price: float |
|||
is_offer: Union[bool, None] = None |
|||
|
|||
|
|||
@app.get("/") |
|||
def read_root(): |
|||
return {"Hello": "World"} |
|||
|
|||
|
|||
@app.get("/items/{item_id}") |
|||
def read_item(item_id: int, q: Union[str, None] = None): |
|||
return {"item_id": item_id, "q": q} |
|||
|
|||
|
|||
@app.put("/items/{item_id}") |
|||
def update_item(item_id: int, item: Item): |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
De `fastapi dev` server zou automatisch moeten herladen. |
|||
|
|||
### Interactieve API documentatie upgrade |
|||
|
|||
Ga nu naar <a href="http://127.0.0.1:8000/docs" class="external-link" target="_blank">http://127.0.0.1:8000/docs</a>. |
|||
|
|||
* De interactieve API-documentatie wordt automatisch bijgewerkt, inclusief de nieuwe body: |
|||
|
|||
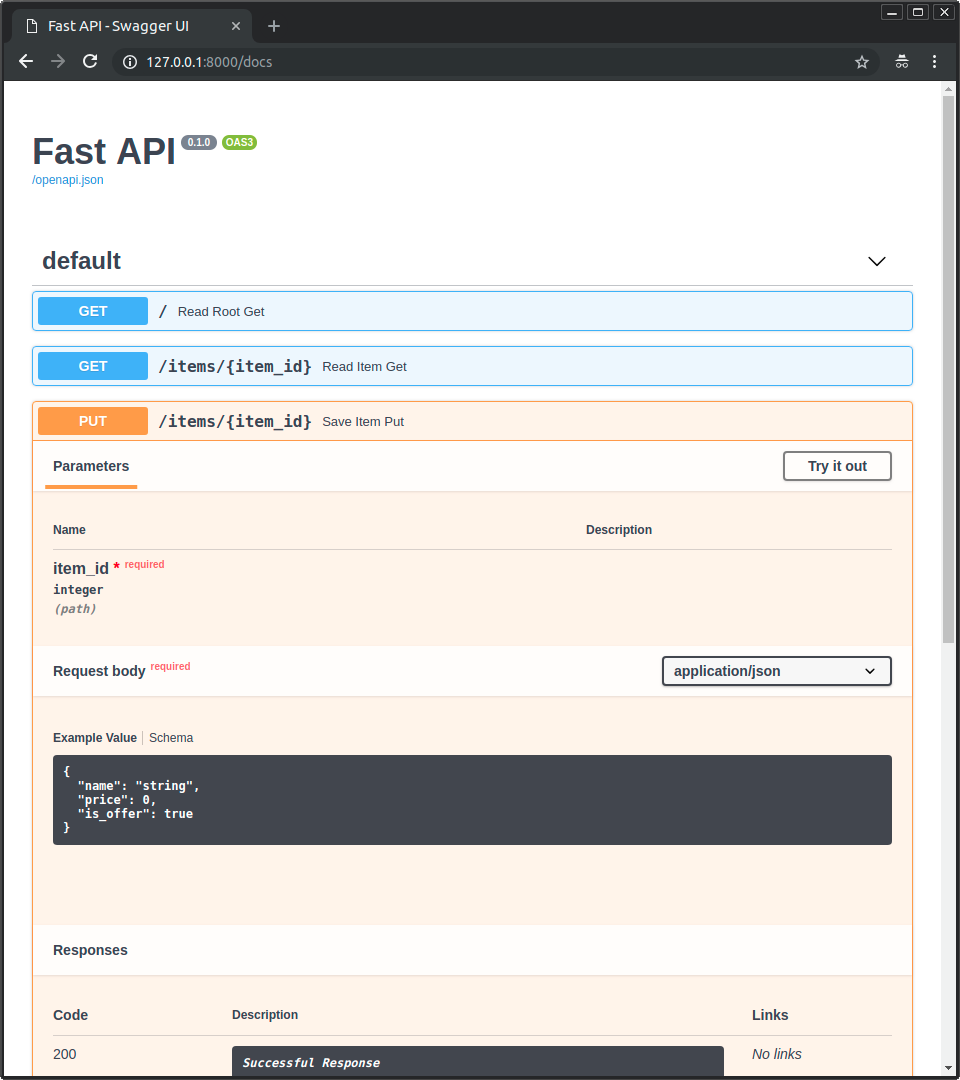 |
|||
|
|||
* Klik op de knop "Try it out", hiermee kan je de parameters invullen en direct met de API interacteren: |
|||
|
|||
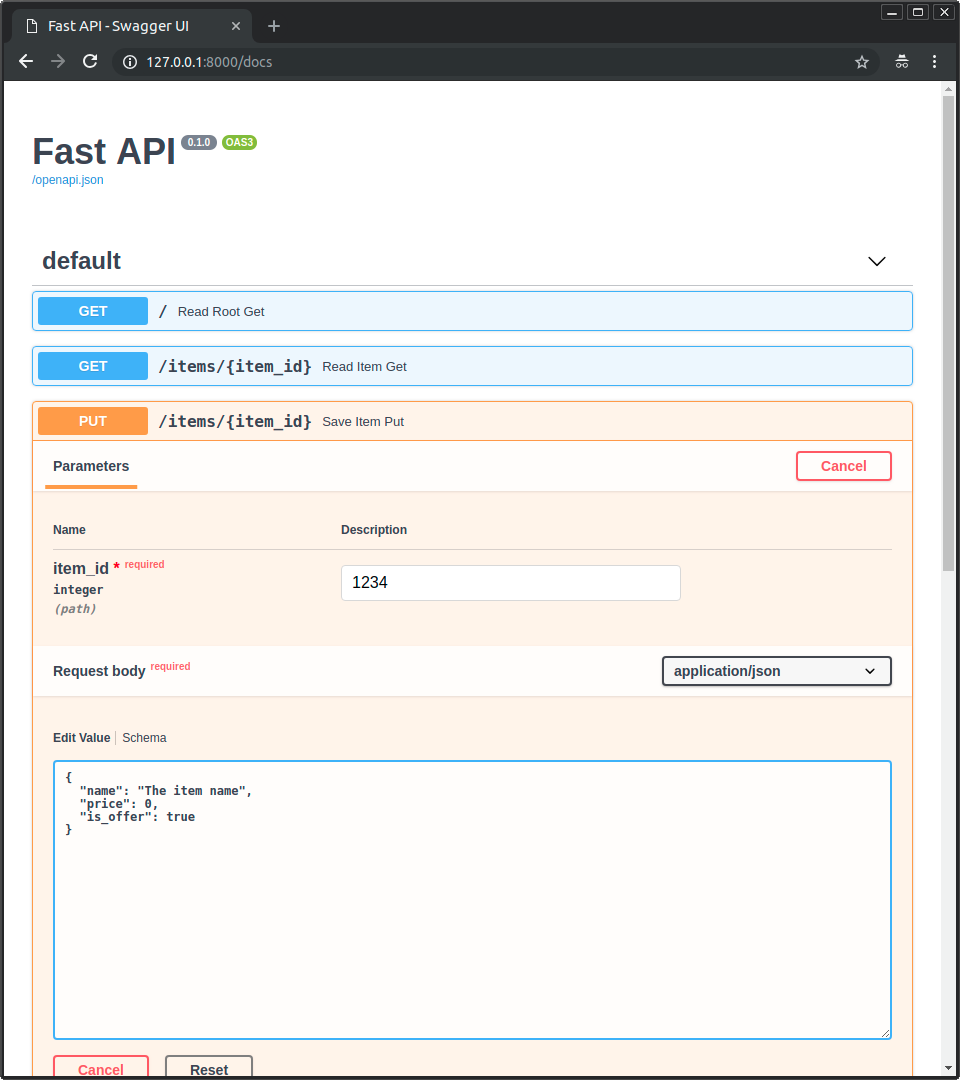 |
|||
|
|||
* Klik vervolgens op de knop "Execute", de gebruikersinterface zal communiceren met jouw API, de parameters verzenden, de resultaten ophalen en deze op het scherm tonen: |
|||
|
|||
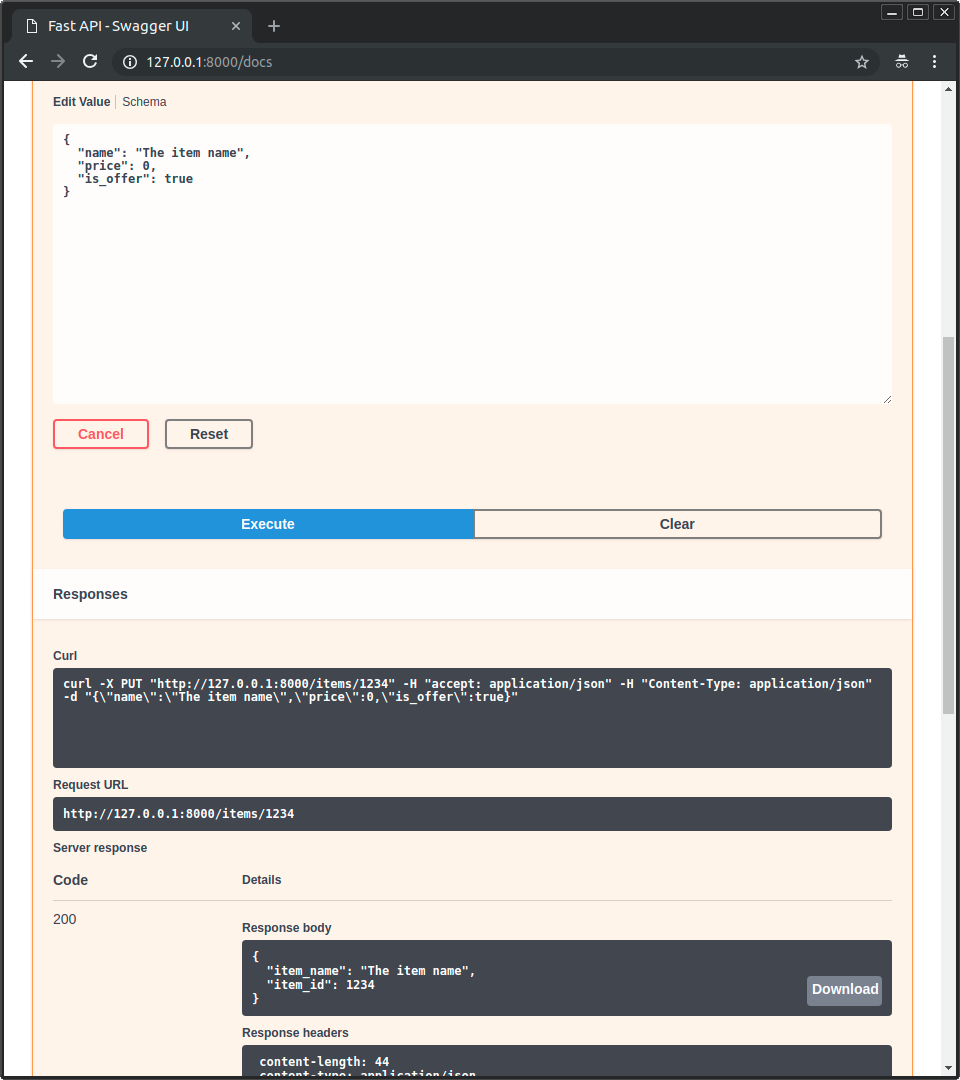 |
|||
|
|||
### Alternatieve API documentatie upgrade |
|||
|
|||
Ga vervolgens naar <a href="http://127.0.0.1:8000/redoc" class="external-link" target="_blank">http://127.0.0.1:8000/redoc</a>. |
|||
|
|||
* De alternatieve documentatie zal ook de nieuwe queryparameter en body weergeven: |
|||
|
|||
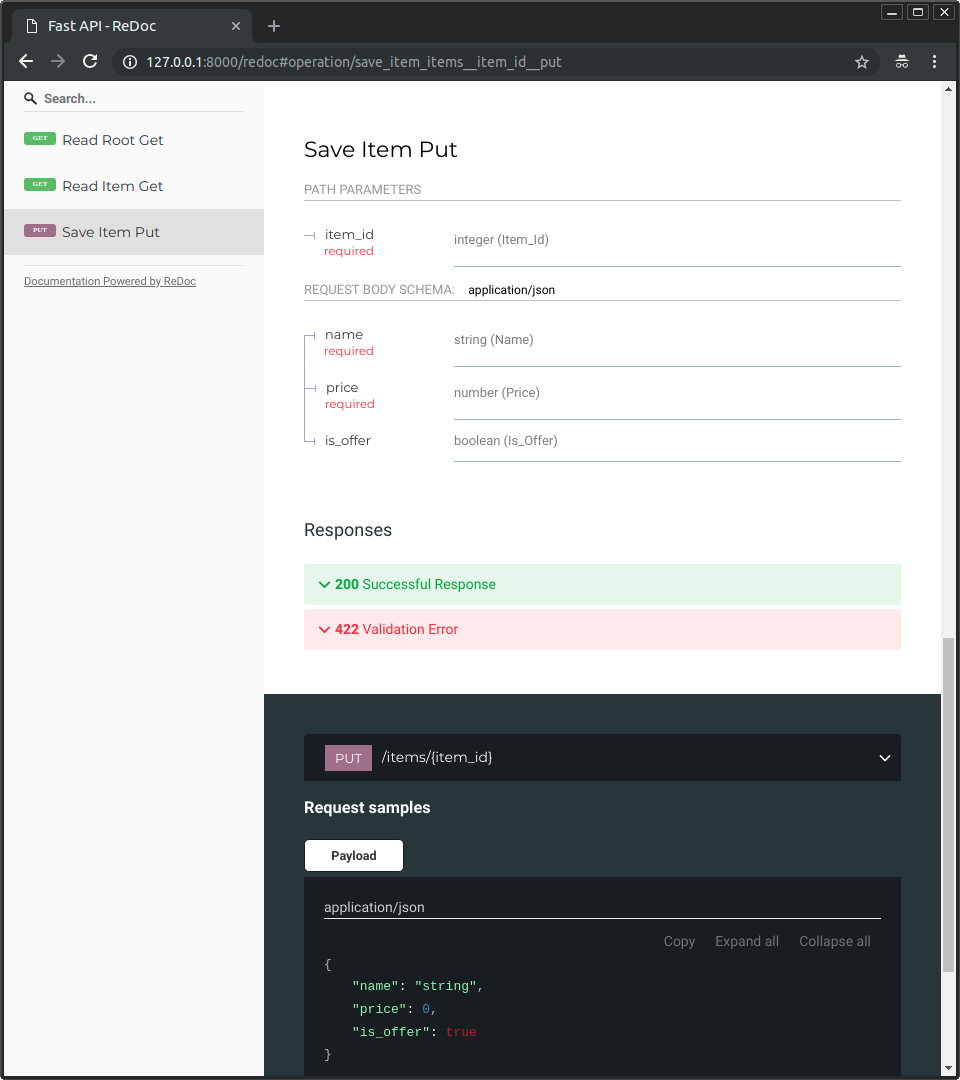 |
|||
|
|||
### Samenvatting |
|||
|
|||
Samengevat declareer je **eenmalig** de types van parameters, body, etc. als functieparameters. |
|||
|
|||
Dat doe je met standaard moderne Python types. |
|||
|
|||
Je hoeft geen nieuwe syntax te leren, de methods of klassen van een specifieke bibliotheek, etc. |
|||
|
|||
Gewoon standaard **Python**. |
|||
|
|||
Bijvoorbeeld, voor een `int`: |
|||
|
|||
```Python |
|||
item_id: int |
|||
``` |
|||
|
|||
of voor een complexer `Item` model: |
|||
|
|||
```Python |
|||
item: Item |
|||
``` |
|||
|
|||
...en met die ene verklaring krijg je: |
|||
|
|||
* Editor ondersteuning, inclusief: |
|||
* Code aanvulling. |
|||
* Type validatie. |
|||
* Validatie van data: |
|||
* Automatische en duidelijke foutboodschappen wanneer de data ongeldig is. |
|||
* Validatie zelfs voor diep geneste JSON objecten. |
|||
* <abbr title="ook bekend als: serialisatie, parsing, marshalling">Conversie</abbr> van invoergegevens: afkomstig van het netwerk naar Python-data en -types. Zoals: |
|||
* JSON. |
|||
* Pad parameters. |
|||
* Query parameters. |
|||
* Cookies. |
|||
* Headers. |
|||
* Formulieren. |
|||
* Bestanden. |
|||
* <abbr title="ook bekend als: serialisatie, parsing, marshalling">Conversie</abbr> van uitvoergegevens: converstie van Python-data en -types naar netwerkgegevens (zoals JSON): |
|||
* Converteer Python types (`str`, `int`, `float`, `bool`, `list`, etc). |
|||
* `datetime` objecten. |
|||
* `UUID` objecten. |
|||
* Database modellen. |
|||
* ...en nog veel meer. |
|||
* Automatische interactieve API-documentatie, inclusief 2 alternatieve gebruikersinterfaces: |
|||
* Swagger UI. |
|||
* ReDoc. |
|||
|
|||
--- |
|||
|
|||
Terugkomend op het vorige code voorbeeld, **FastAPI** zal: |
|||
|
|||
* Valideren dat er een `item_id` bestaat in het pad voor `GET` en `PUT` verzoeken. |
|||
* Valideren dat het `item_id` van het type `int` is voor `GET` en `PUT` verzoeken. |
|||
* Wanneer dat niet het geval is, krijgt de cliënt een nuttige, duidelijke foutmelding. |
|||
* Controleren of er een optionele query parameter is met de naam `q` (zoals in `http://127.0.0.1:8000/items/foo?q=somequery`) voor `GET` verzoeken. |
|||
* Aangezien de `q` parameter werd gedeclareerd met `= None`, is deze optioneel. |
|||
* Zonder de `None` declaratie zou deze verplicht zijn (net als bij de body in het geval met `PUT`). |
|||
* Voor `PUT` verzoeken naar `/items/{item_id}`, lees de body als JSON: |
|||
* Controleer of het een verplicht attribuut `naam` heeft en dat dat een `str` is. |
|||
* Controleer of het een verplicht attribuut `price` heeft en dat dat een`float` is. |
|||
* Controleer of het een optioneel attribuut `is_offer` heeft, dat een `bool` is wanneer het aanwezig is. |
|||
* Dit alles werkt ook voor diep geneste JSON objecten. |
|||
* Converteer automatisch van en naar JSON. |
|||
* Documenteer alles met OpenAPI, dat gebruikt kan worden door: |
|||
* Interactieve documentatiesystemen. |
|||
* Automatische client code generatie systemen, voor vele talen. |
|||
* Biedt 2 interactieve documentatie-webinterfaces aan. |
|||
|
|||
--- |
|||
|
|||
Dit was nog maar een snel overzicht, maar je zou nu toch al een idee moeten hebben over hoe het allemaal werkt. |
|||
|
|||
Probeer deze regel te veranderen: |
|||
|
|||
```Python |
|||
return {"item_name": item.name, "item_id": item_id} |
|||
``` |
|||
|
|||
...van: |
|||
|
|||
```Python |
|||
... "item_name": item.name ... |
|||
``` |
|||
|
|||
...naar: |
|||
|
|||
```Python |
|||
... "item_price": item.price ... |
|||
``` |
|||
|
|||
...en zie hoe je editor de attributen automatisch invult en hun types herkent: |
|||
|
|||
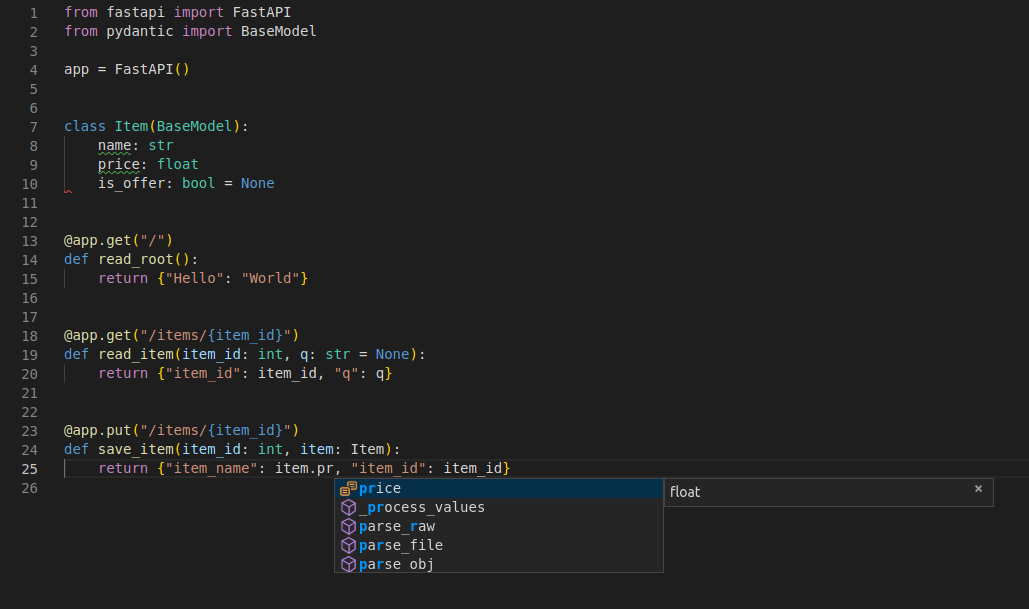 |
|||
|
|||
Voor een vollediger voorbeeld met meer mogelijkheden, zie de <a href="https://fastapi.tiangolo.com/tutorial/">Tutorial - Gebruikershandleiding</a>. |
|||
|
|||
**Spoiler alert**: de tutorial - gebruikershandleiding bevat: |
|||
|
|||
* Declaratie van **parameters** op andere plaatsen zoals: **headers**, **cookies**, **formuliervelden** en **bestanden**. |
|||
* Hoe stel je **validatie restricties** in zoals `maximum_length` of een `regex`. |
|||
* Een zeer krachtig en eenvoudig te gebruiken **<abbr title="ook bekend als componenten, middelen, verstrekkers, diensten, injectables">Dependency Injection</abbr>** systeem. |
|||
* Beveiliging en authenticatie, inclusief ondersteuning voor **OAuth2** met **JWT-tokens** en **HTTP Basic** auth. |
|||
* Meer geavanceerde (maar even eenvoudige) technieken voor het declareren van **diep geneste JSON modellen** (dankzij Pydantic). |
|||
* **GraphQL** integratie met <a href="https://strawberry.rocks" class="external-link" target="_blank">Strawberry</a> en andere packages. |
|||
* Veel extra functies (dankzij Starlette) zoals: |
|||
* **WebSockets** |
|||
* uiterst gemakkelijke tests gebaseerd op HTTPX en `pytest` |
|||
* **CORS** |
|||
* **Cookie Sessions** |
|||
* ...en meer. |
|||
|
|||
## Prestaties |
|||
|
|||
Onafhankelijke TechEmpower benchmarks tonen **FastAPI** applicaties draaiend onder Uvicorn aan als <a href="https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=zijzen-7" class="external-link" target="_blank">een van de snelste Python frameworks beschikbaar</a>, alleen onder Starlette en Uvicorn zelf (intern gebruikt door FastAPI). (*) |
|||
|
|||
Zie de sectie <a href="https://fastapi.tiangolo.com/benchmarks/" class="internal-link" target="_blank">Benchmarks</a> om hier meer over te lezen. |
|||
|
|||
## Afhankelijkheden |
|||
|
|||
FastAPI maakt gebruik van Pydantic en Starlette. |
|||
|
|||
### `standard` Afhankelijkheden |
|||
|
|||
Wanneer je FastAPI installeert met `pip install "fastapi[standard]"`, worden de volgende `standard` optionele afhankelijkheden geïnstalleerd: |
|||
|
|||
Gebruikt door Pydantic: |
|||
|
|||
* <a href="https://github.com/JoshData/python-email-validator" target="_blank"><code>email_validator</code></a> - voor email validatie. |
|||
|
|||
Gebruikt door Starlette: |
|||
|
|||
* <a href="https://www.python-httpx.org" target="_blank"><code>httpx</code></a> - Vereist indien je de `TestClient` wil gebruiken. |
|||
* <a href="https://jinja.palletsprojects.com" target="_blank"><code>jinja2</code></a> - Vereist als je de standaard templateconfiguratie wil gebruiken. |
|||
* <a href="https://github.com/Kludex/python-multipart" target="_blank"><code>python-multipart</code></a> - Vereist indien je <abbr title="het omzetten van de string die uit een HTTP verzoek komt in Python data">"parsen"</abbr> van formulieren wil ondersteunen met `requests.form()`. |
|||
|
|||
Gebruikt door FastAPI / Starlette: |
|||
|
|||
* <a href="https://www.uvicorn.org" target="_blank"><code>uvicorn</code></a> - voor de server die jouw applicatie laadt en bedient. |
|||
* `fastapi-cli` - om het `fastapi` commando te voorzien. |
|||
|
|||
### Zonder `standard` Afhankelijkheden |
|||
|
|||
Indien je de optionele `standard` afhankelijkheden niet wenst te installeren, kan je installeren met `pip install fastapi` in plaats van `pip install "fastapi[standard]"`. |
|||
|
|||
### Bijkomende Optionele Afhankelijkheden |
|||
|
|||
Er zijn nog een aantal bijkomende afhankelijkheden die je eventueel kan installeren. |
|||
|
|||
Bijkomende optionele afhankelijkheden voor Pydantic: |
|||
|
|||
* <a href="https://docs.pydantic.dev/latest/usage/pydantic_settings/" target="_blank"><code>pydantic-settings</code></a> - voor het beheren van settings. |
|||
* <a href="https://docs.pydantic.dev/latest/usage/types/extra_types/extra_types/" target="_blank"><code>pydantic-extra-types</code></a> - voor extra data types die gebruikt kunnen worden met Pydantic. |
|||
|
|||
Bijkomende optionele afhankelijkheden voor FastAPI: |
|||
|
|||
* <a href="https://github.com/ijl/orjson" target="_blank"><code>orjson</code></a> - Vereist indien je `ORJSONResponse` wil gebruiken. |
|||
* <a href="https://github.com/esnme/ultrajson" target="_blank"><code>ujson</code></a> - Vereist indien je `UJSONResponse` wil gebruiken. |
|||
|
|||
## Licentie |
|||
|
|||
Dit project is gelicenseerd onder de voorwaarden van de MIT licentie. |
@ -0,0 +1,597 @@ |
|||
# Introductie tot Python Types |
|||
|
|||
Python biedt ondersteuning voor optionele "type hints" (ook wel "type annotaties" genoemd). |
|||
|
|||
Deze **"type hints"** of annotaties zijn een speciale syntax waarmee het <abbr title="bijvoorbeeld: str, int, float, bool">type</abbr> van een variabele kan worden gedeclareerd. |
|||
|
|||
Door types voor je variabelen te declareren, kunnen editors en hulpmiddelen je beter ondersteunen. |
|||
|
|||
Dit is slechts een **korte tutorial/opfrisser** over Python type hints. Het behandelt enkel het minimum dat nodig is om ze te gebruiken met **FastAPI**... en dat is relatief weinig. |
|||
|
|||
**FastAPI** is helemaal gebaseerd op deze type hints, ze geven veel voordelen. |
|||
|
|||
Maar zelfs als je **FastAPI** nooit gebruikt, heb je er baat bij om er iets over te leren. |
|||
|
|||
/// note |
|||
|
|||
Als je een Python expert bent en alles al weet over type hints, sla dan dit hoofdstuk over. |
|||
|
|||
/// |
|||
|
|||
## Motivatie |
|||
|
|||
Laten we beginnen met een eenvoudig voorbeeld: |
|||
|
|||
```Python |
|||
{!../../../docs_src/python_types/tutorial001.py!} |
|||
``` |
|||
|
|||
Het aanroepen van dit programma leidt tot het volgende resultaat: |
|||
|
|||
``` |
|||
John Doe |
|||
``` |
|||
|
|||
De functie voert het volgende uit: |
|||
|
|||
* Neem een `first_name` en een `last_name` |
|||
* Converteer de eerste letter van elk naar een hoofdletter met `title()`. |
|||
`` |
|||
* <abbr title="Voegt ze samen, als één. Met de inhoud van de een na de ander.">Voeg samen</abbr> met een spatie in het midden. |
|||
|
|||
```Python hl_lines="2" |
|||
{!../../../docs_src/python_types/tutorial001.py!} |
|||
``` |
|||
|
|||
### Bewerk het |
|||
|
|||
Dit is een heel eenvoudig programma. |
|||
|
|||
Maar stel je nu voor dat je het vanaf nul zou moeten maken. |
|||
|
|||
Op een gegeven moment zou je aan de definitie van de functie zijn begonnen, je had de parameters klaar... |
|||
|
|||
Maar dan moet je “die methode die de eerste letter naar hoofdletters converteert” aanroepen. |
|||
|
|||
Was het `upper`? Was het `uppercase`? `first_uppercase`? `capitalize`? |
|||
|
|||
Dan roep je de hulp in van je oude programmeursvriend, (automatische) code aanvulling in je editor. |
|||
|
|||
Je typt de eerste parameter van de functie, `first_name`, dan een punt (`.`) en drukt dan op `Ctrl+Spatie` om de aanvulling te activeren. |
|||
|
|||
Maar helaas krijg je niets bruikbaars: |
|||
|
|||
<img src="/img/python-types/image01.png"> |
|||
|
|||
### Types toevoegen |
|||
|
|||
Laten we een enkele regel uit de vorige versie aanpassen. |
|||
|
|||
We zullen precies dit fragment, de parameters van de functie, wijzigen van: |
|||
|
|||
```Python |
|||
first_name, last_name |
|||
``` |
|||
|
|||
naar: |
|||
|
|||
```Python |
|||
first_name: str, last_name: str |
|||
``` |
|||
|
|||
Dat is alles. |
|||
|
|||
Dat zijn de "type hints": |
|||
|
|||
```Python hl_lines="1" |
|||
{!../../../docs_src/python_types/tutorial002.py!} |
|||
``` |
|||
|
|||
Dit is niet hetzelfde als het declareren van standaardwaarden zoals bij: |
|||
|
|||
```Python |
|||
first_name="john", last_name="doe" |
|||
``` |
|||
|
|||
Het is iets anders. |
|||
|
|||
We gebruiken dubbele punten (`:`), geen gelijkheidstekens (`=`). |
|||
|
|||
Het toevoegen van type hints verandert normaal gesproken niet wat er gebeurt in je programma t.o.v. wat er zonder type hints zou gebeuren. |
|||
|
|||
Maar stel je voor dat je weer bezig bent met het maken van een functie, maar deze keer met type hints. |
|||
|
|||
Op hetzelfde moment probeer je de automatische aanvulling te activeren met `Ctrl+Spatie` en je ziet: |
|||
|
|||
<img src="/img/python-types/image02.png"> |
|||
|
|||
Nu kun je de opties bekijken en er doorheen scrollen totdat je de optie vindt die “een belletje doet rinkelen”: |
|||
|
|||
<img src="/img/python-types/image03.png"> |
|||
|
|||
### Meer motivatie |
|||
|
|||
Bekijk deze functie, deze heeft al type hints: |
|||
|
|||
```Python hl_lines="1" |
|||
{!../../../docs_src/python_types/tutorial003.py!} |
|||
``` |
|||
|
|||
Omdat de editor de types van de variabelen kent, krijgt u niet alleen aanvulling, maar ook controles op fouten: |
|||
|
|||
<img src="/img/python-types/image04.png"> |
|||
|
|||
Nu weet je hoe je het moet oplossen, converteer `age` naar een string met `str(age)`: |
|||
|
|||
```Python hl_lines="2" |
|||
{!../../../docs_src/python_types/tutorial004.py!} |
|||
``` |
|||
|
|||
## Types declareren |
|||
|
|||
Je hebt net de belangrijkste plek om type hints te declareren gezien. Namelijk als functieparameters. |
|||
|
|||
Dit is ook de belangrijkste plek waar je ze gebruikt met **FastAPI**. |
|||
|
|||
### Eenvoudige types |
|||
|
|||
Je kunt alle standaard Python types declareren, niet alleen `str`. |
|||
|
|||
Je kunt bijvoorbeeld het volgende gebruiken: |
|||
|
|||
* `int` |
|||
* `float` |
|||
* `bool` |
|||
* `bytes` |
|||
|
|||
```Python hl_lines="1" |
|||
{!../../../docs_src/python_types/tutorial005.py!} |
|||
``` |
|||
|
|||
### Generieke types met typeparameters |
|||
|
|||
Er zijn enkele datastructuren die andere waarden kunnen bevatten, zoals `dict`, `list`, `set` en `tuple` en waar ook de interne waarden hun eigen type kunnen hebben. |
|||
|
|||
Deze types die interne types hebben worden “**generieke**” types genoemd. Het is mogelijk om ze te declareren, zelfs met hun interne types. |
|||
|
|||
Om deze types en de interne types te declareren, kun je de standaard Python module `typing` gebruiken. Deze module is speciaal gemaakt om deze type hints te ondersteunen. |
|||
|
|||
#### Nieuwere versies van Python |
|||
|
|||
De syntax met `typing` is **verenigbaar** met alle versies, van Python 3.6 tot aan de nieuwste, inclusief Python 3.9, Python 3.10, enz. |
|||
|
|||
Naarmate Python zich ontwikkelt, worden **nieuwere versies**, met verbeterde ondersteuning voor deze type annotaties, beschikbaar. In veel gevallen hoef je niet eens de `typing` module te importeren en te gebruiken om de type annotaties te declareren. |
|||
|
|||
Als je een recentere versie van Python kunt kiezen voor je project, kun je profiteren van die extra eenvoud. |
|||
|
|||
In alle documentatie staan voorbeelden die compatibel zijn met elke versie van Python (als er een verschil is). |
|||
|
|||
Bijvoorbeeld “**Python 3.6+**” betekent dat het compatibel is met Python 3.6 of hoger (inclusief 3.7, 3.8, 3.9, 3.10, etc). En “**Python 3.9+**” betekent dat het compatibel is met Python 3.9 of hoger (inclusief 3.10, etc). |
|||
|
|||
Als je de **laatste versies van Python** kunt gebruiken, gebruik dan de voorbeelden voor de laatste versie, die hebben de **beste en eenvoudigste syntax**, bijvoorbeeld “**Python 3.10+**”. |
|||
|
|||
#### List |
|||
|
|||
Laten we bijvoorbeeld een variabele definiëren als een `list` van `str`. |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
Declareer de variabele met dezelfde dubbele punt (`:`) syntax. |
|||
|
|||
Als type, vul `list` in. |
|||
|
|||
Doordat de list een type is dat enkele interne types bevat, zet je ze tussen vierkante haakjes: |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/python_types/tutorial006_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
Van `typing`, importeer `List` (met een hoofdletter `L`): |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/python_types/tutorial006.py!} |
|||
``` |
|||
|
|||
Declareer de variabele met dezelfde dubbele punt (`:`) syntax. |
|||
|
|||
Zet als type de `List` die je hebt geïmporteerd uit `typing`. |
|||
|
|||
Doordat de list een type is dat enkele interne types bevat, zet je ze tussen vierkante haakjes: |
|||
|
|||
```Python hl_lines="4" |
|||
{!> ../../../docs_src/python_types/tutorial006.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
/// info |
|||
|
|||
De interne types tussen vierkante haakjes worden “typeparameters” genoemd. |
|||
|
|||
In dit geval is `str` de typeparameter die wordt doorgegeven aan `List` (of `list` in Python 3.9 en hoger). |
|||
|
|||
/// |
|||
|
|||
Dat betekent: “de variabele `items` is een `list`, en elk van de items in deze list is een `str`”. |
|||
|
|||
/// tip |
|||
|
|||
Als je Python 3.9 of hoger gebruikt, hoef je `List` niet te importeren uit `typing`, je kunt in plaats daarvan hetzelfde reguliere `list` type gebruiken. |
|||
|
|||
/// |
|||
|
|||
Door dat te doen, kan je editor ondersteuning bieden, zelfs tijdens het verwerken van items uit de list: |
|||
|
|||
<img src="/img/python-types/image05.png"> |
|||
|
|||
Zonder types is dat bijna onmogelijk om te bereiken. |
|||
|
|||
Merk op dat de variabele `item` een van de elementen is in de lijst `items`. |
|||
|
|||
Toch weet de editor dat het een `str` is, en biedt daar vervolgens ondersteuning voor aan. |
|||
|
|||
#### Tuple en Set |
|||
|
|||
Je kunt hetzelfde doen om `tuple`s en `set`s te declareren: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/python_types/tutorial007_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!> ../../../docs_src/python_types/tutorial007.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Dit betekent: |
|||
|
|||
* De variabele `items_t` is een `tuple` met 3 items, een `int`, nog een `int`, en een `str`. |
|||
* De variabele `items_s` is een `set`, en elk van de items is van het type `bytes`. |
|||
|
|||
#### Dict |
|||
|
|||
Om een `dict` te definiëren, geef je 2 typeparameters door, gescheiden door komma's. |
|||
|
|||
De eerste typeparameter is voor de sleutels (keys) van de `dict`. |
|||
|
|||
De tweede typeparameter is voor de waarden (values) van het `dict`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/python_types/tutorial008_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!> ../../../docs_src/python_types/tutorial008.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Dit betekent: |
|||
|
|||
* De variabele `prices` is een `dict`: |
|||
* De sleutels van dit `dict` zijn van het type `str` (bijvoorbeeld de naam van elk item). |
|||
* De waarden van dit `dict` zijn van het type `float` (bijvoorbeeld de prijs van elk item). |
|||
|
|||
#### Union |
|||
|
|||
Je kunt een variable declareren die van **verschillende types** kan zijn, bijvoorbeeld een `int` of een `str`. |
|||
|
|||
In Python 3.6 en hoger (inclusief Python 3.10) kun je het `Union`-type van `typing` gebruiken en de mogelijke types die je wilt accepteren, tussen de vierkante haakjes zetten. |
|||
|
|||
In Python 3.10 is er ook een **nieuwe syntax** waarin je de mogelijke types kunt scheiden door een <abbr title='ook wel "bitwise of operator" genoemd, maar die betekenis is hier niet relevant'>verticale balk (`|`)</abbr>. |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/python_types/tutorial008b_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!> ../../../docs_src/python_types/tutorial008b.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
In beide gevallen betekent dit dat `item` een `int` of een `str` kan zijn. |
|||
|
|||
#### Mogelijk `None` |
|||
|
|||
Je kunt declareren dat een waarde een type kan hebben, zoals `str`, maar dat het ook `None` kan zijn. |
|||
|
|||
In Python 3.6 en hoger (inclusief Python 3.10) kun je het declareren door `Optional` te importeren en te gebruiken vanuit de `typing`-module. |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!../../../docs_src/python_types/tutorial009.py!} |
|||
``` |
|||
|
|||
Door `Optional[str]` te gebruiken in plaats van alleen `str`, kan de editor je helpen fouten te detecteren waarbij je ervan uit zou kunnen gaan dat een waarde altijd een `str` is, terwijl het in werkelijkheid ook `None` zou kunnen zijn. |
|||
|
|||
`Optional[EenType]` is eigenlijk een snelkoppeling voor `Union[EenType, None]`, ze zijn equivalent. |
|||
|
|||
Dit betekent ook dat je in Python 3.10 `EenType | None` kunt gebruiken: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/python_types/tutorial009_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!> ../../../docs_src/python_types/tutorial009.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ alternative |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!> ../../../docs_src/python_types/tutorial009b.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
#### Gebruik van `Union` of `Optional` |
|||
|
|||
Als je een Python versie lager dan 3.10 gebruikt, is dit een tip vanuit mijn **subjectieve** standpunt: |
|||
|
|||
* 🚨 Vermijd het gebruik van `Optional[EenType]`. |
|||
* Gebruik in plaats daarvan **`Union[EenType, None]`** ✨. |
|||
|
|||
Beide zijn gelijkwaardig en onderliggend zijn ze hetzelfde, maar ik zou `Union` aanraden in plaats van `Optional` omdat het woord “**optional**” lijkt te impliceren dat de waarde optioneel is, en het eigenlijk betekent “het kan `None` zijn”, zelfs als het niet optioneel is en nog steeds vereist is. |
|||
|
|||
Ik denk dat `Union[SomeType, None]` explicieter is over wat het betekent. |
|||
|
|||
Het gaat alleen om de woorden en naamgeving. Maar die naamgeving kan invloed hebben op hoe jij en je teamgenoten over de code denken. |
|||
|
|||
Laten we als voorbeeld deze functie nemen: |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!../../../docs_src/python_types/tutorial009c.py!} |
|||
``` |
|||
|
|||
De parameter `name` is gedefinieerd als `Optional[str]`, maar is **niet optioneel**, je kunt de functie niet aanroepen zonder de parameter: |
|||
|
|||
```Python |
|||
say_hi() # Oh, nee, dit geeft een foutmelding! 😱 |
|||
``` |
|||
|
|||
De `name` parameter is **nog steeds vereist** (niet *optioneel*) omdat het geen standaardwaarde heeft. Toch accepteert `name` `None` als waarde: |
|||
|
|||
```Python |
|||
say_hi(name=None) # Dit werkt, None is geldig 🎉 |
|||
``` |
|||
|
|||
Het goede nieuws is dat als je eenmaal Python 3.10 gebruikt, je je daar geen zorgen meer over hoeft te maken, omdat je dan gewoon `|` kunt gebruiken om unions van types te definiëren: |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!../../../docs_src/python_types/tutorial009c_py310.py!} |
|||
``` |
|||
|
|||
Dan hoef je je geen zorgen te maken over namen als `Optional` en `Union`. 😎 |
|||
|
|||
#### Generieke typen |
|||
|
|||
De types die typeparameters in vierkante haakjes gebruiken, worden **Generieke types** of **Generics** genoemd, bijvoorbeeld: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
Je kunt dezelfde ingebouwde types gebruiken als generics (met vierkante haakjes en types erin): |
|||
|
|||
* `list` |
|||
* `tuple` |
|||
* `set` |
|||
* `dict` |
|||
|
|||
Hetzelfde als bij Python 3.8, uit de `typing`-module: |
|||
|
|||
* `Union` |
|||
* `Optional` (hetzelfde als bij Python 3.8) |
|||
* ...en anderen. |
|||
|
|||
In Python 3.10 kun je , als alternatief voor de generieke `Union` en `Optional`, de <abbr title='ook wel "bitwise or operator" genoemd, maar die betekenis is hier niet relevant'>verticale lijn (`|`)</abbr> gebruiken om unions van typen te voorzien, dat is veel beter en eenvoudiger. |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
Je kunt dezelfde ingebouwde types gebruiken als generieke types (met vierkante haakjes en types erin): |
|||
|
|||
* `list` |
|||
* `tuple` |
|||
* `set` |
|||
* `dict` |
|||
|
|||
En hetzelfde als met Python 3.8, vanuit de `typing`-module: |
|||
|
|||
* `Union` |
|||
* `Optional` |
|||
* ...en anderen. |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
* `List` |
|||
* `Tuple` |
|||
* `Set` |
|||
* `Dict` |
|||
* `Union` |
|||
* `Optional` |
|||
* ...en anderen. |
|||
|
|||
//// |
|||
|
|||
### Klassen als types |
|||
|
|||
Je kunt een klasse ook declareren als het type van een variabele. |
|||
|
|||
Stel dat je een klasse `Person` hebt, met een naam: |
|||
|
|||
```Python hl_lines="1-3" |
|||
{!../../../docs_src/python_types/tutorial010.py!} |
|||
``` |
|||
|
|||
Vervolgens kun je een variabele van het type `Persoon` declareren: |
|||
|
|||
```Python hl_lines="6" |
|||
{!../../../docs_src/python_types/tutorial010.py!} |
|||
``` |
|||
|
|||
Dan krijg je ook nog eens volledige editorondersteuning: |
|||
|
|||
<img src="/img/python-types/image06.png"> |
|||
|
|||
Merk op dat dit betekent dat "`one_person` een **instantie** is van de klasse `Person`". |
|||
|
|||
Dit betekent niet dat `one_person` de **klasse** is met de naam `Person`. |
|||
|
|||
## Pydantic modellen |
|||
|
|||
<a href="https://docs.pydantic.dev/" class="external-link" target="_blank">Pydantic</a> is een Python-pakket voor het uitvoeren van datavalidatie. |
|||
|
|||
Je declareert de "vorm" van de data als klassen met attributen. |
|||
|
|||
Elk attribuut heeft een type. |
|||
|
|||
Vervolgens maak je een instantie van die klasse met een aantal waarden en het valideert de waarden, converteert ze naar het juiste type (als dat het geval is) en geeft je een object met alle data terug. |
|||
|
|||
Daarnaast krijg je volledige editorondersteuning met dat resulterende object. |
|||
|
|||
Een voorbeeld uit de officiële Pydantic-documentatie: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/python_types/tutorial011_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/python_types/tutorial011_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/python_types/tutorial011.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
/// info |
|||
|
|||
Om meer te leren over <a href="https://docs.pydantic.dev/" class="external-link" target="_blank">Pydantic, bekijk de documentatie</a>. |
|||
|
|||
/// |
|||
|
|||
**FastAPI** is volledig gebaseerd op Pydantic. |
|||
|
|||
Je zult veel meer van dit alles in de praktijk zien in de [Tutorial - Gebruikershandleiding](tutorial/index.md){.internal-link target=_blank}. |
|||
|
|||
/// tip |
|||
|
|||
Pydantic heeft een speciaal gedrag wanneer je `Optional` of `Union[EenType, None]` gebruikt zonder een standaardwaarde, je kunt er meer over lezen in de Pydantic-documentatie over <a href="https://docs.pydantic.dev/2.3/usage/models/#required-fields" class="external-link" target="_blank">Verplichte optionele velden</a>. |
|||
|
|||
/// |
|||
|
|||
## Type Hints met Metadata Annotaties |
|||
|
|||
Python heeft ook een functie waarmee je **extra <abbr title="Data over de data, in dit geval informatie over het type, bijvoorbeeld een beschrijving.">metadata</abbr>** in deze type hints kunt toevoegen met behulp van `Annotated`. |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
In Python 3.9 is `Annotated` onderdeel van de standaardpakket, dus je kunt het importeren vanuit `typing`. |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!> ../../../docs_src/python_types/tutorial013_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
In versies lager dan Python 3.9 importeer je `Annotated` vanuit `typing_extensions`. |
|||
|
|||
Het wordt al geïnstalleerd met **FastAPI**. |
|||
|
|||
```Python hl_lines="1 4" |
|||
{!> ../../../docs_src/python_types/tutorial013.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Python zelf doet niets met deze `Annotated` en voor editors en andere hulpmiddelen is het type nog steeds een `str`. |
|||
|
|||
Maar je kunt deze ruimte in `Annotated` gebruiken om **FastAPI** te voorzien van extra metadata over hoe je wilt dat je applicatie zich gedraagt. |
|||
|
|||
Het belangrijkste om te onthouden is dat **de eerste *typeparameter*** die je doorgeeft aan `Annotated` het **werkelijke type** is. De rest is gewoon metadata voor andere hulpmiddelen. |
|||
|
|||
Voor nu hoef je alleen te weten dat `Annotated` bestaat en dat het standaard Python is. 😎 |
|||
|
|||
Later zul je zien hoe **krachtig** het kan zijn. |
|||
|
|||
/// tip |
|||
|
|||
Het feit dat dit **standaard Python** is, betekent dat je nog steeds de **best mogelijke ontwikkelaarservaring** krijgt in je editor, met de hulpmiddelen die je gebruikt om je code te analyseren en te refactoren, enz. ✨ |
|||
|
|||
Daarnaast betekent het ook dat je code zeer verenigbaar zal zijn met veel andere Python-hulpmiddelen en -pakketten. 🚀 |
|||
|
|||
/// |
|||
|
|||
## Type hints in **FastAPI** |
|||
|
|||
**FastAPI** maakt gebruik van type hints om verschillende dingen te doen. |
|||
|
|||
Met **FastAPI** declareer je parameters met type hints en krijg je: |
|||
|
|||
* **Editor ondersteuning**. |
|||
* **Type checks**. |
|||
|
|||
...en **FastAPI** gebruikt dezelfde declaraties om: |
|||
|
|||
* **Vereisten te definïeren **: van request pad parameters, query parameters, headers, bodies, dependencies, enz. |
|||
* **Data te converteren**: van de request naar het vereiste type. |
|||
* **Data te valideren**: afkomstig van elke request: |
|||
* **Automatische foutmeldingen** te genereren die naar de client worden geretourneerd wanneer de data ongeldig is. |
|||
* De API met OpenAPI te **documenteren**: |
|||
* die vervolgens wordt gebruikt door de automatische interactieve documentatie gebruikersinterfaces. |
|||
|
|||
Dit klinkt misschien allemaal abstract. Maak je geen zorgen. Je ziet dit allemaal in actie in de [Tutorial - Gebruikershandleiding](tutorial/index.md){.internal-link target=_blank}. |
|||
|
|||
Het belangrijkste is dat door standaard Python types te gebruiken, op één plek (in plaats van meer klassen, decorators, enz. toe te voegen), **FastAPI** een groot deel van het werk voor je doet. |
|||
|
|||
/// info |
|||
|
|||
Als je de hele tutorial al hebt doorgenomen en terug bent gekomen om meer te weten te komen over types, is een goede bron <a href="https://mypy.readthedocs.io/en/latest/cheat_sheet_py3.html" class="external-link" target="_blank">het "cheat sheet" van `mypy`</a>. |
|||
|
|||
/// |
@ -0,0 +1 @@ |
|||
INHERIT: ../en/mkdocs.yml |
@ -0,0 +1,192 @@ |
|||
# HTTP Basic Auth |
|||
|
|||
Para os casos mais simples, você pode utilizar o HTTP Basic Auth. |
|||
|
|||
No HTTP Basic Auth, a aplicação espera um cabeçalho que contém um usuário e uma senha. |
|||
|
|||
Caso ela não receba, ela retorna um erro HTTP 401 "Unauthorized" (*Não Autorizado*). |
|||
|
|||
E retorna um cabeçalho `WWW-Authenticate` com o valor `Basic`, e um parâmetro opcional `realm`. |
|||
|
|||
Isso sinaliza ao navegador para mostrar o prompt integrado para um usuário e senha. |
|||
|
|||
Então, quando você digitar o usuário e senha, o navegador os envia automaticamente no cabeçalho. |
|||
|
|||
## HTTP Basic Auth Simples |
|||
|
|||
* Importe `HTTPBasic` e `HTTPBasicCredentials`. |
|||
* Crie um "esquema `security`" utilizando `HTTPBasic`. |
|||
* Utilize o `security` com uma dependência em sua *operação de rota*. |
|||
* Isso retorna um objeto do tipo `HTTPBasicCredentials`: |
|||
* Isto contém o `username` e o `password` enviado. |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="4 8 12" |
|||
{!> ../../../docs_src/security/tutorial006_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="2 7 11" |
|||
{!> ../../../docs_src/security/tutorial006_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Prefira utilizar a versão `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="2 6 10" |
|||
{!> ../../../docs_src/security/tutorial006.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Quando você tentar abrir a URL pela primeira vez (ou clicar no botão "Executar" nos documentos) o navegador vai pedir pelo seu usuário e senha: |
|||
|
|||
<img src="/img/tutorial/security/image12.png"> |
|||
|
|||
## Verifique o usuário |
|||
|
|||
Aqui está um exemplo mais completo. |
|||
|
|||
Utilize uma dependência para verificar se o usuário e a senha estão corretos. |
|||
|
|||
Para isso, utilize o módulo padrão do Python <a href="https://docs.python.org/3/library/secrets.html" class="external-link" target="_blank">`secrets`</a> para verificar o usuário e senha. |
|||
|
|||
O `secrets.compare_digest()` necessita receber `bytes` ou `str` que possuem apenas caracteres ASCII (os em Inglês). Isso significa que não funcionaria com caracteres como o `á`, como em `Sebastián`. |
|||
|
|||
Para lidar com isso, primeiramente nós convertemos o `username` e o `password` para `bytes`, codificando-os com UTF-8. |
|||
|
|||
Então nós podemos utilizar o `secrets.compare_digest()` para garantir que o `credentials.username` é `"stanleyjobson"`, e que o `credentials.password` é `"swordfish"`. |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="1 12-24" |
|||
{!> ../../../docs_src/security/tutorial007_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="1 12-24" |
|||
{!> ../../../docs_src/security/tutorial007_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Prefira utilizar a versão `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="1 11-21" |
|||
{!> ../../../docs_src/security/tutorial007.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Isso seria parecido com: |
|||
|
|||
```Python |
|||
if not (credentials.username == "stanleyjobson") or not (credentials.password == "swordfish"): |
|||
# Return some error |
|||
... |
|||
``` |
|||
|
|||
Porém ao utilizar o `secrets.compare_digest()`, isso estará seguro contra um tipo de ataque chamado "ataque de temporização (timing attacks)". |
|||
|
|||
### Ataques de Temporização |
|||
|
|||
Mas o que é um "ataque de temporização"? |
|||
|
|||
Vamos imaginar que alguns invasores estão tentando adivinhar o usuário e a senha. |
|||
|
|||
E eles enviam uma requisição com um usuário `johndoe` e uma senha `love123`. |
|||
|
|||
Então o código Python em sua aplicação seria equivalente a algo como: |
|||
|
|||
```Python |
|||
if "johndoe" == "stanleyjobson" and "love123" == "swordfish": |
|||
... |
|||
``` |
|||
|
|||
Mas no exato momento que o Python compara o primeiro `j` em `johndoe` contra o primeiro `s` em `stanleyjobson`, ele retornará `False`, porque ele já sabe que aquelas duas strings não são a mesma, pensando que "não existe a necessidade de desperdiçar mais poder computacional comparando o resto das letras". E a sua aplicação dirá "Usuário ou senha incorretos". |
|||
|
|||
Mas então os invasores vão tentar com o usuário `stanleyjobsox` e a senha `love123`. |
|||
|
|||
E a sua aplicação faz algo como: |
|||
|
|||
```Python |
|||
if "stanleyjobsox" == "stanleyjobson" and "love123" == "swordfish": |
|||
... |
|||
``` |
|||
|
|||
O Python terá que comparar todo o `stanleyjobso` tanto em `stanleyjobsox` como em `stanleyjobson` antes de perceber que as strings não são a mesma. Então isso levará alguns microsegundos a mais para retornar "Usuário ou senha incorretos". |
|||
|
|||
#### O tempo para responder ajuda os invasores |
|||
|
|||
Neste ponto, ao perceber que o servidor demorou alguns microsegundos a mais para enviar o retorno "Usuário ou senha incorretos", os invasores irão saber que eles acertaram _alguma coisa_, algumas das letras iniciais estavam certas. |
|||
|
|||
E eles podem tentar de novo sabendo que provavelmente é algo mais parecido com `stanleyjobsox` do que com `johndoe`. |
|||
|
|||
#### Um ataque "profissional" |
|||
|
|||
Claro, os invasores não tentariam tudo isso de forma manual, eles escreveriam um programa para fazer isso, possivelmente com milhares ou milhões de testes por segundo. E obteriam apenas uma letra a mais por vez. |
|||
|
|||
Mas fazendo isso, em alguns minutos ou horas os invasores teriam adivinhado o usuário e senha corretos, com a "ajuda" da nossa aplicação, apenas usando o tempo levado para responder. |
|||
|
|||
#### Corrija com o `secrets.compare_digest()` |
|||
|
|||
Mas em nosso código nós estamos utilizando o `secrets.compare_digest()`. |
|||
|
|||
Resumindo, levará o mesmo tempo para comparar `stanleyjobsox` com `stanleyjobson` do que comparar `johndoe` com `stanleyjobson`. E o mesmo para a senha. |
|||
|
|||
Deste modo, ao utilizar `secrets.compare_digest()` no código de sua aplicação, ela esterá a salvo contra toda essa gama de ataques de segurança. |
|||
|
|||
|
|||
### Retorne o erro |
|||
|
|||
Depois de detectar que as credenciais estão incorretas, retorne um `HTTPException` com o status 401 (o mesmo retornado quando nenhuma credencial foi informada) e adicione o cabeçalho `WWW-Authenticate` para fazer com que o navegador mostre o prompt de login novamente: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="26-30" |
|||
{!> ../../../docs_src/security/tutorial007_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="26-30" |
|||
{!> ../../../docs_src/security/tutorial007_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Prefira utilizar a versão `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="23-27" |
|||
{!> ../../../docs_src/security/tutorial007.py!} |
|||
``` |
|||
|
|||
//// |
@ -0,0 +1,19 @@ |
|||
# Segurança Avançada |
|||
|
|||
## Funcionalidades Adicionais |
|||
|
|||
Existem algumas funcionalidades adicionais para lidar com segurança além das cobertas em [Tutorial - Guia de Usuário: Segurança](../../tutorial/security/index.md){.internal-link target=_blank}. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
As próximas seções **não são necessariamente "avançadas"**. |
|||
|
|||
E é possível que para o seu caso de uso, a solução está em uma delas. |
|||
|
|||
/// |
|||
|
|||
## Leia o Tutorial primeiro |
|||
|
|||
As próximas seções pressupõem que você já leu o principal [Tutorial - Guia de Usuário: Segurança](../../tutorial/security/index.md){.internal-link target=_blank}. |
|||
|
|||
Todas elas são baseadas nos mesmos conceitos, mas permitem algumas funcionalidades extras. |
@ -0,0 +1,7 @@ |
|||
# Testando Eventos: inicialização - encerramento |
|||
|
|||
Quando você precisa que os seus manipuladores de eventos (`startup` e `shutdown`) sejam executados em seus testes, você pode utilizar o `TestClient` usando a instrução `with`: |
|||
|
|||
```Python hl_lines="9-12 20-24" |
|||
{!../../../docs_src/app_testing/tutorial003.py!} |
|||
``` |
@ -0,0 +1,298 @@ |
|||
# Variáveis de Ambiente |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Se você já sabe o que são "variáveis de ambiente" e como usá-las, pode pular esta seção. |
|||
|
|||
/// |
|||
|
|||
Uma variável de ambiente (também conhecida como "**env var**") é uma variável que existe **fora** do código Python, no **sistema operacional**, e pode ser lida pelo seu código Python (ou por outros programas também). |
|||
|
|||
Variáveis de ambiente podem ser úteis para lidar com **configurações** do aplicativo, como parte da **instalação** do Python, etc. |
|||
|
|||
## Criar e Usar Variáveis de Ambiente |
|||
|
|||
Você pode **criar** e usar variáveis de ambiente no **shell (terminal)**, sem precisar do Python: |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Você pode criar uma variável de ambiente MY_NAME com |
|||
$ export MY_NAME="Wade Wilson" |
|||
|
|||
// Então você pode usá-la com outros programas, como |
|||
$ echo "Hello $MY_NAME" |
|||
|
|||
Hello Wade Wilson |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Criar uma variável de ambiente MY_NAME |
|||
$ $Env:MY_NAME = "Wade Wilson" |
|||
|
|||
// Usá-la com outros programas, como |
|||
$ echo "Hello $Env:MY_NAME" |
|||
|
|||
Hello Wade Wilson |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
## Ler Variáveis de Ambiente no Python |
|||
|
|||
Você também pode criar variáveis de ambiente **fora** do Python, no terminal (ou com qualquer outro método) e depois **lê-las no Python**. |
|||
|
|||
Por exemplo, você poderia ter um arquivo `main.py` com: |
|||
|
|||
```Python hl_lines="3" |
|||
import os |
|||
|
|||
name = os.getenv("MY_NAME", "World") |
|||
print(f"Hello {name} from Python") |
|||
``` |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
O segundo argumento para <a href="https://docs.python.org/3.8/library/os.html#os.getenv" class="external-link" target="_blank">`os.getenv()`</a> é o valor padrão a ser retornado. |
|||
|
|||
Se não for fornecido, é `None` por padrão, Aqui fornecemos `"World"` como o valor padrão a ser usado. |
|||
|
|||
/// |
|||
|
|||
Então você poderia chamar esse programa Python: |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Aqui ainda não definimos a variável de ambiente |
|||
$ python main.py |
|||
|
|||
// Como não definimos a variável de ambiente, obtemos o valor padrão |
|||
|
|||
Hello World from Python |
|||
|
|||
// Mas se criarmos uma variável de ambiente primeiro |
|||
$ export MY_NAME="Wade Wilson" |
|||
|
|||
// E então chamar o programa novamente |
|||
$ python main.py |
|||
|
|||
// Agora ele pode ler a variável de ambiente |
|||
|
|||
Hello Wade Wilson from Python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Aqui ainda não definimos a variável de ambiente |
|||
$ python main.py |
|||
|
|||
// Como não definimos a variável de ambiente, obtemos o valor padrão |
|||
|
|||
Hello World from Python |
|||
|
|||
// Mas se criarmos uma variável de ambiente primeiro |
|||
$ $Env:MY_NAME = "Wade Wilson" |
|||
|
|||
// E então chamar o programa novamente |
|||
$ python main.py |
|||
|
|||
// Agora ele pode ler a variável de ambiente |
|||
|
|||
Hello Wade Wilson from Python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
Como as variáveis de ambiente podem ser definidas fora do código, mas podem ser lidas pelo código e não precisam ser armazenadas (com versão no `git`) com o restante dos arquivos, é comum usá-las para configurações ou **definições**. |
|||
|
|||
Você também pode criar uma variável de ambiente apenas para uma **invocação específica do programa**, que só está disponível para aquele programa e apenas pela duração dele. |
|||
|
|||
Para fazer isso, crie-a na mesma linha, antes do próprio programa: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Criar uma variável de ambiente MY_NAME para esta chamada de programa |
|||
$ MY_NAME="Wade Wilson" python main.py |
|||
|
|||
// Agora ele pode ler a variável de ambiente |
|||
|
|||
Hello Wade Wilson from Python |
|||
|
|||
// A variável de ambiente não existe mais depois |
|||
$ python main.py |
|||
|
|||
Hello World from Python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Você pode ler mais sobre isso em <a href="https://12factor.net/config" class="external-link" target="_blank">The Twelve-Factor App: Config</a>. |
|||
|
|||
/// |
|||
|
|||
## Tipos e Validação |
|||
|
|||
Essas variáveis de ambiente só podem lidar com **strings de texto**, pois são externas ao Python e precisam ser compatíveis com outros programas e com o resto do sistema (e até mesmo com diferentes sistemas operacionais, como Linux, Windows, macOS). |
|||
|
|||
Isso significa que **qualquer valor** lido em Python de uma variável de ambiente **será uma `str`**, e qualquer conversão para um tipo diferente ou qualquer validação precisa ser feita no código. |
|||
|
|||
Você aprenderá mais sobre como usar variáveis de ambiente para lidar com **configurações do aplicativo** no [Guia do Usuário Avançado - Configurações e Variáveis de Ambiente](./advanced/settings.md){.internal-link target=_blank}. |
|||
|
|||
## Variável de Ambiente `PATH` |
|||
|
|||
Existe uma variável de ambiente **especial** chamada **`PATH`** que é usada pelos sistemas operacionais (Linux, macOS, Windows) para encontrar programas para executar. |
|||
|
|||
O valor da variável `PATH` é uma longa string composta por diretórios separados por dois pontos `:` no Linux e macOS, e por ponto e vírgula `;` no Windows. |
|||
|
|||
Por exemplo, a variável de ambiente `PATH` poderia ter esta aparência: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
```plaintext |
|||
/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin |
|||
``` |
|||
|
|||
Isso significa que o sistema deve procurar programas nos diretórios: |
|||
|
|||
* `/usr/local/bin` |
|||
* `/usr/bin` |
|||
* `/bin` |
|||
* `/usr/sbin` |
|||
* `/sbin` |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
```plaintext |
|||
C:\Program Files\Python312\Scripts;C:\Program Files\Python312;C:\Windows\System32 |
|||
``` |
|||
|
|||
Isso significa que o sistema deve procurar programas nos diretórios: |
|||
|
|||
* `C:\Program Files\Python312\Scripts` |
|||
* `C:\Program Files\Python312` |
|||
* `C:\Windows\System32` |
|||
|
|||
//// |
|||
|
|||
Quando você digita um **comando** no terminal, o sistema operacional **procura** o programa em **cada um dos diretórios** listados na variável de ambiente `PATH`. |
|||
|
|||
Por exemplo, quando você digita `python` no terminal, o sistema operacional procura um programa chamado `python` no **primeiro diretório** dessa lista. |
|||
|
|||
Se ele o encontrar, então ele o **usará**. Caso contrário, ele continua procurando nos **outros diretórios**. |
|||
|
|||
### Instalando o Python e Atualizando o `PATH` |
|||
|
|||
Durante a instalação do Python, você pode ser questionado sobre a atualização da variável de ambiente `PATH`. |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
Vamos supor que você instale o Python e ele fique em um diretório `/opt/custompython/bin`. |
|||
|
|||
Se você concordar em atualizar a variável de ambiente `PATH`, o instalador adicionará `/opt/custompython/bin` para a variável de ambiente `PATH`. |
|||
|
|||
Poderia parecer assim: |
|||
|
|||
```plaintext |
|||
/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/opt/custompython/bin |
|||
``` |
|||
|
|||
Dessa forma, ao digitar `python` no terminal, o sistema encontrará o programa Python em `/opt/custompython/bin` (último diretório) e o utilizará. |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
Digamos que você instala o Python e ele acaba em um diretório `C:\opt\custompython\bin`. |
|||
|
|||
Se você disser sim para atualizar a variável de ambiente `PATH`, o instalador adicionará `C:\opt\custompython\bin` à variável de ambiente `PATH`. |
|||
|
|||
```plaintext |
|||
C:\Program Files\Python312\Scripts;C:\Program Files\Python312;C:\Windows\System32;C:\opt\custompython\bin |
|||
``` |
|||
|
|||
Dessa forma, quando você digitar `python` no terminal, o sistema encontrará o programa Python em `C:\opt\custompython\bin` (o último diretório) e o utilizará. |
|||
|
|||
//// |
|||
|
|||
Então, se você digitar: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
O sistema **encontrará** o programa `python` em `/opt/custompython/bin` e o executará. |
|||
|
|||
Seria aproximadamente equivalente a digitar: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ /opt/custompython/bin/python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
O sistema **encontrará** o programa `python` em `C:\opt\custompython\bin\python` e o executará. |
|||
|
|||
Seria aproximadamente equivalente a digitar: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ C:\opt\custompython\bin\python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
Essas informações serão úteis ao aprender sobre [Ambientes Virtuais](virtual-environments.md){.internal-link target=_blank}. |
|||
|
|||
## Conclusão |
|||
|
|||
Com isso, você deve ter uma compreensão básica do que são **variáveis de ambiente** e como usá-las em Python. |
|||
|
|||
Você também pode ler mais sobre elas na <a href="https://en.wikipedia.org/wiki/Environment_variable" class="external-link" target="_blank">Wikipedia para Variáveis de Ambiente</a>. |
|||
|
|||
Em muitos casos, não é muito óbvio como as variáveis de ambiente seriam úteis e aplicáveis imediatamente. Mas elas continuam aparecendo em muitos cenários diferentes quando você está desenvolvendo, então é bom saber sobre elas. |
|||
|
|||
Por exemplo, você precisará dessas informações na próxima seção, sobre [Ambientes Virtuais](virtual-environments.md). |
@ -0,0 +1,115 @@ |
|||
# Depuração |
|||
|
|||
Você pode conectar o depurador no seu editor, por exemplo, com o Visual Studio Code ou PyCharm. |
|||
|
|||
## Chamar `uvicorn` |
|||
|
|||
Em seu aplicativo FastAPI, importe e execute `uvicorn` diretamente: |
|||
|
|||
```Python hl_lines="1 15" |
|||
{!../../../docs_src/debugging/tutorial001.py!} |
|||
``` |
|||
|
|||
### Sobre `__name__ == "__main__"` |
|||
|
|||
O objetivo principal de `__name__ == "__main__"` é ter algum código que seja executado quando seu arquivo for chamado com: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python myapp.py |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
mas não é chamado quando outro arquivo o importa, como em: |
|||
|
|||
```Python |
|||
from myapp import app |
|||
``` |
|||
|
|||
#### Mais detalhes |
|||
|
|||
Digamos que seu arquivo se chama `myapp.py`. |
|||
|
|||
Se você executá-lo com: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python myapp.py |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
então a variável interna `__name__` no seu arquivo, criada automaticamente pelo Python, terá como valor a string `"__main__"`. |
|||
|
|||
Então, a seção: |
|||
|
|||
```Python |
|||
uvicorn.run(app, host="0.0.0.0", port=8000) |
|||
``` |
|||
|
|||
vai executar. |
|||
|
|||
--- |
|||
|
|||
Isso não acontecerá se você importar esse módulo (arquivo). |
|||
|
|||
Então, se você tiver outro arquivo `importer.py` com: |
|||
|
|||
```Python |
|||
from myapp import app |
|||
|
|||
# Mais um pouco de código |
|||
``` |
|||
|
|||
nesse caso, a variável criada automaticamente dentro de `myapp.py` não terá a variável `__name__` com o valor `"__main__"`. |
|||
|
|||
Então, a linha: |
|||
|
|||
```Python |
|||
uvicorn.run(app, host="0.0.0.0", port=8000) |
|||
``` |
|||
|
|||
não será executada. |
|||
|
|||
/// info | "Informação" |
|||
|
|||
Para mais informações, consulte <a href="https://docs.python.org/3/library/__main__.html" class="external-link" target="_blank">a documentação oficial do Python</a>. |
|||
|
|||
/// |
|||
|
|||
## Execute seu código com seu depurador |
|||
|
|||
Como você está executando o servidor Uvicorn diretamente do seu código, você pode chamar seu programa Python (seu aplicativo FastAPI) diretamente do depurador. |
|||
|
|||
--- |
|||
|
|||
Por exemplo, no Visual Studio Code, você pode: |
|||
|
|||
* Ir para o painel "Debug". |
|||
* "Add configuration...". |
|||
* Selecionar "Python" |
|||
* Executar o depurador com a opção "`Python: Current File (Integrated Terminal)`". |
|||
|
|||
Em seguida, ele iniciará o servidor com seu código **FastAPI**, parará em seus pontos de interrupção, etc. |
|||
|
|||
Veja como pode parecer: |
|||
|
|||
<img src="/img/tutorial/debugging/image01.png"> |
|||
|
|||
--- |
|||
|
|||
Se você usar o Pycharm, você pode: |
|||
|
|||
* Abrir o menu "Executar". |
|||
* Selecionar a opção "Depurar...". |
|||
* Então um menu de contexto aparece. |
|||
* Selecionar o arquivo para depurar (neste caso, `main.py`). |
|||
|
|||
Em seguida, ele iniciará o servidor com seu código **FastAPI**, parará em seus pontos de interrupção, etc. |
|||
|
|||
Veja como pode parecer: |
|||
|
|||
<img src="/img/tutorial/debugging/image02.png"> |
@ -0,0 +1,134 @@ |
|||
# Modelos de Formulários |
|||
|
|||
Você pode utilizar **Modelos Pydantic** para declarar **campos de formulários** no FastAPI. |
|||
|
|||
/// info | "Informação" |
|||
|
|||
Para utilizar formulários, instale primeiramente o <a href="https://github.com/Kludex/python-multipart" class="external-link" target="_blank">`python-multipart`</a>. |
|||
|
|||
Certifique-se de criar um [ambiente virtual](../virtual-environments.md){.internal-link target=_blank}, ativá-lo, e então instalar. Por exemplo: |
|||
|
|||
```console |
|||
$ pip install python-multipart |
|||
``` |
|||
|
|||
/// |
|||
|
|||
/// note | "Nota" |
|||
|
|||
Isto é suportado desde a versão `0.113.0` do FastAPI. 🤓 |
|||
|
|||
/// |
|||
|
|||
## Modelos Pydantic para Formulários |
|||
|
|||
Você precisa apenas declarar um **modelo Pydantic** com os campos que deseja receber como **campos de formulários**, e então declarar o parâmetro como um `Form`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9-11 15" |
|||
{!> ../../../docs_src/request_form_models/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="8-10 14" |
|||
{!> ../../../docs_src/request_form_models/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Prefira utilizar a versão `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7-9 13" |
|||
{!> ../../../docs_src/request_form_models/tutorial001.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
O **FastAPI** irá **extrair** as informações para **cada campo** dos **dados do formulário** na requisição e dar para você o modelo Pydantic que você definiu. |
|||
|
|||
## Confira os Documentos |
|||
|
|||
Você pode verificar na UI de documentação em `/docs`: |
|||
|
|||
<div class="screenshot"> |
|||
<img src="/img/tutorial/request-form-models/image01.png"> |
|||
</div> |
|||
|
|||
## Proibir Campos Extras de Formulários |
|||
|
|||
Em alguns casos de uso especiais (provavelmente não muito comum), você pode desejar **restringir** os campos do formulário para aceitar apenas os declarados no modelo Pydantic. E **proibir** qualquer campo **extra**. |
|||
|
|||
/// note | "Nota" |
|||
|
|||
Isso é suportado deste a versão `0.114.0` do FastAPI. 🤓 |
|||
|
|||
/// |
|||
|
|||
Você pode utilizar a configuração de modelo do Pydantic para `proibir` qualquer campo `extra`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="12" |
|||
{!> ../../../docs_src/request_form_models/tutorial002_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="11" |
|||
{!> ../../../docs_src/request_form_models/tutorial002_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip |
|||
|
|||
Prefira utilizar a versão `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="10" |
|||
{!> ../../../docs_src/request_form_models/tutorial002.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Caso um cliente tente enviar informações adicionais, ele receberá um retorno de **erro**. |
|||
|
|||
Por exemplo, se o cliente tentar enviar os campos de formulário: |
|||
|
|||
* `username`: `Rick` |
|||
* `password`: `Portal Gun` |
|||
* `extra`: `Mr. Poopybutthole` |
|||
|
|||
Ele receberá um retorno de erro informando-o que o campo `extra` não é permitido: |
|||
|
|||
```json |
|||
{ |
|||
"detail": [ |
|||
{ |
|||
"type": "extra_forbidden", |
|||
"loc": ["body", "extra"], |
|||
"msg": "Extra inputs are not permitted", |
|||
"input": "Mr. Poopybutthole" |
|||
} |
|||
] |
|||
} |
|||
``` |
|||
|
|||
## Resumo |
|||
|
|||
Você pode utilizar modelos Pydantic para declarar campos de formulários no FastAPI. 😎 |
@ -0,0 +1,418 @@ |
|||
# Arquivos de Requisição |
|||
|
|||
Você pode definir arquivos para serem enviados para o cliente utilizando `File`. |
|||
|
|||
/// info |
|||
|
|||
Para receber arquivos compartilhados, primeiro instale <a href="https://github.com/Kludex/python-multipart" class="external-link" target="_blank">`python-multipart`</a>. |
|||
|
|||
E.g. `pip install python-multipart`. |
|||
|
|||
Isso se deve por que arquivos enviados são enviados como "dados de formulário". |
|||
|
|||
/// |
|||
|
|||
## Importe `File` |
|||
|
|||
Importe `File` e `UploadFile` do `fastapi`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="3" |
|||
{!> ../../../docs_src/request_files/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/request_files/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="1" |
|||
{!> ../../../docs_src/request_files/tutorial001.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
## Defina os parâmetros de `File` |
|||
|
|||
Cria os parâmetros do arquivo da mesma forma que você faria para `Body` ou `Form`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9" |
|||
{!> ../../../docs_src/request_files/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="8" |
|||
{!> ../../../docs_src/request_files/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7" |
|||
{!> ../../../docs_src/request_files/tutorial001.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
/// info | Informação |
|||
|
|||
`File` é uma classe que herda diretamente de `Form`. |
|||
|
|||
Mas lembre-se que quando você importa `Query`,`Path`, `File`, entre outros, do `fastapi`, essas são na verdade funções que retornam classes especiais. |
|||
|
|||
/// |
|||
|
|||
/// tip | Dica |
|||
|
|||
Para declarar o corpo de arquivos, você precisa utilizar `File`, do contrário os parâmetros seriam interpretados como parâmetros de consulta ou corpo (JSON) da requisição. |
|||
|
|||
/// |
|||
|
|||
Os arquivos serão enviados como "form data". |
|||
|
|||
Se você declarar o tipo do seu parâmetro na sua *função de operação de rota* como `bytes`, o **FastAPI** irá ler o arquivo para você e você receberá o conteúdo como `bytes`. |
|||
|
|||
Lembre-se que isso significa que o conteúdo inteiro será armazenado em memória. Isso funciona bem para arquivos pequenos. |
|||
|
|||
Mas existem vários casos em que você pode se beneficiar ao usar `UploadFile`. |
|||
|
|||
## Parâmetros de arquivo com `UploadFile` |
|||
|
|||
Defina um parâmetro de arquivo com o tipo `UploadFile` |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="14" |
|||
{!> ../../../docs_src/request_files/tutorial001_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="13" |
|||
{!> ../../../docs_src/request_files/tutorial001_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="12" |
|||
{!> ../../../docs_src/request_files/tutorial001.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Utilizando `UploadFile` tem várias vantagens sobre `bytes`: |
|||
|
|||
* Você não precisa utilizar `File()` como o valor padrão do parâmetro. |
|||
* A classe utiliza um arquivo em "spool": |
|||
* Um arquivo guardado em memória até um tamanho máximo, depois desse limite ele é guardado em disco. |
|||
* Isso significa que a classe funciona bem com arquivos grandes como imagens, vídeos, binários extensos, etc. Sem consumir toda a memória. |
|||
* Você pode obter metadados do arquivo enviado. |
|||
* Ela possui uma interface <a href="https://docs.python.org/3/glossary.html#term-file-like-object" class="external-link" target="_blank">semelhante a arquivos</a> `async`. |
|||
* Ela expõe um objeto python <a href="https://docs.python.org/3/library/tempfile.html#tempfile.SpooledTemporaryFile" class="external-link" target="_blank">`SpooledTemporaryFile`</a> que você pode repassar para bibliotecas que esperam um objeto com comportamento de arquivo. |
|||
|
|||
### `UploadFile` |
|||
|
|||
`UploadFile` tem os seguintes atributos: |
|||
|
|||
* `filename`: Uma string (`str`) com o nome original do arquivo enviado (e.g. `myimage.jpg`). |
|||
* `content-type`: Uma `str` com o tipo do conteúdo (tipo MIME / media) (e.g. `image/jpeg`). |
|||
* `file`: Um objeto do tipo <a href="https://docs.python.org/3/library/tempfile.html#tempfile.SpooledTemporaryFile" class="external-link" target="_blank">`SpooledTemporaryFile`</a> (um objeto <a href="https://docs.python.org/3/glossary.html#term-file-like-object" class="external-link" target="_blank">file-like</a>). O arquivo propriamente dito que você pode passar diretamente para outras funções ou bibliotecas que esperam um objeto "file-like". |
|||
|
|||
`UploadFile` tem os seguintes métodos `async`. Todos eles chamam os métodos de arquivos por baixo dos panos (usando o objeto `SpooledTemporaryFile` interno). |
|||
|
|||
* `write(data)`: escreve dados (`data`) em `str` ou `bytes` no arquivo. |
|||
* `read(size)`: Lê um número de bytes/caracteres de acordo com a quantidade `size` (`int`). |
|||
* `seek(offset)`: Navega para o byte na posição `offset` (`int`) do arquivo. |
|||
* E.g., `await myfile.seek(0)` navegaria para o ínicio do arquivo. |
|||
* Isso é especialmente útil se você executar `await myfile.read()` uma vez e depois precisar ler os conteúdos do arquivo de novo. |
|||
* `close()`: Fecha o arquivo. |
|||
|
|||
Como todos esses métodos são assíncronos (`async`) você precisa esperar ("await") por eles. |
|||
|
|||
Por exemplo, dentro de uma *função de operação de rota* assíncrona você pode obter os conteúdos com: |
|||
|
|||
```Python |
|||
contents = await myfile.read() |
|||
``` |
|||
|
|||
Se você estiver dentro de uma *função de operação de rota* definida normalmente com `def`, você pode acessar `UploadFile.file` diretamente, por exemplo: |
|||
|
|||
```Python |
|||
contents = myfile.file.read() |
|||
``` |
|||
|
|||
/// note | Detalhes técnicos do `async` |
|||
|
|||
Quando você utiliza métodos assíncronos, o **FastAPI** executa os métodos do arquivo em uma threadpool e espera por eles. |
|||
|
|||
/// |
|||
|
|||
/// note | Detalhes técnicos do Starlette |
|||
|
|||
O `UploadFile` do **FastAPI** herda diretamente do `UploadFile` do **Starlette**, mas adiciona algumas funcionalidades necessárias para ser compatível com o **Pydantic** |
|||
|
|||
/// |
|||
|
|||
## O que é "Form Data" |
|||
|
|||
A forma como formulários HTML(`<form></form>`) enviam dados para o servidor normalmente utilizam uma codificação "especial" para esses dados, que é diferente do JSON. |
|||
|
|||
O **FastAPI** garante que os dados serão lidos da forma correta, em vez do JSON. |
|||
|
|||
/// note | Detalhes Técnicos |
|||
|
|||
Dados vindos de formulários geralmente tem a codificação com o "media type" `application/x-www-form-urlencoded` quando estes não incluem arquivos. |
|||
|
|||
Mas quando os dados incluem arquivos, eles são codificados como `multipart/form-data`. Se você utilizar `File`, **FastAPI** saberá que deve receber os arquivos da parte correta do corpo da requisição. |
|||
|
|||
Se você quer ler mais sobre essas codificações e campos de formulário, veja a documentação online da <a href="https://developer.mozilla.org/en-US/docs/Web/HTTP/Methods/POST" class="external-link" target="_blank"><abbr title="Mozilla Developer Network">MDN</abbr> sobre <code> POST</code> </a>. |
|||
|
|||
/// |
|||
|
|||
/// warning | Aviso |
|||
|
|||
Você pode declarar múltiplos parâmetros `File` e `Form` em uma *operação de rota*, mas você não pode declarar campos `Body`que seriam recebidos como JSON junto desses parâmetros, por que a codificação do corpo da requisição será `multipart/form-data` em vez de `application/json`. |
|||
|
|||
Isso não é uma limitação do **FastAPI**, é uma parte do protocolo HTTP. |
|||
|
|||
/// |
|||
|
|||
## Arquivo de upload opcional |
|||
|
|||
Você pode definir um arquivo como opcional utilizando as anotações de tipo padrão e definindo o valor padrão como `None`: |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python hl_lines="9 17" |
|||
{!> ../../../docs_src/request_files/tutorial001_02_an_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9 17" |
|||
{!> ../../../docs_src/request_files/tutorial001_02_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="10 18" |
|||
{!> ../../../docs_src/request_files/tutorial001_02_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.10+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated`, se possível |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7 15" |
|||
{!> ../../../docs_src/request_files/tutorial001_02_py310.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated`, se possível |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="9 17" |
|||
{!> ../../../docs_src/request_files/tutorial001_02.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
## `UploadFile` com Metadados Adicionais |
|||
|
|||
Você também pode utilizar `File()` com `UploadFile`, por exemplo, para definir metadados adicionais: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="9 15" |
|||
{!> ../../../docs_src/request_files/tutorial001_03_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="8 14" |
|||
{!> ../../../docs_src/request_files/tutorial001_03_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="7 13" |
|||
{!> ../../../docs_src/request_files/tutorial001_03.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
## Envio de Múltiplos Arquivos |
|||
|
|||
É possível enviar múltiplos arquivos ao mesmo tmepo. |
|||
|
|||
Ele ficam associados ao mesmo "campo do formulário" enviado com "form data". |
|||
|
|||
Para usar isso, declare uma lista de `bytes` ou `UploadFile`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="10 15" |
|||
{!> ../../../docs_src/request_files/tutorial002_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="11 16" |
|||
{!> ../../../docs_src/request_files/tutorial002_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="8 13" |
|||
{!> ../../../docs_src/request_files/tutorial002_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="10 15" |
|||
{!> ../../../docs_src/request_files/tutorial002.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
Você irá receber, como delcarado uma lista (`list`) de `bytes` ou `UploadFile`s, |
|||
|
|||
/// note | Detalhes Técnicos |
|||
|
|||
Você também poderia utilizar `from starlette.responses import HTMLResponse`. |
|||
|
|||
O **FastAPI** fornece as mesmas `starlette.responses` como `fastapi.responses` apenas como um facilitador para você, desenvolvedor. Mas a maior parte das respostas vem diretamente do Starlette. |
|||
|
|||
/// |
|||
|
|||
### Enviando Múltiplos Arquivos com Metadados Adicionais |
|||
|
|||
E da mesma forma que antes, você pode utilizar `File()` para definir parâmetros adicionais, até mesmo para `UploadFile`: |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python hl_lines="11 18-20" |
|||
{!> ../../../docs_src/request_files/tutorial003_an_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python hl_lines="12 19-21" |
|||
{!> ../../../docs_src/request_files/tutorial003_an.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="9 16" |
|||
{!> ../../../docs_src/request_files/tutorial003_py39.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | Dica |
|||
|
|||
Utilize a versão com `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python hl_lines="11 18" |
|||
{!> ../../../docs_src/request_files/tutorial003.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
## Recapitulando |
|||
|
|||
Use `File`, `bytes` e `UploadFile` para declarar arquivos que serão enviados na requisição, enviados como dados do formulário. |
@ -0,0 +1,249 @@ |
|||
# Testando |
|||
|
|||
Graças ao <a href="https://www.starlette.io/testclient/" class="external-link" target="_blank">Starlette</a>, testar aplicativos **FastAPI** é fácil e agradável. |
|||
|
|||
Ele é baseado no <a href="https://www.python-httpx.org" class="external-link" target="_blank">HTTPX</a>, que por sua vez é projetado com base em Requests, por isso é muito familiar e intuitivo. |
|||
|
|||
Com ele, você pode usar o <a href="https://docs.pytest.org/" class="external-link" target="_blank">pytest</a> diretamente com **FastAPI**. |
|||
|
|||
## Usando `TestClient` |
|||
|
|||
/// info | "Informação" |
|||
|
|||
Para usar o `TestClient`, primeiro instale o <a href="https://www.python-httpx.org" class="external-link" target="_blank">`httpx`</a>. |
|||
|
|||
Certifique-se de criar um [ambiente virtual](../virtual-environments.md){.internal-link target=_blank}, ativá-lo e instalá-lo, por exemplo: |
|||
|
|||
```console |
|||
$ pip install httpx |
|||
``` |
|||
|
|||
/// |
|||
|
|||
Importe `TestClient`. |
|||
|
|||
Crie um `TestClient` passando seu aplicativo **FastAPI** para ele. |
|||
|
|||
Crie funções com um nome que comece com `test_` (essa é a convenção padrão do `pytest`). |
|||
|
|||
Use o objeto `TestClient` da mesma forma que você faz com `httpx`. |
|||
|
|||
Escreva instruções `assert` simples com as expressões Python padrão que você precisa verificar (novamente, `pytest` padrão). |
|||
|
|||
```Python hl_lines="2 12 15-18" |
|||
{!../../../docs_src/app_testing/tutorial001.py!} |
|||
``` |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Observe que as funções de teste são `def` normais, não `async def`. |
|||
|
|||
E as chamadas para o cliente também são chamadas normais, não usando `await`. |
|||
|
|||
Isso permite que você use `pytest` diretamente sem complicações. |
|||
|
|||
/// |
|||
|
|||
/// note | "Detalhes técnicos" |
|||
|
|||
Você também pode usar `from starlette.testclient import TestClient`. |
|||
|
|||
**FastAPI** fornece o mesmo `starlette.testclient` que `fastapi.testclient` apenas como uma conveniência para você, o desenvolvedor. Mas ele vem diretamente da Starlette. |
|||
|
|||
/// |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Se você quiser chamar funções `async` em seus testes além de enviar solicitações ao seu aplicativo FastAPI (por exemplo, funções de banco de dados assíncronas), dê uma olhada em [Testes assíncronos](../advanced/async-tests.md){.internal-link target=_blank} no tutorial avançado. |
|||
|
|||
/// |
|||
|
|||
## Separando testes |
|||
|
|||
Em uma aplicação real, você provavelmente teria seus testes em um arquivo diferente. |
|||
|
|||
E seu aplicativo **FastAPI** também pode ser composto de vários arquivos/módulos, etc. |
|||
|
|||
### Arquivo do aplicativo **FastAPI** |
|||
|
|||
Digamos que você tenha uma estrutura de arquivo conforme descrito em [Aplicativos maiores](bigger-applications.md){.internal-link target=_blank}: |
|||
|
|||
``` |
|||
. |
|||
├── app |
|||
│ ├── __init__.py |
|||
│ └── main.py |
|||
``` |
|||
|
|||
No arquivo `main.py` você tem seu aplicativo **FastAPI**: |
|||
|
|||
|
|||
```Python |
|||
{!../../../docs_src/app_testing/main.py!} |
|||
``` |
|||
|
|||
### Arquivo de teste |
|||
|
|||
Então você poderia ter um arquivo `test_main.py` com seus testes. Ele poderia estar no mesmo pacote Python (o mesmo diretório com um arquivo `__init__.py`): |
|||
|
|||
``` hl_lines="5" |
|||
. |
|||
├── app |
|||
│ ├── __init__.py |
|||
│ ├── main.py |
|||
│ └── test_main.py |
|||
``` |
|||
|
|||
Como esse arquivo está no mesmo pacote, você pode usar importações relativas para importar o objeto `app` do módulo `main` (`main.py`): |
|||
|
|||
```Python hl_lines="3" |
|||
{!../../../docs_src/app_testing/test_main.py!} |
|||
``` |
|||
|
|||
...e ter o código para os testes como antes. |
|||
|
|||
## Testando: exemplo estendido |
|||
|
|||
Agora vamos estender este exemplo e adicionar mais detalhes para ver como testar diferentes partes. |
|||
|
|||
### Arquivo de aplicativo **FastAPI** estendido |
|||
|
|||
Vamos continuar com a mesma estrutura de arquivo de antes: |
|||
|
|||
``` |
|||
. |
|||
├── app |
|||
│ ├── __init__.py |
|||
│ ├── main.py |
|||
│ └── test_main.py |
|||
``` |
|||
|
|||
Digamos que agora o arquivo `main.py` com seu aplicativo **FastAPI** tenha algumas outras **operações de rotas**. |
|||
|
|||
Ele tem uma operação `GET` que pode retornar um erro. |
|||
|
|||
Ele tem uma operação `POST` que pode retornar vários erros. |
|||
|
|||
Ambas as *operações de rotas* requerem um cabeçalho `X-Token`. |
|||
|
|||
//// tab | Python 3.10+ |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/app_testing/app_b_an_py310/main.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.9+ |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/app_testing/app_b_an_py39/main.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/app_testing/app_b_an/main.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.10+ non-Annotated |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Prefira usar a versão `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/app_testing/app_b_py310/main.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
//// tab | Python 3.8+ non-Annotated |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Prefira usar a versão `Annotated` se possível. |
|||
|
|||
/// |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/app_testing/app_b/main.py!} |
|||
``` |
|||
|
|||
//// |
|||
|
|||
### Arquivo de teste estendido |
|||
|
|||
Você pode então atualizar `test_main.py` com os testes estendidos: |
|||
|
|||
```Python |
|||
{!> ../../../docs_src/app_testing/app_b/test_main.py!} |
|||
``` |
|||
|
|||
Sempre que você precisar que o cliente passe informações na requisição e não souber como, você pode pesquisar (no Google) como fazer isso no `httpx`, ou até mesmo como fazer isso com `requests`, já que o design do HTTPX é baseado no design do Requests. |
|||
|
|||
Depois é só fazer o mesmo nos seus testes. |
|||
|
|||
Por exemplo: |
|||
|
|||
* Para passar um parâmetro *path* ou *query*, adicione-o à própria URL. |
|||
* Para passar um corpo JSON, passe um objeto Python (por exemplo, um `dict`) para o parâmetro `json`. |
|||
* Se você precisar enviar *Dados de Formulário* em vez de JSON, use o parâmetro `data`. |
|||
* Para passar *headers*, use um `dict` no parâmetro `headers`. |
|||
* Para *cookies*, um `dict` no parâmetro `cookies`. |
|||
|
|||
Para mais informações sobre como passar dados para o backend (usando `httpx` ou `TestClient`), consulte a <a href="https://www.python-httpx.org" class="external-link" target="_blank">documentação do HTTPX</a>. |
|||
|
|||
/// info | "Informação" |
|||
|
|||
Observe que o `TestClient` recebe dados que podem ser convertidos para JSON, não para modelos Pydantic. |
|||
|
|||
Se você tiver um modelo Pydantic em seu teste e quiser enviar seus dados para o aplicativo durante o teste, poderá usar o `jsonable_encoder` descrito em [Codificador compatível com JSON](encoder.md){.internal-link target=_blank}. |
|||
|
|||
/// |
|||
|
|||
## Execute-o |
|||
|
|||
Depois disso, você só precisa instalar o `pytest`. |
|||
|
|||
Certifique-se de criar um [ambiente virtual](../virtual-environments.md){.internal-link target=_blank}, ativá-lo e instalá-lo, por exemplo: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install pytest |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
Ele detectará os arquivos e os testes automaticamente, os executará e informará os resultados para você. |
|||
|
|||
Execute os testes com: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pytest |
|||
|
|||
================ test session starts ================ |
|||
platform linux -- Python 3.6.9, pytest-5.3.5, py-1.8.1, pluggy-0.13.1 |
|||
rootdir: /home/user/code/superawesome-cli/app |
|||
plugins: forked-1.1.3, xdist-1.31.0, cov-2.8.1 |
|||
collected 6 items |
|||
|
|||
---> 100% |
|||
|
|||
test_main.py <span style="color: green; white-space: pre;">...... [100%]</span> |
|||
|
|||
<span style="color: green;">================= 1 passed in 0.03s =================</span> |
|||
``` |
|||
|
|||
</div> |
@ -0,0 +1,844 @@ |
|||
# Ambientes Virtuais |
|||
|
|||
Ao trabalhar em projetos Python, você provavelmente deve usar um **ambiente virtual** (ou um mecanismo similar) para isolar os pacotes que você instala para cada projeto. |
|||
|
|||
/// info | "Informação" |
|||
|
|||
Se você já sabe sobre ambientes virtuais, como criá-los e usá-los, talvez seja melhor pular esta seção. 🤓 |
|||
|
|||
/// |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Um **ambiente virtual** é diferente de uma **variável de ambiente**. |
|||
|
|||
Uma **variável de ambiente** é uma variável no sistema que pode ser usada por programas. |
|||
|
|||
Um **ambiente virtual** é um diretório com alguns arquivos. |
|||
|
|||
/// |
|||
|
|||
/// info | "Informação" |
|||
|
|||
Esta página lhe ensinará como usar **ambientes virtuais** e como eles funcionam. |
|||
|
|||
Se você estiver pronto para adotar uma **ferramenta que gerencia tudo** para você (incluindo a instalação do Python), experimente <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">uv</a>. |
|||
|
|||
/// |
|||
|
|||
## Criar um Projeto |
|||
|
|||
Primeiro, crie um diretório para seu projeto. |
|||
|
|||
O que normalmente faço é criar um diretório chamado `code` dentro do meu diretório home/user. |
|||
|
|||
E dentro disso eu crio um diretório por projeto. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Vá para o diretório inicial |
|||
$ cd |
|||
// Crie um diretório para todos os seus projetos de código |
|||
$ mkdir code |
|||
// Entre nesse diretório de código |
|||
$ cd code |
|||
// Crie um diretório para este projeto |
|||
$ mkdir awesome-project |
|||
// Entre no diretório do projeto |
|||
$ cd awesome-project |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Crie um ambiente virtual |
|||
|
|||
Ao começar a trabalhar em um projeto Python **pela primeira vez**, crie um ambiente virtual **<abbr title="existem outras opções, esta é uma diretriz simples">dentro do seu projeto</abbr>**. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Você só precisa fazer isso **uma vez por projeto**, não toda vez que trabalhar. |
|||
|
|||
/// |
|||
|
|||
//// tab | `venv` |
|||
|
|||
Para criar um ambiente virtual, você pode usar o módulo `venv` que vem com o Python. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python -m venv .venv |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// details | O que esse comando significa |
|||
|
|||
* `python`: usa o programa chamado `python` |
|||
* `-m`: chama um módulo como um script, nós diremos a ele qual módulo vem em seguida |
|||
* `venv`: usa o módulo chamado `venv` que normalmente vem instalado com o Python |
|||
* `.venv`: cria o ambiente virtual no novo diretório `.venv` |
|||
|
|||
/// |
|||
|
|||
//// |
|||
|
|||
//// tab | `uv` |
|||
|
|||
Se você tiver o <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a> instalado, poderá usá-lo para criar um ambiente virtual. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uv venv |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Por padrão, `uv` criará um ambiente virtual em um diretório chamado `.venv`. |
|||
|
|||
Mas você pode personalizá-lo passando um argumento adicional com o nome do diretório. |
|||
|
|||
/// |
|||
|
|||
//// |
|||
|
|||
Esse comando cria um novo ambiente virtual em um diretório chamado `.venv`. |
|||
|
|||
/// details | `.venv` ou outro nome |
|||
|
|||
Você pode criar o ambiente virtual em um diretório diferente, mas há uma convenção para chamá-lo de `.venv`. |
|||
|
|||
/// |
|||
|
|||
## Ative o ambiente virtual |
|||
|
|||
Ative o novo ambiente virtual para que qualquer comando Python que você executar ou pacote que você instalar o utilize. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Faça isso **toda vez** que iniciar uma **nova sessão de terminal** para trabalhar no projeto. |
|||
|
|||
/// |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/bin/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ .venv\Scripts\Activate.ps1 |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows Bash |
|||
|
|||
Ou se você usa o Bash para Windows (por exemplo, <a href="https://gitforwindows.org/" class="external-link" target="_blank">Git Bash</a>): |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/Scripts/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Toda vez que você instalar um **novo pacote** naquele ambiente, **ative** o ambiente novamente. |
|||
|
|||
Isso garante que, se você usar um **programa de terminal (<abbr title="interface de linha de comando">CLI</abbr>)** instalado por esse pacote, você usará aquele do seu ambiente virtual e não qualquer outro que possa ser instalado globalmente, provavelmente com uma versão diferente do que você precisa. |
|||
|
|||
/// |
|||
|
|||
## Verifique se o ambiente virtual está ativo |
|||
|
|||
Verifique se o ambiente virtual está ativo (o comando anterior funcionou). |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Isso é **opcional**, mas é uma boa maneira de **verificar** se tudo está funcionando conforme o esperado e se você está usando o ambiente virtual pretendido. |
|||
|
|||
/// |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ which python |
|||
|
|||
/home/user/code/awesome-project/.venv/bin/python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
Se ele mostrar o binário `python` em `.venv/bin/python`, dentro do seu projeto (neste caso `awesome-project`), então funcionou. 🎉 |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ Get-Command python |
|||
|
|||
C:\Users\user\code\awesome-project\.venv\Scripts\python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
Se ele mostrar o binário `python` em `.venv\Scripts\python`, dentro do seu projeto (neste caso `awesome-project`), então funcionou. 🎉 |
|||
|
|||
//// |
|||
|
|||
## Atualizar `pip` |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Se você usar <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a>, você o usará para instalar coisas em vez do `pip`, então não precisará atualizar o `pip`. 😎 |
|||
|
|||
/// |
|||
|
|||
Se você estiver usando `pip` para instalar pacotes (ele vem por padrão com o Python), você deve **atualizá-lo** para a versão mais recente. |
|||
|
|||
Muitos erros exóticos durante a instalação de um pacote são resolvidos apenas atualizando o `pip` primeiro. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Normalmente, você faria isso **uma vez**, logo após criar o ambiente virtual. |
|||
|
|||
/// |
|||
|
|||
Certifique-se de que o ambiente virtual esteja ativo (com o comando acima) e execute: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python -m pip install --upgrade pip |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Adicionar `.gitignore` |
|||
|
|||
Se você estiver usando **Git** (você deveria), adicione um arquivo `.gitignore` para excluir tudo em seu `.venv` do Git. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Se você usou <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a> para criar o ambiente virtual, ele já fez isso para você, você pode pular esta etapa. 😎 |
|||
|
|||
/// |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Faça isso **uma vez**, logo após criar o ambiente virtual. |
|||
|
|||
/// |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ echo "*" > .venv/.gitignore |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
/// details | O que esse comando significa |
|||
|
|||
* `echo "*"`: irá "imprimir" o texto `*` no terminal (a próxima parte muda isso um pouco) |
|||
* `>`: qualquer coisa impressa no terminal pelo comando à esquerda de `>` não deve ser impressa, mas sim escrita no arquivo que vai à direita de `>` |
|||
* `.gitignore`: o nome do arquivo onde o texto deve ser escrito |
|||
|
|||
E `*` para Git significa "tudo". Então, ele ignorará tudo no diretório `.venv`. |
|||
|
|||
Esse comando criará um arquivo `.gitignore` com o conteúdo: |
|||
|
|||
```gitignore |
|||
* |
|||
``` |
|||
|
|||
/// |
|||
|
|||
## Instalar Pacotes |
|||
|
|||
Após ativar o ambiente, você pode instalar pacotes nele. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Faça isso **uma vez** ao instalar ou atualizar os pacotes que seu projeto precisa. |
|||
|
|||
Se precisar atualizar uma versão ou adicionar um novo pacote, você **fará isso novamente**. |
|||
|
|||
/// |
|||
|
|||
### Instalar pacotes diretamente |
|||
|
|||
Se estiver com pressa e não quiser usar um arquivo para declarar os requisitos de pacote do seu projeto, você pode instalá-los diretamente. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
É uma (muito) boa ideia colocar os pacotes e versões que seu programa precisa em um arquivo (por exemplo `requirements.txt` ou `pyproject.toml`). |
|||
|
|||
/// |
|||
|
|||
//// tab | `pip` |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "fastapi[standard]" |
|||
|
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | `uv` |
|||
|
|||
Se você tem o <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a>: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uv pip install "fastapi[standard]" |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
### Instalar a partir de `requirements.txt` |
|||
|
|||
Se você tiver um `requirements.txt`, agora poderá usá-lo para instalar seus pacotes. |
|||
|
|||
//// tab | `pip` |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install -r requirements.txt |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | `uv` |
|||
|
|||
Se você tem o <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">`uv`</a>: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ uv pip install -r requirements.txt |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
/// details | `requirements.txt` |
|||
|
|||
Um `requirements.txt` com alguns pacotes poderia se parecer com: |
|||
|
|||
```requirements.txt |
|||
fastapi[standard]==0.113.0 |
|||
pydantic==2.8.0 |
|||
``` |
|||
|
|||
/// |
|||
|
|||
## Execute seu programa |
|||
|
|||
Depois de ativar o ambiente virtual, você pode executar seu programa, e ele usará o Python dentro do seu ambiente virtual com os pacotes que você instalou lá. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ python main.py |
|||
|
|||
Hello World |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Configure seu editor |
|||
|
|||
Você provavelmente usaria um editor. Certifique-se de configurá-lo para usar o mesmo ambiente virtual que você criou (ele provavelmente o detectará automaticamente) para que você possa obter erros de preenchimento automático e em linha. |
|||
|
|||
Por exemplo: |
|||
|
|||
* <a href="https://code.visualstudio.com/docs/python/environments#_select-and-activate-an-environment" class="external-link" target="_blank">VS Code</a> |
|||
* <a href="https://www.jetbrains.com/help/pycharm/creating-virtual-environment.html" class="external-link" target="_blank">PyCharm</a> |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Normalmente, você só precisa fazer isso **uma vez**, ao criar o ambiente virtual. |
|||
|
|||
/// |
|||
|
|||
## Desativar o ambiente virtual |
|||
|
|||
Quando terminar de trabalhar no seu projeto, você pode **desativar** o ambiente virtual. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ deactivate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
Dessa forma, quando você executar `python`, ele não tentará executá-lo naquele ambiente virtual com os pacotes instalados nele. |
|||
|
|||
## Pronto para trabalhar |
|||
|
|||
Agora você está pronto para começar a trabalhar no seu projeto. |
|||
|
|||
|
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Você quer entender o que é tudo isso acima? |
|||
|
|||
Continue lendo. 👇🤓 |
|||
|
|||
/// |
|||
|
|||
## Por que ambientes virtuais |
|||
|
|||
Para trabalhar com o FastAPI, você precisa instalar o <a href="https://www.python.org/" class="external-link" target="_blank">Python</a>. |
|||
|
|||
Depois disso, você precisará **instalar** o FastAPI e quaisquer outros **pacotes** que queira usar. |
|||
|
|||
Para instalar pacotes, você normalmente usaria o comando `pip` que vem com o Python (ou alternativas semelhantes). |
|||
|
|||
No entanto, se você usar `pip` diretamente, os pacotes serão instalados no seu **ambiente Python global** (a instalação global do Python). |
|||
|
|||
### O Problema |
|||
|
|||
Então, qual é o problema em instalar pacotes no ambiente global do Python? |
|||
|
|||
Em algum momento, você provavelmente acabará escrevendo muitos programas diferentes que dependem de **pacotes diferentes**. E alguns desses projetos em que você trabalha dependerão de **versões diferentes** do mesmo pacote. 😱 |
|||
|
|||
Por exemplo, você pode criar um projeto chamado `philosophers-stone`, este programa depende de outro pacote chamado **`harry`, usando a versão `1`**. Então, você precisa instalar `harry`. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
stone(philosophers-stone) -->|requires| harry-1[harry v1] |
|||
``` |
|||
|
|||
Então, em algum momento depois, você cria outro projeto chamado `prisoner-of-azkaban`, e esse projeto também depende de `harry`, mas esse projeto precisa do **`harry` versão `3`**. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
azkaban(prisoner-of-azkaban) --> |requires| harry-3[harry v3] |
|||
``` |
|||
|
|||
Mas agora o problema é que, se você instalar os pacotes globalmente (no ambiente global) em vez de em um **ambiente virtual** local, você terá que escolher qual versão do `harry` instalar. |
|||
|
|||
Se você quiser executar `philosophers-stone`, precisará primeiro instalar `harry` versão `1`, por exemplo com: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "harry==1" |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
E então você acabaria com `harry` versão `1` instalado em seu ambiente Python global. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
subgraph global[global env] |
|||
harry-1[harry v1] |
|||
end |
|||
subgraph stone-project[philosophers-stone project] |
|||
stone(philosophers-stone) -->|requires| harry-1 |
|||
end |
|||
``` |
|||
|
|||
Mas se você quiser executar `prisoner-of-azkaban`, você precisará desinstalar `harry` versão `1` e instalar `harry` versão `3` (ou apenas instalar a versão `3` desinstalaria automaticamente a versão `1`). |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ pip install "harry==3" |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
E então você acabaria com `harry` versão `3` instalado em seu ambiente Python global. |
|||
|
|||
E se você tentar executar `philosophers-stone` novamente, há uma chance de que **não funcione** porque ele precisa de `harry` versão `1`. |
|||
|
|||
```mermaid |
|||
flowchart LR |
|||
subgraph global[global env] |
|||
harry-1[<strike>harry v1</strike>] |
|||
style harry-1 fill:#ccc,stroke-dasharray: 5 5 |
|||
harry-3[harry v3] |
|||
end |
|||
subgraph stone-project[philosophers-stone project] |
|||
stone(philosophers-stone) -.-x|⛔️| harry-1 |
|||
end |
|||
subgraph azkaban-project[prisoner-of-azkaban project] |
|||
azkaban(prisoner-of-azkaban) --> |requires| harry-3 |
|||
end |
|||
``` |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
É muito comum em pacotes Python tentar ao máximo **evitar alterações drásticas** em **novas versões**, mas é melhor prevenir do que remediar e instalar versões mais recentes intencionalmente e, quando possível, executar os testes para verificar se tudo está funcionando corretamente. |
|||
|
|||
/// |
|||
|
|||
Agora, imagine isso com **muitos** outros **pacotes** dos quais todos os seus **projetos dependem**. Isso é muito difícil de gerenciar. E você provavelmente acabaria executando alguns projetos com algumas **versões incompatíveis** dos pacotes, e não saberia por que algo não está funcionando. |
|||
|
|||
Além disso, dependendo do seu sistema operacional (por exemplo, Linux, Windows, macOS), ele pode ter vindo com o Python já instalado. E, nesse caso, provavelmente tinha alguns pacotes pré-instalados com algumas versões específicas **necessárias para o seu sistema**. Se você instalar pacotes no ambiente global do Python, poderá acabar **quebrando** alguns dos programas que vieram com seu sistema operacional. |
|||
|
|||
## Onde os pacotes são instalados |
|||
|
|||
Quando você instala o Python, ele cria alguns diretórios com alguns arquivos no seu computador. |
|||
|
|||
Alguns desses diretórios são os responsáveis por ter todos os pacotes que você instala. |
|||
|
|||
Quando você executa: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
// Não execute isso agora, é apenas um exemplo 🤓 |
|||
$ pip install "fastapi[standard]" |
|||
---> 100% |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
Isso fará o download de um arquivo compactado com o código FastAPI, normalmente do <a href="https://pypi.org/project/fastapi/" class="external-link" target="_blank">PyPI</a>. |
|||
|
|||
Ele também fará o **download** de arquivos para outros pacotes dos quais o FastAPI depende. |
|||
|
|||
Em seguida, ele **extrairá** todos esses arquivos e os colocará em um diretório no seu computador. |
|||
|
|||
Por padrão, ele colocará os arquivos baixados e extraídos no diretório que vem com a instalação do Python, que é o **ambiente global**. |
|||
|
|||
## O que são ambientes virtuais |
|||
|
|||
A solução para os problemas de ter todos os pacotes no ambiente global é usar um **ambiente virtual para cada projeto** em que você trabalha. |
|||
|
|||
Um ambiente virtual é um **diretório**, muito semelhante ao global, onde você pode instalar os pacotes para um projeto. |
|||
|
|||
Dessa forma, cada projeto terá seu próprio ambiente virtual (diretório `.venv`) com seus próprios pacotes. |
|||
|
|||
```mermaid |
|||
flowchart TB |
|||
subgraph stone-project[philosophers-stone project] |
|||
stone(philosophers-stone) --->|requires| harry-1 |
|||
subgraph venv1[.venv] |
|||
harry-1[harry v1] |
|||
end |
|||
end |
|||
subgraph azkaban-project[prisoner-of-azkaban project] |
|||
azkaban(prisoner-of-azkaban) --->|requires| harry-3 |
|||
subgraph venv2[.venv] |
|||
harry-3[harry v3] |
|||
end |
|||
end |
|||
stone-project ~~~ azkaban-project |
|||
``` |
|||
|
|||
## O que significa ativar um ambiente virtual |
|||
|
|||
Quando você ativa um ambiente virtual, por exemplo com: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/bin/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ .venv\Scripts\Activate.ps1 |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows Bash |
|||
|
|||
Ou se você usa o Bash para Windows (por exemplo, <a href="https://gitforwindows.org/" class="external-link" target="_blank">Git Bash</a>): |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ source .venv/Scripts/activate |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
Esse comando criará ou modificará algumas [variáveis de ambiente](environment-variables.md){.internal-link target=_blank} que estarão disponíveis para os próximos comandos. |
|||
|
|||
Uma dessas variáveis é a variável `PATH`. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
Você pode aprender mais sobre a variável de ambiente `PATH` na seção [Variáveis de ambiente](environment-variables.md#path-environment-variable){.internal-link target=_blank}. |
|||
|
|||
/// |
|||
|
|||
A ativação de um ambiente virtual adiciona seu caminho `.venv/bin` (no Linux e macOS) ou `.venv\Scripts` (no Windows) à variável de ambiente `PATH`. |
|||
|
|||
Digamos que antes de ativar o ambiente, a variável `PATH` estava assim: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
```plaintext |
|||
/usr/bin:/bin:/usr/sbin:/sbin |
|||
``` |
|||
|
|||
Isso significa que o sistema procuraria programas em: |
|||
|
|||
* `/usr/bin` |
|||
* `/bin` |
|||
* `/usr/sbin` |
|||
* `/sbin` |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
```plaintext |
|||
C:\Windows\System32 |
|||
``` |
|||
|
|||
Isso significa que o sistema procuraria programas em: |
|||
|
|||
* `C:\Windows\System32` |
|||
|
|||
//// |
|||
|
|||
Após ativar o ambiente virtual, a variável `PATH` ficaria mais ou menos assim: |
|||
|
|||
//// tab | Linux, macOS |
|||
|
|||
```plaintext |
|||
/home/user/code/awesome-project/.venv/bin:/usr/bin:/bin:/usr/sbin:/sbin |
|||
``` |
|||
|
|||
Isso significa que o sistema agora começará a procurar primeiro por programas em: |
|||
|
|||
```plaintext |
|||
/home/user/code/awesome-project/.venv/bin |
|||
``` |
|||
|
|||
antes de procurar nos outros diretórios. |
|||
|
|||
Então, quando você digita `python` no terminal, o sistema encontrará o programa Python em |
|||
|
|||
```plaintext |
|||
/home/user/code/awesome-project/.venv/bin/python |
|||
``` |
|||
|
|||
e usa esse. |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows |
|||
|
|||
```plaintext |
|||
C:\Users\user\code\awesome-project\.venv\Scripts;C:\Windows\System32 |
|||
``` |
|||
|
|||
Isso significa que o sistema agora começará a procurar primeiro por programas em: |
|||
|
|||
```plaintext |
|||
C:\Users\user\code\awesome-project\.venv\Scripts |
|||
``` |
|||
|
|||
antes de procurar nos outros diretórios. |
|||
|
|||
Então, quando você digita `python` no terminal, o sistema encontrará o programa Python em |
|||
|
|||
```plaintext |
|||
C:\Users\user\code\awesome-project\.venv\Scripts\python |
|||
``` |
|||
|
|||
e usa esse. |
|||
|
|||
//// |
|||
|
|||
Um detalhe importante é que ele colocará o caminho do ambiente virtual no **início** da variável `PATH`. O sistema o encontrará **antes** de encontrar qualquer outro Python disponível. Dessa forma, quando você executar `python`, ele usará o Python **do ambiente virtual** em vez de qualquer outro `python` (por exemplo, um `python` de um ambiente global). |
|||
|
|||
Ativar um ambiente virtual também muda algumas outras coisas, mas esta é uma das mais importantes. |
|||
|
|||
## Verificando um ambiente virtual |
|||
|
|||
Ao verificar se um ambiente virtual está ativo, por exemplo com: |
|||
|
|||
//// tab | Linux, macOS, Windows Bash |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ which python |
|||
|
|||
/home/user/code/awesome-project/.venv/bin/python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
//// tab | Windows PowerShell |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ Get-Command python |
|||
|
|||
C:\Users\user\code\awesome-project\.venv\Scripts\python |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
//// |
|||
|
|||
Isso significa que o programa `python` que será usado é aquele **no ambiente virtual**. |
|||
|
|||
você usa `which` no Linux e macOS e `Get-Command` no Windows PowerShell. |
|||
|
|||
A maneira como esse comando funciona é que ele vai e verifica na variável de ambiente `PATH`, passando por **cada caminho em ordem**, procurando pelo programa chamado `python`. Uma vez que ele o encontre, ele **mostrará o caminho** para esse programa. |
|||
|
|||
A parte mais importante é que quando você chama ``python`, esse é exatamente o "`python`" que será executado. |
|||
|
|||
Assim, você pode confirmar se está no ambiente virtual correto. |
|||
|
|||
/// tip | "Dica" |
|||
|
|||
É fácil ativar um ambiente virtual, obter um Python e então **ir para outro projeto**. |
|||
|
|||
E o segundo projeto **não funcionaria** porque você está usando o **Python incorreto**, de um ambiente virtual para outro projeto. |
|||
|
|||
É útil poder verificar qual `python` está sendo usado. 🤓 |
|||
|
|||
/// |
|||
|
|||
## Por que desativar um ambiente virtual |
|||
|
|||
Por exemplo, você pode estar trabalhando em um projeto `philosophers-stone`, **ativar esse ambiente virtual**, instalar pacotes e trabalhar com esse ambiente. |
|||
|
|||
E então você quer trabalhar em **outro projeto** `prisoner-of-azkaban`. |
|||
|
|||
Você vai para aquele projeto: |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ cd ~/code/prisoner-of-azkaban |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
Se você não desativar o ambiente virtual para `philosophers-stone`, quando você executar `python` no terminal, ele tentará usar o Python de `philosophers-stone`. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ cd ~/code/prisoner-of-azkaban |
|||
|
|||
$ python main.py |
|||
|
|||
// Erro ao importar o Sirius, ele não está instalado 😱 |
|||
Traceback (most recent call last): |
|||
File "main.py", line 1, in <module> |
|||
import sirius |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
Mas se você desativar o ambiente virtual e ativar o novo para `prisoner-of-askaban`, quando você executar `python`, ele usará o Python do ambiente virtual em `prisoner-of-azkaban`. |
|||
|
|||
<div class="termy"> |
|||
|
|||
```console |
|||
$ cd ~/code/prisoner-of-azkaban |
|||
|
|||
// Você não precisa estar no diretório antigo para desativar, você pode fazer isso de onde estiver, mesmo depois de ir para o outro projeto 😎 |
|||
$ deactivate |
|||
|
|||
// Ative o ambiente virtual em prisoner-of-azkaban/.venv 🚀 |
|||
$ source .venv/bin/activate |
|||
|
|||
// Agora, quando você executar o python, ele encontrará o pacote sirius instalado neste ambiente virtual ✨ |
|||
$ python main.py |
|||
|
|||
Eu juro solenemente 🐺 |
|||
``` |
|||
|
|||
</div> |
|||
|
|||
## Alternativas |
|||
|
|||
Este é um guia simples para você começar e lhe ensinar como tudo funciona **por baixo**. |
|||
|
|||
Existem muitas **alternativas** para gerenciar ambientes virtuais, dependências de pacotes (requisitos) e projetos. |
|||
|
|||
Quando estiver pronto e quiser usar uma ferramenta para **gerenciar todo o projeto**, dependências de pacotes, ambientes virtuais, etc., sugiro que você experimente o <a href="https://github.com/astral-sh/uv" class="external-link" target="_blank">uv</a>. |
|||
|
|||
`uv` pode fazer muitas coisas, ele pode: |
|||
|
|||
* **Instalar o Python** para você, incluindo versões diferentes |
|||
* Gerenciar o **ambiente virtual** para seus projetos |
|||
* Instalar **pacotes** |
|||
* Gerenciar **dependências e versões** de pacotes para seu projeto |
|||
* Certifique-se de ter um conjunto **exato** de pacotes e versões para instalar, incluindo suas dependências, para que você possa ter certeza de que pode executar seu projeto em produção exatamente da mesma forma que em seu computador durante o desenvolvimento, isso é chamado de **bloqueio** |
|||
* E muitas outras coisas |
|||
|
|||
## Conclusão |
|||
|
|||
Se você leu e entendeu tudo isso, agora **você sabe muito mais** sobre ambientes virtuais do que muitos desenvolvedores por aí. 🤓 |
|||
|
|||
Saber esses detalhes provavelmente será útil no futuro, quando você estiver depurando algo que parece complexo, mas você saberá **como tudo funciona**. 😎 |
@ -1,84 +1,28 @@ |
|||
# 项目生成 - 模板 |
|||
|
|||
项目生成器一般都会提供很多初始设置、安全措施、数据库,甚至还准备好了第一个 API 端点,能帮助您快速上手。 |
|||
|
|||
项目生成器的设置通常都很主观,您可以按需更新或修改,但对于您的项目来说,它是非常好的起点。 |
|||
|
|||
## 全栈 FastAPI + PostgreSQL |
|||
|
|||
GitHub:<a href="https://github.com/tiangolo/full-stack-fastapi-postgresql" class="external-link" target="_blank">https://github.com/tiangolo/full-stack-fastapi-postgresql</a> |
|||
|
|||
### 全栈 FastAPI + PostgreSQL - 功能 |
|||
|
|||
* 完整的 **Docker** 集成(基于 Docker) |
|||
* Docker Swarm 开发模式 |
|||
* **Docker Compose** 本地开发集成与优化 |
|||
* **生产可用**的 Python 网络服务器,使用 Uvicorn 或 Gunicorn |
|||
* Python <a href="https://github.com/fastapi/fastapi" class="external-link" target="_blank">**FastAPI**</a> 后端: |
|||
* * **速度快**:可与 **NodeJS** 和 **Go** 比肩的极高性能(归功于 Starlette 和 Pydantic) |
|||
* **直观**:强大的编辑器支持,处处皆可<abbr title="也叫自动完成、智能感知">自动补全</abbr>,减少调试时间 |
|||
* **简单**:易学、易用,阅读文档所需时间更短 |
|||
* **简短**:代码重复最小化,每次参数声明都可以实现多个功能 |
|||
* **健壮**: 生产级别的代码,还有自动交互文档 |
|||
* **基于标准**:完全兼容并基于 API 开放标准:<a href="https://github.com/OAI/OpenAPI-Specification" class="external-link" target="_blank">OpenAPI</a> 和 <a href="https://json-schema.org/" class="external-link" target="_blank">JSON Schema</a> |
|||
* <a href="https://fastapi.tiangolo.com/features/" class="external-link" target="_blank">**更多功能**</a>包括自动验证、序列化、交互文档、OAuth2 JWT 令牌身份验证等 |
|||
* **安全密码**,默认使用密码哈希 |
|||
* **JWT 令牌**身份验证 |
|||
* **SQLAlchemy** 模型(独立于 Flask 扩展,可直接用于 Celery Worker) |
|||
* 基础的用户模型(可按需修改或删除) |
|||
* **Alembic** 迁移 |
|||
* **CORS**(跨域资源共享) |
|||
* **Celery** Worker 可从后端其它部分有选择地导入并使用模型和代码 |
|||
* REST 后端测试基于 Pytest,并与 Docker 集成,可独立于数据库实现完整的 API 交互测试。因为是在 Docker 中运行,每次都可从头构建新的数据存储(使用 ElasticSearch、MongoDB、CouchDB 等数据库,仅测试 API 运行) |
|||
* Python 与 **Jupyter Kernels** 集成,用于远程或 Docker 容器内部开发,使用 Atom Hydrogen 或 Visual Studio Code 的 Jupyter 插件 |
|||
* **Vue** 前端: |
|||
* 由 Vue CLI 生成 |
|||
* **JWT 身份验证**处理 |
|||
* 登录视图 |
|||
* 登录后显示主仪表盘视图 |
|||
* 主仪表盘支持用户创建与编辑 |
|||
* 用户信息编辑 |
|||
* **Vuex** |
|||
* **Vue-router** |
|||
* **Vuetify** 美化组件 |
|||
* **TypeScript** |
|||
* 基于 **Nginx** 的 Docker 服务器(优化了 Vue-router 配置) |
|||
* Docker 多阶段构建,无需保存或提交编译的代码 |
|||
* 在构建时运行前端测试(可禁用) |
|||
* 尽量模块化,开箱即用,但仍可使用 Vue CLI 重新生成或创建所需项目,或复用所需内容 |
|||
* 使用 **PGAdmin** 管理 PostgreSQL 数据库,可轻松替换为 PHPMyAdmin 或 MySQL |
|||
* 使用 **Flower** 监控 Celery 任务 |
|||
* 使用 **Traefik** 处理前后端负载平衡,可把前后端放在同一个域下,按路径分隔,但在不同容器中提供服务 |
|||
* Traefik 集成,包括自动生成 Let's Encrypt **HTTPS** 凭证 |
|||
* GitLab **CI**(持续集成),包括前后端测试 |
|||
|
|||
## 全栈 FastAPI + Couchbase |
|||
|
|||
GitHub:<a href="https://github.com/tiangolo/full-stack-fastapi-couchbase" class="external-link" target="_blank">https://github.com/tiangolo/full-stack-fastapi-couchbase</a> |
|||
|
|||
⚠️ **警告** ⚠️ |
|||
|
|||
如果您想从头开始创建新项目,建议使用以下备选方案。 |
|||
|
|||
例如,项目生成器<a href="https://github.com/tiangolo/full-stack-fastapi-postgresql" class="external-link" target="_blank">全栈 FastAPI + PostgreSQL </a>会更适用,这个项目的维护积极,用的人也多,还包括了所有新功能和改进内容。 |
|||
|
|||
当然,您也可以放心使用这个基于 Couchbase 的生成器,它也能正常使用。就算用它生成项目也没有任何问题(为了更好地满足需求,您可以自行更新这个项目)。 |
|||
|
|||
详见资源仓库中的文档。 |
|||
|
|||
## 全栈 FastAPI + MongoDB |
|||
|
|||
……敬请期待,得看我有没有时间做这个项目。😅 🎉 |
|||
|
|||
## FastAPI + spaCy 机器学习模型 |
|||
|
|||
GitHub:<a href="https://github.com/microsoft/cookiecutter-spacy-fastapi" class="external-link" target="_blank">https://github.com/microsoft/cookiecutter-spacy-fastapi</a> |
|||
|
|||
### FastAPI + spaCy 机器学习模型 - 功能 |
|||
|
|||
* 集成 **spaCy** NER 模型 |
|||
* 内置 **Azure 认知搜索**请求格式 |
|||
* **生产可用**的 Python 网络服务器,使用 Uvicorn 与 Gunicorn |
|||
* 内置 **Azure DevOps** Kubernetes (AKS) CI/CD 开发 |
|||
* **多语**支持,可在项目设置时选择 spaCy 内置的语言 |
|||
* 不仅局限于 spaCy,可**轻松扩展**至其它模型框架(Pytorch、TensorFlow) |
|||
# FastAPI全栈模板 |
|||
|
|||
模板通常带有特定的设置,而且被设计为灵活和可定制的。这允许您根据项目的需求修改和调整它们,使它们成为一个很好的起点。🏁 |
|||
|
|||
您可以使用此模板开始,因为它包含了许多已经为您完成的初始设置、安全性、数据库和一些API端点。 |
|||
|
|||
代码仓: <a href="https://github.com/fastapi/full-stack-fastapi-template" class="external-link" target="_blank">Full Stack FastAPI Template</a> |
|||
|
|||
## FastAPI全栈模板 - 技术栈和特性 |
|||
|
|||
- ⚡ [**FastAPI**](https://fastapi.tiangolo.com) 用于Python后端API. |
|||
- 🧰 [SQLModel](https://sqlmodel.tiangolo.com) 用于Python和SQL数据库的集成(ORM)。 |
|||
- 🔍 [Pydantic](https://docs.pydantic.dev) FastAPI的依赖项之一,用于数据验证和配置管理。 |
|||
- 💾 [PostgreSQL](https://www.postgresql.org) 作为SQL数据库。 |
|||
- 🚀 [React](https://react.dev) 用于前端。 |
|||
- 💃 使用了TypeScript、hooks、Vite和其他一些现代化的前端技术栈。 |
|||
- 🎨 [Chakra UI](https://chakra-ui.com) 用于前端组件。 |
|||
- 🤖 一个自动化生成的前端客户端。 |
|||
- 🧪 Playwright用于端到端测试。 |
|||
- 🦇 支持暗黑主题(Dark mode)。 |
|||
- 🐋 [Docker Compose](https://www.docker.com) 用于开发环境和生产环境。 |
|||
- 🔒 默认使用密码哈希来保证安全。 |
|||
- 🔑 JWT令牌用于权限验证。 |
|||
- 📫 使用邮箱来进行密码恢复。 |
|||
- ✅ 单元测试用了[Pytest](https://pytest.org). |
|||
- 📞 [Traefik](https://traefik.io) 用于反向代理和负载均衡。 |
|||
- 🚢 部署指南(Docker Compose)包含了如何起一个Traefik前端代理来自动化HTTPS认证。 |
|||
- 🏭 CI(持续集成)和 CD(持续部署)基于GitHub Actions。 |
|||
|
@ -1,12 +1,14 @@ |
|||
import pytest |
|||
from httpx import AsyncClient |
|||
from httpx import ASGITransport, AsyncClient |
|||
|
|||
from .main import app |
|||
|
|||
|
|||
@pytest.mark.anyio |
|||
async def test_root(): |
|||
async with AsyncClient(app=app, base_url="http://test") as ac: |
|||
async with AsyncClient( |
|||
transport=ASGITransport(app=app), base_url="http://test" |
|||
) as ac: |
|||
response = await ac.get("/") |
|||
assert response.status_code == 200 |
|||
assert response.json() == {"message": "Tomato"} |
|||
|
@ -0,0 +1,17 @@ |
|||
from typing import Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Cookies = Cookie()): |
|||
return cookies |
@ -0,0 +1,18 @@ |
|||
from typing import Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
from typing_extensions import Annotated |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Annotated[Cookies, Cookie()]): |
|||
return cookies |
@ -0,0 +1,17 @@ |
|||
from typing import Annotated |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
session_id: str |
|||
fatebook_tracker: str | None = None |
|||
googall_tracker: str | None = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Annotated[Cookies, Cookie()]): |
|||
return cookies |
@ -0,0 +1,17 @@ |
|||
from typing import Annotated, Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Annotated[Cookies, Cookie()]): |
|||
return cookies |
@ -0,0 +1,15 @@ |
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
session_id: str |
|||
fatebook_tracker: str | None = None |
|||
googall_tracker: str | None = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Cookies = Cookie()): |
|||
return cookies |
@ -0,0 +1,19 @@ |
|||
from typing import Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
model_config = {"extra": "forbid"} |
|||
|
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Cookies = Cookie()): |
|||
return cookies |
@ -0,0 +1,20 @@ |
|||
from typing import Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
from typing_extensions import Annotated |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
model_config = {"extra": "forbid"} |
|||
|
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Annotated[Cookies, Cookie()]): |
|||
return cookies |
@ -0,0 +1,19 @@ |
|||
from typing import Annotated |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
model_config = {"extra": "forbid"} |
|||
|
|||
session_id: str |
|||
fatebook_tracker: str | None = None |
|||
googall_tracker: str | None = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Annotated[Cookies, Cookie()]): |
|||
return cookies |
@ -0,0 +1,19 @@ |
|||
from typing import Annotated, Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
model_config = {"extra": "forbid"} |
|||
|
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Annotated[Cookies, Cookie()]): |
|||
return cookies |
@ -0,0 +1,20 @@ |
|||
from typing import Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
class Config: |
|||
extra = "forbid" |
|||
|
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Cookies = Cookie()): |
|||
return cookies |
@ -0,0 +1,21 @@ |
|||
from typing import Union |
|||
|
|||
from fastapi import Cookie, FastAPI |
|||
from pydantic import BaseModel |
|||
from typing_extensions import Annotated |
|||
|
|||
app = FastAPI() |
|||
|
|||
|
|||
class Cookies(BaseModel): |
|||
class Config: |
|||
extra = "forbid" |
|||
|
|||
session_id: str |
|||
fatebook_tracker: Union[str, None] = None |
|||
googall_tracker: Union[str, None] = None |
|||
|
|||
|
|||
@app.get("/items/") |
|||
async def read_items(cookies: Annotated[Cookies, Cookie()]): |
|||
return cookies |
Some files were not shown because too many files changed in this diff
Loading…
Reference in new issue